url
stringlengths 17
87
| content
stringlengths 152
81.8k
|
---|---|
https://react.dev/blog/2022/03/08/react-18-upgrade-guide | [Blog](https://react.dev/blog)
# How to Upgrade to React 18[Link for this heading]()
March 08, 2022 by [Rick Hanlon](https://twitter.com/rickhanlonii)
* * *
As we shared in the [release post](https://react.dev/blog/2022/03/29/react-v18), React 18 introduces features powered by our new concurrent renderer, with a gradual adoption strategy for existing applications. In this post, we will guide you through the steps for upgrading to React 18.
Please [report any issues](https://github.com/facebook/react/issues/new/choose) you encounter while upgrading to React 18.
### Note
For React Native users, React 18 will ship in a future version of React Native. This is because React 18 relies on the New React Native Architecture to benefit from the new capabilities presented in this blogpost. For more information, see the [React Conf keynote here](https://www.youtube.com/watch?v=FZ0cG47msEk&t=1530s).
* * *
## Installing[Link for Installing]()
To install the latest version of React:
```
npm install react react-dom
```
Or if you’re using yarn:
```
yarn add react react-dom
```
## Updates to Client Rendering APIs[Link for Updates to Client Rendering APIs]()
When you first install React 18, you will see a warning in the console:
Console
ReactDOM.render is no longer supported in React 18. Use createRoot instead. Until you switch to the new API, your app will behave as if it’s running React 17. Learn more: [https://reactjs.org/link/switch-to-createroot](https://reactjs.org/link/switch-to-createroot)
React 18 introduces a new root API which provides better ergonomics for managing roots. The new root API also enables the new concurrent renderer, which allows you to opt-into concurrent features.
```
// Before
import { render } from 'react-dom';
const container = document.getElementById('app');
render(<App tab="home" />, container);
// After
import { createRoot } from 'react-dom/client';
const container = document.getElementById('app');
const root = createRoot(container); // createRoot(container!) if you use TypeScript
root.render(<App tab="home" />);
```
We’ve also changed `unmountComponentAtNode` to `root.unmount`:
```
// Before
unmountComponentAtNode(container);
// After
root.unmount();
```
We’ve also removed the callback from render, since it usually does not have the expected result when using Suspense:
```
// Before
const container = document.getElementById('app');
render(<App tab="home" />, container, () => {
console.log('rendered');
});
// After
function AppWithCallbackAfterRender() {
useEffect(() => {
console.log('rendered');
});
return <App tab="home" />
}
const container = document.getElementById('app');
const root = createRoot(container);
root.render(<AppWithCallbackAfterRender />);
```
### Note
There is no one-to-one replacement for the old render callback API — it depends on your use case. See the working group post for [Replacing render with createRoot](https://github.com/reactwg/react-18/discussions/5) for more information.
Finally, if your app uses server-side rendering with hydration, upgrade `hydrate` to `hydrateRoot`:
```
// Before
import { hydrate } from 'react-dom';
const container = document.getElementById('app');
hydrate(<App tab="home" />, container);
// After
import { hydrateRoot } from 'react-dom/client';
const container = document.getElementById('app');
const root = hydrateRoot(container, <App tab="home" />);
// Unlike with createRoot, you don't need a separate root.render() call here.
```
For more information, see the [working group discussion here](https://github.com/reactwg/react-18/discussions/5).
### Note
**If your app doesn’t work after upgrading, check whether it’s wrapped in `<StrictMode>`.** [Strict Mode has gotten stricter in React 18](), and not all your components may be resilient to the new checks it adds in development mode. If removing Strict Mode fixes your app, you can remove it during the upgrade, and then add it back (either at the top or for a part of the tree) after you fix the issues that it’s pointing out.
## Updates to Server Rendering APIs[Link for Updates to Server Rendering APIs]()
In this release, we’re revamping our `react-dom/server` APIs to fully support Suspense on the server and Streaming SSR. As part of these changes, we’re deprecating the old Node streaming API, which does not support incremental Suspense streaming on the server.
Using this API will now warn:
- `renderToNodeStream`: **Deprecated ⛔️️**
Instead, for streaming in Node environments, use:
- `renderToPipeableStream`: **New ✨**
We’re also introducing a new API to support streaming SSR with Suspense for modern edge runtime environments, such as Deno and Cloudflare workers:
- `renderToReadableStream`: **New ✨**
The following APIs will continue working, but with limited support for Suspense:
- `renderToString`: **Limited** ⚠️
- `renderToStaticMarkup`: **Limited** ⚠️
Finally, this API will continue to work for rendering e-mails:
- `renderToStaticNodeStream`
For more information on the changes to server rendering APIs, see the working group post on [Upgrading to React 18 on the server](https://github.com/reactwg/react-18/discussions/22), a [deep dive on the new Suspense SSR Architecture](https://github.com/reactwg/react-18/discussions/37), and [Shaundai Person’s](https://twitter.com/shaundai) talk on [Streaming Server Rendering with Suspense](https://www.youtube.com/watch?v=pj5N-Khihgc) at React Conf 2021.
## Updates to TypeScript definitions[Link for Updates to TypeScript definitions]()
If your project uses TypeScript, you will need to update your `@types/react` and `@types/react-dom` dependencies to the latest versions. The new types are safer and catch issues that used to be ignored by the type checker. The most notable change is that the `children` prop now needs to be listed explicitly when defining props, for example:
```
interface MyButtonProps {
color: string;
children?: React.ReactNode;
}
```
See the [React 18 typings pull request](https://github.com/DefinitelyTyped/DefinitelyTyped/pull/56210) for a full list of type-only changes. It links to example fixes in library types so you can see how to adjust your code. You can use the [automated migration script](https://github.com/eps1lon/types-react-codemod) to help port your application code to the new and safer typings faster.
If you find a bug in the typings, please [file an issue](https://github.com/DefinitelyTyped/DefinitelyTyped/discussions/new?category=issues-with-a-types-package) in the DefinitelyTyped repo.
## Automatic Batching[Link for Automatic Batching]()
React 18 adds out-of-the-box performance improvements by doing more batching by default. Batching is when React groups multiple state updates into a single re-render for better performance. Before React 18, we only batched updates inside React event handlers. Updates inside of promises, setTimeout, native event handlers, or any other event were not batched in React by default:
```
// Before React 18 only React events were batched
function handleClick() {
setCount(c => c + 1);
setFlag(f => !f);
// React will only re-render once at the end (that's batching!)
}
setTimeout(() => {
setCount(c => c + 1);
setFlag(f => !f);
// React will render twice, once for each state update (no batching)
}, 1000);
```
Starting in React 18 with `createRoot`, all updates will be automatically batched, no matter where they originate from. This means that updates inside of timeouts, promises, native event handlers or any other event will batch the same way as updates inside of React events:
```
// After React 18 updates inside of timeouts, promises,
// native event handlers or any other event are batched.
function handleClick() {
setCount(c => c + 1);
setFlag(f => !f);
// React will only re-render once at the end (that's batching!)
}
setTimeout(() => {
setCount(c => c + 1);
setFlag(f => !f);
// React will only re-render once at the end (that's batching!)
}, 1000);
```
This is a breaking change, but we expect this to result in less work rendering, and therefore better performance in your applications. To opt-out of automatic batching, you can use `flushSync`:
```
import { flushSync } from 'react-dom';
function handleClick() {
flushSync(() => {
setCounter(c => c + 1);
});
// React has updated the DOM by now
flushSync(() => {
setFlag(f => !f);
});
// React has updated the DOM by now
}
```
For more information, see the [Automatic batching deep dive](https://github.com/reactwg/react-18/discussions/21).
## New APIs for Libraries[Link for New APIs for Libraries]()
In the React 18 Working Group we worked with library maintainers to create new APIs needed to support concurrent rendering for use cases specific to their use case in areas like styles, and external stores. To support React 18, some libraries may need to switch to one of the following APIs:
- `useSyncExternalStore` is a new Hook that allows external stores to support concurrent reads by forcing updates to the store to be synchronous. This new API is recommended for any library that integrates with state external to React. For more information, see the [useSyncExternalStore overview post](https://github.com/reactwg/react-18/discussions/70) and [useSyncExternalStore API details](https://github.com/reactwg/react-18/discussions/86).
- `useInsertionEffect` is a new Hook that allows CSS-in-JS libraries to address performance issues of injecting styles in render. Unless you’ve already built a CSS-in-JS library we don’t expect you to ever use this. This Hook will run after the DOM is mutated, but before layout effects read the new layout. This solves an issue that already exists in React 17 and below, but is even more important in React 18 because React yields to the browser during concurrent rendering, giving it a chance to recalculate layout. For more information, see the [Library Upgrade Guide for `<style>`](https://github.com/reactwg/react-18/discussions/110).
React 18 also introduces new APIs for concurrent rendering such as `startTransition`, `useDeferredValue` and `useId`, which we share more about in the [release post](https://react.dev/blog/2022/03/29/react-v18).
## Updates to Strict Mode[Link for Updates to Strict Mode]()
In the future, we’d like to add a feature that allows React to add and remove sections of the UI while preserving state. For example, when a user tabs away from a screen and back, React should be able to immediately show the previous screen. To do this, React would unmount and remount trees using the same component state as before.
This feature will give React better performance out-of-the-box, but requires components to be resilient to effects being mounted and destroyed multiple times. Most effects will work without any changes, but some effects assume they are only mounted or destroyed once.
To help surface these issues, React 18 introduces a new development-only check to Strict Mode. This new check will automatically unmount and remount every component, whenever a component mounts for the first time, restoring the previous state on the second mount.
Before this change, React would mount the component and create the effects:
```
* React mounts the component.
* Layout effects are created.
* Effect effects are created.
```
With Strict Mode in React 18, React will simulate unmounting and remounting the component in development mode:
```
* React mounts the component.
* Layout effects are created.
* Effect effects are created.
* React simulates unmounting the component.
* Layout effects are destroyed.
* Effects are destroyed.
* React simulates mounting the component with the previous state.
* Layout effect setup code runs
* Effect setup code runs
```
For more information, see the Working Group posts for [Adding Reusable State to StrictMode](https://github.com/reactwg/react-18/discussions/19) and [How to support Reusable State in Effects](https://github.com/reactwg/react-18/discussions/18).
## Configuring Your Testing Environment[Link for Configuring Your Testing Environment]()
When you first update your tests to use `createRoot`, you may see this warning in your test console:
Console
The current testing environment is not configured to support act(…)
To fix this, set `globalThis.IS_REACT_ACT_ENVIRONMENT` to `true` before running your test:
```
// In your test setup file
globalThis.IS_REACT_ACT_ENVIRONMENT = true;
```
The purpose of the flag is to tell React that it’s running in a unit test-like environment. React will log helpful warnings if you forget to wrap an update with `act`.
You can also set the flag to `false` to tell React that `act` isn’t needed. This can be useful for end-to-end tests that simulate a full browser environment.
Eventually, we expect testing libraries will configure this for you automatically. For example, the [next version of React Testing Library has built-in support for React 18](https://github.com/testing-library/react-testing-library/issues/509) without any additional configuration.
[More background on the `act` testing API and related changes](https://github.com/reactwg/react-18/discussions/102) is available in the working group.
## Dropping Support for Internet Explorer[Link for Dropping Support for Internet Explorer]()
In this release, React is dropping support for Internet Explorer, which is [going out of support on June 15, 2022](https://blogs.windows.com/windowsexperience/2021/05/19/the-future-of-internet-explorer-on-windows-10-is-in-microsoft-edge). We’re making this change now because new features introduced in React 18 are built using modern browser features such as microtasks which cannot be adequately polyfilled in IE.
If you need to support Internet Explorer we recommend you stay with React 17.
## Deprecations[Link for Deprecations]()
- `react-dom`: `ReactDOM.render` has been deprecated. Using it will warn and run your app in React 17 mode.
- `react-dom`: `ReactDOM.hydrate` has been deprecated. Using it will warn and run your app in React 17 mode.
- `react-dom`: `ReactDOM.unmountComponentAtNode` has been deprecated.
- `react-dom`: `ReactDOM.renderSubtreeIntoContainer` has been deprecated.
- `react-dom/server`: `ReactDOMServer.renderToNodeStream` has been deprecated.
## Other Breaking Changes[Link for Other Breaking Changes]()
- **Consistent useEffect timing**: React now always synchronously flushes effect functions if the update was triggered during a discrete user input event such as a click or a keydown event. Previously, the behavior wasn’t always predictable or consistent.
- **Stricter hydration errors**: Hydration mismatches due to missing or extra text content are now treated like errors instead of warnings. React will no longer attempt to “patch up” individual nodes by inserting or deleting a node on the client in an attempt to match the server markup, and will revert to client rendering up to the closest `<Suspense>` boundary in the tree. This ensures the hydrated tree is consistent and avoids potential privacy and security holes that can be caused by hydration mismatches.
- **Suspense trees are always consistent:** If a component suspends before it’s fully added to the tree, React will not add it to the tree in an incomplete state or fire its effects. Instead, React will throw away the new tree completely, wait for the asynchronous operation to finish, and then retry rendering again from scratch. React will render the retry attempt concurrently, and without blocking the browser.
- **Layout Effects with Suspense**: When a tree re-suspends and reverts to a fallback, React will now clean up layout effects, and then re-create them when the content inside the boundary is shown again. This fixes an issue which prevented component libraries from correctly measuring layout when used with Suspense.
- **New JS Environment Requirements**: React now depends on modern browsers features including `Promise`, `Symbol`, and `Object.assign`. If you support older browsers and devices such as Internet Explorer which do not provide modern browser features natively or have non-compliant implementations, consider including a global polyfill in your bundled application.
## Other Notable Changes[Link for Other Notable Changes]()
### React[Link for React]()
- **Components can now render `undefined`:** React no longer warns if you return `undefined` from a component. This makes the allowed component return values consistent with values that are allowed in the middle of a component tree. We suggest to use a linter to prevent mistakes like forgetting a `return` statement before JSX.
- **In tests, `act` warnings are now opt-in:** If you’re running end-to-end tests, the `act` warnings are unnecessary. We’ve introduced an [opt-in](https://github.com/reactwg/react-18/discussions/102) mechanism so you can enable them only for unit tests where they are useful and beneficial.
- **No warning about `setState` on unmounted components:** Previously, React warned about memory leaks when you call `setState` on an unmounted component. This warning was added for subscriptions, but people primarily run into it in scenarios where setting state is fine, and workarounds make the code worse. We’ve [removed](https://github.com/facebook/react/pull/22114) this warning.
- **No suppression of console logs:** When you use Strict Mode, React renders each component twice to help you find unexpected side effects. In React 17, we’ve suppressed console logs for one of the two renders to make the logs easier to read. In response to [community feedback](https://github.com/facebook/react/issues/21783) about this being confusing, we’ve removed the suppression. Instead, if you have React DevTools installed, the second log’s renders will be displayed in grey, and there will be an option (off by default) to suppress them completely.
- **Improved memory usage:** React now cleans up more internal fields on unmount, making the impact from unfixed memory leaks that may exist in your application code less severe.
### React DOM Server[Link for React DOM Server]()
- **`renderToString`:** Will no longer error when suspending on the server. Instead, it will emit the fallback HTML for the closest `<Suspense>` boundary and then retry rendering the same content on the client. It is still recommended that you switch to a streaming API like `renderToPipeableStream` or `renderToReadableStream` instead.
- **`renderToStaticMarkup`:** Will no longer error when suspending on the server. Instead, it will emit the fallback HTML for the closest `<Suspense>` boundary.
## Changelog[Link for Changelog]()
You can view the [full changelog here](https://github.com/facebook/react/blob/main/CHANGELOG.md).
[PreviousReact v18.0](https://react.dev/blog/2022/03/29/react-v18)
[NextReact Conf 2021 Recap](https://react.dev/blog/2021/12/17/react-conf-2021-recap) |
https://react.dev/blog/2024/02/15/react-labs-what-we-have-been-working-on-february-2024 | [Blog](https://react.dev/blog)
# React Labs: What We've Been Working On – February 2024[Link for this heading]()
February 15, 2024 by [Joseph Savona](https://twitter.com/en_JS), [Ricky Hanlon](https://twitter.com/rickhanlonii), [Andrew Clark](https://twitter.com/acdlite), [Matt Carroll](https://twitter.com/mattcarrollcode), and [Dan Abramov](https://twitter.com/dan_abramov).
* * *
In React Labs posts, we write about projects in active research and development. We’ve made significant progress since our [last update](https://react.dev/blog/2023/03/22/react-labs-what-we-have-been-working-on-march-2023), and we’d like to share our progress.
### Note
React Conf 2024 is scheduled for May 15–16 in Henderson, Nevada! If you’re interested in attending React Conf in person, you can [sign up for the ticket lottery](https://forms.reform.app/bLaLeE/react-conf-2024-ticket-lottery/1aRQLK) until February 28th.
For more info on tickets, free streaming, sponsoring, and more, see [the React Conf website](https://conf.react.dev).
* * *
## React Compiler[Link for React Compiler]()
React Compiler is no longer a research project: the compiler now powers instagram.com in production, and we are working to ship the compiler across additional surfaces at Meta and to prepare the first open source release.
As discussed in our [previous post](https://react.dev/blog/2023/03/22/react-labs-what-we-have-been-working-on-march-2023), React can *sometimes* re-render too much when state changes. Since the early days of React our solution for such cases has been manual memoization. In our current APIs, this means applying the [`useMemo`](https://react.dev/reference/react/useMemo), [`useCallback`](https://react.dev/reference/react/useCallback), and [`memo`](https://react.dev/reference/react/memo) APIs to manually tune how much React re-renders on state changes. But manual memoization is a compromise. It clutters up our code, is easy to get wrong, and requires extra work to keep up to date.
Manual memoization is a reasonable compromise, but we weren’t satisfied. Our vision is for React to *automatically* re-render just the right parts of the UI when state changes, *without compromising on React’s core mental model*. We believe that React’s approach — UI as a simple function of state, with standard JavaScript values and idioms — is a key part of why React has been approachable for so many developers. That’s why we’ve invested in building an optimizing compiler for React.
JavaScript is a notoriously challenging language to optimize, thanks to its loose rules and dynamic nature. React Compiler is able to compile code safely by modeling both the rules of JavaScript *and* the “rules of React”. For example, React components must be idempotent — returning the same value given the same inputs — and can’t mutate props or state values. These rules limit what developers can do and help to carve out a safe space for the compiler to optimize.
Of course, we understand that developers sometimes bend the rules a bit, and our goal is to make React Compiler work out of the box on as much code as possible. The compiler attempts to detect when code doesn’t strictly follow React’s rules and will either compile the code where safe or skip compilation if it isn’t safe. We’re testing against Meta’s large and varied codebase in order to help validate this approach.
For developers who are curious about making sure their code follows React’s rules, we recommend [enabling Strict Mode](https://react.dev/reference/react/StrictMode) and [configuring React’s ESLint plugin](https://react.dev/learn/editor-setup). These tools can help to catch subtle bugs in your React code, improving the quality of your applications today, and future-proofs your applications for upcoming features such as React Compiler. We are also working on consolidated documentation of the rules of React and updates to our ESLint plugin to help teams understand and apply these rules to create more robust apps.
To see the compiler in action, you can check out our [talk from last fall](https://www.youtube.com/watch?v=qOQClO3g8-Y). At the time of the talk, we had early experimental data from trying React Compiler on one page of instagram.com. Since then, we shipped the compiler to production across instagram.com. We’ve also expanded our team to accelerate the rollout to additional surfaces at Meta and to open source. We’re excited about the path ahead and will have more to share in the coming months.
## Actions[Link for Actions]()
We [previously shared](https://react.dev/blog/2023/03/22/react-labs-what-we-have-been-working-on-march-2023) that we were exploring solutions for sending data from the client to the server with Server Actions, so that you can execute database mutations and implement forms. During development of Server Actions, we extended these APIs to support data handling in client-only applications as well.
We refer to this broader collection of features as simply “Actions”. Actions allow you to pass a function to DOM elements such as [`<form/>`](https://react.dev/reference/react-dom/components/form):
```
<form action={search}>
<input name="query" />
<button type="submit">Search</button>
</form>
```
The `action` function can operate synchronously or asynchronously. You can define them on the client side using standard JavaScript or on the server with the [`'use server'`](https://react.dev/reference/rsc/use-server) directive. When using an action, React will manage the life cycle of the data submission for you, providing hooks like [`useFormStatus`](https://react.dev/reference/react-dom/hooks/useFormStatus), and [`useActionState`](https://react.dev/reference/react/useActionState) to access the current state and response of the form action.
By default, Actions are submitted within a [transition](https://react.dev/reference/react/useTransition), keeping the current page interactive while the action is processing. Since Actions support async functions, we’ve also added the ability to use `async/await` in transitions. This allows you to show pending UI with the `isPending` state of a transition when an async request like `fetch` starts, and show the pending UI all the way through the update being applied.
Alongside Actions, we’re introducing a feature named [`useOptimistic`](https://react.dev/reference/react/useOptimistic) for managing optimistic state updates. With this hook, you can apply temporary updates that are automatically reverted once the final state commits. For Actions, this allows you to optimistically set the final state of the data on the client, assuming the submission is successful, and revert to the value for data received from the server. It works using regular `async`/`await`, so it works the same whether you’re using `fetch` on the client, or a Server Action from the server.
Library authors can implement custom `action={fn}` props in their own components with `useTransition`. Our intent is for libraries to adopt the Actions pattern when designing their component APIs, to provide a consistent experience for React developers. For example, if your library provides a `<Calendar onSelect={eventHandler}>` component, consider also exposing a `<Calendar selectAction={action}>` API, too.
While we initially focused on Server Actions for client-server data transfer, our philosophy for React is to provide the same programming model across all platforms and environments. When possible, if we introduce a feature on the client, we aim to make it also work on the server, and vice versa. This philosophy allows us to create a single set of APIs that work no matter where your app runs, making it easier to upgrade to different environments later.
Actions are now available in the Canary channel and will ship in the next release of React.
## New Features in React Canary[Link for New Features in React Canary]()
We introduced [React Canaries](https://react.dev/blog/2023/05/03/react-canaries) as an option to adopt individual new stable features as soon as their design is close to final, before they’re released in a stable semver version.
Canaries are a change to the way we develop React. Previously, features would be researched and built privately inside of Meta, so users would only see the final polished product when released to Stable. With Canaries, we’re building in public with the help of the community to finalize features we share in the React Labs blog series. This means you hear about new features sooner, as they’re being finalized instead of after they’re complete.
React Server Components, Asset Loading, Document Metadata, and Actions have all landed in the React Canary, and we’ve added docs for these features on react.dev:
- **Directives**: [`"use client"`](https://react.dev/reference/rsc/use-client) and [`"use server"`](https://react.dev/reference/rsc/use-server) are bundler features designed for full-stack React frameworks. They mark the “split points” between the two environments: `"use client"` instructs the bundler to generate a `<script>` tag (like [Astro Islands](https://docs.astro.build/en/concepts/islands/)), while `"use server"` tells the bundler to generate a POST endpoint (like [tRPC Mutations](https://trpc.io/docs/concepts)). Together, they let you write reusable components that compose client-side interactivity with the related server-side logic.
- **Document Metadata**: we added built-in support for rendering [`<title>`](https://react.dev/reference/react-dom/components/title), [`<meta>`](https://react.dev/reference/react-dom/components/meta), and metadata [`<link>`](https://react.dev/reference/react-dom/components/link) tags anywhere in your component tree. These work the same way in all environments, including fully client-side code, SSR, and RSC. This provides built-in support for features pioneered by libraries like [React Helmet](https://github.com/nfl/react-helmet).
- **Asset Loading**: we integrated Suspense with the loading lifecycle of resources such as stylesheets, fonts, and scripts so that React takes them into account to determine whether the content in elements like [`<style>`](https://react.dev/reference/react-dom/components/style), [`<link>`](https://react.dev/reference/react-dom/components/link), and [`<script>`](https://react.dev/reference/react-dom/components/script) are ready to be displayed. We’ve also added new [Resource Loading APIs](https://react.dev/reference/react-dom) like `preload` and `preinit` to provide greater control for when a resource should load and initialize.
- **Actions**: As shared above, we’ve added Actions to manage sending data from the client to the server. You can add `action` to elements like [`<form/>`](https://react.dev/reference/react-dom/components/form), access the status with [`useFormStatus`](https://react.dev/reference/react-dom/hooks/useFormStatus), handle the result with [`useActionState`](https://react.dev/reference/react/useActionState), and optimistically update the UI with [`useOptimistic`](https://react.dev/reference/react/useOptimistic).
Since all of these features work together, it’s difficult to release them in the Stable channel individually. Releasing Actions without the complementary hooks for accessing form states would limit the practical usability of Actions. Introducing React Server Components without integrating Server Actions would complicate modifying data on the server.
Before we can release a set of features to the Stable channel, we need to ensure they work cohesively and developers have everything they need to use them in production. React Canaries allow us to develop these features individually, and release the stable APIs incrementally until the entire feature set is complete.
The current set of features in React Canary are complete and ready to release.
## The Next Major Version of React[Link for The Next Major Version of React]()
After a couple of years of iteration, `react@canary` is now ready to ship to `react@latest`. The new features mentioned above are compatible with any environment your app runs in, providing everything needed for production use. Since Asset Loading and Document Metadata may be a breaking change for some apps, the next version of React will be a major version: **React 19**.
There’s still more to be done to prepare for release. In React 19, we’re also adding long-requested improvements which require breaking changes like support for Web Components. Our focus now is to land these changes, prepare for release, finalize docs for new features, and publish announcements for what’s included.
We’ll share more information about everything React 19 includes, how to adopt the new client features, and how to build support for React Server Components in the coming months.
## Offscreen (renamed to Activity).[Link for Offscreen (renamed to Activity).]()
Since our last update, we’ve renamed a capability we’re researching from “Offscreen” to “Activity”. The name “Offscreen” implied that it only applied to parts of the app that were not visible, but while researching the feature we realized that it’s possible for parts of the app to be visible and inactive, such as content behind a modal. The new name more closely reflects the behavior of marking certain parts of the app “active” or “inactive”.
Activity is still under research and our remaining work is to finalize the primitives that are exposed to library developers. We’ve deprioritized this area while we focus on shipping features that are more complete.
* * *
In addition to this update, our team has presented at conferences and made appearances on podcasts to speak more on our work and answer questions.
- [Sathya Gunasekaran](https://react.dev/community/team) spoke about the React Compiler at the [React India](https://www.youtube.com/watch?v=kjOacmVsLSE) conference
- [Dan Abramov](https://react.dev/community/team) gave a talk at [RemixConf](https://www.youtube.com/watch?v=zMf_xeGPn6s) titled “React from Another Dimension” which explores an alternative history of how React Server Components and Actions could have been created
- [Dan Abramov](https://react.dev/community/team) was interviewed on [the Changelog’s JS Party podcast](https://changelog.com/jsparty/311) about React Server Components
- [Matt Carroll](https://react.dev/community/team) was interviewed on the [Front-End Fire podcast](https://www.buzzsprout.com/2226499/14462424-interview-the-two-reacts-with-rachel-nabors-evan-bacon-and-matt-carroll) where he discussed [The Two Reacts](https://overreacted.io/the-two-reacts/)
Thanks [Lauren Tan](https://twitter.com/potetotes), [Sophie Alpert](https://twitter.com/sophiebits), [Jason Bonta](https://threads.net/someextent), [Eli White](https://twitter.com/Eli_White), and [Sathya Gunasekaran](https://twitter.com/_gsathya) for reviewing this post.
Thanks for reading, and [see you at React Conf](https://conf.react.dev/)!
[PreviousReact 19 RC Upgrade Guide](https://react.dev/blog/2024/04/25/react-19-upgrade-guide)
[NextReact Canaries: Enabling Incremental Feature Rollout Outside Meta](https://react.dev/blog/2023/05/03/react-canaries) |
https://react.dev/reference/react-dom/static/prerender | [API Reference](https://react.dev/reference/react)
[Static APIs](https://react.dev/reference/react-dom/static)
# prerender[Link for this heading]()
`prerender` renders a React tree to a static HTML string using a [Web Stream](https://developer.mozilla.org/en-US/docs/Web/API/Streams_API).
```
const {prelude} = await prerender(reactNode, options?)
```
- [Reference]()
- [`prerender(reactNode, options?)`]()
- [Usage]()
- [Rendering a React tree to a stream of static HTML]()
- [Rendering a React tree to a string of static HTML]()
- [Waiting for all data to load]()
- [Troubleshooting]()
- [My stream doesn’t start until the entire app is rendered]()
### Note
This API depends on [Web Streams.](https://developer.mozilla.org/en-US/docs/Web/API/Streams_API) For Node.js, use [`prerenderToNodeStream`](https://react.dev/reference/react-dom/static/prerenderToNodeStream) instead.
* * *
## Reference[Link for Reference]()
### `prerender(reactNode, options?)`[Link for this heading]()
Call `prerender` to render your app to static HTML.
```
import { prerender } from 'react-dom/static';
async function handler(request) {
const {prelude} = await prerender(<App />, {
bootstrapScripts: ['/main.js']
});
return new Response(prelude, {
headers: { 'content-type': 'text/html' },
});
}
```
On the client, call [`hydrateRoot`](https://react.dev/reference/react-dom/client/hydrateRoot) to make the server-generated HTML interactive.
[See more examples below.]()
#### Parameters[Link for Parameters]()
- `reactNode`: A React node you want to render to HTML. For example, a JSX node like `<App />`. It is expected to represent the entire document, so the App component should render the `<html>` tag.
- **optional** `options`: An object with static generation options.
- **optional** `bootstrapScriptContent`: If specified, this string will be placed in an inline `<script>` tag.
- **optional** `bootstrapScripts`: An array of string URLs for the `<script>` tags to emit on the page. Use this to include the `<script>` that calls [`hydrateRoot`.](https://react.dev/reference/react-dom/client/hydrateRoot) Omit it if you don’t want to run React on the client at all.
- **optional** `bootstrapModules`: Like `bootstrapScripts`, but emits [`<script type="module">`](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Guide/Modules) instead.
- **optional** `identifierPrefix`: A string prefix React uses for IDs generated by [`useId`.](https://react.dev/reference/react/useId) Useful to avoid conflicts when using multiple roots on the same page. Must be the same prefix as passed to [`hydrateRoot`.](https://react.dev/reference/react-dom/client/hydrateRoot)
- **optional** `namespaceURI`: A string with the root [namespace URI](https://developer.mozilla.org/en-US/docs/Web/API/Document/createElementNS) for the stream. Defaults to regular HTML. Pass `'http://www.w3.org/2000/svg'` for SVG or `'http://www.w3.org/1998/Math/MathML'` for MathML.
- **optional** `onError`: A callback that fires whenever there is a server error, whether [recoverable]() or [not.]() By default, this only calls `console.error`. If you override it to [log crash reports,]() make sure that you still call `console.error`. You can also use it to [adjust the status code]() before the shell is emitted.
- **optional** `progressiveChunkSize`: The number of bytes in a chunk. [Read more about the default heuristic.](https://github.com/facebook/react/blob/14c2be8dac2d5482fda8a0906a31d239df8551fc/packages/react-server/src/ReactFizzServer.js)
- **optional** `signal`: An [abort signal](https://developer.mozilla.org/en-US/docs/Web/API/AbortSignal) that lets you [abort server rendering]() and render the rest on the client.
#### Returns[Link for Returns]()
`prerender` returns a Promise:
- If rendering the is successful, the Promise will resolve to an object containing:
- `prelude`: a [Web Stream](https://developer.mozilla.org/en-US/docs/Web/API/Streams_API) of HTML. You can use this stream to send a response in chunks, or you can read the entire stream into a string.
- If rendering fails, the Promise will be rejected. [Use this to output a fallback shell.]()
### Note
### When should I use `prerender`?[Link for this heading]()
The static `prerender` API is used for static server-side generation (SSG). Unlike `renderToString`, `prerender` waits for all data to load before resolving. This makes it suitable for generating static HTML for a full page, including data that needs to be fetched using Suspense. To stream content as it loads, use a streaming server-side render (SSR) API like [renderToReadableStream](https://react.dev/reference/react-dom/server/renderToReadableStream).
* * *
## Usage[Link for Usage]()
### Rendering a React tree to a stream of static HTML[Link for Rendering a React tree to a stream of static HTML]()
Call `prerender` to render your React tree to static HTML into a [Readable Web Stream:](https://developer.mozilla.org/en-US/docs/Web/API/ReadableStream):
```
import { prerender } from 'react-dom/static';
async function handler(request) {
const {prelude} = await prerender(<App />, {
bootstrapScripts: ['/main.js']
});
return new Response(prelude, {
headers: { 'content-type': 'text/html' },
});
}
```
Along with the root component, you need to provide a list of bootstrap `<script>` paths. Your root component should return **the entire document including the root `<html>` tag.**
For example, it might look like this:
```
export default function App() {
return (
<html>
<head>
<meta charSet="utf-8" />
<meta name="viewport" content="width=device-width, initial-scale=1" />
<link rel="stylesheet" href="/styles.css"></link>
<title>My app</title>
</head>
<body>
<Router />
</body>
</html>
);
}
```
React will inject the [doctype](https://developer.mozilla.org/en-US/docs/Glossary/Doctype) and your bootstrap `<script>` tags into the resulting HTML stream:
```
<!DOCTYPE html>
<html>
<!-- ... HTML from your components ... -->
</html>
<script src="/main.js" async=""></script>
```
On the client, your bootstrap script should [hydrate the entire `document` with a call to `hydrateRoot`:](https://react.dev/reference/react-dom/client/hydrateRoot)
```
import { hydrateRoot } from 'react-dom/client';
import App from './App.js';
hydrateRoot(document, <App />);
```
This will attach event listeners to the static server-generated HTML and make it interactive.
##### Deep Dive
#### Reading CSS and JS asset paths from the build output[Link for Reading CSS and JS asset paths from the build output]()
Show Details
The final asset URLs (like JavaScript and CSS files) are often hashed after the build. For example, instead of `styles.css` you might end up with `styles.123456.css`. Hashing static asset filenames guarantees that every distinct build of the same asset will have a different filename. This is useful because it lets you safely enable long-term caching for static assets: a file with a certain name would never change content.
However, if you don’t know the asset URLs until after the build, there’s no way for you to put them in the source code. For example, hardcoding `"/styles.css"` into JSX like earlier wouldn’t work. To keep them out of your source code, your root component can read the real filenames from a map passed as a prop:
```
export default function App({ assetMap }) {
return (
<html>
<head>
<title>My app</title>
<link rel="stylesheet" href={assetMap['styles.css']}></link>
</head>
...
</html>
);
}
```
On the server, render `<App assetMap={assetMap} />` and pass your `assetMap` with the asset URLs:
```
// You'd need to get this JSON from your build tooling, e.g. read it from the build output.
const assetMap = {
'styles.css': '/styles.123456.css',
'main.js': '/main.123456.js'
};
async function handler(request) {
const {prelude} = await prerender(<App assetMap={assetMap} />, {
bootstrapScripts: [assetMap['/main.js']]
});
return new Response(prelude, {
headers: { 'content-type': 'text/html' },
});
}
```
Since your server is now rendering `<App assetMap={assetMap} />`, you need to render it with `assetMap` on the client too to avoid hydration errors. You can serialize and pass `assetMap` to the client like this:
```
// You'd need to get this JSON from your build tooling.
const assetMap = {
'styles.css': '/styles.123456.css',
'main.js': '/main.123456.js'
};
async function handler(request) {
const {prelude} = await prerender(<App assetMap={assetMap} />, {
// Careful: It's safe to stringify() this because this data isn't user-generated.
bootstrapScriptContent: `window.assetMap = ${JSON.stringify(assetMap)};`,
bootstrapScripts: [assetMap['/main.js']],
});
return new Response(prelude, {
headers: { 'content-type': 'text/html' },
});
}
```
In the example above, the `bootstrapScriptContent` option adds an extra inline `<script>` tag that sets the global `window.assetMap` variable on the client. This lets the client code read the same `assetMap`:
```
import { hydrateRoot } from 'react-dom/client';
import App from './App.js';
hydrateRoot(document, <App assetMap={window.assetMap} />);
```
Both client and server render `App` with the same `assetMap` prop, so there are no hydration errors.
* * *
### Rendering a React tree to a string of static HTML[Link for Rendering a React tree to a string of static HTML]()
Call `prerender` to render your app to a static HTML string:
```
import { prerender } from 'react-dom/static';
async function renderToString() {
const {prelude} = await prerender(<App />, {
bootstrapScripts: ['/main.js']
});
const reader = stream.getReader();
let content = '';
while (true) {
const {done, value} = await reader.read();
if (done) {
return content;
}
content += Buffer.from(value).toString('utf8');
}
}
```
This will produce the initial non-interactive HTML output of your React components. On the client, you will need to call [`hydrateRoot`](https://react.dev/reference/react-dom/client/hydrateRoot) to *hydrate* that server-generated HTML and make it interactive.
* * *
### Waiting for all data to load[Link for Waiting for all data to load]()
`prerender` waits for all data to load before finishing the static HTML generation and resolving. For example, consider a profile page that shows a cover, a sidebar with friends and photos, and a list of posts:
```
function ProfilePage() {
return (
<ProfileLayout>
<ProfileCover />
<Sidebar>
<Friends />
<Photos />
</Sidebar>
<Suspense fallback={<PostsGlimmer />}>
<Posts />
</Suspense>
</ProfileLayout>
);
}
```
Imagine that `<Posts />` needs to load some data, which takes some time. Ideally, you’d want wait for the posts to finish so it’s included in the HTML. To do this, you can use Suspense to suspend on the data, and `prerender` will wait for the suspended content to finish before resolving to the static HTML.
### Note
**Only Suspense-enabled data sources will activate the Suspense component.** They include:
- Data fetching with Suspense-enabled frameworks like [Relay](https://relay.dev/docs/guided-tour/rendering/loading-states/) and [Next.js](https://nextjs.org/docs/getting-started/react-essentials)
- Lazy-loading component code with [`lazy`](https://react.dev/reference/react/lazy)
- Reading the value of a Promise with [`use`](https://react.dev/reference/react/use)
Suspense **does not** detect when data is fetched inside an Effect or event handler.
The exact way you would load data in the `Posts` component above depends on your framework. If you use a Suspense-enabled framework, you’ll find the details in its data fetching documentation.
Suspense-enabled data fetching without the use of an opinionated framework is not yet supported. The requirements for implementing a Suspense-enabled data source are unstable and undocumented. An official API for integrating data sources with Suspense will be released in a future version of React.
* * *
## Troubleshooting[Link for Troubleshooting]()
### My stream doesn’t start until the entire app is rendered[Link for My stream doesn’t start until the entire app is rendered]()
The `prerender` response waits for the entire app to finish rendering, including waiting for all suspense boundaries to resolve, before resolving. It is designed for static site generation (SSG) ahead of time and does not support streaming more content as it loads.
To stream content as it loads, use a streaming server render API like [renderToReadableStream](https://react.dev/reference/react-dom/server/renderToReadableStream).
[PreviousStatic APIs](https://react.dev/reference/react-dom/static)
[NextprerenderToNodeStream](https://react.dev/reference/react-dom/static/prerenderToNodeStream) |
https://react.dev/reference/rsc/server-components | [API Reference](https://react.dev/reference/react)
# Server Components[Link for this heading]()
### React Server Components
Server Components are for use in [React Server Components](https://react.dev/learn/start-a-new-react-project).
Server Components are a new type of Component that renders ahead of time, before bundling, in an environment separate from your client app or SSR server.
This separate environment is the “server” in React Server Components. Server Components can run once at build time on your CI server, or they can be run for each request using a web server.
- [Server Components without a Server]()
- [Server Components with a Server]()
- [Adding interactivity to Server Components]()
- [Async components with Server Components]()
### Note
#### How do I build support for Server Components?[Link for How do I build support for Server Components?]()
While React Server Components in React 19 are stable and will not break between minor versions, the underlying APIs used to implement a React Server Components bundler or framework do not follow semver and may break between minors in React 19.x.
To support React Server Components as a bundler or framework, we recommend pinning to a specific React version, or using the Canary release. We will continue working with bundlers and frameworks to stabilize the APIs used to implement React Server Components in the future.
### Server Components without a Server[Link for Server Components without a Server]()
Server components can run at build time to read from the filesystem or fetch static content, so a web server is not required. For example, you may want to read static data from a content management system.
Without Server Components, it’s common to fetch static data on the client with an Effect:
```
// bundle.js
import marked from 'marked'; // 35.9K (11.2K gzipped)
import sanitizeHtml from 'sanitize-html'; // 206K (63.3K gzipped)
function Page({page}) {
const [content, setContent] = useState('');
// NOTE: loads *after* first page render.
useEffect(() => {
fetch(`/api/content/${page}`).then((data) => {
setContent(data.content);
});
}, [page]);
return <div>{sanitizeHtml(marked(content))}</div>;
}
```
```
// api.js
app.get(`/api/content/:page`, async (req, res) => {
const page = req.params.page;
const content = await file.readFile(`${page}.md`);
res.send({content});
});
```
This pattern means users need to download and parse an additional 75K (gzipped) of libraries, and wait for a second request to fetch the data after the page loads, just to render static content that will not change for the lifetime of the page.
With Server Components, you can render these components once at build time:
```
import marked from 'marked'; // Not included in bundle
import sanitizeHtml from 'sanitize-html'; // Not included in bundle
async function Page({page}) {
// NOTE: loads *during* render, when the app is built.
const content = await file.readFile(`${page}.md`);
return <div>{sanitizeHtml(marked(content))}</div>;
}
```
The rendered output can then be server-side rendered (SSR) to HTML and uploaded to a CDN. When the app loads, the client will not see the original `Page` component, or the expensive libraries for rendering the markdown. The client will only see the rendered output:
```
<div><!-- html for markdown --></div>
```
This means the content is visible during first page load, and the bundle does not include the expensive libraries needed to render the static content.
### Note
You may notice that the Server Component above is an async function:
```
async function Page({page}) {
//...
}
```
Async Components are a new feature of Server Components that allow you to `await` in render.
See [Async components with Server Components]() below.
### Server Components with a Server[Link for Server Components with a Server]()
Server Components can also run on a web server during a request for a page, letting you access your data layer without having to build an API. They are rendered before your application is bundled, and can pass data and JSX as props to Client Components.
Without Server Components, it’s common to fetch dynamic data on the client in an Effect:
```
// bundle.js
function Note({id}) {
const [note, setNote] = useState('');
// NOTE: loads *after* first render.
useEffect(() => {
fetch(`/api/notes/${id}`).then(data => {
setNote(data.note);
});
}, [id]);
return (
<div>
<Author id={note.authorId} />
<p>{note}</p>
</div>
);
}
function Author({id}) {
const [author, setAuthor] = useState('');
// NOTE: loads *after* Note renders.
// Causing an expensive client-server waterfall.
useEffect(() => {
fetch(`/api/authors/${id}`).then(data => {
setAuthor(data.author);
});
}, [id]);
return <span>By: {author.name}</span>;
}
```
```
// api
import db from './database';
app.get(`/api/notes/:id`, async (req, res) => {
const note = await db.notes.get(id);
res.send({note});
});
app.get(`/api/authors/:id`, async (req, res) => {
const author = await db.authors.get(id);
res.send({author});
});
```
With Server Components, you can read the data and render it in the component:
```
import db from './database';
async function Note({id}) {
// NOTE: loads *during* render.
const note = await db.notes.get(id);
return (
<div>
<Author id={note.authorId} />
<p>{note}</p>
</div>
);
}
async function Author({id}) {
// NOTE: loads *after* Note,
// but is fast if data is co-located.
const author = await db.authors.get(id);
return <span>By: {author.name}</span>;
}
```
The bundler then combines the data, rendered Server Components and dynamic Client Components into a bundle. Optionally, that bundle can then be server-side rendered (SSR) to create the initial HTML for the page. When the page loads, the browser does not see the original `Note` and `Author` components; only the rendered output is sent to the client:
```
<div>
<span>By: The React Team</span>
<p>React 19 is...</p>
</div>
```
Server Components can be made dynamic by re-fetching them from a server, where they can access the data and render again. This new application architecture combines the simple “request/response” mental model of server-centric Multi-Page Apps with the seamless interactivity of client-centric Single-Page Apps, giving you the best of both worlds.
### Adding interactivity to Server Components[Link for Adding interactivity to Server Components]()
Server Components are not sent to the browser, so they cannot use interactive APIs like `useState`. To add interactivity to Server Components, you can compose them with Client Component using the `"use client"` directive.
### Note
#### There is no directive for Server Components.[Link for There is no directive for Server Components.]()
A common misunderstanding is that Server Components are denoted by `"use server"`, but there is no directive for Server Components. The `"use server"` directive is used for Server Functions.
For more info, see the docs for [Directives](https://react.dev/reference/rsc/directives).
In the following example, the `Notes` Server Component imports an `Expandable` Client Component that uses state to toggle its `expanded` state:
```
// Server Component
import Expandable from './Expandable';
async function Notes() {
const notes = await db.notes.getAll();
return (
<div>
{notes.map(note => (
<Expandable key={note.id}>
<p note={note} />
</Expandable>
))}
</div>
)
}
```
```
// Client Component
"use client"
export default function Expandable({children}) {
const [expanded, setExpanded] = useState(false);
return (
<div>
<button
onClick={() => setExpanded(!expanded)}
>
Toggle
</button>
{expanded && children}
</div>
)
}
```
This works by first rendering `Notes` as a Server Component, and then instructing the bundler to create a bundle for the Client Component `Expandable`. In the browser, the Client Components will see output of the Server Components passed as props:
```
<head>
<!-- the bundle for Client Components -->
<script src="bundle.js" />
</head>
<body>
<div>
<Expandable key={1}>
<p>this is the first note</p>
</Expandable>
<Expandable key={2}>
<p>this is the second note</p>
</Expandable>
<!--...-->
</div>
</body>
```
### Async components with Server Components[Link for Async components with Server Components]()
Server Components introduce a new way to write Components using async/await. When you `await` in an async component, React will suspend and wait for the promise to resolve before resuming rendering. This works across server/client boundaries with streaming support for Suspense.
You can even create a promise on the server, and await it on the client:
```
// Server Component
import db from './database';
async function Page({id}) {
// Will suspend the Server Component.
const note = await db.notes.get(id);
// NOTE: not awaited, will start here and await on the client.
const commentsPromise = db.comments.get(note.id);
return (
<div>
{note}
<Suspense fallback={<p>Loading Comments...</p>}>
<Comments commentsPromise={commentsPromise} />
</Suspense>
</div>
);
}
```
```
// Client Component
"use client";
import {use} from 'react';
function Comments({commentsPromise}) {
// NOTE: this will resume the promise from the server.
// It will suspend until the data is available.
const comments = use(commentsPromise);
return comments.map(commment => <p>{comment}</p>);
}
```
The `note` content is important data for the page to render, so we `await` it on the server. The comments are below the fold and lower-priority, so we start the promise on the server, and wait for it on the client with the `use` API. This will Suspend on the client, without blocking the `note` content from rendering.
Since async components are [not supported on the client](), we await the promise with `use`.
[NextServer Functions](https://react.dev/reference/rsc/server-functions) |
https://react.dev/reference/react-dom/static | [API Reference](https://react.dev/reference/react)
# Static React DOM APIs[Link for this heading]()
The `react-dom/static` APIs let you generate static HTML for React components. They have limited functionality compared to the streaming APIs. A [framework](https://react.dev/learn/start-a-new-react-project) may call them for you. Most of your components don’t need to import or use them.
* * *
## Static APIs for Web Streams[Link for Static APIs for Web Streams]()
These methods are only available in the environments with [Web Streams](https://developer.mozilla.org/en-US/docs/Web/API/Streams_API), which includes browsers, Deno, and some modern edge runtimes:
- [`prerender`](https://react.dev/reference/react-dom/static/prerender) renders a React tree to static HTML with a [Readable Web Stream.](https://developer.mozilla.org/en-US/docs/Web/API/ReadableStream)
* * *
## Static APIs for Node.js Streams[Link for Static APIs for Node.js Streams]()
These methods are only available in the environments with [Node.js Streams](https://nodejs.org/api/stream.html):
- [`prerenderToNodeStream`](https://react.dev/reference/react-dom/static/prerenderToNodeStream) renders a React tree to static HTML with a [Node.js Stream.](https://nodejs.org/api/stream.html)
[PreviousrenderToString](https://react.dev/reference/react-dom/server/renderToString)
[Nextprerender](https://react.dev/reference/react-dom/static/prerender) |
https://react.dev/blog/2021/06/08/the-plan-for-react-18 | [Blog](https://react.dev/blog)
# The Plan for React 18[Link for this heading]()
June 8, 2021 by [Andrew Clark](https://twitter.com/acdlite), [Brian Vaughn](https://github.com/bvaughn), [Christine Abernathy](https://twitter.com/abernathyca), [Dan Abramov](https://twitter.com/dan_abramov), [Rachel Nabors](https://twitter.com/rachelnabors), [Rick Hanlon](https://twitter.com/rickhanlonii), [Sebastian Markbåge](https://twitter.com/sebmarkbage), and [Seth Webster](https://twitter.com/sethwebster)
* * *
The React team is excited to share a few updates:
1. We’ve started work on the React 18 release, which will be our next major version.
2. We’ve created a Working Group to prepare the community for gradual adoption of new features in React 18.
3. We’ve published a React 18 Alpha so that library authors can try it and provide feedback.
These updates are primarily aimed at maintainers of third-party libraries. If you’re learning, teaching, or using React to build user-facing applications, you can safely ignore this post. But you are welcome to follow the discussions in the React 18 Working Group if you’re curious!
* * *
## What’s coming in React 18[Link for What’s coming in React 18]()
When it’s released, React 18 will include out-of-the-box improvements (like [automatic batching](https://github.com/reactwg/react-18/discussions/21)), new APIs (like [`startTransition`](https://github.com/reactwg/react-18/discussions/41)), and a [new streaming server renderer](https://github.com/reactwg/react-18/discussions/37) with built-in support for `React.lazy`.
These features are possible thanks to a new opt-in mechanism we’re adding in React 18. It’s called “concurrent rendering” and it lets React prepare multiple versions of the UI at the same time. This change is mostly behind-the-scenes, but it unlocks new possibilities to improve both real and perceived performance of your app.
If you’ve been following our research into the future of React (we don’t expect you to!), you might have heard of something called “concurrent mode” or that it might break your app. In response to this feedback from the community, we’ve redesigned the upgrade strategy for gradual adoption. Instead of an all-or-nothing “mode”, concurrent rendering will only be enabled for updates triggered by one of the new features. In practice, this means **you will be able to adopt React 18 without rewrites and try the new features at your own pace.**
## A gradual adoption strategy[Link for A gradual adoption strategy]()
Since concurrency in React 18 is opt-in, there are no significant out-of-the-box breaking changes to component behavior. **You can upgrade to React 18 with minimal or no changes to your application code, with a level of effort comparable to a typical major React release**. Based on our experience converting several apps to React 18, we expect that many users will be able to upgrade within a single afternoon.
We successfully shipped concurrent features to tens of thousands of components at Facebook, and in our experience, we’ve found that most React components “just work” without additional changes. We’re committed to making sure this is a smooth upgrade for the entire community, so today we’re announcing the React 18 Working Group.
## Working with the community[Link for Working with the community]()
We’re trying something new for this release: We’ve invited a panel of experts, developers, library authors, and educators from across the React community to participate in our [React 18 Working Group](https://github.com/reactwg/react-18) to provide feedback, ask questions, and collaborate on the release. We couldn’t invite everyone we wanted to this initial, small group, but if this experiment works out, we hope there will be more in the future!
**The goal of the React 18 Working Group is to prepare the ecosystem for a smooth, gradual adoption of React 18 by existing applications and libraries.** The Working Group is hosted on [GitHub Discussions](https://github.com/reactwg/react-18/discussions) and is available for the public to read. Members of the working group can leave feedback, ask questions, and share ideas. The core team will also use the discussions repo to share our research findings. As the stable release gets closer, any important information will also be posted on this blog.
For more information on upgrading to React 18, or additional resources about the release, see the [React 18 announcement post](https://github.com/reactwg/react-18/discussions/4).
## Accessing the React 18 Working Group[Link for Accessing the React 18 Working Group]()
Everyone can read the discussions in the [React 18 Working Group repo](https://github.com/reactwg/react-18).
Because we expect an initial surge of interest in the Working Group, only invited members will be allowed to create or comment on threads. However, the threads are fully visible to the public, so everyone has access to the same information. We believe this is a good compromise between creating a productive environment for working group members, while maintaining transparency with the wider community.
As always, you can submit bug reports, questions, and general feedback to our [issue tracker](https://github.com/facebook/react/issues).
## How to try React 18 Alpha today[Link for How to try React 18 Alpha today]()
New alphas are [regularly published to npm using the `@alpha` tag](https://github.com/reactwg/react-18/discussions/9). These releases are built using the most recent commit to our main repo. When a feature or bugfix is merged, it will appear in an alpha the following weekday.
There may be significant behavioral or API changes between alpha releases. Please remember that **alpha releases are not recommended for user-facing, production applications**.
## Projected React 18 release timeline[Link for Projected React 18 release timeline]()
We don’t have a specific release date scheduled, but we expect it will take several months of feedback and iteration before React 18 is ready for most production applications.
- Library Alpha: Available today
- Public Beta: At least several months
- Release Candidate (RC): At least several weeks after Beta
- General Availability: At least several weeks after RC
More details about our projected release timeline are [available in the Working Group](https://github.com/reactwg/react-18/discussions/9). We’ll post updates on this blog when we’re closer to a public release.
[PreviousReact Conf 2021 Recap](https://react.dev/blog/2021/12/17/react-conf-2021-recap)
[NextIntroducing Server Components](https://react.dev/blog/2020/12/21/data-fetching-with-react-server-components) |
https://react.dev/reference/rules | [API Reference](https://react.dev/reference/react)
# Rules of React[Link for this heading]()
Just as different programming languages have their own ways of expressing concepts, React has its own idioms — or rules — for how to express patterns in a way that is easy to understand and yields high-quality applications.
- [Components and Hooks must be pure]()
- [React calls Components and Hooks]()
- [Rules of Hooks]()
* * *
### Note
To learn more about expressing UIs with React, we recommend reading [Thinking in React](https://react.dev/learn/thinking-in-react).
This section describes the rules you need to follow to write idiomatic React code. Writing idiomatic React code can help you write well organized, safe, and composable applications. These properties make your app more resilient to changes and makes it easier to work with other developers, libraries, and tools.
These rules are known as the **Rules of React**. They are rules – and not just guidelines – in the sense that if they are broken, your app likely has bugs. Your code also becomes unidiomatic and harder to understand and reason about.
We strongly recommend using [Strict Mode](https://react.dev/reference/react/StrictMode) alongside React’s [ESLint plugin](https://www.npmjs.com/package/eslint-plugin-react-hooks) to help your codebase follow the Rules of React. By following the Rules of React, you’ll be able to find and address these bugs and keep your application maintainable.
* * *
## Components and Hooks must be pure[Link for Components and Hooks must be pure]()
[Purity in Components and Hooks](https://react.dev/reference/rules/components-and-hooks-must-be-pure) is a key rule of React that makes your app predictable, easy to debug, and allows React to automatically optimize your code.
- [Components must be idempotent](https://react.dev/reference/rules/components-and-hooks-must-be-pure) – React components are assumed to always return the same output with respect to their inputs – props, state, and context.
- [Side effects must run outside of render](https://react.dev/reference/rules/components-and-hooks-must-be-pure) – Side effects should not run in render, as React can render components multiple times to create the best possible user experience.
- [Props and state are immutable](https://react.dev/reference/rules/components-and-hooks-must-be-pure) – A component’s props and state are immutable snapshots with respect to a single render. Never mutate them directly.
- [Return values and arguments to Hooks are immutable](https://react.dev/reference/rules/components-and-hooks-must-be-pure) – Once values are passed to a Hook, you should not modify them. Like props in JSX, values become immutable when passed to a Hook.
- [Values are immutable after being passed to JSX](https://react.dev/reference/rules/components-and-hooks-must-be-pure) – Don’t mutate values after they’ve been used in JSX. Move the mutation before the JSX is created.
* * *
## React calls Components and Hooks[Link for React calls Components and Hooks]()
[React is responsible for rendering components and hooks when necessary to optimize the user experience.](https://react.dev/reference/rules/react-calls-components-and-hooks) It is declarative: you tell React what to render in your component’s logic, and React will figure out how best to display it to your user.
- [Never call component functions directly](https://react.dev/reference/rules/react-calls-components-and-hooks) – Components should only be used in JSX. Don’t call them as regular functions.
- [Never pass around hooks as regular values](https://react.dev/reference/rules/react-calls-components-and-hooks) – Hooks should only be called inside of components. Never pass it around as a regular value.
* * *
## Rules of Hooks[Link for Rules of Hooks]()
Hooks are defined using JavaScript functions, but they represent a special type of reusable UI logic with restrictions on where they can be called. You need to follow the [Rules of Hooks](https://react.dev/reference/rules/rules-of-hooks) when using them.
- [Only call Hooks at the top level](https://react.dev/reference/rules/rules-of-hooks) – Don’t call Hooks inside loops, conditions, or nested functions. Instead, always use Hooks at the top level of your React function, before any early returns.
- [Only call Hooks from React functions](https://react.dev/reference/rules/rules-of-hooks) – Don’t call Hooks from regular JavaScript functions.
[NextComponents and Hooks must be pure](https://react.dev/reference/rules/components-and-hooks-must-be-pure) |
https://react.dev/reference/rules/components-and-hooks-must-be-pure | [API Reference](https://react.dev/reference/react)
[Overview](https://react.dev/reference/rules)
# Components and Hooks must be pure[Link for this heading]()
Pure functions only perform a calculation and nothing more. It makes your code easier to understand, debug, and allows React to automatically optimize your components and Hooks correctly.
### Note
This reference page covers advanced topics and requires familiarity with the concepts covered in the [Keeping Components Pure](https://react.dev/learn/keeping-components-pure) page.
- [Why does purity matter?]()
- [Components and Hooks must be idempotent]()
- [Side effects must run outside of render]()
- [When is it okay to have mutation?]()
- [Props and state are immutable]()
- [Don’t mutate Props]()
- [Don’t mutate State]()
- [Return values and arguments to Hooks are immutable]()
- [Values are immutable after being passed to JSX]()
### Why does purity matter?[Link for Why does purity matter?]()
One of the key concepts that makes React, *React* is *purity*. A pure component or hook is one that is:
- **Idempotent** – You [always get the same result every time](https://react.dev/learn/keeping-components-pure) you run it with the same inputs – props, state, context for component inputs; and arguments for hook inputs.
- **Has no side effects in render** – Code with side effects should run [**separately from rendering**](). For example as an [event handler](https://react.dev/learn/responding-to-events) – where the user interacts with the UI and causes it to update; or as an [Effect](https://react.dev/reference/react/useEffect) – which runs after render.
- **Does not mutate non-local values**: Components and Hooks should [never modify values that aren’t created locally]() in render.
When render is kept pure, React can understand how to prioritize which updates are most important for the user to see first. This is made possible because of render purity: since components don’t have side effects [in render](), React can pause rendering components that aren’t as important to update, and only come back to them later when it’s needed.
Concretely, this means that rendering logic can be run multiple times in a way that allows React to give your user a pleasant user experience. However, if your component has an untracked side effect – like modifying the value of a global variable [during render]() – when React runs your rendering code again, your side effects will be triggered in a way that won’t match what you want. This often leads to unexpected bugs that can degrade how your users experience your app. You can see an [example of this in the Keeping Components Pure page](https://react.dev/learn/keeping-components-pure).
#### How does React run your code?[Link for How does React run your code?]()
React is declarative: you tell React *what* to render, and React will figure out *how* best to display it to your user. To do this, React has a few phases where it runs your code. You don’t need to know about all of these phases to use React well. But at a high level, you should know about what code runs in *render*, and what runs outside of it.
*Rendering* refers to calculating what the next version of your UI should look like. After rendering, [Effects](https://react.dev/reference/react/useEffect) are *flushed* (meaning they are run until there are no more left) and may update the calculation if the Effects have impacts on layout. React takes this new calculation and compares it to the calculation used to create the previous version of your UI, then *commits* just the minimum changes needed to the [DOM](https://developer.mozilla.org/en-US/docs/Web/API/Document_Object_Model) (what your user actually sees) to catch it up to the latest version.
##### Deep Dive
#### How to tell if code runs in render[Link for How to tell if code runs in render]()
Show Details
One quick heuristic to tell if code runs during render is to examine where it is: if it’s written at the top level like in the example below, there’s a good chance it runs during render.
```
function Dropdown() {
const selectedItems = new Set(); // created during render
// ...
}
```
Event handlers and Effects don’t run in render:
```
function Dropdown() {
const selectedItems = new Set();
const onSelect = (item) => {
// this code is in an event handler, so it's only run when the user triggers this
selectedItems.add(item);
}
}
```
```
function Dropdown() {
const selectedItems = new Set();
useEffect(() => {
// this code is inside of an Effect, so it only runs after rendering
logForAnalytics(selectedItems);
}, [selectedItems]);
}
```
* * *
## Components and Hooks must be idempotent[Link for Components and Hooks must be idempotent]()
Components must always return the same output with respect to their inputs – props, state, and context. This is known as *idempotency*. [Idempotency](https://en.wikipedia.org/wiki/Idempotence) is a term popularized in functional programming. It refers to the idea that you [always get the same result every time](https://react.dev/learn/keeping-components-pure) you run that piece of code with the same inputs.
This means that *all* code that runs [during render]() must also be idempotent in order for this rule to hold. For example, this line of code is not idempotent (and therefore, neither is the component):
```
function Clock() {
const time = new Date(); // 🔴 Bad: always returns a different result!
return <span>{time.toLocaleString()}</span>
}
```
`new Date()` is not idempotent as it always returns the current date and changes its result every time it’s called. When you render the above component, the time displayed on the screen will stay stuck on the time that the component was rendered. Similarly, functions like `Math.random()` also aren’t idempotent, because they return different results every time they’re called, even when the inputs are the same.
This doesn’t mean you shouldn’t use non-idempotent functions like `new Date()` *at all* – you should just avoid using them [during render](). In this case, we can *synchronize* the latest date to this component using an [Effect](https://react.dev/reference/react/useEffect):
App.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import { useState, useEffect } from 'react';
function useTime() {
// 1. Keep track of the current date's state. `useState` receives an initializer function as its
// initial state. It only runs once when the hook is called, so only the current date at the
// time the hook is called is set first.
const [time, setTime] = useState(() => new Date());
useEffect(() => {
// 2. Update the current date every second using `setInterval`.
const id = setInterval(() => {
setTime(new Date()); // ✅ Good: non-idempotent code no longer runs in render
}, 1000);
// 3. Return a cleanup function so we don't leak the `setInterval` timer.
return () => clearInterval(id);
}, []);
return time;
}
export default function Clock() {
const time = useTime();
return <span>{time.toLocaleString()}</span>;
}
```
Show more
By wrapping the non-idempotent `new Date()` call in an Effect, it moves that calculation [outside of rendering]().
If you don’t need to synchronize some external state with React, you can also consider using an [event handler](https://react.dev/learn/responding-to-events) if it only needs to be updated in response to a user interaction.
* * *
## Side effects must run outside of render[Link for Side effects must run outside of render]()
[Side effects](https://react.dev/learn/keeping-components-pure) should not run [in render](), as React can render components multiple times to create the best possible user experience.
### Note
Side effects are a broader term than Effects. Effects specifically refer to code that’s wrapped in `useEffect`, while a side effect is a general term for code that has any observable effect other than its primary result of returning a value to the caller.
Side effects are typically written inside of [event handlers](https://react.dev/learn/responding-to-events) or Effects. But never during render.
While render must be kept pure, side effects are necessary at some point in order for your app to do anything interesting, like showing something on the screen! The key point of this rule is that side effects should not run [in render](), as React can render components multiple times. In most cases, you’ll use [event handlers](https://react.dev/learn/responding-to-events) to handle side effects. Using an event handler explicitly tells React that this code doesn’t need to run during render, keeping render pure. If you’ve exhausted all options – and only as a last resort – you can also handle side effects using `useEffect`.
### When is it okay to have mutation?[Link for When is it okay to have mutation?]()
#### Local mutation[Link for Local mutation]()
One common example of a side effect is mutation, which in JavaScript refers to changing the value of a non-[primitive](https://developer.mozilla.org/en-US/docs/Glossary/Primitive) value. In general, while mutation is not idiomatic in React, *local* mutation is absolutely fine:
```
function FriendList({ friends }) {
const items = []; // ✅ Good: locally created
for (let i = 0; i < friends.length; i++) {
const friend = friends[i];
items.push(
<Friend key={friend.id} friend={friend} />
); // ✅ Good: local mutation is okay
}
return <section>{items}</section>;
}
```
There is no need to contort your code to avoid local mutation. [`Array.map`](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Array/map) could also be used here for brevity, but there is nothing wrong with creating a local array and then pushing items into it [during render]().
Even though it looks like we are mutating `items`, the key point to note is that this code only does so *locally* – the mutation isn’t “remembered” when the component is rendered again. In other words, `items` only stays around as long as the component does. Because `items` is always *recreated* every time `<FriendList />` is rendered, the component will always return the same result.
On the other hand, if `items` was created outside of the component, it holds on to its previous values and remembers changes:
```
const items = []; // 🔴 Bad: created outside of the component
function FriendList({ friends }) {
for (let i = 0; i < friends.length; i++) {
const friend = friends[i];
items.push(
<Friend key={friend.id} friend={friend} />
); // 🔴 Bad: mutates a value created outside of render
}
return <section>{items}</section>;
}
```
When `<FriendList />` runs again, we will continue appending `friends` to `items` every time that component is run, leading to multiple duplicated results. This version of `<FriendList />` has observable side effects [during render]() and **breaks the rule**.
#### Lazy initialization[Link for Lazy initialization]()
Lazy initialization is also fine despite not being fully “pure”:
```
function ExpenseForm() {
SuperCalculator.initializeIfNotReady(); // ✅ Good: if it doesn't affect other components
// Continue rendering...
}
```
#### Changing the DOM[Link for Changing the DOM]()
Side effects that are directly visible to the user are not allowed in the render logic of React components. In other words, merely calling a component function shouldn’t by itself produce a change on the screen.
```
function ProductDetailPage({ product }) {
document.title = product.title; // 🔴 Bad: Changes the DOM
}
```
One way to achieve the desired result of updating `document.title` outside of render is to [synchronize the component with `document`](https://react.dev/learn/synchronizing-with-effects).
As long as calling a component multiple times is safe and doesn’t affect the rendering of other components, React doesn’t care if it’s 100% pure in the strict functional programming sense of the word. It is more important that [components must be idempotent](https://react.dev/reference/rules/components-and-hooks-must-be-pure).
* * *
## Props and state are immutable[Link for Props and state are immutable]()
A component’s props and state are immutable [snapshots](https://react.dev/learn/state-as-a-snapshot). Never mutate them directly. Instead, pass new props down, and use the setter function from `useState`.
You can think of the props and state values as snapshots that are updated after rendering. For this reason, you don’t modify the props or state variables directly: instead you pass new props, or use the setter function provided to you to tell React that state needs to update the next time the component is rendered.
### Don’t mutate Props[Link for Don’t mutate Props]()
Props are immutable because if you mutate them, the application will produce inconsistent output, which can be hard to debug since it may or may not work depending on the circumstance.
```
function Post({ item }) {
item.url = new Url(item.url, base); // 🔴 Bad: never mutate props directly
return <Link url={item.url}>{item.title}</Link>;
}
```
```
function Post({ item }) {
const url = new Url(item.url, base); // ✅ Good: make a copy instead
return <Link url={url}>{item.title}</Link>;
}
```
### Don’t mutate State[Link for Don’t mutate State]()
`useState` returns the state variable and a setter to update that state.
```
const [stateVariable, setter] = useState(0);
```
Rather than updating the state variable in-place, we need to update it using the setter function that is returned by `useState`. Changing values on the state variable doesn’t cause the component to update, leaving your users with an outdated UI. Using the setter function informs React that the state has changed, and that we need to queue a re-render to update the UI.
```
function Counter() {
const [count, setCount] = useState(0);
function handleClick() {
count = count + 1; // 🔴 Bad: never mutate state directly
}
return (
<button onClick={handleClick}>
You pressed me {count} times
</button>
);
}
```
```
function Counter() {
const [count, setCount] = useState(0);
function handleClick() {
setCount(count + 1); // ✅ Good: use the setter function returned by useState
}
return (
<button onClick={handleClick}>
You pressed me {count} times
</button>
);
}
```
* * *
## Return values and arguments to Hooks are immutable[Link for Return values and arguments to Hooks are immutable]()
Once values are passed to a hook, you should not modify them. Like props in JSX, values become immutable when passed to a hook.
```
function useIconStyle(icon) {
const theme = useContext(ThemeContext);
if (icon.enabled) {
icon.className = computeStyle(icon, theme); // 🔴 Bad: never mutate hook arguments directly
}
return icon;
}
```
```
function useIconStyle(icon) {
const theme = useContext(ThemeContext);
const newIcon = { ...icon }; // ✅ Good: make a copy instead
if (icon.enabled) {
newIcon.className = computeStyle(icon, theme);
}
return newIcon;
}
```
One important principle in React is *local reasoning*: the ability to understand what a component or hook does by looking at its code in isolation. Hooks should be treated like “black boxes” when they are called. For example, a custom hook might have used its arguments as dependencies to memoize values inside it:
```
function useIconStyle(icon) {
const theme = useContext(ThemeContext);
return useMemo(() => {
const newIcon = { ...icon };
if (icon.enabled) {
newIcon.className = computeStyle(icon, theme);
}
return newIcon;
}, [icon, theme]);
}
```
If you were to mutate the Hooks arguments, the custom hook’s memoization will become incorrect, so it’s important to avoid doing that.
```
style = useIconStyle(icon); // `style` is memoized based on `icon`
icon.enabled = false; // Bad: 🔴 never mutate hook arguments directly
style = useIconStyle(icon); // previously memoized result is returned
```
```
style = useIconStyle(icon); // `style` is memoized based on `icon`
icon = { ...icon, enabled: false }; // Good: ✅ make a copy instead
style = useIconStyle(icon); // new value of `style` is calculated
```
Similarly, it’s important to not modify the return values of Hooks, as they may have been memoized.
* * *
## Values are immutable after being passed to JSX[Link for Values are immutable after being passed to JSX]()
Don’t mutate values after they’ve been used in JSX. Move the mutation before the JSX is created.
When you use JSX in an expression, React may eagerly evaluate the JSX before the component finishes rendering. This means that mutating values after they’ve been passed to JSX can lead to outdated UIs, as React won’t know to update the component’s output.
```
function Page({ colour }) {
const styles = { colour, size: "large" };
const header = <Header styles={styles} />;
styles.size = "small"; // 🔴 Bad: styles was already used in the JSX above
const footer = <Footer styles={styles} />;
return (
<>
{header}
<Content />
{footer}
</>
);
}
```
```
function Page({ colour }) {
const headerStyles = { colour, size: "large" };
const header = <Header styles={headerStyles} />;
const footerStyles = { colour, size: "small" }; // ✅ Good: we created a new value
const footer = <Footer styles={footerStyles} />;
return (
<>
{header}
<Content />
{footer}
</>
);
}
```
[PreviousOverview](https://react.dev/reference/rules)
[NextReact calls Components and Hooks](https://react.dev/reference/rules/react-calls-components-and-hooks) |
https://react.dev/reference/react-dom/static/prerenderToNodeStream | [API Reference](https://react.dev/reference/react)
[Static APIs](https://react.dev/reference/react-dom/static)
# prerenderToNodeStream[Link for this heading]()
`prerenderToNodeStream` renders a React tree to a static HTML string using a [Node.js Stream.](https://nodejs.org/api/stream.html).
```
const {prelude} = await prerenderToNodeStream(reactNode, options?)
```
- [Reference]()
- [`prerenderToNodeStream(reactNode, options?)`]()
- [Usage]()
- [Rendering a React tree to a stream of static HTML]()
- [Rendering a React tree to a string of static HTML]()
- [Waiting for all data to load]()
- [Troubleshooting]()
- [My stream doesn’t start until the entire app is rendered]()
### Note
This API is specific to Node.js. Environments with [Web Streams,](https://developer.mozilla.org/en-US/docs/Web/API/Streams_API) like Deno and modern edge runtimes, should use [`prerender`](https://react.dev/reference/react-dom/static/prerender) instead.
* * *
## Reference[Link for Reference]()
### `prerenderToNodeStream(reactNode, options?)`[Link for this heading]()
Call `prerenderToNodeStream` to render your app to static HTML.
```
import { prerenderToNodeStream } from 'react-dom/static';
// The route handler syntax depends on your backend framework
app.use('/', async (request, response) => {
const { prelude } = await prerenderToNodeStream(<App />, {
bootstrapScripts: ['/main.js'],
});
response.setHeader('Content-Type', 'text/plain');
prelude.pipe(response);
});
```
On the client, call [`hydrateRoot`](https://react.dev/reference/react-dom/client/hydrateRoot) to make the server-generated HTML interactive.
[See more examples below.]()
#### Parameters[Link for Parameters]()
- `reactNode`: A React node you want to render to HTML. For example, a JSX node like `<App />`. It is expected to represent the entire document, so the App component should render the `<html>` tag.
- **optional** `options`: An object with static generation options.
- **optional** `bootstrapScriptContent`: If specified, this string will be placed in an inline `<script>` tag.
- **optional** `bootstrapScripts`: An array of string URLs for the `<script>` tags to emit on the page. Use this to include the `<script>` that calls [`hydrateRoot`.](https://react.dev/reference/react-dom/client/hydrateRoot) Omit it if you don’t want to run React on the client at all.
- **optional** `bootstrapModules`: Like `bootstrapScripts`, but emits [`<script type="module">`](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Guide/Modules) instead.
- **optional** `identifierPrefix`: A string prefix React uses for IDs generated by [`useId`.](https://react.dev/reference/react/useId) Useful to avoid conflicts when using multiple roots on the same page. Must be the same prefix as passed to [`hydrateRoot`.](https://react.dev/reference/react-dom/client/hydrateRoot)
- **optional** `namespaceURI`: A string with the root [namespace URI](https://developer.mozilla.org/en-US/docs/Web/API/Document/createElementNS) for the stream. Defaults to regular HTML. Pass `'http://www.w3.org/2000/svg'` for SVG or `'http://www.w3.org/1998/Math/MathML'` for MathML.
- **optional** `onError`: A callback that fires whenever there is a server error, whether [recoverable]() or [not.]() By default, this only calls `console.error`. If you override it to [log crash reports,]() make sure that you still call `console.error`. You can also use it to [adjust the status code]() before the shell is emitted.
- **optional** `progressiveChunkSize`: The number of bytes in a chunk. [Read more about the default heuristic.](https://github.com/facebook/react/blob/14c2be8dac2d5482fda8a0906a31d239df8551fc/packages/react-server/src/ReactFizzServer.js)
- **optional** `signal`: An [abort signal](https://developer.mozilla.org/en-US/docs/Web/API/AbortSignal) that lets you [abort server rendering]() and render the rest on the client.
#### Returns[Link for Returns]()
`prerenderToNodeStream` returns a Promise:
- If rendering the is successful, the Promise will resolve to an object containing:
- `prelude`: a [Node.js Stream.](https://nodejs.org/api/stream.html) of HTML. You can use this stream to send a response in chunks, or you can read the entire stream into a string.
- If rendering fails, the Promise will be rejected. [Use this to output a fallback shell.]()
### Note
### When should I use `prerenderToNodeStream`?[Link for this heading]()
The static `prerenderToNodeStream` API is used for static server-side generation (SSG). Unlike `renderToString`, `prerenderToNodeStream` waits for all data to load before resolving. This makes it suitable for generating static HTML for a full page, including data that needs to be fetched using Suspense. To stream content as it loads, use a streaming server-side render (SSR) API like [renderToReadableStream](https://react.dev/reference/react-dom/server/renderToReadableStream).
* * *
## Usage[Link for Usage]()
### Rendering a React tree to a stream of static HTML[Link for Rendering a React tree to a stream of static HTML]()
Call `prerenderToNodeStream` to render your React tree to static HTML into a [Node.js Stream.](https://nodejs.org/api/stream.html):
```
import { prerenderToNodeStream } from 'react-dom/static';
// The route handler syntax depends on your backend framework
app.use('/', async (request, response) => {
const { prelude } = await prerenderToNodeStream(<App />, {
bootstrapScripts: ['/main.js'],
});
response.setHeader('Content-Type', 'text/plain');
prelude.pipe(response);
});
```
Along with the root component, you need to provide a list of bootstrap `<script>` paths. Your root component should return **the entire document including the root `<html>` tag.**
For example, it might look like this:
```
export default function App() {
return (
<html>
<head>
<meta charSet="utf-8" />
<meta name="viewport" content="width=device-width, initial-scale=1" />
<link rel="stylesheet" href="/styles.css"></link>
<title>My app</title>
</head>
<body>
<Router />
</body>
</html>
);
}
```
React will inject the [doctype](https://developer.mozilla.org/en-US/docs/Glossary/Doctype) and your bootstrap `<script>` tags into the resulting HTML stream:
```
<!DOCTYPE html>
<html>
<!-- ... HTML from your components ... -->
</html>
<script src="/main.js" async=""></script>
```
On the client, your bootstrap script should [hydrate the entire `document` with a call to `hydrateRoot`:](https://react.dev/reference/react-dom/client/hydrateRoot)
```
import { hydrateRoot } from 'react-dom/client';
import App from './App.js';
hydrateRoot(document, <App />);
```
This will attach event listeners to the static server-generated HTML and make it interactive.
##### Deep Dive
#### Reading CSS and JS asset paths from the build output[Link for Reading CSS and JS asset paths from the build output]()
Show Details
The final asset URLs (like JavaScript and CSS files) are often hashed after the build. For example, instead of `styles.css` you might end up with `styles.123456.css`. Hashing static asset filenames guarantees that every distinct build of the same asset will have a different filename. This is useful because it lets you safely enable long-term caching for static assets: a file with a certain name would never change content.
However, if you don’t know the asset URLs until after the build, there’s no way for you to put them in the source code. For example, hardcoding `"/styles.css"` into JSX like earlier wouldn’t work. To keep them out of your source code, your root component can read the real filenames from a map passed as a prop:
```
export default function App({ assetMap }) {
return (
<html>
<head>
<title>My app</title>
<link rel="stylesheet" href={assetMap['styles.css']}></link>
</head>
...
</html>
);
}
```
On the server, render `<App assetMap={assetMap} />` and pass your `assetMap` with the asset URLs:
```
// You'd need to get this JSON from your build tooling, e.g. read it from the build output.
const assetMap = {
'styles.css': '/styles.123456.css',
'main.js': '/main.123456.js'
};
app.use('/', async (request, response) => {
const { prelude } = await prerenderToNodeStream(<App />, {
bootstrapScripts: [assetMap['/main.js']]
});
response.setHeader('Content-Type', 'text/html');
prelude.pipe(response);
});
```
Since your server is now rendering `<App assetMap={assetMap} />`, you need to render it with `assetMap` on the client too to avoid hydration errors. You can serialize and pass `assetMap` to the client like this:
```
// You'd need to get this JSON from your build tooling.
const assetMap = {
'styles.css': '/styles.123456.css',
'main.js': '/main.123456.js'
};
app.use('/', async (request, response) => {
const { prelude } = await prerenderToNodeStream(<App />, {
// Careful: It's safe to stringify() this because this data isn't user-generated.
bootstrapScriptContent: `window.assetMap = ${JSON.stringify(assetMap)};`,
bootstrapScripts: [assetMap['/main.js']],
});
response.setHeader('Content-Type', 'text/html');
prelude.pipe(response);
});
```
In the example above, the `bootstrapScriptContent` option adds an extra inline `<script>` tag that sets the global `window.assetMap` variable on the client. This lets the client code read the same `assetMap`:
```
import { hydrateRoot } from 'react-dom/client';
import App from './App.js';
hydrateRoot(document, <App assetMap={window.assetMap} />);
```
Both client and server render `App` with the same `assetMap` prop, so there are no hydration errors.
* * *
### Rendering a React tree to a string of static HTML[Link for Rendering a React tree to a string of static HTML]()
Call `prerenderToNodeStream` to render your app to a static HTML string:
```
import { prerenderToNodeStream } from 'react-dom/static';
async function renderToString() {
const {prelude} = await prerenderToNodeStream(<App />, {
bootstrapScripts: ['/main.js']
});
return new Promise((resolve, reject) => {
let data = '';
prelude.on('data', chunk => {
data += chunk;
});
prelude.on('end', () => resolve(data));
prelude.on('error', reject);
});
}
```
This will produce the initial non-interactive HTML output of your React components. On the client, you will need to call [`hydrateRoot`](https://react.dev/reference/react-dom/client/hydrateRoot) to *hydrate* that server-generated HTML and make it interactive.
* * *
### Waiting for all data to load[Link for Waiting for all data to load]()
`prerenderToNodeStream` waits for all data to load before finishing the static HTML generation and resolving. For example, consider a profile page that shows a cover, a sidebar with friends and photos, and a list of posts:
```
function ProfilePage() {
return (
<ProfileLayout>
<ProfileCover />
<Sidebar>
<Friends />
<Photos />
</Sidebar>
<Suspense fallback={<PostsGlimmer />}>
<Posts />
</Suspense>
</ProfileLayout>
);
}
```
Imagine that `<Posts />` needs to load some data, which takes some time. Ideally, you’d want wait for the posts to finish so it’s included in the HTML. To do this, you can use Suspense to suspend on the data, and `prerenderToNodeStream` will wait for the suspended content to finish before resolving to the static HTML.
### Note
**Only Suspense-enabled data sources will activate the Suspense component.** They include:
- Data fetching with Suspense-enabled frameworks like [Relay](https://relay.dev/docs/guided-tour/rendering/loading-states/) and [Next.js](https://nextjs.org/docs/getting-started/react-essentials)
- Lazy-loading component code with [`lazy`](https://react.dev/reference/react/lazy)
- Reading the value of a Promise with [`use`](https://react.dev/reference/react/use)
Suspense **does not** detect when data is fetched inside an Effect or event handler.
The exact way you would load data in the `Posts` component above depends on your framework. If you use a Suspense-enabled framework, you’ll find the details in its data fetching documentation.
Suspense-enabled data fetching without the use of an opinionated framework is not yet supported. The requirements for implementing a Suspense-enabled data source are unstable and undocumented. An official API for integrating data sources with Suspense will be released in a future version of React.
* * *
## Troubleshooting[Link for Troubleshooting]()
### My stream doesn’t start until the entire app is rendered[Link for My stream doesn’t start until the entire app is rendered]()
The `prerenderToNodeStream` response waits for the entire app to finish rendering, including waiting for all suspense boundaries to resolve, before resolving. It is designed for static site generation (SSG) ahead of time and does not support streaming more content as it loads.
To stream content as it loads, use a streaming server render API like [renderToPipeableStream](https://react.dev/reference/react-dom/server/renderToPipeableStream).
[Previousprerender](https://react.dev/reference/react-dom/static/prerender) |
https://react.dev/blog/2022/03/29/react-v18 | [Blog](https://react.dev/blog)
# React v18.0[Link for this heading]()
March 29, 2022 by [The React Team](https://react.dev/community/team)
* * *
React 18 is now available on npm! In our last post, we shared step-by-step instructions for [upgrading your app to React 18](https://react.dev/blog/2022/03/08/react-18-upgrade-guide). In this post, we’ll give an overview of what’s new in React 18, and what it means for the future.
* * *
Our latest major version includes out-of-the-box improvements like automatic batching, new APIs like startTransition, and streaming server-side rendering with support for Suspense.
Many of the features in React 18 are built on top of our new concurrent renderer, a behind-the-scenes change that unlocks powerful new capabilities. Concurrent React is opt-in — it’s only enabled when you use a concurrent feature — but we think it will have a big impact on the way people build applications.
We’ve spent years researching and developing support for concurrency in React, and we’ve taken extra care to provide a gradual adoption path for existing users. Last summer, [we formed the React 18 Working Group](https://react.dev/blog/2021/06/08/the-plan-for-react-18) to gather feedback from experts in the community and ensure a smooth upgrade experience for the entire React ecosystem.
In case you missed it, we shared a lot of this vision at React Conf 2021:
- In [the keynote](https://www.youtube.com/watch?v=FZ0cG47msEk&list=PLNG_1j3cPCaZZ7etkzWA7JfdmKWT0pMsa), we explain how React 18 fits into our mission to make it easy for developers to build great user experiences
- [Shruti Kapoor](https://twitter.com/shrutikapoor08) [demonstrated how to use the new features in React 18](https://www.youtube.com/watch?v=ytudH8je5ko&list=PLNG_1j3cPCaZZ7etkzWA7JfdmKWT0pMsa&index=2)
- [Shaundai Person](https://twitter.com/shaundai) gave us an overview of [streaming server rendering with Suspense](https://www.youtube.com/watch?v=pj5N-Khihgc&list=PLNG_1j3cPCaZZ7etkzWA7JfdmKWT0pMsa&index=3)
Below is a full overview of what to expect in this release, starting with Concurrent Rendering.
### Note
For React Native users, React 18 will ship in React Native with the New React Native Architecture. For more information, see the [React Conf keynote here](https://www.youtube.com/watch?v=FZ0cG47msEk&t=1530s).
## What is Concurrent React?[Link for What is Concurrent React?]()
The most important addition in React 18 is something we hope you never have to think about: concurrency. We think this is largely true for application developers, though the story may be a bit more complicated for library maintainers.
Concurrency is not a feature, per se. It’s a new behind-the-scenes mechanism that enables React to prepare multiple versions of your UI at the same time. You can think of concurrency as an implementation detail — it’s valuable because of the features that it unlocks. React uses sophisticated techniques in its internal implementation, like priority queues and multiple buffering. But you won’t see those concepts anywhere in our public APIs.
When we design APIs, we try to hide implementation details from developers. As a React developer, you focus on *what* you want the user experience to look like, and React handles *how* to deliver that experience. So we don’t expect React developers to know how concurrency works under the hood.
However, Concurrent React is more important than a typical implementation detail — it’s a foundational update to React’s core rendering model. So while it’s not super important to know how concurrency works, it may be worth knowing what it is at a high level.
A key property of Concurrent React is that rendering is interruptible. When you first upgrade to React 18, before adding any concurrent features, updates are rendered the same as in previous versions of React — in a single, uninterrupted, synchronous transaction. With synchronous rendering, once an update starts rendering, nothing can interrupt it until the user can see the result on screen.
In a concurrent render, this is not always the case. React may start rendering an update, pause in the middle, then continue later. It may even abandon an in-progress render altogether. React guarantees that the UI will appear consistent even if a render is interrupted. To do this, it waits to perform DOM mutations until the end, once the entire tree has been evaluated. With this capability, React can prepare new screens in the background without blocking the main thread. This means the UI can respond immediately to user input even if it’s in the middle of a large rendering task, creating a fluid user experience.
Another example is reusable state. Concurrent React can remove sections of the UI from the screen, then add them back later while reusing the previous state. For example, when a user tabs away from a screen and back, React should be able to restore the previous screen in the same state it was in before. In an upcoming minor, we’re planning to add a new component called `<Offscreen>` that implements this pattern. Similarly, you’ll be able to use Offscreen to prepare new UI in the background so that it’s ready before the user reveals it.
Concurrent rendering is a powerful new tool in React and most of our new features are built to take advantage of it, including Suspense, transitions, and streaming server rendering. But React 18 is just the beginning of what we aim to build on this new foundation.
## Gradually Adopting Concurrent Features[Link for Gradually Adopting Concurrent Features]()
Technically, concurrent rendering is a breaking change. Because concurrent rendering is interruptible, components behave slightly differently when it is enabled.
In our testing, we’ve upgraded thousands of components to React 18. What we’ve found is that nearly all existing components “just work” with concurrent rendering, without any changes. However, some of them may require some additional migration effort. Although the changes are usually small, you’ll still have the ability to make them at your own pace. The new rendering behavior in React 18 is **only enabled in the parts of your app that use new features.**
The overall upgrade strategy is to get your application running on React 18 without breaking existing code. Then you can gradually start adding concurrent features at your own pace. You can use [`<StrictMode>`](https://react.dev/reference/react/StrictMode) to help surface concurrency-related bugs during development. Strict Mode doesn’t affect production behavior, but during development it will log extra warnings and double-invoke functions that are expected to be idempotent. It won’t catch everything, but it’s effective at preventing the most common types of mistakes.
After you upgrade to React 18, you’ll be able to start using concurrent features immediately. For example, you can use startTransition to navigate between screens without blocking user input. Or useDeferredValue to throttle expensive re-renders.
However, long term, we expect the main way you’ll add concurrency to your app is by using a concurrent-enabled library or framework. In most cases, you won’t interact with concurrent APIs directly. For example, instead of developers calling startTransition whenever they navigate to a new screen, router libraries will automatically wrap navigations in startTransition.
It may take some time for libraries to upgrade to be concurrent compatible. We’ve provided new APIs to make it easier for libraries to take advantage of concurrent features. In the meantime, please be patient with maintainers as we work to gradually migrate the React ecosystem.
For more info, see our previous post: [How to upgrade to React 18](https://react.dev/blog/2022/03/08/react-18-upgrade-guide).
## Suspense in Data Frameworks[Link for Suspense in Data Frameworks]()
In React 18, you can start using [Suspense](https://react.dev/reference/react/Suspense) for data fetching in opinionated frameworks like Relay, Next.js, Hydrogen, or Remix. Ad hoc data fetching with Suspense is technically possible, but still not recommended as a general strategy.
In the future, we may expose additional primitives that could make it easier to access your data with Suspense, perhaps without the use of an opinionated framework. However, Suspense works best when it’s deeply integrated into your application’s architecture: your router, your data layer, and your server rendering environment. So even long term, we expect that libraries and frameworks will play a crucial role in the React ecosystem.
As in previous versions of React, you can also use Suspense for code splitting on the client with React.lazy. But our vision for Suspense has always been about much more than loading code — the goal is to extend support for Suspense so that eventually, the same declarative Suspense fallback can handle any asynchronous operation (loading code, data, images, etc).
## Server Components is Still in Development[Link for Server Components is Still in Development]()
[**Server Components**](https://react.dev/blog/2020/12/21/data-fetching-with-react-server-components) is an upcoming feature that allows developers to build apps that span the server and client, combining the rich interactivity of client-side apps with the improved performance of traditional server rendering. Server Components is not inherently coupled to Concurrent React, but it’s designed to work best with concurrent features like Suspense and streaming server rendering.
Server Components is still experimental, but we expect to release an initial version in a minor 18.x release. In the meantime, we’re working with frameworks like Next.js, Hydrogen, and Remix to advance the proposal and get it ready for broad adoption.
## What’s New in React 18[Link for What’s New in React 18]()
### New Feature: Automatic Batching[Link for New Feature: Automatic Batching]()
Batching is when React groups multiple state updates into a single re-render for better performance. Without automatic batching, we only batched updates inside React event handlers. Updates inside of promises, setTimeout, native event handlers, or any other event were not batched in React by default. With automatic batching, these updates will be batched automatically:
```
// Before: only React events were batched.
setTimeout(() => {
setCount(c => c + 1);
setFlag(f => !f);
// React will render twice, once for each state update (no batching)
}, 1000);
// After: updates inside of timeouts, promises,
// native event handlers or any other event are batched.
setTimeout(() => {
setCount(c => c + 1);
setFlag(f => !f);
// React will only re-render once at the end (that's batching!)
}, 1000);
```
For more info, see this post for [Automatic batching for fewer renders in React 18](https://github.com/reactwg/react-18/discussions/21).
### New Feature: Transitions[Link for New Feature: Transitions]()
A transition is a new concept in React to distinguish between urgent and non-urgent updates.
- **Urgent updates** reflect direct interaction, like typing, clicking, pressing, and so on.
- **Transition updates** transition the UI from one view to another.
Urgent updates like typing, clicking, or pressing, need immediate response to match our intuitions about how physical objects behave. Otherwise they feel “wrong”. However, transitions are different because the user doesn’t expect to see every intermediate value on screen.
For example, when you select a filter in a dropdown, you expect the filter button itself to respond immediately when you click. However, the actual results may transition separately. A small delay would be imperceptible and often expected. And if you change the filter again before the results are done rendering, you only care to see the latest results.
Typically, for the best user experience, a single user input should result in both an urgent update and a non-urgent one. You can use startTransition API inside an input event to inform React which updates are urgent and which are “transitions”:
```
import { startTransition } from 'react';
// Urgent: Show what was typed
setInputValue(input);
// Mark any state updates inside as transitions
startTransition(() => {
// Transition: Show the results
setSearchQuery(input);
});
```
Updates wrapped in startTransition are handled as non-urgent and will be interrupted if more urgent updates like clicks or key presses come in. If a transition gets interrupted by the user (for example, by typing multiple characters in a row), React will throw out the stale rendering work that wasn’t finished and render only the latest update.
- `useTransition`: a Hook to start transitions, including a value to track the pending state.
- `startTransition`: a method to start transitions when the Hook cannot be used.
Transitions will opt in to concurrent rendering, which allows the update to be interrupted. If the content re-suspends, transitions also tell React to continue showing the current content while rendering the transition content in the background (see the [Suspense RFC](https://github.com/reactjs/rfcs/blob/main/text/0213-suspense-in-react-18.md) for more info).
[See docs for transitions here](https://react.dev/reference/react/useTransition).
### New Suspense Features[Link for New Suspense Features]()
Suspense lets you declaratively specify the loading state for a part of the component tree if it’s not yet ready to be displayed:
```
<Suspense fallback={<Spinner />}>
<Comments />
</Suspense>
```
Suspense makes the “UI loading state” a first-class declarative concept in the React programming model. This lets us build higher-level features on top of it.
We introduced a limited version of Suspense several years ago. However, the only supported use case was code splitting with React.lazy, and it wasn’t supported at all when rendering on the server.
In React 18, we’ve added support for Suspense on the server and expanded its capabilities using concurrent rendering features.
Suspense in React 18 works best when combined with the transition API. If you suspend during a transition, React will prevent already-visible content from being replaced by a fallback. Instead, React will delay the render until enough data has loaded to prevent a bad loading state.
For more, see the RFC for [Suspense in React 18](https://github.com/reactjs/rfcs/blob/main/text/0213-suspense-in-react-18.md).
### New Client and Server Rendering APIs[Link for New Client and Server Rendering APIs]()
In this release we took the opportunity to redesign the APIs we expose for rendering on the client and server. These changes allow users to continue using the old APIs in React 17 mode while they upgrade to the new APIs in React 18.
#### React DOM Client[Link for React DOM Client]()
These new APIs are now exported from `react-dom/client`:
- `createRoot`: New method to create a root to `render` or `unmount`. Use it instead of `ReactDOM.render`. New features in React 18 don’t work without it.
- `hydrateRoot`: New method to hydrate a server rendered application. Use it instead of `ReactDOM.hydrate` in conjunction with the new React DOM Server APIs. New features in React 18 don’t work without it.
Both `createRoot` and `hydrateRoot` accept a new option called `onRecoverableError` in case you want to be notified when React recovers from errors during rendering or hydration for logging. By default, React will use [`reportError`](https://developer.mozilla.org/en-US/docs/Web/API/reportError), or `console.error` in the older browsers.
[See docs for React DOM Client here](https://react.dev/reference/react-dom/client).
#### React DOM Server[Link for React DOM Server]()
These new APIs are now exported from `react-dom/server` and have full support for streaming Suspense on the server:
- `renderToPipeableStream`: for streaming in Node environments.
- `renderToReadableStream`: for modern edge runtime environments, such as Deno and Cloudflare workers.
The existing `renderToString` method keeps working but is discouraged.
[See docs for React DOM Server here](https://react.dev/reference/react-dom/server).
### New Strict Mode Behaviors[Link for New Strict Mode Behaviors]()
In the future, we’d like to add a feature that allows React to add and remove sections of the UI while preserving state. For example, when a user tabs away from a screen and back, React should be able to immediately show the previous screen. To do this, React would unmount and remount trees using the same component state as before.
This feature will give React apps better performance out-of-the-box, but requires components to be resilient to effects being mounted and destroyed multiple times. Most effects will work without any changes, but some effects assume they are only mounted or destroyed once.
To help surface these issues, React 18 introduces a new development-only check to Strict Mode. This new check will automatically unmount and remount every component, whenever a component mounts for the first time, restoring the previous state on the second mount.
Before this change, React would mount the component and create the effects:
```
* React mounts the component.
* Layout effects are created.
* Effects are created.
```
With Strict Mode in React 18, React will simulate unmounting and remounting the component in development mode:
```
* React mounts the component.
* Layout effects are created.
* Effects are created.
* React simulates unmounting the component.
* Layout effects are destroyed.
* Effects are destroyed.
* React simulates mounting the component with the previous state.
* Layout effects are created.
* Effects are created.
```
[See docs for ensuring reusable state here](https://react.dev/reference/react/StrictMode).
### New Hooks[Link for New Hooks]()
#### useId[Link for useId]()
`useId` is a new Hook for generating unique IDs on both the client and server, while avoiding hydration mismatches. It is primarily useful for component libraries integrating with accessibility APIs that require unique IDs. This solves an issue that already exists in React 17 and below, but it’s even more important in React 18 because of how the new streaming server renderer delivers HTML out-of-order. [See docs here](https://react.dev/reference/react/useId).
> Note
>
> `useId` is **not** for generating [keys in a list](https://react.dev/learn/rendering-lists). Keys should be generated from your data.
#### useTransition[Link for useTransition]()
`useTransition` and `startTransition` let you mark some state updates as not urgent. Other state updates are considered urgent by default. React will allow urgent state updates (for example, updating a text input) to interrupt non-urgent state updates (for example, rendering a list of search results). [See docs here](https://react.dev/reference/react/useTransition).
#### useDeferredValue[Link for useDeferredValue]()
`useDeferredValue` lets you defer re-rendering a non-urgent part of the tree. It is similar to debouncing, but has a few advantages compared to it. There is no fixed time delay, so React will attempt the deferred render right after the first render is reflected on the screen. The deferred render is interruptible and doesn’t block user input. [See docs here](https://react.dev/reference/react/useDeferredValue).
#### useSyncExternalStore[Link for useSyncExternalStore]()
`useSyncExternalStore` is a new Hook that allows external stores to support concurrent reads by forcing updates to the store to be synchronous. It removes the need for useEffect when implementing subscriptions to external data sources, and is recommended for any library that integrates with state external to React. [See docs here](https://react.dev/reference/react/useSyncExternalStore).
> Note
>
> `useSyncExternalStore` is intended to be used by libraries, not application code.
#### useInsertionEffect[Link for useInsertionEffect]()
`useInsertionEffect` is a new Hook that allows CSS-in-JS libraries to address performance issues of injecting styles in render. Unless you’ve already built a CSS-in-JS library we don’t expect you to ever use this. This Hook will run after the DOM is mutated, but before layout effects read the new layout. This solves an issue that already exists in React 17 and below, but is even more important in React 18 because React yields to the browser during concurrent rendering, giving it a chance to recalculate layout. [See docs here](https://react.dev/reference/react/useInsertionEffect).
> Note
>
> `useInsertionEffect` is intended to be used by libraries, not application code.
## How to Upgrade[Link for How to Upgrade]()
See [How to Upgrade to React 18](https://react.dev/blog/2022/03/08/react-18-upgrade-guide) for step-by-step instructions and a full list of breaking and notable changes.
## Changelog[Link for Changelog]()
### React[Link for React]()
- Add `useTransition` and `useDeferredValue` to separate urgent updates from transitions. ([#10426](https://github.com/facebook/react/pull/10426), [#10715](https://github.com/facebook/react/pull/10715), [#15593](https://github.com/facebook/react/pull/15593), [#15272](https://github.com/facebook/react/pull/15272), [#15578](https://github.com/facebook/react/pull/15578), [#15769](https://github.com/facebook/react/pull/15769), [#17058](https://github.com/facebook/react/pull/17058), [#18796](https://github.com/facebook/react/pull/18796), [#19121](https://github.com/facebook/react/pull/19121), [#19703](https://github.com/facebook/react/pull/19703), [#19719](https://github.com/facebook/react/pull/19719), [#19724](https://github.com/facebook/react/pull/19724), [#20672](https://github.com/facebook/react/pull/20672), [#20976](https://github.com/facebook/react/pull/20976) by [@acdlite](https://github.com/acdlite), [@lunaruan](https://github.com/lunaruan), [@rickhanlonii](https://github.com/rickhanlonii), and [@sebmarkbage](https://github.com/sebmarkbage))
- Add `useId` for generating unique IDs. ([#17322](https://github.com/facebook/react/pull/17322), [#18576](https://github.com/facebook/react/pull/18576), [#22644](https://github.com/facebook/react/pull/22644), [#22672](https://github.com/facebook/react/pull/22672), [#21260](https://github.com/facebook/react/pull/21260) by [@acdlite](https://github.com/acdlite), [@lunaruan](https://github.com/lunaruan), and [@sebmarkbage](https://github.com/sebmarkbage))
- Add `useSyncExternalStore` to help external store libraries integrate with React. ([#15022](https://github.com/facebook/react/pull/15022), [#18000](https://github.com/facebook/react/pull/18000), [#18771](https://github.com/facebook/react/pull/18771), [#22211](https://github.com/facebook/react/pull/22211), [#22292](https://github.com/facebook/react/pull/22292), [#22239](https://github.com/facebook/react/pull/22239), [#22347](https://github.com/facebook/react/pull/22347), [#23150](https://github.com/facebook/react/pull/23150) by [@acdlite](https://github.com/acdlite), [@bvaughn](https://github.com/bvaughn), and [@drarmstr](https://github.com/drarmstr))
- Add `startTransition` as a version of `useTransition` without pending feedback. ([#19696](https://github.com/facebook/react/pull/19696) by [@rickhanlonii](https://github.com/rickhanlonii))
- Add `useInsertionEffect` for CSS-in-JS libraries. ([#21913](https://github.com/facebook/react/pull/21913) by [@rickhanlonii](https://github.com/rickhanlonii))
- Make Suspense remount layout effects when content reappears. ([#19322](https://github.com/facebook/react/pull/19322), [#19374](https://github.com/facebook/react/pull/19374), [#19523](https://github.com/facebook/react/pull/19523), [#20625](https://github.com/facebook/react/pull/20625), [#21079](https://github.com/facebook/react/pull/21079) by [@acdlite](https://github.com/acdlite), [@bvaughn](https://github.com/bvaughn), and [@lunaruan](https://github.com/lunaruan))
- Make `<StrictMode>` re-run effects to check for restorable state. ([#19523](https://github.com/facebook/react/pull/19523) , [#21418](https://github.com/facebook/react/pull/21418) by [@bvaughn](https://github.com/bvaughn) and [@lunaruan](https://github.com/lunaruan))
- Assume Symbols are always available. ([#23348](https://github.com/facebook/react/pull/23348) by [@sebmarkbage](https://github.com/sebmarkbage))
- Remove `object-assign` polyfill. ([#23351](https://github.com/facebook/react/pull/23351) by [@sebmarkbage](https://github.com/sebmarkbage))
- Remove unsupported `unstable_changedBits` API. ([#20953](https://github.com/facebook/react/pull/20953) by [@acdlite](https://github.com/acdlite))
- Allow components to render undefined. ([#21869](https://github.com/facebook/react/pull/21869) by [@rickhanlonii](https://github.com/rickhanlonii))
- Flush `useEffect` resulting from discrete events like clicks synchronously. ([#21150](https://github.com/facebook/react/pull/21150) by [@acdlite](https://github.com/acdlite))
- Suspense `fallback={undefined}` now behaves the same as `null` and isn’t ignored. ([#21854](https://github.com/facebook/react/pull/21854) by [@rickhanlonii](https://github.com/rickhanlonii))
- Consider all `lazy()` resolving to the same component equivalent. ([#20357](https://github.com/facebook/react/pull/20357) by [@sebmarkbage](https://github.com/sebmarkbage))
- Don’t patch console during first render. ([#22308](https://github.com/facebook/react/pull/22308) by [@lunaruan](https://github.com/lunaruan))
- Improve memory usage. ([#21039](https://github.com/facebook/react/pull/21039) by [@bgirard](https://github.com/bgirard))
- Improve messages if string coercion throws (Temporal.\*, Symbol, etc.) ([#22064](https://github.com/facebook/react/pull/22064) by [@justingrant](https://github.com/justingrant))
- Use `setImmediate` when available over `MessageChannel`. ([#20834](https://github.com/facebook/react/pull/20834) by [@gaearon](https://github.com/gaearon))
- Fix context failing to propagate inside suspended trees. ([#23095](https://github.com/facebook/react/pull/23095) by [@gaearon](https://github.com/gaearon))
- Fix `useReducer` observing incorrect props by removing the eager bailout mechanism. ([#22445](https://github.com/facebook/react/pull/22445) by [@josephsavona](https://github.com/josephsavona))
- Fix `setState` being ignored in Safari when appending iframes. ([#23111](https://github.com/facebook/react/pull/23111) by [@gaearon](https://github.com/gaearon))
- Fix a crash when rendering `ZonedDateTime` in the tree. ([#20617](https://github.com/facebook/react/pull/20617) by [@dimaqq](https://github.com/dimaqq))
- Fix a crash when document is set to `null` in tests. ([#22695](https://github.com/facebook/react/pull/22695) by [@SimenB](https://github.com/SimenB))
- Fix `onLoad` not triggering when concurrent features are on. ([#23316](https://github.com/facebook/react/pull/23316) by [@gnoff](https://github.com/gnoff))
- Fix a warning when a selector returns `NaN`. ([#23333](https://github.com/facebook/react/pull/23333) by [@hachibeeDI](https://github.com/hachibeeDI))
- Fix a crash when document is set to `null` in tests. ([#22695](https://github.com/facebook/react/pull/22695) by [@SimenB](https://github.com/SimenB))
- Fix the generated license header. ([#23004](https://github.com/facebook/react/pull/23004) by [@vitaliemiron](https://github.com/vitaliemiron))
- Add `package.json` as one of the entry points. ([#22954](https://github.com/facebook/react/pull/22954) by [@Jack](https://github.com/Jack-Works))
- Allow suspending outside a Suspense boundary. ([#23267](https://github.com/facebook/react/pull/23267) by [@acdlite](https://github.com/acdlite))
- Log a recoverable error whenever hydration fails. ([#23319](https://github.com/facebook/react/pull/23319) by [@acdlite](https://github.com/acdlite))
### React DOM[Link for React DOM]()
- Add `createRoot` and `hydrateRoot`. ([#10239](https://github.com/facebook/react/pull/10239), [#11225](https://github.com/facebook/react/pull/11225), [#12117](https://github.com/facebook/react/pull/12117), [#13732](https://github.com/facebook/react/pull/13732), [#15502](https://github.com/facebook/react/pull/15502), [#15532](https://github.com/facebook/react/pull/15532), [#17035](https://github.com/facebook/react/pull/17035), [#17165](https://github.com/facebook/react/pull/17165), [#20669](https://github.com/facebook/react/pull/20669), [#20748](https://github.com/facebook/react/pull/20748), [#20888](https://github.com/facebook/react/pull/20888), [#21072](https://github.com/facebook/react/pull/21072), [#21417](https://github.com/facebook/react/pull/21417), [#21652](https://github.com/facebook/react/pull/21652), [#21687](https://github.com/facebook/react/pull/21687), [#23207](https://github.com/facebook/react/pull/23207), [#23385](https://github.com/facebook/react/pull/23385) by [@acdlite](https://github.com/acdlite), [@bvaughn](https://github.com/bvaughn), [@gaearon](https://github.com/gaearon), [@lunaruan](https://github.com/lunaruan), [@rickhanlonii](https://github.com/rickhanlonii), [@trueadm](https://github.com/trueadm), and [@sebmarkbage](https://github.com/sebmarkbage))
- Add selective hydration. ([#14717](https://github.com/facebook/react/pull/14717), [#14884](https://github.com/facebook/react/pull/14884), [#16725](https://github.com/facebook/react/pull/16725), [#16880](https://github.com/facebook/react/pull/16880), [#17004](https://github.com/facebook/react/pull/17004), [#22416](https://github.com/facebook/react/pull/22416), [#22629](https://github.com/facebook/react/pull/22629), [#22448](https://github.com/facebook/react/pull/22448), [#22856](https://github.com/facebook/react/pull/22856), [#23176](https://github.com/facebook/react/pull/23176) by [@acdlite](https://github.com/acdlite), [@gaearon](https://github.com/gaearon), [@salazarm](https://github.com/salazarm), and [@sebmarkbage](https://github.com/sebmarkbage))
- Add `aria-description` to the list of known ARIA attributes. ([#22142](https://github.com/facebook/react/pull/22142) by [@mahyareb](https://github.com/mahyareb))
- Add `onResize` event to video elements. ([#21973](https://github.com/facebook/react/pull/21973) by [@rileyjshaw](https://github.com/rileyjshaw))
- Add `imageSizes` and `imageSrcSet` to known props. ([#22550](https://github.com/facebook/react/pull/22550) by [@eps1lon](https://github.com/eps1lon))
- Allow non-string `<option>` children if `value` is provided. ([#21431](https://github.com/facebook/react/pull/21431) by [@sebmarkbage](https://github.com/sebmarkbage))
- Fix `aspectRatio` style not being applied. ([#21100](https://github.com/facebook/react/pull/21100) by [@gaearon](https://github.com/gaearon))
- Warn if `renderSubtreeIntoContainer` is called. ([#23355](https://github.com/facebook/react/pull/23355) by [@acdlite](https://github.com/acdlite))
### React DOM Server[Link for React DOM Server]()
- Add the new streaming renderer. ([#14144](https://github.com/facebook/react/pull/14144), [#20970](https://github.com/facebook/react/pull/20970), [#21056](https://github.com/facebook/react/pull/21056), [#21255](https://github.com/facebook/react/pull/21255), [#21200](https://github.com/facebook/react/pull/21200), [#21257](https://github.com/facebook/react/pull/21257), [#21276](https://github.com/facebook/react/pull/21276), [#22443](https://github.com/facebook/react/pull/22443), [#22450](https://github.com/facebook/react/pull/22450), [#23247](https://github.com/facebook/react/pull/23247), [#24025](https://github.com/facebook/react/pull/24025), [#24030](https://github.com/facebook/react/pull/24030) by [@sebmarkbage](https://github.com/sebmarkbage))
- Fix context providers in SSR when handling multiple requests. ([#23171](https://github.com/facebook/react/pull/23171) by [@frandiox](https://github.com/frandiox))
- Revert to client render on text mismatch. ([#23354](https://github.com/facebook/react/pull/23354) by [@acdlite](https://github.com/acdlite))
- Deprecate `renderToNodeStream`. ([#23359](https://github.com/facebook/react/pull/23359) by [@sebmarkbage](https://github.com/sebmarkbage))
- Fix a spurious error log in the new server renderer. ([#24043](https://github.com/facebook/react/pull/24043) by [@eps1lon](https://github.com/eps1lon))
- Fix a bug in the new server renderer. ([#22617](https://github.com/facebook/react/pull/22617) by [@shuding](https://github.com/shuding))
- Ignore function and symbol values inside custom elements on the server. ([#21157](https://github.com/facebook/react/pull/21157) by [@sebmarkbage](https://github.com/sebmarkbage))
### React DOM Test Utils[Link for React DOM Test Utils]()
- Throw when `act` is used in production. ([#21686](https://github.com/facebook/react/pull/21686) by [@acdlite](https://github.com/acdlite))
- Support disabling spurious act warnings with `global.IS_REACT_ACT_ENVIRONMENT`. ([#22561](https://github.com/facebook/react/pull/22561) by [@acdlite](https://github.com/acdlite))
- Expand act warning to cover all APIs that might schedule React work. ([#22607](https://github.com/facebook/react/pull/22607) by [@acdlite](https://github.com/acdlite))
- Make `act` batch updates. ([#21797](https://github.com/facebook/react/pull/21797) by [@acdlite](https://github.com/acdlite))
- Remove warning for dangling passive effects. ([#22609](https://github.com/facebook/react/pull/22609) by [@acdlite](https://github.com/acdlite))
### React Refresh[Link for React Refresh]()
- Track late-mounted roots in Fast Refresh. ([#22740](https://github.com/facebook/react/pull/22740) by [@anc95](https://github.com/anc95))
- Add `exports` field to `package.json`. ([#23087](https://github.com/facebook/react/pull/23087) by [@otakustay](https://github.com/otakustay))
### Server Components (Experimental)[Link for Server Components (Experimental)]()
- Add Server Context support. ([#23244](https://github.com/facebook/react/pull/23244) by [@salazarm](https://github.com/salazarm))
- Add `lazy` support. ([#24068](https://github.com/facebook/react/pull/24068) by [@gnoff](https://github.com/gnoff))
- Update webpack plugin for webpack 5 ([#22739](https://github.com/facebook/react/pull/22739) by [@michenly](https://github.com/michenly))
- Fix a mistake in the Node loader. ([#22537](https://github.com/facebook/react/pull/22537) by [@btea](https://github.com/btea))
- Use `globalThis` instead of `window` for edge environments. ([#22777](https://github.com/facebook/react/pull/22777) by [@huozhi](https://github.com/huozhi))
[PreviousReact Labs: What We've Been Working On – June 2022](https://react.dev/blog/2022/06/15/react-labs-what-we-have-been-working-on-june-2022)
[NextHow to Upgrade to React 18](https://react.dev/blog/2022/03/08/react-18-upgrade-guide) |
https://react.dev/reference/react/createRef | [API Reference](https://react.dev/reference/react)
[Legacy React APIs](https://react.dev/reference/react/legacy)
# createRef[Link for this heading]()
### Pitfall
`createRef` is mostly used for [class components.](https://react.dev/reference/react/Component) Function components typically rely on [`useRef`](https://react.dev/reference/react/useRef) instead.
`createRef` creates a [ref](https://react.dev/learn/referencing-values-with-refs) object which can contain arbitrary value.
```
class MyInput extends Component {
inputRef = createRef();
// ...
}
```
- [Reference]()
- [`createRef()`]()
- [Usage]()
- [Declaring a ref in a class component]()
- [Alternatives]()
- [Migrating from a class with `createRef` to a function with `useRef`]()
* * *
## Reference[Link for Reference]()
### `createRef()`[Link for this heading]()
Call `createRef` to declare a [ref](https://react.dev/learn/referencing-values-with-refs) inside a [class component.](https://react.dev/reference/react/Component)
```
import { createRef, Component } from 'react';
class MyComponent extends Component {
intervalRef = createRef();
inputRef = createRef();
// ...
```
[See more examples below.]()
#### Parameters[Link for Parameters]()
`createRef` takes no parameters.
#### Returns[Link for Returns]()
`createRef` returns an object with a single property:
- `current`: Initially, it’s set to the `null`. You can later set it to something else. If you pass the ref object to React as a `ref` attribute to a JSX node, React will set its `current` property.
#### Caveats[Link for Caveats]()
- `createRef` always returns a *different* object. It’s equivalent to writing `{ current: null }` yourself.
- In a function component, you probably want [`useRef`](https://react.dev/reference/react/useRef) instead which always returns the same object.
- `const ref = useRef()` is equivalent to `const [ref, _] = useState(() => createRef(null))`.
* * *
## Usage[Link for Usage]()
### Declaring a ref in a class component[Link for Declaring a ref in a class component]()
To declare a ref inside a [class component,](https://react.dev/reference/react/Component) call `createRef` and assign its result to a class field:
```
import { Component, createRef } from 'react';
class Form extends Component {
inputRef = createRef();
// ...
}
```
If you now pass `ref={this.inputRef}` to an `<input>` in your JSX, React will populate `this.inputRef.current` with the input DOM node. For example, here is how you make a button that focuses the input:
App.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import { Component, createRef } from 'react';
export default class Form extends Component {
inputRef = createRef();
handleClick = () => {
this.inputRef.current.focus();
}
render() {
return (
<>
<input ref={this.inputRef} />
<button onClick={this.handleClick}>
Focus the input
</button>
</>
);
}
}
```
Show more
### Pitfall
`createRef` is mostly used for [class components.](https://react.dev/reference/react/Component) Function components typically rely on [`useRef`](https://react.dev/reference/react/useRef) instead.
* * *
## Alternatives[Link for Alternatives]()
### Migrating from a class with `createRef` to a function with `useRef`[Link for this heading]()
We recommend using function components instead of [class components](https://react.dev/reference/react/Component) in new code. If you have some existing class components using `createRef`, here is how you can convert them. This is the original code:
App.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import { Component, createRef } from 'react';
export default class Form extends Component {
inputRef = createRef();
handleClick = () => {
this.inputRef.current.focus();
}
render() {
return (
<>
<input ref={this.inputRef} />
<button onClick={this.handleClick}>
Focus the input
</button>
</>
);
}
}
```
Show more
When you [convert this component from a class to a function,](https://react.dev/reference/react/Component) replace calls to `createRef` with calls to [`useRef`:](https://react.dev/reference/react/useRef)
App.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import { useRef } from 'react';
export default function Form() {
const inputRef = useRef(null);
function handleClick() {
inputRef.current.focus();
}
return (
<>
<input ref={inputRef} />
<button onClick={handleClick}>
Focus the input
</button>
</>
);
}
```
Show more
[PreviouscreateElement](https://react.dev/reference/react/createElement)
[NextforwardRef](https://react.dev/reference/react/forwardRef) |
https://react.dev/reference/rules/rules-of-hooks | [API Reference](https://react.dev/reference/react)
[Overview](https://react.dev/reference/rules)
# Rules of Hooks[Link for this heading]()
Hooks are defined using JavaScript functions, but they represent a special type of reusable UI logic with restrictions on where they can be called.
- [Only call Hooks at the top level]()
- [Only call Hooks from React functions]()
* * *
## Only call Hooks at the top level[Link for Only call Hooks at the top level]()
Functions whose names start with `use` are called [*Hooks*](https://react.dev/reference/react) in React.
**Don’t call Hooks inside loops, conditions, nested functions, or `try`/`catch`/`finally` blocks.** Instead, always use Hooks at the top level of your React function, before any early returns. You can only call Hooks while React is rendering a function component:
- ✅ Call them at the top level in the body of a [function component](https://react.dev/learn/your-first-component).
- ✅ Call them at the top level in the body of a [custom Hook](https://react.dev/learn/reusing-logic-with-custom-hooks).
```
function Counter() {
// ✅ Good: top-level in a function component
const [count, setCount] = useState(0);
// ...
}
function useWindowWidth() {
// ✅ Good: top-level in a custom Hook
const [width, setWidth] = useState(window.innerWidth);
// ...
}
```
It’s **not** supported to call Hooks (functions starting with `use`) in any other cases, for example:
- 🔴 Do not call Hooks inside conditions or loops.
- 🔴 Do not call Hooks after a conditional `return` statement.
- 🔴 Do not call Hooks in event handlers.
- 🔴 Do not call Hooks in class components.
- 🔴 Do not call Hooks inside functions passed to `useMemo`, `useReducer`, or `useEffect`.
- 🔴 Do not call Hooks inside `try`/`catch`/`finally` blocks.
If you break these rules, you might see this error.
```
function Bad({ cond }) {
if (cond) {
// 🔴 Bad: inside a condition (to fix, move it outside!)
const theme = useContext(ThemeContext);
}
// ...
}
function Bad() {
for (let i = 0; i < 10; i++) {
// 🔴 Bad: inside a loop (to fix, move it outside!)
const theme = useContext(ThemeContext);
}
// ...
}
function Bad({ cond }) {
if (cond) {
return;
}
// 🔴 Bad: after a conditional return (to fix, move it before the return!)
const theme = useContext(ThemeContext);
// ...
}
function Bad() {
function handleClick() {
// 🔴 Bad: inside an event handler (to fix, move it outside!)
const theme = useContext(ThemeContext);
}
// ...
}
function Bad() {
const style = useMemo(() => {
// 🔴 Bad: inside useMemo (to fix, move it outside!)
const theme = useContext(ThemeContext);
return createStyle(theme);
});
// ...
}
class Bad extends React.Component {
render() {
// 🔴 Bad: inside a class component (to fix, write a function component instead of a class!)
useEffect(() => {})
// ...
}
}
function Bad() {
try {
// 🔴 Bad: inside try/catch/finally block (to fix, move it outside!)
const [x, setX] = useState(0);
} catch {
const [x, setX] = useState(1);
}
}
```
You can use the [`eslint-plugin-react-hooks` plugin](https://www.npmjs.com/package/eslint-plugin-react-hooks) to catch these mistakes.
### Note
[Custom Hooks](https://react.dev/learn/reusing-logic-with-custom-hooks) *may* call other Hooks (that’s their whole purpose). This works because custom Hooks are also supposed to only be called while a function component is rendering.
* * *
## Only call Hooks from React functions[Link for Only call Hooks from React functions]()
Don’t call Hooks from regular JavaScript functions. Instead, you can:
✅ Call Hooks from React function components. ✅ Call Hooks from [custom Hooks](https://react.dev/learn/reusing-logic-with-custom-hooks).
By following this rule, you ensure that all stateful logic in a component is clearly visible from its source code.
```
function FriendList() {
const [onlineStatus, setOnlineStatus] = useOnlineStatus(); // ✅
}
function setOnlineStatus() { // ❌ Not a component or custom Hook!
const [onlineStatus, setOnlineStatus] = useOnlineStatus();
}
```
[PreviousReact calls Components and Hooks](https://react.dev/reference/rules/react-calls-components-and-hooks) |
https://react.dev/reference/react/isValidElement | [API Reference](https://react.dev/reference/react)
[Legacy React APIs](https://react.dev/reference/react/legacy)
# isValidElement[Link for this heading]()
`isValidElement` checks whether a value is a React element.
```
const isElement = isValidElement(value)
```
- [Reference]()
- [`isValidElement(value)`]()
- [Usage]()
- [Checking if something is a React element]()
* * *
## Reference[Link for Reference]()
### `isValidElement(value)`[Link for this heading]()
Call `isValidElement(value)` to check whether `value` is a React element.
```
import { isValidElement, createElement } from 'react';
// ✅ React elements
console.log(isValidElement(<p />)); // true
console.log(isValidElement(createElement('p'))); // true
// ❌ Not React elements
console.log(isValidElement(25)); // false
console.log(isValidElement('Hello')); // false
console.log(isValidElement({ age: 42 })); // false
```
[See more examples below.]()
#### Parameters[Link for Parameters]()
- `value`: The `value` you want to check. It can be any a value of any type.
#### Returns[Link for Returns]()
`isValidElement` returns `true` if the `value` is a React element. Otherwise, it returns `false`.
#### Caveats[Link for Caveats]()
- **Only [JSX tags](https://react.dev/learn/writing-markup-with-jsx) and objects returned by [`createElement`](https://react.dev/reference/react/createElement) are considered to be React elements.** For example, even though a number like `42` is a valid React *node* (and can be returned from a component), it is not a valid React element. Arrays and portals created with [`createPortal`](https://react.dev/reference/react-dom/createPortal) are also *not* considered to be React elements.
* * *
## Usage[Link for Usage]()
### Checking if something is a React element[Link for Checking if something is a React element]()
Call `isValidElement` to check if some value is a *React element.*
React elements are:
- Values produced by writing a [JSX tag](https://react.dev/learn/writing-markup-with-jsx)
- Values produced by calling [`createElement`](https://react.dev/reference/react/createElement)
For React elements, `isValidElement` returns `true`:
```
import { isValidElement, createElement } from 'react';
// ✅ JSX tags are React elements
console.log(isValidElement(<p />)); // true
console.log(isValidElement(<MyComponent />)); // true
// ✅ Values returned by createElement are React elements
console.log(isValidElement(createElement('p'))); // true
console.log(isValidElement(createElement(MyComponent))); // true
```
Any other values, such as strings, numbers, or arbitrary objects and arrays, are not React elements.
For them, `isValidElement` returns `false`:
```
// ❌ These are *not* React elements
console.log(isValidElement(null)); // false
console.log(isValidElement(25)); // false
console.log(isValidElement('Hello')); // false
console.log(isValidElement({ age: 42 })); // false
console.log(isValidElement([<div />, <div />])); // false
console.log(isValidElement(MyComponent)); // false
```
It is very uncommon to need `isValidElement`. It’s mostly useful if you’re calling another API that *only* accepts elements (like [`cloneElement`](https://react.dev/reference/react/cloneElement) does) and you want to avoid an error when your argument is not a React element.
Unless you have some very specific reason to add an `isValidElement` check, you probably don’t need it.
##### Deep Dive
#### React elements vs React nodes[Link for React elements vs React nodes]()
Show Details
When you write a component, you can return any kind of *React node* from it:
```
function MyComponent() {
// ... you can return any React node ...
}
```
A React node can be:
- A React element created like `<div />` or `createElement('div')`
- A portal created with [`createPortal`](https://react.dev/reference/react-dom/createPortal)
- A string
- A number
- `true`, `false`, `null`, or `undefined` (which are not displayed)
- An array of other React nodes
**Note `isValidElement` checks whether the argument is a *React element,* not whether it’s a React node.** For example, `42` is not a valid React element. However, it is a perfectly valid React node:
```
function MyComponent() {
return 42; // It's ok to return a number from component
}
```
This is why you shouldn’t use `isValidElement` as a way to check whether something can be rendered.
[PreviousforwardRef](https://react.dev/reference/react/forwardRef)
[NextPureComponent](https://react.dev/reference/react/PureComponent) |
https://react.dev/reference/react/forwardRef | [API Reference](https://react.dev/reference/react)
[Legacy React APIs](https://react.dev/reference/react/legacy)
# forwardRef[Link for this heading]()
### Deprecated
In React 19, `forwardRef` is no longer necessary. Pass `ref` as a prop instead.
`forwardRef` will deprecated in a future release. Learn more [here](https://react.dev/blog/2024/04/25/react-19).
`forwardRef` lets your component expose a DOM node to parent component with a [ref.](https://react.dev/learn/manipulating-the-dom-with-refs)
```
const SomeComponent = forwardRef(render)
```
- [Reference]()
- [`forwardRef(render)`]()
- [`render` function]()
- [Usage]()
- [Exposing a DOM node to the parent component]()
- [Forwarding a ref through multiple components]()
- [Exposing an imperative handle instead of a DOM node]()
- [Troubleshooting]()
- [My component is wrapped in `forwardRef`, but the `ref` to it is always `null`]()
* * *
## Reference[Link for Reference]()
### `forwardRef(render)`[Link for this heading]()
Call `forwardRef()` to let your component receive a ref and forward it to a child component:
```
import { forwardRef } from 'react';
const MyInput = forwardRef(function MyInput(props, ref) {
// ...
});
```
[See more examples below.]()
#### Parameters[Link for Parameters]()
- `render`: The render function for your component. React calls this function with the props and `ref` that your component received from its parent. The JSX you return will be the output of your component.
#### Returns[Link for Returns]()
`forwardRef` returns a React component that you can render in JSX. Unlike React components defined as plain functions, a component returned by `forwardRef` is also able to receive a `ref` prop.
#### Caveats[Link for Caveats]()
- In Strict Mode, React will **call your render function twice** in order to [help you find accidental impurities.](https://react.dev/reference/react/useState) This is development-only behavior and does not affect production. If your render function is pure (as it should be), this should not affect the logic of your component. The result from one of the calls will be ignored.
* * *
### `render` function[Link for this heading]()
`forwardRef` accepts a render function as an argument. React calls this function with `props` and `ref`:
```
const MyInput = forwardRef(function MyInput(props, ref) {
return (
<label>
{props.label}
<input ref={ref} />
</label>
);
});
```
#### Parameters[Link for Parameters]()
- `props`: The props passed by the parent component.
- `ref`: The `ref` attribute passed by the parent component. The `ref` can be an object or a function. If the parent component has not passed a ref, it will be `null`. You should either pass the `ref` you receive to another component, or pass it to [`useImperativeHandle`.](https://react.dev/reference/react/useImperativeHandle)
#### Returns[Link for Returns]()
`forwardRef` returns a React component that you can render in JSX. Unlike React components defined as plain functions, the component returned by `forwardRef` is able to take a `ref` prop.
* * *
## Usage[Link for Usage]()
### Exposing a DOM node to the parent component[Link for Exposing a DOM node to the parent component]()
By default, each component’s DOM nodes are private. However, sometimes it’s useful to expose a DOM node to the parent—for example, to allow focusing it. To opt in, wrap your component definition into `forwardRef()`:
```
import { forwardRef } from 'react';
const MyInput = forwardRef(function MyInput(props, ref) {
const { label, ...otherProps } = props;
return (
<label>
{label}
<input {...otherProps} />
</label>
);
});
```
You will receive a ref as the second argument after props. Pass it to the DOM node that you want to expose:
```
import { forwardRef } from 'react';
const MyInput = forwardRef(function MyInput(props, ref) {
const { label, ...otherProps } = props;
return (
<label>
{label}
<input {...otherProps} ref={ref} />
</label>
);
});
```
This lets the parent `Form` component access the `<input>` DOM node exposed by `MyInput`:
```
function Form() {
const ref = useRef(null);
function handleClick() {
ref.current.focus();
}
return (
<form>
<MyInput label="Enter your name:" ref={ref} />
<button type="button" onClick={handleClick}>
Edit
</button>
</form>
);
}
```
This `Form` component [passes a ref](https://react.dev/reference/react/useRef) to `MyInput`. The `MyInput` component *forwards* that ref to the `<input>` browser tag. As a result, the `Form` component can access that `<input>` DOM node and call [`focus()`](https://developer.mozilla.org/en-US/docs/Web/API/HTMLElement/focus) on it.
Keep in mind that exposing a ref to the DOM node inside your component makes it harder to change your component’s internals later. You will typically expose DOM nodes from reusable low-level components like buttons or text inputs, but you won’t do it for application-level components like an avatar or a comment.
#### Examples of forwarding a ref[Link for Examples of forwarding a ref]()
1\. Focusing a text input 2. Playing and pausing a video
#### Example 1 of 2: Focusing a text input[Link for this heading]()
Clicking the button will focus the input. The `Form` component defines a ref and passes it to the `MyInput` component. The `MyInput` component forwards that ref to the browser `<input>`. This lets the `Form` component focus the `<input>`.
App.jsMyInput.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import { useRef } from 'react';
import MyInput from './MyInput.js';
export default function Form() {
const ref = useRef(null);
function handleClick() {
ref.current.focus();
}
return (
<form>
<MyInput label="Enter your name:" ref={ref} />
<button type="button" onClick={handleClick}>
Edit
</button>
</form>
);
}
```
Show more
Next Example
* * *
### Forwarding a ref through multiple components[Link for Forwarding a ref through multiple components]()
Instead of forwarding a `ref` to a DOM node, you can forward it to your own component like `MyInput`:
```
const FormField = forwardRef(function FormField(props, ref) {
// ...
return (
<>
<MyInput ref={ref} />
...
</>
);
});
```
If that `MyInput` component forwards a ref to its `<input>`, a ref to `FormField` will give you that `<input>`:
```
function Form() {
const ref = useRef(null);
function handleClick() {
ref.current.focus();
}
return (
<form>
<FormField label="Enter your name:" ref={ref} isRequired={true} />
<button type="button" onClick={handleClick}>
Edit
</button>
</form>
);
}
```
The `Form` component defines a ref and passes it to `FormField`. The `FormField` component forwards that ref to `MyInput`, which forwards it to a browser `<input>` DOM node. This is how `Form` accesses that DOM node.
App.jsFormField.jsMyInput.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import { useRef } from 'react';
import FormField from './FormField.js';
export default function Form() {
const ref = useRef(null);
function handleClick() {
ref.current.focus();
}
return (
<form>
<FormField label="Enter your name:" ref={ref} isRequired={true} />
<button type="button" onClick={handleClick}>
Edit
</button>
</form>
);
}
```
Show more
* * *
### Exposing an imperative handle instead of a DOM node[Link for Exposing an imperative handle instead of a DOM node]()
Instead of exposing an entire DOM node, you can expose a custom object, called an *imperative handle,* with a more constrained set of methods. To do this, you’d need to define a separate ref to hold the DOM node:
```
const MyInput = forwardRef(function MyInput(props, ref) {
const inputRef = useRef(null);
// ...
return <input {...props} ref={inputRef} />;
});
```
Pass the `ref` you received to [`useImperativeHandle`](https://react.dev/reference/react/useImperativeHandle) and specify the value you want to expose to the `ref`:
```
import { forwardRef, useRef, useImperativeHandle } from 'react';
const MyInput = forwardRef(function MyInput(props, ref) {
const inputRef = useRef(null);
useImperativeHandle(ref, () => {
return {
focus() {
inputRef.current.focus();
},
scrollIntoView() {
inputRef.current.scrollIntoView();
},
};
}, []);
return <input {...props} ref={inputRef} />;
});
```
If some component gets a ref to `MyInput`, it will only receive your `{ focus, scrollIntoView }` object instead of the DOM node. This lets you limit the information you expose about your DOM node to the minimum.
App.jsMyInput.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import { useRef } from 'react';
import MyInput from './MyInput.js';
export default function Form() {
const ref = useRef(null);
function handleClick() {
ref.current.focus();
// This won't work because the DOM node isn't exposed:
// ref.current.style.opacity = 0.5;
}
return (
<form>
<MyInput placeholder="Enter your name" ref={ref} />
<button type="button" onClick={handleClick}>
Edit
</button>
</form>
);
}
```
Show more
[Read more about using imperative handles.](https://react.dev/reference/react/useImperativeHandle)
### Pitfall
**Do not overuse refs.** You should only use refs for *imperative* behaviors that you can’t express as props: for example, scrolling to a node, focusing a node, triggering an animation, selecting text, and so on.
**If you can express something as a prop, you should not use a ref.** For example, instead of exposing an imperative handle like `{ open, close }` from a `Modal` component, it is better to take `isOpen` as a prop like `<Modal isOpen={isOpen} />`. [Effects](https://react.dev/learn/synchronizing-with-effects) can help you expose imperative behaviors via props.
* * *
## Troubleshooting[Link for Troubleshooting]()
### My component is wrapped in `forwardRef`, but the `ref` to it is always `null`[Link for this heading]()
This usually means that you forgot to actually use the `ref` that you received.
For example, this component doesn’t do anything with its `ref`:
```
const MyInput = forwardRef(function MyInput({ label }, ref) {
return (
<label>
{label}
<input />
</label>
);
});
```
To fix it, pass the `ref` down to a DOM node or another component that can accept a ref:
```
const MyInput = forwardRef(function MyInput({ label }, ref) {
return (
<label>
{label}
<input ref={ref} />
</label>
);
});
```
The `ref` to `MyInput` could also be `null` if some of the logic is conditional:
```
const MyInput = forwardRef(function MyInput({ label, showInput }, ref) {
return (
<label>
{label}
{showInput && <input ref={ref} />}
</label>
);
});
```
If `showInput` is `false`, then the ref won’t be forwarded to any node, and a ref to `MyInput` will remain empty. This is particularly easy to miss if the condition is hidden inside another component, like `Panel` in this example:
```
const MyInput = forwardRef(function MyInput({ label, showInput }, ref) {
return (
<label>
{label}
<Panel isExpanded={showInput}>
<input ref={ref} />
</Panel>
</label>
);
});
```
[PreviouscreateRef](https://react.dev/reference/react/createRef)
[NextisValidElement](https://react.dev/reference/react/isValidElement) |
https://react.dev/reference/react/createElement | [API Reference](https://react.dev/reference/react)
[Legacy React APIs](https://react.dev/reference/react/legacy)
# createElement[Link for this heading]()
`createElement` lets you create a React element. It serves as an alternative to writing [JSX.](https://react.dev/learn/writing-markup-with-jsx)
```
const element = createElement(type, props, ...children)
```
- [Reference]()
- [`createElement(type, props, ...children)`]()
- [Usage]()
- [Creating an element without JSX]()
* * *
## Reference[Link for Reference]()
### `createElement(type, props, ...children)`[Link for this heading]()
Call `createElement` to create a React element with the given `type`, `props`, and `children`.
```
import { createElement } from 'react';
function Greeting({ name }) {
return createElement(
'h1',
{ className: 'greeting' },
'Hello'
);
}
```
[See more examples below.]()
#### Parameters[Link for Parameters]()
- `type`: The `type` argument must be a valid React component type. For example, it could be a tag name string (such as `'div'` or `'span'`), or a React component (a function, a class, or a special component like [`Fragment`](https://react.dev/reference/react/Fragment)).
- `props`: The `props` argument must either be an object or `null`. If you pass `null`, it will be treated the same as an empty object. React will create an element with props matching the `props` you have passed. Note that `ref` and `key` from your `props` object are special and will *not* be available as `element.props.ref` and `element.props.key` on the returned `element`. They will be available as `element.ref` and `element.key`.
- **optional** `...children`: Zero or more child nodes. They can be any React nodes, including React elements, strings, numbers, [portals](https://react.dev/reference/react-dom/createPortal), empty nodes (`null`, `undefined`, `true`, and `false`), and arrays of React nodes.
#### Returns[Link for Returns]()
`createElement` returns a React element object with a few properties:
- `type`: The `type` you have passed.
- `props`: The `props` you have passed except for `ref` and `key`.
- `ref`: The `ref` you have passed. If missing, `null`.
- `key`: The `key` you have passed, coerced to a string. If missing, `null`.
Usually, you’ll return the element from your component or make it a child of another element. Although you may read the element’s properties, it’s best to treat every element as opaque after it’s created, and only render it.
#### Caveats[Link for Caveats]()
- You must **treat React elements and their props as [immutable](https://en.wikipedia.org/wiki/Immutable_object)** and never change their contents after creation. In development, React will [freeze](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Object/freeze) the returned element and its `props` property shallowly to enforce this.
- When you use JSX, **you must start a tag with a capital letter to render your own custom component.** In other words, `<Something />` is equivalent to `createElement(Something)`, but `<something />` (lowercase) is equivalent to `createElement('something')` (note it’s a string, so it will be treated as a built-in HTML tag).
- You should only **pass children as multiple arguments to `createElement` if they are all statically known,** like `createElement('h1', {}, child1, child2, child3)`. If your children are dynamic, pass the entire array as the third argument: `createElement('ul', {}, listItems)`. This ensures that React will [warn you about missing `key`s](https://react.dev/learn/rendering-lists) for any dynamic lists. For static lists this is not necessary because they never reorder.
* * *
## Usage[Link for Usage]()
### Creating an element without JSX[Link for Creating an element without JSX]()
If you don’t like [JSX](https://react.dev/learn/writing-markup-with-jsx) or can’t use it in your project, you can use `createElement` as an alternative.
To create an element without JSX, call `createElement` with some type, props, and children:
```
import { createElement } from 'react';
function Greeting({ name }) {
return createElement(
'h1',
{ className: 'greeting' },
'Hello ',
createElement('i', null, name),
'. Welcome!'
);
}
```
The children are optional, and you can pass as many as you need (the example above has three children). This code will display a `<h1>` header with a greeting. For comparison, here is the same example rewritten with JSX:
```
function Greeting({ name }) {
return (
<h1 className="greeting">
Hello <i>{name}</i>. Welcome!
</h1>
);
}
```
To render your own React component, pass a function like `Greeting` as the type instead of a string like `'h1'`:
```
export default function App() {
return createElement(Greeting, { name: 'Taylor' });
}
```
With JSX, it would look like this:
```
export default function App() {
return <Greeting name="Taylor" />;
}
```
Here is a complete example written with `createElement`:
App.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import { createElement } from 'react';
function Greeting({ name }) {
return createElement(
'h1',
{ className: 'greeting' },
'Hello ',
createElement('i', null, name),
'. Welcome!'
);
}
export default function App() {
return createElement(
Greeting,
{ name: 'Taylor' }
);
}
```
Show more
And here is the same example written using JSX:
App.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
function Greeting({ name }) {
return (
<h1 className="greeting">
Hello <i>{name}</i>. Welcome!
</h1>
);
}
export default function App() {
return <Greeting name="Taylor" />;
}
```
Both coding styles are fine, so you can use whichever one you prefer for your project. The main benefit of using JSX compared to `createElement` is that it’s easy to see which closing tag corresponds to which opening tag.
##### Deep Dive
#### What is a React element, exactly?[Link for What is a React element, exactly?]()
Show Details
An element is a lightweight description of a piece of the user interface. For example, both `<Greeting name="Taylor" />` and `createElement(Greeting, { name: 'Taylor' })` produce an object like this:
```
// Slightly simplified
{
type: Greeting,
props: {
name: 'Taylor'
},
key: null,
ref: null,
}
```
**Note that creating this object does not render the `Greeting` component or create any DOM elements.**
A React element is more like a description—an instruction for React to later render the `Greeting` component. By returning this object from your `App` component, you tell React what to do next.
Creating elements is extremely cheap so you don’t need to try to optimize or avoid it.
[PreviousComponent](https://react.dev/reference/react/Component)
[NextcreateRef](https://react.dev/reference/react/createRef) |
https://react.dev/reference/rsc/use-server | [API Reference](https://react.dev/reference/react)
[Directives](https://react.dev/reference/rsc/directives)
# 'use server'[Link for this heading]()
### React Server Components
`'use server'` is for use with [using React Server Components](https://react.dev/learn/start-a-new-react-project).
`'use server'` marks server-side functions that can be called from client-side code.
- [Reference]()
- [`'use server'`]()
- [Security considerations]()
- [Serializable arguments and return values]()
- [Usage]()
- [Server Functions in forms]()
- [Calling a Server Function outside of `<form>`]()
* * *
## Reference[Link for Reference]()
### `'use server'`[Link for this heading]()
Add `'use server'` at the top of an async function body to mark the function as callable by the client. We call these functions [*Server Functions*](https://react.dev/reference/rsc/server-functions).
```
async function addToCart(data) {
'use server';
// ...
}
```
When calling a Server Function on the client, it will make a network request to the server that includes a serialized copy of any arguments passed. If the Server Function returns a value, that value will be serialized and returned to the client.
Instead of individually marking functions with `'use server'`, you can add the directive to the top of a file to mark all exports within that file as Server Functions that can be used anywhere, including imported in client code.
#### Caveats[Link for Caveats]()
- `'use server'` must be at the very beginning of their function or module; above any other code including imports (comments above directives are OK). They must be written with single or double quotes, not backticks.
- `'use server'` can only be used in server-side files. The resulting Server Functions can be passed to Client Components through props. See supported [types for serialization]().
- To import a Server Functions from [client code](https://react.dev/reference/rsc/use-client), the directive must be used on a module level.
- Because the underlying network calls are always asynchronous, `'use server'` can only be used on async functions.
- Always treat arguments to Server Functions as untrusted input and authorize any mutations. See [security considerations]().
- Server Functions should be called in a [Transition](https://react.dev/reference/react/useTransition). Server Functions passed to [`<form action>`](https://react.dev/reference/react-dom/components/form) or [`formAction`](https://react.dev/reference/react-dom/components/input) will automatically be called in a transition.
- Server Functions are designed for mutations that update server-side state; they are not recommended for data fetching. Accordingly, frameworks implementing Server Functions typically process one action at a time and do not have a way to cache the return value.
### Security considerations[Link for Security considerations]()
Arguments to Server Functions are fully client-controlled. For security, always treat them as untrusted input, and make sure to validate and escape arguments as appropriate.
In any Server Function, make sure to validate that the logged-in user is allowed to perform that action.
### Under Construction
To prevent sending sensitive data from a Server Function, there are experimental taint APIs to prevent unique values and objects from being passed to client code.
See [experimental\_taintUniqueValue](https://react.dev/reference/react/experimental_taintUniqueValue) and [experimental\_taintObjectReference](https://react.dev/reference/react/experimental_taintObjectReference).
### Serializable arguments and return values[Link for Serializable arguments and return values]()
Since client code calls the Server Function over the network, any arguments passed will need to be serializable.
Here are supported types for Server Function arguments:
- Primitives
- [string](https://developer.mozilla.org/en-US/docs/Glossary/String)
- [number](https://developer.mozilla.org/en-US/docs/Glossary/Number)
- [bigint](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/BigInt)
- [boolean](https://developer.mozilla.org/en-US/docs/Glossary/Boolean)
- [undefined](https://developer.mozilla.org/en-US/docs/Glossary/Undefined)
- [null](https://developer.mozilla.org/en-US/docs/Glossary/Null)
- [symbol](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Symbol), only symbols registered in the global Symbol registry via [`Symbol.for`](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Symbol/for)
- Iterables containing serializable values
- [String](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/String)
- [Array](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Array)
- [Map](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Map)
- [Set](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Set)
- [TypedArray](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/TypedArray) and [ArrayBuffer](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/ArrayBuffer)
- [Date](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Date)
- [FormData](https://developer.mozilla.org/en-US/docs/Web/API/FormData) instances
- Plain [objects](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Object): those created with [object initializers](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Operators/Object_initializer), with serializable properties
- Functions that are Server Functions
- [Promises](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Promise)
Notably, these are not supported:
- React elements, or [JSX](https://react.dev/learn/writing-markup-with-jsx)
- Functions, including component functions or any other function that is not a Server Function
- [Classes](https://developer.mozilla.org/en-US/docs/Learn/JavaScript/Objects/Classes_in_JavaScript)
- Objects that are instances of any class (other than the built-ins mentioned) or objects with [a null prototype](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Object)
- Symbols not registered globally, ex. `Symbol('my new symbol')`
- Events from event handlers
Supported serializable return values are the same as [serializable props](https://react.dev/reference/rsc/use-client) for a boundary Client Component.
## Usage[Link for Usage]()
### Server Functions in forms[Link for Server Functions in forms]()
The most common use case of Server Functions will be calling functions that mutate data. On the browser, the [HTML form element](https://developer.mozilla.org/en-US/docs/Web/HTML/Element/form) is the traditional approach for a user to submit a mutation. With React Server Components, React introduces first-class support for Server Functions as Actions in [forms](https://react.dev/reference/react-dom/components/form).
Here is a form that allows a user to request a username.
```
// App.js
async function requestUsername(formData) {
'use server';
const username = formData.get('username');
// ...
}
export default function App() {
return (
<form action={requestUsername}>
<input type="text" name="username" />
<button type="submit">Request</button>
</form>
);
}
```
In this example `requestUsername` is a Server Function passed to a `<form>`. When a user submits this form, there is a network request to the server function `requestUsername`. When calling a Server Function in a form, React will supply the form’s [FormData](https://developer.mozilla.org/en-US/docs/Web/API/FormData) as the first argument to the Server Function.
By passing a Server Function to the form `action`, React can [progressively enhance](https://developer.mozilla.org/en-US/docs/Glossary/Progressive_Enhancement) the form. This means that forms can be submitted before the JavaScript bundle is loaded.
#### Handling return values in forms[Link for Handling return values in forms]()
In the username request form, there might be the chance that a username is not available. `requestUsername` should tell us if it fails or not.
To update the UI based on the result of a Server Function while supporting progressive enhancement, use [`useActionState`](https://react.dev/reference/react/useActionState).
```
// requestUsername.js
'use server';
export default async function requestUsername(formData) {
const username = formData.get('username');
if (canRequest(username)) {
// ...
return 'successful';
}
return 'failed';
}
```
```
// UsernameForm.js
'use client';
import { useActionState } from 'react';
import requestUsername from './requestUsername';
function UsernameForm() {
const [state, action] = useActionState(requestUsername, null, 'n/a');
return (
<>
<form action={action}>
<input type="text" name="username" />
<button type="submit">Request</button>
</form>
<p>Last submission request returned: {state}</p>
</>
);
}
```
Note that like most Hooks, `useActionState` can only be called in [client code](https://react.dev/reference/rsc/use-client).
### Calling a Server Function outside of `<form>`[Link for this heading]()
Server Functions are exposed server endpoints and can be called anywhere in client code.
When using a Server Function outside a [form](https://react.dev/reference/react-dom/components/form), call the Server Function in a [Transition](https://react.dev/reference/react/useTransition), which allows you to display a loading indicator, show [optimistic state updates](https://react.dev/reference/react/useOptimistic), and handle unexpected errors. Forms will automatically wrap Server Functions in transitions.
```
import incrementLike from './actions';
import { useState, useTransition } from 'react';
function LikeButton() {
const [isPending, startTransition] = useTransition();
const [likeCount, setLikeCount] = useState(0);
const onClick = () => {
startTransition(async () => {
const currentCount = await incrementLike();
setLikeCount(currentCount);
});
};
return (
<>
<p>Total Likes: {likeCount}</p>
<button onClick={onClick} disabled={isPending}>Like</button>;
</>
);
}
```
```
// actions.js
'use server';
let likeCount = 0;
export default async function incrementLike() {
likeCount++;
return likeCount;
}
```
To read a Server Function return value, you’ll need to `await` the promise returned.
[Previous'use client'](https://react.dev/reference/rsc/use-client) |
https://react.dev/reference/rsc/server-functions | [API Reference](https://react.dev/reference/react)
# Server Functions[Link for this heading]()
### React Server Components
Server Functions are for use in [React Server Components](https://react.dev/learn/start-a-new-react-project).
**Note:** Until September 2024, we referred to all Server Functions as “Server Actions”. If a Server Function is passed to an action prop or called from inside an action then it is a Server Action, but not all Server Functions are Server Actions. The naming in this documentation has been updated to reflect that Server Functions can be used for multiple purposes.
Server Functions allow Client Components to call async functions executed on the server.
### Note
#### How do I build support for Server Functions?[Link for How do I build support for Server Functions?]()
While Server Functions in React 19 are stable and will not break between minor versions, the underlying APIs used to implement Server Functions in a React Server Components bundler or framework do not follow semver and may break between minors in React 19.x.
To support Server Functions as a bundler or framework, we recommend pinning to a specific React version, or using the Canary release. We will continue working with bundlers and frameworks to stabilize the APIs used to implement Server Functions in the future.
When a Server Functions is defined with the [`"use server"`](https://react.dev/reference/rsc/use-server) directive, your framework will automatically create a reference to the server function, and pass that reference to the Client Component. When that function is called on the client, React will send a request to the server to execute the function, and return the result.
Server Functions can be created in Server Components and passed as props to Client Components, or they can be imported and used in Client Components.
## Usage[Link for Usage]()
### Creating a Server Function from a Server Component[Link for Creating a Server Function from a Server Component]()
Server Components can define Server Functions with the `"use server"` directive:
```
// Server Component
import Button from './Button';
function EmptyNote () {
async function createNoteAction() {
// Server Function
'use server';
await db.notes.create();
}
return <Button onClick={createNoteAction}/>;
}
```
When React renders the `EmptyNote` Server Function, it will create a reference to the `createNoteAction` function, and pass that reference to the `Button` Client Component. When the button is clicked, React will send a request to the server to execute the `createNoteAction` function with the reference provided:
```
"use client";
export default function Button({onClick}) {
console.log(onClick);
// {$$typeof: Symbol.for("react.server.reference"), $$id: 'createNoteAction'}
return <button onClick={() => onClick()}>Create Empty Note</button>
}
```
For more, see the docs for [`"use server"`](https://react.dev/reference/rsc/use-server).
### Importing Server Functions from Client Components[Link for Importing Server Functions from Client Components]()
Client Components can import Server Functions from files that use the `"use server"` directive:
```
"use server";
export async function createNote() {
await db.notes.create();
}
```
When the bundler builds the `EmptyNote` Client Component, it will create a reference to the `createNoteAction` function in the bundle. When the `button` is clicked, React will send a request to the server to execute the `createNoteAction` function using the reference provided:
```
"use client";
import {createNote} from './actions';
function EmptyNote() {
console.log(createNote);
// {$$typeof: Symbol.for("react.server.reference"), $$id: 'createNoteAction'}
<button onClick={() => createNote()} />
}
```
For more, see the docs for [`"use server"`](https://react.dev/reference/rsc/use-server).
### Server Functions with Actions[Link for Server Functions with Actions]()
Server Functions can be called from Actions on the client:
```
"use server";
export async function updateName(name) {
if (!name) {
return {error: 'Name is required'};
}
await db.users.updateName(name);
}
```
```
"use client";
import {updateName} from './actions';
function UpdateName() {
const [name, setName] = useState('');
const [error, setError] = useState(null);
const [isPending, startTransition] = useTransition();
const submitAction = async () => {
startTransition(async () => {
const {error} = await updateName(name);
if (!error) {
setError(error);
} else {
setName('');
}
})
}
return (
<form action={submitAction}>
<input type="text" name="name" disabled={isPending}/>
{state.error && <span>Failed: {state.error}</span>}
</form>
)
}
```
This allows you to access the `isPending` state of the Server Function by wrapping it in an Action on the client.
For more, see the docs for [Calling a Server Function outside of `<form>`](https://react.dev/reference/rsc/use-server)
### Server Functions with Form Actions[Link for Server Functions with Form Actions]()
Server Functions work with the new Form features in React 19.
You can pass a Server Function to a Form to automatically submit the form to the server:
```
"use client";
import {updateName} from './actions';
function UpdateName() {
return (
<form action={updateName}>
<input type="text" name="name" />
</form>
)
}
```
When the Form submission succeeds, React will automatically reset the form. You can add `useActionState` to access the pending state, last response, or to support progressive enhancement.
For more, see the docs for [Server Functions in Forms](https://react.dev/reference/rsc/use-server).
### Server Functions with `useActionState`[Link for this heading]()
You can call Server Functions with `useActionState` for the common case where you just need access to the action pending state and last returned response:
```
"use client";
import {updateName} from './actions';
function UpdateName() {
const [state, submitAction, isPending] = useActionState(updateName, {error: null});
return (
<form action={submitAction}>
<input type="text" name="name" disabled={isPending}/>
{state.error && <span>Failed: {state.error}</span>}
</form>
);
}
```
When using `useActionState` with Server Functions, React will also automatically replay form submissions entered before hydration finishes. This means users can interact with your app even before the app has hydrated.
For more, see the docs for [`useActionState`](https://react.dev/reference/react-dom/hooks/useFormState).
### Progressive enhancement with `useActionState`[Link for this heading]()
Server Functions also support progressive enhancement with the third argument of `useActionState`.
```
"use client";
import {updateName} from './actions';
function UpdateName() {
const [, submitAction] = useActionState(updateName, null, `/name/update`);
return (
<form action={submitAction}>
...
</form>
);
}
```
When the permalink is provided to `useActionState`, React will redirect to the provided URL if the form is submitted before the JavaScript bundle loads.
For more, see the docs for [`useActionState`](https://react.dev/reference/react-dom/hooks/useFormState).
[PreviousServer Components](https://react.dev/reference/rsc/server-components)
[NextDirectives](https://react.dev/reference/rsc/directives) |
https://react.dev/reference/react/cloneElement | [API Reference](https://react.dev/reference/react)
[Legacy React APIs](https://react.dev/reference/react/legacy)
# cloneElement[Link for this heading]()
### Pitfall
Using `cloneElement` is uncommon and can lead to fragile code. [See common alternatives.]()
`cloneElement` lets you create a new React element using another element as a starting point.
```
const clonedElement = cloneElement(element, props, ...children)
```
- [Reference]()
- [`cloneElement(element, props, ...children)`]()
- [Usage]()
- [Overriding props of an element]()
- [Alternatives]()
- [Passing data with a render prop]()
- [Passing data through context]()
- [Extracting logic into a custom Hook]()
* * *
## Reference[Link for Reference]()
### `cloneElement(element, props, ...children)`[Link for this heading]()
Call `cloneElement` to create a React element based on the `element`, but with different `props` and `children`:
```
import { cloneElement } from 'react';
// ...
const clonedElement = cloneElement(
<Row title="Cabbage">
Hello
</Row>,
{ isHighlighted: true },
'Goodbye'
);
console.log(clonedElement); // <Row title="Cabbage" isHighlighted={true}>Goodbye</Row>
```
[See more examples below.]()
#### Parameters[Link for Parameters]()
- `element`: The `element` argument must be a valid React element. For example, it could be a JSX node like `<Something />`, the result of calling [`createElement`](https://react.dev/reference/react/createElement), or the result of another `cloneElement` call.
- `props`: The `props` argument must either be an object or `null`. If you pass `null`, the cloned element will retain all of the original `element.props`. Otherwise, for every prop in the `props` object, the returned element will “prefer” the value from `props` over the value from `element.props`. The rest of the props will be filled from the original `element.props`. If you pass `props.key` or `props.ref`, they will replace the original ones.
- **optional** `...children`: Zero or more child nodes. They can be any React nodes, including React elements, strings, numbers, [portals](https://react.dev/reference/react-dom/createPortal), empty nodes (`null`, `undefined`, `true`, and `false`), and arrays of React nodes. If you don’t pass any `...children` arguments, the original `element.props.children` will be preserved.
#### Returns[Link for Returns]()
`cloneElement` returns a React element object with a few properties:
- `type`: Same as `element.type`.
- `props`: The result of shallowly merging `element.props` with the overriding `props` you have passed.
- `ref`: The original `element.ref`, unless it was overridden by `props.ref`.
- `key`: The original `element.key`, unless it was overridden by `props.key`.
Usually, you’ll return the element from your component or make it a child of another element. Although you may read the element’s properties, it’s best to treat every element as opaque after it’s created, and only render it.
#### Caveats[Link for Caveats]()
- Cloning an element **does not modify the original element.**
- You should only **pass children as multiple arguments to `cloneElement` if they are all statically known,** like `cloneElement(element, null, child1, child2, child3)`. If your children are dynamic, pass the entire array as the third argument: `cloneElement(element, null, listItems)`. This ensures that React will [warn you about missing `key`s](https://react.dev/learn/rendering-lists) for any dynamic lists. For static lists this is not necessary because they never reorder.
- `cloneElement` makes it harder to trace the data flow, so **try the [alternatives]() instead.**
* * *
## Usage[Link for Usage]()
### Overriding props of an element[Link for Overriding props of an element]()
To override the props of some React element, pass it to `cloneElement` with the props you want to override:
```
import { cloneElement } from 'react';
// ...
const clonedElement = cloneElement(
<Row title="Cabbage" />,
{ isHighlighted: true }
);
```
Here, the resulting cloned element will be `<Row title="Cabbage" isHighlighted={true} />`.
**Let’s walk through an example to see when it’s useful.**
Imagine a `List` component that renders its [`children`](https://react.dev/learn/passing-props-to-a-component) as a list of selectable rows with a “Next” button that changes which row is selected. The `List` component needs to render the selected `Row` differently, so it clones every `<Row>` child that it has received, and adds an extra `isHighlighted: true` or `isHighlighted: false` prop:
```
export default function List({ children }) {
const [selectedIndex, setSelectedIndex] = useState(0);
return (
<div className="List">
{Children.map(children, (child, index) =>
cloneElement(child, {
isHighlighted: index === selectedIndex
})
)}
```
Let’s say the original JSX received by `List` looks like this:
```
<List>
<Row title="Cabbage" />
<Row title="Garlic" />
<Row title="Apple" />
</List>
```
By cloning its children, the `List` can pass extra information to every `Row` inside. The result looks like this:
```
<List>
<Row
title="Cabbage"
isHighlighted={true}
/>
<Row
title="Garlic"
isHighlighted={false}
/>
<Row
title="Apple"
isHighlighted={false}
/>
</List>
```
Notice how pressing “Next” updates the state of the `List`, and highlights a different row:
App.jsList.jsRow.jsdata.js
List.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import { Children, cloneElement, useState } from 'react';
export default function List({ children }) {
const [selectedIndex, setSelectedIndex] = useState(0);
return (
<div className="List">
{Children.map(children, (child, index) =>
cloneElement(child, {
isHighlighted: index === selectedIndex
})
)}
<hr />
<button onClick={() => {
setSelectedIndex(i =>
(i + 1) % Children.count(children)
);
}}>
Next
</button>
</div>
);
}
```
Show more
To summarize, the `List` cloned the `<Row />` elements it received and added an extra prop to them.
### Pitfall
Cloning children makes it hard to tell how the data flows through your app. Try one of the [alternatives.]()
* * *
## Alternatives[Link for Alternatives]()
### Passing data with a render prop[Link for Passing data with a render prop]()
Instead of using `cloneElement`, consider accepting a *render prop* like `renderItem`. Here, `List` receives `renderItem` as a prop. `List` calls `renderItem` for every item and passes `isHighlighted` as an argument:
```
export default function List({ items, renderItem }) {
const [selectedIndex, setSelectedIndex] = useState(0);
return (
<div className="List">
{items.map((item, index) => {
const isHighlighted = index === selectedIndex;
return renderItem(item, isHighlighted);
})}
```
The `renderItem` prop is called a “render prop” because it’s a prop that specifies how to render something. For example, you can pass a `renderItem` implementation that renders a `<Row>` with the given `isHighlighted` value:
```
<List
items={products}
renderItem={(product, isHighlighted) =>
<Row
key={product.id}
title={product.title}
isHighlighted={isHighlighted}
/>
}
/>
```
The end result is the same as with `cloneElement`:
```
<List>
<Row
title="Cabbage"
isHighlighted={true}
/>
<Row
title="Garlic"
isHighlighted={false}
/>
<Row
title="Apple"
isHighlighted={false}
/>
</List>
```
However, you can clearly trace where the `isHighlighted` value is coming from.
App.jsList.jsRow.jsdata.js
List.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import { useState } from 'react';
export default function List({ items, renderItem }) {
const [selectedIndex, setSelectedIndex] = useState(0);
return (
<div className="List">
{items.map((item, index) => {
const isHighlighted = index === selectedIndex;
return renderItem(item, isHighlighted);
})}
<hr />
<button onClick={() => {
setSelectedIndex(i =>
(i + 1) % items.length
);
}}>
Next
</button>
</div>
);
}
```
Show more
This pattern is preferred to `cloneElement` because it is more explicit.
* * *
### Passing data through context[Link for Passing data through context]()
Another alternative to `cloneElement` is to [pass data through context.](https://react.dev/learn/passing-data-deeply-with-context)
For example, you can call [`createContext`](https://react.dev/reference/react/createContext) to define a `HighlightContext`:
```
export const HighlightContext = createContext(false);
```
Your `List` component can wrap every item it renders into a `HighlightContext` provider:
```
export default function List({ items, renderItem }) {
const [selectedIndex, setSelectedIndex] = useState(0);
return (
<div className="List">
{items.map((item, index) => {
const isHighlighted = index === selectedIndex;
return (
<HighlightContext.Provider key={item.id} value={isHighlighted}>
{renderItem(item)}
</HighlightContext.Provider>
);
})}
```
With this approach, `Row` does not need to receive an `isHighlighted` prop at all. Instead, it reads the context:
```
export default function Row({ title }) {
const isHighlighted = useContext(HighlightContext);
// ...
```
This allows the calling component to not know or worry about passing `isHighlighted` to `<Row>`:
```
<List
items={products}
renderItem={product =>
<Row title={product.title} />
}
/>
```
Instead, `List` and `Row` coordinate the highlighting logic through context.
App.jsList.jsRow.jsHighlightContext.jsdata.js
List.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import { useState } from 'react';
import { HighlightContext } from './HighlightContext.js';
export default function List({ items, renderItem }) {
const [selectedIndex, setSelectedIndex] = useState(0);
return (
<div className="List">
{items.map((item, index) => {
const isHighlighted = index === selectedIndex;
return (
<HighlightContext.Provider
key={item.id}
value={isHighlighted}
>
{renderItem(item)}
</HighlightContext.Provider>
);
})}
<hr />
<button onClick={() => {
setSelectedIndex(i =>
(i + 1) % items.length
);
}}>
Next
</button>
</div>
);
}
```
Show more
[Learn more about passing data through context.](https://react.dev/reference/react/useContext)
* * *
### Extracting logic into a custom Hook[Link for Extracting logic into a custom Hook]()
Another approach you can try is to extract the “non-visual” logic into your own Hook, and use the information returned by your Hook to decide what to render. For example, you could write a `useList` custom Hook like this:
```
import { useState } from 'react';
export default function useList(items) {
const [selectedIndex, setSelectedIndex] = useState(0);
function onNext() {
setSelectedIndex(i =>
(i + 1) % items.length
);
}
const selected = items[selectedIndex];
return [selected, onNext];
}
```
Then you could use it like this:
```
export default function App() {
const [selected, onNext] = useList(products);
return (
<div className="List">
{products.map(product =>
<Row
key={product.id}
title={product.title}
isHighlighted={selected === product}
/>
)}
<hr />
<button onClick={onNext}>
Next
</button>
</div>
);
}
```
The data flow is explicit, but the state is inside the `useList` custom Hook that you can use from any component:
App.jsuseList.jsRow.jsdata.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import Row from './Row.js';
import useList from './useList.js';
import { products } from './data.js';
export default function App() {
const [selected, onNext] = useList(products);
return (
<div className="List">
{products.map(product =>
<Row
key={product.id}
title={product.title}
isHighlighted={selected === product}
/>
)}
<hr />
<button onClick={onNext}>
Next
</button>
</div>
);
}
```
Show more
This approach is particularly useful if you want to reuse this logic between different components.
[PreviousChildren](https://react.dev/reference/react/Children)
[NextComponent](https://react.dev/reference/react/Component) |
https://react.dev/reference/react/Children | [API Reference](https://react.dev/reference/react)
[Legacy React APIs](https://react.dev/reference/react/legacy)
# Children[Link for this heading]()
### Pitfall
Using `Children` is uncommon and can lead to fragile code. [See common alternatives.]()
`Children` lets you manipulate and transform the JSX you received as the [`children` prop.](https://react.dev/learn/passing-props-to-a-component)
```
const mappedChildren = Children.map(children, child =>
<div className="Row">
{child}
</div>
);
```
- [Reference]()
- [`Children.count(children)`]()
- [`Children.forEach(children, fn, thisArg?)`]()
- [`Children.map(children, fn, thisArg?)`]()
- [`Children.only(children)`]()
- [`Children.toArray(children)`]()
- [Usage]()
- [Transforming children]()
- [Running some code for each child]()
- [Counting children]()
- [Converting children to an array]()
- [Alternatives]()
- [Exposing multiple components]()
- [Accepting an array of objects as a prop]()
- [Calling a render prop to customize rendering]()
- [Troubleshooting]()
- [I pass a custom component, but the `Children` methods don’t show its render result]()
* * *
## Reference[Link for Reference]()
### `Children.count(children)`[Link for this heading]()
Call `Children.count(children)` to count the number of children in the `children` data structure.
```
import { Children } from 'react';
function RowList({ children }) {
return (
<>
<h1>Total rows: {Children.count(children)}</h1>
...
</>
);
}
```
[See more examples below.]()
#### Parameters[Link for Parameters]()
- `children`: The value of the [`children` prop](https://react.dev/learn/passing-props-to-a-component) received by your component.
#### Returns[Link for Returns]()
The number of nodes inside these `children`.
#### Caveats[Link for Caveats]()
- Empty nodes (`null`, `undefined`, and Booleans), strings, numbers, and [React elements](https://react.dev/reference/react/createElement) count as individual nodes. Arrays don’t count as individual nodes, but their children do. **The traversal does not go deeper than React elements:** they don’t get rendered, and their children aren’t traversed. [Fragments](https://react.dev/reference/react/Fragment) don’t get traversed.
* * *
### `Children.forEach(children, fn, thisArg?)`[Link for this heading]()
Call `Children.forEach(children, fn, thisArg?)` to run some code for each child in the `children` data structure.
```
import { Children } from 'react';
function SeparatorList({ children }) {
const result = [];
Children.forEach(children, (child, index) => {
result.push(child);
result.push(<hr key={index} />);
});
// ...
```
[See more examples below.]()
#### Parameters[Link for Parameters]()
- `children`: The value of the [`children` prop](https://react.dev/learn/passing-props-to-a-component) received by your component.
- `fn`: The function you want to run for each child, similar to the [array `forEach` method](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Array/forEach) callback. It will be called with the child as the first argument and its index as the second argument. The index starts at `0` and increments on each call.
- **optional** `thisArg`: The [`this` value](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Operators/this) with which the `fn` function should be called. If omitted, it’s `undefined`.
#### Returns[Link for Returns]()
`Children.forEach` returns `undefined`.
#### Caveats[Link for Caveats]()
- Empty nodes (`null`, `undefined`, and Booleans), strings, numbers, and [React elements](https://react.dev/reference/react/createElement) count as individual nodes. Arrays don’t count as individual nodes, but their children do. **The traversal does not go deeper than React elements:** they don’t get rendered, and their children aren’t traversed. [Fragments](https://react.dev/reference/react/Fragment) don’t get traversed.
* * *
### `Children.map(children, fn, thisArg?)`[Link for this heading]()
Call `Children.map(children, fn, thisArg?)` to map or transform each child in the `children` data structure.
```
import { Children } from 'react';
function RowList({ children }) {
return (
<div className="RowList">
{Children.map(children, child =>
<div className="Row">
{child}
</div>
)}
</div>
);
}
```
[See more examples below.]()
#### Parameters[Link for Parameters]()
- `children`: The value of the [`children` prop](https://react.dev/learn/passing-props-to-a-component) received by your component.
- `fn`: The mapping function, similar to the [array `map` method](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Array/map) callback. It will be called with the child as the first argument and its index as the second argument. The index starts at `0` and increments on each call. You need to return a React node from this function. This may be an empty node (`null`, `undefined`, or a Boolean), a string, a number, a React element, or an array of other React nodes.
- **optional** `thisArg`: The [`this` value](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Operators/this) with which the `fn` function should be called. If omitted, it’s `undefined`.
#### Returns[Link for Returns]()
If `children` is `null` or `undefined`, returns the same value.
Otherwise, returns a flat array consisting of the nodes you’ve returned from the `fn` function. The returned array will contain all nodes you returned except for `null` and `undefined`.
#### Caveats[Link for Caveats]()
- Empty nodes (`null`, `undefined`, and Booleans), strings, numbers, and [React elements](https://react.dev/reference/react/createElement) count as individual nodes. Arrays don’t count as individual nodes, but their children do. **The traversal does not go deeper than React elements:** they don’t get rendered, and their children aren’t traversed. [Fragments](https://react.dev/reference/react/Fragment) don’t get traversed.
- If you return an element or an array of elements with keys from `fn`, **the returned elements’ keys will be automatically combined with the key of the corresponding original item from `children`.** When you return multiple elements from `fn` in an array, their keys only need to be unique locally amongst each other.
* * *
### `Children.only(children)`[Link for this heading]()
Call `Children.only(children)` to assert that `children` represent a single React element.
```
function Box({ children }) {
const element = Children.only(children);
// ...
```
#### Parameters[Link for Parameters]()
- `children`: The value of the [`children` prop](https://react.dev/learn/passing-props-to-a-component) received by your component.
#### Returns[Link for Returns]()
If `children` [is a valid element,](https://react.dev/reference/react/isValidElement) returns that element.
Otherwise, throws an error.
#### Caveats[Link for Caveats]()
- This method always **throws if you pass an array (such as the return value of `Children.map`) as `children`.** In other words, it enforces that `children` is a single React element, not that it’s an array with a single element.
* * *
### `Children.toArray(children)`[Link for this heading]()
Call `Children.toArray(children)` to create an array out of the `children` data structure.
```
import { Children } from 'react';
export default function ReversedList({ children }) {
const result = Children.toArray(children);
result.reverse();
// ...
```
#### Parameters[Link for Parameters]()
- `children`: The value of the [`children` prop](https://react.dev/learn/passing-props-to-a-component) received by your component.
#### Returns[Link for Returns]()
Returns a flat array of elements in `children`.
#### Caveats[Link for Caveats]()
- Empty nodes (`null`, `undefined`, and Booleans) will be omitted in the returned array. **The returned elements’ keys will be calculated from the original elements’ keys and their level of nesting and position.** This ensures that flattening the array does not introduce changes in behavior.
* * *
## Usage[Link for Usage]()
### Transforming children[Link for Transforming children]()
To transform the children JSX that your component [receives as the `children` prop,](https://react.dev/learn/passing-props-to-a-component) call `Children.map`:
```
import { Children } from 'react';
function RowList({ children }) {
return (
<div className="RowList">
{Children.map(children, child =>
<div className="Row">
{child}
</div>
)}
</div>
);
}
```
In the example above, the `RowList` wraps every child it receives into a `<div className="Row">` container. For example, let’s say the parent component passes three `<p>` tags as the `children` prop to `RowList`:
```
<RowList>
<p>This is the first item.</p>
<p>This is the second item.</p>
<p>This is the third item.</p>
</RowList>
```
Then, with the `RowList` implementation above, the final rendered result will look like this:
```
<div className="RowList">
<div className="Row">
<p>This is the first item.</p>
</div>
<div className="Row">
<p>This is the second item.</p>
</div>
<div className="Row">
<p>This is the third item.</p>
</div>
</div>
```
`Children.map` is similar to [to transforming arrays with `map()`.](https://react.dev/learn/rendering-lists) The difference is that the `children` data structure is considered *opaque.* This means that even if it’s sometimes an array, you should not assume it’s an array or any other particular data type. This is why you should use `Children.map` if you need to transform it.
App.jsRowList.js
RowList.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import { Children } from 'react';
export default function RowList({ children }) {
return (
<div className="RowList">
{Children.map(children, child =>
<div className="Row">
{child}
</div>
)}
</div>
);
}
```
##### Deep Dive
#### Why is the children prop not always an array?[Link for Why is the children prop not always an array?]()
Show Details
In React, the `children` prop is considered an *opaque* data structure. This means that you shouldn’t rely on how it is structured. To transform, filter, or count children, you should use the `Children` methods.
In practice, the `children` data structure is often represented as an array internally. However, if there is only a single child, then React won’t create an extra array since this would lead to unnecessary memory overhead. As long as you use the `Children` methods instead of directly introspecting the `children` prop, your code will not break even if React changes how the data structure is actually implemented.
Even when `children` is an array, `Children.map` has useful special behavior. For example, `Children.map` combines the [keys](https://react.dev/learn/rendering-lists) on the returned elements with the keys on the `children` you’ve passed to it. This ensures the original JSX children don’t “lose” keys even if they get wrapped like in the example above.
### Pitfall
The `children` data structure **does not include rendered output** of the components you pass as JSX. In the example below, the `children` received by the `RowList` only contains two items rather than three:
1. `<p>This is the first item.</p>`
2. `<MoreRows />`
This is why only two row wrappers are generated in this example:
App.jsRowList.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import RowList from './RowList.js';
export default function App() {
return (
<RowList>
<p>This is the first item.</p>
<MoreRows />
</RowList>
);
}
function MoreRows() {
return (
<>
<p>This is the second item.</p>
<p>This is the third item.</p>
</>
);
}
```
Show more
**There is no way to get the rendered output of an inner component** like `<MoreRows />` when manipulating `children`. This is why [it’s usually better to use one of the alternative solutions.]()
* * *
### Running some code for each child[Link for Running some code for each child]()
Call `Children.forEach` to iterate over each child in the `children` data structure. It does not return any value and is similar to the [array `forEach` method.](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Array/forEach) You can use it to run custom logic like constructing your own array.
App.jsSeparatorList.js
SeparatorList.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import { Children } from 'react';
export default function SeparatorList({ children }) {
const result = [];
Children.forEach(children, (child, index) => {
result.push(child);
result.push(<hr key={index} />);
});
result.pop(); // Remove the last separator
return result;
}
```
### Pitfall
As mentioned earlier, there is no way to get the rendered output of an inner component when manipulating `children`. This is why [it’s usually better to use one of the alternative solutions.]()
* * *
### Counting children[Link for Counting children]()
Call `Children.count(children)` to calculate the number of children.
App.jsRowList.js
RowList.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import { Children } from 'react';
export default function RowList({ children }) {
return (
<div className="RowList">
<h1 className="RowListHeader">
Total rows: {Children.count(children)}
</h1>
{Children.map(children, child =>
<div className="Row">
{child}
</div>
)}
</div>
);
}
```
Show more
### Pitfall
As mentioned earlier, there is no way to get the rendered output of an inner component when manipulating `children`. This is why [it’s usually better to use one of the alternative solutions.]()
* * *
### Converting children to an array[Link for Converting children to an array]()
Call `Children.toArray(children)` to turn the `children` data structure into a regular JavaScript array. This lets you manipulate the array with built-in array methods like [`filter`](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Array/filter), [`sort`](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Array/sort), or [`reverse`.](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Array/reverse)
App.jsReversedList.js
ReversedList.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import { Children } from 'react';
export default function ReversedList({ children }) {
const result = Children.toArray(children);
result.reverse();
return result;
}
```
### Pitfall
As mentioned earlier, there is no way to get the rendered output of an inner component when manipulating `children`. This is why [it’s usually better to use one of the alternative solutions.]()
* * *
## Alternatives[Link for Alternatives]()
### Note
This section describes alternatives to the `Children` API (with capital `C`) that’s imported like this:
```
import { Children } from 'react';
```
Don’t confuse it with [using the `children` prop](https://react.dev/learn/passing-props-to-a-component) (lowercase `c`), which is good and encouraged.
### Exposing multiple components[Link for Exposing multiple components]()
Manipulating children with the `Children` methods often leads to fragile code. When you pass children to a component in JSX, you don’t usually expect the component to manipulate or transform the individual children.
When you can, try to avoid using the `Children` methods. For example, if you want every child of `RowList` to be wrapped in `<div className="Row">`, export a `Row` component, and manually wrap every row into it like this:
App.jsRowList.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import { RowList, Row } from './RowList.js';
export default function App() {
return (
<RowList>
<Row>
<p>This is the first item.</p>
</Row>
<Row>
<p>This is the second item.</p>
</Row>
<Row>
<p>This is the third item.</p>
</Row>
</RowList>
);
}
```
Show more
Unlike using `Children.map`, this approach does not wrap every child automatically. **However, this approach has a significant benefit compared to the [earlier example with `Children.map`]() because it works even if you keep extracting more components.** For example, it still works if you extract your own `MoreRows` component:
App.jsRowList.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import { RowList, Row } from './RowList.js';
export default function App() {
return (
<RowList>
<Row>
<p>This is the first item.</p>
</Row>
<MoreRows />
</RowList>
);
}
function MoreRows() {
return (
<>
<Row>
<p>This is the second item.</p>
</Row>
<Row>
<p>This is the third item.</p>
</Row>
</>
);
}
```
Show more
This wouldn’t work with `Children.map` because it would “see” `<MoreRows />` as a single child (and a single row).
* * *
### Accepting an array of objects as a prop[Link for Accepting an array of objects as a prop]()
You can also explicitly pass an array as a prop. For example, this `RowList` accepts a `rows` array as a prop:
App.jsRowList.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import { RowList, Row } from './RowList.js';
export default function App() {
return (
<RowList rows={[
{ id: 'first', content: <p>This is the first item.</p> },
{ id: 'second', content: <p>This is the second item.</p> },
{ id: 'third', content: <p>This is the third item.</p> }
]} />
);
}
```
Since `rows` is a regular JavaScript array, the `RowList` component can use built-in array methods like [`map`](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Array/map) on it.
This pattern is especially useful when you want to be able to pass more information as structured data together with children. In the below example, the `TabSwitcher` component receives an array of objects as the `tabs` prop:
App.jsTabSwitcher.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import TabSwitcher from './TabSwitcher.js';
export default function App() {
return (
<TabSwitcher tabs={[
{
id: 'first',
header: 'First',
content: <p>This is the first item.</p>
},
{
id: 'second',
header: 'Second',
content: <p>This is the second item.</p>
},
{
id: 'third',
header: 'Third',
content: <p>This is the third item.</p>
}
]} />
);
}
```
Show more
Unlike passing the children as JSX, this approach lets you associate some extra data like `header` with each item. Because you are working with the `tabs` directly, and it is an array, you do not need the `Children` methods.
* * *
### Calling a render prop to customize rendering[Link for Calling a render prop to customize rendering]()
Instead of producing JSX for every single item, you can also pass a function that returns JSX, and call that function when necessary. In this example, the `App` component passes a `renderContent` function to the `TabSwitcher` component. The `TabSwitcher` component calls `renderContent` only for the selected tab:
App.jsTabSwitcher.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import TabSwitcher from './TabSwitcher.js';
export default function App() {
return (
<TabSwitcher
tabIds={['first', 'second', 'third']}
getHeader={tabId => {
return tabId[0].toUpperCase() + tabId.slice(1);
}}
renderContent={tabId => {
return <p>This is the {tabId} item.</p>;
}}
/>
);
}
```
A prop like `renderContent` is called a *render prop* because it is a prop that specifies how to render a piece of the user interface. However, there is nothing special about it: it is a regular prop which happens to be a function.
Render props are functions, so you can pass information to them. For example, this `RowList` component passes the `id` and the `index` of each row to the `renderRow` render prop, which uses `index` to highlight even rows:
App.jsRowList.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import { RowList, Row } from './RowList.js';
export default function App() {
return (
<RowList
rowIds={['first', 'second', 'third']}
renderRow={(id, index) => {
return (
<Row isHighlighted={index % 2 === 0}>
<p>This is the {id} item.</p>
</Row>
);
}}
/>
);
}
```
Show more
This is another example of how parent and child components can cooperate without manipulating the children.
* * *
## Troubleshooting[Link for Troubleshooting]()
### I pass a custom component, but the `Children` methods don’t show its render result[Link for this heading]()
Suppose you pass two children to `RowList` like this:
```
<RowList>
<p>First item</p>
<MoreRows />
</RowList>
```
If you do `Children.count(children)` inside `RowList`, you will get `2`. Even if `MoreRows` renders 10 different items, or if it returns `null`, `Children.count(children)` will still be `2`. From the `RowList`’s perspective, it only “sees” the JSX it has received. It does not “see” the internals of the `MoreRows` component.
The limitation makes it hard to extract a component. This is why [alternatives]() are preferred to using `Children`.
[PreviousLegacy React APIs](https://react.dev/reference/react/legacy)
[NextcloneElement](https://react.dev/reference/react/cloneElement) |
https://react.dev/reference/rules/react-calls-components-and-hooks | [API Reference](https://react.dev/reference/react)
[Overview](https://react.dev/reference/rules)
# React calls Components and Hooks[Link for this heading]()
React is responsible for rendering components and Hooks when necessary to optimize the user experience. It is declarative: you tell React what to render in your component’s logic, and React will figure out how best to display it to your user.
- [Never call component functions directly]()
- [Never pass around Hooks as regular values]()
- [Don’t dynamically mutate a Hook]()
- [Don’t dynamically use Hooks]()
* * *
## Never call component functions directly[Link for Never call component functions directly]()
Components should only be used in JSX. Don’t call them as regular functions. React should call it.
React must decide when your component function is called [during rendering](https://react.dev/reference/rules/components-and-hooks-must-be-pure). In React, you do this using JSX.
```
function BlogPost() {
return <Layout><Article /></Layout>; // ✅ Good: Only use components in JSX
}
```
```
function BlogPost() {
return <Layout>{Article()}</Layout>; // 🔴 Bad: Never call them directly
}
```
If a component contains Hooks, it’s easy to violate the [Rules of Hooks](https://react.dev/reference/rules/rules-of-hooks) when components are called directly in a loop or conditionally.
Letting React orchestrate rendering also allows a number of benefits:
- **Components become more than functions.** React can augment them with features like *local state* through Hooks that are tied to the component’s identity in the tree.
- **Component types participate in reconciliation.** By letting React call your components, you also tell it more about the conceptual structure of your tree. For example, when you move from rendering `<Feed>` to the `<Profile>` page, React won’t attempt to re-use them.
- **React can enhance your user experience.** For example, it can let the browser do some work between component calls so that re-rendering a large component tree doesn’t block the main thread.
- **A better debugging story.** If components are first-class citizens that the library is aware of, we can build rich developer tools for introspection in development.
- **More efficient reconciliation.** React can decide exactly which components in the tree need re-rendering and skip over the ones that don’t. That makes your app faster and more snappy.
* * *
## Never pass around Hooks as regular values[Link for Never pass around Hooks as regular values]()
Hooks should only be called inside of components or Hooks. Never pass it around as a regular value.
Hooks allow you to augment a component with React features. They should always be called as a function, and never passed around as a regular value. This enables *local reasoning*, or the ability for developers to understand everything a component can do by looking at that component in isolation.
Breaking this rule will cause React to not automatically optimize your component.
### Don’t dynamically mutate a Hook[Link for Don’t dynamically mutate a Hook]()
Hooks should be as “static” as possible. This means you shouldn’t dynamically mutate them. For example, this means you shouldn’t write higher order Hooks:
```
function ChatInput() {
const useDataWithLogging = withLogging(useData); // 🔴 Bad: don't write higher order Hooks
const data = useDataWithLogging();
}
```
Hooks should be immutable and not be mutated. Instead of mutating a Hook dynamically, create a static version of the Hook with the desired functionality.
```
function ChatInput() {
const data = useDataWithLogging(); // ✅ Good: Create a new version of the Hook
}
function useDataWithLogging() {
// ... Create a new version of the Hook and inline the logic here
}
```
### Don’t dynamically use Hooks[Link for Don’t dynamically use Hooks]()
Hooks should also not be dynamically used: for example, instead of doing dependency injection in a component by passing a Hook as a value:
```
function ChatInput() {
return <Button useData={useDataWithLogging} /> // 🔴 Bad: don't pass Hooks as props
}
```
You should always inline the call of the Hook into that component and handle any logic in there.
```
function ChatInput() {
return <Button />
}
function Button() {
const data = useDataWithLogging(); // ✅ Good: Use the Hook directly
}
function useDataWithLogging() {
// If there's any conditional logic to change the Hook's behavior, it should be inlined into
// the Hook
}
```
This way, `<Button />` is much easier to understand and debug. When Hooks are used in dynamic ways, it increases the complexity of your app greatly and inhibits local reasoning, making your team less productive in the long term. It also makes it easier to accidentally break the [Rules of Hooks](https://react.dev/reference/rules/rules-of-hooks) that Hooks should not be called conditionally. If you find yourself needing to mock components for tests, it’s better to mock the server instead to respond with canned data. If possible, it’s also usually more effective to test your app with end-to-end tests.
[PreviousComponents and Hooks must be pure](https://react.dev/reference/rules/components-and-hooks-must-be-pure)
[NextRules of Hooks](https://react.dev/reference/rules/rules-of-hooks) |
https://react.dev/blog/2020/12/21/data-fetching-with-react-server-components | [Blog](https://react.dev/blog)
# Introducing Zero-Bundle-Size React Server Components[Link for this heading]()
December 21, 2020 by [Dan Abramov](https://twitter.com/dan_abramov), [Lauren Tan](https://twitter.com/potetotes), [Joseph Savona](https://twitter.com/en_JS), and [Sebastian Markbåge](https://twitter.com/sebmarkbage)
* * *
2020 has been a long year. As it comes to an end we wanted to share a special Holiday Update on our research into zero-bundle-size **React Server Components**.
* * *
To introduce React Server Components, we have prepared a talk and a demo. If you want, you can check them out during the holidays, or later when work picks back up in the new year.
**React Server Components are still in research and development.** We are sharing this work in the spirit of transparency and to get initial feedback from the React community. There will be plenty of time for that, so **don’t feel like you have to catch up right now!**
If you want to check them out, we recommend going in the following order:
1. **Watch the talk** to learn about React Server Components and see the demo.
2. [**Clone the demo**](http://github.com/reactjs/server-components-demo) to play with React Server Components on your computer.
3. [**Read the RFC (with FAQ at the end)**](https://github.com/reactjs/rfcs/pull/188) for a deeper technical breakdown and to provide feedback.
We are excited to hear from you on the RFC or in replies to the [@reactjs](https://twitter.com/reactjs) Twitter handle. Happy holidays, stay safe, and see you next year!
[PreviousThe Plan for React 18](https://react.dev/blog/2021/06/08/the-plan-for-react-18)
[NextOlder posts](https://reactjs.org/blog/all.html) |
https://react.dev/reference/react/PureComponent | [API Reference](https://react.dev/reference/react)
[Legacy React APIs](https://react.dev/reference/react/legacy)
# PureComponent[Link for this heading]()
### Pitfall
We recommend defining components as functions instead of classes. [See how to migrate.]()
`PureComponent` is similar to [`Component`](https://react.dev/reference/react/Component) but it skips re-renders for same props and state. Class components are still supported by React, but we don’t recommend using them in new code.
```
class Greeting extends PureComponent {
render() {
return <h1>Hello, {this.props.name}!</h1>;
}
}
```
- [Reference]()
- [`PureComponent`]()
- [Usage]()
- [Skipping unnecessary re-renders for class components]()
- [Alternatives]()
- [Migrating from a `PureComponent` class component to a function]()
* * *
## Reference[Link for Reference]()
### `PureComponent`[Link for this heading]()
To skip re-rendering a class component for same props and state, extend `PureComponent` instead of [`Component`:](https://react.dev/reference/react/Component)
```
import { PureComponent } from 'react';
class Greeting extends PureComponent {
render() {
return <h1>Hello, {this.props.name}!</h1>;
}
}
```
`PureComponent` is a subclass of `Component` and supports [all the `Component` APIs.](https://react.dev/reference/react/Component) Extending `PureComponent` is equivalent to defining a custom [`shouldComponentUpdate`](https://react.dev/reference/react/Component) method that shallowly compares props and state.
[See more examples below.]()
* * *
## Usage[Link for Usage]()
### Skipping unnecessary re-renders for class components[Link for Skipping unnecessary re-renders for class components]()
React normally re-renders a component whenever its parent re-renders. As an optimization, you can create a component that React will not re-render when its parent re-renders so long as its new props and state are the same as the old props and state. [Class components](https://react.dev/reference/react/Component) can opt into this behavior by extending `PureComponent`:
```
class Greeting extends PureComponent {
render() {
return <h1>Hello, {this.props.name}!</h1>;
}
}
```
A React component should always have [pure rendering logic.](https://react.dev/learn/keeping-components-pure) This means that it must return the same output if its props, state, and context haven’t changed. By using `PureComponent`, you are telling React that your component complies with this requirement, so React doesn’t need to re-render as long as its props and state haven’t changed. However, your component will still re-render if a context that it’s using changes.
In this example, notice that the `Greeting` component re-renders whenever `name` is changed (because that’s one of its props), but not when `address` is changed (because it’s not passed to `Greeting` as a prop):
App.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import { PureComponent, useState } from 'react';
class Greeting extends PureComponent {
render() {
console.log("Greeting was rendered at", new Date().toLocaleTimeString());
return <h3>Hello{this.props.name && ', '}{this.props.name}!</h3>;
}
}
export default function MyApp() {
const [name, setName] = useState('');
const [address, setAddress] = useState('');
return (
<>
<label>
Name{': '}
<input value={name} onChange={e => setName(e.target.value)} />
</label>
<label>
Address{': '}
<input value={address} onChange={e => setAddress(e.target.value)} />
</label>
<Greeting name={name} />
</>
);
}
```
Show more
### Pitfall
We recommend defining components as functions instead of classes. [See how to migrate.]()
* * *
## Alternatives[Link for Alternatives]()
### Migrating from a `PureComponent` class component to a function[Link for this heading]()
We recommend using function components instead of [class components](https://react.dev/reference/react/Component) in new code. If you have some existing class components using `PureComponent`, here is how you can convert them. This is the original code:
App.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import { PureComponent, useState } from 'react';
class Greeting extends PureComponent {
render() {
console.log("Greeting was rendered at", new Date().toLocaleTimeString());
return <h3>Hello{this.props.name && ', '}{this.props.name}!</h3>;
}
}
export default function MyApp() {
const [name, setName] = useState('');
const [address, setAddress] = useState('');
return (
<>
<label>
Name{': '}
<input value={name} onChange={e => setName(e.target.value)} />
</label>
<label>
Address{': '}
<input value={address} onChange={e => setAddress(e.target.value)} />
</label>
<Greeting name={name} />
</>
);
}
```
Show more
When you [convert this component from a class to a function,](https://react.dev/reference/react/Component) wrap it in [`memo`:](https://react.dev/reference/react/memo)
App.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import { memo, useState } from 'react';
const Greeting = memo(function Greeting({ name }) {
console.log("Greeting was rendered at", new Date().toLocaleTimeString());
return <h3>Hello{name && ', '}{name}!</h3>;
});
export default function MyApp() {
const [name, setName] = useState('');
const [address, setAddress] = useState('');
return (
<>
<label>
Name{': '}
<input value={name} onChange={e => setName(e.target.value)} />
</label>
<label>
Address{': '}
<input value={address} onChange={e => setAddress(e.target.value)} />
</label>
<Greeting name={name} />
</>
);
}
```
Show more
### Note
Unlike `PureComponent`, [`memo`](https://react.dev/reference/react/memo) does not compare the new and the old state. In function components, calling the [`set` function](https://react.dev/reference/react/useState) with the same state [already prevents re-renders by default,](https://react.dev/reference/react/memo) even without `memo`.
[PreviousisValidElement](https://react.dev/reference/react/isValidElement) |
https://react.dev/learn/typescript | [Learn React](https://react.dev/learn)
[Installation](https://react.dev/learn/installation)
# Using TypeScript[Link for this heading]()
TypeScript is a popular way to add type definitions to JavaScript codebases. Out of the box, TypeScript [supports JSX](https://react.dev/learn/writing-markup-with-jsx) and you can get full React Web support by adding [`@types/react`](https://www.npmjs.com/package/@types/react) and [`@types/react-dom`](https://www.npmjs.com/package/@types/react-dom) to your project.
### You will learn
- [TypeScript with React Components](https://react.dev/learn/typescript)
- [Examples of typing with Hooks](https://react.dev/learn/typescript)
- [Common types from `@types/react`](https://react.dev/learn/typescript)
- [Further learning locations](https://react.dev/learn/typescript)
## Installation[Link for Installation]()
All [production-grade React frameworks](https://react.dev/learn/start-a-new-react-project) offer support for using TypeScript. Follow the framework specific guide for installation:
- [Next.js](https://nextjs.org/docs/app/building-your-application/configuring/typescript)
- [Remix](https://remix.run/docs/en/1.19.2/guides/typescript)
- [Gatsby](https://www.gatsbyjs.com/docs/how-to/custom-configuration/typescript/)
- [Expo](https://docs.expo.dev/guides/typescript/)
### Adding TypeScript to an existing React project[Link for Adding TypeScript to an existing React project]()
To install the latest version of React’s type definitions:
Terminal
Copy
npm install @types/react @types/react-dom
The following compiler options need to be set in your `tsconfig.json`:
1. `dom` must be included in [`lib`](https://www.typescriptlang.org/tsconfig/) (Note: If no `lib` option is specified, `dom` is included by default).
2. [`jsx`](https://www.typescriptlang.org/tsconfig/) must be set to one of the valid options. `preserve` should suffice for most applications. If you’re publishing a library, consult the [`jsx` documentation](https://www.typescriptlang.org/tsconfig/) on what value to choose.
## TypeScript with React Components[Link for TypeScript with React Components]()
### Note
Every file containing JSX must use the `.tsx` file extension. This is a TypeScript-specific extension that tells TypeScript that this file contains JSX.
Writing TypeScript with React is very similar to writing JavaScript with React. The key difference when working with a component is that you can provide types for your component’s props. These types can be used for correctness checking and providing inline documentation in editors.
Taking the [`MyButton` component](https://react.dev/learn) from the [Quick Start](https://react.dev/learn) guide, we can add a type describing the `title` for the button:
App.tsx
App.tsx
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")[TypeScript Playground](https://www.typescriptlang.org/play "Open in TypeScript Playground")
```
function MyButton({ title }: { title: string }) {
return (
<button>{title}</button>
);
}
export default function MyApp() {
return (
<div>
<h1>Welcome to my app</h1>
<MyButton title="I'm a button" />
</div>
);
}
```
### Note
These sandboxes can handle TypeScript code, but they do not run the type-checker. This means you can amend the TypeScript sandboxes to learn, but you won’t get any type errors or warnings. To get type-checking, you can use the [TypeScript Playground](https://www.typescriptlang.org/play) or use a more fully-featured online sandbox.
This inline syntax is the simplest way to provide types for a component, though once you start to have a few fields to describe it can become unwieldy. Instead, you can use an `interface` or `type` to describe the component’s props:
App.tsx
App.tsx
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")[TypeScript Playground](https://www.typescriptlang.org/play "Open in TypeScript Playground")
```
interface MyButtonProps {
/** The text to display inside the button */
title: string;
/** Whether the button can be interacted with */
disabled: boolean;
}
function MyButton({ title, disabled }: MyButtonProps) {
return (
<button disabled={disabled}>{title}</button>
);
}
export default function MyApp() {
return (
<div>
<h1>Welcome to my app</h1>
<MyButton title="I'm a disabled button" disabled={true}/>
</div>
);
}
```
Show more
The type describing your component’s props can be as simple or as complex as you need, though they should be an object type described with either a `type` or `interface`. You can learn about how TypeScript describes objects in [Object Types](https://www.typescriptlang.org/docs/handbook/2/objects.html) but you may also be interested in using [Union Types](https://www.typescriptlang.org/docs/handbook/2/everyday-types.html) to describe a prop that can be one of a few different types and the [Creating Types from Types](https://www.typescriptlang.org/docs/handbook/2/types-from-types.html) guide for more advanced use cases.
## Example Hooks[Link for Example Hooks]()
The type definitions from `@types/react` include types for the built-in Hooks, so you can use them in your components without any additional setup. They are built to take into account the code you write in your component, so you will get [inferred types](https://www.typescriptlang.org/docs/handbook/type-inference.html) a lot of the time and ideally do not need to handle the minutiae of providing the types.
However, we can look at a few examples of how to provide types for Hooks.
### `useState`[Link for this heading]()
The [`useState` Hook](https://react.dev/reference/react/useState) will re-use the value passed in as the initial state to determine what the type of the value should be. For example:
```
// Infer the type as "boolean"
const [enabled, setEnabled] = useState(false);
```
This will assign the type of `boolean` to `enabled`, and `setEnabled` will be a function accepting either a `boolean` argument, or a function that returns a `boolean`. If you want to explicitly provide a type for the state, you can do so by providing a type argument to the `useState` call:
```
// Explicitly set the type to "boolean"
const [enabled, setEnabled] = useState<boolean>(false);
```
This isn’t very useful in this case, but a common case where you may want to provide a type is when you have a union type. For example, `status` here can be one of a few different strings:
```
type Status = "idle" | "loading" | "success" | "error";
const [status, setStatus] = useState<Status>("idle");
```
Or, as recommended in [Principles for structuring state](https://react.dev/learn/choosing-the-state-structure), you can group related state as an object and describe the different possibilities via object types:
```
type RequestState =
| { status: 'idle' }
| { status: 'loading' }
| { status: 'success', data: any }
| { status: 'error', error: Error };
const [requestState, setRequestState] = useState<RequestState>({ status: 'idle' });
```
### `useReducer`[Link for this heading]()
The [`useReducer` Hook](https://react.dev/reference/react/useReducer) is a more complex Hook that takes a reducer function and an initial state. The types for the reducer function are inferred from the initial state. You can optionally provide a type argument to the `useReducer` call to provide a type for the state, but it is often better to set the type on the initial state instead:
App.tsx
App.tsx
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")[TypeScript Playground](https://www.typescriptlang.org/play "Open in TypeScript Playground")
```
import {useReducer} from 'react';
interface State {
count: number
};
type CounterAction =
| { type: "reset" }
| { type: "setCount"; value: State["count"] }
const initialState: State = { count: 0 };
function stateReducer(state: State, action: CounterAction): State {
switch (action.type) {
case "reset":
return initialState;
case "setCount":
return { ...state, count: action.value };
default:
throw new Error("Unknown action");
}
}
export default function App() {
const [state, dispatch] = useReducer(stateReducer, initialState);
const addFive = () => dispatch({ type: "setCount", value: state.count + 5 });
const reset = () => dispatch({ type: "reset" });
return (
<div>
<h1>Welcome to my counter</h1>
<p>Count: {state.count}</p>
<button onClick={addFive}>Add 5</button>
<button onClick={reset}>Reset</button>
</div>
);
}
```
Show more
We are using TypeScript in a few key places:
- `interface State` describes the shape of the reducer’s state.
- `type CounterAction` describes the different actions which can be dispatched to the reducer.
- `const initialState: State` provides a type for the initial state, and also the type which is used by `useReducer` by default.
- `stateReducer(state: State, action: CounterAction): State` sets the types for the reducer function’s arguments and return value.
A more explicit alternative to setting the type on `initialState` is to provide a type argument to `useReducer`:
```
import { stateReducer, State } from './your-reducer-implementation';
const initialState = { count: 0 };
export default function App() {
const [state, dispatch] = useReducer<State>(stateReducer, initialState);
}
```
### `useContext`[Link for this heading]()
The [`useContext` Hook](https://react.dev/reference/react/useContext) is a technique for passing data down the component tree without having to pass props through components. It is used by creating a provider component and often by creating a Hook to consume the value in a child component.
The type of the value provided by the context is inferred from the value passed to the `createContext` call:
App.tsx
App.tsx
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")[TypeScript Playground](https://www.typescriptlang.org/play "Open in TypeScript Playground")
```
import { createContext, useContext, useState } from 'react';
type Theme = "light" | "dark" | "system";
const ThemeContext = createContext<Theme>("system");
const useGetTheme = () => useContext(ThemeContext);
export default function MyApp() {
const [theme, setTheme] = useState<Theme>('light');
return (
<ThemeContext.Provider value={theme}>
<MyComponent />
</ThemeContext.Provider>
)
}
function MyComponent() {
const theme = useGetTheme();
return (
<div>
<p>Current theme: {theme}</p>
</div>
)
}
```
Show more
This technique works when you have a default value which makes sense - but there are occasionally cases when you do not, and in those cases `null` can feel reasonable as a default value. However, to allow the type-system to understand your code, you need to explicitly set `ContextShape | null` on the `createContext`.
This causes the issue that you need to eliminate the `| null` in the type for context consumers. Our recommendation is to have the Hook do a runtime check for it’s existence and throw an error when not present:
```
import { createContext, useContext, useState, useMemo } from 'react';
// This is a simpler example, but you can imagine a more complex object here
type ComplexObject = {
kind: string
};
// The context is created with `| null` in the type, to accurately reflect the default value.
const Context = createContext<ComplexObject | null>(null);
// The `| null` will be removed via the check in the Hook.
const useGetComplexObject = () => {
const object = useContext(Context);
if (!object) { throw new Error("useGetComplexObject must be used within a Provider") }
return object;
}
export default function MyApp() {
const object = useMemo(() => ({ kind: "complex" }), []);
return (
<Context.Provider value={object}>
<MyComponent />
</Context.Provider>
)
}
function MyComponent() {
const object = useGetComplexObject();
return (
<div>
<p>Current object: {object.kind}</p>
</div>
)
}
```
### `useMemo`[Link for this heading]()
The [`useMemo`](https://react.dev/reference/react/useMemo) Hooks will create/re-access a memorized value from a function call, re-running the function only when dependencies passed as the 2nd parameter are changed. The result of calling the Hook is inferred from the return value from the function in the first parameter. You can be more explicit by providing a type argument to the Hook.
```
// The type of visibleTodos is inferred from the return value of filterTodos
const visibleTodos = useMemo(() => filterTodos(todos, tab), [todos, tab]);
```
### `useCallback`[Link for this heading]()
The [`useCallback`](https://react.dev/reference/react/useCallback) provide a stable reference to a function as long as the dependencies passed into the second parameter are the same. Like `useMemo`, the function’s type is inferred from the return value of the function in the first parameter, and you can be more explicit by providing a type argument to the Hook.
```
const handleClick = useCallback(() => {
// ...
}, [todos]);
```
When working in TypeScript strict mode `useCallback` requires adding types for the parameters in your callback. This is because the type of the callback is inferred from the return value of the function, and without parameters the type cannot be fully understood.
Depending on your code-style preferences, you could use the `*EventHandler` functions from the React types to provide the type for the event handler at the same time as defining the callback:
```
import { useState, useCallback } from 'react';
export default function Form() {
const [value, setValue] = useState("Change me");
const handleChange = useCallback<React.ChangeEventHandler<HTMLInputElement>>((event) => {
setValue(event.currentTarget.value);
}, [setValue])
return (
<>
<input value={value} onChange={handleChange} />
<p>Value: {value}</p>
</>
);
}
```
## Useful Types[Link for Useful Types]()
There is quite an expansive set of types which come from the `@types/react` package, it is worth a read when you feel comfortable with how React and TypeScript interact. You can find them [in React’s folder in DefinitelyTyped](https://github.com/DefinitelyTyped/DefinitelyTyped/blob/master/types/react/index.d.ts). We will cover a few of the more common types here.
### DOM Events[Link for DOM Events]()
When working with DOM events in React, the type of the event can often be inferred from the event handler. However, when you want to extract a function to be passed to an event handler, you will need to explicitly set the type of the event.
App.tsx
App.tsx
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")[TypeScript Playground](https://www.typescriptlang.org/play "Open in TypeScript Playground")
```
import { useState } from 'react';
export default function Form() {
const [value, setValue] = useState("Change me");
function handleChange(event: React.ChangeEvent<HTMLInputElement>) {
setValue(event.currentTarget.value);
}
return (
<>
<input value={value} onChange={handleChange} />
<p>Value: {value}</p>
</>
);
}
```
Show more
There are many types of events provided in the React types - the full list can be found [here](https://github.com/DefinitelyTyped/DefinitelyTyped/blob/b580df54c0819ec9df62b0835a315dd48b8594a9/types/react/index.d.ts) which is based on the [most popular events from the DOM](https://developer.mozilla.org/en-US/docs/Web/Events).
When determining the type you are looking for you can first look at the hover information for the event handler you are using, which will show the type of the event.
If you need to use an event that is not included in this list, you can use the `React.SyntheticEvent` type, which is the base type for all events.
### Children[Link for Children]()
There are two common paths to describing the children of a component. The first is to use the `React.ReactNode` type, which is a union of all the possible types that can be passed as children in JSX:
```
interface ModalRendererProps {
title: string;
children: React.ReactNode;
}
```
This is a very broad definition of children. The second is to use the `React.ReactElement` type, which is only JSX elements and not JavaScript primitives like strings or numbers:
```
interface ModalRendererProps {
title: string;
children: React.ReactElement;
}
```
Note, that you cannot use TypeScript to describe that the children are a certain type of JSX elements, so you cannot use the type-system to describe a component which only accepts `<li>` children.
You can see an example of both `React.ReactNode` and `React.ReactElement` with the type-checker in [this TypeScript playground](https://www.typescriptlang.org/play?).
### Style Props[Link for Style Props]()
When using inline styles in React, you can use `React.CSSProperties` to describe the object passed to the `style` prop. This type is a union of all the possible CSS properties, and is a good way to ensure you are passing valid CSS properties to the `style` prop, and to get auto-complete in your editor.
```
interface MyComponentProps {
style: React.CSSProperties;
}
```
## Further learning[Link for Further learning]()
This guide has covered the basics of using TypeScript with React, but there is a lot more to learn. Individual API pages on the docs may contain more in-depth documentation on how to use them with TypeScript.
We recommend the following resources:
- [The TypeScript handbook](https://www.typescriptlang.org/docs/handbook/) is the official documentation for TypeScript, and covers most key language features.
- [The TypeScript release notes](https://devblogs.microsoft.com/typescript/) cover new features in depth.
- [React TypeScript Cheatsheet](https://react-typescript-cheatsheet.netlify.app/) is a community-maintained cheatsheet for using TypeScript with React, covering a lot of useful edge cases and providing more breadth than this document.
- [TypeScript Community Discord](https://discord.com/invite/typescript) is a great place to ask questions and get help with TypeScript and React issues.
[PreviousEditor Setup](https://react.dev/learn/editor-setup)
[NextReact Developer Tools](https://react.dev/learn/react-developer-tools) |
https://react.dev/learn/editor-setup | [Learn React](https://react.dev/learn)
[Installation](https://react.dev/learn/installation)
# Editor Setup[Link for this heading]()
A properly configured editor can make code clearer to read and faster to write. It can even help you catch bugs as you write them! If this is your first time setting up an editor or you’re looking to tune up your current editor, we have a few recommendations.
### You will learn
- What the most popular editors are
- How to format your code automatically
## Your editor[Link for Your editor]()
[VS Code](https://code.visualstudio.com/) is one of the most popular editors in use today. It has a large marketplace of extensions and integrates well with popular services like GitHub. Most of the features listed below can be added to VS Code as extensions as well, making it highly configurable!
Other popular text editors used in the React community include:
- [WebStorm](https://www.jetbrains.com/webstorm/) is an integrated development environment designed specifically for JavaScript.
- [Sublime Text](https://www.sublimetext.com/) has support for JSX and TypeScript, [syntax highlighting](https://stackoverflow.com/a/70960574/458193) and autocomplete built in.
- [Vim](https://www.vim.org/) is a highly configurable text editor built to make creating and changing any kind of text very efficient. It is included as “vi” with most UNIX systems and with Apple OS X.
## Recommended text editor features[Link for Recommended text editor features]()
Some editors come with these features built in, but others might require adding an extension. Check to see what support your editor of choice provides to be sure!
### Linting[Link for Linting]()
Code linters find problems in your code as you write, helping you fix them early. [ESLint](https://eslint.org/) is a popular, open source linter for JavaScript.
- [Install ESLint with the recommended configuration for React](https://www.npmjs.com/package/eslint-config-react-app) (be sure you have [Node installed!](https://nodejs.org/en/download/current/))
- [Integrate ESLint in VSCode with the official extension](https://marketplace.visualstudio.com/items?itemName=dbaeumer.vscode-eslint)
**Make sure that you’ve enabled all the [`eslint-plugin-react-hooks`](https://www.npmjs.com/package/eslint-plugin-react-hooks) rules for your project.** They are essential and catch the most severe bugs early. The recommended [`eslint-config-react-app`](https://www.npmjs.com/package/eslint-config-react-app) preset already includes them.
### Formatting[Link for Formatting]()
The last thing you want to do when sharing your code with another contributor is get into a discussion about [tabs vs spaces](https://www.google.com/search?q=tabs%20vs%20spaces)! Fortunately, [Prettier](https://prettier.io/) will clean up your code by reformatting it to conform to preset, configurable rules. Run Prettier, and all your tabs will be converted to spaces—and your indentation, quotes, etc will also all be changed to conform to the configuration. In the ideal setup, Prettier will run when you save your file, quickly making these edits for you.
You can install the [Prettier extension in VSCode](https://marketplace.visualstudio.com/items?itemName=esbenp.prettier-vscode) by following these steps:
1. Launch VS Code
2. Use Quick Open (press Ctrl/Cmd+P)
3. Paste in `ext install esbenp.prettier-vscode`
4. Press Enter
#### Formatting on save[Link for Formatting on save]()
Ideally, you should format your code on every save. VS Code has settings for this!
1. In VS Code, press `CTRL/CMD + SHIFT + P`.
2. Type “settings”
3. Hit Enter
4. In the search bar, type “format on save”
5. Be sure the “format on save” option is ticked!
> If your ESLint preset has formatting rules, they may conflict with Prettier. We recommend disabling all formatting rules in your ESLint preset using [`eslint-config-prettier`](https://github.com/prettier/eslint-config-prettier) so that ESLint is *only* used for catching logical mistakes. If you want to enforce that files are formatted before a pull request is merged, use [`prettier --check`](https://prettier.io/docs/en/cli.html) for your continuous integration.
[PreviousAdd React to an Existing Project](https://react.dev/learn/add-react-to-an-existing-project)
[NextUsing TypeScript](https://react.dev/learn/typescript) |
https://react.dev/learn/writing-markup-with-jsx | [Learn React](https://react.dev/learn)
[Describing the UI](https://react.dev/learn/describing-the-ui)
# Writing Markup with JSX[Link for this heading]()
*JSX* is a syntax extension for JavaScript that lets you write HTML-like markup inside a JavaScript file. Although there are other ways to write components, most React developers prefer the conciseness of JSX, and most codebases use it.
### You will learn
- Why React mixes markup with rendering logic
- How JSX is different from HTML
- How to display information with JSX
## JSX: Putting markup into JavaScript[Link for JSX: Putting markup into JavaScript]()
The Web has been built on HTML, CSS, and JavaScript. For many years, web developers kept content in HTML, design in CSS, and logic in JavaScript—often in separate files! Content was marked up inside HTML while the page’s logic lived separately in JavaScript:


HTML


JavaScript
But as the Web became more interactive, logic increasingly determined content. JavaScript was in charge of the HTML! This is why **in React, rendering logic and markup live together in the same place—components.**


`Sidebar.js` React component


`Form.js` React component
Keeping a button’s rendering logic and markup together ensures that they stay in sync with each other on every edit. Conversely, details that are unrelated, such as the button’s markup and a sidebar’s markup, are isolated from each other, making it safer to change either of them on their own.
Each React component is a JavaScript function that may contain some markup that React renders into the browser. React components use a syntax extension called JSX to represent that markup. JSX looks a lot like HTML, but it is a bit stricter and can display dynamic information. The best way to understand this is to convert some HTML markup to JSX markup.
### Note
JSX and React are two separate things. They’re often used together, but you *can* [use them independently](https://reactjs.org/blog/2020/09/22/introducing-the-new-jsx-transform.html) of each other. JSX is a syntax extension, while React is a JavaScript library.
## Converting HTML to JSX[Link for Converting HTML to JSX]()
Suppose that you have some (perfectly valid) HTML:
```
<h1>Hedy Lamarr's Todos</h1>
<img
src="https://i.imgur.com/yXOvdOSs.jpg"
alt="Hedy Lamarr"
class="photo"
>
<ul>
<li>Invent new traffic lights
<li>Rehearse a movie scene
<li>Improve the spectrum technology
</ul>
```
And you want to put it into your component:
```
export default function TodoList() {
return (
// ???
)
}
```
If you copy and paste it as is, it will not work:
App.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
export default function TodoList() {
return (
// This doesn't quite work!
<h1>Hedy Lamarr's Todos</h1>
<img
src="https://i.imgur.com/yXOvdOSs.jpg"
alt="Hedy Lamarr"
class="photo"
>
<ul>
<li>Invent new traffic lights
<li>Rehearse a movie scene
<li>Improve the spectrum technology
</ul>
```
Show more
This is because JSX is stricter and has a few more rules than HTML! If you read the error messages above, they’ll guide you to fix the markup, or you can follow the guide below.
### Note
Most of the time, React’s on-screen error messages will help you find where the problem is. Give them a read if you get stuck!
## The Rules of JSX[Link for The Rules of JSX]()
### 1. Return a single root element[Link for 1. Return a single root element]()
To return multiple elements from a component, **wrap them with a single parent tag.**
For example, you can use a `<div>`:
```
<div>
<h1>Hedy Lamarr's Todos</h1>
<img
src="https://i.imgur.com/yXOvdOSs.jpg"
alt="Hedy Lamarr"
class="photo"
>
<ul>
...
</ul>
</div>
```
If you don’t want to add an extra `<div>` to your markup, you can write `<>` and `</>` instead:
```
<>
<h1>Hedy Lamarr's Todos</h1>
<img
src="https://i.imgur.com/yXOvdOSs.jpg"
alt="Hedy Lamarr"
class="photo"
>
<ul>
...
</ul>
</>
```
This empty tag is called a [*Fragment.*](https://react.dev/reference/react/Fragment) Fragments let you group things without leaving any trace in the browser HTML tree.
##### Deep Dive
#### Why do multiple JSX tags need to be wrapped?[Link for Why do multiple JSX tags need to be wrapped?]()
Show Details
JSX looks like HTML, but under the hood it is transformed into plain JavaScript objects. You can’t return two objects from a function without wrapping them into an array. This explains why you also can’t return two JSX tags without wrapping them into another tag or a Fragment.
### 2. Close all the tags[Link for 2. Close all the tags]()
JSX requires tags to be explicitly closed: self-closing tags like `<img>` must become `<img />`, and wrapping tags like `<li>oranges` must be written as `<li>oranges</li>`.
This is how Hedy Lamarr’s image and list items look closed:
```
<>
<img
src="https://i.imgur.com/yXOvdOSs.jpg"
alt="Hedy Lamarr"
class="photo"
/>
<ul>
<li>Invent new traffic lights</li>
<li>Rehearse a movie scene</li>
<li>Improve the spectrum technology</li>
</ul>
</>
```
### 3. camelCase all most of the things\![Link for this heading]()
JSX turns into JavaScript and attributes written in JSX become keys of JavaScript objects. In your own components, you will often want to read those attributes into variables. But JavaScript has limitations on variable names. For example, their names can’t contain dashes or be reserved words like `class`.
This is why, in React, many HTML and SVG attributes are written in camelCase. For example, instead of `stroke-width` you use `strokeWidth`. Since `class` is a reserved word, in React you write `className` instead, named after the [corresponding DOM property](https://developer.mozilla.org/en-US/docs/Web/API/Element/className):
```
<img
src="https://i.imgur.com/yXOvdOSs.jpg"
alt="Hedy Lamarr"
className="photo"
/>
```
You can [find all these attributes in the list of DOM component props.](https://react.dev/reference/react-dom/components/common) If you get one wrong, don’t worry—React will print a message with a possible correction to the [browser console.](https://developer.mozilla.org/docs/Tools/Browser_Console)
### Pitfall
For historical reasons, [`aria-*`](https://developer.mozilla.org/docs/Web/Accessibility/ARIA) and [`data-*`](https://developer.mozilla.org/docs/Learn/HTML/Howto/Use_data_attributes) attributes are written as in HTML with dashes.
### Pro-tip: Use a JSX Converter[Link for Pro-tip: Use a JSX Converter]()
Converting all these attributes in existing markup can be tedious! We recommend using a [converter](https://transform.tools/html-to-jsx) to translate your existing HTML and SVG to JSX. Converters are very useful in practice, but it’s still worth understanding what is going on so that you can comfortably write JSX on your own.
Here is your final result:
App.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
export default function TodoList() {
return (
<>
<h1>Hedy Lamarr's Todos</h1>
<img
src="https://i.imgur.com/yXOvdOSs.jpg"
alt="Hedy Lamarr"
className="photo"
/>
<ul>
<li>Invent new traffic lights</li>
<li>Rehearse a movie scene</li>
<li>Improve the spectrum technology</li>
</ul>
</>
);
}
```
Show more
## Recap[Link for Recap]()
Now you know why JSX exists and how to use it in components:
- React components group rendering logic together with markup because they are related.
- JSX is similar to HTML, with a few differences. You can use a [converter](https://transform.tools/html-to-jsx) if you need to.
- Error messages will often point you in the right direction to fixing your markup.
## Try out some challenges[Link for Try out some challenges]()
#### Challenge 1 of 1: Convert some HTML to JSX[Link for this heading]()
This HTML was pasted into a component, but it’s not valid JSX. Fix it:
App.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
export default function Bio() {
return (
<div class="intro">
<h1>Welcome to my website!</h1>
</div>
<p class="summary">
You can find my thoughts here.
<br><br>
<b>And <i>pictures</b></i> of scientists!
</p>
);
}
```
Whether to do it by hand or using the converter is up to you!
Show solution
[PreviousImporting and Exporting Components](https://react.dev/learn/importing-and-exporting-components)
[NextJavaScript in JSX with Curly Braces](https://react.dev/learn/javascript-in-jsx-with-curly-braces) |
https://react.dev/learn/thinking-in-react | [Learn React](https://react.dev/learn)
[Quick Start](https://react.dev/learn)
# Thinking in React[Link for this heading]()
React can change how you think about the designs you look at and the apps you build. When you build a user interface with React, you will first break it apart into pieces called *components*. Then, you will describe the different visual states for each of your components. Finally, you will connect your components together so that the data flows through them. In this tutorial, we’ll guide you through the thought process of building a searchable product data table with React.
## Start with the mockup[Link for Start with the mockup]()
Imagine that you already have a JSON API and a mockup from a designer.
The JSON API returns some data that looks like this:
```
[
{ category: "Fruits", price: "$1", stocked: true, name: "Apple" },
{ category: "Fruits", price: "$1", stocked: true, name: "Dragonfruit" },
{ category: "Fruits", price: "$2", stocked: false, name: "Passionfruit" },
{ category: "Vegetables", price: "$2", stocked: true, name: "Spinach" },
{ category: "Vegetables", price: "$4", stocked: false, name: "Pumpkin" },
{ category: "Vegetables", price: "$1", stocked: true, name: "Peas" }
]
```
The mockup looks like this:

To implement a UI in React, you will usually follow the same five steps.
## Step 1: Break the UI into a component hierarchy[Link for Step 1: Break the UI into a component hierarchy]()
Start by drawing boxes around every component and subcomponent in the mockup and naming them. If you work with a designer, they may have already named these components in their design tool. Ask them!
Depending on your background, you can think about splitting up a design into components in different ways:
- **Programming**—use the same techniques for deciding if you should create a new function or object. One such technique is the [single responsibility principle](https://en.wikipedia.org/wiki/Single_responsibility_principle), that is, a component should ideally only do one thing. If it ends up growing, it should be decomposed into smaller subcomponents.
- **CSS**—consider what you would make class selectors for. (However, components are a bit less granular.)
- **Design**—consider how you would organize the design’s layers.
If your JSON is well-structured, you’ll often find that it naturally maps to the component structure of your UI. That’s because UI and data models often have the same information architecture—that is, the same shape. Separate your UI into components, where each component matches one piece of your data model.
There are five components on this screen:

1. `FilterableProductTable` (grey) contains the entire app.
2. `SearchBar` (blue) receives the user input.
3. `ProductTable` (lavender) displays and filters the list according to the user input.
4. `ProductCategoryRow` (green) displays a heading for each category.
5. `ProductRow` (yellow) displays a row for each product.
If you look at `ProductTable` (lavender), you’ll see that the table header (containing the “Name” and “Price” labels) isn’t its own component. This is a matter of preference, and you could go either way. For this example, it is a part of `ProductTable` because it appears inside the `ProductTable`’s list. However, if this header grows to be complex (e.g., if you add sorting), you can move it into its own `ProductTableHeader` component.
Now that you’ve identified the components in the mockup, arrange them into a hierarchy. Components that appear within another component in the mockup should appear as a child in the hierarchy:
- `FilterableProductTable`
- `SearchBar`
- `ProductTable`
- `ProductCategoryRow`
- `ProductRow`
## Step 2: Build a static version in React[Link for Step 2: Build a static version in React]()
Now that you have your component hierarchy, it’s time to implement your app. The most straightforward approach is to build a version that renders the UI from your data model without adding any interactivity… yet! It’s often easier to build the static version first and add interactivity later. Building a static version requires a lot of typing and no thinking, but adding interactivity requires a lot of thinking and not a lot of typing.
To build a static version of your app that renders your data model, you’ll want to build [components](https://react.dev/learn/your-first-component) that reuse other components and pass data using [props.](https://react.dev/learn/passing-props-to-a-component) Props are a way of passing data from parent to child. (If you’re familiar with the concept of [state](https://react.dev/learn/state-a-components-memory), don’t use state at all to build this static version. State is reserved only for interactivity, that is, data that changes over time. Since this is a static version of the app, you don’t need it.)
You can either build “top down” by starting with building the components higher up in the hierarchy (like `FilterableProductTable`) or “bottom up” by working from components lower down (like `ProductRow`). In simpler examples, it’s usually easier to go top-down, and on larger projects, it’s easier to go bottom-up.
App.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
function ProductCategoryRow({ category }) {
return (
<tr>
<th colSpan="2">
{category}
</th>
</tr>
);
}
function ProductRow({ product }) {
const name = product.stocked ? product.name :
<span style={{ color: 'red' }}>
{product.name}
</span>;
return (
<tr>
<td>{name}</td>
<td>{product.price}</td>
</tr>
);
}
function ProductTable({ products }) {
const rows = [];
let lastCategory = null;
products.forEach((product) => {
if (product.category !== lastCategory) {
rows.push(
<ProductCategoryRow
category={product.category}
key={product.category} />
);
}
rows.push(
<ProductRow
product={product}
key={product.name} />
);
lastCategory = product.category;
});
return (
<table>
<thead>
<tr>
<th>Name</th>
<th>Price</th>
</tr>
</thead>
<tbody>{rows}</tbody>
</table>
);
}
function SearchBar() {
return (
<form>
<input type="text" placeholder="Search..." />
<label>
<input type="checkbox" />
{' '}
Only show products in stock
</label>
</form>
);
}
function FilterableProductTable({ products }) {
return (
<div>
<SearchBar />
<ProductTable products={products} />
</div>
);
}
const PRODUCTS = [
{category: "Fruits", price: "$1", stocked: true, name: "Apple"},
{category: "Fruits", price: "$1", stocked: true, name: "Dragonfruit"},
{category: "Fruits", price: "$2", stocked: false, name: "Passionfruit"},
{category: "Vegetables", price: "$2", stocked: true, name: "Spinach"},
{category: "Vegetables", price: "$4", stocked: false, name: "Pumpkin"},
{category: "Vegetables", price: "$1", stocked: true, name: "Peas"}
];
export default function App() {
return <FilterableProductTable products={PRODUCTS} />;
}
```
Show more
(If this code looks intimidating, go through the [Quick Start](https://react.dev/learn) first!)
After building your components, you’ll have a library of reusable components that render your data model. Because this is a static app, the components will only return JSX. The component at the top of the hierarchy (`FilterableProductTable`) will take your data model as a prop. This is called *one-way data flow* because the data flows down from the top-level component to the ones at the bottom of the tree.
### Pitfall
At this point, you should not be using any state values. That’s for the next step!
## Step 3: Find the minimal but complete representation of UI state[Link for Step 3: Find the minimal but complete representation of UI state]()
To make the UI interactive, you need to let users change your underlying data model. You will use *state* for this.
Think of state as the minimal set of changing data that your app needs to remember. The most important principle for structuring state is to keep it [DRY (Don’t Repeat Yourself).](https://en.wikipedia.org/wiki/Don%27t_repeat_yourself) Figure out the absolute minimal representation of the state your application needs and compute everything else on-demand. For example, if you’re building a shopping list, you can store the items as an array in state. If you want to also display the number of items in the list, don’t store the number of items as another state value—instead, read the length of your array.
Now think of all of the pieces of data in this example application:
1. The original list of products
2. The search text the user has entered
3. The value of the checkbox
4. The filtered list of products
Which of these are state? Identify the ones that are not:
- Does it **remain unchanged** over time? If so, it isn’t state.
- Is it **passed in from a parent** via props? If so, it isn’t state.
- **Can you compute it** based on existing state or props in your component? If so, it *definitely* isn’t state!
What’s left is probably state.
Let’s go through them one by one again:
1. The original list of products is **passed in as props, so it’s not state.**
2. The search text seems to be state since it changes over time and can’t be computed from anything.
3. The value of the checkbox seems to be state since it changes over time and can’t be computed from anything.
4. The filtered list of products **isn’t state because it can be computed** by taking the original list of products and filtering it according to the search text and value of the checkbox.
This means only the search text and the value of the checkbox are state! Nicely done!
##### Deep Dive
#### Props vs State[Link for Props vs State]()
Show Details
There are two types of “model” data in React: props and state. The two are very different:
- [**Props** are like arguments you pass](https://react.dev/learn/passing-props-to-a-component) to a function. They let a parent component pass data to a child component and customize its appearance. For example, a `Form` can pass a `color` prop to a `Button`.
- [**State** is like a component’s memory.](https://react.dev/learn/state-a-components-memory) It lets a component keep track of some information and change it in response to interactions. For example, a `Button` might keep track of `isHovered` state.
Props and state are different, but they work together. A parent component will often keep some information in state (so that it can change it), and *pass it down* to child components as their props. It’s okay if the difference still feels fuzzy on the first read. It takes a bit of practice for it to really stick!
## Step 4: Identify where your state should live[Link for Step 4: Identify where your state should live]()
After identifying your app’s minimal state data, you need to identify which component is responsible for changing this state, or *owns* the state. Remember: React uses one-way data flow, passing data down the component hierarchy from parent to child component. It may not be immediately clear which component should own what state. This can be challenging if you’re new to this concept, but you can figure it out by following these steps!
For each piece of state in your application:
1. Identify *every* component that renders something based on that state.
2. Find their closest common parent component—a component above them all in the hierarchy.
3. Decide where the state should live:
1. Often, you can put the state directly into their common parent.
2. You can also put the state into some component above their common parent.
3. If you can’t find a component where it makes sense to own the state, create a new component solely for holding the state and add it somewhere in the hierarchy above the common parent component.
In the previous step, you found two pieces of state in this application: the search input text, and the value of the checkbox. In this example, they always appear together, so it makes sense to put them into the same place.
Now let’s run through our strategy for them:
1. **Identify components that use state:**
- `ProductTable` needs to filter the product list based on that state (search text and checkbox value).
- `SearchBar` needs to display that state (search text and checkbox value).
2. **Find their common parent:** The first parent component both components share is `FilterableProductTable`.
3. **Decide where the state lives**: We’ll keep the filter text and checked state values in `FilterableProductTable`.
So the state values will live in `FilterableProductTable`.
Add state to the component with the [`useState()` Hook.](https://react.dev/reference/react/useState) Hooks are special functions that let you “hook into” React. Add two state variables at the top of `FilterableProductTable` and specify their initial state:
```
function FilterableProductTable({ products }) {
const [filterText, setFilterText] = useState('');
const [inStockOnly, setInStockOnly] = useState(false);
```
Then, pass `filterText` and `inStockOnly` to `ProductTable` and `SearchBar` as props:
```
<div>
<SearchBar
filterText={filterText}
inStockOnly={inStockOnly} />
<ProductTable
products={products}
filterText={filterText}
inStockOnly={inStockOnly} />
</div>
```
You can start seeing how your application will behave. Edit the `filterText` initial value from `useState('')` to `useState('fruit')` in the sandbox code below. You’ll see both the search input text and the table update:
App.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import { useState } from 'react';
function FilterableProductTable({ products }) {
const [filterText, setFilterText] = useState('');
const [inStockOnly, setInStockOnly] = useState(false);
return (
<div>
<SearchBar
filterText={filterText}
inStockOnly={inStockOnly} />
<ProductTable
products={products}
filterText={filterText}
inStockOnly={inStockOnly} />
</div>
);
}
function ProductCategoryRow({ category }) {
return (
<tr>
<th colSpan="2">
{category}
</th>
</tr>
);
}
function ProductRow({ product }) {
const name = product.stocked ? product.name :
<span style={{ color: 'red' }}>
{product.name}
</span>;
return (
<tr>
<td>{name}</td>
<td>{product.price}</td>
</tr>
);
}
function ProductTable({ products, filterText, inStockOnly }) {
const rows = [];
let lastCategory = null;
products.forEach((product) => {
if (
product.name.toLowerCase().indexOf(
filterText.toLowerCase()
) === -1
) {
return;
}
if (inStockOnly && !product.stocked) {
return;
}
if (product.category !== lastCategory) {
rows.push(
<ProductCategoryRow
category={product.category}
key={product.category} />
);
}
rows.push(
<ProductRow
product={product}
key={product.name} />
);
lastCategory = product.category;
});
return (
<table>
<thead>
<tr>
<th>Name</th>
<th>Price</th>
</tr>
</thead>
<tbody>{rows}</tbody>
</table>
);
}
function SearchBar({ filterText, inStockOnly }) {
return (
<form>
<input
type="text"
value={filterText}
placeholder="Search..."/>
<label>
<input
type="checkbox"
checked={inStockOnly} />
{' '}
Only show products in stock
</label>
</form>
);
}
const PRODUCTS = [
{category: "Fruits", price: "$1", stocked: true, name: "Apple"},
{category: "Fruits", price: "$1", stocked: true, name: "Dragonfruit"},
{category: "Fruits", price: "$2", stocked: false, name: "Passionfruit"},
{category: "Vegetables", price: "$2", stocked: true, name: "Spinach"},
{category: "Vegetables", price: "$4", stocked: false, name: "Pumpkin"},
{category: "Vegetables", price: "$1", stocked: true, name: "Peas"}
];
export default function App() {
return <FilterableProductTable products={PRODUCTS} />;
}
```
Show more
Notice that editing the form doesn’t work yet. There is a console error in the sandbox above explaining why:
Console
You provided a \`value\` prop to a form field without an \`onChange\` handler. This will render a read-only field.
In the sandbox above, `ProductTable` and `SearchBar` read the `filterText` and `inStockOnly` props to render the table, the input, and the checkbox. For example, here is how `SearchBar` populates the input value:
```
function SearchBar({ filterText, inStockOnly }) {
return (
<form>
<input
type="text"
value={filterText}
placeholder="Search..."/>
```
However, you haven’t added any code to respond to the user actions like typing yet. This will be your final step.
## Step 5: Add inverse data flow[Link for Step 5: Add inverse data flow]()
Currently your app renders correctly with props and state flowing down the hierarchy. But to change the state according to user input, you will need to support data flowing the other way: the form components deep in the hierarchy need to update the state in `FilterableProductTable`.
React makes this data flow explicit, but it requires a little more typing than two-way data binding. If you try to type or check the box in the example above, you’ll see that React ignores your input. This is intentional. By writing `<input value={filterText} />`, you’ve set the `value` prop of the `input` to always be equal to the `filterText` state passed in from `FilterableProductTable`. Since `filterText` state is never set, the input never changes.
You want to make it so whenever the user changes the form inputs, the state updates to reflect those changes. The state is owned by `FilterableProductTable`, so only it can call `setFilterText` and `setInStockOnly`. To let `SearchBar` update the `FilterableProductTable`’s state, you need to pass these functions down to `SearchBar`:
```
function FilterableProductTable({ products }) {
const [filterText, setFilterText] = useState('');
const [inStockOnly, setInStockOnly] = useState(false);
return (
<div>
<SearchBar
filterText={filterText}
inStockOnly={inStockOnly}
onFilterTextChange={setFilterText}
onInStockOnlyChange={setInStockOnly} />
```
Inside the `SearchBar`, you will add the `onChange` event handlers and set the parent state from them:
```
function SearchBar({
filterText,
inStockOnly,
onFilterTextChange,
onInStockOnlyChange
}) {
return (
<form>
<input
type="text"
value={filterText}
placeholder="Search..."
onChange={(e) => onFilterTextChange(e.target.value)}
/>
<label>
<input
type="checkbox"
checked={inStockOnly}
onChange={(e) => onInStockOnlyChange(e.target.checked)}
```
Now the application fully works!
App.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import { useState } from 'react';
function FilterableProductTable({ products }) {
const [filterText, setFilterText] = useState('');
const [inStockOnly, setInStockOnly] = useState(false);
return (
<div>
<SearchBar
filterText={filterText}
inStockOnly={inStockOnly}
onFilterTextChange={setFilterText}
onInStockOnlyChange={setInStockOnly} />
<ProductTable
products={products}
filterText={filterText}
inStockOnly={inStockOnly} />
</div>
);
}
function ProductCategoryRow({ category }) {
return (
<tr>
<th colSpan="2">
{category}
</th>
</tr>
);
}
function ProductRow({ product }) {
const name = product.stocked ? product.name :
<span style={{ color: 'red' }}>
{product.name}
</span>;
return (
<tr>
<td>{name}</td>
<td>{product.price}</td>
</tr>
);
}
function ProductTable({ products, filterText, inStockOnly }) {
const rows = [];
let lastCategory = null;
products.forEach((product) => {
if (
product.name.toLowerCase().indexOf(
filterText.toLowerCase()
) === -1
) {
return;
}
if (inStockOnly && !product.stocked) {
return;
}
if (product.category !== lastCategory) {
rows.push(
<ProductCategoryRow
category={product.category}
key={product.category} />
);
}
rows.push(
<ProductRow
product={product}
key={product.name} />
);
lastCategory = product.category;
});
return (
<table>
<thead>
<tr>
<th>Name</th>
<th>Price</th>
</tr>
</thead>
<tbody>{rows}</tbody>
</table>
);
}
function SearchBar({
filterText,
inStockOnly,
onFilterTextChange,
onInStockOnlyChange
}) {
return (
<form>
<input
type="text"
value={filterText} placeholder="Search..."
onChange={(e) => onFilterTextChange(e.target.value)} />
<label>
<input
type="checkbox"
checked={inStockOnly}
onChange={(e) => onInStockOnlyChange(e.target.checked)} />
{' '}
Only show products in stock
</label>
</form>
);
}
const PRODUCTS = [
{category: "Fruits", price: "$1", stocked: true, name: "Apple"},
{category: "Fruits", price: "$1", stocked: true, name: "Dragonfruit"},
{category: "Fruits", price: "$2", stocked: false, name: "Passionfruit"},
{category: "Vegetables", price: "$2", stocked: true, name: "Spinach"},
{category: "Vegetables", price: "$4", stocked: false, name: "Pumpkin"},
{category: "Vegetables", price: "$1", stocked: true, name: "Peas"}
];
export default function App() {
return <FilterableProductTable products={PRODUCTS} />;
}
```
Show more
You can learn all about handling events and updating state in the [Adding Interactivity](https://react.dev/learn/adding-interactivity) section.
## Where to go from here[Link for Where to go from here]()
This was a very brief introduction to how to think about building components and applications with React. You can [start a React project](https://react.dev/learn/installation) right now or [dive deeper on all the syntax](https://react.dev/learn/describing-the-ui) used in this tutorial.
[PreviousTutorial: Tic-Tac-Toe](https://react.dev/learn/tutorial-tic-tac-toe)
[NextInstallation](https://react.dev/learn/installation) |
https://react.dev/reference/react/Component | [API Reference](https://react.dev/reference/react)
[Legacy React APIs](https://react.dev/reference/react/legacy)
# Component[Link for this heading]()
### Pitfall
We recommend defining components as functions instead of classes. [See how to migrate.]()
`Component` is the base class for the React components defined as [JavaScript classes.](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Classes) Class components are still supported by React, but we don’t recommend using them in new code.
```
class Greeting extends Component {
render() {
return <h1>Hello, {this.props.name}!</h1>;
}
}
```
- [Reference]()
- [`Component`]()
- [`context`]()
- [`props`]()
- [`state`]()
- [`constructor(props)`]()
- [`componentDidCatch(error, info)`]()
- [`componentDidMount()`]()
- [`componentDidUpdate(prevProps, prevState, snapshot?)`]()
- [`componentWillMount()`]()
- [`componentWillReceiveProps(nextProps)`]()
- [`componentWillUpdate(nextProps, nextState)`]()
- [`componentWillUnmount()`]()
- [`forceUpdate(callback?)`]()
- [`getSnapshotBeforeUpdate(prevProps, prevState)`]()
- [`render()`]()
- [`setState(nextState, callback?)`]()
- [`shouldComponentUpdate(nextProps, nextState, nextContext)`]()
- [`UNSAFE_componentWillMount()`]()
- [`UNSAFE_componentWillReceiveProps(nextProps, nextContext)`]()
- [`UNSAFE_componentWillUpdate(nextProps, nextState)`]()
- [`static contextType`]()
- [`static defaultProps`]()
- [`static getDerivedStateFromError(error)`]()
- [`static getDerivedStateFromProps(props, state)`]()
- [Usage]()
- [Defining a class component]()
- [Adding state to a class component]()
- [Adding lifecycle methods to a class component]()
- [Catching rendering errors with an error boundary]()
- [Alternatives]()
- [Migrating a simple component from a class to a function]()
- [Migrating a component with state from a class to a function]()
- [Migrating a component with lifecycle methods from a class to a function]()
- [Migrating a component with context from a class to a function]()
* * *
## Reference[Link for Reference]()
### `Component`[Link for this heading]()
To define a React component as a class, extend the built-in `Component` class and define a [`render` method:]()
```
import { Component } from 'react';
class Greeting extends Component {
render() {
return <h1>Hello, {this.props.name}!</h1>;
}
}
```
Only the `render` method is required, other methods are optional.
[See more examples below.]()
* * *
### `context`[Link for this heading]()
The [context](https://react.dev/learn/passing-data-deeply-with-context) of a class component is available as `this.context`. It is only available if you specify *which* context you want to receive using [`static contextType`]().
A class component can only read one context at a time.
```
class Button extends Component {
static contextType = ThemeContext;
render() {
const theme = this.context;
const className = 'button-' + theme;
return (
<button className={className}>
{this.props.children}
</button>
);
}
}
```
### Note
Reading `this.context` in class components is equivalent to [`useContext`](https://react.dev/reference/react/useContext) in function components.
[See how to migrate.]()
* * *
### `props`[Link for this heading]()
The props passed to a class component are available as `this.props`.
```
class Greeting extends Component {
render() {
return <h1>Hello, {this.props.name}!</h1>;
}
}
<Greeting name="Taylor" />
```
### Note
Reading `this.props` in class components is equivalent to [declaring props](https://react.dev/learn/passing-props-to-a-component) in function components.
[See how to migrate.]()
* * *
### `state`[Link for this heading]()
The state of a class component is available as `this.state`. The `state` field must be an object. Do not mutate the state directly. If you wish to change the state, call `setState` with the new state.
```
class Counter extends Component {
state = {
age: 42,
};
handleAgeChange = () => {
this.setState({
age: this.state.age + 1
});
};
render() {
return (
<>
<button onClick={this.handleAgeChange}>
Increment age
</button>
<p>You are {this.state.age}.</p>
</>
);
}
}
```
### Note
Defining `state` in class components is equivalent to calling [`useState`](https://react.dev/reference/react/useState) in function components.
[See how to migrate.]()
* * *
### `constructor(props)`[Link for this heading]()
The [constructor](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Classes/constructor) runs before your class component *mounts* (gets added to the screen). Typically, a constructor is only used for two purposes in React. It lets you declare state and [bind](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_objects/Function/bind) your class methods to the class instance:
```
class Counter extends Component {
constructor(props) {
super(props);
this.state = { counter: 0 };
this.handleClick = this.handleClick.bind(this);
}
handleClick() {
// ...
}
```
If you use modern JavaScript syntax, constructors are rarely needed. Instead, you can rewrite this code above using the [public class field syntax](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Classes/Public_class_fields) which is supported both by modern browsers and tools like [Babel:](https://babeljs.io/)
```
class Counter extends Component {
state = { counter: 0 };
handleClick = () => {
// ...
}
```
A constructor should not contain any side effects or subscriptions.
#### Parameters[Link for Parameters]()
- `props`: The component’s initial props.
#### Returns[Link for Returns]()
`constructor` should not return anything.
#### Caveats[Link for Caveats]()
- Do not run any side effects or subscriptions in the constructor. Instead, use [`componentDidMount`]() for that.
- Inside a constructor, you need to call `super(props)` before any other statement. If you don’t do that, `this.props` will be `undefined` while the constructor runs, which can be confusing and cause bugs.
- Constructor is the only place where you can assign [`this.state`]() directly. In all other methods, you need to use [`this.setState()`]() instead. Do not call `setState` in the constructor.
- When you use [server rendering,](https://react.dev/reference/react-dom/server) the constructor will run on the server too, followed by the [`render`]() method. However, lifecycle methods like `componentDidMount` or `componentWillUnmount` will not run on the server.
- When [Strict Mode](https://react.dev/reference/react/StrictMode) is on, React will call `constructor` twice in development and then throw away one of the instances. This helps you notice the accidental side effects that need to be moved out of the `constructor`.
### Note
There is no exact equivalent for `constructor` in function components. To declare state in a function component, call [`useState`.](https://react.dev/reference/react/useState) To avoid recalculating the initial state, [pass a function to `useState`.](https://react.dev/reference/react/useState)
* * *
### `componentDidCatch(error, info)`[Link for this heading]()
If you define `componentDidCatch`, React will call it when some child component (including distant children) throws an error during rendering. This lets you log that error to an error reporting service in production.
Typically, it is used together with [`static getDerivedStateFromError`]() which lets you update state in response to an error and display an error message to the user. A component with these methods is called an *error boundary.*
[See an example.]()
#### Parameters[Link for Parameters]()
- `error`: The error that was thrown. In practice, it will usually be an instance of [`Error`](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Error) but this is not guaranteed because JavaScript allows to [`throw`](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Statements/throw) any value, including strings or even `null`.
- `info`: An object containing additional information about the error. Its `componentStack` field contains a stack trace with the component that threw, as well as the names and source locations of all its parent components. In production, the component names will be minified. If you set up production error reporting, you can decode the component stack using sourcemaps the same way as you would do for regular JavaScript error stacks.
#### Returns[Link for Returns]()
`componentDidCatch` should not return anything.
#### Caveats[Link for Caveats]()
- In the past, it was common to call `setState` inside `componentDidCatch` in order to update the UI and display the fallback error message. This is deprecated in favor of defining [`static getDerivedStateFromError`.]()
- Production and development builds of React slightly differ in the way `componentDidCatch` handles errors. In development, the errors will bubble up to `window`, which means that any `window.onerror` or `window.addEventListener('error', callback)` will intercept the errors that have been caught by `componentDidCatch`. In production, instead, the errors will not bubble up, which means any ancestor error handler will only receive errors not explicitly caught by `componentDidCatch`.
### Note
There is no direct equivalent for `componentDidCatch` in function components yet. If you’d like to avoid creating class components, write a single `ErrorBoundary` component like above and use it throughout your app. Alternatively, you can use the [`react-error-boundary`](https://github.com/bvaughn/react-error-boundary) package which does that for you.
* * *
### `componentDidMount()`[Link for this heading]()
If you define the `componentDidMount` method, React will call it when your component is added *(mounted)* to the screen. This is a common place to start data fetching, set up subscriptions, or manipulate the DOM nodes.
If you implement `componentDidMount`, you usually need to implement other lifecycle methods to avoid bugs. For example, if `componentDidMount` reads some state or props, you also have to implement [`componentDidUpdate`]() to handle their changes, and [`componentWillUnmount`]() to clean up whatever `componentDidMount` was doing.
```
class ChatRoom extends Component {
state = {
serverUrl: 'https://localhost:1234'
};
componentDidMount() {
this.setupConnection();
}
componentDidUpdate(prevProps, prevState) {
if (
this.props.roomId !== prevProps.roomId ||
this.state.serverUrl !== prevState.serverUrl
) {
this.destroyConnection();
this.setupConnection();
}
}
componentWillUnmount() {
this.destroyConnection();
}
// ...
}
```
[See more examples.]()
#### Parameters[Link for Parameters]()
`componentDidMount` does not take any parameters.
#### Returns[Link for Returns]()
`componentDidMount` should not return anything.
#### Caveats[Link for Caveats]()
- When [Strict Mode](https://react.dev/reference/react/StrictMode) is on, in development React will call `componentDidMount`, then immediately call [`componentWillUnmount`,]() and then call `componentDidMount` again. This helps you notice if you forgot to implement `componentWillUnmount` or if its logic doesn’t fully “mirror” what `componentDidMount` does.
- Although you may call [`setState`]() immediately in `componentDidMount`, it’s best to avoid that when you can. It will trigger an extra rendering, but it will happen before the browser updates the screen. This guarantees that even though the [`render`]() will be called twice in this case, the user won’t see the intermediate state. Use this pattern with caution because it often causes performance issues. In most cases, you should be able to assign the initial state in the [`constructor`]() instead. It can, however, be necessary for cases like modals and tooltips when you need to measure a DOM node before rendering something that depends on its size or position.
### Note
For many use cases, defining `componentDidMount`, `componentDidUpdate`, and `componentWillUnmount` together in class components is equivalent to calling [`useEffect`](https://react.dev/reference/react/useEffect) in function components. In the rare cases where it’s important for the code to run before browser paint, [`useLayoutEffect`](https://react.dev/reference/react/useLayoutEffect) is a closer match.
[See how to migrate.]()
* * *
### `componentDidUpdate(prevProps, prevState, snapshot?)`[Link for this heading]()
If you define the `componentDidUpdate` method, React will call it immediately after your component has been re-rendered with updated props or state. This method is not called for the initial render.
You can use it to manipulate the DOM after an update. This is also a common place to do network requests as long as you compare the current props to previous props (e.g. a network request may not be necessary if the props have not changed). Typically, you’d use it together with [`componentDidMount`]() and [`componentWillUnmount`:]()
```
class ChatRoom extends Component {
state = {
serverUrl: 'https://localhost:1234'
};
componentDidMount() {
this.setupConnection();
}
componentDidUpdate(prevProps, prevState) {
if (
this.props.roomId !== prevProps.roomId ||
this.state.serverUrl !== prevState.serverUrl
) {
this.destroyConnection();
this.setupConnection();
}
}
componentWillUnmount() {
this.destroyConnection();
}
// ...
}
```
[See more examples.]()
#### Parameters[Link for Parameters]()
- `prevProps`: Props before the update. Compare `prevProps` to [`this.props`]() to determine what changed.
- `prevState`: State before the update. Compare `prevState` to [`this.state`]() to determine what changed.
- `snapshot`: If you implemented [`getSnapshotBeforeUpdate`](), `snapshot` will contain the value you returned from that method. Otherwise, it will be `undefined`.
#### Returns[Link for Returns]()
`componentDidUpdate` should not return anything.
#### Caveats[Link for Caveats]()
- `componentDidUpdate` will not get called if [`shouldComponentUpdate`]() is defined and returns `false`.
- The logic inside `componentDidUpdate` should usually be wrapped in conditions comparing `this.props` with `prevProps`, and `this.state` with `prevState`. Otherwise, there’s a risk of creating infinite loops.
- Although you may call [`setState`]() immediately in `componentDidUpdate`, it’s best to avoid that when you can. It will trigger an extra rendering, but it will happen before the browser updates the screen. This guarantees that even though the [`render`]() will be called twice in this case, the user won’t see the intermediate state. This pattern often causes performance issues, but it may be necessary for rare cases like modals and tooltips when you need to measure a DOM node before rendering something that depends on its size or position.
### Note
For many use cases, defining `componentDidMount`, `componentDidUpdate`, and `componentWillUnmount` together in class components is equivalent to calling [`useEffect`](https://react.dev/reference/react/useEffect) in function components. In the rare cases where it’s important for the code to run before browser paint, [`useLayoutEffect`](https://react.dev/reference/react/useLayoutEffect) is a closer match.
[See how to migrate.]()
* * *
### `componentWillMount()`[Link for this heading]()
### Deprecated
This API has been renamed from `componentWillMount` to [`UNSAFE_componentWillMount`.]() The old name has been deprecated. In a future major version of React, only the new name will work.
Run the [`rename-unsafe-lifecycles` codemod](https://github.com/reactjs/react-codemod) to automatically update your components.
* * *
### `componentWillReceiveProps(nextProps)`[Link for this heading]()
### Deprecated
This API has been renamed from `componentWillReceiveProps` to [`UNSAFE_componentWillReceiveProps`.]() The old name has been deprecated. In a future major version of React, only the new name will work.
Run the [`rename-unsafe-lifecycles` codemod](https://github.com/reactjs/react-codemod) to automatically update your components.
* * *
### `componentWillUpdate(nextProps, nextState)`[Link for this heading]()
### Deprecated
This API has been renamed from `componentWillUpdate` to [`UNSAFE_componentWillUpdate`.]() The old name has been deprecated. In a future major version of React, only the new name will work.
Run the [`rename-unsafe-lifecycles` codemod](https://github.com/reactjs/react-codemod) to automatically update your components.
* * *
### `componentWillUnmount()`[Link for this heading]()
If you define the `componentWillUnmount` method, React will call it before your component is removed *(unmounted)* from the screen. This is a common place to cancel data fetching or remove subscriptions.
The logic inside `componentWillUnmount` should “mirror” the logic inside [`componentDidMount`.]() For example, if `componentDidMount` sets up a subscription, `componentWillUnmount` should clean up that subscription. If the cleanup logic in your `componentWillUnmount` reads some props or state, you will usually also need to implement [`componentDidUpdate`]() to clean up resources (such as subscriptions) corresponding to the old props and state.
```
class ChatRoom extends Component {
state = {
serverUrl: 'https://localhost:1234'
};
componentDidMount() {
this.setupConnection();
}
componentDidUpdate(prevProps, prevState) {
if (
this.props.roomId !== prevProps.roomId ||
this.state.serverUrl !== prevState.serverUrl
) {
this.destroyConnection();
this.setupConnection();
}
}
componentWillUnmount() {
this.destroyConnection();
}
// ...
}
```
[See more examples.]()
#### Parameters[Link for Parameters]()
`componentWillUnmount` does not take any parameters.
#### Returns[Link for Returns]()
`componentWillUnmount` should not return anything.
#### Caveats[Link for Caveats]()
- When [Strict Mode](https://react.dev/reference/react/StrictMode) is on, in development React will call [`componentDidMount`,]() then immediately call `componentWillUnmount`, and then call `componentDidMount` again. This helps you notice if you forgot to implement `componentWillUnmount` or if its logic doesn’t fully “mirror” what `componentDidMount` does.
### Note
For many use cases, defining `componentDidMount`, `componentDidUpdate`, and `componentWillUnmount` together in class components is equivalent to calling [`useEffect`](https://react.dev/reference/react/useEffect) in function components. In the rare cases where it’s important for the code to run before browser paint, [`useLayoutEffect`](https://react.dev/reference/react/useLayoutEffect) is a closer match.
[See how to migrate.]()
* * *
### `forceUpdate(callback?)`[Link for this heading]()
Forces a component to re-render.
Usually, this is not necessary. If your component’s [`render`]() method only reads from [`this.props`](), [`this.state`](), or [`this.context`,]() it will re-render automatically when you call [`setState`]() inside your component or one of its parents. However, if your component’s `render` method reads directly from an external data source, you have to tell React to update the user interface when that data source changes. That’s what `forceUpdate` lets you do.
Try to avoid all uses of `forceUpdate` and only read from `this.props` and `this.state` in `render`.
#### Parameters[Link for Parameters]()
- **optional** `callback` If specified, React will call the `callback` you’ve provided after the update is committed.
#### Returns[Link for Returns]()
`forceUpdate` does not return anything.
#### Caveats[Link for Caveats]()
- If you call `forceUpdate`, React will re-render without calling [`shouldComponentUpdate`.]()
### Note
Reading an external data source and forcing class components to re-render in response to its changes with `forceUpdate` has been superseded by [`useSyncExternalStore`](https://react.dev/reference/react/useSyncExternalStore) in function components.
* * *
### `getSnapshotBeforeUpdate(prevProps, prevState)`[Link for this heading]()
If you implement `getSnapshotBeforeUpdate`, React will call it immediately before React updates the DOM. It enables your component to capture some information from the DOM (e.g. scroll position) before it is potentially changed. Any value returned by this lifecycle method will be passed as a parameter to [`componentDidUpdate`.]()
For example, you can use it in a UI like a chat thread that needs to preserve its scroll position during updates:
```
class ScrollingList extends React.Component {
constructor(props) {
super(props);
this.listRef = React.createRef();
}
getSnapshotBeforeUpdate(prevProps, prevState) {
// Are we adding new items to the list?
// Capture the scroll position so we can adjust scroll later.
if (prevProps.list.length < this.props.list.length) {
const list = this.listRef.current;
return list.scrollHeight - list.scrollTop;
}
return null;
}
componentDidUpdate(prevProps, prevState, snapshot) {
// If we have a snapshot value, we've just added new items.
// Adjust scroll so these new items don't push the old ones out of view.
// (snapshot here is the value returned from getSnapshotBeforeUpdate)
if (snapshot !== null) {
const list = this.listRef.current;
list.scrollTop = list.scrollHeight - snapshot;
}
}
render() {
return (
<div ref={this.listRef}>{/* ...contents... */}</div>
);
}
}
```
In the above example, it is important to read the `scrollHeight` property directly in `getSnapshotBeforeUpdate`. It is not safe to read it in [`render`](), [`UNSAFE_componentWillReceiveProps`](), or [`UNSAFE_componentWillUpdate`]() because there is a potential time gap between these methods getting called and React updating the DOM.
#### Parameters[Link for Parameters]()
- `prevProps`: Props before the update. Compare `prevProps` to [`this.props`]() to determine what changed.
- `prevState`: State before the update. Compare `prevState` to [`this.state`]() to determine what changed.
#### Returns[Link for Returns]()
You should return a snapshot value of any type that you’d like, or `null`. The value you returned will be passed as the third argument to [`componentDidUpdate`.]()
#### Caveats[Link for Caveats]()
- `getSnapshotBeforeUpdate` will not get called if [`shouldComponentUpdate`]() is defined and returns `false`.
### Note
At the moment, there is no equivalent to `getSnapshotBeforeUpdate` for function components. This use case is very uncommon, but if you have the need for it, for now you’ll have to write a class component.
* * *
### `render()`[Link for this heading]()
The `render` method is the only required method in a class component.
The `render` method should specify what you want to appear on the screen, for example:
```
import { Component } from 'react';
class Greeting extends Component {
render() {
return <h1>Hello, {this.props.name}!</h1>;
}
}
```
React may call `render` at any moment, so you shouldn’t assume that it runs at a particular time. Usually, the `render` method should return a piece of [JSX](https://react.dev/learn/writing-markup-with-jsx), but a few [other return types]() (like strings) are supported. To calculate the returned JSX, the `render` method can read [`this.props`](), [`this.state`](), and [`this.context`]().
You should write the `render` method as a pure function, meaning that it should return the same result if props, state, and context are the same. It also shouldn’t contain side effects (like setting up subscriptions) or interact with the browser APIs. Side effects should happen either in event handlers or methods like [`componentDidMount`.]()
#### Parameters[Link for Parameters]()
`render` does not take any parameters.
#### Returns[Link for Returns]()
`render` can return any valid React node. This includes React elements such as `<div />`, strings, numbers, [portals](https://react.dev/reference/react-dom/createPortal), empty nodes (`null`, `undefined`, `true`, and `false`), and arrays of React nodes.
#### Caveats[Link for Caveats]()
- `render` should be written as a pure function of props, state, and context. It should not have side effects.
- `render` will not get called if [`shouldComponentUpdate`]() is defined and returns `false`.
- When [Strict Mode](https://react.dev/reference/react/StrictMode) is on, React will call `render` twice in development and then throw away one of the results. This helps you notice the accidental side effects that need to be moved out of the `render` method.
- There is no one-to-one correspondence between the `render` call and the subsequent `componentDidMount` or `componentDidUpdate` call. Some of the `render` call results may be discarded by React when it’s beneficial.
* * *
### `setState(nextState, callback?)`[Link for this heading]()
Call `setState` to update the state of your React component.
```
class Form extends Component {
state = {
name: 'Taylor',
};
handleNameChange = (e) => {
const newName = e.target.value;
this.setState({
name: newName
});
}
render() {
return (
<>
<input value={this.state.name} onChange={this.handleNameChange} />
<p>Hello, {this.state.name}.</p>
</>
);
}
}
```
`setState` enqueues changes to the component state. It tells React that this component and its children need to re-render with the new state. This is the main way you’ll update the user interface in response to interactions.
### Pitfall
Calling `setState` **does not** change the current state in the already executing code:
```
function handleClick() {
console.log(this.state.name); // "Taylor"
this.setState({
name: 'Robin'
});
console.log(this.state.name); // Still "Taylor"!
}
```
It only affects what `this.state` will return starting from the *next* render.
You can also pass a function to `setState`. It lets you update state based on the previous state:
```
handleIncreaseAge = () => {
this.setState(prevState => {
return {
age: prevState.age + 1
};
});
}
```
You don’t have to do this, but it’s handy if you want to update state multiple times during the same event.
#### Parameters[Link for Parameters]()
- `nextState`: Either an object or a function.
- If you pass an object as `nextState`, it will be shallowly merged into `this.state`.
- If you pass a function as `nextState`, it will be treated as an *updater function*. It must be pure, should take the pending state and props as arguments, and should return the object to be shallowly merged into `this.state`. React will put your updater function in a queue and re-render your component. During the next render, React will calculate the next state by applying all of the queued updaters to the previous state.
- **optional** `callback`: If specified, React will call the `callback` you’ve provided after the update is committed.
#### Returns[Link for Returns]()
`setState` does not return anything.
#### Caveats[Link for Caveats]()
- Think of `setState` as a *request* rather than an immediate command to update the component. When multiple components update their state in response to an event, React will batch their updates and re-render them together in a single pass at the end of the event. In the rare case that you need to force a particular state update to be applied synchronously, you may wrap it in [`flushSync`,](https://react.dev/reference/react-dom/flushSync) but this may hurt performance.
- `setState` does not update `this.state` immediately. This makes reading `this.state` right after calling `setState` a potential pitfall. Instead, use [`componentDidUpdate`]() or the setState `callback` argument, either of which are guaranteed to fire after the update has been applied. If you need to set the state based on the previous state, you can pass a function to `nextState` as described above.
### Note
Calling `setState` in class components is similar to calling a [`set` function](https://react.dev/reference/react/useState) in function components.
[See how to migrate.]()
* * *
### `shouldComponentUpdate(nextProps, nextState, nextContext)`[Link for this heading]()
If you define `shouldComponentUpdate`, React will call it to determine whether a re-render can be skipped.
If you are confident you want to write it by hand, you may compare `this.props` with `nextProps` and `this.state` with `nextState` and return `false` to tell React the update can be skipped.
```
class Rectangle extends Component {
state = {
isHovered: false
};
shouldComponentUpdate(nextProps, nextState) {
if (
nextProps.position.x === this.props.position.x &&
nextProps.position.y === this.props.position.y &&
nextProps.size.width === this.props.size.width &&
nextProps.size.height === this.props.size.height &&
nextState.isHovered === this.state.isHovered
) {
// Nothing has changed, so a re-render is unnecessary
return false;
}
return true;
}
// ...
}
```
React calls `shouldComponentUpdate` before rendering when new props or state are being received. Defaults to `true`. This method is not called for the initial render or when [`forceUpdate`]() is used.
#### Parameters[Link for Parameters]()
- `nextProps`: The next props that the component is about to render with. Compare `nextProps` to [`this.props`]() to determine what changed.
- `nextState`: The next state that the component is about to render with. Compare `nextState` to [`this.state`]() to determine what changed.
- `nextContext`: The next context that the component is about to render with. Compare `nextContext` to [`this.context`]() to determine what changed. Only available if you specify [`static contextType`]().
#### Returns[Link for Returns]()
Return `true` if you want the component to re-render. That’s the default behavior.
Return `false` to tell React that re-rendering can be skipped.
#### Caveats[Link for Caveats]()
- This method *only* exists as a performance optimization. If your component breaks without it, fix that first.
- Consider using [`PureComponent`](https://react.dev/reference/react/PureComponent) instead of writing `shouldComponentUpdate` by hand. `PureComponent` shallowly compares props and state, and reduces the chance that you’ll skip a necessary update.
- We do not recommend doing deep equality checks or using `JSON.stringify` in `shouldComponentUpdate`. It makes performance unpredictable and dependent on the data structure of every prop and state. In the best case, you risk introducing multi-second stalls to your application, and in the worst case you risk crashing it.
- Returning `false` does not prevent child components from re-rendering when *their* state changes.
- Returning `false` does not *guarantee* that the component will not re-render. React will use the return value as a hint but it may still choose to re-render your component if it makes sense to do for other reasons.
### Note
Optimizing class components with `shouldComponentUpdate` is similar to optimizing function components with [`memo`.](https://react.dev/reference/react/memo) Function components also offer more granular optimization with [`useMemo`.](https://react.dev/reference/react/useMemo)
* * *
### `UNSAFE_componentWillMount()`[Link for this heading]()
If you define `UNSAFE_componentWillMount`, React will call it immediately after the [`constructor`.]() It only exists for historical reasons and should not be used in any new code. Instead, use one of the alternatives:
- To initialize state, declare [`state`]() as a class field or set `this.state` inside the [`constructor`.]()
- If you need to run a side effect or set up a subscription, move that logic to [`componentDidMount`]() instead.
[See examples of migrating away from unsafe lifecycles.](https://legacy.reactjs.org/blog/2018/03/27/update-on-async-rendering.html)
#### Parameters[Link for Parameters]()
`UNSAFE_componentWillMount` does not take any parameters.
#### Returns[Link for Returns]()
`UNSAFE_componentWillMount` should not return anything.
#### Caveats[Link for Caveats]()
- `UNSAFE_componentWillMount` will not get called if the component implements [`static getDerivedStateFromProps`]() or [`getSnapshotBeforeUpdate`.]()
- Despite its naming, `UNSAFE_componentWillMount` does not guarantee that the component *will* get mounted if your app uses modern React features like [`Suspense`.](https://react.dev/reference/react/Suspense) If a render attempt is suspended (for example, because the code for some child component has not loaded yet), React will throw the in-progress tree away and attempt to construct the component from scratch during the next attempt. This is why this method is “unsafe”. Code that relies on mounting (like adding a subscription) should go into [`componentDidMount`.]()
- `UNSAFE_componentWillMount` is the only lifecycle method that runs during [server rendering.](https://react.dev/reference/react-dom/server) For all practical purposes, it is identical to [`constructor`,]() so you should use the `constructor` for this type of logic instead.
### Note
Calling [`setState`]() inside `UNSAFE_componentWillMount` in a class component to initialize state is equivalent to passing that state as the initial state to [`useState`](https://react.dev/reference/react/useState) in a function component.
* * *
### `UNSAFE_componentWillReceiveProps(nextProps, nextContext)`[Link for this heading]()
If you define `UNSAFE_componentWillReceiveProps`, React will call it when the component receives new props. It only exists for historical reasons and should not be used in any new code. Instead, use one of the alternatives:
- If you need to **run a side effect** (for example, fetch data, run an animation, or reinitialize a subscription) in response to prop changes, move that logic to [`componentDidUpdate`]() instead.
- If you need to **avoid re-computing some data only when a prop changes,** use a [memoization helper](https://legacy.reactjs.org/blog/2018/06/07/you-probably-dont-need-derived-state.html) instead.
- If you need to **“reset” some state when a prop changes,** consider either making a component [fully controlled](https://legacy.reactjs.org/blog/2018/06/07/you-probably-dont-need-derived-state.html) or [fully uncontrolled with a key](https://legacy.reactjs.org/blog/2018/06/07/you-probably-dont-need-derived-state.html) instead.
- If you need to **“adjust” some state when a prop changes,** check whether you can compute all the necessary information from props alone during rendering. If you can’t, use [`static getDerivedStateFromProps`](https://react.dev/reference/react/Component) instead.
[See examples of migrating away from unsafe lifecycles.](https://legacy.reactjs.org/blog/2018/03/27/update-on-async-rendering.html)
#### Parameters[Link for Parameters]()
- `nextProps`: The next props that the component is about to receive from its parent component. Compare `nextProps` to [`this.props`]() to determine what changed.
- `nextContext`: The next context that the component is about to receive from the closest provider. Compare `nextContext` to [`this.context`]() to determine what changed. Only available if you specify [`static contextType`]().
#### Returns[Link for Returns]()
`UNSAFE_componentWillReceiveProps` should not return anything.
#### Caveats[Link for Caveats]()
- `UNSAFE_componentWillReceiveProps` will not get called if the component implements [`static getDerivedStateFromProps`]() or [`getSnapshotBeforeUpdate`.]()
- Despite its naming, `UNSAFE_componentWillReceiveProps` does not guarantee that the component *will* receive those props if your app uses modern React features like [`Suspense`.](https://react.dev/reference/react/Suspense) If a render attempt is suspended (for example, because the code for some child component has not loaded yet), React will throw the in-progress tree away and attempt to construct the component from scratch during the next attempt. By the time of the next render attempt, the props might be different. This is why this method is “unsafe”. Code that should run only for committed updates (like resetting a subscription) should go into [`componentDidUpdate`.]()
- `UNSAFE_componentWillReceiveProps` does not mean that the component has received *different* props than the last time. You need to compare `nextProps` and `this.props` yourself to check if something changed.
- React doesn’t call `UNSAFE_componentWillReceiveProps` with initial props during mounting. It only calls this method if some of component’s props are going to be updated. For example, calling [`setState`]() doesn’t generally trigger `UNSAFE_componentWillReceiveProps` inside the same component.
### Note
Calling [`setState`]() inside `UNSAFE_componentWillReceiveProps` in a class component to “adjust” state is equivalent to [calling the `set` function from `useState` during rendering](https://react.dev/reference/react/useState) in a function component.
* * *
### `UNSAFE_componentWillUpdate(nextProps, nextState)`[Link for this heading]()
If you define `UNSAFE_componentWillUpdate`, React will call it before rendering with the new props or state. It only exists for historical reasons and should not be used in any new code. Instead, use one of the alternatives:
- If you need to run a side effect (for example, fetch data, run an animation, or reinitialize a subscription) in response to prop or state changes, move that logic to [`componentDidUpdate`]() instead.
- If you need to read some information from the DOM (for example, to save the current scroll position) so that you can use it in [`componentDidUpdate`]() later, read it inside [`getSnapshotBeforeUpdate`]() instead.
[See examples of migrating away from unsafe lifecycles.](https://legacy.reactjs.org/blog/2018/03/27/update-on-async-rendering.html)
#### Parameters[Link for Parameters]()
- `nextProps`: The next props that the component is about to render with. Compare `nextProps` to [`this.props`]() to determine what changed.
- `nextState`: The next state that the component is about to render with. Compare `nextState` to [`this.state`]() to determine what changed.
#### Returns[Link for Returns]()
`UNSAFE_componentWillUpdate` should not return anything.
#### Caveats[Link for Caveats]()
- `UNSAFE_componentWillUpdate` will not get called if [`shouldComponentUpdate`]() is defined and returns `false`.
- `UNSAFE_componentWillUpdate` will not get called if the component implements [`static getDerivedStateFromProps`]() or [`getSnapshotBeforeUpdate`.]()
- It’s not supported to call [`setState`]() (or any method that leads to `setState` being called, like dispatching a Redux action) during `componentWillUpdate`.
- Despite its naming, `UNSAFE_componentWillUpdate` does not guarantee that the component *will* update if your app uses modern React features like [`Suspense`.](https://react.dev/reference/react/Suspense) If a render attempt is suspended (for example, because the code for some child component has not loaded yet), React will throw the in-progress tree away and attempt to construct the component from scratch during the next attempt. By the time of the next render attempt, the props and state might be different. This is why this method is “unsafe”. Code that should run only for committed updates (like resetting a subscription) should go into [`componentDidUpdate`.]()
- `UNSAFE_componentWillUpdate` does not mean that the component has received *different* props or state than the last time. You need to compare `nextProps` with `this.props` and `nextState` with `this.state` yourself to check if something changed.
- React doesn’t call `UNSAFE_componentWillUpdate` with initial props and state during mounting.
### Note
There is no direct equivalent to `UNSAFE_componentWillUpdate` in function components.
* * *
### `static contextType`[Link for this heading]()
If you want to read [`this.context`]() from your class component, you must specify which context it needs to read. The context you specify as the `static contextType` must be a value previously created by [`createContext`.](https://react.dev/reference/react/createContext)
```
class Button extends Component {
static contextType = ThemeContext;
render() {
const theme = this.context;
const className = 'button-' + theme;
return (
<button className={className}>
{this.props.children}
</button>
);
}
}
```
### Note
Reading `this.context` in class components is equivalent to [`useContext`](https://react.dev/reference/react/useContext) in function components.
[See how to migrate.]()
* * *
### `static defaultProps`[Link for this heading]()
You can define `static defaultProps` to set the default props for the class. They will be used for `undefined` and missing props, but not for `null` props.
For example, here is how you define that the `color` prop should default to `'blue'`:
```
class Button extends Component {
static defaultProps = {
color: 'blue'
};
render() {
return <button className={this.props.color}>click me</button>;
}
}
```
If the `color` prop is not provided or is `undefined`, it will be set by default to `'blue'`:
```
<>
{/* this.props.color is "blue" */}
<Button />
{/* this.props.color is "blue" */}
<Button color={undefined} />
{/* this.props.color is null */}
<Button color={null} />
{/* this.props.color is "red" */}
<Button color="red" />
</>
```
### Note
Defining `defaultProps` in class components is similar to using [default values](https://react.dev/learn/passing-props-to-a-component) in function components.
* * *
### `static getDerivedStateFromError(error)`[Link for this heading]()
If you define `static getDerivedStateFromError`, React will call it when a child component (including distant children) throws an error during rendering. This lets you display an error message instead of clearing the UI.
Typically, it is used together with [`componentDidCatch`]() which lets you send the error report to some analytics service. A component with these methods is called an *error boundary.*
[See an example.]()
#### Parameters[Link for Parameters]()
- `error`: The error that was thrown. In practice, it will usually be an instance of [`Error`](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Error) but this is not guaranteed because JavaScript allows to [`throw`](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Statements/throw) any value, including strings or even `null`.
#### Returns[Link for Returns]()
`static getDerivedStateFromError` should return the state telling the component to display the error message.
#### Caveats[Link for Caveats]()
- `static getDerivedStateFromError` should be a pure function. If you want to perform a side effect (for example, to call an analytics service), you need to also implement [`componentDidCatch`.]()
### Note
There is no direct equivalent for `static getDerivedStateFromError` in function components yet. If you’d like to avoid creating class components, write a single `ErrorBoundary` component like above and use it throughout your app. Alternatively, use the [`react-error-boundary`](https://github.com/bvaughn/react-error-boundary) package which does that.
* * *
### `static getDerivedStateFromProps(props, state)`[Link for this heading]()
If you define `static getDerivedStateFromProps`, React will call it right before calling [`render`,]() both on the initial mount and on subsequent updates. It should return an object to update the state, or `null` to update nothing.
This method exists for [rare use cases](https://legacy.reactjs.org/blog/2018/06/07/you-probably-dont-need-derived-state.html) where the state depends on changes in props over time. For example, this `Form` component resets the `email` state when the `userID` prop changes:
```
class Form extends Component {
state = {
email: this.props.defaultEmail,
prevUserID: this.props.userID
};
static getDerivedStateFromProps(props, state) {
// Any time the current user changes,
// Reset any parts of state that are tied to that user.
// In this simple example, that's just the email.
if (props.userID !== state.prevUserID) {
return {
prevUserID: props.userID,
email: props.defaultEmail
};
}
return null;
}
// ...
}
```
Note that this pattern requires you to keep a previous value of the prop (like `userID`) in state (like `prevUserID`).
### Pitfall
Deriving state leads to verbose code and makes your components difficult to think about. [Make sure you’re familiar with simpler alternatives:](https://legacy.reactjs.org/blog/2018/06/07/you-probably-dont-need-derived-state.html)
- If you need to **perform a side effect** (for example, data fetching or an animation) in response to a change in props, use [`componentDidUpdate`]() method instead.
- If you want to **re-compute some data only when a prop changes,** [use a memoization helper instead.](https://legacy.reactjs.org/blog/2018/06/07/you-probably-dont-need-derived-state.html)
- If you want to **“reset” some state when a prop changes,** consider either making a component [fully controlled](https://legacy.reactjs.org/blog/2018/06/07/you-probably-dont-need-derived-state.html) or [fully uncontrolled with a key](https://legacy.reactjs.org/blog/2018/06/07/you-probably-dont-need-derived-state.html) instead.
#### Parameters[Link for Parameters]()
- `props`: The next props that the component is about to render with.
- `state`: The next state that the component is about to render with.
#### Returns[Link for Returns]()
`static getDerivedStateFromProps` return an object to update the state, or `null` to update nothing.
#### Caveats[Link for Caveats]()
- This method is fired on *every* render, regardless of the cause. This is different from [`UNSAFE_componentWillReceiveProps`](), which only fires when the parent causes a re-render and not as a result of a local `setState`.
- This method doesn’t have access to the component instance. If you’d like, you can reuse some code between `static getDerivedStateFromProps` and the other class methods by extracting pure functions of the component props and state outside the class definition.
### Note
Implementing `static getDerivedStateFromProps` in a class component is equivalent to [calling the `set` function from `useState` during rendering](https://react.dev/reference/react/useState) in a function component.
* * *
## Usage[Link for Usage]()
### Defining a class component[Link for Defining a class component]()
To define a React component as a class, extend the built-in `Component` class and define a [`render` method:]()
```
import { Component } from 'react';
class Greeting extends Component {
render() {
return <h1>Hello, {this.props.name}!</h1>;
}
}
```
React will call your [`render`]() method whenever it needs to figure out what to display on the screen. Usually, you will return some [JSX](https://react.dev/learn/writing-markup-with-jsx) from it. Your `render` method should be a [pure function:](https://en.wikipedia.org/wiki/Pure_function) it should only calculate the JSX.
Similarly to [function components,](https://react.dev/learn/your-first-component) a class component can [receive information by props](https://react.dev/learn/your-first-component) from its parent component. However, the syntax for reading props is different. For example, if the parent component renders `<Greeting name="Taylor" />`, then you can read the `name` prop from [`this.props`](), like `this.props.name`:
App.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import { Component } from 'react';
class Greeting extends Component {
render() {
return <h1>Hello, {this.props.name}!</h1>;
}
}
export default function App() {
return (
<>
<Greeting name="Sara" />
<Greeting name="Cahal" />
<Greeting name="Edite" />
</>
);
}
```
Show more
Note that Hooks (functions starting with `use`, like [`useState`](https://react.dev/reference/react/useState)) are not supported inside class components.
### Pitfall
We recommend defining components as functions instead of classes. [See how to migrate.]()
* * *
### Adding state to a class component[Link for Adding state to a class component]()
To add [state](https://react.dev/learn/state-a-components-memory) to a class, assign an object to a property called [`state`](). To update state, call [`this.setState`]().
App.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import { Component } from 'react';
export default class Counter extends Component {
state = {
name: 'Taylor',
age: 42,
};
handleNameChange = (e) => {
this.setState({
name: e.target.value
});
}
handleAgeChange = () => {
this.setState({
age: this.state.age + 1
});
};
render() {
return (
<>
<input
value={this.state.name}
onChange={this.handleNameChange}
/>
<button onClick={this.handleAgeChange}>
Increment age
</button>
<p>Hello, {this.state.name}. You are {this.state.age}.</p>
</>
);
}
}
```
Show more
### Pitfall
We recommend defining components as functions instead of classes. [See how to migrate.]()
* * *
### Adding lifecycle methods to a class component[Link for Adding lifecycle methods to a class component]()
There are a few special methods you can define on your class.
If you define the [`componentDidMount`]() method, React will call it when your component is added *(mounted)* to the screen. React will call [`componentDidUpdate`]() after your component re-renders due to changed props or state. React will call [`componentWillUnmount`]() after your component has been removed *(unmounted)* from the screen.
If you implement `componentDidMount`, you usually need to implement all three lifecycles to avoid bugs. For example, if `componentDidMount` reads some state or props, you also have to implement `componentDidUpdate` to handle their changes, and `componentWillUnmount` to clean up whatever `componentDidMount` was doing.
For example, this `ChatRoom` component keeps a chat connection synchronized with props and state:
App.jsChatRoom.jschat.js
ChatRoom.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import { Component } from 'react';
import { createConnection } from './chat.js';
export default class ChatRoom extends Component {
state = {
serverUrl: 'https://localhost:1234'
};
componentDidMount() {
this.setupConnection();
}
componentDidUpdate(prevProps, prevState) {
if (
this.props.roomId !== prevProps.roomId ||
this.state.serverUrl !== prevState.serverUrl
) {
this.destroyConnection();
this.setupConnection();
}
}
componentWillUnmount() {
this.destroyConnection();
}
setupConnection() {
this.connection = createConnection(
this.state.serverUrl,
this.props.roomId
);
this.connection.connect();
}
destroyConnection() {
this.connection.disconnect();
this.connection = null;
}
render() {
return (
<>
<label>
Server URL:{' '}
<input
value={this.state.serverUrl}
onChange={e => {
this.setState({
serverUrl: e.target.value
});
}}
/>
</label>
<h1>Welcome to the {this.props.roomId} room!</h1>
</>
);
}
}
```
Show more
Note that in development when [Strict Mode](https://react.dev/reference/react/StrictMode) is on, React will call `componentDidMount`, immediately call `componentWillUnmount`, and then call `componentDidMount` again. This helps you notice if you forgot to implement `componentWillUnmount` or if its logic doesn’t fully “mirror” what `componentDidMount` does.
### Pitfall
We recommend defining components as functions instead of classes. [See how to migrate.]()
* * *
### Catching rendering errors with an error boundary[Link for Catching rendering errors with an error boundary]()
By default, if your application throws an error during rendering, React will remove its UI from the screen. To prevent this, you can wrap a part of your UI into an *error boundary*. An error boundary is a special component that lets you display some fallback UI instead of the part that crashed—for example, an error message.
To implement an error boundary component, you need to provide [`static getDerivedStateFromError`]() which lets you update state in response to an error and display an error message to the user. You can also optionally implement [`componentDidCatch`]() to add some extra logic, for example, to log the error to an analytics service.
```
class ErrorBoundary extends React.Component {
constructor(props) {
super(props);
this.state = { hasError: false };
}
static getDerivedStateFromError(error) {
// Update state so the next render will show the fallback UI.
return { hasError: true };
}
componentDidCatch(error, info) {
// Example "componentStack":
// in ComponentThatThrows (created by App)
// in ErrorBoundary (created by App)
// in div (created by App)
// in App
logErrorToMyService(error, info.componentStack);
}
render() {
if (this.state.hasError) {
// You can render any custom fallback UI
return this.props.fallback;
}
return this.props.children;
}
}
```
Then you can wrap a part of your component tree with it:
```
<ErrorBoundary fallback={<p>Something went wrong</p>}>
<Profile />
</ErrorBoundary>
```
If `Profile` or its child component throws an error, `ErrorBoundary` will “catch” that error, display a fallback UI with the error message you’ve provided, and send a production error report to your error reporting service.
You don’t need to wrap every component into a separate error boundary. When you think about the [granularity of error boundaries,](https://www.brandondail.com/posts/fault-tolerance-react) consider where it makes sense to display an error message. For example, in a messaging app, it makes sense to place an error boundary around the list of conversations. It also makes sense to place one around every individual message. However, it wouldn’t make sense to place a boundary around every avatar.
### Note
There is currently no way to write an error boundary as a function component. However, you don’t have to write the error boundary class yourself. For example, you can use [`react-error-boundary`](https://github.com/bvaughn/react-error-boundary) instead.
* * *
## Alternatives[Link for Alternatives]()
### Migrating a simple component from a class to a function[Link for Migrating a simple component from a class to a function]()
Typically, you will [define components as functions](https://react.dev/learn/your-first-component) instead.
For example, suppose you’re converting this `Greeting` class component to a function:
App.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import { Component } from 'react';
class Greeting extends Component {
render() {
return <h1>Hello, {this.props.name}!</h1>;
}
}
export default function App() {
return (
<>
<Greeting name="Sara" />
<Greeting name="Cahal" />
<Greeting name="Edite" />
</>
);
}
```
Show more
Define a function called `Greeting`. This is where you will move the body of your `render` function.
```
function Greeting() {
// ... move the code from the render method here ...
}
```
Instead of `this.props.name`, define the `name` prop [using the destructuring syntax](https://react.dev/learn/passing-props-to-a-component) and read it directly:
```
function Greeting({ name }) {
return <h1>Hello, {name}!</h1>;
}
```
Here is a complete example:
App.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
function Greeting({ name }) {
return <h1>Hello, {name}!</h1>;
}
export default function App() {
return (
<>
<Greeting name="Sara" />
<Greeting name="Cahal" />
<Greeting name="Edite" />
</>
);
}
```
* * *
### Migrating a component with state from a class to a function[Link for Migrating a component with state from a class to a function]()
Suppose you’re converting this `Counter` class component to a function:
App.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import { Component } from 'react';
export default class Counter extends Component {
state = {
name: 'Taylor',
age: 42,
};
handleNameChange = (e) => {
this.setState({
name: e.target.value
});
}
handleAgeChange = (e) => {
this.setState({
age: this.state.age + 1
});
};
render() {
return (
<>
<input
value={this.state.name}
onChange={this.handleNameChange}
/>
<button onClick={this.handleAgeChange}>
Increment age
</button>
<p>Hello, {this.state.name}. You are {this.state.age}.</p>
</>
);
}
}
```
Show more
Start by declaring a function with the necessary [state variables:](https://react.dev/reference/react/useState)
```
import { useState } from 'react';
function Counter() {
const [name, setName] = useState('Taylor');
const [age, setAge] = useState(42);
// ...
```
Next, convert the event handlers:
```
function Counter() {
const [name, setName] = useState('Taylor');
const [age, setAge] = useState(42);
function handleNameChange(e) {
setName(e.target.value);
}
function handleAgeChange() {
setAge(age + 1);
}
// ...
```
Finally, replace all references starting with `this` with the variables and functions you defined in your component. For example, replace `this.state.age` with `age`, and replace `this.handleNameChange` with `handleNameChange`.
Here is a fully converted component:
App.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import { useState } from 'react';
export default function Counter() {
const [name, setName] = useState('Taylor');
const [age, setAge] = useState(42);
function handleNameChange(e) {
setName(e.target.value);
}
function handleAgeChange() {
setAge(age + 1);
}
return (
<>
<input
value={name}
onChange={handleNameChange}
/>
<button onClick={handleAgeChange}>
Increment age
</button>
<p>Hello, {name}. You are {age}.</p>
</>
)
}
```
Show more
* * *
### Migrating a component with lifecycle methods from a class to a function[Link for Migrating a component with lifecycle methods from a class to a function]()
Suppose you’re converting this `ChatRoom` class component with lifecycle methods to a function:
App.jsChatRoom.jschat.js
ChatRoom.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import { Component } from 'react';
import { createConnection } from './chat.js';
export default class ChatRoom extends Component {
state = {
serverUrl: 'https://localhost:1234'
};
componentDidMount() {
this.setupConnection();
}
componentDidUpdate(prevProps, prevState) {
if (
this.props.roomId !== prevProps.roomId ||
this.state.serverUrl !== prevState.serverUrl
) {
this.destroyConnection();
this.setupConnection();
}
}
componentWillUnmount() {
this.destroyConnection();
}
setupConnection() {
this.connection = createConnection(
this.state.serverUrl,
this.props.roomId
);
this.connection.connect();
}
destroyConnection() {
this.connection.disconnect();
this.connection = null;
}
render() {
return (
<>
<label>
Server URL:{' '}
<input
value={this.state.serverUrl}
onChange={e => {
this.setState({
serverUrl: e.target.value
});
}}
/>
</label>
<h1>Welcome to the {this.props.roomId} room!</h1>
</>
);
}
}
```
Show more
First, verify that your [`componentWillUnmount`]() does the opposite of [`componentDidMount`.]() In the above example, that’s true: it disconnects the connection that `componentDidMount` sets up. If such logic is missing, add it first.
Next, verify that your [`componentDidUpdate`]() method handles changes to any props and state you’re using in `componentDidMount`. In the above example, `componentDidMount` calls `setupConnection` which reads `this.state.serverUrl` and `this.props.roomId`. This is why `componentDidUpdate` checks whether `this.state.serverUrl` and `this.props.roomId` have changed, and resets the connection if they did. If your `componentDidUpdate` logic is missing or doesn’t handle changes to all relevant props and state, fix that first.
In the above example, the logic inside the lifecycle methods connects the component to a system outside of React (a chat server). To connect a component to an external system, [describe this logic as a single Effect:](https://react.dev/reference/react/useEffect)
```
import { useState, useEffect } from 'react';
function ChatRoom({ roomId }) {
const [serverUrl, setServerUrl] = useState('https://localhost:1234');
useEffect(() => {
const connection = createConnection(serverUrl, roomId);
connection.connect();
return () => {
connection.disconnect();
};
}, [serverUrl, roomId]);
// ...
}
```
This [`useEffect`](https://react.dev/reference/react/useEffect) call is equivalent to the logic in the lifecycle methods above. If your lifecycle methods do multiple unrelated things, [split them into multiple independent Effects.](https://react.dev/learn/removing-effect-dependencies) Here is a complete example you can play with:
App.jsChatRoom.jschat.js
ChatRoom.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import { useState, useEffect } from 'react';
import { createConnection } from './chat.js';
export default function ChatRoom({ roomId }) {
const [serverUrl, setServerUrl] = useState('https://localhost:1234');
useEffect(() => {
const connection = createConnection(serverUrl, roomId);
connection.connect();
return () => {
connection.disconnect();
};
}, [roomId, serverUrl]);
return (
<>
<label>
Server URL:{' '}
<input
value={serverUrl}
onChange={e => setServerUrl(e.target.value)}
/>
</label>
<h1>Welcome to the {roomId} room!</h1>
</>
);
}
```
Show more
### Note
If your component does not synchronize with any external systems, [you might not need an Effect.](https://react.dev/learn/you-might-not-need-an-effect)
* * *
### Migrating a component with context from a class to a function[Link for Migrating a component with context from a class to a function]()
In this example, the `Panel` and `Button` class components read [context](https://react.dev/learn/passing-data-deeply-with-context) from [`this.context`:]()
App.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import { createContext, Component } from 'react';
const ThemeContext = createContext(null);
class Panel extends Component {
static contextType = ThemeContext;
render() {
const theme = this.context;
const className = 'panel-' + theme;
return (
<section className={className}>
<h1>{this.props.title}</h1>
{this.props.children}
</section>
);
}
}
class Button extends Component {
static contextType = ThemeContext;
render() {
const theme = this.context;
const className = 'button-' + theme;
return (
<button className={className}>
{this.props.children}
</button>
);
}
}
function Form() {
return (
<Panel title="Welcome">
<Button>Sign up</Button>
<Button>Log in</Button>
</Panel>
);
}
export default function MyApp() {
return (
<ThemeContext.Provider value="dark">
<Form />
</ThemeContext.Provider>
)
}
```
Show more
When you convert them to function components, replace `this.context` with [`useContext`](https://react.dev/reference/react/useContext) calls:
App.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import { createContext, useContext } from 'react';
const ThemeContext = createContext(null);
function Panel({ title, children }) {
const theme = useContext(ThemeContext);
const className = 'panel-' + theme;
return (
<section className={className}>
<h1>{title}</h1>
{children}
</section>
)
}
function Button({ children }) {
const theme = useContext(ThemeContext);
const className = 'button-' + theme;
return (
<button className={className}>
{children}
</button>
);
}
function Form() {
return (
<Panel title="Welcome">
<Button>Sign up</Button>
<Button>Log in</Button>
</Panel>
);
}
export default function MyApp() {
return (
<ThemeContext.Provider value="dark">
<Form />
</ThemeContext.Provider>
)
}
```
Show more
[PreviouscloneElement](https://react.dev/reference/react/cloneElement)
[NextcreateElement](https://react.dev/reference/react/createElement) |
https://react.dev/learn/your-first-component | [Learn React](https://react.dev/learn)
[Describing the UI](https://react.dev/learn/describing-the-ui)
# Your First Component[Link for this heading]()
*Components* are one of the core concepts of React. They are the foundation upon which you build user interfaces (UI), which makes them the perfect place to start your React journey!
### You will learn
- What a component is
- What role components play in a React application
- How to write your first React component
## Components: UI building blocks[Link for Components: UI building blocks]()
On the Web, HTML lets us create rich structured documents with its built-in set of tags like `<h1>` and `<li>`:
```
<article>
<h1>My First Component</h1>
<ol>
<li>Components: UI Building Blocks</li>
<li>Defining a Component</li>
<li>Using a Component</li>
</ol>
</article>
```
This markup represents this article `<article>`, its heading `<h1>`, and an (abbreviated) table of contents as an ordered list `<ol>`. Markup like this, combined with CSS for style, and JavaScript for interactivity, lies behind every sidebar, avatar, modal, dropdown—every piece of UI you see on the Web.
React lets you combine your markup, CSS, and JavaScript into custom “components”, **reusable UI elements for your app.** The table of contents code you saw above could be turned into a `<TableOfContents />` component you could render on every page. Under the hood, it still uses the same HTML tags like `<article>`, `<h1>`, etc.
Just like with HTML tags, you can compose, order and nest components to design whole pages. For example, the documentation page you’re reading is made out of React components:
```
<PageLayout>
<NavigationHeader>
<SearchBar />
<Link to="/docs">Docs</Link>
</NavigationHeader>
<Sidebar />
<PageContent>
<TableOfContents />
<DocumentationText />
</PageContent>
</PageLayout>
```
As your project grows, you will notice that many of your designs can be composed by reusing components you already wrote, speeding up your development. Our table of contents above could be added to any screen with `<TableOfContents />`! You can even jumpstart your project with the thousands of components shared by the React open source community like [Chakra UI](https://chakra-ui.com/) and [Material UI.](https://material-ui.com/)
## Defining a component[Link for Defining a component]()
Traditionally when creating web pages, web developers marked up their content and then added interaction by sprinkling on some JavaScript. This worked great when interaction was a nice-to-have on the web. Now it is expected for many sites and all apps. React puts interactivity first while still using the same technology: **a React component is a JavaScript function that you can *sprinkle with markup*.** Here’s what that looks like (you can edit the example below):
App.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
export default function Profile() {
return (
<img
src="https://i.imgur.com/MK3eW3Am.jpg"
alt="Katherine Johnson"
/>
)
}
```
And here’s how to build a component:
### Step 1: Export the component[Link for Step 1: Export the component]()
The `export default` prefix is a [standard JavaScript syntax](https://developer.mozilla.org/docs/web/javascript/reference/statements/export) (not specific to React). It lets you mark the main function in a file so that you can later import it from other files. (More on importing in [Importing and Exporting Components](https://react.dev/learn/importing-and-exporting-components)!)
### Step 2: Define the function[Link for Step 2: Define the function]()
With `function Profile() { }` you define a JavaScript function with the name `Profile`.
### Pitfall
React components are regular JavaScript functions, but **their names must start with a capital letter** or they won’t work!
### Step 3: Add markup[Link for Step 3: Add markup]()
The component returns an `<img />` tag with `src` and `alt` attributes. `<img />` is written like HTML, but it is actually JavaScript under the hood! This syntax is called [JSX](https://react.dev/learn/writing-markup-with-jsx), and it lets you embed markup inside JavaScript.
Return statements can be written all on one line, as in this component:
```
return <img src="https://i.imgur.com/MK3eW3As.jpg" alt="Katherine Johnson" />;
```
But if your markup isn’t all on the same line as the `return` keyword, you must wrap it in a pair of parentheses:
```
return (
<div>
<img src="https://i.imgur.com/MK3eW3As.jpg" alt="Katherine Johnson" />
</div>
);
```
### Pitfall
Without parentheses, any code on the lines after `return` [will be ignored](https://stackoverflow.com/questions/2846283/what-are-the-rules-for-javascripts-automatic-semicolon-insertion-asi)!
## Using a component[Link for Using a component]()
Now that you’ve defined your `Profile` component, you can nest it inside other components. For example, you can export a `Gallery` component that uses multiple `Profile` components:
App.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
function Profile() {
return (
<img
src="https://i.imgur.com/MK3eW3As.jpg"
alt="Katherine Johnson"
/>
);
}
export default function Gallery() {
return (
<section>
<h1>Amazing scientists</h1>
<Profile />
<Profile />
<Profile />
</section>
);
}
```
Show more
### What the browser sees[Link for What the browser sees]()
Notice the difference in casing:
- `<section>` is lowercase, so React knows we refer to an HTML tag.
- `<Profile />` starts with a capital `P`, so React knows that we want to use our component called `Profile`.
And `Profile` contains even more HTML: `<img />`. In the end, this is what the browser sees:
```
<section>
<h1>Amazing scientists</h1>
<img src="https://i.imgur.com/MK3eW3As.jpg" alt="Katherine Johnson" />
<img src="https://i.imgur.com/MK3eW3As.jpg" alt="Katherine Johnson" />
<img src="https://i.imgur.com/MK3eW3As.jpg" alt="Katherine Johnson" />
</section>
```
### Nesting and organizing components[Link for Nesting and organizing components]()
Components are regular JavaScript functions, so you can keep multiple components in the same file. This is convenient when components are relatively small or tightly related to each other. If this file gets crowded, you can always move `Profile` to a separate file. You will learn how to do this shortly on the [page about imports.](https://react.dev/learn/importing-and-exporting-components)
Because the `Profile` components are rendered inside `Gallery`—even several times!—we can say that `Gallery` is a **parent component,** rendering each `Profile` as a “child”. This is part of the magic of React: you can define a component once, and then use it in as many places and as many times as you like.
### Pitfall
Components can render other components, but **you must never nest their definitions:**
```
export default function Gallery() {
// 🔴 Never define a component inside another component!
function Profile() {
// ...
}
// ...
}
```
The snippet above is [very slow and causes bugs.](https://react.dev/learn/preserving-and-resetting-state) Instead, define every component at the top level:
```
export default function Gallery() {
// ...
}
// ✅ Declare components at the top level
function Profile() {
// ...
}
```
When a child component needs some data from a parent, [pass it by props](https://react.dev/learn/passing-props-to-a-component) instead of nesting definitions.
##### Deep Dive
#### Components all the way down[Link for Components all the way down]()
Show Details
Your React application begins at a “root” component. Usually, it is created automatically when you start a new project. For example, if you use [CodeSandbox](https://codesandbox.io/) or if you use the framework [Next.js](https://nextjs.org/), the root component is defined in `pages/index.js`. In these examples, you’ve been exporting root components.
Most React apps use components all the way down. This means that you won’t only use components for reusable pieces like buttons, but also for larger pieces like sidebars, lists, and ultimately, complete pages! Components are a handy way to organize UI code and markup, even if some of them are only used once.
[React-based frameworks](https://react.dev/learn/start-a-new-react-project) take this a step further. Instead of using an empty HTML file and letting React “take over” managing the page with JavaScript, they *also* generate the HTML automatically from your React components. This allows your app to show some content before the JavaScript code loads.
Still, many websites only use React to [add interactivity to existing HTML pages.](https://react.dev/learn/add-react-to-an-existing-project) They have many root components instead of a single one for the entire page. You can use as much—or as little—React as you need.
## Recap[Link for Recap]()
You’ve just gotten your first taste of React! Let’s recap some key points.
- React lets you create components, **reusable UI elements for your app.**
- In a React app, every piece of UI is a component.
- React components are regular JavaScript functions except:
1. Their names always begin with a capital letter.
2. They return JSX markup.
## Try out some challenges[Link for Try out some challenges]()
1\. Export the component 2. Fix the return statement 3. Spot the mistake 4. Your own component
#### Challenge 1 of 4: Export the component[Link for this heading]()
This sandbox doesn’t work because the root component is not exported:
App.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
function Profile() {
return (
<img
src="https://i.imgur.com/lICfvbD.jpg"
alt="Aklilu Lemma"
/>
);
}
```
Try to fix it yourself before looking at the solution!
Show solutionNext Challenge
[PreviousDescribing the UI](https://react.dev/learn/describing-the-ui)
[NextImporting and Exporting Components](https://react.dev/learn/importing-and-exporting-components) |
https://react.dev/learn/understanding-your-ui-as-a-tree | [Learn React](https://react.dev/learn)
[Describing the UI](https://react.dev/learn/describing-the-ui)
# Understanding Your UI as a Tree[Link for this heading]()
Your React app is taking shape with many components being nested within each other. How does React keep track of your app’s component structure?
React, and many other UI libraries, model UI as a tree. Thinking of your app as a tree is useful for understanding the relationship between components. This understanding will help you debug future concepts like performance and state management.
### You will learn
- How React “sees” component structures
- What a render tree is and what it is useful for
- What a module dependency tree is and what it is useful for
## Your UI as a tree[Link for Your UI as a tree]()
Trees are a relationship model between items and UI is often represented using tree structures. For example, browsers use tree structures to model HTML ([DOM](https://developer.mozilla.org/docs/Web/API/Document_Object_Model/Introduction)) and CSS ([CSSOM](https://developer.mozilla.org/docs/Web/API/CSS_Object_Model)). Mobile platforms also use trees to represent their view hierarchy.


React creates a UI tree from your components. In this example, the UI tree is then used to render to the DOM.
Like browsers and mobile platforms, React also uses tree structures to manage and model the relationship between components in a React app. These trees are useful tools to understand how data flows through a React app and how to optimize rendering and app size.
## The Render Tree[Link for The Render Tree]()
A major feature of components is the ability to compose components of other components. As we [nest components](https://react.dev/learn/your-first-component), we have the concept of parent and child components, where each parent component may itself be a child of another component.
When we render a React app, we can model this relationship in a tree, known as the render tree.
Here is a React app that renders inspirational quotes.
App.jsFancyText.jsInspirationGenerator.jsCopyright.jsquotes.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import FancyText from './FancyText';
import InspirationGenerator from './InspirationGenerator';
import Copyright from './Copyright';
export default function App() {
return (
<>
<FancyText title text="Get Inspired App" />
<InspirationGenerator>
<Copyright year={2004} />
</InspirationGenerator>
</>
);
}
```


React creates a *render tree*, a UI tree, composed of the rendered components.
From the example app, we can construct the above render tree.
The tree is composed of nodes, each of which represents a component. `App`, `FancyText`, `Copyright`, to name a few, are all nodes in our tree.
The root node in a React render tree is the [root component](https://react.dev/learn/importing-and-exporting-components) of the app. In this case, the root component is `App` and it is the first component React renders. Each arrow in the tree points from a parent component to a child component.
##### Deep Dive
#### Where are the HTML tags in the render tree?[Link for Where are the HTML tags in the render tree?]()
Show Details
You’ll notice in the above render tree, there is no mention of the HTML tags that each component renders. This is because the render tree is only composed of React [components](https://react.dev/learn/your-first-component).
React, as a UI framework, is platform agnostic. On react.dev, we showcase examples that render to the web, which uses HTML markup as its UI primitives. But a React app could just as likely render to a mobile or desktop platform, which may use different UI primitives like [UIView](https://developer.apple.com/documentation/uikit/uiview) or [FrameworkElement](https://learn.microsoft.com/en-us/dotnet/api/system.windows.frameworkelement?view=windowsdesktop-7.0).
These platform UI primitives are not a part of React. React render trees can provide insight to our React app regardless of what platform your app renders to.
A render tree represents a single render pass of a React application. With [conditional rendering](https://react.dev/learn/conditional-rendering), a parent component may render different children depending on the data passed.
We can update the app to conditionally render either an inspirational quote or color.
App.jsFancyText.jsColor.jsInspirationGenerator.jsCopyright.jsinspirations.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import FancyText from './FancyText';
import InspirationGenerator from './InspirationGenerator';
import Copyright from './Copyright';
export default function App() {
return (
<>
<FancyText title text="Get Inspired App" />
<InspirationGenerator>
<Copyright year={2004} />
</InspirationGenerator>
</>
);
}
```


With conditional rendering, across different renders, the render tree may render different components.
In this example, depending on what `inspiration.type` is, we may render `<FancyText>` or `<Color>`. The render tree may be different for each render pass.
Although render trees may differ across render passes, these trees are generally helpful for identifying what the *top-level* and *leaf components* are in a React app. Top-level components are the components nearest to the root component and affect the rendering performance of all the components beneath them and often contain the most complexity. Leaf components are near the bottom of the tree and have no child components and are often frequently re-rendered.
Identifying these categories of components are useful for understanding data flow and performance of your app.
## The Module Dependency Tree[Link for The Module Dependency Tree]()
Another relationship in a React app that can be modeled with a tree are an app’s module dependencies. As we [break up our components](https://react.dev/learn/importing-and-exporting-components) and logic into separate files, we create [JS modules](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Guide/Modules) where we may export components, functions, or constants.
Each node in a module dependency tree is a module and each branch represents an `import` statement in that module.
If we take the previous Inspirations app, we can build a module dependency tree, or dependency tree for short.


The module dependency tree for the Inspirations app.
The root node of the tree is the root module, also known as the entrypoint file. It often is the module that contains the root component.
Comparing to the render tree of the same app, there are similar structures but some notable differences:
- The nodes that make-up the tree represent modules, not components.
- Non-component modules, like `inspirations.js`, are also represented in this tree. The render tree only encapsulates components.
- `Copyright.js` appears under `App.js` but in the render tree, `Copyright`, the component, appears as a child of `InspirationGenerator`. This is because `InspirationGenerator` accepts JSX as [children props](https://react.dev/learn/passing-props-to-a-component), so it renders `Copyright` as a child component but does not import the module.
Dependency trees are useful to determine what modules are necessary to run your React app. When building a React app for production, there is typically a build step that will bundle all the necessary JavaScript to ship to the client. The tool responsible for this is called a [bundler](https://developer.mozilla.org/en-US/docs/Learn/Tools_and_testing/Understanding_client-side_tools/Overview), and bundlers will use the dependency tree to determine what modules should be included.
As your app grows, often the bundle size does too. Large bundle sizes are expensive for a client to download and run. Large bundle sizes can delay the time for your UI to get drawn. Getting a sense of your app’s dependency tree may help with debugging these issues.
## Recap[Link for Recap]()
- Trees are a common way to represent the relationship between entities. They are often used to model UI.
- Render trees represent the nested relationship between React components across a single render.
- With conditional rendering, the render tree may change across different renders. With different prop values, components may render different children components.
- Render trees help identify what the top-level and leaf components are. Top-level components affect the rendering performance of all components beneath them and leaf components are often re-rendered frequently. Identifying them is useful for understanding and debugging rendering performance.
- Dependency trees represent the module dependencies in a React app.
- Dependency trees are used by build tools to bundle the necessary code to ship an app.
- Dependency trees are useful for debugging large bundle sizes that slow time to paint and expose opportunities for optimizing what code is bundled.
[PreviousKeeping Components Pure](https://react.dev/learn/keeping-components-pure)
[NextAdding Interactivity](https://react.dev/learn/adding-interactivity) |
https://react.dev/learn/react-compiler | [Learn React](https://react.dev/learn)
[Installation](https://react.dev/learn/installation)
# React Compiler[Link for this heading]()
This page will give you an introduction to React Compiler and how to try it out successfully.
### Under Construction
These docs are still a work in progress. More documentation is available in the [React Compiler Working Group repo](https://github.com/reactwg/react-compiler/discussions), and will be upstreamed into these docs when they are more stable.
### You will learn
- Getting started with the compiler
- Installing the compiler and ESLint plugin
- Troubleshooting
### Note
React Compiler is a new compiler currently in Beta, that we’ve open sourced to get early feedback from the community. While it has been used in production at companies like Meta, rolling out the compiler to production for your app will depend on the health of your codebase and how well you’ve followed the [Rules of React](https://react.dev/reference/rules).
The latest Beta release can be found with the `@beta` tag, and daily experimental releases with `@experimental`.
React Compiler is a new compiler that we’ve open sourced to get early feedback from the community. It is a build-time only tool that automatically optimizes your React app. It works with plain JavaScript, and understands the [Rules of React](https://react.dev/reference/rules), so you don’t need to rewrite any code to use it.
The compiler also includes an [ESLint plugin]() that surfaces the analysis from the compiler right in your editor. **We strongly recommend everyone use the linter today.** The linter does not require that you have the compiler installed, so you can use it even if you are not ready to try out the compiler.
The compiler is currently released as `beta`, and is available to try out on React 17+ apps and libraries. To install the Beta:
Terminal
Copy
npm install -D babel-plugin-react-compiler@beta eslint-plugin-react-compiler@beta
Or, if you’re using Yarn:
Terminal
Copy
yarn add -D babel-plugin-react-compiler@beta eslint-plugin-react-compiler@beta
If you are not using React 19 yet, please see [the section below]() for further instructions.
### What does the compiler do?[Link for What does the compiler do?]()
In order to optimize applications, React Compiler automatically memoizes your code. You may be familiar today with memoization through APIs such as `useMemo`, `useCallback`, and `React.memo`. With these APIs you can tell React that certain parts of your application don’t need to recompute if their inputs haven’t changed, reducing work on updates. While powerful, it’s easy to forget to apply memoization or apply them incorrectly. This can lead to inefficient updates as React has to check parts of your UI that don’t have any *meaningful* changes.
The compiler uses its knowledge of JavaScript and React’s rules to automatically memoize values or groups of values within your components and hooks. If it detects breakages of the rules, it will automatically skip over just those components or hooks, and continue safely compiling other code.
### Note
React Compiler can statically detect when Rules of React are broken, and safely opt-out of optimizing just the affected components or hooks. It is not necessary for the compiler to optimize 100% of your codebase.
If your codebase is already very well-memoized, you might not expect to see major performance improvements with the compiler. However, in practice memoizing the correct dependencies that cause performance issues is tricky to get right by hand.
##### Deep Dive
#### What kind of memoization does React Compiler add?[Link for What kind of memoization does React Compiler add?]()
Show Details
The initial release of React Compiler is primarily focused on **improving update performance** (re-rendering existing components), so it focuses on these two use cases:
1. **Skipping cascading re-rendering of components**
- Re-rendering `<Parent />` causes many components in its component tree to re-render, even though only `<Parent />` has changed
2. **Skipping expensive calculations from outside of React**
- For example, calling `expensivelyProcessAReallyLargeArrayOfObjects()` inside of your component or hook that needs that data
#### Optimizing Re-renders[Link for Optimizing Re-renders]()
React lets you express your UI as a function of their current state (more concretely: their props, state, and context). In its current implementation, when a component’s state changes, React will re-render that component *and all of its children* — unless you have applied some form of manual memoization with `useMemo()`, `useCallback()`, or `React.memo()`. For example, in the following example, `<MessageButton>` will re-render whenever `<FriendList>`’s state changes:
```
function FriendList({ friends }) {
const onlineCount = useFriendOnlineCount();
if (friends.length === 0) {
return <NoFriends />;
}
return (
<div>
<span>{onlineCount} online</span>
{friends.map((friend) => (
<FriendListCard key={friend.id} friend={friend} />
))}
<MessageButton />
</div>
);
}
```
[*See this example in the React Compiler Playground*](https://playground.react.dev/)
React Compiler automatically applies the equivalent of manual memoization, ensuring that only the relevant parts of an app re-render as state changes, which is sometimes referred to as “fine-grained reactivity”. In the above example, React Compiler determines that the return value of `<FriendListCard />` can be reused even as `friends` changes, and can avoid recreating this JSX *and* avoid re-rendering `<MessageButton>` as the count changes.
#### Expensive calculations also get memoized[Link for Expensive calculations also get memoized]()
The compiler can also automatically memoize for expensive calculations used during rendering:
```
// **Not** memoized by React Compiler, since this is not a component or hook
function expensivelyProcessAReallyLargeArrayOfObjects() { /* ... */ }
// Memoized by React Compiler since this is a component
function TableContainer({ items }) {
// This function call would be memoized:
const data = expensivelyProcessAReallyLargeArrayOfObjects(items);
// ...
}
```
[*See this example in the React Compiler Playground*](https://playground.react.dev/)
However, if `expensivelyProcessAReallyLargeArrayOfObjects` is truly an expensive function, you may want to consider implementing its own memoization outside of React, because:
- React Compiler only memoizes React components and hooks, not every function
- React Compiler’s memoization is not shared across multiple components or hooks
So if `expensivelyProcessAReallyLargeArrayOfObjects` was used in many different components, even if the same exact items were passed down, that expensive calculation would be run repeatedly. We recommend [profiling](https://react.dev/reference/react/useMemo) first to see if it really is that expensive before making code more complicated.
### Should I try out the compiler?[Link for Should I try out the compiler?]()
Please note that the compiler is still in Beta and has many rough edges. While it has been used in production at companies like Meta, rolling out the compiler to production for your app will depend on the health of your codebase and how well you’ve followed the [Rules of React](https://react.dev/reference/rules).
**You don’t have to rush into using the compiler now. It’s okay to wait until it reaches a stable release before adopting it.** However, we do appreciate trying it out in small experiments in your apps so that you can [provide feedback]() to us to help make the compiler better.
## Getting Started[Link for Getting Started]()
In addition to these docs, we recommend checking the [React Compiler Working Group](https://github.com/reactwg/react-compiler) for additional information and discussion about the compiler.
### Installing eslint-plugin-react-compiler[Link for Installing eslint-plugin-react-compiler]()
React Compiler also powers an ESLint plugin. The ESLint plugin can be used **independently** of the compiler, meaning you can use the ESLint plugin even if you don’t use the compiler.
Terminal
Copy
npm install -D eslint-plugin-react-compiler@beta
Then, add it to your ESLint config:
```
import reactCompiler from 'eslint-plugin-react-compiler'
export default [
{
plugins: {
'react-compiler': reactCompiler,
},
rules: {
'react-compiler/react-compiler': 'error',
},
},
]
```
Or, in the deprecated eslintrc config format:
```
module.exports = {
plugins: [
'eslint-plugin-react-compiler',
],
rules: {
'react-compiler/react-compiler': 'error',
},
}
```
The ESLint plugin will display any violations of the rules of React in your editor. When it does this, it means that the compiler has skipped over optimizing that component or hook. This is perfectly okay, and the compiler can recover and continue optimizing other components in your codebase.
### Note
**You don’t have to fix all ESLint violations straight away.** You can address them at your own pace to increase the amount of components and hooks being optimized, but it is not required to fix everything before you can use the compiler.
### Rolling out the compiler to your codebase[Link for Rolling out the compiler to your codebase]()
#### Existing projects[Link for Existing projects]()
The compiler is designed to compile functional components and hooks that follow the [Rules of React](https://react.dev/reference/rules). It can also handle code that breaks those rules by bailing out (skipping over) those components or hooks. However, due to the flexible nature of JavaScript, the compiler cannot catch every possible violation and may compile with false negatives: that is, the compiler may accidentally compile a component/hook that breaks the Rules of React which can lead to undefined behavior.
For this reason, to adopt the compiler successfully on existing projects, we recommend running it on a small directory in your product code first. You can do this by configuring the compiler to only run on a specific set of directories:
```
const ReactCompilerConfig = {
sources: (filename) => {
return filename.indexOf('src/path/to/dir') !== -1;
},
};
```
When you have more confidence with rolling out the compiler, you can expand coverage to other directories as well and slowly roll it out to your whole app.
#### New projects[Link for New projects]()
If you’re starting a new project, you can enable the compiler on your entire codebase, which is the default behavior.
### Using React Compiler with React 17 or 18[Link for Using React Compiler with React 17 or 18]()
React Compiler works best with React 19 RC. If you are unable to upgrade, you can install the extra `react-compiler-runtime` package which will allow the compiled code to run on versions prior to 19. However, note that the minimum supported version is 17.
Terminal
Copy
npm install react-compiler-runtime@beta
You should also add the correct `target` to your compiler config, where `target` is the major version of React you are targeting:
```
// babel.config.js
const ReactCompilerConfig = {
target: '18' // '17' | '18' | '19'
};
module.exports = function () {
return {
plugins: [
['babel-plugin-react-compiler', ReactCompilerConfig],
],
};
};
```
### Using the compiler on libraries[Link for Using the compiler on libraries]()
React Compiler can also be used to compile libraries. Because React Compiler needs to run on the original source code prior to any code transformations, it is not possible for an application’s build pipeline to compile the libraries they use. Hence, our recommendation is for library maintainers to independently compile and test their libraries with the compiler, and ship compiled code to npm.
Because your code is pre-compiled, users of your library will not need to have the compiler enabled in order to benefit from the automatic memoization applied to your library. If your library targets apps not yet on React 19, specify a minimum [`target` and add `react-compiler-runtime` as a direct dependency](). The runtime package will use the correct implementation of APIs depending on the application’s version, and polyfill the missing APIs if necessary.
Library code can often require more complex patterns and usage of escape hatches. For this reason, we recommend ensuring that you have sufficient testing in order to identify any issues that might arise from using the compiler on your library. If you identify any issues, you can always opt-out the specific components or hooks with the [`'use no memo'` directive]().
Similarly to apps, it is not necessary to fully compile 100% of your components or hooks to see benefits in your library. A good starting point might be to identify the most performance sensitive parts of your library and ensuring that they don’t break the [Rules of React](https://react.dev/reference/rules), which you can use `eslint-plugin-react-compiler` to identify.
## Usage[Link for Usage]()
### Babel[Link for Babel]()
Terminal
Copy
npm install babel-plugin-react-compiler@beta
The compiler includes a Babel plugin which you can use in your build pipeline to run the compiler.
After installing, add it to your Babel config. Please note that it’s critical that the compiler run **first** in the pipeline:
```
// babel.config.js
const ReactCompilerConfig = { /* ... */ };
module.exports = function () {
return {
plugins: [
['babel-plugin-react-compiler', ReactCompilerConfig], // must run first!
// ...
],
};
};
```
`babel-plugin-react-compiler` should run first before other Babel plugins as the compiler requires the input source information for sound analysis.
### Vite[Link for Vite]()
If you use Vite, you can add the plugin to vite-plugin-react:
```
// vite.config.js
const ReactCompilerConfig = { /* ... */ };
export default defineConfig(() => {
return {
plugins: [
react({
babel: {
plugins: [
["babel-plugin-react-compiler", ReactCompilerConfig],
],
},
}),
],
// ...
};
});
```
### Next.js[Link for Next.js]()
Please refer to the [Next.js docs](https://nextjs.org/docs/app/api-reference/next-config-js/reactCompiler) for more information.
### Remix[Link for Remix]()
Install `vite-plugin-babel`, and add the compiler’s Babel plugin to it:
Terminal
Copy
npm install vite-plugin-babel
```
// vite.config.js
import babel from "vite-plugin-babel";
const ReactCompilerConfig = { /* ... */ };
export default defineConfig({
plugins: [
remix({ /* ... */}),
babel({
filter: /\.[jt]sx?$/,
babelConfig: {
presets: ["@babel/preset-typescript"], // if you use TypeScript
plugins: [
["babel-plugin-react-compiler", ReactCompilerConfig],
],
},
}),
],
});
```
### Webpack[Link for Webpack]()
A community Webpack loader is [now available here](https://github.com/SukkaW/react-compiler-webpack).
### Expo[Link for Expo]()
Please refer to [Expo’s docs](https://docs.expo.dev/guides/react-compiler/) to enable and use the React Compiler in Expo apps.
### Metro (React Native)[Link for Metro (React Native)]()
React Native uses Babel via Metro, so refer to the [Usage with Babel]() section for installation instructions.
### Rspack[Link for Rspack]()
Please refer to [Rspack’s docs](https://rspack.dev/guide/tech/react) to enable and use the React Compiler in Rspack apps.
### Rsbuild[Link for Rsbuild]()
Please refer to [Rsbuild’s docs](https://rsbuild.dev/guide/framework/react) to enable and use the React Compiler in Rsbuild apps.
## Troubleshooting[Link for Troubleshooting]()
To report issues, please first create a minimal repro on the [React Compiler Playground](https://playground.react.dev/) and include it in your bug report. You can open issues in the [facebook/react](https://github.com/facebook/react/issues) repo.
You can also provide feedback in the React Compiler Working Group by applying to be a member. Please see [the README for more details on joining](https://github.com/reactwg/react-compiler).
### What does the compiler assume?[Link for What does the compiler assume?]()
React Compiler assumes that your code:
1. Is valid, semantic JavaScript.
2. Tests that nullable/optional values and properties are defined before accessing them (for example, by enabling [`strictNullChecks`](https://www.typescriptlang.org/tsconfig/) if using TypeScript), i.e., `if (object.nullableProperty) { object.nullableProperty.foo }` or with optional-chaining `object.nullableProperty?.foo`.
3. Follows the [Rules of React](https://react.dev/reference/rules).
React Compiler can verify many of the Rules of React statically, and will safely skip compilation when it detects an error. To see the errors we recommend also installing [eslint-plugin-react-compiler](https://www.npmjs.com/package/eslint-plugin-react-compiler).
### How do I know my components have been optimized?[Link for How do I know my components have been optimized?]()
[React DevTools](https://react.dev/learn/react-developer-tools) (v5.0+) and [React Native DevTools](https://reactnative.dev/docs/react-native-devtools) have built-in support for React Compiler and will display a “Memo ✨” badge next to components that have been optimized by the compiler.
### Something is not working after compilation[Link for Something is not working after compilation]()
If you have eslint-plugin-react-compiler installed, the compiler will display any violations of the rules of React in your editor. When it does this, it means that the compiler has skipped over optimizing that component or hook. This is perfectly okay, and the compiler can recover and continue optimizing other components in your codebase. **You don’t have to fix all ESLint violations straight away.** You can address them at your own pace to increase the amount of components and hooks being optimized.
Due to the flexible and dynamic nature of JavaScript however, it’s not possible to comprehensively detect all cases. Bugs and undefined behavior such as infinite loops may occur in those cases.
If your app doesn’t work properly after compilation and you aren’t seeing any ESLint errors, the compiler may be incorrectly compiling your code. To confirm this, try to make the issue go away by aggressively opting out any component or hook you think might be related via the [`"use no memo"` directive]().
```
function SuspiciousComponent() {
"use no memo"; // opts out this component from being compiled by React Compiler
// ...
}
```
### Note
#### `"use no memo"`[Link for this heading]()
`"use no memo"` is a *temporary* escape hatch that lets you opt-out components and hooks from being compiled by the React Compiler. This directive is not meant to be long lived the same way as eg [`"use client"`](https://react.dev/reference/rsc/use-client) is.
It is not recommended to reach for this directive unless it’s strictly necessary. Once you opt-out a component or hook, it is opted-out forever until the directive is removed. This means that even if you fix the code, the compiler will still skip over compiling it unless you remove the directive.
When you make the error go away, confirm that removing the opt out directive makes the issue come back. Then share a bug report with us (you can try to reduce it to a small repro, or if it’s open source code you can also just paste the entire source) using the [React Compiler Playground](https://playground.react.dev) so we can identify and help fix the issue.
### Other issues[Link for Other issues]()
Please see [https://github.com/reactwg/react-compiler/discussions/7](https://github.com/reactwg/react-compiler/discussions/7).
[PreviousReact Developer Tools](https://react.dev/learn/react-developer-tools) |
https://react.dev/learn/react-developer-tools | [Learn React](https://react.dev/learn)
[Installation](https://react.dev/learn/installation)
# React Developer Tools[Link for this heading]()
Use React Developer Tools to inspect React [components](https://react.dev/learn/your-first-component), edit [props](https://react.dev/learn/passing-props-to-a-component) and [state](https://react.dev/learn/state-a-components-memory), and identify performance problems.
### You will learn
- How to install React Developer Tools
## Browser extension[Link for Browser extension]()
The easiest way to debug websites built with React is to install the React Developer Tools browser extension. It is available for several popular browsers:
- [Install for **Chrome**](https://chrome.google.com/webstore/detail/react-developer-tools/fmkadmapgofadopljbjfkapdkoienihi?hl=en)
- [Install for **Firefox**](https://addons.mozilla.org/en-US/firefox/addon/react-devtools/)
- [Install for **Edge**](https://microsoftedge.microsoft.com/addons/detail/react-developer-tools/gpphkfbcpidddadnkolkpfckpihlkkil)
Now, if you visit a website **built with React,** you will see the *Components* and *Profiler* panels.

### Safari and other browsers[Link for Safari and other browsers]()
For other browsers (for example, Safari), install the [`react-devtools`](https://www.npmjs.com/package/react-devtools) npm package:
```
# Yarn
yarn global add react-devtools
# Npm
npm install -g react-devtools
```
Next open the developer tools from the terminal:
```
react-devtools
```
Then connect your website by adding the following `<script>` tag to the beginning of your website’s `<head>`:
```
<html>
<head>
<script src="http://localhost:8097"></script>
```
Reload your website in the browser now to view it in developer tools.

## Mobile (React Native)[Link for Mobile (React Native)]()
To inspect apps built with [React Native](https://reactnative.dev/), you can use [React Native DevTools](https://reactnative.dev/docs/react-native-devtools), the built-in debugger that deeply integrates React Developer Tools. All features work identically to the browser extension, including native element highlighting and selection.
[Learn more about debugging in React Native.](https://reactnative.dev/docs/debugging)
> For versions of React Native earlier than 0.76, please use the standalone build of React DevTools by following the [Safari and other browsers]() guide above.
[PreviousUsing TypeScript](https://react.dev/learn/typescript)
[NextReact Compiler](https://react.dev/learn/react-compiler) |
https://react.dev/learn/importing-and-exporting-components | [Learn React](https://react.dev/learn)
[Describing the UI](https://react.dev/learn/describing-the-ui)
# Importing and Exporting Components[Link for this heading]()
The magic of components lies in their reusability: you can create components that are composed of other components. But as you nest more and more components, it often makes sense to start splitting them into different files. This lets you keep your files easy to scan and reuse components in more places.
### You will learn
- What a root component file is
- How to import and export a component
- When to use default and named imports and exports
- How to import and export multiple components from one file
- How to split components into multiple files
## The root component file[Link for The root component file]()
In [Your First Component](https://react.dev/learn/your-first-component), you made a `Profile` component and a `Gallery` component that renders it:
App.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
function Profile() {
return (
<img
src="https://i.imgur.com/MK3eW3As.jpg"
alt="Katherine Johnson"
/>
);
}
export default function Gallery() {
return (
<section>
<h1>Amazing scientists</h1>
<Profile />
<Profile />
<Profile />
</section>
);
}
```
Show more
These currently live in a **root component file,** named `App.js` in this example. Depending on your setup, your root component could be in another file, though. If you use a framework with file-based routing, such as Next.js, your root component will be different for every page.
## Exporting and importing a component[Link for Exporting and importing a component]()
What if you want to change the landing screen in the future and put a list of science books there? Or place all the profiles somewhere else? It makes sense to move `Gallery` and `Profile` out of the root component file. This will make them more modular and reusable in other files. You can move a component in three steps:
1. **Make** a new JS file to put the components in.
2. **Export** your function component from that file (using either [default](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Statements/export) or [named](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Statements/export) exports).
3. **Import** it in the file where you’ll use the component (using the corresponding technique for importing [default](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Statements/import) or [named](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Statements/import) exports).
Here both `Profile` and `Gallery` have been moved out of `App.js` into a new file called `Gallery.js`. Now you can change `App.js` to import `Gallery` from `Gallery.js`:
App.jsGallery.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import Gallery from './Gallery.js';
export default function App() {
return (
<Gallery />
);
}
```
Notice how this example is broken down into two component files now:
1. `Gallery.js`:
- Defines the `Profile` component which is only used within the same file and is not exported.
- Exports the `Gallery` component as a **default export.**
2. `App.js`:
- Imports `Gallery` as a **default import** from `Gallery.js`.
- Exports the root `App` component as a **default export.**
### Note
You may encounter files that leave off the `.js` file extension like so:
```
import Gallery from './Gallery';
```
Either `'./Gallery.js'` or `'./Gallery'` will work with React, though the former is closer to how [native ES Modules](https://developer.mozilla.org/docs/Web/JavaScript/Guide/Modules) work.
##### Deep Dive
#### Default vs named exports[Link for Default vs named exports]()
Show Details
There are two primary ways to export values with JavaScript: default exports and named exports. So far, our examples have only used default exports. But you can use one or both of them in the same file. **A file can have no more than one *default* export, but it can have as many *named* exports as you like.**

How you export your component dictates how you must import it. You will get an error if you try to import a default export the same way you would a named export! This chart can help you keep track:
SyntaxExport statementImport statementDefault`export default function Button() {}``import Button from './Button.js';`Named`export function Button() {}``import { Button } from './Button.js';`
When you write a *default* import, you can put any name you want after `import`. For example, you could write `import Banana from './Button.js'` instead and it would still provide you with the same default export. In contrast, with named imports, the name has to match on both sides. That’s why they are called *named* imports!
**People often use default exports if the file exports only one component, and use named exports if it exports multiple components and values.** Regardless of which coding style you prefer, always give meaningful names to your component functions and the files that contain them. Components without names, like `export default () => {}`, are discouraged because they make debugging harder.
## Exporting and importing multiple components from the same file[Link for Exporting and importing multiple components from the same file]()
What if you want to show just one `Profile` instead of a gallery? You can export the `Profile` component, too. But `Gallery.js` already has a *default* export, and you can’t have *two* default exports. You could create a new file with a default export, or you could add a *named* export for `Profile`. **A file can only have one default export, but it can have numerous named exports!**
### Note
To reduce the potential confusion between default and named exports, some teams choose to only stick to one style (default or named), or avoid mixing them in a single file. Do what works best for you!
First, **export** `Profile` from `Gallery.js` using a named export (no `default` keyword):
```
export function Profile() {
// ...
}
```
Then, **import** `Profile` from `Gallery.js` to `App.js` using a named import (with the curly braces):
```
import { Profile } from './Gallery.js';
```
Finally, **render** `<Profile />` from the `App` component:
```
export default function App() {
return <Profile />;
}
```
Now `Gallery.js` contains two exports: a default `Gallery` export, and a named `Profile` export. `App.js` imports both of them. Try editing `<Profile />` to `<Gallery />` and back in this example:
App.jsGallery.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import Gallery from './Gallery.js';
import { Profile } from './Gallery.js';
export default function App() {
return (
<Profile />
);
}
```
Now you’re using a mix of default and named exports:
- `Gallery.js`:
- Exports the `Profile` component as a **named export called `Profile`.**
- Exports the `Gallery` component as a **default export.**
- `App.js`:
- Imports `Profile` as a **named import called `Profile`** from `Gallery.js`.
- Imports `Gallery` as a **default import** from `Gallery.js`.
- Exports the root `App` component as a **default export.**
## Recap[Link for Recap]()
On this page you learned:
- What a root component file is
- How to import and export a component
- When and how to use default and named imports and exports
- How to export multiple components from the same file
## Try out some challenges[Link for Try out some challenges]()
#### Challenge 1 of 1: Split the components further[Link for this heading]()
Currently, `Gallery.js` exports both `Profile` and `Gallery`, which is a bit confusing.
Move the `Profile` component to its own `Profile.js`, and then change the `App` component to render both `<Profile />` and `<Gallery />` one after another.
You may use either a default or a named export for `Profile`, but make sure that you use the corresponding import syntax in both `App.js` and `Gallery.js`! You can refer to the table from the deep dive above:
SyntaxExport statementImport statementDefault`export default function Button() {}``import Button from './Button.js';`Named`export function Button() {}``import { Button } from './Button.js';`
App.jsGallery.jsProfile.js
Gallery.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
// Move me to Profile.js!
export function Profile() {
return (
<img
src="https://i.imgur.com/QIrZWGIs.jpg"
alt="Alan L. Hart"
/>
);
}
export default function Gallery() {
return (
<section>
<h1>Amazing scientists</h1>
<Profile />
<Profile />
<Profile />
</section>
);
}
```
Show more
After you get it working with one kind of exports, make it work with the other kind.
Show hint Show solution
[PreviousYour First Component](https://react.dev/learn/your-first-component)
[NextWriting Markup with JSX](https://react.dev/learn/writing-markup-with-jsx) |
https://react.dev/learn/rendering-lists | [Learn React](https://react.dev/learn)
[Describing the UI](https://react.dev/learn/describing-the-ui)
# Rendering Lists[Link for this heading]()
You will often want to display multiple similar components from a collection of data. You can use the [JavaScript array methods](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Array) to manipulate an array of data. On this page, you’ll use [`filter()`](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Array/filter) and [`map()`](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Array/map) with React to filter and transform your array of data into an array of components.
### You will learn
- How to render components from an array using JavaScript’s `map()`
- How to render only specific components using JavaScript’s `filter()`
- When and why to use React keys
## Rendering data from arrays[Link for Rendering data from arrays]()
Say that you have a list of content.
```
<ul>
<li>Creola Katherine Johnson: mathematician</li>
<li>Mario José Molina-Pasquel Henríquez: chemist</li>
<li>Mohammad Abdus Salam: physicist</li>
<li>Percy Lavon Julian: chemist</li>
<li>Subrahmanyan Chandrasekhar: astrophysicist</li>
</ul>
```
The only difference among those list items is their contents, their data. You will often need to show several instances of the same component using different data when building interfaces: from lists of comments to galleries of profile images. In these situations, you can store that data in JavaScript objects and arrays and use methods like [`map()`](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Array/map) and [`filter()`](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Array/filter) to render lists of components from them.
Here’s a short example of how to generate a list of items from an array:
1. **Move** the data into an array:
```
const people = [
'Creola Katherine Johnson: mathematician',
'Mario José Molina-Pasquel Henríquez: chemist',
'Mohammad Abdus Salam: physicist',
'Percy Lavon Julian: chemist',
'Subrahmanyan Chandrasekhar: astrophysicist'
];
```
2. **Map** the `people` members into a new array of JSX nodes, `listItems`:
```
const listItems = people.map(person => <li>{person}</li>);
```
3. **Return** `listItems` from your component wrapped in a `<ul>`:
```
return <ul>{listItems}</ul>;
```
Here is the result:
App.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
const people = [
'Creola Katherine Johnson: mathematician',
'Mario José Molina-Pasquel Henríquez: chemist',
'Mohammad Abdus Salam: physicist',
'Percy Lavon Julian: chemist',
'Subrahmanyan Chandrasekhar: astrophysicist'
];
export default function List() {
const listItems = people.map(person =>
<li>{person}</li>
);
return <ul>{listItems}</ul>;
}
```
Notice the sandbox above displays a console error:
Console
Warning: Each child in a list should have a unique “key” prop.
You’ll learn how to fix this error later on this page. Before we get to that, let’s add some structure to your data.
## Filtering arrays of items[Link for Filtering arrays of items]()
This data can be structured even more.
```
const people = [{
id: 0,
name: 'Creola Katherine Johnson',
profession: 'mathematician',
}, {
id: 1,
name: 'Mario José Molina-Pasquel Henríquez',
profession: 'chemist',
}, {
id: 2,
name: 'Mohammad Abdus Salam',
profession: 'physicist',
}, {
id: 3,
name: 'Percy Lavon Julian',
profession: 'chemist',
}, {
id: 4,
name: 'Subrahmanyan Chandrasekhar',
profession: 'astrophysicist',
}];
```
Let’s say you want a way to only show people whose profession is `'chemist'`. You can use JavaScript’s `filter()` method to return just those people. This method takes an array of items, passes them through a “test” (a function that returns `true` or `false`), and returns a new array of only those items that passed the test (returned `true`).
You only want the items where `profession` is `'chemist'`. The “test” function for this looks like `(person) => person.profession === 'chemist'`. Here’s how to put it together:
1. **Create** a new array of just “chemist” people, `chemists`, by calling `filter()` on the `people` filtering by `person.profession === 'chemist'`:
```
const chemists = people.filter(person =>
person.profession === 'chemist'
);
```
2. Now **map** over `chemists`:
```
const listItems = chemists.map(person =>
<li>
<img
src={getImageUrl(person)}
alt={person.name}
/>
<p>
<b>{person.name}:</b>
{' ' + person.profession + ' '}
known for {person.accomplishment}
</p>
</li>
);
```
3. Lastly, **return** the `listItems` from your component:
```
return <ul>{listItems}</ul>;
```
App.jsdata.jsutils.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import { people } from './data.js';
import { getImageUrl } from './utils.js';
export default function List() {
const chemists = people.filter(person =>
person.profession === 'chemist'
);
const listItems = chemists.map(person =>
<li>
<img
src={getImageUrl(person)}
alt={person.name}
/>
<p>
<b>{person.name}:</b>
{' ' + person.profession + ' '}
known for {person.accomplishment}
</p>
</li>
);
return <ul>{listItems}</ul>;
}
```
Show more
### Pitfall
Arrow functions implicitly return the expression right after `=>`, so you didn’t need a `return` statement:
```
const listItems = chemists.map(person =>
<li>...</li> // Implicit return!
);
```
However, **you must write `return` explicitly if your `=>` is followed by a `{` curly brace!**
```
const listItems = chemists.map(person => { // Curly brace
return <li>...</li>;
});
```
Arrow functions containing `=> {` are said to have a [“block body”.](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Functions/Arrow_functions) They let you write more than a single line of code, but you *have to* write a `return` statement yourself. If you forget it, nothing gets returned!
## Keeping list items in order with `key`[Link for this heading]()
Notice that all the sandboxes above show an error in the console:
Console
Warning: Each child in a list should have a unique “key” prop.
You need to give each array item a `key` — a string or a number that uniquely identifies it among other items in that array:
```
<li key={person.id}>...</li>
```
### Note
JSX elements directly inside a `map()` call always need keys!
Keys tell React which array item each component corresponds to, so that it can match them up later. This becomes important if your array items can move (e.g. due to sorting), get inserted, or get deleted. A well-chosen `key` helps React infer what exactly has happened, and make the correct updates to the DOM tree.
Rather than generating keys on the fly, you should include them in your data:
App.jsdata.jsutils.js
data.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
export const people = [{
id: 0, // Used in JSX as a key
name: 'Creola Katherine Johnson',
profession: 'mathematician',
accomplishment: 'spaceflight calculations',
imageId: 'MK3eW3A'
}, {
id: 1, // Used in JSX as a key
name: 'Mario José Molina-Pasquel Henríquez',
profession: 'chemist',
accomplishment: 'discovery of Arctic ozone hole',
imageId: 'mynHUSa'
}, {
id: 2, // Used in JSX as a key
name: 'Mohammad Abdus Salam',
profession: 'physicist',
accomplishment: 'electromagnetism theory',
imageId: 'bE7W1ji'
}, {
id: 3, // Used in JSX as a key
name: 'Percy Lavon Julian',
profession: 'chemist',
accomplishment: 'pioneering cortisone drugs, steroids and birth control pills',
imageId: 'IOjWm71'
}, {
id: 4, // Used in JSX as a key
name: 'Subrahmanyan Chandrasekhar',
profession: 'astrophysicist',
accomplishment: 'white dwarf star mass calculations',
imageId: 'lrWQx8l'
}];
```
Show more
##### Deep Dive
#### Displaying several DOM nodes for each list item[Link for Displaying several DOM nodes for each list item]()
Show Details
What do you do when each item needs to render not one, but several DOM nodes?
The short [`<>...</>` Fragment](https://react.dev/reference/react/Fragment) syntax won’t let you pass a key, so you need to either group them into a single `<div>`, or use the slightly longer and [more explicit `<Fragment>` syntax:](https://react.dev/reference/react/Fragment)
```
import { Fragment } from 'react';
// ...
const listItems = people.map(person =>
<Fragment key={person.id}>
<h1>{person.name}</h1>
<p>{person.bio}</p>
</Fragment>
);
```
Fragments disappear from the DOM, so this will produce a flat list of `<h1>`, `<p>`, `<h1>`, `<p>`, and so on.
### Where to get your `key`[Link for this heading]()
Different sources of data provide different sources of keys:
- **Data from a database:** If your data is coming from a database, you can use the database keys/IDs, which are unique by nature.
- **Locally generated data:** If your data is generated and persisted locally (e.g. notes in a note-taking app), use an incrementing counter, [`crypto.randomUUID()`](https://developer.mozilla.org/en-US/docs/Web/API/Crypto/randomUUID) or a package like [`uuid`](https://www.npmjs.com/package/uuid) when creating items.
### Rules of keys[Link for Rules of keys]()
- **Keys must be unique among siblings.** However, it’s okay to use the same keys for JSX nodes in *different* arrays.
- **Keys must not change** or that defeats their purpose! Don’t generate them while rendering.
### Why does React need keys?[Link for Why does React need keys?]()
Imagine that files on your desktop didn’t have names. Instead, you’d refer to them by their order — the first file, the second file, and so on. You could get used to it, but once you delete a file, it would get confusing. The second file would become the first file, the third file would be the second file, and so on.
File names in a folder and JSX keys in an array serve a similar purpose. They let us uniquely identify an item between its siblings. A well-chosen key provides more information than the position within the array. Even if the *position* changes due to reordering, the `key` lets React identify the item throughout its lifetime.
### Pitfall
You might be tempted to use an item’s index in the array as its key. In fact, that’s what React will use if you don’t specify a `key` at all. But the order in which you render items will change over time if an item is inserted, deleted, or if the array gets reordered. Index as a key often leads to subtle and confusing bugs.
Similarly, do not generate keys on the fly, e.g. with `key={Math.random()}`. This will cause keys to never match up between renders, leading to all your components and DOM being recreated every time. Not only is this slow, but it will also lose any user input inside the list items. Instead, use a stable ID based on the data.
Note that your components won’t receive `key` as a prop. It’s only used as a hint by React itself. If your component needs an ID, you have to pass it as a separate prop: `<Profile key={id} userId={id} />`.
## Recap[Link for Recap]()
On this page you learned:
- How to move data out of components and into data structures like arrays and objects.
- How to generate sets of similar components with JavaScript’s `map()`.
- How to create arrays of filtered items with JavaScript’s `filter()`.
- Why and how to set `key` on each component in a collection so React can keep track of each of them even if their position or data changes.
## Try out some challenges[Link for Try out some challenges]()
1\. Splitting a list in two 2. Nested lists in one component 3. Extracting a list item component 4. List with a separator
#### Challenge 1 of 4: Splitting a list in two[Link for this heading]()
This example shows a list of all people.
Change it to show two separate lists one after another: **Chemists** and **Everyone Else.** Like previously, you can determine whether a person is a chemist by checking if `person.profession === 'chemist'`.
App.jsdata.jsutils.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import { people } from './data.js';
import { getImageUrl } from './utils.js';
export default function List() {
const listItems = people.map(person =>
<li key={person.id}>
<img
src={getImageUrl(person)}
alt={person.name}
/>
<p>
<b>{person.name}:</b>
{' ' + person.profession + ' '}
known for {person.accomplishment}
</p>
</li>
);
return (
<article>
<h1>Scientists</h1>
<ul>{listItems}</ul>
</article>
);
}
```
Show more
Show solutionNext Challenge
[PreviousConditional Rendering](https://react.dev/learn/conditional-rendering)
[NextKeeping Components Pure](https://react.dev/learn/keeping-components-pure) |
https://react.dev/learn/keeping-components-pure | [Learn React](https://react.dev/learn)
[Describing the UI](https://react.dev/learn/describing-the-ui)
# Keeping Components Pure[Link for this heading]()
Some JavaScript functions are *pure.* Pure functions only perform a calculation and nothing more. By strictly only writing your components as pure functions, you can avoid an entire class of baffling bugs and unpredictable behavior as your codebase grows. To get these benefits, though, there are a few rules you must follow.
### You will learn
- What purity is and how it helps you avoid bugs
- How to keep components pure by keeping changes out of the render phase
- How to use Strict Mode to find mistakes in your components
## Purity: Components as formulas[Link for Purity: Components as formulas]()
In computer science (and especially the world of functional programming), [a pure function](https://wikipedia.org/wiki/Pure_function) is a function with the following characteristics:
- **It minds its own business.** It does not change any objects or variables that existed before it was called.
- **Same inputs, same output.** Given the same inputs, a pure function should always return the same result.
You might already be familiar with one example of pure functions: formulas in math.
Consider this math formula: y = 2x.
If x = 2 then y = 4. Always.
If x = 3 then y = 6. Always.
If x = 3, y won’t sometimes be 9 or –1 or 2.5 depending on the time of day or the state of the stock market.
If y = 2x and x = 3, y will *always* be 6.
If we made this into a JavaScript function, it would look like this:
```
function double(number) {
return 2 * number;
}
```
In the above example, `double` is a **pure function.** If you pass it `3`, it will return `6`. Always.
React is designed around this concept. **React assumes that every component you write is a pure function.** This means that React components you write must always return the same JSX given the same inputs:
App.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
function Recipe({ drinkers }) {
return (
<ol>
<li>Boil {drinkers} cups of water.</li>
<li>Add {drinkers} spoons of tea and {0.5 * drinkers} spoons of spice.</li>
<li>Add {0.5 * drinkers} cups of milk to boil and sugar to taste.</li>
</ol>
);
}
export default function App() {
return (
<section>
<h1>Spiced Chai Recipe</h1>
<h2>For two</h2>
<Recipe drinkers={2} />
<h2>For a gathering</h2>
<Recipe drinkers={4} />
</section>
);
}
```
Show more
When you pass `drinkers={2}` to `Recipe`, it will return JSX containing `2 cups of water`. Always.
If you pass `drinkers={4}`, it will return JSX containing `4 cups of water`. Always.
Just like a math formula.
You could think of your components as recipes: if you follow them and don’t introduce new ingredients during the cooking process, you will get the same dish every time. That “dish” is the JSX that the component serves to React to [render.](https://react.dev/learn/render-and-commit)

Illustrated by [Rachel Lee Nabors](https://nearestnabors.com/)
## Side Effects: (un)intended consequences[Link for Side Effects: (un)intended consequences]()
React’s rendering process must always be pure. Components should only *return* their JSX, and not *change* any objects or variables that existed before rendering—that would make them impure!
Here is a component that breaks this rule:
App.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
let guest = 0;
function Cup() {
// Bad: changing a preexisting variable!
guest = guest + 1;
return <h2>Tea cup for guest #{guest}</h2>;
}
export default function TeaSet() {
return (
<>
<Cup />
<Cup />
<Cup />
</>
);
}
```
Show more
This component is reading and writing a `guest` variable declared outside of it. This means that **calling this component multiple times will produce different JSX!** And what’s more, if *other* components read `guest`, they will produce different JSX, too, depending on when they were rendered! That’s not predictable.
Going back to our formula y = 2x, now even if x = 2, we cannot trust that y = 4. Our tests could fail, our users would be baffled, planes would fall out of the sky—you can see how this would lead to confusing bugs!
You can fix this component by [passing `guest` as a prop instead](https://react.dev/learn/passing-props-to-a-component):
App.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
function Cup({ guest }) {
return <h2>Tea cup for guest #{guest}</h2>;
}
export default function TeaSet() {
return (
<>
<Cup guest={1} />
<Cup guest={2} />
<Cup guest={3} />
</>
);
}
```
Now your component is pure, as the JSX it returns only depends on the `guest` prop.
In general, you should not expect your components to be rendered in any particular order. It doesn’t matter if you call y = 2x before or after y = 5x: both formulas will resolve independently of each other. In the same way, each component should only “think for itself”, and not attempt to coordinate with or depend upon others during rendering. Rendering is like a school exam: each component should calculate JSX on their own!
##### Deep Dive
#### Detecting impure calculations with StrictMode[Link for Detecting impure calculations with StrictMode]()
Show Details
Although you might not have used them all yet, in React there are three kinds of inputs that you can read while rendering: [props](https://react.dev/learn/passing-props-to-a-component), [state](https://react.dev/learn/state-a-components-memory), and [context.](https://react.dev/learn/passing-data-deeply-with-context) You should always treat these inputs as read-only.
When you want to *change* something in response to user input, you should [set state](https://react.dev/learn/state-a-components-memory) instead of writing to a variable. You should never change preexisting variables or objects while your component is rendering.
React offers a “Strict Mode” in which it calls each component’s function twice during development. **By calling the component functions twice, Strict Mode helps find components that break these rules.**
Notice how the original example displayed “Guest #2”, “Guest #4”, and “Guest #6” instead of “Guest #1”, “Guest #2”, and “Guest #3”. The original function was impure, so calling it twice broke it. But the fixed pure version works even if the function is called twice every time. **Pure functions only calculate, so calling them twice won’t change anything**—just like calling `double(2)` twice doesn’t change what’s returned, and solving y = 2x twice doesn’t change what y is. Same inputs, same outputs. Always.
Strict Mode has no effect in production, so it won’t slow down the app for your users. To opt into Strict Mode, you can wrap your root component into `<React.StrictMode>`. Some frameworks do this by default.
### Local mutation: Your component’s little secret[Link for Local mutation: Your component’s little secret]()
In the above example, the problem was that the component changed a *preexisting* variable while rendering. This is often called a **“mutation”** to make it sound a bit scarier. Pure functions don’t mutate variables outside of the function’s scope or objects that were created before the call—that makes them impure!
However, **it’s completely fine to change variables and objects that you’ve *just* created while rendering.** In this example, you create an `[]` array, assign it to a `cups` variable, and then `push` a dozen cups into it:
App.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
function Cup({ guest }) {
return <h2>Tea cup for guest #{guest}</h2>;
}
export default function TeaGathering() {
let cups = [];
for (let i = 1; i <= 12; i++) {
cups.push(<Cup key={i} guest={i} />);
}
return cups;
}
```
If the `cups` variable or the `[]` array were created outside the `TeaGathering` function, this would be a huge problem! You would be changing a *preexisting* object by pushing items into that array.
However, it’s fine because you’ve created them *during the same render*, inside `TeaGathering`. No code outside of `TeaGathering` will ever know that this happened. This is called **“local mutation”**—it’s like your component’s little secret.
## Where you *can* cause side effects[Link for this heading]()
While functional programming relies heavily on purity, at some point, somewhere, *something* has to change. That’s kind of the point of programming! These changes—updating the screen, starting an animation, changing the data—are called **side effects.** They’re things that happen *“on the side”*, not during rendering.
In React, **side effects usually belong inside [event handlers.](https://react.dev/learn/responding-to-events)** Event handlers are functions that React runs when you perform some action—for example, when you click a button. Even though event handlers are defined *inside* your component, they don’t run *during* rendering! **So event handlers don’t need to be pure.**
If you’ve exhausted all other options and can’t find the right event handler for your side effect, you can still attach it to your returned JSX with a [`useEffect`](https://react.dev/reference/react/useEffect) call in your component. This tells React to execute it later, after rendering, when side effects are allowed. **However, this approach should be your last resort.**
When possible, try to express your logic with rendering alone. You’ll be surprised how far this can take you!
##### Deep Dive
#### Why does React care about purity?[Link for Why does React care about purity?]()
Show Details
Writing pure functions takes some habit and discipline. But it also unlocks marvelous opportunities:
- Your components could run in a different environment—for example, on the server! Since they return the same result for the same inputs, one component can serve many user requests.
- You can improve performance by [skipping rendering](https://react.dev/reference/react/memo) components whose inputs have not changed. This is safe because pure functions always return the same results, so they are safe to cache.
- If some data changes in the middle of rendering a deep component tree, React can restart rendering without wasting time to finish the outdated render. Purity makes it safe to stop calculating at any time.
Every new React feature we’re building takes advantage of purity. From data fetching to animations to performance, keeping components pure unlocks the power of the React paradigm.
## Recap[Link for Recap]()
- A component must be pure, meaning:
- **It minds its own business.** It should not change any objects or variables that existed before rendering.
- **Same inputs, same output.** Given the same inputs, a component should always return the same JSX.
- Rendering can happen at any time, so components should not depend on each others’ rendering sequence.
- You should not mutate any of the inputs that your components use for rendering. That includes props, state, and context. To update the screen, [“set” state](https://react.dev/learn/state-a-components-memory) instead of mutating preexisting objects.
- Strive to express your component’s logic in the JSX you return. When you need to “change things”, you’ll usually want to do it in an event handler. As a last resort, you can `useEffect`.
- Writing pure functions takes a bit of practice, but it unlocks the power of React’s paradigm.
## Try out some challenges[Link for Try out some challenges]()
1\. Fix a broken clock 2. Fix a broken profile 3. Fix a broken story tray
#### Challenge 1 of 3: Fix a broken clock[Link for this heading]()
This component tries to set the `<h1>`’s CSS class to `"night"` during the time from midnight to six hours in the morning, and `"day"` at all other times. However, it doesn’t work. Can you fix this component?
You can verify whether your solution works by temporarily changing the computer’s timezone. When the current time is between midnight and six in the morning, the clock should have inverted colors!
Clock.js
Clock.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
export default function Clock({ time }) {
let hours = time.getHours();
if (hours >= 0 && hours <= 6) {
document.getElementById('time').className = 'night';
} else {
document.getElementById('time').className = 'day';
}
return (
<h1 id="time">
{time.toLocaleTimeString()}
</h1>
);
}
```
Show hint Show solution
Next Challenge
[PreviousRendering Lists](https://react.dev/learn/rendering-lists)
[NextYour UI as a Tree](https://react.dev/learn/understanding-your-ui-as-a-tree) |
https://react.dev/learn/state-a-components-memory | [Learn React](https://react.dev/learn)
[Adding Interactivity](https://react.dev/learn/adding-interactivity)
# State: A Component's Memory[Link for this heading]()
Components often need to change what’s on the screen as a result of an interaction. Typing into the form should update the input field, clicking “next” on an image carousel should change which image is displayed, clicking “buy” should put a product in the shopping cart. Components need to “remember” things: the current input value, the current image, the shopping cart. In React, this kind of component-specific memory is called *state*.
### You will learn
- How to add a state variable with the [`useState`](https://react.dev/reference/react/useState) Hook
- What pair of values the `useState` Hook returns
- How to add more than one state variable
- Why state is called local
## When a regular variable isn’t enough[Link for When a regular variable isn’t enough]()
Here’s a component that renders a sculpture image. Clicking the “Next” button should show the next sculpture by changing the `index` to `1`, then `2`, and so on. However, this **won’t work** (you can try it!):
App.jsdata.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import { sculptureList } from './data.js';
export default function Gallery() {
let index = 0;
function handleClick() {
index = index + 1;
}
let sculpture = sculptureList[index];
return (
<>
<button onClick={handleClick}>
Next
</button>
<h2>
<i>{sculpture.name} </i>
by {sculpture.artist}
</h2>
<h3>
({index + 1} of {sculptureList.length})
</h3>
<img
src={sculpture.url}
alt={sculpture.alt}
/>
<p>
{sculpture.description}
</p>
</>
);
}
```
Show more
The `handleClick` event handler is updating a local variable, `index`. But two things prevent that change from being visible:
1. **Local variables don’t persist between renders.** When React renders this component a second time, it renders it from scratch—it doesn’t consider any changes to the local variables.
2. **Changes to local variables won’t trigger renders.** React doesn’t realize it needs to render the component again with the new data.
To update a component with new data, two things need to happen:
1. **Retain** the data between renders.
2. **Trigger** React to render the component with new data (re-rendering).
The [`useState`](https://react.dev/reference/react/useState) Hook provides those two things:
1. A **state variable** to retain the data between renders.
2. A **state setter function** to update the variable and trigger React to render the component again.
## Adding a state variable[Link for Adding a state variable]()
To add a state variable, import `useState` from React at the top of the file:
```
import { useState } from 'react';
```
Then, replace this line:
```
let index = 0;
```
with
```
const [index, setIndex] = useState(0);
```
`index` is a state variable and `setIndex` is the setter function.
> The `[` and `]` syntax here is called [array destructuring](https://javascript.info/destructuring-assignment) and it lets you read values from an array. The array returned by `useState` always has exactly two items.
This is how they work together in `handleClick`:
```
function handleClick() {
setIndex(index + 1);
}
```
Now clicking the “Next” button switches the current sculpture:
App.jsdata.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import { useState } from 'react';
import { sculptureList } from './data.js';
export default function Gallery() {
const [index, setIndex] = useState(0);
function handleClick() {
setIndex(index + 1);
}
let sculpture = sculptureList[index];
return (
<>
<button onClick={handleClick}>
Next
</button>
<h2>
<i>{sculpture.name} </i>
by {sculpture.artist}
</h2>
<h3>
({index + 1} of {sculptureList.length})
</h3>
<img
src={sculpture.url}
alt={sculpture.alt}
/>
<p>
{sculpture.description}
</p>
</>
);
}
```
Show more
### Meet your first Hook[Link for Meet your first Hook]()
In React, `useState`, as well as any other function starting with “`use`”, is called a Hook.
*Hooks* are special functions that are only available while React is [rendering](https://react.dev/learn/render-and-commit) (which we’ll get into in more detail on the next page). They let you “hook into” different React features.
State is just one of those features, but you will meet the other Hooks later.
### Pitfall
**Hooks—functions starting with `use`—can only be called at the top level of your components or [your own Hooks.](https://react.dev/learn/reusing-logic-with-custom-hooks)** You can’t call Hooks inside conditions, loops, or other nested functions. Hooks are functions, but it’s helpful to think of them as unconditional declarations about your component’s needs. You “use” React features at the top of your component similar to how you “import” modules at the top of your file.
### Anatomy of `useState`[Link for this heading]()
When you call [`useState`](https://react.dev/reference/react/useState), you are telling React that you want this component to remember something:
```
const [index, setIndex] = useState(0);
```
In this case, you want React to remember `index`.
### Note
The convention is to name this pair like `const [something, setSomething]`. You could name it anything you like, but conventions make things easier to understand across projects.
The only argument to `useState` is the **initial value** of your state variable. In this example, the `index`’s initial value is set to `0` with `useState(0)`.
Every time your component renders, `useState` gives you an array containing two values:
1. The **state variable** (`index`) with the value you stored.
2. The **state setter function** (`setIndex`) which can update the state variable and trigger React to render the component again.
Here’s how that happens in action:
```
const [index, setIndex] = useState(0);
```
1. **Your component renders the first time.** Because you passed `0` to `useState` as the initial value for `index`, it will return `[0, setIndex]`. React remembers `0` is the latest state value.
2. **You update the state.** When a user clicks the button, it calls `setIndex(index + 1)`. `index` is `0`, so it’s `setIndex(1)`. This tells React to remember `index` is `1` now and triggers another render.
3. **Your component’s second render.** React still sees `useState(0)`, but because React *remembers* that you set `index` to `1`, it returns `[1, setIndex]` instead.
4. And so on!
## Giving a component multiple state variables[Link for Giving a component multiple state variables]()
You can have as many state variables of as many types as you like in one component. This component has two state variables, a number `index` and a boolean `showMore` that’s toggled when you click “Show details”:
App.jsdata.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import { useState } from 'react';
import { sculptureList } from './data.js';
export default function Gallery() {
const [index, setIndex] = useState(0);
const [showMore, setShowMore] = useState(false);
function handleNextClick() {
setIndex(index + 1);
}
function handleMoreClick() {
setShowMore(!showMore);
}
let sculpture = sculptureList[index];
return (
<>
<button onClick={handleNextClick}>
Next
</button>
<h2>
<i>{sculpture.name} </i>
by {sculpture.artist}
</h2>
<h3>
({index + 1} of {sculptureList.length})
</h3>
<button onClick={handleMoreClick}>
{showMore ? 'Hide' : 'Show'} details
</button>
{showMore && <p>{sculpture.description}</p>}
<img
src={sculpture.url}
alt={sculpture.alt}
/>
</>
);
}
```
Show more
It is a good idea to have multiple state variables if their state is unrelated, like `index` and `showMore` in this example. But if you find that you often change two state variables together, it might be easier to combine them into one. For example, if you have a form with many fields, it’s more convenient to have a single state variable that holds an object than state variable per field. Read [Choosing the State Structure](https://react.dev/learn/choosing-the-state-structure) for more tips.
##### Deep Dive
#### How does React know which state to return?[Link for How does React know which state to return?]()
Show Details
You might have noticed that the `useState` call does not receive any information about *which* state variable it refers to. There is no “identifier” that is passed to `useState`, so how does it know which of the state variables to return? Does it rely on some magic like parsing your functions? The answer is no.
Instead, to enable their concise syntax, Hooks **rely on a stable call order on every render of the same component.** This works well in practice because if you follow the rule above (“only call Hooks at the top level”), Hooks will always be called in the same order. Additionally, a [linter plugin](https://www.npmjs.com/package/eslint-plugin-react-hooks) catches most mistakes.
Internally, React holds an array of state pairs for every component. It also maintains the current pair index, which is set to `0` before rendering. Each time you call `useState`, React gives you the next state pair and increments the index. You can read more about this mechanism in [React Hooks: Not Magic, Just Arrays.](https://medium.com/@ryardley/react-hooks-not-magic-just-arrays-cd4f1857236e)
This example **doesn’t use React** but it gives you an idea of how `useState` works internally:
index.jsindex.html
index.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
let componentHooks = [];
let currentHookIndex = 0;
// How useState works inside React (simplified).
function useState(initialState) {
let pair = componentHooks[currentHookIndex];
if (pair) {
// This is not the first render,
// so the state pair already exists.
// Return it and prepare for next Hook call.
currentHookIndex++;
return pair;
}
// This is the first time we're rendering,
// so create a state pair and store it.
pair = [initialState, setState];
function setState(nextState) {
// When the user requests a state change,
// put the new value into the pair.
pair[0] = nextState;
updateDOM();
}
// Store the pair for future renders
// and prepare for the next Hook call.
componentHooks[currentHookIndex] = pair;
currentHookIndex++;
return pair;
}
function Gallery() {
// Each useState() call will get the next pair.
const [index, setIndex] = useState(0);
const [showMore, setShowMore] = useState(false);
function handleNextClick() {
setIndex(index + 1);
}
function handleMoreClick() {
setShowMore(!showMore);
}
let sculpture = sculptureList[index];
// This example doesn't use React, so
// return an output object instead of JSX.
return {
onNextClick: handleNextClick,
onMoreClick: handleMoreClick,
header: `${sculpture.name} by ${sculpture.artist}`,
counter: `${index + 1} of ${sculptureList.length}`,
more: `${showMore ? 'Hide' : 'Show'} details`,
description: showMore ? sculpture.description : null,
imageSrc: sculpture.url,
imageAlt: sculpture.alt
};
}
function updateDOM() {
// Reset the current Hook index
// before rendering the component.
currentHookIndex = 0;
let output = Gallery();
// Update the DOM to match the output.
// This is the part React does for you.
nextButton.onclick = output.onNextClick;
header.textContent = output.header;
moreButton.onclick = output.onMoreClick;
moreButton.textContent = output.more;
image.src = output.imageSrc;
image.alt = output.imageAlt;
if (output.description !== null) {
description.textContent = output.description;
description.style.display = '';
} else {
description.style.display = 'none';
}
}
let nextButton = document.getElementById('nextButton');
let header = document.getElementById('header');
let moreButton = document.getElementById('moreButton');
let description = document.getElementById('description');
let image = document.getElementById('image');
let sculptureList = [{
name: 'Homenaje a la Neurocirugía',
artist: 'Marta Colvin Andrade',
description: 'Although Colvin is predominantly known for abstract themes that allude to pre-Hispanic symbols, this gigantic sculpture, an homage to neurosurgery, is one of her most recognizable public art pieces.',
url: 'https://i.imgur.com/Mx7dA2Y.jpg',
alt: 'A bronze statue of two crossed hands delicately holding a human brain in their fingertips.'
}, {
name: 'Floralis Genérica',
artist: 'Eduardo Catalano',
description: 'This enormous (75 ft. or 23m) silver flower is located in Buenos Aires. It is designed to move, closing its petals in the evening or when strong winds blow and opening them in the morning.',
url: 'https://i.imgur.com/ZF6s192m.jpg',
alt: 'A gigantic metallic flower sculpture with reflective mirror-like petals and strong stamens.'
}, {
name: 'Eternal Presence',
artist: 'John Woodrow Wilson',
description: 'Wilson was known for his preoccupation with equality, social justice, as well as the essential and spiritual qualities of humankind. This massive (7ft. or 2,13m) bronze represents what he described as "a symbolic Black presence infused with a sense of universal humanity."',
url: 'https://i.imgur.com/aTtVpES.jpg',
alt: 'The sculpture depicting a human head seems ever-present and solemn. It radiates calm and serenity.'
}, {
name: 'Moai',
artist: 'Unknown Artist',
description: 'Located on the Easter Island, there are 1,000 moai, or extant monumental statues, created by the early Rapa Nui people, which some believe represented deified ancestors.',
url: 'https://i.imgur.com/RCwLEoQm.jpg',
alt: 'Three monumental stone busts with the heads that are disproportionately large with somber faces.'
}, {
name: 'Blue Nana',
artist: 'Niki de Saint Phalle',
description: 'The Nanas are triumphant creatures, symbols of femininity and maternity. Initially, Saint Phalle used fabric and found objects for the Nanas, and later on introduced polyester to achieve a more vibrant effect.',
url: 'https://i.imgur.com/Sd1AgUOm.jpg',
alt: 'A large mosaic sculpture of a whimsical dancing female figure in a colorful costume emanating joy.'
}, {
name: 'Ultimate Form',
artist: 'Barbara Hepworth',
description: 'This abstract bronze sculpture is a part of The Family of Man series located at Yorkshire Sculpture Park. Hepworth chose not to create literal representations of the world but developed abstract forms inspired by people and landscapes.',
url: 'https://i.imgur.com/2heNQDcm.jpg',
alt: 'A tall sculpture made of three elements stacked on each other reminding of a human figure.'
}, {
name: 'Cavaliere',
artist: 'Lamidi Olonade Fakeye',
description: "Descended from four generations of woodcarvers, Fakeye's work blended traditional and contemporary Yoruba themes.",
url: 'https://i.imgur.com/wIdGuZwm.png',
alt: 'An intricate wood sculpture of a warrior with a focused face on a horse adorned with patterns.'
}, {
name: 'Big Bellies',
artist: 'Alina Szapocznikow',
description: "Szapocznikow is known for her sculptures of the fragmented body as a metaphor for the fragility and impermanence of youth and beauty. This sculpture depicts two very realistic large bellies stacked on top of each other, each around five feet (1,5m) tall.",
url: 'https://i.imgur.com/AlHTAdDm.jpg',
alt: 'The sculpture reminds a cascade of folds, quite different from bellies in classical sculptures.'
}, {
name: 'Terracotta Army',
artist: 'Unknown Artist',
description: 'The Terracotta Army is a collection of terracotta sculptures depicting the armies of Qin Shi Huang, the first Emperor of China. The army consisted of more than 8,000 soldiers, 130 chariots with 520 horses, and 150 cavalry horses.',
url: 'https://i.imgur.com/HMFmH6m.jpg',
alt: '12 terracotta sculptures of solemn warriors, each with a unique facial expression and armor.'
}, {
name: 'Lunar Landscape',
artist: 'Louise Nevelson',
description: 'Nevelson was known for scavenging objects from New York City debris, which she would later assemble into monumental constructions. In this one, she used disparate parts like a bedpost, juggling pin, and seat fragment, nailing and gluing them into boxes that reflect the influence of Cubism’s geometric abstraction of space and form.',
url: 'https://i.imgur.com/rN7hY6om.jpg',
alt: 'A black matte sculpture where the individual elements are initially indistinguishable.'
}, {
name: 'Aureole',
artist: 'Ranjani Shettar',
description: 'Shettar merges the traditional and the modern, the natural and the industrial. Her art focuses on the relationship between man and nature. Her work was described as compelling both abstractly and figuratively, gravity defying, and a "fine synthesis of unlikely materials."',
url: 'https://i.imgur.com/okTpbHhm.jpg',
alt: 'A pale wire-like sculpture mounted on concrete wall and descending on the floor. It appears light.'
}, {
name: 'Hippos',
artist: 'Taipei Zoo',
description: 'The Taipei Zoo commissioned a Hippo Square featuring submerged hippos at play.',
url: 'https://i.imgur.com/6o5Vuyu.jpg',
alt: 'A group of bronze hippo sculptures emerging from the sett sidewalk as if they were swimming.'
}];
// Make UI match the initial state.
updateDOM();
```
Show more
You don’t have to understand it to use React, but you might find this a helpful mental model.
## State is isolated and private[Link for State is isolated and private]()
State is local to a component instance on the screen. In other words, **if you render the same component twice, each copy will have completely isolated state!** Changing one of them will not affect the other.
In this example, the `Gallery` component from earlier is rendered twice with no changes to its logic. Try clicking the buttons inside each of the galleries. Notice that their state is independent:
App.jsGallery.jsdata.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import Gallery from './Gallery.js';
export default function Page() {
return (
<div className="Page">
<Gallery />
<Gallery />
</div>
);
}
```
This is what makes state different from regular variables that you might declare at the top of your module. State is not tied to a particular function call or a place in the code, but it’s “local” to the specific place on the screen. You rendered two `<Gallery />` components, so their state is stored separately.
Also notice how the `Page` component doesn’t “know” anything about the `Gallery` state or even whether it has any. Unlike props, **state is fully private to the component declaring it.** The parent component can’t change it. This lets you add state to any component or remove it without impacting the rest of the components.
What if you wanted both galleries to keep their states in sync? The right way to do it in React is to *remove* state from child components and add it to their closest shared parent. The next few pages will focus on organizing state of a single component, but we will return to this topic in [Sharing State Between Components.](https://react.dev/learn/sharing-state-between-components)
## Recap[Link for Recap]()
- Use a state variable when a component needs to “remember” some information between renders.
- State variables are declared by calling the `useState` Hook.
- Hooks are special functions that start with `use`. They let you “hook into” React features like state.
- Hooks might remind you of imports: they need to be called unconditionally. Calling Hooks, including `useState`, is only valid at the top level of a component or another Hook.
- The `useState` Hook returns a pair of values: the current state and the function to update it.
- You can have more than one state variable. Internally, React matches them up by their order.
- State is private to the component. If you render it in two places, each copy gets its own state.
## Try out some challenges[Link for Try out some challenges]()
1\. Complete the gallery 2. Fix stuck form inputs 3. Fix a crash 4. Remove unnecessary state
#### Challenge 1 of 4: Complete the gallery[Link for this heading]()
When you press “Next” on the last sculpture, the code crashes. Fix the logic to prevent the crash. You may do this by adding extra logic to event handler or by disabling the button when the action is not possible.
After fixing the crash, add a “Previous” button that shows the previous sculpture. It shouldn’t crash on the first sculpture.
App.jsdata.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import { useState } from 'react';
import { sculptureList } from './data.js';
export default function Gallery() {
const [index, setIndex] = useState(0);
const [showMore, setShowMore] = useState(false);
function handleNextClick() {
setIndex(index + 1);
}
function handleMoreClick() {
setShowMore(!showMore);
}
let sculpture = sculptureList[index];
return (
<>
<button onClick={handleNextClick}>
Next
</button>
<h2>
<i>{sculpture.name} </i>
by {sculpture.artist}
</h2>
<h3>
({index + 1} of {sculptureList.length})
</h3>
<button onClick={handleMoreClick}>
{showMore ? 'Hide' : 'Show'} details
</button>
{showMore && <p>{sculpture.description}</p>}
<img
src={sculpture.url}
alt={sculpture.alt}
/>
</>
);
}
```
Show more
Show solutionNext Challenge
[PreviousResponding to Events](https://react.dev/learn/responding-to-events)
[NextRender and Commit](https://react.dev/learn/render-and-commit) |
https://react.dev/learn/updating-objects-in-state | [Learn React](https://react.dev/learn)
[Adding Interactivity](https://react.dev/learn/adding-interactivity)
# Updating Objects in State[Link for this heading]()
State can hold any kind of JavaScript value, including objects. But you shouldn’t change objects that you hold in the React state directly. Instead, when you want to update an object, you need to create a new one (or make a copy of an existing one), and then set the state to use that copy.
### You will learn
- How to correctly update an object in React state
- How to update a nested object without mutating it
- What immutability is, and how not to break it
- How to make object copying less repetitive with Immer
## What’s a mutation?[Link for What’s a mutation?]()
You can store any kind of JavaScript value in state.
```
const [x, setX] = useState(0);
```
So far you’ve been working with numbers, strings, and booleans. These kinds of JavaScript values are “immutable”, meaning unchangeable or “read-only”. You can trigger a re-render to *replace* a value:
```
setX(5);
```
The `x` state changed from `0` to `5`, but the *number `0` itself* did not change. It’s not possible to make any changes to the built-in primitive values like numbers, strings, and booleans in JavaScript.
Now consider an object in state:
```
const [position, setPosition] = useState({ x: 0, y: 0 });
```
Technically, it is possible to change the contents of *the object itself*. **This is called a mutation:**
```
position.x = 5;
```
However, although objects in React state are technically mutable, you should treat them **as if** they were immutable—like numbers, booleans, and strings. Instead of mutating them, you should always replace them.
## Treat state as read-only[Link for Treat state as read-only]()
In other words, you should **treat any JavaScript object that you put into state as read-only.**
This example holds an object in state to represent the current pointer position. The red dot is supposed to move when you touch or move the cursor over the preview area. But the dot stays in the initial position:
App.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import { useState } from 'react';
export default function MovingDot() {
const [position, setPosition] = useState({
x: 0,
y: 0
});
return (
<div
onPointerMove={e => {
position.x = e.clientX;
position.y = e.clientY;
}}
style={{
position: 'relative',
width: '100vw',
height: '100vh',
}}>
<div style={{
position: 'absolute',
backgroundColor: 'red',
borderRadius: '50%',
transform: `translate(${position.x}px, ${position.y}px)`,
left: -10,
top: -10,
width: 20,
height: 20,
}} />
</div>
);
}
```
Show more
The problem is with this bit of code.
```
onPointerMove={e => {
position.x = e.clientX;
position.y = e.clientY;
}}
```
This code modifies the object assigned to `position` from [the previous render.](https://react.dev/learn/state-as-a-snapshot) But without using the state setting function, React has no idea that object has changed. So React does not do anything in response. It’s like trying to change the order after you’ve already eaten the meal. While mutating state can work in some cases, we don’t recommend it. You should treat the state value you have access to in a render as read-only.
To actually [trigger a re-render](https://react.dev/learn/state-as-a-snapshot) in this case, **create a *new* object and pass it to the state setting function:**
```
onPointerMove={e => {
setPosition({
x: e.clientX,
y: e.clientY
});
}}
```
With `setPosition`, you’re telling React:
- Replace `position` with this new object
- And render this component again
Notice how the red dot now follows your pointer when you touch or hover over the preview area:
App.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import { useState } from 'react';
export default function MovingDot() {
const [position, setPosition] = useState({
x: 0,
y: 0
});
return (
<div
onPointerMove={e => {
setPosition({
x: e.clientX,
y: e.clientY
});
}}
style={{
position: 'relative',
width: '100vw',
height: '100vh',
}}>
<div style={{
position: 'absolute',
backgroundColor: 'red',
borderRadius: '50%',
transform: `translate(${position.x}px, ${position.y}px)`,
left: -10,
top: -10,
width: 20,
height: 20,
}} />
</div>
);
}
```
Show more
##### Deep Dive
#### Local mutation is fine[Link for Local mutation is fine]()
Show Details
Code like this is a problem because it modifies an *existing* object in state:
```
position.x = e.clientX;
position.y = e.clientY;
```
But code like this is **absolutely fine** because you’re mutating a fresh object you have *just created*:
```
const nextPosition = {};
nextPosition.x = e.clientX;
nextPosition.y = e.clientY;
setPosition(nextPosition);
```
In fact, it is completely equivalent to writing this:
```
setPosition({
x: e.clientX,
y: e.clientY
});
```
Mutation is only a problem when you change *existing* objects that are already in state. Mutating an object you’ve just created is okay because *no other code references it yet.* Changing it isn’t going to accidentally impact something that depends on it. This is called a “local mutation”. You can even do local mutation [while rendering.](https://react.dev/learn/keeping-components-pure) Very convenient and completely okay!
## Copying objects with the spread syntax[Link for Copying objects with the spread syntax]()
In the previous example, the `position` object is always created fresh from the current cursor position. But often, you will want to include *existing* data as a part of the new object you’re creating. For example, you may want to update *only one* field in a form, but keep the previous values for all other fields.
These input fields don’t work because the `onChange` handlers mutate the state:
App.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import { useState } from 'react';
export default function Form() {
const [person, setPerson] = useState({
firstName: 'Barbara',
lastName: 'Hepworth',
email: '[email protected]'
});
function handleFirstNameChange(e) {
person.firstName = e.target.value;
}
function handleLastNameChange(e) {
person.lastName = e.target.value;
}
function handleEmailChange(e) {
person.email = e.target.value;
}
return (
<>
<label>
First name:
<input
value={person.firstName}
onChange={handleFirstNameChange}
/>
</label>
<label>
Last name:
<input
value={person.lastName}
onChange={handleLastNameChange}
/>
</label>
<label>
Email:
<input
value={person.email}
onChange={handleEmailChange}
/>
</label>
<p>
{person.firstName}{' '}
{person.lastName}{' '}
({person.email})
</p>
</>
);
}
```
Show more
For example, this line mutates the state from a past render:
```
person.firstName = e.target.value;
```
The reliable way to get the behavior you’re looking for is to create a new object and pass it to `setPerson`. But here, you want to also **copy the existing data into it** because only one of the fields has changed:
```
setPerson({
firstName: e.target.value, // New first name from the input
lastName: person.lastName,
email: person.email
});
```
You can use the `...` [object spread](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Operators/Spread_syntax) syntax so that you don’t need to copy every property separately.
```
setPerson({
...person, // Copy the old fields
firstName: e.target.value // But override this one
});
```
Now the form works!
Notice how you didn’t declare a separate state variable for each input field. For large forms, keeping all data grouped in an object is very convenient—as long as you update it correctly!
App.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import { useState } from 'react';
export default function Form() {
const [person, setPerson] = useState({
firstName: 'Barbara',
lastName: 'Hepworth',
email: '[email protected]'
});
function handleFirstNameChange(e) {
setPerson({
...person,
firstName: e.target.value
});
}
function handleLastNameChange(e) {
setPerson({
...person,
lastName: e.target.value
});
}
function handleEmailChange(e) {
setPerson({
...person,
email: e.target.value
});
}
return (
<>
<label>
First name:
<input
value={person.firstName}
onChange={handleFirstNameChange}
/>
</label>
<label>
Last name:
<input
value={person.lastName}
onChange={handleLastNameChange}
/>
</label>
<label>
Email:
<input
value={person.email}
onChange={handleEmailChange}
/>
</label>
<p>
{person.firstName}{' '}
{person.lastName}{' '}
({person.email})
</p>
</>
);
}
```
Show more
Note that the `...` spread syntax is “shallow”—it only copies things one level deep. This makes it fast, but it also means that if you want to update a nested property, you’ll have to use it more than once.
##### Deep Dive
#### Using a single event handler for multiple fields[Link for Using a single event handler for multiple fields]()
Show Details
You can also use the `[` and `]` braces inside your object definition to specify a property with a dynamic name. Here is the same example, but with a single event handler instead of three different ones:
App.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import { useState } from 'react';
export default function Form() {
const [person, setPerson] = useState({
firstName: 'Barbara',
lastName: 'Hepworth',
email: '[email protected]'
});
function handleChange(e) {
setPerson({
...person,
[e.target.name]: e.target.value
});
}
return (
<>
<label>
First name:
<input
name="firstName"
value={person.firstName}
onChange={handleChange}
/>
</label>
<label>
Last name:
<input
name="lastName"
value={person.lastName}
onChange={handleChange}
/>
</label>
<label>
Email:
<input
name="email"
value={person.email}
onChange={handleChange}
/>
</label>
<p>
{person.firstName}{' '}
{person.lastName}{' '}
({person.email})
</p>
</>
);
}
```
Show more
Here, `e.target.name` refers to the `name` property given to the `<input>` DOM element.
## Updating a nested object[Link for Updating a nested object]()
Consider a nested object structure like this:
```
const [person, setPerson] = useState({
name: 'Niki de Saint Phalle',
artwork: {
title: 'Blue Nana',
city: 'Hamburg',
image: 'https://i.imgur.com/Sd1AgUOm.jpg',
}
});
```
If you wanted to update `person.artwork.city`, it’s clear how to do it with mutation:
```
person.artwork.city = 'New Delhi';
```
But in React, you treat state as immutable! In order to change `city`, you would first need to produce the new `artwork` object (pre-populated with data from the previous one), and then produce the new `person` object which points at the new `artwork`:
```
const nextArtwork = { ...person.artwork, city: 'New Delhi' };
const nextPerson = { ...person, artwork: nextArtwork };
setPerson(nextPerson);
```
Or, written as a single function call:
```
setPerson({
...person, // Copy other fields
artwork: { // but replace the artwork
...person.artwork, // with the same one
city: 'New Delhi' // but in New Delhi!
}
});
```
This gets a bit wordy, but it works fine for many cases:
App.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import { useState } from 'react';
export default function Form() {
const [person, setPerson] = useState({
name: 'Niki de Saint Phalle',
artwork: {
title: 'Blue Nana',
city: 'Hamburg',
image: 'https://i.imgur.com/Sd1AgUOm.jpg',
}
});
function handleNameChange(e) {
setPerson({
...person,
name: e.target.value
});
}
function handleTitleChange(e) {
setPerson({
...person,
artwork: {
...person.artwork,
title: e.target.value
}
});
}
function handleCityChange(e) {
setPerson({
...person,
artwork: {
...person.artwork,
city: e.target.value
}
});
}
function handleImageChange(e) {
setPerson({
...person,
artwork: {
...person.artwork,
image: e.target.value
}
});
}
return (
<>
<label>
Name:
<input
value={person.name}
onChange={handleNameChange}
/>
</label>
<label>
Title:
<input
value={person.artwork.title}
onChange={handleTitleChange}
/>
</label>
<label>
City:
<input
value={person.artwork.city}
onChange={handleCityChange}
/>
</label>
<label>
Image:
<input
value={person.artwork.image}
onChange={handleImageChange}
/>
</label>
<p>
<i>{person.artwork.title}</i>
{' by '}
{person.name}
<br />
(located in {person.artwork.city})
</p>
<img
src={person.artwork.image}
alt={person.artwork.title}
/>
</>
);
}
```
Show more
##### Deep Dive
#### Objects are not really nested[Link for Objects are not really nested]()
Show Details
An object like this appears “nested” in code:
```
let obj = {
name: 'Niki de Saint Phalle',
artwork: {
title: 'Blue Nana',
city: 'Hamburg',
image: 'https://i.imgur.com/Sd1AgUOm.jpg',
}
};
```
However, “nesting” is an inaccurate way to think about how objects behave. When the code executes, there is no such thing as a “nested” object. You are really looking at two different objects:
```
let obj1 = {
title: 'Blue Nana',
city: 'Hamburg',
image: 'https://i.imgur.com/Sd1AgUOm.jpg',
};
let obj2 = {
name: 'Niki de Saint Phalle',
artwork: obj1
};
```
The `obj1` object is not “inside” `obj2`. For example, `obj3` could “point” at `obj1` too:
```
let obj1 = {
title: 'Blue Nana',
city: 'Hamburg',
image: 'https://i.imgur.com/Sd1AgUOm.jpg',
};
let obj2 = {
name: 'Niki de Saint Phalle',
artwork: obj1
};
let obj3 = {
name: 'Copycat',
artwork: obj1
};
```
If you were to mutate `obj3.artwork.city`, it would affect both `obj2.artwork.city` and `obj1.city`. This is because `obj3.artwork`, `obj2.artwork`, and `obj1` are the same object. This is difficult to see when you think of objects as “nested”. Instead, they are separate objects “pointing” at each other with properties.
### Write concise update logic with Immer[Link for Write concise update logic with Immer]()
If your state is deeply nested, you might want to consider [flattening it.](https://react.dev/learn/choosing-the-state-structure) But, if you don’t want to change your state structure, you might prefer a shortcut to nested spreads. [Immer](https://github.com/immerjs/use-immer) is a popular library that lets you write using the convenient but mutating syntax and takes care of producing the copies for you. With Immer, the code you write looks like you are “breaking the rules” and mutating an object:
```
updatePerson(draft => {
draft.artwork.city = 'Lagos';
});
```
But unlike a regular mutation, it doesn’t overwrite the past state!
##### Deep Dive
#### How does Immer work?[Link for How does Immer work?]()
Show Details
The `draft` provided by Immer is a special type of object, called a [Proxy](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Proxy), that “records” what you do with it. This is why you can mutate it freely as much as you like! Under the hood, Immer figures out which parts of the `draft` have been changed, and produces a completely new object that contains your edits.
To try Immer:
1. Run `npm install use-immer` to add Immer as a dependency
2. Then replace `import { useState } from 'react'` with `import { useImmer } from 'use-immer'`
Here is the above example converted to Immer:
package.jsonApp.js
package.json
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
{
"dependencies": {
"immer": "1.7.3",
"react": "latest",
"react-dom": "latest",
"react-scripts": "latest",
"use-immer": "0.5.1"
},
"scripts": {
"start": "react-scripts start",
"build": "react-scripts build",
"test": "react-scripts test --env=jsdom",
"eject": "react-scripts eject"
},
"devDependencies": {}
}
```
Notice how much more concise the event handlers have become. You can mix and match `useState` and `useImmer` in a single component as much as you like. Immer is a great way to keep the update handlers concise, especially if there’s nesting in your state, and copying objects leads to repetitive code.
##### Deep Dive
#### Why is mutating state not recommended in React?[Link for Why is mutating state not recommended in React?]()
Show Details
There are a few reasons:
- **Debugging:** If you use `console.log` and don’t mutate state, your past logs won’t get clobbered by the more recent state changes. So you can clearly see how state has changed between renders.
- **Optimizations:** Common React [optimization strategies](https://react.dev/reference/react/memo) rely on skipping work if previous props or state are the same as the next ones. If you never mutate state, it is very fast to check whether there were any changes. If `prevObj === obj`, you can be sure that nothing could have changed inside of it.
- **New Features:** The new React features we’re building rely on state being [treated like a snapshot.](https://react.dev/learn/state-as-a-snapshot) If you’re mutating past versions of state, that may prevent you from using the new features.
- **Requirement Changes:** Some application features, like implementing Undo/Redo, showing a history of changes, or letting the user reset a form to earlier values, are easier to do when nothing is mutated. This is because you can keep past copies of state in memory, and reuse them when appropriate. If you start with a mutative approach, features like this can be difficult to add later on.
- **Simpler Implementation:** Because React does not rely on mutation, it does not need to do anything special with your objects. It does not need to hijack their properties, always wrap them into Proxies, or do other work at initialization as many “reactive” solutions do. This is also why React lets you put any object into state—no matter how large—without additional performance or correctness pitfalls.
In practice, you can often “get away” with mutating state in React, but we strongly advise you not to do that so that you can use new React features developed with this approach in mind. Future contributors and perhaps even your future self will thank you!
## Recap[Link for Recap]()
- Treat all state in React as immutable.
- When you store objects in state, mutating them will not trigger renders and will change the state in previous render “snapshots”.
- Instead of mutating an object, create a *new* version of it, and trigger a re-render by setting state to it.
- You can use the `{...obj, something: 'newValue'}` object spread syntax to create copies of objects.
- Spread syntax is shallow: it only copies one level deep.
- To update a nested object, you need to create copies all the way up from the place you’re updating.
- To reduce repetitive copying code, use Immer.
## Try out some challenges[Link for Try out some challenges]()
1\. Fix incorrect state updates 2. Find and fix the mutation 3. Update an object with Immer
#### Challenge 1 of 3: Fix incorrect state updates[Link for this heading]()
This form has a few bugs. Click the button that increases the score a few times. Notice that it does not increase. Then edit the first name, and notice that the score has suddenly “caught up” with your changes. Finally, edit the last name, and notice that the score has disappeared completely.
Your task is to fix all of these bugs. As you fix them, explain why each of them happens.
App.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import { useState } from 'react';
export default function Scoreboard() {
const [player, setPlayer] = useState({
firstName: 'Ranjani',
lastName: 'Shettar',
score: 10,
});
function handlePlusClick() {
player.score++;
}
function handleFirstNameChange(e) {
setPlayer({
...player,
firstName: e.target.value,
});
}
function handleLastNameChange(e) {
setPlayer({
lastName: e.target.value
});
}
return (
<>
<label>
Score: <b>{player.score}</b>
{' '}
<button onClick={handlePlusClick}>
+1
</button>
</label>
<label>
First name:
<input
value={player.firstName}
onChange={handleFirstNameChange}
/>
</label>
<label>
Last name:
<input
value={player.lastName}
onChange={handleLastNameChange}
/>
</label>
</>
);
}
```
Show more
Show solutionNext Challenge
[PreviousQueueing a Series of State Updates](https://react.dev/learn/queueing-a-series-of-state-updates)
[NextUpdating Arrays in State](https://react.dev/learn/updating-arrays-in-state) |
https://react.dev/learn/passing-props-to-a-component | [Learn React](https://react.dev/learn)
[Describing the UI](https://react.dev/learn/describing-the-ui)
# Passing Props to a Component[Link for this heading]()
React components use *props* to communicate with each other. Every parent component can pass some information to its child components by giving them props. Props might remind you of HTML attributes, but you can pass any JavaScript value through them, including objects, arrays, and functions.
### You will learn
- How to pass props to a component
- How to read props from a component
- How to specify default values for props
- How to pass some JSX to a component
- How props change over time
## Familiar props[Link for Familiar props]()
Props are the information that you pass to a JSX tag. For example, `className`, `src`, `alt`, `width`, and `height` are some of the props you can pass to an `<img>`:
App.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
function Avatar() {
return (
<img
className="avatar"
src="https://i.imgur.com/1bX5QH6.jpg"
alt="Lin Lanying"
width={100}
height={100}
/>
);
}
export default function Profile() {
return (
<Avatar />
);
}
```
Show more
The props you can pass to an `<img>` tag are predefined (ReactDOM conforms to [the HTML standard](https://www.w3.org/TR/html52/semantics-embedded-content.html)). But you can pass any props to *your own* components, such as `<Avatar>`, to customize them. Here’s how!
## Passing props to a component[Link for Passing props to a component]()
In this code, the `Profile` component isn’t passing any props to its child component, `Avatar`:
```
export default function Profile() {
return (
<Avatar />
);
}
```
You can give `Avatar` some props in two steps.
### Step 1: Pass props to the child component[Link for Step 1: Pass props to the child component]()
First, pass some props to `Avatar`. For example, let’s pass two props: `person` (an object), and `size` (a number):
```
export default function Profile() {
return (
<Avatar
person={{ name: 'Lin Lanying', imageId: '1bX5QH6' }}
size={100}
/>
);
}
```
### Note
If double curly braces after `person=` confuse you, recall [they’re merely an object](https://react.dev/learn/javascript-in-jsx-with-curly-braces) inside the JSX curlies.
Now you can read these props inside the `Avatar` component.
### Step 2: Read props inside the child component[Link for Step 2: Read props inside the child component]()
You can read these props by listing their names `person, size` separated by the commas inside `({` and `})` directly after `function Avatar`. This lets you use them inside the `Avatar` code, like you would with a variable.
```
function Avatar({ person, size }) {
// person and size are available here
}
```
Add some logic to `Avatar` that uses the `person` and `size` props for rendering, and you’re done.
Now you can configure `Avatar` to render in many different ways with different props. Try tweaking the values!
App.jsutils.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import { getImageUrl } from './utils.js';
function Avatar({ person, size }) {
return (
<img
className="avatar"
src={getImageUrl(person)}
alt={person.name}
width={size}
height={size}
/>
);
}
export default function Profile() {
return (
<div>
<Avatar
size={100}
person={{
name: 'Katsuko Saruhashi',
imageId: 'YfeOqp2'
}}
/>
<Avatar
size={80}
person={{
name: 'Aklilu Lemma',
imageId: 'OKS67lh'
}}
/>
<Avatar
size={50}
person={{
name: 'Lin Lanying',
imageId: '1bX5QH6'
}}
/>
</div>
);
}
```
Show more
Props let you think about parent and child components independently. For example, you can change the `person` or the `size` props inside `Profile` without having to think about how `Avatar` uses them. Similarly, you can change how the `Avatar` uses these props, without looking at the `Profile`.
You can think of props like “knobs” that you can adjust. They serve the same role as arguments serve for functions—in fact, props *are* the only argument to your component! React component functions accept a single argument, a `props` object:
```
function Avatar(props) {
let person = props.person;
let size = props.size;
// ...
}
```
Usually you don’t need the whole `props` object itself, so you destructure it into individual props.
### Pitfall
**Don’t miss the pair of `{` and `}` curlies** inside of `(` and `)` when declaring props:
```
function Avatar({ person, size }) {
// ...
}
```
This syntax is called [“destructuring”](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Operators/Destructuring_assignment) and is equivalent to reading properties from a function parameter:
```
function Avatar(props) {
let person = props.person;
let size = props.size;
// ...
}
```
## Specifying a default value for a prop[Link for Specifying a default value for a prop]()
If you want to give a prop a default value to fall back on when no value is specified, you can do it with the destructuring by putting `=` and the default value right after the parameter:
```
function Avatar({ person, size = 100 }) {
// ...
}
```
Now, if `<Avatar person={...} />` is rendered with no `size` prop, the `size` will be set to `100`.
The default value is only used if the `size` prop is missing or if you pass `size={undefined}`. But if you pass `size={null}` or `size={0}`, the default value will **not** be used.
## Forwarding props with the JSX spread syntax[Link for Forwarding props with the JSX spread syntax]()
Sometimes, passing props gets very repetitive:
```
function Profile({ person, size, isSepia, thickBorder }) {
return (
<div className="card">
<Avatar
person={person}
size={size}
isSepia={isSepia}
thickBorder={thickBorder}
/>
</div>
);
}
```
There’s nothing wrong with repetitive code—it can be more legible. But at times you may value conciseness. Some components forward all of their props to their children, like how this `Profile` does with `Avatar`. Because they don’t use any of their props directly, it can make sense to use a more concise “spread” syntax:
```
function Profile(props) {
return (
<div className="card">
<Avatar {...props} />
</div>
);
}
```
This forwards all of `Profile`’s props to the `Avatar` without listing each of their names.
**Use spread syntax with restraint.** If you’re using it in every other component, something is wrong. Often, it indicates that you should split your components and pass children as JSX. More on that next!
## Passing JSX as children[Link for Passing JSX as children]()
It is common to nest built-in browser tags:
```
<div>
<img />
</div>
```
Sometimes you’ll want to nest your own components the same way:
```
<Card>
<Avatar />
</Card>
```
When you nest content inside a JSX tag, the parent component will receive that content in a prop called `children`. For example, the `Card` component below will receive a `children` prop set to `<Avatar />` and render it in a wrapper div:
App.jsAvatar.jsutils.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import Avatar from './Avatar.js';
function Card({ children }) {
return (
<div className="card">
{children}
</div>
);
}
export default function Profile() {
return (
<Card>
<Avatar
size={100}
person={{
name: 'Katsuko Saruhashi',
imageId: 'YfeOqp2'
}}
/>
</Card>
);
}
```
Show more
Try replacing the `<Avatar>` inside `<Card>` with some text to see how the `Card` component can wrap any nested content. It doesn’t need to “know” what’s being rendered inside of it. You will see this flexible pattern in many places.
You can think of a component with a `children` prop as having a “hole” that can be “filled in” by its parent components with arbitrary JSX. You will often use the `children` prop for visual wrappers: panels, grids, etc.

Illustrated by [Rachel Lee Nabors](https://nearestnabors.com/)
## How props change over time[Link for How props change over time]()
The `Clock` component below receives two props from its parent component: `color` and `time`. (The parent component’s code is omitted because it uses [state](https://react.dev/learn/state-a-components-memory), which we won’t dive into just yet.)
Try changing the color in the select box below:
Clock.js
Clock.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
export default function Clock({ color, time }) {
return (
<h1 style={{ color: color }}>
{time}
</h1>
);
}
```
This example illustrates that **a component may receive different props over time.** Props are not always static! Here, the `time` prop changes every second, and the `color` prop changes when you select another color. Props reflect a component’s data at any point in time, rather than only in the beginning.
However, props are [immutable](https://en.wikipedia.org/wiki/Immutable_object)—a term from computer science meaning “unchangeable”. When a component needs to change its props (for example, in response to a user interaction or new data), it will have to “ask” its parent component to pass it *different props*—a new object! Its old props will then be cast aside, and eventually the JavaScript engine will reclaim the memory taken by them.
**Don’t try to “change props”.** When you need to respond to the user input (like changing the selected color), you will need to “set state”, which you can learn about in [State: A Component’s Memory.](https://react.dev/learn/state-a-components-memory)
## Recap[Link for Recap]()
- To pass props, add them to the JSX, just like you would with HTML attributes.
- To read props, use the `function Avatar({ person, size })` destructuring syntax.
- You can specify a default value like `size = 100`, which is used for missing and `undefined` props.
- You can forward all props with `<Avatar {...props} />` JSX spread syntax, but don’t overuse it!
- Nested JSX like `<Card><Avatar /></Card>` will appear as `Card` component’s `children` prop.
- Props are read-only snapshots in time: every render receives a new version of props.
- You can’t change props. When you need interactivity, you’ll need to set state.
## Try out some challenges[Link for Try out some challenges]()
1\. Extract a component 2. Adjust the image size based on a prop 3. Passing JSX in a `children` prop
#### Challenge 1 of 3: Extract a component[Link for this heading]()
This `Gallery` component contains some very similar markup for two profiles. Extract a `Profile` component out of it to reduce the duplication. You’ll need to choose what props to pass to it.
App.jsutils.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import { getImageUrl } from './utils.js';
export default function Gallery() {
return (
<div>
<h1>Notable Scientists</h1>
<section className="profile">
<h2>Maria Skłodowska-Curie</h2>
<img
className="avatar"
src={getImageUrl('szV5sdG')}
alt="Maria Skłodowska-Curie"
width={70}
height={70}
/>
<ul>
<li>
<b>Profession: </b>
physicist and chemist
</li>
<li>
<b>Awards: 4 </b>
(Nobel Prize in Physics, Nobel Prize in Chemistry, Davy Medal, Matteucci Medal)
</li>
<li>
<b>Discovered: </b>
polonium (chemical element)
</li>
</ul>
</section>
<section className="profile">
<h2>Katsuko Saruhashi</h2>
<img
className="avatar"
src={getImageUrl('YfeOqp2')}
alt="Katsuko Saruhashi"
width={70}
height={70}
/>
<ul>
<li>
<b>Profession: </b>
geochemist
</li>
<li>
<b>Awards: 2 </b>
(Miyake Prize for geochemistry, Tanaka Prize)
</li>
<li>
<b>Discovered: </b>
a method for measuring carbon dioxide in seawater
</li>
</ul>
</section>
</div>
);
}
```
Show more
Show hint Show solution
Next Challenge
[PreviousJavaScript in JSX with Curly Braces](https://react.dev/learn/javascript-in-jsx-with-curly-braces)
[NextConditional Rendering](https://react.dev/learn/conditional-rendering) |
https://react.dev/learn/render-and-commit | [Learn React](https://react.dev/learn)
[Adding Interactivity](https://react.dev/learn/adding-interactivity)
# Render and Commit[Link for this heading]()
Before your components are displayed on screen, they must be rendered by React. Understanding the steps in this process will help you think about how your code executes and explain its behavior.
### You will learn
- What rendering means in React
- When and why React renders a component
- The steps involved in displaying a component on screen
- Why rendering does not always produce a DOM update
Imagine that your components are cooks in the kitchen, assembling tasty dishes from ingredients. In this scenario, React is the waiter who puts in requests from customers and brings them their orders. This process of requesting and serving UI has three steps:
1. **Triggering** a render (delivering the guest’s order to the kitchen)
2. **Rendering** the component (preparing the order in the kitchen)
3. **Committing** to the DOM (placing the order on the table)
<!--THE END-->
1. 
Trigger
2. 
Render
3. 
Commit
Illustrated by [Rachel Lee Nabors](https://nearestnabors.com/)
## Step 1: Trigger a render[Link for Step 1: Trigger a render]()
There are two reasons for a component to render:
1. It’s the component’s **initial render.**
2. The component’s (or one of its ancestors’) **state has been updated.**
### Initial render[Link for Initial render]()
When your app starts, you need to trigger the initial render. Frameworks and sandboxes sometimes hide this code, but it’s done by calling [`createRoot`](https://react.dev/reference/react-dom/client/createRoot) with the target DOM node, and then calling its `render` method with your component:
index.jsImage.js
index.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import Image from './Image.js';
import { createRoot } from 'react-dom/client';
const root = createRoot(document.getElementById('root'))
root.render(<Image />);
```
Try commenting out the `root.render()` call and see the component disappear!
### Re-renders when state updates[Link for Re-renders when state updates]()
Once the component has been initially rendered, you can trigger further renders by updating its state with the [`set` function.](https://react.dev/reference/react/useState) Updating your component’s state automatically queues a render. (You can imagine these as a restaurant guest ordering tea, dessert, and all sorts of things after putting in their first order, depending on the state of their thirst or hunger.)
1. 
State update...
2. 
...triggers...
3. 
...render!
Illustrated by [Rachel Lee Nabors](https://nearestnabors.com/)
## Step 2: React renders your components[Link for Step 2: React renders your components]()
After you trigger a render, React calls your components to figure out what to display on screen. **“Rendering” is React calling your components.**
- **On initial render,** React will call the root component.
- **For subsequent renders,** React will call the function component whose state update triggered the render.
This process is recursive: if the updated component returns some other component, React will render *that* component next, and if that component also returns something, it will render *that* component next, and so on. The process will continue until there are no more nested components and React knows exactly what should be displayed on screen.
In the following example, React will call `Gallery()` and `Image()` several times:
index.jsGallery.js
Gallery.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
export default function Gallery() {
return (
<section>
<h1>Inspiring Sculptures</h1>
<Image />
<Image />
<Image />
</section>
);
}
function Image() {
return (
<img
src="https://i.imgur.com/ZF6s192.jpg"
alt="'Floralis Genérica' by Eduardo Catalano: a gigantic metallic flower sculpture with reflective petals"
/>
);
}
```
Show more
- **During the initial render,** React will [create the DOM nodes](https://developer.mozilla.org/docs/Web/API/Document/createElement) for `<section>`, `<h1>`, and three `<img>` tags.
- **During a re-render,** React will calculate which of their properties, if any, have changed since the previous render. It won’t do anything with that information until the next step, the commit phase.
### Pitfall
Rendering must always be a [pure calculation](https://react.dev/learn/keeping-components-pure):
- **Same inputs, same output.** Given the same inputs, a component should always return the same JSX. (When someone orders a salad with tomatoes, they should not receive a salad with onions!)
- **It minds its own business.** It should not change any objects or variables that existed before rendering. (One order should not change anyone else’s order.)
Otherwise, you can encounter confusing bugs and unpredictable behavior as your codebase grows in complexity. When developing in “Strict Mode”, React calls each component’s function twice, which can help surface mistakes caused by impure functions.
##### Deep Dive
#### Optimizing performance[Link for Optimizing performance]()
Show Details
The default behavior of rendering all components nested within the updated component is not optimal for performance if the updated component is very high in the tree. If you run into a performance issue, there are several opt-in ways to solve it described in the [Performance](https://reactjs.org/docs/optimizing-performance.html) section. **Don’t optimize prematurely!**
## Step 3: React commits changes to the DOM[Link for Step 3: React commits changes to the DOM]()
After rendering (calling) your components, React will modify the DOM.
- **For the initial render,** React will use the [`appendChild()`](https://developer.mozilla.org/docs/Web/API/Node/appendChild) DOM API to put all the DOM nodes it has created on screen.
- **For re-renders,** React will apply the minimal necessary operations (calculated while rendering!) to make the DOM match the latest rendering output.
**React only changes the DOM nodes if there’s a difference between renders.** For example, here is a component that re-renders with different props passed from its parent every second. Notice how you can add some text into the `<input>`, updating its `value`, but the text doesn’t disappear when the component re-renders:
Clock.js
Clock.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
export default function Clock({ time }) {
return (
<>
<h1>{time}</h1>
<input />
</>
);
}
```
This works because during this last step, React only updates the content of `<h1>` with the new `time`. It sees that the `<input>` appears in the JSX in the same place as last time, so React doesn’t touch the `<input>`—or its `value`!
## Epilogue: Browser paint[Link for Epilogue: Browser paint]()
After rendering is done and React updated the DOM, the browser will repaint the screen. Although this process is known as “browser rendering”, we’ll refer to it as “painting” to avoid confusion throughout the docs.

Illustrated by [Rachel Lee Nabors](https://nearestnabors.com/)
## Recap[Link for Recap]()
- Any screen update in a React app happens in three steps:
1. Trigger
2. Render
3. Commit
- You can use Strict Mode to find mistakes in your components
- React does not touch the DOM if the rendering result is the same as last time
[PreviousState: A Component's Memory](https://react.dev/learn/state-a-components-memory)
[NextState as a Snapshot](https://react.dev/learn/state-as-a-snapshot) |
https://react.dev/learn/queueing-a-series-of-state-updates | [Learn React](https://react.dev/learn)
[Adding Interactivity](https://react.dev/learn/adding-interactivity)
# Queueing a Series of State Updates[Link for this heading]()
Setting a state variable will queue another render. But sometimes you might want to perform multiple operations on the value before queueing the next render. To do this, it helps to understand how React batches state updates.
### You will learn
- What “batching” is and how React uses it to process multiple state updates
- How to apply several updates to the same state variable in a row
## React batches state updates[Link for React batches state updates]()
You might expect that clicking the “+3” button will increment the counter three times because it calls `setNumber(number + 1)` three times:
App.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import { useState } from 'react';
export default function Counter() {
const [number, setNumber] = useState(0);
return (
<>
<h1>{number}</h1>
<button onClick={() => {
setNumber(number + 1);
setNumber(number + 1);
setNumber(number + 1);
}}>+3</button>
</>
)
}
```
Show more
However, as you might recall from the previous section, [each render’s state values are fixed](https://react.dev/learn/state-as-a-snapshot), so the value of `number` inside the first render’s event handler is always `0`, no matter how many times you call `setNumber(1)`:
```
setNumber(0 + 1);
setNumber(0 + 1);
setNumber(0 + 1);
```
But there is one other factor at play here. **React waits until *all* code in the event handlers has run before processing your state updates.** This is why the re-render only happens *after* all these `setNumber()` calls.
This might remind you of a waiter taking an order at the restaurant. A waiter doesn’t run to the kitchen at the mention of your first dish! Instead, they let you finish your order, let you make changes to it, and even take orders from other people at the table.

Illustrated by [Rachel Lee Nabors](https://nearestnabors.com/)
This lets you update multiple state variables—even from multiple components—without triggering too many [re-renders.](https://react.dev/learn/render-and-commit) But this also means that the UI won’t be updated until *after* your event handler, and any code in it, completes. This behavior, also known as **batching,** makes your React app run much faster. It also avoids dealing with confusing “half-finished” renders where only some of the variables have been updated.
**React does not batch across *multiple* intentional events like clicks**—each click is handled separately. Rest assured that React only does batching when it’s generally safe to do. This ensures that, for example, if the first button click disables a form, the second click would not submit it again.
## Updating the same state multiple times before the next render[Link for Updating the same state multiple times before the next render]()
It is an uncommon use case, but if you would like to update the same state variable multiple times before the next render, instead of passing the *next state value* like `setNumber(number + 1)`, you can pass a *function* that calculates the next state based on the previous one in the queue, like `setNumber(n => n + 1)`. It is a way to tell React to “do something with the state value” instead of just replacing it.
Try incrementing the counter now:
App.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import { useState } from 'react';
export default function Counter() {
const [number, setNumber] = useState(0);
return (
<>
<h1>{number}</h1>
<button onClick={() => {
setNumber(n => n + 1);
setNumber(n => n + 1);
setNumber(n => n + 1);
}}>+3</button>
</>
)
}
```
Show more
Here, `n => n + 1` is called an **updater function.** When you pass it to a state setter:
1. React queues this function to be processed after all the other code in the event handler has run.
2. During the next render, React goes through the queue and gives you the final updated state.
```
setNumber(n => n + 1);
setNumber(n => n + 1);
setNumber(n => n + 1);
```
Here’s how React works through these lines of code while executing the event handler:
1. `setNumber(n => n + 1)`: `n => n + 1` is a function. React adds it to a queue.
2. `setNumber(n => n + 1)`: `n => n + 1` is a function. React adds it to a queue.
3. `setNumber(n => n + 1)`: `n => n + 1` is a function. React adds it to a queue.
When you call `useState` during the next render, React goes through the queue. The previous `number` state was `0`, so that’s what React passes to the first updater function as the `n` argument. Then React takes the return value of your previous updater function and passes it to the next updater as `n`, and so on:
queued update`n`returns`n => n + 1``0``0 + 1 = 1``n => n + 1``1``1 + 1 = 2``n => n + 1``2``2 + 1 = 3`
React stores `3` as the final result and returns it from `useState`.
This is why clicking “+3” in the above example correctly increments the value by 3.
### What happens if you update state after replacing it[Link for What happens if you update state after replacing it]()
What about this event handler? What do you think `number` will be in the next render?
```
<button onClick={() => {
setNumber(number + 5);
setNumber(n => n + 1);
}}>
```
App.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import { useState } from 'react';
export default function Counter() {
const [number, setNumber] = useState(0);
return (
<>
<h1>{number}</h1>
<button onClick={() => {
setNumber(number + 5);
setNumber(n => n + 1);
}}>Increase the number</button>
</>
)
}
```
Here’s what this event handler tells React to do:
1. `setNumber(number + 5)`: `number` is `0`, so `setNumber(0 + 5)`. React adds *“replace with `5`”* to its queue.
2. `setNumber(n => n + 1)`: `n => n + 1` is an updater function. React adds *that function* to its queue.
During the next render, React goes through the state queue:
queued update`n`returns”replace with `5`”`0` (unused)`5``n => n + 1``5``5 + 1 = 6`
React stores `6` as the final result and returns it from `useState`.
### Note
You may have noticed that `setState(5)` actually works like `setState(n => 5)`, but `n` is unused!
### What happens if you replace state after updating it[Link for What happens if you replace state after updating it]()
Let’s try one more example. What do you think `number` will be in the next render?
```
<button onClick={() => {
setNumber(number + 5);
setNumber(n => n + 1);
setNumber(42);
}}>
```
App.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import { useState } from 'react';
export default function Counter() {
const [number, setNumber] = useState(0);
return (
<>
<h1>{number}</h1>
<button onClick={() => {
setNumber(number + 5);
setNumber(n => n + 1);
setNumber(42);
}}>Increase the number</button>
</>
)
}
```
Show more
Here’s how React works through these lines of code while executing this event handler:
1. `setNumber(number + 5)`: `number` is `0`, so `setNumber(0 + 5)`. React adds *“replace with `5`”* to its queue.
2. `setNumber(n => n + 1)`: `n => n + 1` is an updater function. React adds *that function* to its queue.
3. `setNumber(42)`: React adds *“replace with `42`”* to its queue.
During the next render, React goes through the state queue:
queued update`n`returns”replace with `5`”`0` (unused)`5``n => n + 1``5``5 + 1 = 6`”replace with `42`”`6` (unused)`42`
Then React stores `42` as the final result and returns it from `useState`.
To summarize, here’s how you can think of what you’re passing to the `setNumber` state setter:
- **An updater function** (e.g. `n => n + 1`) gets added to the queue.
- **Any other value** (e.g. number `5`) adds “replace with `5`” to the queue, ignoring what’s already queued.
After the event handler completes, React will trigger a re-render. During the re-render, React will process the queue. Updater functions run during rendering, so **updater functions must be [pure](https://react.dev/learn/keeping-components-pure)** and only *return* the result. Don’t try to set state from inside of them or run other side effects. In Strict Mode, React will run each updater function twice (but discard the second result) to help you find mistakes.
### Naming conventions[Link for Naming conventions]()
It’s common to name the updater function argument by the first letters of the corresponding state variable:
```
setEnabled(e => !e);
setLastName(ln => ln.reverse());
setFriendCount(fc => fc * 2);
```
If you prefer more verbose code, another common convention is to repeat the full state variable name, like `setEnabled(enabled => !enabled)`, or to use a prefix like `setEnabled(prevEnabled => !prevEnabled)`.
## Recap[Link for Recap]()
- Setting state does not change the variable in the existing render, but it requests a new render.
- React processes state updates after event handlers have finished running. This is called batching.
- To update some state multiple times in one event, you can use `setNumber(n => n + 1)` updater function.
## Try out some challenges[Link for Try out some challenges]()
1\. Fix a request counter 2. Implement the state queue yourself
#### Challenge 1 of 2: Fix a request counter[Link for this heading]()
You’re working on an art marketplace app that lets the user submit multiple orders for an art item at the same time. Each time the user presses the “Buy” button, the “Pending” counter should increase by one. After three seconds, the “Pending” counter should decrease, and the “Completed” counter should increase.
However, the “Pending” counter does not behave as intended. When you press “Buy”, it decreases to `-1` (which should not be possible!). And if you click fast twice, both counters seem to behave unpredictably.
Why does this happen? Fix both counters.
App.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import { useState } from 'react';
export default function RequestTracker() {
const [pending, setPending] = useState(0);
const [completed, setCompleted] = useState(0);
async function handleClick() {
setPending(pending + 1);
await delay(3000);
setPending(pending - 1);
setCompleted(completed + 1);
}
return (
<>
<h3>
Pending: {pending}
</h3>
<h3>
Completed: {completed}
</h3>
<button onClick={handleClick}>
Buy
</button>
</>
);
}
function delay(ms) {
return new Promise(resolve => {
setTimeout(resolve, ms);
});
}
```
Show more
Show solutionNext Challenge
[PreviousState as a Snapshot](https://react.dev/learn/state-as-a-snapshot)
[NextUpdating Objects in State](https://react.dev/learn/updating-objects-in-state) |
https://react.dev/learn/responding-to-events | [Learn React](https://react.dev/learn)
[Adding Interactivity](https://react.dev/learn/adding-interactivity)
# Responding to Events[Link for this heading]()
React lets you add *event handlers* to your JSX. Event handlers are your own functions that will be triggered in response to interactions like clicking, hovering, focusing form inputs, and so on.
### You will learn
- Different ways to write an event handler
- How to pass event handling logic from a parent component
- How events propagate and how to stop them
## Adding event handlers[Link for Adding event handlers]()
To add an event handler, you will first define a function and then [pass it as a prop](https://react.dev/learn/passing-props-to-a-component) to the appropriate JSX tag. For example, here is a button that doesn’t do anything yet:
App.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
export default function Button() {
return (
<button>
I don't do anything
</button>
);
}
```
You can make it show a message when a user clicks by following these three steps:
1. Declare a function called `handleClick` *inside* your `Button` component.
2. Implement the logic inside that function (use `alert` to show the message).
3. Add `onClick={handleClick}` to the `<button>` JSX.
App.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
export default function Button() {
function handleClick() {
alert('You clicked me!');
}
return (
<button onClick={handleClick}>
Click me
</button>
);
}
```
You defined the `handleClick` function and then [passed it as a prop](https://react.dev/learn/passing-props-to-a-component) to `<button>`. `handleClick` is an **event handler.** Event handler functions:
- Are usually defined *inside* your components.
- Have names that start with `handle`, followed by the name of the event.
By convention, it is common to name event handlers as `handle` followed by the event name. You’ll often see `onClick={handleClick}`, `onMouseEnter={handleMouseEnter}`, and so on.
Alternatively, you can define an event handler inline in the JSX:
```
<button onClick={function handleClick() {
alert('You clicked me!');
}}>
```
Or, more concisely, using an arrow function:
```
<button onClick={() => {
alert('You clicked me!');
}}>
```
All of these styles are equivalent. Inline event handlers are convenient for short functions.
### Pitfall
Functions passed to event handlers must be passed, not called. For example:
passing a function (correct)calling a function (incorrect)`<button onClick={handleClick}>``<button onClick={handleClick()}>`
The difference is subtle. In the first example, the `handleClick` function is passed as an `onClick` event handler. This tells React to remember it and only call your function when the user clicks the button.
In the second example, the `()` at the end of `handleClick()` fires the function *immediately* during [rendering](https://react.dev/learn/render-and-commit), without any clicks. This is because JavaScript inside the [JSX `{` and `}`](https://react.dev/learn/javascript-in-jsx-with-curly-braces) executes right away.
When you write code inline, the same pitfall presents itself in a different way:
passing a function (correct)calling a function (incorrect)`<button onClick={() => alert('...')}>``<button onClick={alert('...')}>`
Passing inline code like this won’t fire on click—it fires every time the component renders:
```
// This alert fires when the component renders, not when clicked!
<button onClick={alert('You clicked me!')}>
```
If you want to define your event handler inline, wrap it in an anonymous function like so:
```
<button onClick={() => alert('You clicked me!')}>
```
Rather than executing the code inside with every render, this creates a function to be called later.
In both cases, what you want to pass is a function:
- `<button onClick={handleClick}>` passes the `handleClick` function.
- `<button onClick={() => alert('...')}>` passes the `() => alert('...')` function.
[Read more about arrow functions.](https://javascript.info/arrow-functions-basics)
### Reading props in event handlers[Link for Reading props in event handlers]()
Because event handlers are declared inside of a component, they have access to the component’s props. Here is a button that, when clicked, shows an alert with its `message` prop:
App.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
function AlertButton({ message, children }) {
return (
<button onClick={() => alert(message)}>
{children}
</button>
);
}
export default function Toolbar() {
return (
<div>
<AlertButton message="Playing!">
Play Movie
</AlertButton>
<AlertButton message="Uploading!">
Upload Image
</AlertButton>
</div>
);
}
```
Show more
This lets these two buttons show different messages. Try changing the messages passed to them.
### Passing event handlers as props[Link for Passing event handlers as props]()
Often you’ll want the parent component to specify a child’s event handler. Consider buttons: depending on where you’re using a `Button` component, you might want to execute a different function—perhaps one plays a movie and another uploads an image.
To do this, pass a prop the component receives from its parent as the event handler like so:
App.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
function Button({ onClick, children }) {
return (
<button onClick={onClick}>
{children}
</button>
);
}
function PlayButton({ movieName }) {
function handlePlayClick() {
alert(`Playing ${movieName}!`);
}
return (
<Button onClick={handlePlayClick}>
Play "{movieName}"
</Button>
);
}
function UploadButton() {
return (
<Button onClick={() => alert('Uploading!')}>
Upload Image
</Button>
);
}
export default function Toolbar() {
return (
<div>
<PlayButton movieName="Kiki's Delivery Service" />
<UploadButton />
</div>
);
}
```
Show more
Here, the `Toolbar` component renders a `PlayButton` and an `UploadButton`:
- `PlayButton` passes `handlePlayClick` as the `onClick` prop to the `Button` inside.
- `UploadButton` passes `() => alert('Uploading!')` as the `onClick` prop to the `Button` inside.
Finally, your `Button` component accepts a prop called `onClick`. It passes that prop directly to the built-in browser `<button>` with `onClick={onClick}`. This tells React to call the passed function on click.
If you use a [design system](https://uxdesign.cc/everything-you-need-to-know-about-design-systems-54b109851969), it’s common for components like buttons to contain styling but not specify behavior. Instead, components like `PlayButton` and `UploadButton` will pass event handlers down.
### Naming event handler props[Link for Naming event handler props]()
Built-in components like `<button>` and `<div>` only support [browser event names](https://react.dev/reference/react-dom/components/common) like `onClick`. However, when you’re building your own components, you can name their event handler props any way that you like.
By convention, event handler props should start with `on`, followed by a capital letter.
For example, the `Button` component’s `onClick` prop could have been called `onSmash`:
App.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
function Button({ onSmash, children }) {
return (
<button onClick={onSmash}>
{children}
</button>
);
}
export default function App() {
return (
<div>
<Button onSmash={() => alert('Playing!')}>
Play Movie
</Button>
<Button onSmash={() => alert('Uploading!')}>
Upload Image
</Button>
</div>
);
}
```
Show more
In this example, `<button onClick={onSmash}>` shows that the browser `<button>` (lowercase) still needs a prop called `onClick`, but the prop name received by your custom `Button` component is up to you!
When your component supports multiple interactions, you might name event handler props for app-specific concepts. For example, this `Toolbar` component receives `onPlayMovie` and `onUploadImage` event handlers:
App.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
export default function App() {
return (
<Toolbar
onPlayMovie={() => alert('Playing!')}
onUploadImage={() => alert('Uploading!')}
/>
);
}
function Toolbar({ onPlayMovie, onUploadImage }) {
return (
<div>
<Button onClick={onPlayMovie}>
Play Movie
</Button>
<Button onClick={onUploadImage}>
Upload Image
</Button>
</div>
);
}
function Button({ onClick, children }) {
return (
<button onClick={onClick}>
{children}
</button>
);
}
```
Show more
Notice how the `App` component does not need to know *what* `Toolbar` will do with `onPlayMovie` or `onUploadImage`. That’s an implementation detail of the `Toolbar`. Here, `Toolbar` passes them down as `onClick` handlers to its `Button`s, but it could later also trigger them on a keyboard shortcut. Naming props after app-specific interactions like `onPlayMovie` gives you the flexibility to change how they’re used later.
### Note
Make sure that you use the appropriate HTML tags for your event handlers. For example, to handle clicks, use [`<button onClick={handleClick}>`](https://developer.mozilla.org/en-US/docs/Web/HTML/Element/button) instead of `<div onClick={handleClick}>`. Using a real browser `<button>` enables built-in browser behaviors like keyboard navigation. If you don’t like the default browser styling of a button and want to make it look more like a link or a different UI element, you can achieve it with CSS. [Learn more about writing accessible markup.](https://developer.mozilla.org/en-US/docs/Learn/Accessibility/HTML)
## Event propagation[Link for Event propagation]()
Event handlers will also catch events from any children your component might have. We say that an event “bubbles” or “propagates” up the tree: it starts with where the event happened, and then goes up the tree.
This `<div>` contains two buttons. Both the `<div>` *and* each button have their own `onClick` handlers. Which handlers do you think will fire when you click a button?
App.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
export default function Toolbar() {
return (
<div className="Toolbar" onClick={() => {
alert('You clicked on the toolbar!');
}}>
<button onClick={() => alert('Playing!')}>
Play Movie
</button>
<button onClick={() => alert('Uploading!')}>
Upload Image
</button>
</div>
);
}
```
If you click on either button, its `onClick` will run first, followed by the parent `<div>`’s `onClick`. So two messages will appear. If you click the toolbar itself, only the parent `<div>`’s `onClick` will run.
### Pitfall
All events propagate in React except `onScroll`, which only works on the JSX tag you attach it to.
### Stopping propagation[Link for Stopping propagation]()
Event handlers receive an **event object** as their only argument. By convention, it’s usually called `e`, which stands for “event”. You can use this object to read information about the event.
That event object also lets you stop the propagation. If you want to prevent an event from reaching parent components, you need to call `e.stopPropagation()` like this `Button` component does:
App.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
function Button({ onClick, children }) {
return (
<button onClick={e => {
e.stopPropagation();
onClick();
}}>
{children}
</button>
);
}
export default function Toolbar() {
return (
<div className="Toolbar" onClick={() => {
alert('You clicked on the toolbar!');
}}>
<Button onClick={() => alert('Playing!')}>
Play Movie
</Button>
<Button onClick={() => alert('Uploading!')}>
Upload Image
</Button>
</div>
);
}
```
Show more
When you click on a button:
1. React calls the `onClick` handler passed to `<button>`.
2. That handler, defined in `Button`, does the following:
- Calls `e.stopPropagation()`, preventing the event from bubbling further.
- Calls the `onClick` function, which is a prop passed from the `Toolbar` component.
3. That function, defined in the `Toolbar` component, displays the button’s own alert.
4. Since the propagation was stopped, the parent `<div>`’s `onClick` handler does *not* run.
As a result of `e.stopPropagation()`, clicking on the buttons now only shows a single alert (from the `<button>`) rather than the two of them (from the `<button>` and the parent toolbar `<div>`). Clicking a button is not the same thing as clicking the surrounding toolbar, so stopping the propagation makes sense for this UI.
##### Deep Dive
#### Capture phase events[Link for Capture phase events]()
Show Details
In rare cases, you might need to catch all events on child elements, *even if they stopped propagation*. For example, maybe you want to log every click to analytics, regardless of the propagation logic. You can do this by adding `Capture` at the end of the event name:
```
<div onClickCapture={() => { /* this runs first */ }}>
<button onClick={e => e.stopPropagation()} />
<button onClick={e => e.stopPropagation()} />
</div>
```
Each event propagates in three phases:
1. It travels down, calling all `onClickCapture` handlers.
2. It runs the clicked element’s `onClick` handler.
3. It travels upwards, calling all `onClick` handlers.
Capture events are useful for code like routers or analytics, but you probably won’t use them in app code.
### Passing handlers as alternative to propagation[Link for Passing handlers as alternative to propagation]()
Notice how this click handler runs a line of code *and then* calls the `onClick` prop passed by the parent:
```
function Button({ onClick, children }) {
return (
<button onClick={e => {
e.stopPropagation();
onClick();
}}>
{children}
</button>
);
}
```
You could add more code to this handler before calling the parent `onClick` event handler, too. This pattern provides an *alternative* to propagation. It lets the child component handle the event, while also letting the parent component specify some additional behavior. Unlike propagation, it’s not automatic. But the benefit of this pattern is that you can clearly follow the whole chain of code that executes as a result of some event.
If you rely on propagation and it’s difficult to trace which handlers execute and why, try this approach instead.
### Preventing default behavior[Link for Preventing default behavior]()
Some browser events have default behavior associated with them. For example, a `<form>` submit event, which happens when a button inside of it is clicked, will reload the whole page by default:
App.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
export default function Signup() {
return (
<form onSubmit={() => alert('Submitting!')}>
<input />
<button>Send</button>
</form>
);
}
```
You can call `e.preventDefault()` on the event object to stop this from happening:
App.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
export default function Signup() {
return (
<form onSubmit={e => {
e.preventDefault();
alert('Submitting!');
}}>
<input />
<button>Send</button>
</form>
);
}
```
Don’t confuse `e.stopPropagation()` and `e.preventDefault()`. They are both useful, but are unrelated:
- [`e.stopPropagation()`](https://developer.mozilla.org/docs/Web/API/Event/stopPropagation) stops the event handlers attached to the tags above from firing.
- [`e.preventDefault()`](https://developer.mozilla.org/docs/Web/API/Event/preventDefault) prevents the default browser behavior for the few events that have it.
## Can event handlers have side effects?[Link for Can event handlers have side effects?]()
Absolutely! Event handlers are the best place for side effects.
Unlike rendering functions, event handlers don’t need to be [pure](https://react.dev/learn/keeping-components-pure), so it’s a great place to *change* something—for example, change an input’s value in response to typing, or change a list in response to a button press. However, in order to change some information, you first need some way to store it. In React, this is done by using [state, a component’s memory.](https://react.dev/learn/state-a-components-memory) You will learn all about it on the next page.
## Recap[Link for Recap]()
- You can handle events by passing a function as a prop to an element like `<button>`.
- Event handlers must be passed, **not called!** `onClick={handleClick}`, not `onClick={handleClick()}`.
- You can define an event handler function separately or inline.
- Event handlers are defined inside a component, so they can access props.
- You can declare an event handler in a parent and pass it as a prop to a child.
- You can define your own event handler props with application-specific names.
- Events propagate upwards. Call `e.stopPropagation()` on the first argument to prevent that.
- Events may have unwanted default browser behavior. Call `e.preventDefault()` to prevent that.
- Explicitly calling an event handler prop from a child handler is a good alternative to propagation.
## Try out some challenges[Link for Try out some challenges]()
1\. Fix an event handler 2. Wire up the events
#### Challenge 1 of 2: Fix an event handler[Link for this heading]()
Clicking this button is supposed to switch the page background between white and black. However, nothing happens when you click it. Fix the problem. (Don’t worry about the logic inside `handleClick`—that part is fine.)
App.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
export default function LightSwitch() {
function handleClick() {
let bodyStyle = document.body.style;
if (bodyStyle.backgroundColor === 'black') {
bodyStyle.backgroundColor = 'white';
} else {
bodyStyle.backgroundColor = 'black';
}
}
return (
<button onClick={handleClick()}>
Toggle the lights
</button>
);
}
```
Show more
Show solutionNext Challenge
[PreviousAdding Interactivity](https://react.dev/learn/adding-interactivity)
[NextState: A Component's Memory](https://react.dev/learn/state-a-components-memory) |
https://react.dev/learn/state-as-a-snapshot | [Learn React](https://react.dev/learn)
[Adding Interactivity](https://react.dev/learn/adding-interactivity)
# State as a Snapshot[Link for this heading]()
State variables might look like regular JavaScript variables that you can read and write to. However, state behaves more like a snapshot. Setting it does not change the state variable you already have, but instead triggers a re-render.
### You will learn
- How setting state triggers re-renders
- When and how state updates
- Why state does not update immediately after you set it
- How event handlers access a “snapshot” of the state
## Setting state triggers renders[Link for Setting state triggers renders]()
You might think of your user interface as changing directly in response to the user event like a click. In React, it works a little differently from this mental model. On the previous page, you saw that [setting state requests a re-render](https://react.dev/learn/render-and-commit) from React. This means that for an interface to react to the event, you need to *update the state*.
In this example, when you press “send”, `setIsSent(true)` tells React to re-render the UI:
App.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import { useState } from 'react';
export default function Form() {
const [isSent, setIsSent] = useState(false);
const [message, setMessage] = useState('Hi!');
if (isSent) {
return <h1>Your message is on its way!</h1>
}
return (
<form onSubmit={(e) => {
e.preventDefault();
setIsSent(true);
sendMessage(message);
}}>
<textarea
placeholder="Message"
value={message}
onChange={e => setMessage(e.target.value)}
/>
<button type="submit">Send</button>
</form>
);
}
function sendMessage(message) {
// ...
}
```
Show more
Here’s what happens when you click the button:
1. The `onSubmit` event handler executes.
2. `setIsSent(true)` sets `isSent` to `true` and queues a new render.
3. React re-renders the component according to the new `isSent` value.
Let’s take a closer look at the relationship between state and rendering.
## Rendering takes a snapshot in time[Link for Rendering takes a snapshot in time]()
[“Rendering”](https://react.dev/learn/render-and-commit) means that React is calling your component, which is a function. The JSX you return from that function is like a snapshot of the UI in time. Its props, event handlers, and local variables were all calculated **using its state at the time of the render.**
Unlike a photograph or a movie frame, the UI “snapshot” you return is interactive. It includes logic like event handlers that specify what happens in response to inputs. React updates the screen to match this snapshot and connects the event handlers. As a result, pressing a button will trigger the click handler from your JSX.
When React re-renders a component:
1. React calls your function again.
2. Your function returns a new JSX snapshot.
3. React then updates the screen to match the snapshot your function returned.
<!--THE END-->
1. 
React executing the function
2. 
Calculating the snapshot
3. 
Updating the DOM tree
Illustrated by [Rachel Lee Nabors](https://nearestnabors.com/)
As a component’s memory, state is not like a regular variable that disappears after your function returns. State actually “lives” in React itself—as if on a shelf!—outside of your function. When React calls your component, it gives you a snapshot of the state for that particular render. Your component returns a snapshot of the UI with a fresh set of props and event handlers in its JSX, all calculated **using the state values from that render!**
1. 
You tell React to update the state
2. 
React updates the state value
3. 
React passes a snapshot of the state value into the component
Illustrated by [Rachel Lee Nabors](https://nearestnabors.com/)
Here’s a little experiment to show you how this works. In this example, you might expect that clicking the “+3” button would increment the counter three times because it calls `setNumber(number + 1)` three times.
See what happens when you click the “+3” button:
App.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import { useState } from 'react';
export default function Counter() {
const [number, setNumber] = useState(0);
return (
<>
<h1>{number}</h1>
<button onClick={() => {
setNumber(number + 1);
setNumber(number + 1);
setNumber(number + 1);
}}>+3</button>
</>
)
}
```
Show more
Notice that `number` only increments once per click!
**Setting state only changes it for the *next* render.** During the first render, `number` was `0`. This is why, in *that render’s* `onClick` handler, the value of `number` is still `0` even after `setNumber(number + 1)` was called:
```
<button onClick={() => {
setNumber(number + 1);
setNumber(number + 1);
setNumber(number + 1);
}}>+3</button>
```
Here is what this button’s click handler tells React to do:
1. `setNumber(number + 1)`: `number` is `0` so `setNumber(0 + 1)`.
- React prepares to change `number` to `1` on the next render.
2. `setNumber(number + 1)`: `number` is `0` so `setNumber(0 + 1)`.
- React prepares to change `number` to `1` on the next render.
3. `setNumber(number + 1)`: `number` is `0` so `setNumber(0 + 1)`.
- React prepares to change `number` to `1` on the next render.
Even though you called `setNumber(number + 1)` three times, in *this render’s* event handler `number` is always `0`, so you set the state to `1` three times. This is why, after your event handler finishes, React re-renders the component with `number` equal to `1` rather than `3`.
You can also visualize this by mentally substituting state variables with their values in your code. Since the `number` state variable is `0` for *this render*, its event handler looks like this:
```
<button onClick={() => {
setNumber(0 + 1);
setNumber(0 + 1);
setNumber(0 + 1);
}}>+3</button>
```
For the next render, `number` is `1`, so *that render’s* click handler looks like this:
```
<button onClick={() => {
setNumber(1 + 1);
setNumber(1 + 1);
setNumber(1 + 1);
}}>+3</button>
```
This is why clicking the button again will set the counter to `2`, then to `3` on the next click, and so on.
## State over time[Link for State over time]()
Well, that was fun. Try to guess what clicking this button will alert:
App.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import { useState } from 'react';
export default function Counter() {
const [number, setNumber] = useState(0);
return (
<>
<h1>{number}</h1>
<button onClick={() => {
setNumber(number + 5);
alert(number);
}}>+5</button>
</>
)
}
```
If you use the substitution method from before, you can guess that the alert shows “0”:
```
setNumber(0 + 5);
alert(0);
```
But what if you put a timer on the alert, so it only fires *after* the component re-rendered? Would it say “0” or “5”? Have a guess!
App.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import { useState } from 'react';
export default function Counter() {
const [number, setNumber] = useState(0);
return (
<>
<h1>{number}</h1>
<button onClick={() => {
setNumber(number + 5);
setTimeout(() => {
alert(number);
}, 3000);
}}>+5</button>
</>
)
}
```
Show more
Surprised? If you use the substitution method, you can see the “snapshot” of the state passed to the alert.
```
setNumber(0 + 5);
setTimeout(() => {
alert(0);
}, 3000);
```
The state stored in React may have changed by the time the alert runs, but it was scheduled using a snapshot of the state at the time the user interacted with it!
**A state variable’s value never changes within a render,** even if its event handler’s code is asynchronous. Inside *that render’s* `onClick`, the value of `number` continues to be `0` even after `setNumber(number + 5)` was called. Its value was “fixed” when React “took the snapshot” of the UI by calling your component.
Here is an example of how that makes your event handlers less prone to timing mistakes. Below is a form that sends a message with a five-second delay. Imagine this scenario:
1. You press the “Send” button, sending “Hello” to Alice.
2. Before the five-second delay ends, you change the value of the “To” field to “Bob”.
What do you expect the `alert` to display? Would it display, “You said Hello to Alice”? Or would it display, “You said Hello to Bob”? Make a guess based on what you know, and then try it:
App.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import { useState } from 'react';
export default function Form() {
const [to, setTo] = useState('Alice');
const [message, setMessage] = useState('Hello');
function handleSubmit(e) {
e.preventDefault();
setTimeout(() => {
alert(`You said ${message} to ${to}`);
}, 5000);
}
return (
<form onSubmit={handleSubmit}>
<label>
To:{' '}
<select
value={to}
onChange={e => setTo(e.target.value)}>
<option value="Alice">Alice</option>
<option value="Bob">Bob</option>
</select>
</label>
<textarea
placeholder="Message"
value={message}
onChange={e => setMessage(e.target.value)}
/>
<button type="submit">Send</button>
</form>
);
}
```
Show more
**React keeps the state values “fixed” within one render’s event handlers.** You don’t need to worry whether the state has changed while the code is running.
But what if you wanted to read the latest state before a re-render? You’ll want to use a [state updater function](https://react.dev/learn/queueing-a-series-of-state-updates), covered on the next page!
## Recap[Link for Recap]()
- Setting state requests a new render.
- React stores state outside of your component, as if on a shelf.
- When you call `useState`, React gives you a snapshot of the state *for that render*.
- Variables and event handlers don’t “survive” re-renders. Every render has its own event handlers.
- Every render (and functions inside it) will always “see” the snapshot of the state that React gave to *that* render.
- You can mentally substitute state in event handlers, similarly to how you think about the rendered JSX.
- Event handlers created in the past have the state values from the render in which they were created.
## Try out some challenges[Link for Try out some challenges]()
#### Challenge 1 of 1: Implement a traffic light[Link for this heading]()
Here is a crosswalk light component that toggles when the button is pressed:
App.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import { useState } from 'react';
export default function TrafficLight() {
const [walk, setWalk] = useState(true);
function handleClick() {
setWalk(!walk);
}
return (
<>
<button onClick={handleClick}>
Change to {walk ? 'Stop' : 'Walk'}
</button>
<h1 style={{
color: walk ? 'darkgreen' : 'darkred'
}}>
{walk ? 'Walk' : 'Stop'}
</h1>
</>
);
}
```
Show more
Add an `alert` to the click handler. When the light is green and says “Walk”, clicking the button should say “Stop is next”. When the light is red and says “Stop”, clicking the button should say “Walk is next”.
Does it make a difference whether you put the `alert` before or after the `setWalk` call?
Show solution
[PreviousRender and Commit](https://react.dev/learn/render-and-commit)
[NextQueueing a Series of State Updates](https://react.dev/learn/queueing-a-series-of-state-updates) |
https://react.dev/learn/tutorial-tic-tac-toe | [Learn React](https://react.dev/learn)
[Quick Start](https://react.dev/learn)
# Tutorial: Tic-Tac-Toe[Link for this heading]()
You will build a small tic-tac-toe game during this tutorial. This tutorial does not assume any existing React knowledge. The techniques you’ll learn in the tutorial are fundamental to building any React app, and fully understanding it will give you a deep understanding of React.
### Note
This tutorial is designed for people who prefer to **learn by doing** and want to quickly try making something tangible. If you prefer learning each concept step by step, start with [Describing the UI.](https://react.dev/learn/describing-the-ui)
The tutorial is divided into several sections:
- [Setup for the tutorial]() will give you **a starting point** to follow the tutorial.
- [Overview]() will teach you **the fundamentals** of React: components, props, and state.
- [Completing the game]() will teach you **the most common techniques** in React development.
- [Adding time travel]() will give you **a deeper insight** into the unique strengths of React.
### What are you building?[Link for What are you building?]()
In this tutorial, you’ll build an interactive tic-tac-toe game with React.
You can see what it will look like when you’re finished here:
App.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import { useState } from 'react';
function Square({ value, onSquareClick }) {
return (
<button className="square" onClick={onSquareClick}>
{value}
</button>
);
}
function Board({ xIsNext, squares, onPlay }) {
function handleClick(i) {
if (calculateWinner(squares) || squares[i]) {
return;
}
const nextSquares = squares.slice();
if (xIsNext) {
nextSquares[i] = 'X';
} else {
nextSquares[i] = 'O';
}
onPlay(nextSquares);
}
const winner = calculateWinner(squares);
let status;
if (winner) {
status = 'Winner: ' + winner;
} else {
status = 'Next player: ' + (xIsNext ? 'X' : 'O');
}
return (
<>
<div className="status">{status}</div>
<div className="board-row">
<Square value={squares[0]} onSquareClick={() => handleClick(0)} />
<Square value={squares[1]} onSquareClick={() => handleClick(1)} />
<Square value={squares[2]} onSquareClick={() => handleClick(2)} />
</div>
<div className="board-row">
<Square value={squares[3]} onSquareClick={() => handleClick(3)} />
<Square value={squares[4]} onSquareClick={() => handleClick(4)} />
<Square value={squares[5]} onSquareClick={() => handleClick(5)} />
</div>
<div className="board-row">
<Square value={squares[6]} onSquareClick={() => handleClick(6)} />
<Square value={squares[7]} onSquareClick={() => handleClick(7)} />
<Square value={squares[8]} onSquareClick={() => handleClick(8)} />
</div>
</>
);
}
export default function Game() {
const [history, setHistory] = useState([Array(9).fill(null)]);
const [currentMove, setCurrentMove] = useState(0);
const xIsNext = currentMove % 2 === 0;
const currentSquares = history[currentMove];
function handlePlay(nextSquares) {
const nextHistory = [...history.slice(0, currentMove + 1), nextSquares];
setHistory(nextHistory);
setCurrentMove(nextHistory.length - 1);
}
function jumpTo(nextMove) {
setCurrentMove(nextMove);
}
const moves = history.map((squares, move) => {
let description;
if (move > 0) {
description = 'Go to move #' + move;
} else {
description = 'Go to game start';
}
return (
<li key={move}>
<button onClick={() => jumpTo(move)}>{description}</button>
</li>
);
});
return (
<div className="game">
<div className="game-board">
<Board xIsNext={xIsNext} squares={currentSquares} onPlay={handlePlay} />
</div>
<div className="game-info">
<ol>{moves}</ol>
</div>
</div>
);
}
function calculateWinner(squares) {
const lines = [
[0, 1, 2],
[3, 4, 5],
[6, 7, 8],
[0, 3, 6],
[1, 4, 7],
[2, 5, 8],
[0, 4, 8],
[2, 4, 6],
];
for (let i = 0; i < lines.length; i++) {
const [a, b, c] = lines[i];
if (squares[a] && squares[a] === squares[b] && squares[a] === squares[c]) {
return squares[a];
}
}
return null;
}
```
Show more
If the code doesn’t make sense to you yet, or if you are unfamiliar with the code’s syntax, don’t worry! The goal of this tutorial is to help you understand React and its syntax.
We recommend that you check out the tic-tac-toe game above before continuing with the tutorial. One of the features that you’ll notice is that there is a numbered list to the right of the game’s board. This list gives you a history of all of the moves that have occurred in the game, and it is updated as the game progresses.
Once you’ve played around with the finished tic-tac-toe game, keep scrolling. You’ll start with a simpler template in this tutorial. Our next step is to set you up so that you can start building the game.
## Setup for the tutorial[Link for Setup for the tutorial]()
In the live code editor below, click **Fork** in the top-right corner to open the editor in a new tab using the website CodeSandbox. CodeSandbox lets you write code in your browser and preview how your users will see the app you’ve created. The new tab should display an empty square and the starter code for this tutorial.
App.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
export default function Square() {
return <button className="square">X</button>;
}
```
### Note
You can also follow this tutorial using your local development environment. To do this, you need to:
1. Install [Node.js](https://nodejs.org/en/)
2. In the CodeSandbox tab you opened earlier, press the top-left corner button to open the menu, and then choose **Download Sandbox** in that menu to download an archive of the files locally
3. Unzip the archive, then open a terminal and `cd` to the directory you unzipped
4. Install the dependencies with `npm install`
5. Run `npm start` to start a local server and follow the prompts to view the code running in a browser
If you get stuck, don’t let this stop you! Follow along online instead and try a local setup again later.
## Overview[Link for Overview]()
Now that you’re set up, let’s get an overview of React!
### Inspecting the starter code[Link for Inspecting the starter code]()
In CodeSandbox you’ll see three main sections:

1. The *Files* section with a list of files like `App.js`, `index.js`, `styles.css` and a folder called `public`
2. The *code editor* where you’ll see the source code of your selected file
3. The *browser* section where you’ll see how the code you’ve written will be displayed
The `App.js` file should be selected in the *Files* section. The contents of that file in the *code editor* should be:
```
export default function Square() {
return <button className="square">X</button>;
}
```
The *browser* section should be displaying a square with a X in it like this:

Now let’s have a look at the files in the starter code.
#### `App.js`[Link for this heading]()
The code in `App.js` creates a *component*. In React, a component is a piece of reusable code that represents a part of a user interface. Components are used to render, manage, and update the UI elements in your application. Let’s look at the component line by line to see what’s going on:
```
export default function Square() {
return <button className="square">X</button>;
}
```
The first line defines a function called `Square`. The `export` JavaScript keyword makes this function accessible outside of this file. The `default` keyword tells other files using your code that it’s the main function in your file.
```
export default function Square() {
return <button className="square">X</button>;
}
```
The second line returns a button. The `return` JavaScript keyword means whatever comes after is returned as a value to the caller of the function. `<button>` is a *JSX element*. A JSX element is a combination of JavaScript code and HTML tags that describes what you’d like to display. `className="square"` is a button property or *prop* that tells CSS how to style the button. `X` is the text displayed inside of the button and `</button>` closes the JSX element to indicate that any following content shouldn’t be placed inside the button.
#### `styles.css`[Link for this heading]()
Click on the file labeled `styles.css` in the *Files* section of CodeSandbox. This file defines the styles for your React app. The first two *CSS selectors* (`*` and `body`) define the style of large parts of your app while the `.square` selector defines the style of any component where the `className` property is set to `square`. In your code, that would match the button from your Square component in the `App.js` file.
#### `index.js`[Link for this heading]()
Click on the file labeled `index.js` in the *Files* section of CodeSandbox. You won’t be editing this file during the tutorial but it is the bridge between the component you created in the `App.js` file and the web browser.
```
import { StrictMode } from 'react';
import { createRoot } from 'react-dom/client';
import './styles.css';
import App from './App';
```
Lines 1-5 bring all the necessary pieces together:
- React
- React’s library to talk to web browsers (React DOM)
- the styles for your components
- the component you created in `App.js`.
The remainder of the file brings all the pieces together and injects the final product into `index.html` in the `public` folder.
### Building the board[Link for Building the board]()
Let’s get back to `App.js`. This is where you’ll spend the rest of the tutorial.
Currently the board is only a single square, but you need nine! If you just try and copy paste your square to make two squares like this:
```
export default function Square() {
return <button className="square">X</button><button className="square">X</button>;
}
```
You’ll get this error:
Console
/src/App.js: Adjacent JSX elements must be wrapped in an enclosing tag. Did you want a JSX Fragment `<>...</>`?
React components need to return a single JSX element and not multiple adjacent JSX elements like two buttons. To fix this you can use *Fragments* (`<>` and `</>`) to wrap multiple adjacent JSX elements like this:
```
export default function Square() {
return (
<>
<button className="square">X</button>
<button className="square">X</button>
</>
);
}
```
Now you should see:

Great! Now you just need to copy-paste a few times to add nine squares and…

Oh no! The squares are all in a single line, not in a grid like you need for our board. To fix this you’ll need to group your squares into rows with `div`s and add some CSS classes. While you’re at it, you’ll give each square a number to make sure you know where each square is displayed.
In the `App.js` file, update the `Square` component to look like this:
```
export default function Square() {
return (
<>
<div className="board-row">
<button className="square">1</button>
<button className="square">2</button>
<button className="square">3</button>
</div>
<div className="board-row">
<button className="square">4</button>
<button className="square">5</button>
<button className="square">6</button>
</div>
<div className="board-row">
<button className="square">7</button>
<button className="square">8</button>
<button className="square">9</button>
</div>
</>
);
}
```
The CSS defined in `styles.css` styles the divs with the `className` of `board-row`. Now that you’ve grouped your components into rows with the styled `div`s you have your tic-tac-toe board:

But you now have a problem. Your component named `Square`, really isn’t a square anymore. Let’s fix that by changing the name to `Board`:
```
export default function Board() {
//...
}
```
At this point your code should look something like this:
App.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
export default function Board() {
return (
<>
<div className="board-row">
<button className="square">1</button>
<button className="square">2</button>
<button className="square">3</button>
</div>
<div className="board-row">
<button className="square">4</button>
<button className="square">5</button>
<button className="square">6</button>
</div>
<div className="board-row">
<button className="square">7</button>
<button className="square">8</button>
<button className="square">9</button>
</div>
</>
);
}
```
Show more
### Note
Psssst… That’s a lot to type! It’s okay to copy and paste code from this page. However, if you’re up for a little challenge, we recommend only copying code that you’ve manually typed at least once yourself.
### Passing data through props[Link for Passing data through props]()
Next, you’ll want to change the value of a square from empty to “X” when the user clicks on the square. With how you’ve built the board so far you would need to copy-paste the code that updates the square nine times (once for each square you have)! Instead of copy-pasting, React’s component architecture allows you to create a reusable component to avoid messy, duplicated code.
First, you are going to copy the line defining your first square (`<button className="square">1</button>`) from your `Board` component into a new `Square` component:
```
function Square() {
return <button className="square">1</button>;
}
export default function Board() {
// ...
}
```
Then you’ll update the Board component to render that `Square` component using JSX syntax:
```
// ...
export default function Board() {
return (
<>
<div className="board-row">
<Square />
<Square />
<Square />
</div>
<div className="board-row">
<Square />
<Square />
<Square />
</div>
<div className="board-row">
<Square />
<Square />
<Square />
</div>
</>
);
}
```
Note how unlike the browser `div`s, your own components `Board` and `Square` must start with a capital letter.
Let’s take a look:

Oh no! You lost the numbered squares you had before. Now each square says “1”. To fix this, you will use *props* to pass the value each square should have from the parent component (`Board`) to its child (`Square`).
Update the `Square` component to read the `value` prop that you’ll pass from the `Board`:
```
function Square({ value }) {
return <button className="square">1</button>;
}
```
`function Square({ value })` indicates the Square component can be passed a prop called `value`.
Now you want to display that `value` instead of `1` inside every square. Try doing it like this:
```
function Square({ value }) {
return <button className="square">value</button>;
}
```
Oops, this is not what you wanted:

You wanted to render the JavaScript variable called `value` from your component, not the word “value”. To “escape into JavaScript” from JSX, you need curly braces. Add curly braces around `value` in JSX like so:
```
function Square({ value }) {
return <button className="square">{value}</button>;
}
```
For now, you should see an empty board:

This is because the `Board` component hasn’t passed the `value` prop to each `Square` component it renders yet. To fix it you’ll add the `value` prop to each `Square` component rendered by the `Board` component:
```
export default function Board() {
return (
<>
<div className="board-row">
<Square value="1" />
<Square value="2" />
<Square value="3" />
</div>
<div className="board-row">
<Square value="4" />
<Square value="5" />
<Square value="6" />
</div>
<div className="board-row">
<Square value="7" />
<Square value="8" />
<Square value="9" />
</div>
</>
);
}
```
Now you should see a grid of numbers again:

Your updated code should look like this:
App.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
function Square({ value }) {
return <button className="square">{value}</button>;
}
export default function Board() {
return (
<>
<div className="board-row">
<Square value="1" />
<Square value="2" />
<Square value="3" />
</div>
<div className="board-row">
<Square value="4" />
<Square value="5" />
<Square value="6" />
</div>
<div className="board-row">
<Square value="7" />
<Square value="8" />
<Square value="9" />
</div>
</>
);
}
```
Show more
### Making an interactive component[Link for Making an interactive component]()
Let’s fill the `Square` component with an `X` when you click it. Declare a function called `handleClick` inside of the `Square`. Then, add `onClick` to the props of the button JSX element returned from the `Square`:
```
function Square({ value }) {
function handleClick() {
console.log('clicked!');
}
return (
<button
className="square"
onClick={handleClick}
>
{value}
</button>
);
}
```
If you click on a square now, you should see a log saying `"clicked!"` in the *Console* tab at the bottom of the *Browser* section in CodeSandbox. Clicking the square more than once will log `"clicked!"` again. Repeated console logs with the same message will not create more lines in the console. Instead, you will see an incrementing counter next to your first `"clicked!"` log.
### Note
If you are following this tutorial using your local development environment, you need to open your browser’s Console. For example, if you use the Chrome browser, you can view the Console with the keyboard shortcut **Shift + Ctrl + J** (on Windows/Linux) or **Option + ⌘ + J** (on macOS).
As a next step, you want the Square component to “remember” that it got clicked, and fill it with an “X” mark. To “remember” things, components use *state*.
React provides a special function called `useState` that you can call from your component to let it “remember” things. Let’s store the current value of the `Square` in state, and change it when the `Square` is clicked.
Import `useState` at the top of the file. Remove the `value` prop from the `Square` component. Instead, add a new line at the start of the `Square` that calls `useState`. Have it return a state variable called `value`:
```
import { useState } from 'react';
function Square() {
const [value, setValue] = useState(null);
function handleClick() {
//...
```
`value` stores the value and `setValue` is a function that can be used to change the value. The `null` passed to `useState` is used as the initial value for this state variable, so `value` here starts off equal to `null`.
Since the `Square` component no longer accepts props anymore, you’ll remove the `value` prop from all nine of the Square components created by the Board component:
```
// ...
export default function Board() {
return (
<>
<div className="board-row">
<Square />
<Square />
<Square />
</div>
<div className="board-row">
<Square />
<Square />
<Square />
</div>
<div className="board-row">
<Square />
<Square />
<Square />
</div>
</>
);
}
```
Now you’ll change `Square` to display an “X” when clicked. Replace the `console.log("clicked!");` event handler with `setValue('X');`. Now your `Square` component looks like this:
```
function Square() {
const [value, setValue] = useState(null);
function handleClick() {
setValue('X');
}
return (
<button
className="square"
onClick={handleClick}
>
{value}
</button>
);
}
```
By calling this `set` function from an `onClick` handler, you’re telling React to re-render that `Square` whenever its `<button>` is clicked. After the update, the `Square`’s `value` will be `'X'`, so you’ll see the “X” on the game board. Click on any Square, and “X” should show up:

Each Square has its own state: the `value` stored in each Square is completely independent of the others. When you call a `set` function in a component, React automatically updates the child components inside too.
After you’ve made the above changes, your code will look like this:
App.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import { useState } from 'react';
function Square() {
const [value, setValue] = useState(null);
function handleClick() {
setValue('X');
}
return (
<button
className="square"
onClick={handleClick}
>
{value}
</button>
);
}
export default function Board() {
return (
<>
<div className="board-row">
<Square />
<Square />
<Square />
</div>
<div className="board-row">
<Square />
<Square />
<Square />
</div>
<div className="board-row">
<Square />
<Square />
<Square />
</div>
</>
);
}
```
Show more
### React Developer Tools[Link for React Developer Tools]()
React DevTools let you check the props and the state of your React components. You can find the React DevTools tab at the bottom of the *browser* section in CodeSandbox:

To inspect a particular component on the screen, use the button in the top left corner of React DevTools:

### Note
For local development, React DevTools is available as a [Chrome](https://chrome.google.com/webstore/detail/react-developer-tools/fmkadmapgofadopljbjfkapdkoienihi?hl=en), [Firefox](https://addons.mozilla.org/en-US/firefox/addon/react-devtools/), and [Edge](https://microsoftedge.microsoft.com/addons/detail/react-developer-tools/gpphkfbcpidddadnkolkpfckpihlkkil) browser extension. Install it, and the *Components* tab will appear in your browser Developer Tools for sites using React.
## Completing the game[Link for Completing the game]()
By this point, you have all the basic building blocks for your tic-tac-toe game. To have a complete game, you now need to alternate placing “X”s and “O”s on the board, and you need a way to determine a winner.
### Lifting state up[Link for Lifting state up]()
Currently, each `Square` component maintains a part of the game’s state. To check for a winner in a tic-tac-toe game, the `Board` would need to somehow know the state of each of the 9 `Square` components.
How would you approach that? At first, you might guess that the `Board` needs to “ask” each `Square` for that `Square`’s state. Although this approach is technically possible in React, we discourage it because the code becomes difficult to understand, susceptible to bugs, and hard to refactor. Instead, the best approach is to store the game’s state in the parent `Board` component instead of in each `Square`. The `Board` component can tell each `Square` what to display by passing a prop, like you did when you passed a number to each Square.
**To collect data from multiple children, or to have two child components communicate with each other, declare the shared state in their parent component instead. The parent component can pass that state back down to the children via props. This keeps the child components in sync with each other and with their parent.**
Lifting state into a parent component is common when React components are refactored.
Let’s take this opportunity to try it out. Edit the `Board` component so that it declares a state variable named `squares` that defaults to an array of 9 nulls corresponding to the 9 squares:
```
// ...
export default function Board() {
const [squares, setSquares] = useState(Array(9).fill(null));
return (
// ...
);
}
```
`Array(9).fill(null)` creates an array with nine elements and sets each of them to `null`. The `useState()` call around it declares a `squares` state variable that’s initially set to that array. Each entry in the array corresponds to the value of a square. When you fill the board in later, the `squares` array will look like this:
```
['O', null, 'X', 'X', 'X', 'O', 'O', null, null]
```
Now your `Board` component needs to pass the `value` prop down to each `Square` that it renders:
```
export default function Board() {
const [squares, setSquares] = useState(Array(9).fill(null));
return (
<>
<div className="board-row">
<Square value={squares[0]} />
<Square value={squares[1]} />
<Square value={squares[2]} />
</div>
<div className="board-row">
<Square value={squares[3]} />
<Square value={squares[4]} />
<Square value={squares[5]} />
</div>
<div className="board-row">
<Square value={squares[6]} />
<Square value={squares[7]} />
<Square value={squares[8]} />
</div>
</>
);
}
```
Next, you’ll edit the `Square` component to receive the `value` prop from the Board component. This will require removing the Square component’s own stateful tracking of `value` and the button’s `onClick` prop:
```
function Square({value}) {
return <button className="square">{value}</button>;
}
```
At this point you should see an empty tic-tac-toe board:

And your code should look like this:
App.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import { useState } from 'react';
function Square({ value }) {
return <button className="square">{value}</button>;
}
export default function Board() {
const [squares, setSquares] = useState(Array(9).fill(null));
return (
<>
<div className="board-row">
<Square value={squares[0]} />
<Square value={squares[1]} />
<Square value={squares[2]} />
</div>
<div className="board-row">
<Square value={squares[3]} />
<Square value={squares[4]} />
<Square value={squares[5]} />
</div>
<div className="board-row">
<Square value={squares[6]} />
<Square value={squares[7]} />
<Square value={squares[8]} />
</div>
</>
);
}
```
Show more
Each Square will now receive a `value` prop that will either be `'X'`, `'O'`, or `null` for empty squares.
Next, you need to change what happens when a `Square` is clicked. The `Board` component now maintains which squares are filled. You’ll need to create a way for the `Square` to update the `Board`’s state. Since state is private to a component that defines it, you cannot update the `Board`’s state directly from `Square`.
Instead, you’ll pass down a function from the `Board` component to the `Square` component, and you’ll have `Square` call that function when a square is clicked. You’ll start with the function that the `Square` component will call when it is clicked. You’ll call that function `onSquareClick`:
```
function Square({ value }) {
return (
<button className="square" onClick={onSquareClick}>
{value}
</button>
);
}
```
Next, you’ll add the `onSquareClick` function to the `Square` component’s props:
```
function Square({ value, onSquareClick }) {
return (
<button className="square" onClick={onSquareClick}>
{value}
</button>
);
}
```
Now you’ll connect the `onSquareClick` prop to a function in the `Board` component that you’ll name `handleClick`. To connect `onSquareClick` to `handleClick` you’ll pass a function to the `onSquareClick` prop of the first `Square` component:
```
export default function Board() {
const [squares, setSquares] = useState(Array(9).fill(null));
return (
<>
<div className="board-row">
<Square value={squares[0]} onSquareClick={handleClick} />
//...
);
}
```
Lastly, you will define the `handleClick` function inside the Board component to update the `squares` array holding your board’s state:
```
export default function Board() {
const [squares, setSquares] = useState(Array(9).fill(null));
function handleClick() {
const nextSquares = squares.slice();
nextSquares[0] = "X";
setSquares(nextSquares);
}
return (
// ...
)
}
```
The `handleClick` function creates a copy of the `squares` array (`nextSquares`) with the JavaScript `slice()` Array method. Then, `handleClick` updates the `nextSquares` array to add `X` to the first (`[0]` index) square.
Calling the `setSquares` function lets React know the state of the component has changed. This will trigger a re-render of the components that use the `squares` state (`Board`) as well as its child components (the `Square` components that make up the board).
### Note
JavaScript supports [closures](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Closures) which means an inner function (e.g. `handleClick`) has access to variables and functions defined in an outer function (e.g. `Board`). The `handleClick` function can read the `squares` state and call the `setSquares` method because they are both defined inside of the `Board` function.
Now you can add X’s to the board… but only to the upper left square. Your `handleClick` function is hardcoded to update the index for the upper left square (`0`). Let’s update `handleClick` to be able to update any square. Add an argument `i` to the `handleClick` function that takes the index of the square to update:
```
export default function Board() {
const [squares, setSquares] = useState(Array(9).fill(null));
function handleClick(i) {
const nextSquares = squares.slice();
nextSquares[i] = "X";
setSquares(nextSquares);
}
return (
// ...
)
}
```
Next, you will need to pass that `i` to `handleClick`. You could try to set the `onSquareClick` prop of square to be `handleClick(0)` directly in the JSX like this, but it won’t work:
```
<Square value={squares[0]} onSquareClick={handleClick(0)} />
```
Here is why this doesn’t work. The `handleClick(0)` call will be a part of rendering the board component. Because `handleClick(0)` alters the state of the board component by calling `setSquares`, your entire board component will be re-rendered again. But this runs `handleClick(0)` again, leading to an infinite loop:
Console
Too many re-renders. React limits the number of renders to prevent an infinite loop.
Why didn’t this problem happen earlier?
When you were passing `onSquareClick={handleClick}`, you were passing the `handleClick` function down as a prop. You were not calling it! But now you are *calling* that function right away—notice the parentheses in `handleClick(0)`—and that’s why it runs too early. You don’t *want* to call `handleClick` until the user clicks!
You could fix this by creating a function like `handleFirstSquareClick` that calls `handleClick(0)`, a function like `handleSecondSquareClick` that calls `handleClick(1)`, and so on. You would pass (rather than call) these functions down as props like `onSquareClick={handleFirstSquareClick}`. This would solve the infinite loop.
However, defining nine different functions and giving each of them a name is too verbose. Instead, let’s do this:
```
export default function Board() {
// ...
return (
<>
<div className="board-row">
<Square value={squares[0]} onSquareClick={() => handleClick(0)} />
// ...
);
}
```
Notice the new `() =>` syntax. Here, `() => handleClick(0)` is an *arrow function,* which is a shorter way to define functions. When the square is clicked, the code after the `=>` “arrow” will run, calling `handleClick(0)`.
Now you need to update the other eight squares to call `handleClick` from the arrow functions you pass. Make sure that the argument for each call of the `handleClick` corresponds to the index of the correct square:
```
export default function Board() {
// ...
return (
<>
<div className="board-row">
<Square value={squares[0]} onSquareClick={() => handleClick(0)} />
<Square value={squares[1]} onSquareClick={() => handleClick(1)} />
<Square value={squares[2]} onSquareClick={() => handleClick(2)} />
</div>
<div className="board-row">
<Square value={squares[3]} onSquareClick={() => handleClick(3)} />
<Square value={squares[4]} onSquareClick={() => handleClick(4)} />
<Square value={squares[5]} onSquareClick={() => handleClick(5)} />
</div>
<div className="board-row">
<Square value={squares[6]} onSquareClick={() => handleClick(6)} />
<Square value={squares[7]} onSquareClick={() => handleClick(7)} />
<Square value={squares[8]} onSquareClick={() => handleClick(8)} />
</div>
</>
);
};
```
Now you can again add X’s to any square on the board by clicking on them:

But this time all the state management is handled by the `Board` component!
This is what your code should look like:
App.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import { useState } from 'react';
function Square({ value, onSquareClick }) {
return (
<button className="square" onClick={onSquareClick}>
{value}
</button>
);
}
export default function Board() {
const [squares, setSquares] = useState(Array(9).fill(null));
function handleClick(i) {
const nextSquares = squares.slice();
nextSquares[i] = 'X';
setSquares(nextSquares);
}
return (
<>
<div className="board-row">
<Square value={squares[0]} onSquareClick={() => handleClick(0)} />
<Square value={squares[1]} onSquareClick={() => handleClick(1)} />
<Square value={squares[2]} onSquareClick={() => handleClick(2)} />
</div>
<div className="board-row">
<Square value={squares[3]} onSquareClick={() => handleClick(3)} />
<Square value={squares[4]} onSquareClick={() => handleClick(4)} />
<Square value={squares[5]} onSquareClick={() => handleClick(5)} />
</div>
<div className="board-row">
<Square value={squares[6]} onSquareClick={() => handleClick(6)} />
<Square value={squares[7]} onSquareClick={() => handleClick(7)} />
<Square value={squares[8]} onSquareClick={() => handleClick(8)} />
</div>
</>
);
}
```
Show more
Now that your state handling is in the `Board` component, the parent `Board` component passes props to the child `Square` components so that they can be displayed correctly. When clicking on a `Square`, the child `Square` component now asks the parent `Board` component to update the state of the board. When the `Board`’s state changes, both the `Board` component and every child `Square` re-renders automatically. Keeping the state of all squares in the `Board` component will allow it to determine the winner in the future.
Let’s recap what happens when a user clicks the top left square on your board to add an `X` to it:
1. Clicking on the upper left square runs the function that the `button` received as its `onClick` prop from the `Square`. The `Square` component received that function as its `onSquareClick` prop from the `Board`. The `Board` component defined that function directly in the JSX. It calls `handleClick` with an argument of `0`.
2. `handleClick` uses the argument (`0`) to update the first element of the `squares` array from `null` to `X`.
3. The `squares` state of the `Board` component was updated, so the `Board` and all of its children re-render. This causes the `value` prop of the `Square` component with index `0` to change from `null` to `X`.
In the end the user sees that the upper left square has changed from empty to having a `X` after clicking it.
### Note
The DOM `<button>` element’s `onClick` attribute has a special meaning to React because it is a built-in component. For custom components like Square, the naming is up to you. You could give any name to the `Square`’s `onSquareClick` prop or `Board`’s `handleClick` function, and the code would work the same. In React, it’s conventional to use `onSomething` names for props which represent events and `handleSomething` for the function definitions which handle those events.
### Why immutability is important[Link for Why immutability is important]()
Note how in `handleClick`, you call `.slice()` to create a copy of the `squares` array instead of modifying the existing array. To explain why, we need to discuss immutability and why immutability is important to learn.
There are generally two approaches to changing data. The first approach is to *mutate* the data by directly changing the data’s values. The second approach is to replace the data with a new copy which has the desired changes. Here is what it would look like if you mutated the `squares` array:
```
const squares = [null, null, null, null, null, null, null, null, null];
squares[0] = 'X';
// Now `squares` is ["X", null, null, null, null, null, null, null, null];
```
And here is what it would look like if you changed data without mutating the `squares` array:
```
const squares = [null, null, null, null, null, null, null, null, null];
const nextSquares = ['X', null, null, null, null, null, null, null, null];
// Now `squares` is unchanged, but `nextSquares` first element is 'X' rather than `null`
```
The result is the same but by not mutating (changing the underlying data) directly, you gain several benefits.
Immutability makes complex features much easier to implement. Later in this tutorial, you will implement a “time travel” feature that lets you review the game’s history and “jump back” to past moves. This functionality isn’t specific to games—an ability to undo and redo certain actions is a common requirement for apps. Avoiding direct data mutation lets you keep previous versions of the data intact, and reuse them later.
There is also another benefit of immutability. By default, all child components re-render automatically when the state of a parent component changes. This includes even the child components that weren’t affected by the change. Although re-rendering is not by itself noticeable to the user (you shouldn’t actively try to avoid it!), you might want to skip re-rendering a part of the tree that clearly wasn’t affected by it for performance reasons. Immutability makes it very cheap for components to compare whether their data has changed or not. You can learn more about how React chooses when to re-render a component in [the `memo` API reference](https://react.dev/reference/react/memo).
### Taking turns[Link for Taking turns]()
It’s now time to fix a major defect in this tic-tac-toe game: the “O”s cannot be marked on the board.
You’ll set the first move to be “X” by default. Let’s keep track of this by adding another piece of state to the Board component:
```
function Board() {
const [xIsNext, setXIsNext] = useState(true);
const [squares, setSquares] = useState(Array(9).fill(null));
// ...
}
```
Each time a player moves, `xIsNext` (a boolean) will be flipped to determine which player goes next and the game’s state will be saved. You’ll update the `Board`’s `handleClick` function to flip the value of `xIsNext`:
```
export default function Board() {
const [xIsNext, setXIsNext] = useState(true);
const [squares, setSquares] = useState(Array(9).fill(null));
function handleClick(i) {
const nextSquares = squares.slice();
if (xIsNext) {
nextSquares[i] = "X";
} else {
nextSquares[i] = "O";
}
setSquares(nextSquares);
setXIsNext(!xIsNext);
}
return (
//...
);
}
```
Now, as you click on different squares, they will alternate between `X` and `O`, as they should!
But wait, there’s a problem. Try clicking on the same square multiple times:

The `X` is overwritten by an `O`! While this would add a very interesting twist to the game, we’re going to stick to the original rules for now.
When you mark a square with a `X` or an `O` you aren’t first checking to see if the square already has a `X` or `O` value. You can fix this by *returning early*. You’ll check to see if the square already has a `X` or an `O`. If the square is already filled, you will `return` in the `handleClick` function early—before it tries to update the board state.
```
function handleClick(i) {
if (squares[i]) {
return;
}
const nextSquares = squares.slice();
//...
}
```
Now you can only add `X`’s or `O`’s to empty squares! Here is what your code should look like at this point:
App.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import { useState } from 'react';
function Square({value, onSquareClick}) {
return (
<button className="square" onClick={onSquareClick}>
{value}
</button>
);
}
export default function Board() {
const [xIsNext, setXIsNext] = useState(true);
const [squares, setSquares] = useState(Array(9).fill(null));
function handleClick(i) {
if (squares[i]) {
return;
}
const nextSquares = squares.slice();
if (xIsNext) {
nextSquares[i] = 'X';
} else {
nextSquares[i] = 'O';
}
setSquares(nextSquares);
setXIsNext(!xIsNext);
}
return (
<>
<div className="board-row">
<Square value={squares[0]} onSquareClick={() => handleClick(0)} />
<Square value={squares[1]} onSquareClick={() => handleClick(1)} />
<Square value={squares[2]} onSquareClick={() => handleClick(2)} />
</div>
<div className="board-row">
<Square value={squares[3]} onSquareClick={() => handleClick(3)} />
<Square value={squares[4]} onSquareClick={() => handleClick(4)} />
<Square value={squares[5]} onSquareClick={() => handleClick(5)} />
</div>
<div className="board-row">
<Square value={squares[6]} onSquareClick={() => handleClick(6)} />
<Square value={squares[7]} onSquareClick={() => handleClick(7)} />
<Square value={squares[8]} onSquareClick={() => handleClick(8)} />
</div>
</>
);
}
```
Show more
### Declaring a winner[Link for Declaring a winner]()
Now that the players can take turns, you’ll want to show when the game is won and there are no more turns to make. To do this you’ll add a helper function called `calculateWinner` that takes an array of 9 squares, checks for a winner and returns `'X'`, `'O'`, or `null` as appropriate. Don’t worry too much about the `calculateWinner` function; it’s not specific to React:
```
export default function Board() {
//...
}
function calculateWinner(squares) {
const lines = [
[0, 1, 2],
[3, 4, 5],
[6, 7, 8],
[0, 3, 6],
[1, 4, 7],
[2, 5, 8],
[0, 4, 8],
[2, 4, 6]
];
for (let i = 0; i < lines.length; i++) {
const [a, b, c] = lines[i];
if (squares[a] && squares[a] === squares[b] && squares[a] === squares[c]) {
return squares[a];
}
}
return null;
}
```
### Note
It does not matter whether you define `calculateWinner` before or after the `Board`. Let’s put it at the end so that you don’t have to scroll past it every time you edit your components.
You will call `calculateWinner(squares)` in the `Board` component’s `handleClick` function to check if a player has won. You can perform this check at the same time you check if a user has clicked a square that already has a `X` or and `O`. We’d like to return early in both cases:
```
function handleClick(i) {
if (squares[i] || calculateWinner(squares)) {
return;
}
const nextSquares = squares.slice();
//...
}
```
To let the players know when the game is over, you can display text such as “Winner: X” or “Winner: O”. To do that you’ll add a `status` section to the `Board` component. The status will display the winner if the game is over and if the game is ongoing you’ll display which player’s turn is next:
```
export default function Board() {
// ...
const winner = calculateWinner(squares);
let status;
if (winner) {
status = "Winner: " + winner;
} else {
status = "Next player: " + (xIsNext ? "X" : "O");
}
return (
<>
<div className="status">{status}</div>
<div className="board-row">
// ...
)
}
```
Congratulations! You now have a working tic-tac-toe game. And you’ve just learned the basics of React too. So *you* are the real winner here. Here is what the code should look like:
App.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import { useState } from 'react';
function Square({value, onSquareClick}) {
return (
<button className="square" onClick={onSquareClick}>
{value}
</button>
);
}
export default function Board() {
const [xIsNext, setXIsNext] = useState(true);
const [squares, setSquares] = useState(Array(9).fill(null));
function handleClick(i) {
if (calculateWinner(squares) || squares[i]) {
return;
}
const nextSquares = squares.slice();
if (xIsNext) {
nextSquares[i] = 'X';
} else {
nextSquares[i] = 'O';
}
setSquares(nextSquares);
setXIsNext(!xIsNext);
}
const winner = calculateWinner(squares);
let status;
if (winner) {
status = 'Winner: ' + winner;
} else {
status = 'Next player: ' + (xIsNext ? 'X' : 'O');
}
return (
<>
<div className="status">{status}</div>
<div className="board-row">
<Square value={squares[0]} onSquareClick={() => handleClick(0)} />
<Square value={squares[1]} onSquareClick={() => handleClick(1)} />
<Square value={squares[2]} onSquareClick={() => handleClick(2)} />
</div>
<div className="board-row">
<Square value={squares[3]} onSquareClick={() => handleClick(3)} />
<Square value={squares[4]} onSquareClick={() => handleClick(4)} />
<Square value={squares[5]} onSquareClick={() => handleClick(5)} />
</div>
<div className="board-row">
<Square value={squares[6]} onSquareClick={() => handleClick(6)} />
<Square value={squares[7]} onSquareClick={() => handleClick(7)} />
<Square value={squares[8]} onSquareClick={() => handleClick(8)} />
</div>
</>
);
}
function calculateWinner(squares) {
const lines = [
[0, 1, 2],
[3, 4, 5],
[6, 7, 8],
[0, 3, 6],
[1, 4, 7],
[2, 5, 8],
[0, 4, 8],
[2, 4, 6],
];
for (let i = 0; i < lines.length; i++) {
const [a, b, c] = lines[i];
if (squares[a] && squares[a] === squares[b] && squares[a] === squares[c]) {
return squares[a];
}
}
return null;
}
```
Show more
## Adding time travel[Link for Adding time travel]()
As a final exercise, let’s make it possible to “go back in time” to the previous moves in the game.
### Storing a history of moves[Link for Storing a history of moves]()
If you mutated the `squares` array, implementing time travel would be very difficult.
However, you used `slice()` to create a new copy of the `squares` array after every move, and treated it as immutable. This will allow you to store every past version of the `squares` array, and navigate between the turns that have already happened.
You’ll store the past `squares` arrays in another array called `history`, which you’ll store as a new state variable. The `history` array represents all board states, from the first to the last move, and has a shape like this:
```
[
// Before first move
[null, null, null, null, null, null, null, null, null],
// After first move
[null, null, null, null, 'X', null, null, null, null],
// After second move
[null, null, null, null, 'X', null, null, null, 'O'],
// ...
]
```
### Lifting state up, again[Link for Lifting state up, again]()
You will now write a new top-level component called `Game` to display a list of past moves. That’s where you will place the `history` state that contains the entire game history.
Placing the `history` state into the `Game` component will let you remove the `squares` state from its child `Board` component. Just like you “lifted state up” from the `Square` component into the `Board` component, you will now lift it up from the `Board` into the top-level `Game` component. This gives the `Game` component full control over the `Board`’s data and lets it instruct the `Board` to render previous turns from the `history`.
First, add a `Game` component with `export default`. Have it render the `Board` component and some markup:
```
function Board() {
// ...
}
export default function Game() {
return (
<div className="game">
<div className="game-board">
<Board />
</div>
<div className="game-info">
<ol>{/*TODO*/}</ol>
</div>
</div>
);
}
```
Note that you are removing the `export default` keywords before the `function Board() {` declaration and adding them before the `function Game() {` declaration. This tells your `index.js` file to use the `Game` component as the top-level component instead of your `Board` component. The additional `div`s returned by the `Game` component are making room for the game information you’ll add to the board later.
Add some state to the `Game` component to track which player is next and the history of moves:
```
export default function Game() {
const [xIsNext, setXIsNext] = useState(true);
const [history, setHistory] = useState([Array(9).fill(null)]);
// ...
```
Notice how `[Array(9).fill(null)]` is an array with a single item, which itself is an array of 9 `null`s.
To render the squares for the current move, you’ll want to read the last squares array from the `history`. You don’t need `useState` for this—you already have enough information to calculate it during rendering:
```
export default function Game() {
const [xIsNext, setXIsNext] = useState(true);
const [history, setHistory] = useState([Array(9).fill(null)]);
const currentSquares = history[history.length - 1];
// ...
```
Next, create a `handlePlay` function inside the `Game` component that will be called by the `Board` component to update the game. Pass `xIsNext`, `currentSquares` and `handlePlay` as props to the `Board` component:
```
export default function Game() {
const [xIsNext, setXIsNext] = useState(true);
const [history, setHistory] = useState([Array(9).fill(null)]);
const currentSquares = history[history.length - 1];
function handlePlay(nextSquares) {
// TODO
}
return (
<div className="game">
<div className="game-board">
<Board xIsNext={xIsNext} squares={currentSquares} onPlay={handlePlay} />
//...
)
}
```
Let’s make the `Board` component fully controlled by the props it receives. Change the `Board` component to take three props: `xIsNext`, `squares`, and a new `onPlay` function that `Board` can call with the updated squares array when a player makes a move. Next, remove the first two lines of the `Board` function that call `useState`:
```
function Board({ xIsNext, squares, onPlay }) {
function handleClick(i) {
//...
}
// ...
}
```
Now replace the `setSquares` and `setXIsNext` calls in `handleClick` in the `Board` component with a single call to your new `onPlay` function so the `Game` component can update the `Board` when the user clicks a square:
```
function Board({ xIsNext, squares, onPlay }) {
function handleClick(i) {
if (calculateWinner(squares) || squares[i]) {
return;
}
const nextSquares = squares.slice();
if (xIsNext) {
nextSquares[i] = "X";
} else {
nextSquares[i] = "O";
}
onPlay(nextSquares);
}
//...
}
```
The `Board` component is fully controlled by the props passed to it by the `Game` component. You need to implement the `handlePlay` function in the `Game` component to get the game working again.
What should `handlePlay` do when called? Remember that Board used to call `setSquares` with an updated array; now it passes the updated `squares` array to `onPlay`.
The `handlePlay` function needs to update `Game`’s state to trigger a re-render, but you don’t have a `setSquares` function that you can call any more—you’re now using the `history` state variable to store this information. You’ll want to update `history` by appending the updated `squares` array as a new history entry. You also want to toggle `xIsNext`, just as Board used to do:
```
export default function Game() {
//...
function handlePlay(nextSquares) {
setHistory([...history, nextSquares]);
setXIsNext(!xIsNext);
}
//...
}
```
Here, `[...history, nextSquares]` creates a new array that contains all the items in `history`, followed by `nextSquares`. (You can read the `...history` [*spread syntax*](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Operators/Spread_syntax) as “enumerate all the items in `history`”.)
For example, if `history` is `[[null,null,null], ["X",null,null]]` and `nextSquares` is `["X",null,"O"]`, then the new `[...history, nextSquares]` array will be `[[null,null,null], ["X",null,null], ["X",null,"O"]]`.
At this point, you’ve moved the state to live in the `Game` component, and the UI should be fully working, just as it was before the refactor. Here is what the code should look like at this point:
App.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import { useState } from 'react';
function Square({ value, onSquareClick }) {
return (
<button className="square" onClick={onSquareClick}>
{value}
</button>
);
}
function Board({ xIsNext, squares, onPlay }) {
function handleClick(i) {
if (calculateWinner(squares) || squares[i]) {
return;
}
const nextSquares = squares.slice();
if (xIsNext) {
nextSquares[i] = 'X';
} else {
nextSquares[i] = 'O';
}
onPlay(nextSquares);
}
const winner = calculateWinner(squares);
let status;
if (winner) {
status = 'Winner: ' + winner;
} else {
status = 'Next player: ' + (xIsNext ? 'X' : 'O');
}
return (
<>
<div className="status">{status}</div>
<div className="board-row">
<Square value={squares[0]} onSquareClick={() => handleClick(0)} />
<Square value={squares[1]} onSquareClick={() => handleClick(1)} />
<Square value={squares[2]} onSquareClick={() => handleClick(2)} />
</div>
<div className="board-row">
<Square value={squares[3]} onSquareClick={() => handleClick(3)} />
<Square value={squares[4]} onSquareClick={() => handleClick(4)} />
<Square value={squares[5]} onSquareClick={() => handleClick(5)} />
</div>
<div className="board-row">
<Square value={squares[6]} onSquareClick={() => handleClick(6)} />
<Square value={squares[7]} onSquareClick={() => handleClick(7)} />
<Square value={squares[8]} onSquareClick={() => handleClick(8)} />
</div>
</>
);
}
export default function Game() {
const [xIsNext, setXIsNext] = useState(true);
const [history, setHistory] = useState([Array(9).fill(null)]);
const currentSquares = history[history.length - 1];
function handlePlay(nextSquares) {
setHistory([...history, nextSquares]);
setXIsNext(!xIsNext);
}
return (
<div className="game">
<div className="game-board">
<Board xIsNext={xIsNext} squares={currentSquares} onPlay={handlePlay} />
</div>
<div className="game-info">
<ol>{/*TODO*/}</ol>
</div>
</div>
);
}
function calculateWinner(squares) {
const lines = [
[0, 1, 2],
[3, 4, 5],
[6, 7, 8],
[0, 3, 6],
[1, 4, 7],
[2, 5, 8],
[0, 4, 8],
[2, 4, 6],
];
for (let i = 0; i < lines.length; i++) {
const [a, b, c] = lines[i];
if (squares[a] && squares[a] === squares[b] && squares[a] === squares[c]) {
return squares[a];
}
}
return null;
}
```
Show more
### Showing the past moves[Link for Showing the past moves]()
Since you are recording the tic-tac-toe game’s history, you can now display a list of past moves to the player.
React elements like `<button>` are regular JavaScript objects; you can pass them around in your application. To render multiple items in React, you can use an array of React elements.
You already have an array of `history` moves in state, so now you need to transform it to an array of React elements. In JavaScript, to transform one array into another, you can use the [array `map` method:](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Array/map)
```
[1, 2, 3].map((x) => x * 2) // [2, 4, 6]
```
You’ll use `map` to transform your `history` of moves into React elements representing buttons on the screen, and display a list of buttons to “jump” to past moves. Let’s `map` over the `history` in the Game component:
```
export default function Game() {
const [xIsNext, setXIsNext] = useState(true);
const [history, setHistory] = useState([Array(9).fill(null)]);
const currentSquares = history[history.length - 1];
function handlePlay(nextSquares) {
setHistory([...history, nextSquares]);
setXIsNext(!xIsNext);
}
function jumpTo(nextMove) {
// TODO
}
const moves = history.map((squares, move) => {
let description;
if (move > 0) {
description = 'Go to move #' + move;
} else {
description = 'Go to game start';
}
return (
<li>
<button onClick={() => jumpTo(move)}>{description}</button>
</li>
);
});
return (
<div className="game">
<div className="game-board">
<Board xIsNext={xIsNext} squares={currentSquares} onPlay={handlePlay} />
</div>
<div className="game-info">
<ol>{moves}</ol>
</div>
</div>
);
}
```
You can see what your code should look like below. Note that you should see an error in the developer tools console that says:
Console
Warning: Each child in an array or iterator should have a unique “key” prop. Check the render method of \`Game\`.
You’ll fix this error in the next section.
App.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import { useState } from 'react';
function Square({ value, onSquareClick }) {
return (
<button className="square" onClick={onSquareClick}>
{value}
</button>
);
}
function Board({ xIsNext, squares, onPlay }) {
function handleClick(i) {
if (calculateWinner(squares) || squares[i]) {
return;
}
const nextSquares = squares.slice();
if (xIsNext) {
nextSquares[i] = 'X';
} else {
nextSquares[i] = 'O';
}
onPlay(nextSquares);
}
const winner = calculateWinner(squares);
let status;
if (winner) {
status = 'Winner: ' + winner;
} else {
status = 'Next player: ' + (xIsNext ? 'X' : 'O');
}
return (
<>
<div className="status">{status}</div>
<div className="board-row">
<Square value={squares[0]} onSquareClick={() => handleClick(0)} />
<Square value={squares[1]} onSquareClick={() => handleClick(1)} />
<Square value={squares[2]} onSquareClick={() => handleClick(2)} />
</div>
<div className="board-row">
<Square value={squares[3]} onSquareClick={() => handleClick(3)} />
<Square value={squares[4]} onSquareClick={() => handleClick(4)} />
<Square value={squares[5]} onSquareClick={() => handleClick(5)} />
</div>
<div className="board-row">
<Square value={squares[6]} onSquareClick={() => handleClick(6)} />
<Square value={squares[7]} onSquareClick={() => handleClick(7)} />
<Square value={squares[8]} onSquareClick={() => handleClick(8)} />
</div>
</>
);
}
export default function Game() {
const [xIsNext, setXIsNext] = useState(true);
const [history, setHistory] = useState([Array(9).fill(null)]);
const currentSquares = history[history.length - 1];
function handlePlay(nextSquares) {
setHistory([...history, nextSquares]);
setXIsNext(!xIsNext);
}
function jumpTo(nextMove) {
// TODO
}
const moves = history.map((squares, move) => {
let description;
if (move > 0) {
description = 'Go to move #' + move;
} else {
description = 'Go to game start';
}
return (
<li>
<button onClick={() => jumpTo(move)}>{description}</button>
</li>
);
});
return (
<div className="game">
<div className="game-board">
<Board xIsNext={xIsNext} squares={currentSquares} onPlay={handlePlay} />
</div>
<div className="game-info">
<ol>{moves}</ol>
</div>
</div>
);
}
function calculateWinner(squares) {
const lines = [
[0, 1, 2],
[3, 4, 5],
[6, 7, 8],
[0, 3, 6],
[1, 4, 7],
[2, 5, 8],
[0, 4, 8],
[2, 4, 6],
];
for (let i = 0; i < lines.length; i++) {
const [a, b, c] = lines[i];
if (squares[a] && squares[a] === squares[b] && squares[a] === squares[c]) {
return squares[a];
}
}
return null;
}
```
Show more
As you iterate through `history` array inside the function you passed to `map`, the `squares` argument goes through each element of `history`, and the `move` argument goes through each array index: `0`, `1`, `2`, …. (In most cases, you’d need the actual array elements, but to render a list of moves you will only need indexes.)
For each move in the tic-tac-toe game’s history, you create a list item `<li>` which contains a button `<button>`. The button has an `onClick` handler which calls a function called `jumpTo` (that you haven’t implemented yet).
For now, you should see a list of the moves that occurred in the game and an error in the developer tools console. Let’s discuss what the “key” error means.
### Picking a key[Link for Picking a key]()
When you render a list, React stores some information about each rendered list item. When you update a list, React needs to determine what has changed. You could have added, removed, re-arranged, or updated the list’s items.
Imagine transitioning from
```
<li>Alexa: 7 tasks left</li>
<li>Ben: 5 tasks left</li>
```
to
```
<li>Ben: 9 tasks left</li>
<li>Claudia: 8 tasks left</li>
<li>Alexa: 5 tasks left</li>
```
In addition to the updated counts, a human reading this would probably say that you swapped Alexa and Ben’s ordering and inserted Claudia between Alexa and Ben. However, React is a computer program and does not know what you intended, so you need to specify a *key* property for each list item to differentiate each list item from its siblings. If your data was from a database, Alexa, Ben, and Claudia’s database IDs could be used as keys.
```
<li key={user.id}>
{user.name}: {user.taskCount} tasks left
</li>
```
When a list is re-rendered, React takes each list item’s key and searches the previous list’s items for a matching key. If the current list has a key that didn’t exist before, React creates a component. If the current list is missing a key that existed in the previous list, React destroys the previous component. If two keys match, the corresponding component is moved.
Keys tell React about the identity of each component, which allows React to maintain state between re-renders. If a component’s key changes, the component will be destroyed and re-created with a new state.
`key` is a special and reserved property in React. When an element is created, React extracts the `key` property and stores the key directly on the returned element. Even though `key` may look like it is passed as props, React automatically uses `key` to decide which components to update. There’s no way for a component to ask what `key` its parent specified.
**It’s strongly recommended that you assign proper keys whenever you build dynamic lists.** If you don’t have an appropriate key, you may want to consider restructuring your data so that you do.
If no key is specified, React will report an error and use the array index as a key by default. Using the array index as a key is problematic when trying to re-order a list’s items or inserting/removing list items. Explicitly passing `key={i}` silences the error but has the same problems as array indices and is not recommended in most cases.
Keys do not need to be globally unique; they only need to be unique between components and their siblings.
### Implementing time travel[Link for Implementing time travel]()
In the tic-tac-toe game’s history, each past move has a unique ID associated with it: it’s the sequential number of the move. Moves will never be re-ordered, deleted, or inserted in the middle, so it’s safe to use the move index as a key.
In the `Game` function, you can add the key as `<li key={move}>`, and if you reload the rendered game, React’s “key” error should disappear:
```
const moves = history.map((squares, move) => {
//...
return (
<li key={move}>
<button onClick={() => jumpTo(move)}>{description}</button>
</li>
);
});
```
App.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import { useState } from 'react';
function Square({ value, onSquareClick }) {
return (
<button className="square" onClick={onSquareClick}>
{value}
</button>
);
}
function Board({ xIsNext, squares, onPlay }) {
function handleClick(i) {
if (calculateWinner(squares) || squares[i]) {
return;
}
const nextSquares = squares.slice();
if (xIsNext) {
nextSquares[i] = 'X';
} else {
nextSquares[i] = 'O';
}
onPlay(nextSquares);
}
const winner = calculateWinner(squares);
let status;
if (winner) {
status = 'Winner: ' + winner;
} else {
status = 'Next player: ' + (xIsNext ? 'X' : 'O');
}
return (
<>
<div className="status">{status}</div>
<div className="board-row">
<Square value={squares[0]} onSquareClick={() => handleClick(0)} />
<Square value={squares[1]} onSquareClick={() => handleClick(1)} />
<Square value={squares[2]} onSquareClick={() => handleClick(2)} />
</div>
<div className="board-row">
<Square value={squares[3]} onSquareClick={() => handleClick(3)} />
<Square value={squares[4]} onSquareClick={() => handleClick(4)} />
<Square value={squares[5]} onSquareClick={() => handleClick(5)} />
</div>
<div className="board-row">
<Square value={squares[6]} onSquareClick={() => handleClick(6)} />
<Square value={squares[7]} onSquareClick={() => handleClick(7)} />
<Square value={squares[8]} onSquareClick={() => handleClick(8)} />
</div>
</>
);
}
export default function Game() {
const [xIsNext, setXIsNext] = useState(true);
const [history, setHistory] = useState([Array(9).fill(null)]);
const currentSquares = history[history.length - 1];
function handlePlay(nextSquares) {
setHistory([...history, nextSquares]);
setXIsNext(!xIsNext);
}
function jumpTo(nextMove) {
// TODO
}
const moves = history.map((squares, move) => {
let description;
if (move > 0) {
description = 'Go to move #' + move;
} else {
description = 'Go to game start';
}
return (
<li key={move}>
<button onClick={() => jumpTo(move)}>{description}</button>
</li>
);
});
return (
<div className="game">
<div className="game-board">
<Board xIsNext={xIsNext} squares={currentSquares} onPlay={handlePlay} />
</div>
<div className="game-info">
<ol>{moves}</ol>
</div>
</div>
);
}
function calculateWinner(squares) {
const lines = [
[0, 1, 2],
[3, 4, 5],
[6, 7, 8],
[0, 3, 6],
[1, 4, 7],
[2, 5, 8],
[0, 4, 8],
[2, 4, 6],
];
for (let i = 0; i < lines.length; i++) {
const [a, b, c] = lines[i];
if (squares[a] && squares[a] === squares[b] && squares[a] === squares[c]) {
return squares[a];
}
}
return null;
}
```
Show more
Before you can implement `jumpTo`, you need the `Game` component to keep track of which step the user is currently viewing. To do this, define a new state variable called `currentMove`, defaulting to `0`:
```
export default function Game() {
const [xIsNext, setXIsNext] = useState(true);
const [history, setHistory] = useState([Array(9).fill(null)]);
const [currentMove, setCurrentMove] = useState(0);
const currentSquares = history[history.length - 1];
//...
}
```
Next, update the `jumpTo` function inside `Game` to update that `currentMove`. You’ll also set `xIsNext` to `true` if the number that you’re changing `currentMove` to is even.
```
export default function Game() {
// ...
function jumpTo(nextMove) {
setCurrentMove(nextMove);
setXIsNext(nextMove % 2 === 0);
}
//...
}
```
You will now make two changes to the `Game`’s `handlePlay` function which is called when you click on a square.
- If you “go back in time” and then make a new move from that point, you only want to keep the history up to that point. Instead of adding `nextSquares` after all items (`...` spread syntax) in `history`, you’ll add it after all items in `history.slice(0, currentMove + 1)` so that you’re only keeping that portion of the old history.
- Each time a move is made, you need to update `currentMove` to point to the latest history entry.
```
function handlePlay(nextSquares) {
const nextHistory = [...history.slice(0, currentMove + 1), nextSquares];
setHistory(nextHistory);
setCurrentMove(nextHistory.length - 1);
setXIsNext(!xIsNext);
}
```
Finally, you will modify the `Game` component to render the currently selected move, instead of always rendering the final move:
```
export default function Game() {
const [xIsNext, setXIsNext] = useState(true);
const [history, setHistory] = useState([Array(9).fill(null)]);
const [currentMove, setCurrentMove] = useState(0);
const currentSquares = history[currentMove];
// ...
}
```
If you click on any step in the game’s history, the tic-tac-toe board should immediately update to show what the board looked like after that step occurred.
App.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import { useState } from 'react';
function Square({value, onSquareClick}) {
return (
<button className="square" onClick={onSquareClick}>
{value}
</button>
);
}
function Board({ xIsNext, squares, onPlay }) {
function handleClick(i) {
if (calculateWinner(squares) || squares[i]) {
return;
}
const nextSquares = squares.slice();
if (xIsNext) {
nextSquares[i] = 'X';
} else {
nextSquares[i] = 'O';
}
onPlay(nextSquares);
}
const winner = calculateWinner(squares);
let status;
if (winner) {
status = 'Winner: ' + winner;
} else {
status = 'Next player: ' + (xIsNext ? 'X' : 'O');
}
return (
<>
<div className="status">{status}</div>
<div className="board-row">
<Square value={squares[0]} onSquareClick={() => handleClick(0)} />
<Square value={squares[1]} onSquareClick={() => handleClick(1)} />
<Square value={squares[2]} onSquareClick={() => handleClick(2)} />
</div>
<div className="board-row">
<Square value={squares[3]} onSquareClick={() => handleClick(3)} />
<Square value={squares[4]} onSquareClick={() => handleClick(4)} />
<Square value={squares[5]} onSquareClick={() => handleClick(5)} />
</div>
<div className="board-row">
<Square value={squares[6]} onSquareClick={() => handleClick(6)} />
<Square value={squares[7]} onSquareClick={() => handleClick(7)} />
<Square value={squares[8]} onSquareClick={() => handleClick(8)} />
</div>
</>
);
}
export default function Game() {
const [xIsNext, setXIsNext] = useState(true);
const [history, setHistory] = useState([Array(9).fill(null)]);
const [currentMove, setCurrentMove] = useState(0);
const currentSquares = history[currentMove];
function handlePlay(nextSquares) {
const nextHistory = [...history.slice(0, currentMove + 1), nextSquares];
setHistory(nextHistory);
setCurrentMove(nextHistory.length - 1);
setXIsNext(!xIsNext);
}
function jumpTo(nextMove) {
setCurrentMove(nextMove);
setXIsNext(nextMove % 2 === 0);
}
const moves = history.map((squares, move) => {
let description;
if (move > 0) {
description = 'Go to move #' + move;
} else {
description = 'Go to game start';
}
return (
<li key={move}>
<button onClick={() => jumpTo(move)}>{description}</button>
</li>
);
});
return (
<div className="game">
<div className="game-board">
<Board xIsNext={xIsNext} squares={currentSquares} onPlay={handlePlay} />
</div>
<div className="game-info">
<ol>{moves}</ol>
</div>
</div>
);
}
function calculateWinner(squares) {
const lines = [
[0, 1, 2],
[3, 4, 5],
[6, 7, 8],
[0, 3, 6],
[1, 4, 7],
[2, 5, 8],
[0, 4, 8],
[2, 4, 6],
];
for (let i = 0; i < lines.length; i++) {
const [a, b, c] = lines[i];
if (squares[a] && squares[a] === squares[b] && squares[a] === squares[c]) {
return squares[a];
}
}
return null;
}
```
Show more
### Final cleanup[Link for Final cleanup]()
If you look at the code very closely, you may notice that `xIsNext === true` when `currentMove` is even and `xIsNext === false` when `currentMove` is odd. In other words, if you know the value of `currentMove`, then you can always figure out what `xIsNext` should be.
There’s no reason for you to store both of these in state. In fact, always try to avoid redundant state. Simplifying what you store in state reduces bugs and makes your code easier to understand. Change `Game` so that it doesn’t store `xIsNext` as a separate state variable and instead figures it out based on the `currentMove`:
```
export default function Game() {
const [history, setHistory] = useState([Array(9).fill(null)]);
const [currentMove, setCurrentMove] = useState(0);
const xIsNext = currentMove % 2 === 0;
const currentSquares = history[currentMove];
function handlePlay(nextSquares) {
const nextHistory = [...history.slice(0, currentMove + 1), nextSquares];
setHistory(nextHistory);
setCurrentMove(nextHistory.length - 1);
}
function jumpTo(nextMove) {
setCurrentMove(nextMove);
}
// ...
}
```
You no longer need the `xIsNext` state declaration or the calls to `setXIsNext`. Now, there’s no chance for `xIsNext` to get out of sync with `currentMove`, even if you make a mistake while coding the components.
### Wrapping up[Link for Wrapping up]()
Congratulations! You’ve created a tic-tac-toe game that:
- Lets you play tic-tac-toe,
- Indicates when a player has won the game,
- Stores a game’s history as a game progresses,
- Allows players to review a game’s history and see previous versions of a game’s board.
Nice work! We hope you now feel like you have a decent grasp of how React works.
Check out the final result here:
App.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import { useState } from 'react';
function Square({ value, onSquareClick }) {
return (
<button className="square" onClick={onSquareClick}>
{value}
</button>
);
}
function Board({ xIsNext, squares, onPlay }) {
function handleClick(i) {
if (calculateWinner(squares) || squares[i]) {
return;
}
const nextSquares = squares.slice();
if (xIsNext) {
nextSquares[i] = 'X';
} else {
nextSquares[i] = 'O';
}
onPlay(nextSquares);
}
const winner = calculateWinner(squares);
let status;
if (winner) {
status = 'Winner: ' + winner;
} else {
status = 'Next player: ' + (xIsNext ? 'X' : 'O');
}
return (
<>
<div className="status">{status}</div>
<div className="board-row">
<Square value={squares[0]} onSquareClick={() => handleClick(0)} />
<Square value={squares[1]} onSquareClick={() => handleClick(1)} />
<Square value={squares[2]} onSquareClick={() => handleClick(2)} />
</div>
<div className="board-row">
<Square value={squares[3]} onSquareClick={() => handleClick(3)} />
<Square value={squares[4]} onSquareClick={() => handleClick(4)} />
<Square value={squares[5]} onSquareClick={() => handleClick(5)} />
</div>
<div className="board-row">
<Square value={squares[6]} onSquareClick={() => handleClick(6)} />
<Square value={squares[7]} onSquareClick={() => handleClick(7)} />
<Square value={squares[8]} onSquareClick={() => handleClick(8)} />
</div>
</>
);
}
export default function Game() {
const [history, setHistory] = useState([Array(9).fill(null)]);
const [currentMove, setCurrentMove] = useState(0);
const xIsNext = currentMove % 2 === 0;
const currentSquares = history[currentMove];
function handlePlay(nextSquares) {
const nextHistory = [...history.slice(0, currentMove + 1), nextSquares];
setHistory(nextHistory);
setCurrentMove(nextHistory.length - 1);
}
function jumpTo(nextMove) {
setCurrentMove(nextMove);
}
const moves = history.map((squares, move) => {
let description;
if (move > 0) {
description = 'Go to move #' + move;
} else {
description = 'Go to game start';
}
return (
<li key={move}>
<button onClick={() => jumpTo(move)}>{description}</button>
</li>
);
});
return (
<div className="game">
<div className="game-board">
<Board xIsNext={xIsNext} squares={currentSquares} onPlay={handlePlay} />
</div>
<div className="game-info">
<ol>{moves}</ol>
</div>
</div>
);
}
function calculateWinner(squares) {
const lines = [
[0, 1, 2],
[3, 4, 5],
[6, 7, 8],
[0, 3, 6],
[1, 4, 7],
[2, 5, 8],
[0, 4, 8],
[2, 4, 6],
];
for (let i = 0; i < lines.length; i++) {
const [a, b, c] = lines[i];
if (squares[a] && squares[a] === squares[b] && squares[a] === squares[c]) {
return squares[a];
}
}
return null;
}
```
Show more
If you have extra time or want to practice your new React skills, here are some ideas for improvements that you could make to the tic-tac-toe game, listed in order of increasing difficulty:
1. For the current move only, show “You are at move #…” instead of a button.
2. Rewrite `Board` to use two loops to make the squares instead of hardcoding them.
3. Add a toggle button that lets you sort the moves in either ascending or descending order.
4. When someone wins, highlight the three squares that caused the win (and when no one wins, display a message about the result being a draw).
5. Display the location for each move in the format (row, col) in the move history list.
Throughout this tutorial, you’ve touched on React concepts including elements, components, props, and state. Now that you’ve seen how these concepts work when building a game, check out [Thinking in React](https://react.dev/learn/thinking-in-react) to see how the same React concepts work when building an app’s UI.
[PreviousQuick Start](https://react.dev/learn)
[NextThinking in React](https://react.dev/learn/thinking-in-react) |
https://react.dev/learn/conditional-rendering | [Learn React](https://react.dev/learn)
[Describing the UI](https://react.dev/learn/describing-the-ui)
# Conditional Rendering[Link for this heading]()
Your components will often need to display different things depending on different conditions. In React, you can conditionally render JSX using JavaScript syntax like `if` statements, `&&`, and `? :` operators.
### You will learn
- How to return different JSX depending on a condition
- How to conditionally include or exclude a piece of JSX
- Common conditional syntax shortcuts you’ll encounter in React codebases
## Conditionally returning JSX[Link for Conditionally returning JSX]()
Let’s say you have a `PackingList` component rendering several `Item`s, which can be marked as packed or not:
App.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
function Item({ name, isPacked }) {
return <li className="item">{name}</li>;
}
export default function PackingList() {
return (
<section>
<h1>Sally Ride's Packing List</h1>
<ul>
<Item
isPacked={true}
name="Space suit"
/>
<Item
isPacked={true}
name="Helmet with a golden leaf"
/>
<Item
isPacked={false}
name="Photo of Tam"
/>
</ul>
</section>
);
}
```
Show more
Notice that some of the `Item` components have their `isPacked` prop set to `true` instead of `false`. You want to add a checkmark (✅) to packed items if `isPacked={true}`.
You can write this as an [`if`/`else` statement](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Statements/if...else) like so:
```
if (isPacked) {
return <li className="item">{name} ✅</li>;
}
return <li className="item">{name}</li>;
```
If the `isPacked` prop is `true`, this code **returns a different JSX tree.** With this change, some of the items get a checkmark at the end:
App.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
function Item({ name, isPacked }) {
if (isPacked) {
return <li className="item">{name} ✅</li>;
}
return <li className="item">{name}</li>;
}
export default function PackingList() {
return (
<section>
<h1>Sally Ride's Packing List</h1>
<ul>
<Item
isPacked={true}
name="Space suit"
/>
<Item
isPacked={true}
name="Helmet with a golden leaf"
/>
<Item
isPacked={false}
name="Photo of Tam"
/>
</ul>
</section>
);
}
```
Show more
Try editing what gets returned in either case, and see how the result changes!
Notice how you’re creating branching logic with JavaScript’s `if` and `return` statements. In React, control flow (like conditions) is handled by JavaScript.
### Conditionally returning nothing with `null`[Link for this heading]()
In some situations, you won’t want to render anything at all. For example, say you don’t want to show packed items at all. A component must return something. In this case, you can return `null`:
```
if (isPacked) {
return null;
}
return <li className="item">{name}</li>;
```
If `isPacked` is true, the component will return nothing, `null`. Otherwise, it will return JSX to render.
App.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
function Item({ name, isPacked }) {
if (isPacked) {
return null;
}
return <li className="item">{name}</li>;
}
export default function PackingList() {
return (
<section>
<h1>Sally Ride's Packing List</h1>
<ul>
<Item
isPacked={true}
name="Space suit"
/>
<Item
isPacked={true}
name="Helmet with a golden leaf"
/>
<Item
isPacked={false}
name="Photo of Tam"
/>
</ul>
</section>
);
}
```
Show more
In practice, returning `null` from a component isn’t common because it might surprise a developer trying to render it. More often, you would conditionally include or exclude the component in the parent component’s JSX. Here’s how to do that!
## Conditionally including JSX[Link for Conditionally including JSX]()
In the previous example, you controlled which (if any!) JSX tree would be returned by the component. You may already have noticed some duplication in the render output:
```
<li className="item">{name} ✅</li>
```
is very similar to
```
<li className="item">{name}</li>
```
Both of the conditional branches return `<li className="item">...</li>`:
```
if (isPacked) {
return <li className="item">{name} ✅</li>;
}
return <li className="item">{name}</li>;
```
While this duplication isn’t harmful, it could make your code harder to maintain. What if you want to change the `className`? You’d have to do it in two places in your code! In such a situation, you could conditionally include a little JSX to make your code more [DRY.](https://en.wikipedia.org/wiki/Don%27t_repeat_yourself)
### Conditional (ternary) operator (`? :`)[Link for this heading]()
JavaScript has a compact syntax for writing a conditional expression — the [conditional operator](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Operators/Conditional_Operator) or “ternary operator”.
Instead of this:
```
if (isPacked) {
return <li className="item">{name} ✅</li>;
}
return <li className="item">{name}</li>;
```
You can write this:
```
return (
<li className="item">
{isPacked ? name + ' ✅' : name}
</li>
);
```
You can read it as *“if `isPacked` is true, then (`?`) render `name + ' ✅'`, otherwise (`:`) render `name`”*.
##### Deep Dive
#### Are these two examples fully equivalent?[Link for Are these two examples fully equivalent?]()
Show Details
If you’re coming from an object-oriented programming background, you might assume that the two examples above are subtly different because one of them may create two different “instances” of `<li>`. But JSX elements aren’t “instances” because they don’t hold any internal state and aren’t real DOM nodes. They’re lightweight descriptions, like blueprints. So these two examples, in fact, *are* completely equivalent. [Preserving and Resetting State](https://react.dev/learn/preserving-and-resetting-state) goes into detail about how this works.
Now let’s say you want to wrap the completed item’s text into another HTML tag, like `<del>` to strike it out. You can add even more newlines and parentheses so that it’s easier to nest more JSX in each of the cases:
App.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
function Item({ name, isPacked }) {
return (
<li className="item">
{isPacked ? (
<del>
{name + ' ✅'}
</del>
) : (
name
)}
</li>
);
}
export default function PackingList() {
return (
<section>
<h1>Sally Ride's Packing List</h1>
<ul>
<Item
isPacked={true}
name="Space suit"
/>
<Item
isPacked={true}
name="Helmet with a golden leaf"
/>
<Item
isPacked={false}
name="Photo of Tam"
/>
</ul>
</section>
);
}
```
Show more
This style works well for simple conditions, but use it in moderation. If your components get messy with too much nested conditional markup, consider extracting child components to clean things up. In React, markup is a part of your code, so you can use tools like variables and functions to tidy up complex expressions.
### Logical AND operator (`&&`)[Link for this heading]()
Another common shortcut you’ll encounter is the [JavaScript logical AND (`&&`) operator.](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Operators/Logical_AND) Inside React components, it often comes up when you want to render some JSX when the condition is true, **or render nothing otherwise.** With `&&`, you could conditionally render the checkmark only if `isPacked` is `true`:
```
return (
<li className="item">
{name} {isPacked && '✅'}
</li>
);
```
You can read this as *“if `isPacked`, then (`&&`) render the checkmark, otherwise, render nothing”*.
Here it is in action:
App.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
function Item({ name, isPacked }) {
return (
<li className="item">
{name} {isPacked && '✅'}
</li>
);
}
export default function PackingList() {
return (
<section>
<h1>Sally Ride's Packing List</h1>
<ul>
<Item
isPacked={true}
name="Space suit"
/>
<Item
isPacked={true}
name="Helmet with a golden leaf"
/>
<Item
isPacked={false}
name="Photo of Tam"
/>
</ul>
</section>
);
}
```
Show more
A [JavaScript && expression](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Operators/Logical_AND) returns the value of its right side (in our case, the checkmark) if the left side (our condition) is `true`. But if the condition is `false`, the whole expression becomes `false`. React considers `false` as a “hole” in the JSX tree, just like `null` or `undefined`, and doesn’t render anything in its place.
### Pitfall
**Don’t put numbers on the left side of `&&`.**
To test the condition, JavaScript converts the left side to a boolean automatically. However, if the left side is `0`, then the whole expression gets that value (`0`), and React will happily render `0` rather than nothing.
For example, a common mistake is to write code like `messageCount && <p>New messages</p>`. It’s easy to assume that it renders nothing when `messageCount` is `0`, but it really renders the `0` itself!
To fix it, make the left side a boolean: `messageCount > 0 && <p>New messages</p>`.
### Conditionally assigning JSX to a variable[Link for Conditionally assigning JSX to a variable]()
When the shortcuts get in the way of writing plain code, try using an `if` statement and a variable. You can reassign variables defined with [`let`](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Statements/let), so start by providing the default content you want to display, the name:
```
let itemContent = name;
```
Use an `if` statement to reassign a JSX expression to `itemContent` if `isPacked` is `true`:
```
if (isPacked) {
itemContent = name + " ✅";
}
```
[Curly braces open the “window into JavaScript”.](https://react.dev/learn/javascript-in-jsx-with-curly-braces) Embed the variable with curly braces in the returned JSX tree, nesting the previously calculated expression inside of JSX:
```
<li className="item">
{itemContent}
</li>
```
This style is the most verbose, but it’s also the most flexible. Here it is in action:
App.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
function Item({ name, isPacked }) {
let itemContent = name;
if (isPacked) {
itemContent = name + " ✅";
}
return (
<li className="item">
{itemContent}
</li>
);
}
export default function PackingList() {
return (
<section>
<h1>Sally Ride's Packing List</h1>
<ul>
<Item
isPacked={true}
name="Space suit"
/>
<Item
isPacked={true}
name="Helmet with a golden leaf"
/>
<Item
isPacked={false}
name="Photo of Tam"
/>
</ul>
</section>
);
}
```
Show more
Like before, this works not only for text, but for arbitrary JSX too:
App.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
function Item({ name, isPacked }) {
let itemContent = name;
if (isPacked) {
itemContent = (
<del>
{name + " ✅"}
</del>
);
}
return (
<li className="item">
{itemContent}
</li>
);
}
export default function PackingList() {
return (
<section>
<h1>Sally Ride's Packing List</h1>
<ul>
<Item
isPacked={true}
name="Space suit"
/>
<Item
isPacked={true}
name="Helmet with a golden leaf"
/>
<Item
isPacked={false}
name="Photo of Tam"
/>
</ul>
</section>
);
}
```
Show more
If you’re not familiar with JavaScript, this variety of styles might seem overwhelming at first. However, learning them will help you read and write any JavaScript code — and not just React components! Pick the one you prefer for a start, and then consult this reference again if you forget how the other ones work.
## Recap[Link for Recap]()
- In React, you control branching logic with JavaScript.
- You can return a JSX expression conditionally with an `if` statement.
- You can conditionally save some JSX to a variable and then include it inside other JSX by using the curly braces.
- In JSX, `{cond ? <A /> : <B />}` means *“if `cond`, render `<A />`, otherwise `<B />`”*.
- In JSX, `{cond && <A />}` means *“if `cond`, render `<A />`, otherwise nothing”*.
- The shortcuts are common, but you don’t have to use them if you prefer plain `if`.
## Try out some challenges[Link for Try out some challenges]()
1\. Show an icon for incomplete items with `? :` 2. Show the item importance with `&&` 3. Refactor a series of `? :` to `if` and variables
#### Challenge 1 of 3: Show an icon for incomplete items with `? :`[Link for this heading]()
Use the conditional operator (`cond ? a : b`) to render a ❌ if `isPacked` isn’t `true`.
App.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
function Item({ name, isPacked }) {
return (
<li className="item">
{name} {isPacked && '✅'}
</li>
);
}
export default function PackingList() {
return (
<section>
<h1>Sally Ride's Packing List</h1>
<ul>
<Item
isPacked={true}
name="Space suit"
/>
<Item
isPacked={true}
name="Helmet with a golden leaf"
/>
<Item
isPacked={false}
name="Photo of Tam"
/>
</ul>
</section>
);
}
```
Show more
Show solutionNext Challenge
[PreviousPassing Props to a Component](https://react.dev/learn/passing-props-to-a-component)
[NextRendering Lists](https://react.dev/learn/rendering-lists) |
https://react.dev/learn/reacting-to-input-with-state | [Learn React](https://react.dev/learn)
[Managing State](https://react.dev/learn/managing-state)
# Reacting to Input with State[Link for this heading]()
React provides a declarative way to manipulate the UI. Instead of manipulating individual pieces of the UI directly, you describe the different states that your component can be in, and switch between them in response to the user input. This is similar to how designers think about the UI.
### You will learn
- How declarative UI programming differs from imperative UI programming
- How to enumerate the different visual states your component can be in
- How to trigger the changes between the different visual states from code
## How declarative UI compares to imperative[Link for How declarative UI compares to imperative]()
When you design UI interactions, you probably think about how the UI *changes* in response to user actions. Consider a form that lets the user submit an answer:
- When you type something into the form, the “Submit” button **becomes enabled.**
- When you press “Submit”, both the form and the button **become disabled,** and a spinner **appears.**
- If the network request succeeds, the form **gets hidden,** and the “Thank you” message **appears.**
- If the network request fails, an error message **appears,** and the form **becomes enabled** again.
In **imperative programming,** the above corresponds directly to how you implement interaction. You have to write the exact instructions to manipulate the UI depending on what just happened. Here’s another way to think about this: imagine riding next to someone in a car and telling them turn by turn where to go.

Illustrated by [Rachel Lee Nabors](https://nearestnabors.com/)
They don’t know where you want to go, they just follow your commands. (And if you get the directions wrong, you end up in the wrong place!) It’s called *imperative* because you have to “command” each element, from the spinner to the button, telling the computer *how* to update the UI.
In this example of imperative UI programming, the form is built *without* React. It only uses the browser [DOM](https://developer.mozilla.org/en-US/docs/Web/API/Document_Object_Model):
index.jsindex.html
index.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
async function handleFormSubmit(e) {
e.preventDefault();
disable(textarea);
disable(button);
show(loadingMessage);
hide(errorMessage);
try {
await submitForm(textarea.value);
show(successMessage);
hide(form);
} catch (err) {
show(errorMessage);
errorMessage.textContent = err.message;
} finally {
hide(loadingMessage);
enable(textarea);
enable(button);
}
}
function handleTextareaChange() {
if (textarea.value.length === 0) {
disable(button);
} else {
enable(button);
}
}
function hide(el) {
el.style.display = 'none';
}
function show(el) {
el.style.display = '';
}
function enable(el) {
el.disabled = false;
}
function disable(el) {
el.disabled = true;
}
function submitForm(answer) {
// Pretend it's hitting the network.
return new Promise((resolve, reject) => {
setTimeout(() => {
if (answer.toLowerCase() === 'istanbul') {
resolve();
} else {
reject(new Error('Good guess but a wrong answer. Try again!'));
}
}, 1500);
});
}
let form = document.getElementById('form');
let textarea = document.getElementById('textarea');
let button = document.getElementById('button');
let loadingMessage = document.getElementById('loading');
let errorMessage = document.getElementById('error');
let successMessage = document.getElementById('success');
form.onsubmit = handleFormSubmit;
textarea.oninput = handleTextareaChange;
```
Show more
Manipulating the UI imperatively works well enough for isolated examples, but it gets exponentially more difficult to manage in more complex systems. Imagine updating a page full of different forms like this one. Adding a new UI element or a new interaction would require carefully checking all existing code to make sure you haven’t introduced a bug (for example, forgetting to show or hide something).
React was built to solve this problem.
In React, you don’t directly manipulate the UI—meaning you don’t enable, disable, show, or hide components directly. Instead, you **declare what you want to show,** and React figures out how to update the UI. Think of getting into a taxi and telling the driver where you want to go instead of telling them exactly where to turn. It’s the driver’s job to get you there, and they might even know some shortcuts you haven’t considered!

Illustrated by [Rachel Lee Nabors](https://nearestnabors.com/)
## Thinking about UI declaratively[Link for Thinking about UI declaratively]()
You’ve seen how to implement a form imperatively above. To better understand how to think in React, you’ll walk through reimplementing this UI in React below:
1. **Identify** your component’s different visual states
2. **Determine** what triggers those state changes
3. **Represent** the state in memory using `useState`
4. **Remove** any non-essential state variables
5. **Connect** the event handlers to set the state
### Step 1: Identify your component’s different visual states[Link for Step 1: Identify your component’s different visual states]()
In computer science, you may hear about a [“state machine”](https://en.wikipedia.org/wiki/Finite-state_machine) being in one of several “states”. If you work with a designer, you may have seen mockups for different “visual states”. React stands at the intersection of design and computer science, so both of these ideas are sources of inspiration.
First, you need to visualize all the different “states” of the UI the user might see:
- **Empty**: Form has a disabled “Submit” button.
- **Typing**: Form has an enabled “Submit” button.
- **Submitting**: Form is completely disabled. Spinner is shown.
- **Success**: “Thank you” message is shown instead of a form.
- **Error**: Same as Typing state, but with an extra error message.
Just like a designer, you’ll want to “mock up” or create “mocks” for the different states before you add logic. For example, here is a mock for just the visual part of the form. This mock is controlled by a prop called `status` with a default value of `'empty'`:
App.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
export default function Form({
status = 'empty'
}) {
if (status === 'success') {
return <h1>That's right!</h1>
}
return (
<>
<h2>City quiz</h2>
<p>
In which city is there a billboard that turns air into drinkable water?
</p>
<form>
<textarea />
<br />
<button>
Submit
</button>
</form>
</>
)
}
```
Show more
You could call that prop anything you like, the naming is not important. Try editing `status = 'empty'` to `status = 'success'` to see the success message appear. Mocking lets you quickly iterate on the UI before you wire up any logic. Here is a more fleshed out prototype of the same component, still “controlled” by the `status` prop:
App.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
export default function Form({
// Try 'submitting', 'error', 'success':
status = 'empty'
}) {
if (status === 'success') {
return <h1>That's right!</h1>
}
return (
<>
<h2>City quiz</h2>
<p>
In which city is there a billboard that turns air into drinkable water?
</p>
<form>
<textarea disabled={
status === 'submitting'
} />
<br />
<button disabled={
status === 'empty' ||
status === 'submitting'
}>
Submit
</button>
{status === 'error' &&
<p className="Error">
Good guess but a wrong answer. Try again!
</p>
}
</form>
</>
);
}
```
Show more
##### Deep Dive
#### Displaying many visual states at once[Link for Displaying many visual states at once]()
Show Details
If a component has a lot of visual states, it can be convenient to show them all on one page:
App.jsForm.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import Form from './Form.js';
let statuses = [
'empty',
'typing',
'submitting',
'success',
'error',
];
export default function App() {
return (
<>
{statuses.map(status => (
<section key={status}>
<h4>Form ({status}):</h4>
<Form status={status} />
</section>
))}
</>
);
}
```
Show more
Pages like this are often called “living styleguides” or “storybooks”.
### Step 2: Determine what triggers those state changes[Link for Step 2: Determine what triggers those state changes]()
You can trigger state updates in response to two kinds of inputs:
- **Human inputs,** like clicking a button, typing in a field, navigating a link.
- **Computer inputs,** like a network response arriving, a timeout completing, an image loading.

Human inputs

Computer inputs
Illustrated by [Rachel Lee Nabors](https://nearestnabors.com/)
In both cases, **you must set [state variables](https://react.dev/learn/state-a-components-memory) to update the UI.** For the form you’re developing, you will need to change state in response to a few different inputs:
- **Changing the text input** (human) should switch it from the *Empty* state to the *Typing* state or back, depending on whether the text box is empty or not.
- **Clicking the Submit button** (human) should switch it to the *Submitting* state.
- **Successful network response** (computer) should switch it to the *Success* state.
- **Failed network response** (computer) should switch it to the *Error* state with the matching error message.
### Note
Notice that human inputs often require [event handlers](https://react.dev/learn/responding-to-events)!
To help visualize this flow, try drawing each state on paper as a labeled circle, and each change between two states as an arrow. You can sketch out many flows this way and sort out bugs long before implementation.


Form states
### Step 3: Represent the state in memory with `useState`[Link for this heading]()
Next you’ll need to represent the visual states of your component in memory with [`useState`.](https://react.dev/reference/react/useState) Simplicity is key: each piece of state is a “moving piece”, and **you want as few “moving pieces” as possible.** More complexity leads to more bugs!
Start with the state that *absolutely must* be there. For example, you’ll need to store the `answer` for the input, and the `error` (if it exists) to store the last error:
```
const [answer, setAnswer] = useState('');
const [error, setError] = useState(null);
```
Then, you’ll need a state variable representing which one of the visual states that you want to display. There’s usually more than a single way to represent that in memory, so you’ll need to experiment with it.
If you struggle to think of the best way immediately, start by adding enough state that you’re *definitely* sure that all the possible visual states are covered:
```
const [isEmpty, setIsEmpty] = useState(true);
const [isTyping, setIsTyping] = useState(false);
const [isSubmitting, setIsSubmitting] = useState(false);
const [isSuccess, setIsSuccess] = useState(false);
const [isError, setIsError] = useState(false);
```
Your first idea likely won’t be the best, but that’s ok—refactoring state is a part of the process!
### Step 4: Remove any non-essential state variables[Link for Step 4: Remove any non-essential state variables]()
You want to avoid duplication in the state content so you’re only tracking what is essential. Spending a little time on refactoring your state structure will make your components easier to understand, reduce duplication, and avoid unintended meanings. Your goal is to **prevent the cases where the state in memory doesn’t represent any valid UI that you’d want a user to see.** (For example, you never want to show an error message and disable the input at the same time, or the user won’t be able to correct the error!)
Here are some questions you can ask about your state variables:
- **Does this state cause a paradox?** For example, `isTyping` and `isSubmitting` can’t both be `true`. A paradox usually means that the state is not constrained enough. There are four possible combinations of two booleans, but only three correspond to valid states. To remove the “impossible” state, you can combine these into a `status` that must be one of three values: `'typing'`, `'submitting'`, or `'success'`.
- **Is the same information available in another state variable already?** Another paradox: `isEmpty` and `isTyping` can’t be `true` at the same time. By making them separate state variables, you risk them going out of sync and causing bugs. Fortunately, you can remove `isEmpty` and instead check `answer.length === 0`.
- **Can you get the same information from the inverse of another state variable?** `isError` is not needed because you can check `error !== null` instead.
After this clean-up, you’re left with 3 (down from 7!) *essential* state variables:
```
const [answer, setAnswer] = useState('');
const [error, setError] = useState(null);
const [status, setStatus] = useState('typing'); // 'typing', 'submitting', or 'success'
```
You know they are essential, because you can’t remove any of them without breaking the functionality.
##### Deep Dive
#### Eliminating “impossible” states with a reducer[Link for Eliminating “impossible” states with a reducer]()
Show Details
These three variables are a good enough representation of this form’s state. However, there are still some intermediate states that don’t fully make sense. For example, a non-null `error` doesn’t make sense when `status` is `'success'`. To model the state more precisely, you can [extract it into a reducer.](https://react.dev/learn/extracting-state-logic-into-a-reducer) Reducers let you unify multiple state variables into a single object and consolidate all the related logic!
### Step 5: Connect the event handlers to set state[Link for Step 5: Connect the event handlers to set state]()
Lastly, create event handlers that update the state. Below is the final form, with all event handlers wired up:
App.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import { useState } from 'react';
export default function Form() {
const [answer, setAnswer] = useState('');
const [error, setError] = useState(null);
const [status, setStatus] = useState('typing');
if (status === 'success') {
return <h1>That's right!</h1>
}
async function handleSubmit(e) {
e.preventDefault();
setStatus('submitting');
try {
await submitForm(answer);
setStatus('success');
} catch (err) {
setStatus('typing');
setError(err);
}
}
function handleTextareaChange(e) {
setAnswer(e.target.value);
}
return (
<>
<h2>City quiz</h2>
<p>
In which city is there a billboard that turns air into drinkable water?
</p>
<form onSubmit={handleSubmit}>
<textarea
value={answer}
onChange={handleTextareaChange}
disabled={status === 'submitting'}
/>
<br />
<button disabled={
answer.length === 0 ||
status === 'submitting'
}>
Submit
</button>
{error !== null &&
<p className="Error">
{error.message}
</p>
}
</form>
</>
);
}
function submitForm(answer) {
// Pretend it's hitting the network.
return new Promise((resolve, reject) => {
setTimeout(() => {
let shouldError = answer.toLowerCase() !== 'lima'
if (shouldError) {
reject(new Error('Good guess but a wrong answer. Try again!'));
} else {
resolve();
}
}, 1500);
});
}
```
Show more
Although this code is longer than the original imperative example, it is much less fragile. Expressing all interactions as state changes lets you later introduce new visual states without breaking existing ones. It also lets you change what should be displayed in each state without changing the logic of the interaction itself.
## Recap[Link for Recap]()
- Declarative programming means describing the UI for each visual state rather than micromanaging the UI (imperative).
- When developing a component:
1. Identify all its visual states.
2. Determine the human and computer triggers for state changes.
3. Model the state with `useState`.
4. Remove non-essential state to avoid bugs and paradoxes.
5. Connect the event handlers to set state.
## Try out some challenges[Link for Try out some challenges]()
1\. Add and remove a CSS class 2. Profile editor 3. Refactor the imperative solution without React
#### Challenge 1 of 3: Add and remove a CSS class[Link for this heading]()
Make it so that clicking on the picture *removes* the `background--active` CSS class from the outer `<div>`, but *adds* the `picture--active` class to the `<img>`. Clicking the background again should restore the original CSS classes.
Visually, you should expect that clicking on the picture removes the purple background and highlights the picture border. Clicking outside the picture highlights the background, but removes the picture border highlight.
App.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
export default function Picture() {
return (
<div className="background background--active">
<img
className="picture"
alt="Rainbow houses in Kampung Pelangi, Indonesia"
src="https://i.imgur.com/5qwVYb1.jpeg"
/>
</div>
);
}
```
Show solutionNext Challenge
[PreviousManaging State](https://react.dev/learn/managing-state)
[NextChoosing the State Structure](https://react.dev/learn/choosing-the-state-structure) |
https://react.dev/learn/preserving-and-resetting-state | [Learn React](https://react.dev/learn)
[Managing State](https://react.dev/learn/managing-state)
# Preserving and Resetting State[Link for this heading]()
State is isolated between components. React keeps track of which state belongs to which component based on their place in the UI tree. You can control when to preserve state and when to reset it between re-renders.
### You will learn
- When React chooses to preserve or reset the state
- How to force React to reset component’s state
- How keys and types affect whether the state is preserved
## State is tied to a position in the render tree[Link for State is tied to a position in the render tree]()
React builds [render trees](https://react.dev/learn/understanding-your-ui-as-a-tree) for the component structure in your UI.
When you give a component state, you might think the state “lives” inside the component. But the state is actually held inside React. React associates each piece of state it’s holding with the correct component by where that component sits in the render tree.
Here, there is only one `<Counter />` JSX tag, but it’s rendered at two different positions:
App.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import { useState } from 'react';
export default function App() {
const counter = <Counter />;
return (
<div>
{counter}
{counter}
</div>
);
}
function Counter() {
const [score, setScore] = useState(0);
const [hover, setHover] = useState(false);
let className = 'counter';
if (hover) {
className += ' hover';
}
return (
<div
className={className}
onPointerEnter={() => setHover(true)}
onPointerLeave={() => setHover(false)}
>
<h1>{score}</h1>
<button onClick={() => setScore(score + 1)}>
Add one
</button>
</div>
);
}
```
Show more
Here’s how these look as a tree:


React tree
**These are two separate counters because each is rendered at its own position in the tree.** You don’t usually have to think about these positions to use React, but it can be useful to understand how it works.
In React, each component on the screen has fully isolated state. For example, if you render two `Counter` components side by side, each of them will get its own, independent, `score` and `hover` states.
Try clicking both counters and notice they don’t affect each other:
App.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import { useState } from 'react';
export default function App() {
return (
<div>
<Counter />
<Counter />
</div>
);
}
function Counter() {
const [score, setScore] = useState(0);
const [hover, setHover] = useState(false);
let className = 'counter';
if (hover) {
className += ' hover';
}
return (
<div
className={className}
onPointerEnter={() => setHover(true)}
onPointerLeave={() => setHover(false)}
>
<h1>{score}</h1>
<button onClick={() => setScore(score + 1)}>
Add one
</button>
</div>
);
}
```
Show more
As you can see, when one counter is updated, only the state for that component is updated:


Updating state
React will keep the state around for as long as you render the same component at the same position in the tree. To see this, increment both counters, then remove the second component by unchecking “Render the second counter” checkbox, and then add it back by ticking it again:
App.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import { useState } from 'react';
export default function App() {
const [showB, setShowB] = useState(true);
return (
<div>
<Counter />
{showB && <Counter />}
<label>
<input
type="checkbox"
checked={showB}
onChange={e => {
setShowB(e.target.checked)
}}
/>
Render the second counter
</label>
</div>
);
}
function Counter() {
const [score, setScore] = useState(0);
const [hover, setHover] = useState(false);
let className = 'counter';
if (hover) {
className += ' hover';
}
return (
<div
className={className}
onPointerEnter={() => setHover(true)}
onPointerLeave={() => setHover(false)}
>
<h1>{score}</h1>
<button onClick={() => setScore(score + 1)}>
Add one
</button>
</div>
);
}
```
Show more
Notice how the moment you stop rendering the second counter, its state disappears completely. That’s because when React removes a component, it destroys its state.


Deleting a component
When you tick “Render the second counter”, a second `Counter` and its state are initialized from scratch (`score = 0`) and added to the DOM.


Adding a component
**React preserves a component’s state for as long as it’s being rendered at its position in the UI tree.** If it gets removed, or a different component gets rendered at the same position, React discards its state.
## Same component at the same position preserves state[Link for Same component at the same position preserves state]()
In this example, there are two different `<Counter />` tags:
App.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import { useState } from 'react';
export default function App() {
const [isFancy, setIsFancy] = useState(false);
return (
<div>
{isFancy ? (
<Counter isFancy={true} />
) : (
<Counter isFancy={false} />
)}
<label>
<input
type="checkbox"
checked={isFancy}
onChange={e => {
setIsFancy(e.target.checked)
}}
/>
Use fancy styling
</label>
</div>
);
}
function Counter({ isFancy }) {
const [score, setScore] = useState(0);
const [hover, setHover] = useState(false);
let className = 'counter';
if (hover) {
className += ' hover';
}
if (isFancy) {
className += ' fancy';
}
return (
<div
className={className}
onPointerEnter={() => setHover(true)}
onPointerLeave={() => setHover(false)}
>
<h1>{score}</h1>
<button onClick={() => setScore(score + 1)}>
Add one
</button>
</div>
);
}
```
Show more
When you tick or clear the checkbox, the counter state does not get reset. Whether `isFancy` is `true` or `false`, you always have a `<Counter />` as the first child of the `div` returned from the root `App` component:


Updating the `App` state does not reset the `Counter` because `Counter` stays in the same position
It’s the same component at the same position, so from React’s perspective, it’s the same counter.
### Pitfall
Remember that **it’s the position in the UI tree—not in the JSX markup—that matters to React!** This component has two `return` clauses with different `<Counter />` JSX tags inside and outside the `if`:
App.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import { useState } from 'react';
export default function App() {
const [isFancy, setIsFancy] = useState(false);
if (isFancy) {
return (
<div>
<Counter isFancy={true} />
<label>
<input
type="checkbox"
checked={isFancy}
onChange={e => {
setIsFancy(e.target.checked)
}}
/>
Use fancy styling
</label>
</div>
);
}
return (
<div>
<Counter isFancy={false} />
<label>
<input
type="checkbox"
checked={isFancy}
onChange={e => {
setIsFancy(e.target.checked)
}}
/>
Use fancy styling
</label>
</div>
);
}
function Counter({ isFancy }) {
const [score, setScore] = useState(0);
const [hover, setHover] = useState(false);
let className = 'counter';
if (hover) {
className += ' hover';
}
if (isFancy) {
className += ' fancy';
}
return (
<div
className={className}
onPointerEnter={() => setHover(true)}
onPointerLeave={() => setHover(false)}
>
<h1>{score}</h1>
<button onClick={() => setScore(score + 1)}>
Add one
</button>
</div>
);
}
```
Show more
You might expect the state to reset when you tick checkbox, but it doesn’t! This is because **both of these `<Counter />` tags are rendered at the same position.** React doesn’t know where you place the conditions in your function. All it “sees” is the tree you return.
In both cases, the `App` component returns a `<div>` with `<Counter />` as a first child. To React, these two counters have the same “address”: the first child of the first child of the root. This is how React matches them up between the previous and next renders, regardless of how you structure your logic.
## Different components at the same position reset state[Link for Different components at the same position reset state]()
In this example, ticking the checkbox will replace `<Counter>` with a `<p>`:
App.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import { useState } from 'react';
export default function App() {
const [isPaused, setIsPaused] = useState(false);
return (
<div>
{isPaused ? (
<p>See you later!</p>
) : (
<Counter />
)}
<label>
<input
type="checkbox"
checked={isPaused}
onChange={e => {
setIsPaused(e.target.checked)
}}
/>
Take a break
</label>
</div>
);
}
function Counter() {
const [score, setScore] = useState(0);
const [hover, setHover] = useState(false);
let className = 'counter';
if (hover) {
className += ' hover';
}
return (
<div
className={className}
onPointerEnter={() => setHover(true)}
onPointerLeave={() => setHover(false)}
>
<h1>{score}</h1>
<button onClick={() => setScore(score + 1)}>
Add one
</button>
</div>
);
}
```
Show more
Here, you switch between *different* component types at the same position. Initially, the first child of the `<div>` contained a `Counter`. But when you swapped in a `p`, React removed the `Counter` from the UI tree and destroyed its state.


When `Counter` changes to `p`, the `Counter` is deleted and the `p` is added


When switching back, the `p` is deleted and the `Counter` is added
Also, **when you render a different component in the same position, it resets the state of its entire subtree.** To see how this works, increment the counter and then tick the checkbox:
App.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import { useState } from 'react';
export default function App() {
const [isFancy, setIsFancy] = useState(false);
return (
<div>
{isFancy ? (
<div>
<Counter isFancy={true} />
</div>
) : (
<section>
<Counter isFancy={false} />
</section>
)}
<label>
<input
type="checkbox"
checked={isFancy}
onChange={e => {
setIsFancy(e.target.checked)
}}
/>
Use fancy styling
</label>
</div>
);
}
function Counter({ isFancy }) {
const [score, setScore] = useState(0);
const [hover, setHover] = useState(false);
let className = 'counter';
if (hover) {
className += ' hover';
}
if (isFancy) {
className += ' fancy';
}
return (
<div
className={className}
onPointerEnter={() => setHover(true)}
onPointerLeave={() => setHover(false)}
>
<h1>{score}</h1>
<button onClick={() => setScore(score + 1)}>
Add one
</button>
</div>
);
}
```
Show more
The counter state gets reset when you click the checkbox. Although you render a `Counter`, the first child of the `div` changes from a `div` to a `section`. When the child `div` was removed from the DOM, the whole tree below it (including the `Counter` and its state) was destroyed as well.


When `section` changes to `div`, the `section` is deleted and the new `div` is added


When switching back, the `div` is deleted and the new `section` is added
As a rule of thumb, **if you want to preserve the state between re-renders, the structure of your tree needs to “match up”** from one render to another. If the structure is different, the state gets destroyed because React destroys state when it removes a component from the tree.
### Pitfall
This is why you should not nest component function definitions.
Here, the `MyTextField` component function is defined *inside* `MyComponent`:
App.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import { useState } from 'react';
export default function MyComponent() {
const [counter, setCounter] = useState(0);
function MyTextField() {
const [text, setText] = useState('');
return (
<input
value={text}
onChange={e => setText(e.target.value)}
/>
);
}
return (
<>
<MyTextField />
<button onClick={() => {
setCounter(counter + 1)
}}>Clicked {counter} times</button>
</>
);
}
```
Show more
Every time you click the button, the input state disappears! This is because a *different* `MyTextField` function is created for every render of `MyComponent`. You’re rendering a *different* component in the same position, so React resets all state below. This leads to bugs and performance problems. To avoid this problem, **always declare component functions at the top level, and don’t nest their definitions.**
## Resetting state at the same position[Link for Resetting state at the same position]()
By default, React preserves state of a component while it stays at the same position. Usually, this is exactly what you want, so it makes sense as the default behavior. But sometimes, you may want to reset a component’s state. Consider this app that lets two players keep track of their scores during each turn:
App.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import { useState } from 'react';
export default function Scoreboard() {
const [isPlayerA, setIsPlayerA] = useState(true);
return (
<div>
{isPlayerA ? (
<Counter person="Taylor" />
) : (
<Counter person="Sarah" />
)}
<button onClick={() => {
setIsPlayerA(!isPlayerA);
}}>
Next player!
</button>
</div>
);
}
function Counter({ person }) {
const [score, setScore] = useState(0);
const [hover, setHover] = useState(false);
let className = 'counter';
if (hover) {
className += ' hover';
}
return (
<div
className={className}
onPointerEnter={() => setHover(true)}
onPointerLeave={() => setHover(false)}
>
<h1>{person}'s score: {score}</h1>
<button onClick={() => setScore(score + 1)}>
Add one
</button>
</div>
);
}
```
Show more
Currently, when you change the player, the score is preserved. The two `Counter`s appear in the same position, so React sees them as *the same* `Counter` whose `person` prop has changed.
But conceptually, in this app they should be two separate counters. They might appear in the same place in the UI, but one is a counter for Taylor, and another is a counter for Sarah.
There are two ways to reset state when switching between them:
1. Render components in different positions
2. Give each component an explicit identity with `key`
### Option 1: Rendering a component in different positions[Link for Option 1: Rendering a component in different positions]()
If you want these two `Counter`s to be independent, you can render them in two different positions:
App.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import { useState } from 'react';
export default function Scoreboard() {
const [isPlayerA, setIsPlayerA] = useState(true);
return (
<div>
{isPlayerA &&
<Counter person="Taylor" />
}
{!isPlayerA &&
<Counter person="Sarah" />
}
<button onClick={() => {
setIsPlayerA(!isPlayerA);
}}>
Next player!
</button>
</div>
);
}
function Counter({ person }) {
const [score, setScore] = useState(0);
const [hover, setHover] = useState(false);
let className = 'counter';
if (hover) {
className += ' hover';
}
return (
<div
className={className}
onPointerEnter={() => setHover(true)}
onPointerLeave={() => setHover(false)}
>
<h1>{person}'s score: {score}</h1>
<button onClick={() => setScore(score + 1)}>
Add one
</button>
</div>
);
}
```
Show more
- Initially, `isPlayerA` is `true`. So the first position contains `Counter` state, and the second one is empty.
- When you click the “Next player” button the first position clears but the second one now contains a `Counter`.


Initial state


Clicking “next”


Clicking “next” again
Each `Counter`’s state gets destroyed each time it’s removed from the DOM. This is why they reset every time you click the button.
This solution is convenient when you only have a few independent components rendered in the same place. In this example, you only have two, so it’s not a hassle to render both separately in the JSX.
### Option 2: Resetting state with a key[Link for Option 2: Resetting state with a key]()
There is also another, more generic, way to reset a component’s state.
You might have seen `key`s when [rendering lists.](https://react.dev/learn/rendering-lists) Keys aren’t just for lists! You can use keys to make React distinguish between any components. By default, React uses order within the parent (“first counter”, “second counter”) to discern between components. But keys let you tell React that this is not just a *first* counter, or a *second* counter, but a specific counter—for example, *Taylor’s* counter. This way, React will know *Taylor’s* counter wherever it appears in the tree!
In this example, the two `<Counter />`s don’t share state even though they appear in the same place in JSX:
App.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import { useState } from 'react';
export default function Scoreboard() {
const [isPlayerA, setIsPlayerA] = useState(true);
return (
<div>
{isPlayerA ? (
<Counter key="Taylor" person="Taylor" />
) : (
<Counter key="Sarah" person="Sarah" />
)}
<button onClick={() => {
setIsPlayerA(!isPlayerA);
}}>
Next player!
</button>
</div>
);
}
function Counter({ person }) {
const [score, setScore] = useState(0);
const [hover, setHover] = useState(false);
let className = 'counter';
if (hover) {
className += ' hover';
}
return (
<div
className={className}
onPointerEnter={() => setHover(true)}
onPointerLeave={() => setHover(false)}
>
<h1>{person}'s score: {score}</h1>
<button onClick={() => setScore(score + 1)}>
Add one
</button>
</div>
);
}
```
Show more
Switching between Taylor and Sarah does not preserve the state. This is because **you gave them different `key`s:**
```
{isPlayerA ? (
<Counter key="Taylor" person="Taylor" />
) : (
<Counter key="Sarah" person="Sarah" />
)}
```
Specifying a `key` tells React to use the `key` itself as part of the position, instead of their order within the parent. This is why, even though you render them in the same place in JSX, React sees them as two different counters, and so they will never share state. Every time a counter appears on the screen, its state is created. Every time it is removed, its state is destroyed. Toggling between them resets their state over and over.
### Note
Remember that keys are not globally unique. They only specify the position *within the parent*.
### Resetting a form with a key[Link for Resetting a form with a key]()
Resetting state with a key is particularly useful when dealing with forms.
In this chat app, the `<Chat>` component contains the text input state:
App.jsContactList.jsChat.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import { useState } from 'react';
import Chat from './Chat.js';
import ContactList from './ContactList.js';
export default function Messenger() {
const [to, setTo] = useState(contacts[0]);
return (
<div>
<ContactList
contacts={contacts}
selectedContact={to}
onSelect={contact => setTo(contact)}
/>
<Chat contact={to} />
</div>
)
}
const contacts = [
{ id: 0, name: 'Taylor', email: '[email protected]' },
{ id: 1, name: 'Alice', email: '[email protected]' },
{ id: 2, name: 'Bob', email: '[email protected]' }
];
```
Show more
Try entering something into the input, and then press “Alice” or “Bob” to choose a different recipient. You will notice that the input state is preserved because the `<Chat>` is rendered at the same position in the tree.
**In many apps, this may be the desired behavior, but not in a chat app!** You don’t want to let the user send a message they already typed to a wrong person due to an accidental click. To fix it, add a `key`:
```
<Chat key={to.id} contact={to} />
```
This ensures that when you select a different recipient, the `Chat` component will be recreated from scratch, including any state in the tree below it. React will also re-create the DOM elements instead of reusing them.
Now switching the recipient always clears the text field:
App.jsContactList.jsChat.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import { useState } from 'react';
import Chat from './Chat.js';
import ContactList from './ContactList.js';
export default function Messenger() {
const [to, setTo] = useState(contacts[0]);
return (
<div>
<ContactList
contacts={contacts}
selectedContact={to}
onSelect={contact => setTo(contact)}
/>
<Chat key={to.id} contact={to} />
</div>
)
}
const contacts = [
{ id: 0, name: 'Taylor', email: '[email protected]' },
{ id: 1, name: 'Alice', email: '[email protected]' },
{ id: 2, name: 'Bob', email: '[email protected]' }
];
```
Show more
##### Deep Dive
#### Preserving state for removed components[Link for Preserving state for removed components]()
Show Details
In a real chat app, you’d probably want to recover the input state when the user selects the previous recipient again. There are a few ways to keep the state “alive” for a component that’s no longer visible:
- You could render *all* chats instead of just the current one, but hide all the others with CSS. The chats would not get removed from the tree, so their local state would be preserved. This solution works great for simple UIs. But it can get very slow if the hidden trees are large and contain a lot of DOM nodes.
- You could [lift the state up](https://react.dev/learn/sharing-state-between-components) and hold the pending message for each recipient in the parent component. This way, when the child components get removed, it doesn’t matter, because it’s the parent that keeps the important information. This is the most common solution.
- You might also use a different source in addition to React state. For example, you probably want a message draft to persist even if the user accidentally closes the page. To implement this, you could have the `Chat` component initialize its state by reading from the [`localStorage`](https://developer.mozilla.org/en-US/docs/Web/API/Window/localStorage), and save the drafts there too.
No matter which strategy you pick, a chat *with Alice* is conceptually distinct from a chat *with Bob*, so it makes sense to give a `key` to the `<Chat>` tree based on the current recipient.
## Recap[Link for Recap]()
- React keeps state for as long as the same component is rendered at the same position.
- State is not kept in JSX tags. It’s associated with the tree position in which you put that JSX.
- You can force a subtree to reset its state by giving it a different key.
- Don’t nest component definitions, or you’ll reset state by accident.
## Try out some challenges[Link for Try out some challenges]()
1\. Fix disappearing input text 2. Swap two form fields 3. Reset a detail form 4. Clear an image while it’s loading 5. Fix misplaced state in the list
#### Challenge 1 of 5: Fix disappearing input text[Link for this heading]()
This example shows a message when you press the button. However, pressing the button also accidentally resets the input. Why does this happen? Fix it so that pressing the button does not reset the input text.
App.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import { useState } from 'react';
export default function App() {
const [showHint, setShowHint] = useState(false);
if (showHint) {
return (
<div>
<p><i>Hint: Your favorite city?</i></p>
<Form />
<button onClick={() => {
setShowHint(false);
}}>Hide hint</button>
</div>
);
}
return (
<div>
<Form />
<button onClick={() => {
setShowHint(true);
}}>Show hint</button>
</div>
);
}
function Form() {
const [text, setText] = useState('');
return (
<textarea
value={text}
onChange={e => setText(e.target.value)}
/>
);
}
```
Show more
Show solutionNext Challenge
[PreviousSharing State Between Components](https://react.dev/learn/sharing-state-between-components)
[NextExtracting State Logic into a Reducer](https://react.dev/learn/extracting-state-logic-into-a-reducer) |
https://react.dev/learn/choosing-the-state-structure | [Learn React](https://react.dev/learn)
[Managing State](https://react.dev/learn/managing-state)
# Choosing the State Structure[Link for this heading]()
Structuring state well can make a difference between a component that is pleasant to modify and debug, and one that is a constant source of bugs. Here are some tips you should consider when structuring state.
### You will learn
- When to use a single vs multiple state variables
- What to avoid when organizing state
- How to fix common issues with the state structure
## Principles for structuring state[Link for Principles for structuring state]()
When you write a component that holds some state, you’ll have to make choices about how many state variables to use and what the shape of their data should be. While it’s possible to write correct programs even with a suboptimal state structure, there are a few principles that can guide you to make better choices:
1. **Group related state.** If you always update two or more state variables at the same time, consider merging them into a single state variable.
2. **Avoid contradictions in state.** When the state is structured in a way that several pieces of state may contradict and “disagree” with each other, you leave room for mistakes. Try to avoid this.
3. **Avoid redundant state.** If you can calculate some information from the component’s props or its existing state variables during rendering, you should not put that information into that component’s state.
4. **Avoid duplication in state.** When the same data is duplicated between multiple state variables, or within nested objects, it is difficult to keep them in sync. Reduce duplication when you can.
5. **Avoid deeply nested state.** Deeply hierarchical state is not very convenient to update. When possible, prefer to structure state in a flat way.
The goal behind these principles is to *make state easy to update without introducing mistakes*. Removing redundant and duplicate data from state helps ensure that all its pieces stay in sync. This is similar to how a database engineer might want to [“normalize” the database structure](https://docs.microsoft.com/en-us/office/troubleshoot/access/database-normalization-description) to reduce the chance of bugs. To paraphrase Albert Einstein, **“Make your state as simple as it can be—but no simpler.”**
Now let’s see how these principles apply in action.
## Group related state[Link for Group related state]()
You might sometimes be unsure between using a single or multiple state variables.
Should you do this?
```
const [x, setX] = useState(0);
const [y, setY] = useState(0);
```
Or this?
```
const [position, setPosition] = useState({ x: 0, y: 0 });
```
Technically, you can use either of these approaches. But **if some two state variables always change together, it might be a good idea to unify them into a single state variable.** Then you won’t forget to always keep them in sync, like in this example where moving the cursor updates both coordinates of the red dot:
App.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import { useState } from 'react';
export default function MovingDot() {
const [position, setPosition] = useState({
x: 0,
y: 0
});
return (
<div
onPointerMove={e => {
setPosition({
x: e.clientX,
y: e.clientY
});
}}
style={{
position: 'relative',
width: '100vw',
height: '100vh',
}}>
<div style={{
position: 'absolute',
backgroundColor: 'red',
borderRadius: '50%',
transform: `translate(${position.x}px, ${position.y}px)`,
left: -10,
top: -10,
width: 20,
height: 20,
}} />
</div>
)
}
```
Show more
Another case where you’ll group data into an object or an array is when you don’t know how many pieces of state you’ll need. For example, it’s helpful when you have a form where the user can add custom fields.
### Pitfall
If your state variable is an object, remember that [you can’t update only one field in it](https://react.dev/learn/updating-objects-in-state) without explicitly copying the other fields. For example, you can’t do `setPosition({ x: 100 })` in the above example because it would not have the `y` property at all! Instead, if you wanted to set `x` alone, you would either do `setPosition({ ...position, x: 100 })`, or split them into two state variables and do `setX(100)`.
## Avoid contradictions in state[Link for Avoid contradictions in state]()
Here is a hotel feedback form with `isSending` and `isSent` state variables:
App.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import { useState } from 'react';
export default function FeedbackForm() {
const [text, setText] = useState('');
const [isSending, setIsSending] = useState(false);
const [isSent, setIsSent] = useState(false);
async function handleSubmit(e) {
e.preventDefault();
setIsSending(true);
await sendMessage(text);
setIsSending(false);
setIsSent(true);
}
if (isSent) {
return <h1>Thanks for feedback!</h1>
}
return (
<form onSubmit={handleSubmit}>
<p>How was your stay at The Prancing Pony?</p>
<textarea
disabled={isSending}
value={text}
onChange={e => setText(e.target.value)}
/>
<br />
<button
disabled={isSending}
type="submit"
>
Send
</button>
{isSending && <p>Sending...</p>}
</form>
);
}
// Pretend to send a message.
function sendMessage(text) {
return new Promise(resolve => {
setTimeout(resolve, 2000);
});
}
```
Show more
While this code works, it leaves the door open for “impossible” states. For example, if you forget to call `setIsSent` and `setIsSending` together, you may end up in a situation where both `isSending` and `isSent` are `true` at the same time. The more complex your component is, the harder it is to understand what happened.
**Since `isSending` and `isSent` should never be `true` at the same time, it is better to replace them with one `status` state variable that may take one of *three* valid states:** `'typing'` (initial), `'sending'`, and `'sent'`:
App.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import { useState } from 'react';
export default function FeedbackForm() {
const [text, setText] = useState('');
const [status, setStatus] = useState('typing');
async function handleSubmit(e) {
e.preventDefault();
setStatus('sending');
await sendMessage(text);
setStatus('sent');
}
const isSending = status === 'sending';
const isSent = status === 'sent';
if (isSent) {
return <h1>Thanks for feedback!</h1>
}
return (
<form onSubmit={handleSubmit}>
<p>How was your stay at The Prancing Pony?</p>
<textarea
disabled={isSending}
value={text}
onChange={e => setText(e.target.value)}
/>
<br />
<button
disabled={isSending}
type="submit"
>
Send
</button>
{isSending && <p>Sending...</p>}
</form>
);
}
// Pretend to send a message.
function sendMessage(text) {
return new Promise(resolve => {
setTimeout(resolve, 2000);
});
}
```
Show more
You can still declare some constants for readability:
```
const isSending = status === 'sending';
const isSent = status === 'sent';
```
But they’re not state variables, so you don’t need to worry about them getting out of sync with each other.
## Avoid redundant state[Link for Avoid redundant state]()
If you can calculate some information from the component’s props or its existing state variables during rendering, you **should not** put that information into that component’s state.
For example, take this form. It works, but can you find any redundant state in it?
App.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import { useState } from 'react';
export default function Form() {
const [firstName, setFirstName] = useState('');
const [lastName, setLastName] = useState('');
const [fullName, setFullName] = useState('');
function handleFirstNameChange(e) {
setFirstName(e.target.value);
setFullName(e.target.value + ' ' + lastName);
}
function handleLastNameChange(e) {
setLastName(e.target.value);
setFullName(firstName + ' ' + e.target.value);
}
return (
<>
<h2>Let’s check you in</h2>
<label>
First name:{' '}
<input
value={firstName}
onChange={handleFirstNameChange}
/>
</label>
<label>
Last name:{' '}
<input
value={lastName}
onChange={handleLastNameChange}
/>
</label>
<p>
Your ticket will be issued to: <b>{fullName}</b>
</p>
</>
);
}
```
Show more
This form has three state variables: `firstName`, `lastName`, and `fullName`. However, `fullName` is redundant. **You can always calculate `fullName` from `firstName` and `lastName` during render, so remove it from state.**
This is how you can do it:
App.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import { useState } from 'react';
export default function Form() {
const [firstName, setFirstName] = useState('');
const [lastName, setLastName] = useState('');
const fullName = firstName + ' ' + lastName;
function handleFirstNameChange(e) {
setFirstName(e.target.value);
}
function handleLastNameChange(e) {
setLastName(e.target.value);
}
return (
<>
<h2>Let’s check you in</h2>
<label>
First name:{' '}
<input
value={firstName}
onChange={handleFirstNameChange}
/>
</label>
<label>
Last name:{' '}
<input
value={lastName}
onChange={handleLastNameChange}
/>
</label>
<p>
Your ticket will be issued to: <b>{fullName}</b>
</p>
</>
);
}
```
Show more
Here, `fullName` is *not* a state variable. Instead, it’s calculated during render:
```
const fullName = firstName + ' ' + lastName;
```
As a result, the change handlers don’t need to do anything special to update it. When you call `setFirstName` or `setLastName`, you trigger a re-render, and then the next `fullName` will be calculated from the fresh data.
##### Deep Dive
#### Don’t mirror props in state[Link for Don’t mirror props in state]()
Show Details
A common example of redundant state is code like this:
```
function Message({ messageColor }) {
const [color, setColor] = useState(messageColor);
```
Here, a `color` state variable is initialized to the `messageColor` prop. The problem is that **if the parent component passes a different value of `messageColor` later (for example, `'red'` instead of `'blue'`), the `color` *state variable* would not be updated!** The state is only initialized during the first render.
This is why “mirroring” some prop in a state variable can lead to confusion. Instead, use the `messageColor` prop directly in your code. If you want to give it a shorter name, use a constant:
```
function Message({ messageColor }) {
const color = messageColor;
```
This way it won’t get out of sync with the prop passed from the parent component.
”Mirroring” props into state only makes sense when you *want* to ignore all updates for a specific prop. By convention, start the prop name with `initial` or `default` to clarify that its new values are ignored:
```
function Message({ initialColor }) {
// The `color` state variable holds the *first* value of `initialColor`.
// Further changes to the `initialColor` prop are ignored.
const [color, setColor] = useState(initialColor);
```
## Avoid duplication in state[Link for Avoid duplication in state]()
This menu list component lets you choose a single travel snack out of several:
App.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import { useState } from 'react';
const initialItems = [
{ title: 'pretzels', id: 0 },
{ title: 'crispy seaweed', id: 1 },
{ title: 'granola bar', id: 2 },
];
export default function Menu() {
const [items, setItems] = useState(initialItems);
const [selectedItem, setSelectedItem] = useState(
items[0]
);
return (
<>
<h2>What's your travel snack?</h2>
<ul>
{items.map(item => (
<li key={item.id}>
{item.title}
{' '}
<button onClick={() => {
setSelectedItem(item);
}}>Choose</button>
</li>
))}
</ul>
<p>You picked {selectedItem.title}.</p>
</>
);
}
```
Show more
Currently, it stores the selected item as an object in the `selectedItem` state variable. However, this is not great: **the contents of the `selectedItem` is the same object as one of the items inside the `items` list.** This means that the information about the item itself is duplicated in two places.
Why is this a problem? Let’s make each item editable:
App.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import { useState } from 'react';
const initialItems = [
{ title: 'pretzels', id: 0 },
{ title: 'crispy seaweed', id: 1 },
{ title: 'granola bar', id: 2 },
];
export default function Menu() {
const [items, setItems] = useState(initialItems);
const [selectedItem, setSelectedItem] = useState(
items[0]
);
function handleItemChange(id, e) {
setItems(items.map(item => {
if (item.id === id) {
return {
...item,
title: e.target.value,
};
} else {
return item;
}
}));
}
return (
<>
<h2>What's your travel snack?</h2>
<ul>
{items.map((item, index) => (
<li key={item.id}>
<input
value={item.title}
onChange={e => {
handleItemChange(item.id, e)
}}
/>
{' '}
<button onClick={() => {
setSelectedItem(item);
}}>Choose</button>
</li>
))}
</ul>
<p>You picked {selectedItem.title}.</p>
</>
);
}
```
Show more
Notice how if you first click “Choose” on an item and *then* edit it, **the input updates but the label at the bottom does not reflect the edits.** This is because you have duplicated state, and you forgot to update `selectedItem`.
Although you could update `selectedItem` too, an easier fix is to remove duplication. In this example, instead of a `selectedItem` object (which creates a duplication with objects inside `items`), you hold the `selectedId` in state, and *then* get the `selectedItem` by searching the `items` array for an item with that ID:
App.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import { useState } from 'react';
const initialItems = [
{ title: 'pretzels', id: 0 },
{ title: 'crispy seaweed', id: 1 },
{ title: 'granola bar', id: 2 },
];
export default function Menu() {
const [items, setItems] = useState(initialItems);
const [selectedId, setSelectedId] = useState(0);
const selectedItem = items.find(item =>
item.id === selectedId
);
function handleItemChange(id, e) {
setItems(items.map(item => {
if (item.id === id) {
return {
...item,
title: e.target.value,
};
} else {
return item;
}
}));
}
return (
<>
<h2>What's your travel snack?</h2>
<ul>
{items.map((item, index) => (
<li key={item.id}>
<input
value={item.title}
onChange={e => {
handleItemChange(item.id, e)
}}
/>
{' '}
<button onClick={() => {
setSelectedId(item.id);
}}>Choose</button>
</li>
))}
</ul>
<p>You picked {selectedItem.title}.</p>
</>
);
}
```
Show more
The state used to be duplicated like this:
- `items = [{ id: 0, title: 'pretzels'}, ...]`
- `selectedItem = {id: 0, title: 'pretzels'}`
But after the change it’s like this:
- `items = [{ id: 0, title: 'pretzels'}, ...]`
- `selectedId = 0`
The duplication is gone, and you only keep the essential state!
Now if you edit the *selected* item, the message below will update immediately. This is because `setItems` triggers a re-render, and `items.find(...)` would find the item with the updated title. You didn’t need to hold *the selected item* in state, because only the *selected ID* is essential. The rest could be calculated during render.
## Avoid deeply nested state[Link for Avoid deeply nested state]()
Imagine a travel plan consisting of planets, continents, and countries. You might be tempted to structure its state using nested objects and arrays, like in this example:
App.jsplaces.js
places.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
export const initialTravelPlan = {
id: 0,
title: '(Root)',
childPlaces: [{
id: 1,
title: 'Earth',
childPlaces: [{
id: 2,
title: 'Africa',
childPlaces: [{
id: 3,
title: 'Botswana',
childPlaces: []
}, {
id: 4,
title: 'Egypt',
childPlaces: []
}, {
id: 5,
title: 'Kenya',
childPlaces: []
}, {
id: 6,
title: 'Madagascar',
childPlaces: []
}, {
id: 7,
title: 'Morocco',
childPlaces: []
}, {
id: 8,
title: 'Nigeria',
childPlaces: []
}, {
id: 9,
title: 'South Africa',
childPlaces: []
}]
}, {
id: 10,
title: 'Americas',
childPlaces: [{
id: 11,
title: 'Argentina',
childPlaces: []
}, {
id: 12,
title: 'Brazil',
childPlaces: []
}, {
id: 13,
title: 'Barbados',
childPlaces: []
}, {
id: 14,
title: 'Canada',
childPlaces: []
}, {
id: 15,
title: 'Jamaica',
childPlaces: []
}, {
id: 16,
title: 'Mexico',
childPlaces: []
}, {
id: 17,
title: 'Trinidad and Tobago',
childPlaces: []
}, {
id: 18,
title: 'Venezuela',
childPlaces: []
}]
}, {
id: 19,
title: 'Asia',
childPlaces: [{
id: 20,
title: 'China',
childPlaces: []
}, {
id: 21,
title: 'India',
childPlaces: []
}, {
id: 22,
title: 'Singapore',
childPlaces: []
}, {
id: 23,
title: 'South Korea',
childPlaces: []
}, {
id: 24,
title: 'Thailand',
childPlaces: []
}, {
id: 25,
title: 'Vietnam',
childPlaces: []
}]
}, {
id: 26,
title: 'Europe',
childPlaces: [{
id: 27,
title: 'Croatia',
childPlaces: [],
}, {
id: 28,
title: 'France',
childPlaces: [],
}, {
id: 29,
title: 'Germany',
childPlaces: [],
}, {
id: 30,
title: 'Italy',
childPlaces: [],
}, {
id: 31,
title: 'Portugal',
childPlaces: [],
}, {
id: 32,
title: 'Spain',
childPlaces: [],
}, {
id: 33,
title: 'Turkey',
childPlaces: [],
}]
}, {
id: 34,
title: 'Oceania',
childPlaces: [{
id: 35,
title: 'Australia',
childPlaces: [],
}, {
id: 36,
title: 'Bora Bora (French Polynesia)',
childPlaces: [],
}, {
id: 37,
title: 'Easter Island (Chile)',
childPlaces: [],
}, {
id: 38,
title: 'Fiji',
childPlaces: [],
}, {
id: 39,
title: 'Hawaii (the USA)',
childPlaces: [],
}, {
id: 40,
title: 'New Zealand',
childPlaces: [],
}, {
id: 41,
title: 'Vanuatu',
childPlaces: [],
}]
}]
}, {
id: 42,
title: 'Moon',
childPlaces: [{
id: 43,
title: 'Rheita',
childPlaces: []
}, {
id: 44,
title: 'Piccolomini',
childPlaces: []
}, {
id: 45,
title: 'Tycho',
childPlaces: []
}]
}, {
id: 46,
title: 'Mars',
childPlaces: [{
id: 47,
title: 'Corn Town',
childPlaces: []
}, {
id: 48,
title: 'Green Hill',
childPlaces: []
}]
}]
};
```
Show more
Now let’s say you want to add a button to delete a place you’ve already visited. How would you go about it? [Updating nested state](https://react.dev/learn/updating-objects-in-state) involves making copies of objects all the way up from the part that changed. Deleting a deeply nested place would involve copying its entire parent place chain. Such code can be very verbose.
**If the state is too nested to update easily, consider making it “flat”.** Here is one way you can restructure this data. Instead of a tree-like structure where each `place` has an array of *its child places*, you can have each place hold an array of *its child place IDs*. Then store a mapping from each place ID to the corresponding place.
This data restructuring might remind you of seeing a database table:
App.jsplaces.js
places.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
export const initialTravelPlan = {
0: {
id: 0,
title: '(Root)',
childIds: [1, 42, 46],
},
1: {
id: 1,
title: 'Earth',
childIds: [2, 10, 19, 26, 34]
},
2: {
id: 2,
title: 'Africa',
childIds: [3, 4, 5, 6 , 7, 8, 9]
},
3: {
id: 3,
title: 'Botswana',
childIds: []
},
4: {
id: 4,
title: 'Egypt',
childIds: []
},
5: {
id: 5,
title: 'Kenya',
childIds: []
},
6: {
id: 6,
title: 'Madagascar',
childIds: []
},
7: {
id: 7,
title: 'Morocco',
childIds: []
},
8: {
id: 8,
title: 'Nigeria',
childIds: []
},
9: {
id: 9,
title: 'South Africa',
childIds: []
},
10: {
id: 10,
title: 'Americas',
childIds: [11, 12, 13, 14, 15, 16, 17, 18],
},
11: {
id: 11,
title: 'Argentina',
childIds: []
},
12: {
id: 12,
title: 'Brazil',
childIds: []
},
13: {
id: 13,
title: 'Barbados',
childIds: []
},
14: {
id: 14,
title: 'Canada',
childIds: []
},
15: {
id: 15,
title: 'Jamaica',
childIds: []
},
16: {
id: 16,
title: 'Mexico',
childIds: []
},
17: {
id: 17,
title: 'Trinidad and Tobago',
childIds: []
},
18: {
id: 18,
title: 'Venezuela',
childIds: []
},
19: {
id: 19,
title: 'Asia',
childIds: [20, 21, 22, 23, 24, 25],
},
20: {
id: 20,
title: 'China',
childIds: []
},
21: {
id: 21,
title: 'India',
childIds: []
},
22: {
id: 22,
title: 'Singapore',
childIds: []
},
23: {
id: 23,
title: 'South Korea',
childIds: []
},
24: {
id: 24,
title: 'Thailand',
childIds: []
},
25: {
id: 25,
title: 'Vietnam',
childIds: []
},
26: {
id: 26,
title: 'Europe',
childIds: [27, 28, 29, 30, 31, 32, 33],
},
27: {
id: 27,
title: 'Croatia',
childIds: []
},
28: {
id: 28,
title: 'France',
childIds: []
},
29: {
id: 29,
title: 'Germany',
childIds: []
},
30: {
id: 30,
title: 'Italy',
childIds: []
},
31: {
id: 31,
title: 'Portugal',
childIds: []
},
32: {
id: 32,
title: 'Spain',
childIds: []
},
33: {
id: 33,
title: 'Turkey',
childIds: []
},
34: {
id: 34,
title: 'Oceania',
childIds: [35, 36, 37, 38, 39, 40, 41],
},
35: {
id: 35,
title: 'Australia',
childIds: []
},
36: {
id: 36,
title: 'Bora Bora (French Polynesia)',
childIds: []
},
37: {
id: 37,
title: 'Easter Island (Chile)',
childIds: []
},
38: {
id: 38,
title: 'Fiji',
childIds: []
},
39: {
id: 40,
title: 'Hawaii (the USA)',
childIds: []
},
40: {
id: 40,
title: 'New Zealand',
childIds: []
},
41: {
id: 41,
title: 'Vanuatu',
childIds: []
},
42: {
id: 42,
title: 'Moon',
childIds: [43, 44, 45]
},
43: {
id: 43,
title: 'Rheita',
childIds: []
},
44: {
id: 44,
title: 'Piccolomini',
childIds: []
},
45: {
id: 45,
title: 'Tycho',
childIds: []
},
46: {
id: 46,
title: 'Mars',
childIds: [47, 48]
},
47: {
id: 47,
title: 'Corn Town',
childIds: []
},
48: {
id: 48,
title: 'Green Hill',
childIds: []
}
};
```
Show more
**Now that the state is “flat” (also known as “normalized”), updating nested items becomes easier.**
In order to remove a place now, you only need to update two levels of state:
- The updated version of its *parent* place should exclude the removed ID from its `childIds` array.
- The updated version of the root “table” object should include the updated version of the parent place.
Here is an example of how you could go about it:
App.jsplaces.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import { useState } from 'react';
import { initialTravelPlan } from './places.js';
export default function TravelPlan() {
const [plan, setPlan] = useState(initialTravelPlan);
function handleComplete(parentId, childId) {
const parent = plan[parentId];
// Create a new version of the parent place
// that doesn't include this child ID.
const nextParent = {
...parent,
childIds: parent.childIds
.filter(id => id !== childId)
};
// Update the root state object...
setPlan({
...plan,
// ...so that it has the updated parent.
[parentId]: nextParent
});
}
const root = plan[0];
const planetIds = root.childIds;
return (
<>
<h2>Places to visit</h2>
<ol>
{planetIds.map(id => (
<PlaceTree
key={id}
id={id}
parentId={0}
placesById={plan}
onComplete={handleComplete}
/>
))}
</ol>
</>
);
}
function PlaceTree({ id, parentId, placesById, onComplete }) {
const place = placesById[id];
const childIds = place.childIds;
return (
<li>
{place.title}
<button onClick={() => {
onComplete(parentId, id);
}}>
Complete
</button>
{childIds.length > 0 &&
<ol>
{childIds.map(childId => (
<PlaceTree
key={childId}
id={childId}
parentId={id}
placesById={placesById}
onComplete={onComplete}
/>
))}
</ol>
}
</li>
);
}
```
Show more
You can nest state as much as you like, but making it “flat” can solve numerous problems. It makes state easier to update, and it helps ensure you don’t have duplication in different parts of a nested object.
##### Deep Dive
#### Improving memory usage[Link for Improving memory usage]()
Show Details
Ideally, you would also remove the deleted items (and their children!) from the “table” object to improve memory usage. This version does that. It also [uses Immer](https://react.dev/learn/updating-objects-in-state) to make the update logic more concise.
package.jsonApp.jsplaces.js
package.json
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
{
"dependencies": {
"immer": "1.7.3",
"react": "latest",
"react-dom": "latest",
"react-scripts": "latest",
"use-immer": "0.5.1"
},
"scripts": {
"start": "react-scripts start",
"build": "react-scripts build",
"test": "react-scripts test --env=jsdom",
"eject": "react-scripts eject"
},
"devDependencies": {}
}
```
Sometimes, you can also reduce state nesting by moving some of the nested state into the child components. This works well for ephemeral UI state that doesn’t need to be stored, like whether an item is hovered.
## Recap[Link for Recap]()
- If two state variables always update together, consider merging them into one.
- Choose your state variables carefully to avoid creating “impossible” states.
- Structure your state in a way that reduces the chances that you’ll make a mistake updating it.
- Avoid redundant and duplicate state so that you don’t need to keep it in sync.
- Don’t put props *into* state unless you specifically want to prevent updates.
- For UI patterns like selection, keep ID or index in state instead of the object itself.
- If updating deeply nested state is complicated, try flattening it.
## Try out some challenges[Link for Try out some challenges]()
1\. Fix a component that’s not updating 2. Fix a broken packing list 3. Fix the disappearing selection 4. Implement multiple selection
#### Challenge 1 of 4: Fix a component that’s not updating[Link for this heading]()
This `Clock` component receives two props: `color` and `time`. When you select a different color in the select box, the `Clock` component receives a different `color` prop from its parent component. However, for some reason, the displayed color doesn’t update. Why? Fix the problem.
Clock.js
Clock.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import { useState } from 'react';
export default function Clock(props) {
const [color, setColor] = useState(props.color);
return (
<h1 style={{ color: color }}>
{props.time}
</h1>
);
}
```
Show solutionNext Challenge
[PreviousReacting to Input with State](https://react.dev/learn/reacting-to-input-with-state)
[NextSharing State Between Components](https://react.dev/learn/sharing-state-between-components) |
https://react.dev/learn/scaling-up-with-reducer-and-context | [Learn React](https://react.dev/learn)
[Managing State](https://react.dev/learn/managing-state)
# Scaling Up with Reducer and Context[Link for this heading]()
Reducers let you consolidate a component’s state update logic. Context lets you pass information deep down to other components. You can combine reducers and context together to manage state of a complex screen.
### You will learn
- How to combine a reducer with context
- How to avoid passing state and dispatch through props
- How to keep context and state logic in a separate file
## Combining a reducer with context[Link for Combining a reducer with context]()
In this example from [the introduction to reducers](https://react.dev/learn/extracting-state-logic-into-a-reducer), the state is managed by a reducer. The reducer function contains all of the state update logic and is declared at the bottom of this file:
App.jsAddTask.jsTaskList.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import { useReducer } from 'react';
import AddTask from './AddTask.js';
import TaskList from './TaskList.js';
export default function TaskApp() {
const [tasks, dispatch] = useReducer(
tasksReducer,
initialTasks
);
function handleAddTask(text) {
dispatch({
type: 'added',
id: nextId++,
text: text,
});
}
function handleChangeTask(task) {
dispatch({
type: 'changed',
task: task
});
}
function handleDeleteTask(taskId) {
dispatch({
type: 'deleted',
id: taskId
});
}
return (
<>
<h1>Day off in Kyoto</h1>
<AddTask
onAddTask={handleAddTask}
/>
<TaskList
tasks={tasks}
onChangeTask={handleChangeTask}
onDeleteTask={handleDeleteTask}
/>
</>
);
}
function tasksReducer(tasks, action) {
switch (action.type) {
case 'added': {
return [...tasks, {
id: action.id,
text: action.text,
done: false
}];
}
case 'changed': {
return tasks.map(t => {
if (t.id === action.task.id) {
return action.task;
} else {
return t;
}
});
}
case 'deleted': {
return tasks.filter(t => t.id !== action.id);
}
default: {
throw Error('Unknown action: ' + action.type);
}
}
}
let nextId = 3;
const initialTasks = [
{ id: 0, text: 'Philosopher’s Path', done: true },
{ id: 1, text: 'Visit the temple', done: false },
{ id: 2, text: 'Drink matcha', done: false }
];
```
Show more
A reducer helps keep the event handlers short and concise. However, as your app grows, you might run into another difficulty. **Currently, the `tasks` state and the `dispatch` function are only available in the top-level `TaskApp` component.** To let other components read the list of tasks or change it, you have to explicitly [pass down](https://react.dev/learn/passing-props-to-a-component) the current state and the event handlers that change it as props.
For example, `TaskApp` passes a list of tasks and the event handlers to `TaskList`:
```
<TaskList
tasks={tasks}
onChangeTask={handleChangeTask}
onDeleteTask={handleDeleteTask}
/>
```
And `TaskList` passes the event handlers to `Task`:
```
<Task
task={task}
onChange={onChangeTask}
onDelete={onDeleteTask}
/>
```
In a small example like this, this works well, but if you have tens or hundreds of components in the middle, passing down all state and functions can be quite frustrating!
This is why, as an alternative to passing them through props, you might want to put both the `tasks` state and the `dispatch` function [into context.](https://react.dev/learn/passing-data-deeply-with-context) **This way, any component below `TaskApp` in the tree can read the tasks and dispatch actions without the repetitive “prop drilling”.**
Here is how you can combine a reducer with context:
1. **Create** the context.
2. **Put** state and dispatch into context.
3. **Use** context anywhere in the tree.
### Step 1: Create the context[Link for Step 1: Create the context]()
The `useReducer` Hook returns the current `tasks` and the `dispatch` function that lets you update them:
```
const [tasks, dispatch] = useReducer(tasksReducer, initialTasks);
```
To pass them down the tree, you will [create](https://react.dev/learn/passing-data-deeply-with-context) two separate contexts:
- `TasksContext` provides the current list of tasks.
- `TasksDispatchContext` provides the function that lets components dispatch actions.
Export them from a separate file so that you can later import them from other files:
App.jsTasksContext.jsAddTask.jsTaskList.js
TasksContext.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import { createContext } from 'react';
export const TasksContext = createContext(null);
export const TasksDispatchContext = createContext(null);
```
Here, you’re passing `null` as the default value to both contexts. The actual values will be provided by the `TaskApp` component.
### Step 2: Put state and dispatch into context[Link for Step 2: Put state and dispatch into context]()
Now you can import both contexts in your `TaskApp` component. Take the `tasks` and `dispatch` returned by `useReducer()` and [provide them](https://react.dev/learn/passing-data-deeply-with-context) to the entire tree below:
```
import { TasksContext, TasksDispatchContext } from './TasksContext.js';
export default function TaskApp() {
const [tasks, dispatch] = useReducer(tasksReducer, initialTasks);
// ...
return (
<TasksContext.Provider value={tasks}>
<TasksDispatchContext.Provider value={dispatch}>
...
</TasksDispatchContext.Provider>
</TasksContext.Provider>
);
}
```
For now, you pass the information both via props and in context:
App.jsTasksContext.jsAddTask.jsTaskList.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import { useReducer } from 'react';
import AddTask from './AddTask.js';
import TaskList from './TaskList.js';
import { TasksContext, TasksDispatchContext } from './TasksContext.js';
export default function TaskApp() {
const [tasks, dispatch] = useReducer(
tasksReducer,
initialTasks
);
function handleAddTask(text) {
dispatch({
type: 'added',
id: nextId++,
text: text,
});
}
function handleChangeTask(task) {
dispatch({
type: 'changed',
task: task
});
}
function handleDeleteTask(taskId) {
dispatch({
type: 'deleted',
id: taskId
});
}
return (
<TasksContext.Provider value={tasks}>
<TasksDispatchContext.Provider value={dispatch}>
<h1>Day off in Kyoto</h1>
<AddTask
onAddTask={handleAddTask}
/>
<TaskList
tasks={tasks}
onChangeTask={handleChangeTask}
onDeleteTask={handleDeleteTask}
/>
</TasksDispatchContext.Provider>
</TasksContext.Provider>
);
}
function tasksReducer(tasks, action) {
switch (action.type) {
case 'added': {
return [...tasks, {
id: action.id,
text: action.text,
done: false
}];
}
case 'changed': {
return tasks.map(t => {
if (t.id === action.task.id) {
return action.task;
} else {
return t;
}
});
}
case 'deleted': {
return tasks.filter(t => t.id !== action.id);
}
default: {
throw Error('Unknown action: ' + action.type);
}
}
}
let nextId = 3;
const initialTasks = [
{ id: 0, text: 'Philosopher’s Path', done: true },
{ id: 1, text: 'Visit the temple', done: false },
{ id: 2, text: 'Drink matcha', done: false }
];
```
Show more
In the next step, you will remove prop passing.
### Step 3: Use context anywhere in the tree[Link for Step 3: Use context anywhere in the tree]()
Now you don’t need to pass the list of tasks or the event handlers down the tree:
```
<TasksContext.Provider value={tasks}>
<TasksDispatchContext.Provider value={dispatch}>
<h1>Day off in Kyoto</h1>
<AddTask />
<TaskList />
</TasksDispatchContext.Provider>
</TasksContext.Provider>
```
Instead, any component that needs the task list can read it from the `TaskContext`:
```
export default function TaskList() {
const tasks = useContext(TasksContext);
// ...
```
To update the task list, any component can read the `dispatch` function from context and call it:
```
export default function AddTask() {
const [text, setText] = useState('');
const dispatch = useContext(TasksDispatchContext);
// ...
return (
// ...
<button onClick={() => {
setText('');
dispatch({
type: 'added',
id: nextId++,
text: text,
});
}}>Add</button>
// ...
```
**The `TaskApp` component does not pass any event handlers down, and the `TaskList` does not pass any event handlers to the `Task` component either.** Each component reads the context that it needs:
App.jsTasksContext.jsAddTask.jsTaskList.js
TaskList.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import { useState, useContext } from 'react';
import { TasksContext, TasksDispatchContext } from './TasksContext.js';
export default function TaskList() {
const tasks = useContext(TasksContext);
return (
<ul>
{tasks.map(task => (
<li key={task.id}>
<Task task={task} />
</li>
))}
</ul>
);
}
function Task({ task }) {
const [isEditing, setIsEditing] = useState(false);
const dispatch = useContext(TasksDispatchContext);
let taskContent;
if (isEditing) {
taskContent = (
<>
<input
value={task.text}
onChange={e => {
dispatch({
type: 'changed',
task: {
...task,
text: e.target.value
}
});
}} />
<button onClick={() => setIsEditing(false)}>
Save
</button>
</>
);
} else {
taskContent = (
<>
{task.text}
<button onClick={() => setIsEditing(true)}>
Edit
</button>
</>
);
}
return (
<label>
<input
type="checkbox"
checked={task.done}
onChange={e => {
dispatch({
type: 'changed',
task: {
...task,
done: e.target.checked
}
});
}}
/>
{taskContent}
<button onClick={() => {
dispatch({
type: 'deleted',
id: task.id
});
}}>
Delete
</button>
</label>
);
}
```
Show more
**The state still “lives” in the top-level `TaskApp` component, managed with `useReducer`.** But its `tasks` and `dispatch` are now available to every component below in the tree by importing and using these contexts.
## Moving all wiring into a single file[Link for Moving all wiring into a single file]()
You don’t have to do this, but you could further declutter the components by moving both reducer and context into a single file. Currently, `TasksContext.js` contains only two context declarations:
```
import { createContext } from 'react';
export const TasksContext = createContext(null);
export const TasksDispatchContext = createContext(null);
```
This file is about to get crowded! You’ll move the reducer into that same file. Then you’ll declare a new `TasksProvider` component in the same file. This component will tie all the pieces together:
1. It will manage the state with a reducer.
2. It will provide both contexts to components below.
3. It will [take `children` as a prop](https://react.dev/learn/passing-props-to-a-component) so you can pass JSX to it.
```
export function TasksProvider({ children }) {
const [tasks, dispatch] = useReducer(tasksReducer, initialTasks);
return (
<TasksContext.Provider value={tasks}>
<TasksDispatchContext.Provider value={dispatch}>
{children}
</TasksDispatchContext.Provider>
</TasksContext.Provider>
);
}
```
**This removes all the complexity and wiring from your `TaskApp` component:**
App.jsTasksContext.jsAddTask.jsTaskList.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import AddTask from './AddTask.js';
import TaskList from './TaskList.js';
import { TasksProvider } from './TasksContext.js';
export default function TaskApp() {
return (
<TasksProvider>
<h1>Day off in Kyoto</h1>
<AddTask />
<TaskList />
</TasksProvider>
);
}
```
You can also export functions that *use* the context from `TasksContext.js`:
```
export function useTasks() {
return useContext(TasksContext);
}
export function useTasksDispatch() {
return useContext(TasksDispatchContext);
}
```
When a component needs to read context, it can do it through these functions:
```
const tasks = useTasks();
const dispatch = useTasksDispatch();
```
This doesn’t change the behavior in any way, but it lets you later split these contexts further or add some logic to these functions. **Now all of the context and reducer wiring is in `TasksContext.js`. This keeps the components clean and uncluttered, focused on what they display rather than where they get the data:**
App.jsTasksContext.jsAddTask.jsTaskList.js
TaskList.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import { useState } from 'react';
import { useTasks, useTasksDispatch } from './TasksContext.js';
export default function TaskList() {
const tasks = useTasks();
return (
<ul>
{tasks.map(task => (
<li key={task.id}>
<Task task={task} />
</li>
))}
</ul>
);
}
function Task({ task }) {
const [isEditing, setIsEditing] = useState(false);
const dispatch = useTasksDispatch();
let taskContent;
if (isEditing) {
taskContent = (
<>
<input
value={task.text}
onChange={e => {
dispatch({
type: 'changed',
task: {
...task,
text: e.target.value
}
});
}} />
<button onClick={() => setIsEditing(false)}>
Save
</button>
</>
);
} else {
taskContent = (
<>
{task.text}
<button onClick={() => setIsEditing(true)}>
Edit
</button>
</>
);
}
return (
<label>
<input
type="checkbox"
checked={task.done}
onChange={e => {
dispatch({
type: 'changed',
task: {
...task,
done: e.target.checked
}
});
}}
/>
{taskContent}
<button onClick={() => {
dispatch({
type: 'deleted',
id: task.id
});
}}>
Delete
</button>
</label>
);
}
```
Show more
You can think of `TasksProvider` as a part of the screen that knows how to deal with tasks, `useTasks` as a way to read them, and `useTasksDispatch` as a way to update them from any component below in the tree.
### Note
Functions like `useTasks` and `useTasksDispatch` are called [*Custom Hooks.*](https://react.dev/learn/reusing-logic-with-custom-hooks) Your function is considered a custom Hook if its name starts with `use`. This lets you use other Hooks, like `useContext`, inside it.
As your app grows, you may have many context-reducer pairs like this. This is a powerful way to scale your app and [lift state up](https://react.dev/learn/sharing-state-between-components) without too much work whenever you want to access the data deep in the tree.
## Recap[Link for Recap]()
- You can combine reducer with context to let any component read and update state above it.
- To provide state and the dispatch function to components below:
1. Create two contexts (for state and for dispatch functions).
2. Provide both contexts from the component that uses the reducer.
3. Use either context from components that need to read them.
- You can further declutter the components by moving all wiring into one file.
- You can export a component like `TasksProvider` that provides context.
- You can also export custom Hooks like `useTasks` and `useTasksDispatch` to read it.
- You can have many context-reducer pairs like this in your app.
[PreviousPassing Data Deeply with Context](https://react.dev/learn/passing-data-deeply-with-context)
[NextEscape Hatches](https://react.dev/learn/escape-hatches) |
https://react.dev/learn/referencing-values-with-refs | [Learn React](https://react.dev/learn)
[Escape Hatches](https://react.dev/learn/escape-hatches)
# Referencing Values with Refs[Link for this heading]()
When you want a component to “remember” some information, but you don’t want that information to [trigger new renders](https://react.dev/learn/render-and-commit), you can use a *ref*.
### You will learn
- How to add a ref to your component
- How to update a ref’s value
- How refs are different from state
- How to use refs safely
## Adding a ref to your component[Link for Adding a ref to your component]()
You can add a ref to your component by importing the `useRef` Hook from React:
```
import { useRef } from 'react';
```
Inside your component, call the `useRef` Hook and pass the initial value that you want to reference as the only argument. For example, here is a ref to the value `0`:
```
const ref = useRef(0);
```
`useRef` returns an object like this:
```
{
current: 0 // The value you passed to useRef
}
```

Illustrated by [Rachel Lee Nabors](https://nearestnabors.com/)
You can access the current value of that ref through the `ref.current` property. This value is intentionally mutable, meaning you can both read and write to it. It’s like a secret pocket of your component that React doesn’t track. (This is what makes it an “escape hatch” from React’s one-way data flow—more on that below!)
Here, a button will increment `ref.current` on every click:
App.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import { useRef } from 'react';
export default function Counter() {
let ref = useRef(0);
function handleClick() {
ref.current = ref.current + 1;
alert('You clicked ' + ref.current + ' times!');
}
return (
<button onClick={handleClick}>
Click me!
</button>
);
}
```
Show more
The ref points to a number, but, like [state](https://react.dev/learn/state-a-components-memory), you could point to anything: a string, an object, or even a function. Unlike state, ref is a plain JavaScript object with the `current` property that you can read and modify.
Note that **the component doesn’t re-render with every increment.** Like state, refs are retained by React between re-renders. However, setting state re-renders a component. Changing a ref does not!
## Example: building a stopwatch[Link for Example: building a stopwatch]()
You can combine refs and state in a single component. For example, let’s make a stopwatch that the user can start or stop by pressing a button. In order to display how much time has passed since the user pressed “Start”, you will need to keep track of when the Start button was pressed and what the current time is. **This information is used for rendering, so you’ll keep it in state:**
```
const [startTime, setStartTime] = useState(null);
const [now, setNow] = useState(null);
```
When the user presses “Start”, you’ll use [`setInterval`](https://developer.mozilla.org/docs/Web/API/setInterval) in order to update the time every 10 milliseconds:
App.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import { useState } from 'react';
export default function Stopwatch() {
const [startTime, setStartTime] = useState(null);
const [now, setNow] = useState(null);
function handleStart() {
// Start counting.
setStartTime(Date.now());
setNow(Date.now());
setInterval(() => {
// Update the current time every 10ms.
setNow(Date.now());
}, 10);
}
let secondsPassed = 0;
if (startTime != null && now != null) {
secondsPassed = (now - startTime) / 1000;
}
return (
<>
<h1>Time passed: {secondsPassed.toFixed(3)}</h1>
<button onClick={handleStart}>
Start
</button>
</>
);
}
```
Show more
When the “Stop” button is pressed, you need to cancel the existing interval so that it stops updating the `now` state variable. You can do this by calling [`clearInterval`](https://developer.mozilla.org/en-US/docs/Web/API/clearInterval), but you need to give it the interval ID that was previously returned by the `setInterval` call when the user pressed Start. You need to keep the interval ID somewhere. **Since the interval ID is not used for rendering, you can keep it in a ref:**
App.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import { useState, useRef } from 'react';
export default function Stopwatch() {
const [startTime, setStartTime] = useState(null);
const [now, setNow] = useState(null);
const intervalRef = useRef(null);
function handleStart() {
setStartTime(Date.now());
setNow(Date.now());
clearInterval(intervalRef.current);
intervalRef.current = setInterval(() => {
setNow(Date.now());
}, 10);
}
function handleStop() {
clearInterval(intervalRef.current);
}
let secondsPassed = 0;
if (startTime != null && now != null) {
secondsPassed = (now - startTime) / 1000;
}
return (
<>
<h1>Time passed: {secondsPassed.toFixed(3)}</h1>
<button onClick={handleStart}>
Start
</button>
<button onClick={handleStop}>
Stop
</button>
</>
);
}
```
Show more
When a piece of information is used for rendering, keep it in state. When a piece of information is only needed by event handlers and changing it doesn’t require a re-render, using a ref may be more efficient.
## Differences between refs and state[Link for Differences between refs and state]()
Perhaps you’re thinking refs seem less “strict” than state—you can mutate them instead of always having to use a state setting function, for instance. But in most cases, you’ll want to use state. Refs are an “escape hatch” you won’t need often. Here’s how state and refs compare:
refsstate`useRef(initialValue)` returns `{ current: initialValue }``useState(initialValue)` returns the current value of a state variable and a state setter function ( `[value, setValue]`)Doesn’t trigger re-render when you change it.Triggers re-render when you change it.Mutable—you can modify and update `current`’s value outside of the rendering process.”Immutable”—you must use the state setting function to modify state variables to queue a re-render.You shouldn’t read (or write) the `current` value during rendering.You can read state at any time. However, each render has its own [snapshot](https://react.dev/learn/state-as-a-snapshot) of state which does not change.
Here is a counter button that’s implemented with state:
App.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import { useState } from 'react';
export default function Counter() {
const [count, setCount] = useState(0);
function handleClick() {
setCount(count + 1);
}
return (
<button onClick={handleClick}>
You clicked {count} times
</button>
);
}
```
Because the `count` value is displayed, it makes sense to use a state value for it. When the counter’s value is set with `setCount()`, React re-renders the component and the screen updates to reflect the new count.
If you tried to implement this with a ref, React would never re-render the component, so you’d never see the count change! See how clicking this button **does not update its text**:
App.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import { useRef } from 'react';
export default function Counter() {
let countRef = useRef(0);
function handleClick() {
// This doesn't re-render the component!
countRef.current = countRef.current + 1;
}
return (
<button onClick={handleClick}>
You clicked {countRef.current} times
</button>
);
}
```
Show more
This is why reading `ref.current` during render leads to unreliable code. If you need that, use state instead.
##### Deep Dive
#### How does useRef work inside?[Link for How does useRef work inside?]()
Show Details
Although both `useState` and `useRef` are provided by React, in principle `useRef` could be implemented *on top of* `useState`. You can imagine that inside of React, `useRef` is implemented like this:
```
// Inside of React
function useRef(initialValue) {
const [ref, unused] = useState({ current: initialValue });
return ref;
}
```
During the first render, `useRef` returns `{ current: initialValue }`. This object is stored by React, so during the next render the same object will be returned. Note how the state setter is unused in this example. It is unnecessary because `useRef` always needs to return the same object!
React provides a built-in version of `useRef` because it is common enough in practice. But you can think of it as a regular state variable without a setter. If you’re familiar with object-oriented programming, refs might remind you of instance fields—but instead of `this.something` you write `somethingRef.current`.
## When to use refs[Link for When to use refs]()
Typically, you will use a ref when your component needs to “step outside” React and communicate with external APIs—often a browser API that won’t impact the appearance of the component. Here are a few of these rare situations:
- Storing [timeout IDs](https://developer.mozilla.org/docs/Web/API/setTimeout)
- Storing and manipulating [DOM elements](https://developer.mozilla.org/docs/Web/API/Element), which we cover on [the next page](https://react.dev/learn/manipulating-the-dom-with-refs)
- Storing other objects that aren’t necessary to calculate the JSX.
If your component needs to store some value, but it doesn’t impact the rendering logic, choose refs.
## Best practices for refs[Link for Best practices for refs]()
Following these principles will make your components more predictable:
- **Treat refs as an escape hatch.** Refs are useful when you work with external systems or browser APIs. If much of your application logic and data flow relies on refs, you might want to rethink your approach.
- **Don’t read or write `ref.current` during rendering.** If some information is needed during rendering, use [state](https://react.dev/learn/state-a-components-memory) instead. Since React doesn’t know when `ref.current` changes, even reading it while rendering makes your component’s behavior difficult to predict. (The only exception to this is code like `if (!ref.current) ref.current = new Thing()` which only sets the ref once during the first render.)
Limitations of React state don’t apply to refs. For example, state acts like a [snapshot for every render](https://react.dev/learn/state-as-a-snapshot) and [doesn’t update synchronously.](https://react.dev/learn/queueing-a-series-of-state-updates) But when you mutate the current value of a ref, it changes immediately:
```
ref.current = 5;
console.log(ref.current); // 5
```
This is because **the ref itself is a regular JavaScript object,** and so it behaves like one.
You also don’t need to worry about [avoiding mutation](https://react.dev/learn/updating-objects-in-state) when you work with a ref. As long as the object you’re mutating isn’t used for rendering, React doesn’t care what you do with the ref or its contents.
## Refs and the DOM[Link for Refs and the DOM]()
You can point a ref to any value. However, the most common use case for a ref is to access a DOM element. For example, this is handy if you want to focus an input programmatically. When you pass a ref to a `ref` attribute in JSX, like `<div ref={myRef}>`, React will put the corresponding DOM element into `myRef.current`. Once the element is removed from the DOM, React will update `myRef.current` to be `null`. You can read more about this in [Manipulating the DOM with Refs.](https://react.dev/learn/manipulating-the-dom-with-refs)
## Recap[Link for Recap]()
- Refs are an escape hatch to hold onto values that aren’t used for rendering. You won’t need them often.
- A ref is a plain JavaScript object with a single property called `current`, which you can read or set.
- You can ask React to give you a ref by calling the `useRef` Hook.
- Like state, refs let you retain information between re-renders of a component.
- Unlike state, setting the ref’s `current` value does not trigger a re-render.
- Don’t read or write `ref.current` during rendering. This makes your component hard to predict.
## Try out some challenges[Link for Try out some challenges]()
1\. Fix a broken chat input 2. Fix a component failing to re-render 3. Fix debouncing 4. Read the latest state
#### Challenge 1 of 4: Fix a broken chat input[Link for this heading]()
Type a message and click “Send”. You will notice there is a three second delay before you see the “Sent!” alert. During this delay, you can see an “Undo” button. Click it. This “Undo” button is supposed to stop the “Sent!” message from appearing. It does this by calling [`clearTimeout`](https://developer.mozilla.org/en-US/docs/Web/API/clearTimeout) for the timeout ID saved during `handleSend`. However, even after “Undo” is clicked, the “Sent!” message still appears. Find why it doesn’t work, and fix it.
App.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import { useState } from 'react';
export default function Chat() {
const [text, setText] = useState('');
const [isSending, setIsSending] = useState(false);
let timeoutID = null;
function handleSend() {
setIsSending(true);
timeoutID = setTimeout(() => {
alert('Sent!');
setIsSending(false);
}, 3000);
}
function handleUndo() {
setIsSending(false);
clearTimeout(timeoutID);
}
return (
<>
<input
disabled={isSending}
value={text}
onChange={e => setText(e.target.value)}
/>
<button
disabled={isSending}
onClick={handleSend}>
{isSending ? 'Sending...' : 'Send'}
</button>
{isSending &&
<button onClick={handleUndo}>
Undo
</button>
}
</>
);
}
```
Show more
Show hint Show solution
Next Challenge
[PreviousEscape Hatches](https://react.dev/learn/escape-hatches)
[NextManipulating the DOM with Refs](https://react.dev/learn/manipulating-the-dom-with-refs) |
https://react.dev/learn/javascript-in-jsx-with-curly-braces | [Learn React](https://react.dev/learn)
[Describing the UI](https://react.dev/learn/describing-the-ui)
# JavaScript in JSX with Curly Braces[Link for this heading]()
JSX lets you write HTML-like markup inside a JavaScript file, keeping rendering logic and content in the same place. Sometimes you will want to add a little JavaScript logic or reference a dynamic property inside that markup. In this situation, you can use curly braces in your JSX to open a window to JavaScript.
### You will learn
- How to pass strings with quotes
- How to reference a JavaScript variable inside JSX with curly braces
- How to call a JavaScript function inside JSX with curly braces
- How to use a JavaScript object inside JSX with curly braces
## Passing strings with quotes[Link for Passing strings with quotes]()
When you want to pass a string attribute to JSX, you put it in single or double quotes:
App.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
export default function Avatar() {
return (
<img
className="avatar"
src="https://i.imgur.com/7vQD0fPs.jpg"
alt="Gregorio Y. Zara"
/>
);
}
```
Here, `"https://i.imgur.com/7vQD0fPs.jpg"` and `"Gregorio Y. Zara"` are being passed as strings.
But what if you want to dynamically specify the `src` or `alt` text? You could **use a value from JavaScript by replacing `"` and `"` with `{` and `}`** :
App.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
export default function Avatar() {
const avatar = 'https://i.imgur.com/7vQD0fPs.jpg';
const description = 'Gregorio Y. Zara';
return (
<img
className="avatar"
src={avatar}
alt={description}
/>
);
}
```
Notice the difference between `className="avatar"`, which specifies an `"avatar"` CSS class name that makes the image round, and `src={avatar}` that reads the value of the JavaScript variable called `avatar`. That’s because curly braces let you work with JavaScript right there in your markup!
## Using curly braces: A window into the JavaScript world[Link for Using curly braces: A window into the JavaScript world]()
JSX is a special way of writing JavaScript. That means it’s possible to use JavaScript inside it—with curly braces `{ }`. The example below first declares a name for the scientist, `name`, then embeds it with curly braces inside the `<h1>`:
App.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
export default function TodoList() {
const name = 'Gregorio Y. Zara';
return (
<h1>{name}'s To Do List</h1>
);
}
```
Try changing the `name`’s value from `'Gregorio Y. Zara'` to `'Hedy Lamarr'`. See how the list title changes?
Any JavaScript expression will work between curly braces, including function calls like `formatDate()`:
App.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
const today = new Date();
function formatDate(date) {
return new Intl.DateTimeFormat(
'en-US',
{ weekday: 'long' }
).format(date);
}
export default function TodoList() {
return (
<h1>To Do List for {formatDate(today)}</h1>
);
}
```
### Where to use curly braces[Link for Where to use curly braces]()
You can only use curly braces in two ways inside JSX:
1. **As text** directly inside a JSX tag: `<h1>{name}'s To Do List</h1>` works, but `<{tag}>Gregorio Y. Zara's To Do List</{tag}>` will not.
2. **As attributes** immediately following the `=` sign: `src={avatar}` will read the `avatar` variable, but `src="{avatar}"` will pass the string `"{avatar}"`.
## Using “double curlies”: CSS and other objects in JSX[Link for Using “double curlies”: CSS and other objects in JSX]()
In addition to strings, numbers, and other JavaScript expressions, you can even pass objects in JSX. Objects are also denoted with curly braces, like `{ name: "Hedy Lamarr", inventions: 5 }`. Therefore, to pass a JS object in JSX, you must wrap the object in another pair of curly braces: `person={{ name: "Hedy Lamarr", inventions: 5 }}`.
You may see this with inline CSS styles in JSX. React does not require you to use inline styles (CSS classes work great for most cases). But when you need an inline style, you pass an object to the `style` attribute:
App.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
export default function TodoList() {
return (
<ul style={{
backgroundColor: 'black',
color: 'pink'
}}>
<li>Improve the videophone</li>
<li>Prepare aeronautics lectures</li>
<li>Work on the alcohol-fuelled engine</li>
</ul>
);
}
```
Try changing the values of `backgroundColor` and `color`.
You can really see the JavaScript object inside the curly braces when you write it like this:
```
<ul style={
{
backgroundColor: 'black',
color: 'pink'
}
}>
```
The next time you see `{{` and `}}` in JSX, know that it’s nothing more than an object inside the JSX curlies!
### Pitfall
Inline `style` properties are written in camelCase. For example, HTML `<ul style="background-color: black">` would be written as `<ul style={{ backgroundColor: 'black' }}>` in your component.
## More fun with JavaScript objects and curly braces[Link for More fun with JavaScript objects and curly braces]()
You can move several expressions into one object, and reference them in your JSX inside curly braces:
App.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
const person = {
name: 'Gregorio Y. Zara',
theme: {
backgroundColor: 'black',
color: 'pink'
}
};
export default function TodoList() {
return (
<div style={person.theme}>
<h1>{person.name}'s Todos</h1>
<img
className="avatar"
src="https://i.imgur.com/7vQD0fPs.jpg"
alt="Gregorio Y. Zara"
/>
<ul>
<li>Improve the videophone</li>
<li>Prepare aeronautics lectures</li>
<li>Work on the alcohol-fuelled engine</li>
</ul>
</div>
);
}
```
Show more
In this example, the `person` JavaScript object contains a `name` string and a `theme` object:
```
const person = {
name: 'Gregorio Y. Zara',
theme: {
backgroundColor: 'black',
color: 'pink'
}
};
```
The component can use these values from `person` like so:
```
<div style={person.theme}>
<h1>{person.name}'s Todos</h1>
```
JSX is very minimal as a templating language because it lets you organize data and logic using JavaScript.
## Recap[Link for Recap]()
Now you know almost everything about JSX:
- JSX attributes inside quotes are passed as strings.
- Curly braces let you bring JavaScript logic and variables into your markup.
- They work inside the JSX tag content or immediately after `=` in attributes.
- `{{` and `}}` is not special syntax: it’s a JavaScript object tucked inside JSX curly braces.
## Try out some challenges[Link for Try out some challenges]()
1\. Fix the mistake 2. Extract information into an object 3. Write an expression inside JSX curly braces
#### Challenge 1 of 3: Fix the mistake[Link for this heading]()
This code crashes with an error saying `Objects are not valid as a React child`:
App.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
const person = {
name: 'Gregorio Y. Zara',
theme: {
backgroundColor: 'black',
color: 'pink'
}
};
export default function TodoList() {
return (
<div style={person.theme}>
<h1>{person}'s Todos</h1>
<img
className="avatar"
src="https://i.imgur.com/7vQD0fPs.jpg"
alt="Gregorio Y. Zara"
/>
<ul>
<li>Improve the videophone</li>
<li>Prepare aeronautics lectures</li>
<li>Work on the alcohol-fuelled engine</li>
</ul>
</div>
);
}
```
Show more
Can you find the problem?
Show hint Show solution
Next Challenge
[PreviousWriting Markup with JSX](https://react.dev/learn/writing-markup-with-jsx)
[NextPassing Props to a Component](https://react.dev/learn/passing-props-to-a-component) |
https://react.dev/learn/extracting-state-logic-into-a-reducer | [Learn React](https://react.dev/learn)
[Managing State](https://react.dev/learn/managing-state)
# Extracting State Logic into a Reducer[Link for this heading]()
Components with many state updates spread across many event handlers can get overwhelming. For these cases, you can consolidate all the state update logic outside your component in a single function, called a *reducer.*
### You will learn
- What a reducer function is
- How to refactor `useState` to `useReducer`
- When to use a reducer
- How to write one well
## Consolidate state logic with a reducer[Link for Consolidate state logic with a reducer]()
As your components grow in complexity, it can get harder to see at a glance all the different ways in which a component’s state gets updated. For example, the `TaskApp` component below holds an array of `tasks` in state and uses three different event handlers to add, remove, and edit tasks:
App.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import { useState } from 'react';
import AddTask from './AddTask.js';
import TaskList from './TaskList.js';
export default function TaskApp() {
const [tasks, setTasks] = useState(initialTasks);
function handleAddTask(text) {
setTasks([
...tasks,
{
id: nextId++,
text: text,
done: false,
},
]);
}
function handleChangeTask(task) {
setTasks(
tasks.map((t) => {
if (t.id === task.id) {
return task;
} else {
return t;
}
})
);
}
function handleDeleteTask(taskId) {
setTasks(tasks.filter((t) => t.id !== taskId));
}
return (
<>
<h1>Prague itinerary</h1>
<AddTask onAddTask={handleAddTask} />
<TaskList
tasks={tasks}
onChangeTask={handleChangeTask}
onDeleteTask={handleDeleteTask}
/>
</>
);
}
let nextId = 3;
const initialTasks = [
{id: 0, text: 'Visit Kafka Museum', done: true},
{id: 1, text: 'Watch a puppet show', done: false},
{id: 2, text: 'Lennon Wall pic', done: false},
];
```
Show more
Each of its event handlers calls `setTasks` in order to update the state. As this component grows, so does the amount of state logic sprinkled throughout it. To reduce this complexity and keep all your logic in one easy-to-access place, you can move that state logic into a single function outside your component, **called a “reducer”.**
Reducers are a different way to handle state. You can migrate from `useState` to `useReducer` in three steps:
1. **Move** from setting state to dispatching actions.
2. **Write** a reducer function.
3. **Use** the reducer from your component.
### Step 1: Move from setting state to dispatching actions[Link for Step 1: Move from setting state to dispatching actions]()
Your event handlers currently specify *what to do* by setting state:
```
function handleAddTask(text) {
setTasks([
...tasks,
{
id: nextId++,
text: text,
done: false,
},
]);
}
function handleChangeTask(task) {
setTasks(
tasks.map((t) => {
if (t.id === task.id) {
return task;
} else {
return t;
}
})
);
}
function handleDeleteTask(taskId) {
setTasks(tasks.filter((t) => t.id !== taskId));
}
```
Remove all the state setting logic. What you are left with are three event handlers:
- `handleAddTask(text)` is called when the user presses “Add”.
- `handleChangeTask(task)` is called when the user toggles a task or presses “Save”.
- `handleDeleteTask(taskId)` is called when the user presses “Delete”.
Managing state with reducers is slightly different from directly setting state. Instead of telling React “what to do” by setting state, you specify “what the user just did” by dispatching “actions” from your event handlers. (The state update logic will live elsewhere!) So instead of “setting `tasks`” via an event handler, you’re dispatching an “added/changed/deleted a task” action. This is more descriptive of the user’s intent.
```
function handleAddTask(text) {
dispatch({
type: 'added',
id: nextId++,
text: text,
});
}
function handleChangeTask(task) {
dispatch({
type: 'changed',
task: task,
});
}
function handleDeleteTask(taskId) {
dispatch({
type: 'deleted',
id: taskId,
});
}
```
The object you pass to `dispatch` is called an “action”:
```
function handleDeleteTask(taskId) {
dispatch(
// "action" object:
{
type: 'deleted',
id: taskId,
}
);
}
```
It is a regular JavaScript object. You decide what to put in it, but generally it should contain the minimal information about *what happened*. (You will add the `dispatch` function itself in a later step.)
### Note
An action object can have any shape.
By convention, it is common to give it a string `type` that describes what happened, and pass any additional information in other fields. The `type` is specific to a component, so in this example either `'added'` or `'added_task'` would be fine. Choose a name that says what happened!
```
dispatch({
// specific to component
type: 'what_happened',
// other fields go here
});
```
### Step 2: Write a reducer function[Link for Step 2: Write a reducer function]()
A reducer function is where you will put your state logic. It takes two arguments, the current state and the action object, and it returns the next state:
```
function yourReducer(state, action) {
// return next state for React to set
}
```
React will set the state to what you return from the reducer.
To move your state setting logic from your event handlers to a reducer function in this example, you will:
1. Declare the current state (`tasks`) as the first argument.
2. Declare the `action` object as the second argument.
3. Return the *next* state from the reducer (which React will set the state to).
Here is all the state setting logic migrated to a reducer function:
```
function tasksReducer(tasks, action) {
if (action.type === 'added') {
return [
...tasks,
{
id: action.id,
text: action.text,
done: false,
},
];
} else if (action.type === 'changed') {
return tasks.map((t) => {
if (t.id === action.task.id) {
return action.task;
} else {
return t;
}
});
} else if (action.type === 'deleted') {
return tasks.filter((t) => t.id !== action.id);
} else {
throw Error('Unknown action: ' + action.type);
}
}
```
Because the reducer function takes state (`tasks`) as an argument, you can **declare it outside of your component.** This decreases the indentation level and can make your code easier to read.
### Note
The code above uses if/else statements, but it’s a convention to use [switch statements](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Statements/switch) inside reducers. The result is the same, but it can be easier to read switch statements at a glance.
We’ll be using them throughout the rest of this documentation like so:
```
function tasksReducer(tasks, action) {
switch (action.type) {
case 'added': {
return [
...tasks,
{
id: action.id,
text: action.text,
done: false,
},
];
}
case 'changed': {
return tasks.map((t) => {
if (t.id === action.task.id) {
return action.task;
} else {
return t;
}
});
}
case 'deleted': {
return tasks.filter((t) => t.id !== action.id);
}
default: {
throw Error('Unknown action: ' + action.type);
}
}
}
```
We recommend wrapping each `case` block into the `{` and `}` curly braces so that variables declared inside of different `case`s don’t clash with each other. Also, a `case` should usually end with a `return`. If you forget to `return`, the code will “fall through” to the next `case`, which can lead to mistakes!
If you’re not yet comfortable with switch statements, using if/else is completely fine.
##### Deep Dive
#### Why are reducers called this way?[Link for Why are reducers called this way?]()
Show Details
Although reducers can “reduce” the amount of code inside your component, they are actually named after the [`reduce()`](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Array/Reduce) operation that you can perform on arrays.
The `reduce()` operation lets you take an array and “accumulate” a single value out of many:
```
const arr = [1, 2, 3, 4, 5];
const sum = arr.reduce(
(result, number) => result + number
); // 1 + 2 + 3 + 4 + 5
```
The function you pass to `reduce` is known as a “reducer”. It takes the *result so far* and the *current item,* then it returns the *next result.* React reducers are an example of the same idea: they take the *state so far* and the *action*, and return the *next state.* In this way, they accumulate actions over time into state.
You could even use the `reduce()` method with an `initialState` and an array of `actions` to calculate the final state by passing your reducer function to it:
index.jsindex.htmltasksReducer.js
index.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import tasksReducer from './tasksReducer.js';
let initialState = [];
let actions = [
{type: 'added', id: 1, text: 'Visit Kafka Museum'},
{type: 'added', id: 2, text: 'Watch a puppet show'},
{type: 'deleted', id: 1},
{type: 'added', id: 3, text: 'Lennon Wall pic'},
];
let finalState = actions.reduce(tasksReducer, initialState);
const output = document.getElementById('output');
output.textContent = JSON.stringify(finalState, null, 2);
```
You probably won’t need to do this yourself, but this is similar to what React does!
### Step 3: Use the reducer from your component[Link for Step 3: Use the reducer from your component]()
Finally, you need to hook up the `tasksReducer` to your component. Import the `useReducer` Hook from React:
```
import { useReducer } from 'react';
```
Then you can replace `useState`:
```
const [tasks, setTasks] = useState(initialTasks);
```
with `useReducer` like so:
```
const [tasks, dispatch] = useReducer(tasksReducer, initialTasks);
```
The `useReducer` Hook is similar to `useState`—you must pass it an initial state and it returns a stateful value and a way to set state (in this case, the dispatch function). But it’s a little different.
The `useReducer` Hook takes two arguments:
1. A reducer function
2. An initial state
And it returns:
1. A stateful value
2. A dispatch function (to “dispatch” user actions to the reducer)
Now it’s fully wired up! Here, the reducer is declared at the bottom of the component file:
App.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import { useReducer } from 'react';
import AddTask from './AddTask.js';
import TaskList from './TaskList.js';
export default function TaskApp() {
const [tasks, dispatch] = useReducer(tasksReducer, initialTasks);
function handleAddTask(text) {
dispatch({
type: 'added',
id: nextId++,
text: text,
});
}
function handleChangeTask(task) {
dispatch({
type: 'changed',
task: task,
});
}
function handleDeleteTask(taskId) {
dispatch({
type: 'deleted',
id: taskId,
});
}
return (
<>
<h1>Prague itinerary</h1>
<AddTask onAddTask={handleAddTask} />
<TaskList
tasks={tasks}
onChangeTask={handleChangeTask}
onDeleteTask={handleDeleteTask}
/>
</>
);
}
function tasksReducer(tasks, action) {
switch (action.type) {
case 'added': {
return [
...tasks,
{
id: action.id,
text: action.text,
done: false,
},
];
}
case 'changed': {
return tasks.map((t) => {
if (t.id === action.task.id) {
return action.task;
} else {
return t;
}
});
}
case 'deleted': {
return tasks.filter((t) => t.id !== action.id);
}
default: {
throw Error('Unknown action: ' + action.type);
}
}
}
let nextId = 3;
const initialTasks = [
{id: 0, text: 'Visit Kafka Museum', done: true},
{id: 1, text: 'Watch a puppet show', done: false},
{id: 2, text: 'Lennon Wall pic', done: false},
];
```
Show more
If you want, you can even move the reducer to a different file:
App.jstasksReducer.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import { useReducer } from 'react';
import AddTask from './AddTask.js';
import TaskList from './TaskList.js';
import tasksReducer from './tasksReducer.js';
export default function TaskApp() {
const [tasks, dispatch] = useReducer(tasksReducer, initialTasks);
function handleAddTask(text) {
dispatch({
type: 'added',
id: nextId++,
text: text,
});
}
function handleChangeTask(task) {
dispatch({
type: 'changed',
task: task,
});
}
function handleDeleteTask(taskId) {
dispatch({
type: 'deleted',
id: taskId,
});
}
return (
<>
<h1>Prague itinerary</h1>
<AddTask onAddTask={handleAddTask} />
<TaskList
tasks={tasks}
onChangeTask={handleChangeTask}
onDeleteTask={handleDeleteTask}
/>
</>
);
}
let nextId = 3;
const initialTasks = [
{id: 0, text: 'Visit Kafka Museum', done: true},
{id: 1, text: 'Watch a puppet show', done: false},
{id: 2, text: 'Lennon Wall pic', done: false},
];
```
Show more
Component logic can be easier to read when you separate concerns like this. Now the event handlers only specify *what happened* by dispatching actions, and the reducer function determines *how the state updates* in response to them.
## Comparing `useState` and `useReducer`[Link for this heading]()
Reducers are not without downsides! Here’s a few ways you can compare them:
- **Code size:** Generally, with `useState` you have to write less code upfront. With `useReducer`, you have to write both a reducer function *and* dispatch actions. However, `useReducer` can help cut down on the code if many event handlers modify state in a similar way.
- **Readability:** `useState` is very easy to read when the state updates are simple. When they get more complex, they can bloat your component’s code and make it difficult to scan. In this case, `useReducer` lets you cleanly separate the *how* of update logic from the *what happened* of event handlers.
- **Debugging:** When you have a bug with `useState`, it can be difficult to tell *where* the state was set incorrectly, and *why*. With `useReducer`, you can add a console log into your reducer to see every state update, and *why* it happened (due to which `action`). If each `action` is correct, you’ll know that the mistake is in the reducer logic itself. However, you have to step through more code than with `useState`.
- **Testing:** A reducer is a pure function that doesn’t depend on your component. This means that you can export and test it separately in isolation. While generally it’s best to test components in a more realistic environment, for complex state update logic it can be useful to assert that your reducer returns a particular state for a particular initial state and action.
- **Personal preference:** Some people like reducers, others don’t. That’s okay. It’s a matter of preference. You can always convert between `useState` and `useReducer` back and forth: they are equivalent!
We recommend using a reducer if you often encounter bugs due to incorrect state updates in some component, and want to introduce more structure to its code. You don’t have to use reducers for everything: feel free to mix and match! You can even `useState` and `useReducer` in the same component.
## Writing reducers well[Link for Writing reducers well]()
Keep these two tips in mind when writing reducers:
- **Reducers must be pure.** Similar to [state updater functions](https://react.dev/learn/queueing-a-series-of-state-updates), reducers run during rendering! (Actions are queued until the next render.) This means that reducers [must be pure](https://react.dev/learn/keeping-components-pure)—same inputs always result in the same output. They should not send requests, schedule timeouts, or perform any side effects (operations that impact things outside the component). They should update [objects](https://react.dev/learn/updating-objects-in-state) and [arrays](https://react.dev/learn/updating-arrays-in-state) without mutations.
- **Each action describes a single user interaction, even if that leads to multiple changes in the data.** For example, if a user presses “Reset” on a form with five fields managed by a reducer, it makes more sense to dispatch one `reset_form` action rather than five separate `set_field` actions. If you log every action in a reducer, that log should be clear enough for you to reconstruct what interactions or responses happened in what order. This helps with debugging!
## Writing concise reducers with Immer[Link for Writing concise reducers with Immer]()
Just like with [updating objects](https://react.dev/learn/updating-objects-in-state) and [arrays](https://react.dev/learn/updating-arrays-in-state) in regular state, you can use the Immer library to make reducers more concise. Here, [`useImmerReducer`](https://github.com/immerjs/use-immer) lets you mutate the state with `push` or `arr[i] =` assignment:
package.jsonApp.js
package.json
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
{
"dependencies": {
"immer": "1.7.3",
"react": "latest",
"react-dom": "latest",
"react-scripts": "latest",
"use-immer": "0.5.1"
},
"scripts": {
"start": "react-scripts start",
"build": "react-scripts build",
"test": "react-scripts test --env=jsdom",
"eject": "react-scripts eject"
},
"devDependencies": {}
}
```
Reducers must be pure, so they shouldn’t mutate state. But Immer provides you with a special `draft` object which is safe to mutate. Under the hood, Immer will create a copy of your state with the changes you made to the `draft`. This is why reducers managed by `useImmerReducer` can mutate their first argument and don’t need to return state.
## Recap[Link for Recap]()
- To convert from `useState` to `useReducer`:
1. Dispatch actions from event handlers.
2. Write a reducer function that returns the next state for a given state and action.
3. Replace `useState` with `useReducer`.
- Reducers require you to write a bit more code, but they help with debugging and testing.
- Reducers must be pure.
- Each action describes a single user interaction.
- Use Immer if you want to write reducers in a mutating style.
## Try out some challenges[Link for Try out some challenges]()
1\. Dispatch actions from event handlers 2. Clear the input on sending a message 3. Restore input values when switching between tabs 4. Implement `useReducer` from scratch
#### Challenge 1 of 4: Dispatch actions from event handlers[Link for this heading]()
Currently, the event handlers in `ContactList.js` and `Chat.js` have `// TODO` comments. This is why typing into the input doesn’t work, and clicking on the buttons doesn’t change the selected recipient.
Replace these two `// TODO`s with the code to `dispatch` the corresponding actions. To see the expected shape and the type of the actions, check the reducer in `messengerReducer.js`. The reducer is already written so you won’t need to change it. You only need to dispatch the actions in `ContactList.js` and `Chat.js`.
App.jsmessengerReducer.jsContactList.jsChat.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import { useReducer } from 'react';
import Chat from './Chat.js';
import ContactList from './ContactList.js';
import { initialState, messengerReducer } from './messengerReducer';
export default function Messenger() {
const [state, dispatch] = useReducer(messengerReducer, initialState);
const message = state.message;
const contact = contacts.find((c) => c.id === state.selectedId);
return (
<div>
<ContactList
contacts={contacts}
selectedId={state.selectedId}
dispatch={dispatch}
/>
<Chat
key={contact.id}
message={message}
contact={contact}
dispatch={dispatch}
/>
</div>
);
}
const contacts = [
{id: 0, name: 'Taylor', email: '[email protected]'},
{id: 1, name: 'Alice', email: '[email protected]'},
{id: 2, name: 'Bob', email: '[email protected]'},
];
```
Show more
Show hint Show solution
Next Challenge
[PreviousPreserving and Resetting State](https://react.dev/learn/preserving-and-resetting-state)
[NextPassing Data Deeply with Context](https://react.dev/learn/passing-data-deeply-with-context) |
https://react.dev/learn/sharing-state-between-components | [Learn React](https://react.dev/learn)
[Managing State](https://react.dev/learn/managing-state)
# Sharing State Between Components[Link for this heading]()
Sometimes, you want the state of two components to always change together. To do it, remove state from both of them, move it to their closest common parent, and then pass it down to them via props. This is known as *lifting state up,* and it’s one of the most common things you will do writing React code.
### You will learn
- How to share state between components by lifting it up
- What are controlled and uncontrolled components
## Lifting state up by example[Link for Lifting state up by example]()
In this example, a parent `Accordion` component renders two separate `Panel`s:
- `Accordion`
- `Panel`
- `Panel`
Each `Panel` component has a boolean `isActive` state that determines whether its content is visible.
Press the Show button for both panels:
App.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import { useState } from 'react';
function Panel({ title, children }) {
const [isActive, setIsActive] = useState(false);
return (
<section className="panel">
<h3>{title}</h3>
{isActive ? (
<p>{children}</p>
) : (
<button onClick={() => setIsActive(true)}>
Show
</button>
)}
</section>
);
}
export default function Accordion() {
return (
<>
<h2>Almaty, Kazakhstan</h2>
<Panel title="About">
With a population of about 2 million, Almaty is Kazakhstan's largest city. From 1929 to 1997, it was its capital city.
</Panel>
<Panel title="Etymology">
The name comes from <span lang="kk-KZ">алма</span>, the Kazakh word for "apple" and is often translated as "full of apples". In fact, the region surrounding Almaty is thought to be the ancestral home of the apple, and the wild <i lang="la">Malus sieversii</i> is considered a likely candidate for the ancestor of the modern domestic apple.
</Panel>
</>
);
}
```
Show more
Notice how pressing one panel’s button does not affect the other panel—they are independent.


Initially, each `Panel`’s `isActive` state is `false`, so they both appear collapsed


Clicking either `Panel`’s button will only update that `Panel`’s `isActive` state alone
**But now let’s say you want to change it so that only one panel is expanded at any given time.** With that design, expanding the second panel should collapse the first one. How would you do that?
To coordinate these two panels, you need to “lift their state up” to a parent component in three steps:
1. **Remove** state from the child components.
2. **Pass** hardcoded data from the common parent.
3. **Add** state to the common parent and pass it down together with the event handlers.
This will allow the `Accordion` component to coordinate both `Panel`s and only expand one at a time.
### Step 1: Remove state from the child components[Link for Step 1: Remove state from the child components]()
You will give control of the `Panel`’s `isActive` to its parent component. This means that the parent component will pass `isActive` to `Panel` as a prop instead. Start by **removing this line** from the `Panel` component:
```
const [isActive, setIsActive] = useState(false);
```
And instead, add `isActive` to the `Panel`’s list of props:
```
function Panel({ title, children, isActive }) {
```
Now the `Panel`’s parent component can *control* `isActive` by [passing it down as a prop.](https://react.dev/learn/passing-props-to-a-component) Conversely, the `Panel` component now has *no control* over the value of `isActive`—it’s now up to the parent component!
### Step 2: Pass hardcoded data from the common parent[Link for Step 2: Pass hardcoded data from the common parent]()
To lift state up, you must locate the closest common parent component of *both* of the child components that you want to coordinate:
- `Accordion` *(closest common parent)*
- `Panel`
- `Panel`
In this example, it’s the `Accordion` component. Since it’s above both panels and can control their props, it will become the “source of truth” for which panel is currently active. Make the `Accordion` component pass a hardcoded value of `isActive` (for example, `true`) to both panels:
App.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import { useState } from 'react';
export default function Accordion() {
return (
<>
<h2>Almaty, Kazakhstan</h2>
<Panel title="About" isActive={true}>
With a population of about 2 million, Almaty is Kazakhstan's largest city. From 1929 to 1997, it was its capital city.
</Panel>
<Panel title="Etymology" isActive={true}>
The name comes from <span lang="kk-KZ">алма</span>, the Kazakh word for "apple" and is often translated as "full of apples". In fact, the region surrounding Almaty is thought to be the ancestral home of the apple, and the wild <i lang="la">Malus sieversii</i> is considered a likely candidate for the ancestor of the modern domestic apple.
</Panel>
</>
);
}
function Panel({ title, children, isActive }) {
return (
<section className="panel">
<h3>{title}</h3>
{isActive ? (
<p>{children}</p>
) : (
<button onClick={() => setIsActive(true)}>
Show
</button>
)}
</section>
);
}
```
Show more
Try editing the hardcoded `isActive` values in the `Accordion` component and see the result on the screen.
### Step 3: Add state to the common parent[Link for Step 3: Add state to the common parent]()
Lifting state up often changes the nature of what you’re storing as state.
In this case, only one panel should be active at a time. This means that the `Accordion` common parent component needs to keep track of *which* panel is the active one. Instead of a `boolean` value, it could use a number as the index of the active `Panel` for the state variable:
```
const [activeIndex, setActiveIndex] = useState(0);
```
When the `activeIndex` is `0`, the first panel is active, and when it’s `1`, it’s the second one.
Clicking the “Show” button in either `Panel` needs to change the active index in `Accordion`. A `Panel` can’t set the `activeIndex` state directly because it’s defined inside the `Accordion`. The `Accordion` component needs to *explicitly allow* the `Panel` component to change its state by [passing an event handler down as a prop](https://react.dev/learn/responding-to-events):
```
<>
<Panel
isActive={activeIndex === 0}
onShow={() => setActiveIndex(0)}
>
...
</Panel>
<Panel
isActive={activeIndex === 1}
onShow={() => setActiveIndex(1)}
>
...
</Panel>
</>
```
The `<button>` inside the `Panel` will now use the `onShow` prop as its click event handler:
App.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import { useState } from 'react';
export default function Accordion() {
const [activeIndex, setActiveIndex] = useState(0);
return (
<>
<h2>Almaty, Kazakhstan</h2>
<Panel
title="About"
isActive={activeIndex === 0}
onShow={() => setActiveIndex(0)}
>
With a population of about 2 million, Almaty is Kazakhstan's largest city. From 1929 to 1997, it was its capital city.
</Panel>
<Panel
title="Etymology"
isActive={activeIndex === 1}
onShow={() => setActiveIndex(1)}
>
The name comes from <span lang="kk-KZ">алма</span>, the Kazakh word for "apple" and is often translated as "full of apples". In fact, the region surrounding Almaty is thought to be the ancestral home of the apple, and the wild <i lang="la">Malus sieversii</i> is considered a likely candidate for the ancestor of the modern domestic apple.
</Panel>
</>
);
}
function Panel({
title,
children,
isActive,
onShow
}) {
return (
<section className="panel">
<h3>{title}</h3>
{isActive ? (
<p>{children}</p>
) : (
<button onClick={onShow}>
Show
</button>
)}
</section>
);
}
```
Show more
This completes lifting state up! Moving state into the common parent component allowed you to coordinate the two panels. Using the active index instead of two “is shown” flags ensured that only one panel is active at a given time. And passing down the event handler to the child allowed the child to change the parent’s state.


Initially, `Accordion`’s `activeIndex` is `0`, so the first `Panel` receives `isActive = true`


When `Accordion`’s `activeIndex` state changes to `1`, the second `Panel` receives `isActive = true` instead
##### Deep Dive
#### Controlled and uncontrolled components[Link for Controlled and uncontrolled components]()
Show Details
It is common to call a component with some local state “uncontrolled”. For example, the original `Panel` component with an `isActive` state variable is uncontrolled because its parent cannot influence whether the panel is active or not.
In contrast, you might say a component is “controlled” when the important information in it is driven by props rather than its own local state. This lets the parent component fully specify its behavior. The final `Panel` component with the `isActive` prop is controlled by the `Accordion` component.
Uncontrolled components are easier to use within their parents because they require less configuration. But they’re less flexible when you want to coordinate them together. Controlled components are maximally flexible, but they require the parent components to fully configure them with props.
In practice, “controlled” and “uncontrolled” aren’t strict technical terms—each component usually has some mix of both local state and props. However, this is a useful way to talk about how components are designed and what capabilities they offer.
When writing a component, consider which information in it should be controlled (via props), and which information should be uncontrolled (via state). But you can always change your mind and refactor later.
## A single source of truth for each state[Link for A single source of truth for each state]()
In a React application, many components will have their own state. Some state may “live” close to the leaf components (components at the bottom of the tree) like inputs. Other state may “live” closer to the top of the app. For example, even client-side routing libraries are usually implemented by storing the current route in the React state, and passing it down by props!
**For each unique piece of state, you will choose the component that “owns” it.** This principle is also known as having a [“single source of truth”.](https://en.wikipedia.org/wiki/Single_source_of_truth) It doesn’t mean that all state lives in one place—but that for *each* piece of state, there is a *specific* component that holds that piece of information. Instead of duplicating shared state between components, *lift it up* to their common shared parent, and *pass it down* to the children that need it.
Your app will change as you work on it. It is common that you will move state down or back up while you’re still figuring out where each piece of the state “lives”. This is all part of the process!
To see what this feels like in practice with a few more components, read [Thinking in React.](https://react.dev/learn/thinking-in-react)
## Recap[Link for Recap]()
- When you want to coordinate two components, move their state to their common parent.
- Then pass the information down through props from their common parent.
- Finally, pass the event handlers down so that the children can change the parent’s state.
- It’s useful to consider components as “controlled” (driven by props) or “uncontrolled” (driven by state).
## Try out some challenges[Link for Try out some challenges]()
1\. Synced inputs 2. Filtering a list
#### Challenge 1 of 2: Synced inputs[Link for this heading]()
These two inputs are independent. Make them stay in sync: editing one input should update the other input with the same text, and vice versa.
App.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import { useState } from 'react';
export default function SyncedInputs() {
return (
<>
<Input label="First input" />
<Input label="Second input" />
</>
);
}
function Input({ label }) {
const [text, setText] = useState('');
function handleChange(e) {
setText(e.target.value);
}
return (
<label>
{label}
{' '}
<input
value={text}
onChange={handleChange}
/>
</label>
);
}
```
Show more
Show hint Show solution
Next Challenge
[PreviousChoosing the State Structure](https://react.dev/learn/choosing-the-state-structure)
[NextPreserving and Resetting State](https://react.dev/learn/preserving-and-resetting-state) |
https://react.dev/learn/passing-data-deeply-with-context | [Learn React](https://react.dev/learn)
[Managing State](https://react.dev/learn/managing-state)
# Passing Data Deeply with Context[Link for this heading]()
Usually, you will pass information from a parent component to a child component via props. But passing props can become verbose and inconvenient if you have to pass them through many components in the middle, or if many components in your app need the same information. *Context* lets the parent component make some information available to any component in the tree below it—no matter how deep—without passing it explicitly through props.
### You will learn
- What “prop drilling” is
- How to replace repetitive prop passing with context
- Common use cases for context
- Common alternatives to context
## The problem with passing props[Link for The problem with passing props]()
[Passing props](https://react.dev/learn/passing-props-to-a-component) is a great way to explicitly pipe data through your UI tree to the components that use it.
But passing props can become verbose and inconvenient when you need to pass some prop deeply through the tree, or if many components need the same prop. The nearest common ancestor could be far removed from the components that need data, and [lifting state up](https://react.dev/learn/sharing-state-between-components) that high can lead to a situation called “prop drilling”.
Lifting state up


Prop drilling


Wouldn’t it be great if there were a way to “teleport” data to the components in the tree that need it without passing props? With React’s context feature, there is!
## Context: an alternative to passing props[Link for Context: an alternative to passing props]()
Context lets a parent component provide data to the entire tree below it. There are many uses for context. Here is one example. Consider this `Heading` component that accepts a `level` for its size:
App.jsSection.jsHeading.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import Heading from './Heading.js';
import Section from './Section.js';
export default function Page() {
return (
<Section>
<Heading level={1}>Title</Heading>
<Heading level={2}>Heading</Heading>
<Heading level={3}>Sub-heading</Heading>
<Heading level={4}>Sub-sub-heading</Heading>
<Heading level={5}>Sub-sub-sub-heading</Heading>
<Heading level={6}>Sub-sub-sub-sub-heading</Heading>
</Section>
);
}
```
Let’s say you want multiple headings within the same `Section` to always have the same size:
App.jsSection.jsHeading.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import Heading from './Heading.js';
import Section from './Section.js';
export default function Page() {
return (
<Section>
<Heading level={1}>Title</Heading>
<Section>
<Heading level={2}>Heading</Heading>
<Heading level={2}>Heading</Heading>
<Heading level={2}>Heading</Heading>
<Section>
<Heading level={3}>Sub-heading</Heading>
<Heading level={3}>Sub-heading</Heading>
<Heading level={3}>Sub-heading</Heading>
<Section>
<Heading level={4}>Sub-sub-heading</Heading>
<Heading level={4}>Sub-sub-heading</Heading>
<Heading level={4}>Sub-sub-heading</Heading>
</Section>
</Section>
</Section>
</Section>
);
}
```
Show more
Currently, you pass the `level` prop to each `<Heading>` separately:
```
<Section>
<Heading level={3}>About</Heading>
<Heading level={3}>Photos</Heading>
<Heading level={3}>Videos</Heading>
</Section>
```
It would be nice if you could pass the `level` prop to the `<Section>` component instead and remove it from the `<Heading>`. This way you could enforce that all headings in the same section have the same size:
```
<Section level={3}>
<Heading>About</Heading>
<Heading>Photos</Heading>
<Heading>Videos</Heading>
</Section>
```
But how can the `<Heading>` component know the level of its closest `<Section>`? **That would require some way for a child to “ask” for data from somewhere above in the tree.**
You can’t do it with props alone. This is where context comes into play. You will do it in three steps:
1. **Create** a context. (You can call it `LevelContext`, since it’s for the heading level.)
2. **Use** that context from the component that needs the data. (`Heading` will use `LevelContext`.)
3. **Provide** that context from the component that specifies the data. (`Section` will provide `LevelContext`.)
Context lets a parent—even a distant one!—provide some data to the entire tree inside of it.
Using context in close children


Using context in distant children


### Step 1: Create the context[Link for Step 1: Create the context]()
First, you need to create the context. You’ll need to **export it from a file** so that your components can use it:
App.jsSection.jsHeading.jsLevelContext.js
LevelContext.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import { createContext } from 'react';
export const LevelContext = createContext(1);
```
The only argument to `createContext` is the *default* value. Here, `1` refers to the biggest heading level, but you could pass any kind of value (even an object). You will see the significance of the default value in the next step.
### Step 2: Use the context[Link for Step 2: Use the context]()
Import the `useContext` Hook from React and your context:
```
import { useContext } from 'react';
import { LevelContext } from './LevelContext.js';
```
Currently, the `Heading` component reads `level` from props:
```
export default function Heading({ level, children }) {
// ...
}
```
Instead, remove the `level` prop and read the value from the context you just imported, `LevelContext`:
```
export default function Heading({ children }) {
const level = useContext(LevelContext);
// ...
}
```
`useContext` is a Hook. Just like `useState` and `useReducer`, you can only call a Hook immediately inside a React component (not inside loops or conditions). **`useContext` tells React that the `Heading` component wants to read the `LevelContext`.**
Now that the `Heading` component doesn’t have a `level` prop, you don’t need to pass the level prop to `Heading` in your JSX like this anymore:
```
<Section>
<Heading level={4}>Sub-sub-heading</Heading>
<Heading level={4}>Sub-sub-heading</Heading>
<Heading level={4}>Sub-sub-heading</Heading>
</Section>
```
Update the JSX so that it’s the `Section` that receives it instead:
```
<Section level={4}>
<Heading>Sub-sub-heading</Heading>
<Heading>Sub-sub-heading</Heading>
<Heading>Sub-sub-heading</Heading>
</Section>
```
As a reminder, this is the markup that you were trying to get working:
App.jsSection.jsHeading.jsLevelContext.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import Heading from './Heading.js';
import Section from './Section.js';
export default function Page() {
return (
<Section level={1}>
<Heading>Title</Heading>
<Section level={2}>
<Heading>Heading</Heading>
<Heading>Heading</Heading>
<Heading>Heading</Heading>
<Section level={3}>
<Heading>Sub-heading</Heading>
<Heading>Sub-heading</Heading>
<Heading>Sub-heading</Heading>
<Section level={4}>
<Heading>Sub-sub-heading</Heading>
<Heading>Sub-sub-heading</Heading>
<Heading>Sub-sub-heading</Heading>
</Section>
</Section>
</Section>
</Section>
);
}
```
Show more
Notice this example doesn’t quite work, yet! All the headings have the same size because **even though you’re *using* the context, you have not *provided* it yet.** React doesn’t know where to get it!
If you don’t provide the context, React will use the default value you’ve specified in the previous step. In this example, you specified `1` as the argument to `createContext`, so `useContext(LevelContext)` returns `1`, setting all those headings to `<h1>`. Let’s fix this problem by having each `Section` provide its own context.
### Step 3: Provide the context[Link for Step 3: Provide the context]()
The `Section` component currently renders its children:
```
export default function Section({ children }) {
return (
<section className="section">
{children}
</section>
);
}
```
**Wrap them with a context provider** to provide the `LevelContext` to them:
```
import { LevelContext } from './LevelContext.js';
export default function Section({ level, children }) {
return (
<section className="section">
<LevelContext.Provider value={level}>
{children}
</LevelContext.Provider>
</section>
);
}
```
This tells React: “if any component inside this `<Section>` asks for `LevelContext`, give them this `level`.” The component will use the value of the nearest `<LevelContext.Provider>` in the UI tree above it.
App.jsSection.jsHeading.jsLevelContext.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import Heading from './Heading.js';
import Section from './Section.js';
export default function Page() {
return (
<Section level={1}>
<Heading>Title</Heading>
<Section level={2}>
<Heading>Heading</Heading>
<Heading>Heading</Heading>
<Heading>Heading</Heading>
<Section level={3}>
<Heading>Sub-heading</Heading>
<Heading>Sub-heading</Heading>
<Heading>Sub-heading</Heading>
<Section level={4}>
<Heading>Sub-sub-heading</Heading>
<Heading>Sub-sub-heading</Heading>
<Heading>Sub-sub-heading</Heading>
</Section>
</Section>
</Section>
</Section>
);
}
```
Show more
It’s the same result as the original code, but you did not need to pass the `level` prop to each `Heading` component! Instead, it “figures out” its heading level by asking the closest `Section` above:
1. You pass a `level` prop to the `<Section>`.
2. `Section` wraps its children into `<LevelContext.Provider value={level}>`.
3. `Heading` asks the closest value of `LevelContext` above with `useContext(LevelContext)`.
## Using and providing context from the same component[Link for Using and providing context from the same component]()
Currently, you still have to specify each section’s `level` manually:
```
export default function Page() {
return (
<Section level={1}>
...
<Section level={2}>
...
<Section level={3}>
...
```
Since context lets you read information from a component above, each `Section` could read the `level` from the `Section` above, and pass `level + 1` down automatically. Here is how you could do it:
```
import { useContext } from 'react';
import { LevelContext } from './LevelContext.js';
export default function Section({ children }) {
const level = useContext(LevelContext);
return (
<section className="section">
<LevelContext.Provider value={level + 1}>
{children}
</LevelContext.Provider>
</section>
);
}
```
With this change, you don’t need to pass the `level` prop *either* to the `<Section>` or to the `<Heading>`:
App.jsSection.jsHeading.jsLevelContext.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import Heading from './Heading.js';
import Section from './Section.js';
export default function Page() {
return (
<Section>
<Heading>Title</Heading>
<Section>
<Heading>Heading</Heading>
<Heading>Heading</Heading>
<Heading>Heading</Heading>
<Section>
<Heading>Sub-heading</Heading>
<Heading>Sub-heading</Heading>
<Heading>Sub-heading</Heading>
<Section>
<Heading>Sub-sub-heading</Heading>
<Heading>Sub-sub-heading</Heading>
<Heading>Sub-sub-heading</Heading>
</Section>
</Section>
</Section>
</Section>
);
}
```
Show more
Now both `Heading` and `Section` read the `LevelContext` to figure out how “deep” they are. And the `Section` wraps its children into the `LevelContext` to specify that anything inside of it is at a “deeper” level.
### Note
This example uses heading levels because they show visually how nested components can override context. But context is useful for many other use cases too. You can pass down any information needed by the entire subtree: the current color theme, the currently logged in user, and so on.
## Context passes through intermediate components[Link for Context passes through intermediate components]()
You can insert as many components as you like between the component that provides context and the one that uses it. This includes both built-in components like `<div>` and components you might build yourself.
In this example, the same `Post` component (with a dashed border) is rendered at two different nesting levels. Notice that the `<Heading>` inside of it gets its level automatically from the closest `<Section>`:
App.jsSection.jsHeading.jsLevelContext.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import Heading from './Heading.js';
import Section from './Section.js';
export default function ProfilePage() {
return (
<Section>
<Heading>My Profile</Heading>
<Post
title="Hello traveller!"
body="Read about my adventures."
/>
<AllPosts />
</Section>
);
}
function AllPosts() {
return (
<Section>
<Heading>Posts</Heading>
<RecentPosts />
</Section>
);
}
function RecentPosts() {
return (
<Section>
<Heading>Recent Posts</Heading>
<Post
title="Flavors of Lisbon"
body="...those pastéis de nata!"
/>
<Post
title="Buenos Aires in the rhythm of tango"
body="I loved it!"
/>
</Section>
);
}
function Post({ title, body }) {
return (
<Section isFancy={true}>
<Heading>
{title}
</Heading>
<p><i>{body}</i></p>
</Section>
);
}
```
Show more
You didn’t do anything special for this to work. A `Section` specifies the context for the tree inside it, so you can insert a `<Heading>` anywhere, and it will have the correct size. Try it in the sandbox above!
**Context lets you write components that “adapt to their surroundings” and display themselves differently depending on *where* (or, in other words, *in which context*) they are being rendered.**
How context works might remind you of [CSS property inheritance.](https://developer.mozilla.org/en-US/docs/Web/CSS/inheritance) In CSS, you can specify `color: blue` for a `<div>`, and any DOM node inside of it, no matter how deep, will inherit that color unless some other DOM node in the middle overrides it with `color: green`. Similarly, in React, the only way to override some context coming from above is to wrap children into a context provider with a different value.
In CSS, different properties like `color` and `background-color` don’t override each other. You can set all `<div>`’s `color` to red without impacting `background-color`. Similarly, **different React contexts don’t override each other.** Each context that you make with `createContext()` is completely separate from other ones, and ties together components using and providing *that particular* context. One component may use or provide many different contexts without a problem.
## Before you use context[Link for Before you use context]()
Context is very tempting to use! However, this also means it’s too easy to overuse it. **Just because you need to pass some props several levels deep doesn’t mean you should put that information into context.**
Here’s a few alternatives you should consider before using context:
1. **Start by [passing props.](https://react.dev/learn/passing-props-to-a-component)** If your components are not trivial, it’s not unusual to pass a dozen props down through a dozen components. It may feel like a slog, but it makes it very clear which components use which data! The person maintaining your code will be glad you’ve made the data flow explicit with props.
2. **Extract components and [pass JSX as `children`](https://react.dev/learn/passing-props-to-a-component) to them.** If you pass some data through many layers of intermediate components that don’t use that data (and only pass it further down), this often means that you forgot to extract some components along the way. For example, maybe you pass data props like `posts` to visual components that don’t use them directly, like `<Layout posts={posts} />`. Instead, make `Layout` take `children` as a prop, and render `<Layout><Posts posts={posts} /></Layout>`. This reduces the number of layers between the component specifying the data and the one that needs it.
If neither of these approaches works well for you, consider context.
## Use cases for context[Link for Use cases for context]()
- **Theming:** If your app lets the user change its appearance (e.g. dark mode), you can put a context provider at the top of your app, and use that context in components that need to adjust their visual look.
- **Current account:** Many components might need to know the currently logged in user. Putting it in context makes it convenient to read it anywhere in the tree. Some apps also let you operate multiple accounts at the same time (e.g. to leave a comment as a different user). In those cases, it can be convenient to wrap a part of the UI into a nested provider with a different current account value.
- **Routing:** Most routing solutions use context internally to hold the current route. This is how every link “knows” whether it’s active or not. If you build your own router, you might want to do it too.
- **Managing state:** As your app grows, you might end up with a lot of state closer to the top of your app. Many distant components below may want to change it. It is common to [use a reducer together with context](https://react.dev/learn/scaling-up-with-reducer-and-context) to manage complex state and pass it down to distant components without too much hassle.
Context is not limited to static values. If you pass a different value on the next render, React will update all the components reading it below! This is why context is often used in combination with state.
In general, if some information is needed by distant components in different parts of the tree, it’s a good indication that context will help you.
## Recap[Link for Recap]()
- Context lets a component provide some information to the entire tree below it.
- To pass context:
1. Create and export it with `export const MyContext = createContext(defaultValue)`.
2. Pass it to the `useContext(MyContext)` Hook to read it in any child component, no matter how deep.
3. Wrap children into `<MyContext.Provider value={...}>` to provide it from a parent.
- Context passes through any components in the middle.
- Context lets you write components that “adapt to their surroundings”.
- Before you use context, try passing props or passing JSX as `children`.
## Try out some challenges[Link for Try out some challenges]()
#### Challenge 1 of 1: Replace prop drilling with context[Link for this heading]()
In this example, toggling the checkbox changes the `imageSize` prop passed to each `<PlaceImage>`. The checkbox state is held in the top-level `App` component, but each `<PlaceImage>` needs to be aware of it.
Currently, `App` passes `imageSize` to `List`, which passes it to each `Place`, which passes it to the `PlaceImage`. Remove the `imageSize` prop, and instead pass it from the `App` component directly to `PlaceImage`.
You can declare context in `Context.js`.
App.jsContext.jsdata.jsutils.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import { useState } from 'react';
import { places } from './data.js';
import { getImageUrl } from './utils.js';
export default function App() {
const [isLarge, setIsLarge] = useState(false);
const imageSize = isLarge ? 150 : 100;
return (
<>
<label>
<input
type="checkbox"
checked={isLarge}
onChange={e => {
setIsLarge(e.target.checked);
}}
/>
Use large images
</label>
<hr />
<List imageSize={imageSize} />
</>
)
}
function List({ imageSize }) {
const listItems = places.map(place =>
<li key={place.id}>
<Place
place={place}
imageSize={imageSize}
/>
</li>
);
return <ul>{listItems}</ul>;
}
function Place({ place, imageSize }) {
return (
<>
<PlaceImage
place={place}
imageSize={imageSize}
/>
<p>
<b>{place.name}</b>
{': ' + place.description}
</p>
</>
);
}
function PlaceImage({ place, imageSize }) {
return (
<img
src={getImageUrl(place)}
alt={place.name}
width={imageSize}
height={imageSize}
/>
);
}
```
Show more
Show solution
[PreviousExtracting State Logic into a Reducer](https://react.dev/learn/extracting-state-logic-into-a-reducer)
[NextScaling Up with Reducer and Context](https://react.dev/learn/scaling-up-with-reducer-and-context) |
https://react.dev/learn/you-might-not-need-an-effect | [Learn React](https://react.dev/learn)
[Escape Hatches](https://react.dev/learn/escape-hatches)
# You Might Not Need an Effect[Link for this heading]()
Effects are an escape hatch from the React paradigm. They let you “step outside” of React and synchronize your components with some external system like a non-React widget, network, or the browser DOM. If there is no external system involved (for example, if you want to update a component’s state when some props or state change), you shouldn’t need an Effect. Removing unnecessary Effects will make your code easier to follow, faster to run, and less error-prone.
### You will learn
- Why and how to remove unnecessary Effects from your components
- How to cache expensive computations without Effects
- How to reset and adjust component state without Effects
- How to share logic between event handlers
- Which logic should be moved to event handlers
- How to notify parent components about changes
## How to remove unnecessary Effects[Link for How to remove unnecessary Effects]()
There are two common cases in which you don’t need Effects:
- **You don’t need Effects to transform data for rendering.** For example, let’s say you want to filter a list before displaying it. You might feel tempted to write an Effect that updates a state variable when the list changes. However, this is inefficient. When you update the state, React will first call your component functions to calculate what should be on the screen. Then React will [“commit”](https://react.dev/learn/render-and-commit) these changes to the DOM, updating the screen. Then React will run your Effects. If your Effect *also* immediately updates the state, this restarts the whole process from scratch! To avoid the unnecessary render passes, transform all the data at the top level of your components. That code will automatically re-run whenever your props or state change.
- **You don’t need Effects to handle user events.** For example, let’s say you want to send an `/api/buy` POST request and show a notification when the user buys a product. In the Buy button click event handler, you know exactly what happened. By the time an Effect runs, you don’t know *what* the user did (for example, which button was clicked). This is why you’ll usually handle user events in the corresponding event handlers.
You *do* need Effects to [synchronize](https://react.dev/learn/synchronizing-with-effects) with external systems. For example, you can write an Effect that keeps a jQuery widget synchronized with the React state. You can also fetch data with Effects: for example, you can synchronize the search results with the current search query. Keep in mind that modern [frameworks](https://react.dev/learn/start-a-new-react-project) provide more efficient built-in data fetching mechanisms than writing Effects directly in your components.
To help you gain the right intuition, let’s look at some common concrete examples!
### Updating state based on props or state[Link for Updating state based on props or state]()
Suppose you have a component with two state variables: `firstName` and `lastName`. You want to calculate a `fullName` from them by concatenating them. Moreover, you’d like `fullName` to update whenever `firstName` or `lastName` change. Your first instinct might be to add a `fullName` state variable and update it in an Effect:
```
function Form() {
const [firstName, setFirstName] = useState('Taylor');
const [lastName, setLastName] = useState('Swift');
// 🔴 Avoid: redundant state and unnecessary Effect
const [fullName, setFullName] = useState('');
useEffect(() => {
setFullName(firstName + ' ' + lastName);
}, [firstName, lastName]);
// ...
}
```
This is more complicated than necessary. It is inefficient too: it does an entire render pass with a stale value for `fullName`, then immediately re-renders with the updated value. Remove the state variable and the Effect:
```
function Form() {
const [firstName, setFirstName] = useState('Taylor');
const [lastName, setLastName] = useState('Swift');
// ✅ Good: calculated during rendering
const fullName = firstName + ' ' + lastName;
// ...
}
```
**When something can be calculated from the existing props or state, [don’t put it in state.](https://react.dev/learn/choosing-the-state-structure) Instead, calculate it during rendering.** This makes your code faster (you avoid the extra “cascading” updates), simpler (you remove some code), and less error-prone (you avoid bugs caused by different state variables getting out of sync with each other). If this approach feels new to you, [Thinking in React](https://react.dev/learn/thinking-in-react) explains what should go into state.
### Caching expensive calculations[Link for Caching expensive calculations]()
This component computes `visibleTodos` by taking the `todos` it receives by props and filtering them according to the `filter` prop. You might feel tempted to store the result in state and update it from an Effect:
```
function TodoList({ todos, filter }) {
const [newTodo, setNewTodo] = useState('');
// 🔴 Avoid: redundant state and unnecessary Effect
const [visibleTodos, setVisibleTodos] = useState([]);
useEffect(() => {
setVisibleTodos(getFilteredTodos(todos, filter));
}, [todos, filter]);
// ...
}
```
Like in the earlier example, this is both unnecessary and inefficient. First, remove the state and the Effect:
```
function TodoList({ todos, filter }) {
const [newTodo, setNewTodo] = useState('');
// ✅ This is fine if getFilteredTodos() is not slow.
const visibleTodos = getFilteredTodos(todos, filter);
// ...
}
```
Usually, this code is fine! But maybe `getFilteredTodos()` is slow or you have a lot of `todos`. In that case you don’t want to recalculate `getFilteredTodos()` if some unrelated state variable like `newTodo` has changed.
You can cache (or [“memoize”](https://en.wikipedia.org/wiki/Memoization)) an expensive calculation by wrapping it in a [`useMemo`](https://react.dev/reference/react/useMemo) Hook:
```
import { useMemo, useState } from 'react';
function TodoList({ todos, filter }) {
const [newTodo, setNewTodo] = useState('');
const visibleTodos = useMemo(() => {
// ✅ Does not re-run unless todos or filter change
return getFilteredTodos(todos, filter);
}, [todos, filter]);
// ...
}
```
Or, written as a single line:
```
import { useMemo, useState } from 'react';
function TodoList({ todos, filter }) {
const [newTodo, setNewTodo] = useState('');
// ✅ Does not re-run getFilteredTodos() unless todos or filter change
const visibleTodos = useMemo(() => getFilteredTodos(todos, filter), [todos, filter]);
// ...
}
```
**This tells React that you don’t want the inner function to re-run unless either `todos` or `filter` have changed.** React will remember the return value of `getFilteredTodos()` during the initial render. During the next renders, it will check if `todos` or `filter` are different. If they’re the same as last time, `useMemo` will return the last result it has stored. But if they are different, React will call the inner function again (and store its result).
The function you wrap in [`useMemo`](https://react.dev/reference/react/useMemo) runs during rendering, so this only works for [pure calculations.](https://react.dev/learn/keeping-components-pure)
##### Deep Dive
#### How to tell if a calculation is expensive?[Link for How to tell if a calculation is expensive?]()
Show Details
In general, unless you’re creating or looping over thousands of objects, it’s probably not expensive. If you want to get more confidence, you can add a console log to measure the time spent in a piece of code:
```
console.time('filter array');
const visibleTodos = getFilteredTodos(todos, filter);
console.timeEnd('filter array');
```
Perform the interaction you’re measuring (for example, typing into the input). You will then see logs like `filter array: 0.15ms` in your console. If the overall logged time adds up to a significant amount (say, `1ms` or more), it might make sense to memoize that calculation. As an experiment, you can then wrap the calculation in `useMemo` to verify whether the total logged time has decreased for that interaction or not:
```
console.time('filter array');
const visibleTodos = useMemo(() => {
return getFilteredTodos(todos, filter); // Skipped if todos and filter haven't changed
}, [todos, filter]);
console.timeEnd('filter array');
```
`useMemo` won’t make the *first* render faster. It only helps you skip unnecessary work on updates.
Keep in mind that your machine is probably faster than your users’ so it’s a good idea to test the performance with an artificial slowdown. For example, Chrome offers a [CPU Throttling](https://developer.chrome.com/blog/new-in-devtools-61/) option for this.
Also note that measuring performance in development will not give you the most accurate results. (For example, when [Strict Mode](https://react.dev/reference/react/StrictMode) is on, you will see each component render twice rather than once.) To get the most accurate timings, build your app for production and test it on a device like your users have.
### Resetting all state when a prop changes[Link for Resetting all state when a prop changes]()
This `ProfilePage` component receives a `userId` prop. The page contains a comment input, and you use a `comment` state variable to hold its value. One day, you notice a problem: when you navigate from one profile to another, the `comment` state does not get reset. As a result, it’s easy to accidentally post a comment on a wrong user’s profile. To fix the issue, you want to clear out the `comment` state variable whenever the `userId` changes:
```
export default function ProfilePage({ userId }) {
const [comment, setComment] = useState('');
// 🔴 Avoid: Resetting state on prop change in an Effect
useEffect(() => {
setComment('');
}, [userId]);
// ...
}
```
This is inefficient because `ProfilePage` and its children will first render with the stale value, and then render again. It is also complicated because you’d need to do this in *every* component that has some state inside `ProfilePage`. For example, if the comment UI is nested, you’d want to clear out nested comment state too.
Instead, you can tell React that each user’s profile is conceptually a *different* profile by giving it an explicit key. Split your component in two and pass a `key` attribute from the outer component to the inner one:
```
export default function ProfilePage({ userId }) {
return (
<Profile
userId={userId}
key={userId}
/>
);
}
function Profile({ userId }) {
// ✅ This and any other state below will reset on key change automatically
const [comment, setComment] = useState('');
// ...
}
```
Normally, React preserves the state when the same component is rendered in the same spot. **By passing `userId` as a `key` to the `Profile` component, you’re asking React to treat two `Profile` components with different `userId` as two different components that should not share any state.** Whenever the key (which you’ve set to `userId`) changes, React will recreate the DOM and [reset the state](https://react.dev/learn/preserving-and-resetting-state) of the `Profile` component and all of its children. Now the `comment` field will clear out automatically when navigating between profiles.
Note that in this example, only the outer `ProfilePage` component is exported and visible to other files in the project. Components rendering `ProfilePage` don’t need to pass the key to it: they pass `userId` as a regular prop. The fact `ProfilePage` passes it as a `key` to the inner `Profile` component is an implementation detail.
### Adjusting some state when a prop changes[Link for Adjusting some state when a prop changes]()
Sometimes, you might want to reset or adjust a part of the state on a prop change, but not all of it.
This `List` component receives a list of `items` as a prop, and maintains the selected item in the `selection` state variable. You want to reset the `selection` to `null` whenever the `items` prop receives a different array:
```
function List({ items }) {
const [isReverse, setIsReverse] = useState(false);
const [selection, setSelection] = useState(null);
// 🔴 Avoid: Adjusting state on prop change in an Effect
useEffect(() => {
setSelection(null);
}, [items]);
// ...
}
```
This, too, is not ideal. Every time the `items` change, the `List` and its child components will render with a stale `selection` value at first. Then React will update the DOM and run the Effects. Finally, the `setSelection(null)` call will cause another re-render of the `List` and its child components, restarting this whole process again.
Start by deleting the Effect. Instead, adjust the state directly during rendering:
```
function List({ items }) {
const [isReverse, setIsReverse] = useState(false);
const [selection, setSelection] = useState(null);
// Better: Adjust the state while rendering
const [prevItems, setPrevItems] = useState(items);
if (items !== prevItems) {
setPrevItems(items);
setSelection(null);
}
// ...
}
```
[Storing information from previous renders](https://react.dev/reference/react/useState) like this can be hard to understand, but it’s better than updating the same state in an Effect. In the above example, `setSelection` is called directly during a render. React will re-render the `List` *immediately* after it exits with a `return` statement. React has not rendered the `List` children or updated the DOM yet, so this lets the `List` children skip rendering the stale `selection` value.
When you update a component during rendering, React throws away the returned JSX and immediately retries rendering. To avoid very slow cascading retries, React only lets you update the *same* component’s state during a render. If you update another component’s state during a render, you’ll see an error. A condition like `items !== prevItems` is necessary to avoid loops. You may adjust state like this, but any other side effects (like changing the DOM or setting timeouts) should stay in event handlers or Effects to [keep components pure.](https://react.dev/learn/keeping-components-pure)
**Although this pattern is more efficient than an Effect, most components shouldn’t need it either.** No matter how you do it, adjusting state based on props or other state makes your data flow more difficult to understand and debug. Always check whether you can [reset all state with a key]() or [calculate everything during rendering]() instead. For example, instead of storing (and resetting) the selected *item*, you can store the selected *item ID:*
```
function List({ items }) {
const [isReverse, setIsReverse] = useState(false);
const [selectedId, setSelectedId] = useState(null);
// ✅ Best: Calculate everything during rendering
const selection = items.find(item => item.id === selectedId) ?? null;
// ...
}
```
Now there is no need to “adjust” the state at all. If the item with the selected ID is in the list, it remains selected. If it’s not, the `selection` calculated during rendering will be `null` because no matching item was found. This behavior is different, but arguably better because most changes to `items` preserve the selection.
### Sharing logic between event handlers[Link for Sharing logic between event handlers]()
Let’s say you have a product page with two buttons (Buy and Checkout) that both let you buy that product. You want to show a notification whenever the user puts the product in the cart. Calling `showNotification()` in both buttons’ click handlers feels repetitive so you might be tempted to place this logic in an Effect:
```
function ProductPage({ product, addToCart }) {
// 🔴 Avoid: Event-specific logic inside an Effect
useEffect(() => {
if (product.isInCart) {
showNotification(`Added ${product.name} to the shopping cart!`);
}
}, [product]);
function handleBuyClick() {
addToCart(product);
}
function handleCheckoutClick() {
addToCart(product);
navigateTo('/checkout');
}
// ...
}
```
This Effect is unnecessary. It will also most likely cause bugs. For example, let’s say that your app “remembers” the shopping cart between the page reloads. If you add a product to the cart once and refresh the page, the notification will appear again. It will keep appearing every time you refresh that product’s page. This is because `product.isInCart` will already be `true` on the page load, so the Effect above will call `showNotification()`.
**When you’re not sure whether some code should be in an Effect or in an event handler, ask yourself *why* this code needs to run. Use Effects only for code that should run *because* the component was displayed to the user.** In this example, the notification should appear because the user *pressed the button*, not because the page was displayed! Delete the Effect and put the shared logic into a function called from both event handlers:
```
function ProductPage({ product, addToCart }) {
// ✅ Good: Event-specific logic is called from event handlers
function buyProduct() {
addToCart(product);
showNotification(`Added ${product.name} to the shopping cart!`);
}
function handleBuyClick() {
buyProduct();
}
function handleCheckoutClick() {
buyProduct();
navigateTo('/checkout');
}
// ...
}
```
This both removes the unnecessary Effect and fixes the bug.
### Sending a POST request[Link for Sending a POST request]()
This `Form` component sends two kinds of POST requests. It sends an analytics event when it mounts. When you fill in the form and click the Submit button, it will send a POST request to the `/api/register` endpoint:
```
function Form() {
const [firstName, setFirstName] = useState('');
const [lastName, setLastName] = useState('');
// ✅ Good: This logic should run because the component was displayed
useEffect(() => {
post('/analytics/event', { eventName: 'visit_form' });
}, []);
// 🔴 Avoid: Event-specific logic inside an Effect
const [jsonToSubmit, setJsonToSubmit] = useState(null);
useEffect(() => {
if (jsonToSubmit !== null) {
post('/api/register', jsonToSubmit);
}
}, [jsonToSubmit]);
function handleSubmit(e) {
e.preventDefault();
setJsonToSubmit({ firstName, lastName });
}
// ...
}
```
Let’s apply the same criteria as in the example before.
The analytics POST request should remain in an Effect. This is because the *reason* to send the analytics event is that the form was displayed. (It would fire twice in development, but [see here](https://react.dev/learn/synchronizing-with-effects) for how to deal with that.)
However, the `/api/register` POST request is not caused by the form being *displayed*. You only want to send the request at one specific moment in time: when the user presses the button. It should only ever happen *on that particular interaction*. Delete the second Effect and move that POST request into the event handler:
```
function Form() {
const [firstName, setFirstName] = useState('');
const [lastName, setLastName] = useState('');
// ✅ Good: This logic runs because the component was displayed
useEffect(() => {
post('/analytics/event', { eventName: 'visit_form' });
}, []);
function handleSubmit(e) {
e.preventDefault();
// ✅ Good: Event-specific logic is in the event handler
post('/api/register', { firstName, lastName });
}
// ...
}
```
When you choose whether to put some logic into an event handler or an Effect, the main question you need to answer is *what kind of logic* it is from the user’s perspective. If this logic is caused by a particular interaction, keep it in the event handler. If it’s caused by the user *seeing* the component on the screen, keep it in the Effect.
### Chains of computations[Link for Chains of computations]()
Sometimes you might feel tempted to chain Effects that each adjust a piece of state based on other state:
```
function Game() {
const [card, setCard] = useState(null);
const [goldCardCount, setGoldCardCount] = useState(0);
const [round, setRound] = useState(1);
const [isGameOver, setIsGameOver] = useState(false);
// 🔴 Avoid: Chains of Effects that adjust the state solely to trigger each other
useEffect(() => {
if (card !== null && card.gold) {
setGoldCardCount(c => c + 1);
}
}, [card]);
useEffect(() => {
if (goldCardCount > 3) {
setRound(r => r + 1)
setGoldCardCount(0);
}
}, [goldCardCount]);
useEffect(() => {
if (round > 5) {
setIsGameOver(true);
}
}, [round]);
useEffect(() => {
alert('Good game!');
}, [isGameOver]);
function handlePlaceCard(nextCard) {
if (isGameOver) {
throw Error('Game already ended.');
} else {
setCard(nextCard);
}
}
// ...
```
There are two problems with this code.
The first problem is that it is very inefficient: the component (and its children) have to re-render between each `set` call in the chain. In the example above, in the worst case (`setCard` → render → `setGoldCardCount` → render → `setRound` → render → `setIsGameOver` → render) there are three unnecessary re-renders of the tree below.
The second problem is that even if it weren’t slow, as your code evolves, you will run into cases where the “chain” you wrote doesn’t fit the new requirements. Imagine you are adding a way to step through the history of the game moves. You’d do it by updating each state variable to a value from the past. However, setting the `card` state to a value from the past would trigger the Effect chain again and change the data you’re showing. Such code is often rigid and fragile.
In this case, it’s better to calculate what you can during rendering, and adjust the state in the event handler:
```
function Game() {
const [card, setCard] = useState(null);
const [goldCardCount, setGoldCardCount] = useState(0);
const [round, setRound] = useState(1);
// ✅ Calculate what you can during rendering
const isGameOver = round > 5;
function handlePlaceCard(nextCard) {
if (isGameOver) {
throw Error('Game already ended.');
}
// ✅ Calculate all the next state in the event handler
setCard(nextCard);
if (nextCard.gold) {
if (goldCardCount <= 3) {
setGoldCardCount(goldCardCount + 1);
} else {
setGoldCardCount(0);
setRound(round + 1);
if (round === 5) {
alert('Good game!');
}
}
}
}
// ...
```
This is a lot more efficient. Also, if you implement a way to view game history, now you will be able to set each state variable to a move from the past without triggering the Effect chain that adjusts every other value. If you need to reuse logic between several event handlers, you can [extract a function]() and call it from those handlers.
Remember that inside event handlers, [state behaves like a snapshot.](https://react.dev/learn/state-as-a-snapshot) For example, even after you call `setRound(round + 1)`, the `round` variable will reflect the value at the time the user clicked the button. If you need to use the next value for calculations, define it manually like `const nextRound = round + 1`.
In some cases, you *can’t* calculate the next state directly in the event handler. For example, imagine a form with multiple dropdowns where the options of the next dropdown depend on the selected value of the previous dropdown. Then, a chain of Effects is appropriate because you are synchronizing with network.
### Initializing the application[Link for Initializing the application]()
Some logic should only run once when the app loads.
You might be tempted to place it in an Effect in the top-level component:
```
function App() {
// 🔴 Avoid: Effects with logic that should only ever run once
useEffect(() => {
loadDataFromLocalStorage();
checkAuthToken();
}, []);
// ...
}
```
However, you’ll quickly discover that it [runs twice in development.](https://react.dev/learn/synchronizing-with-effects) This can cause issues—for example, maybe it invalidates the authentication token because the function wasn’t designed to be called twice. In general, your components should be resilient to being remounted. This includes your top-level `App` component.
Although it may not ever get remounted in practice in production, following the same constraints in all components makes it easier to move and reuse code. If some logic must run *once per app load* rather than *once per component mount*, add a top-level variable to track whether it has already executed:
```
let didInit = false;
function App() {
useEffect(() => {
if (!didInit) {
didInit = true;
// ✅ Only runs once per app load
loadDataFromLocalStorage();
checkAuthToken();
}
}, []);
// ...
}
```
You can also run it during module initialization and before the app renders:
```
if (typeof window !== 'undefined') { // Check if we're running in the browser.
// ✅ Only runs once per app load
checkAuthToken();
loadDataFromLocalStorage();
}
function App() {
// ...
}
```
Code at the top level runs once when your component is imported—even if it doesn’t end up being rendered. To avoid slowdown or surprising behavior when importing arbitrary components, don’t overuse this pattern. Keep app-wide initialization logic to root component modules like `App.js` or in your application’s entry point.
### Notifying parent components about state changes[Link for Notifying parent components about state changes]()
Let’s say you’re writing a `Toggle` component with an internal `isOn` state which can be either `true` or `false`. There are a few different ways to toggle it (by clicking or dragging). You want to notify the parent component whenever the `Toggle` internal state changes, so you expose an `onChange` event and call it from an Effect:
```
function Toggle({ onChange }) {
const [isOn, setIsOn] = useState(false);
// 🔴 Avoid: The onChange handler runs too late
useEffect(() => {
onChange(isOn);
}, [isOn, onChange])
function handleClick() {
setIsOn(!isOn);
}
function handleDragEnd(e) {
if (isCloserToRightEdge(e)) {
setIsOn(true);
} else {
setIsOn(false);
}
}
// ...
}
```
Like earlier, this is not ideal. The `Toggle` updates its state first, and React updates the screen. Then React runs the Effect, which calls the `onChange` function passed from a parent component. Now the parent component will update its own state, starting another render pass. It would be better to do everything in a single pass.
Delete the Effect and instead update the state of *both* components within the same event handler:
```
function Toggle({ onChange }) {
const [isOn, setIsOn] = useState(false);
function updateToggle(nextIsOn) {
// ✅ Good: Perform all updates during the event that caused them
setIsOn(nextIsOn);
onChange(nextIsOn);
}
function handleClick() {
updateToggle(!isOn);
}
function handleDragEnd(e) {
if (isCloserToRightEdge(e)) {
updateToggle(true);
} else {
updateToggle(false);
}
}
// ...
}
```
With this approach, both the `Toggle` component and its parent component update their state during the event. React [batches updates](https://react.dev/learn/queueing-a-series-of-state-updates) from different components together, so there will only be one render pass.
You might also be able to remove the state altogether, and instead receive `isOn` from the parent component:
```
// ✅ Also good: the component is fully controlled by its parent
function Toggle({ isOn, onChange }) {
function handleClick() {
onChange(!isOn);
}
function handleDragEnd(e) {
if (isCloserToRightEdge(e)) {
onChange(true);
} else {
onChange(false);
}
}
// ...
}
```
[“Lifting state up”](https://react.dev/learn/sharing-state-between-components) lets the parent component fully control the `Toggle` by toggling the parent’s own state. This means the parent component will have to contain more logic, but there will be less state overall to worry about. Whenever you try to keep two different state variables synchronized, try lifting state up instead!
### Passing data to the parent[Link for Passing data to the parent]()
This `Child` component fetches some data and then passes it to the `Parent` component in an Effect:
```
function Parent() {
const [data, setData] = useState(null);
// ...
return <Child onFetched={setData} />;
}
function Child({ onFetched }) {
const data = useSomeAPI();
// 🔴 Avoid: Passing data to the parent in an Effect
useEffect(() => {
if (data) {
onFetched(data);
}
}, [onFetched, data]);
// ...
}
```
In React, data flows from the parent components to their children. When you see something wrong on the screen, you can trace where the information comes from by going up the component chain until you find which component passes the wrong prop or has the wrong state. When child components update the state of their parent components in Effects, the data flow becomes very difficult to trace. Since both the child and the parent need the same data, let the parent component fetch that data, and *pass it down* to the child instead:
```
function Parent() {
const data = useSomeAPI();
// ...
// ✅ Good: Passing data down to the child
return <Child data={data} />;
}
function Child({ data }) {
// ...
}
```
This is simpler and keeps the data flow predictable: the data flows down from the parent to the child.
### Subscribing to an external store[Link for Subscribing to an external store]()
Sometimes, your components may need to subscribe to some data outside of the React state. This data could be from a third-party library or a built-in browser API. Since this data can change without React’s knowledge, you need to manually subscribe your components to it. This is often done with an Effect, for example:
```
function useOnlineStatus() {
// Not ideal: Manual store subscription in an Effect
const [isOnline, setIsOnline] = useState(true);
useEffect(() => {
function updateState() {
setIsOnline(navigator.onLine);
}
updateState();
window.addEventListener('online', updateState);
window.addEventListener('offline', updateState);
return () => {
window.removeEventListener('online', updateState);
window.removeEventListener('offline', updateState);
};
}, []);
return isOnline;
}
function ChatIndicator() {
const isOnline = useOnlineStatus();
// ...
}
```
Here, the component subscribes to an external data store (in this case, the browser `navigator.onLine` API). Since this API does not exist on the server (so it can’t be used for the initial HTML), initially the state is set to `true`. Whenever the value of that data store changes in the browser, the component updates its state.
Although it’s common to use Effects for this, React has a purpose-built Hook for subscribing to an external store that is preferred instead. Delete the Effect and replace it with a call to [`useSyncExternalStore`](https://react.dev/reference/react/useSyncExternalStore):
```
function subscribe(callback) {
window.addEventListener('online', callback);
window.addEventListener('offline', callback);
return () => {
window.removeEventListener('online', callback);
window.removeEventListener('offline', callback);
};
}
function useOnlineStatus() {
// ✅ Good: Subscribing to an external store with a built-in Hook
return useSyncExternalStore(
subscribe, // React won't resubscribe for as long as you pass the same function
() => navigator.onLine, // How to get the value on the client
() => true // How to get the value on the server
);
}
function ChatIndicator() {
const isOnline = useOnlineStatus();
// ...
}
```
This approach is less error-prone than manually syncing mutable data to React state with an Effect. Typically, you’ll write a custom Hook like `useOnlineStatus()` above so that you don’t need to repeat this code in the individual components. [Read more about subscribing to external stores from React components.](https://react.dev/reference/react/useSyncExternalStore)
### Fetching data[Link for Fetching data]()
Many apps use Effects to kick off data fetching. It is quite common to write a data fetching Effect like this:
```
function SearchResults({ query }) {
const [results, setResults] = useState([]);
const [page, setPage] = useState(1);
useEffect(() => {
// 🔴 Avoid: Fetching without cleanup logic
fetchResults(query, page).then(json => {
setResults(json);
});
}, [query, page]);
function handleNextPageClick() {
setPage(page + 1);
}
// ...
}
```
You *don’t* need to move this fetch to an event handler.
This might seem like a contradiction with the earlier examples where you needed to put the logic into the event handlers! However, consider that it’s not *the typing event* that’s the main reason to fetch. Search inputs are often prepopulated from the URL, and the user might navigate Back and Forward without touching the input.
It doesn’t matter where `page` and `query` come from. While this component is visible, you want to keep `results` [synchronized](https://react.dev/learn/synchronizing-with-effects) with data from the network for the current `page` and `query`. This is why it’s an Effect.
However, the code above has a bug. Imagine you type `"hello"` fast. Then the `query` will change from `"h"`, to `"he"`, `"hel"`, `"hell"`, and `"hello"`. This will kick off separate fetches, but there is no guarantee about which order the responses will arrive in. For example, the `"hell"` response may arrive *after* the `"hello"` response. Since it will call `setResults()` last, you will be displaying the wrong search results. This is called a [“race condition”](https://en.wikipedia.org/wiki/Race_condition): two different requests “raced” against each other and came in a different order than you expected.
**To fix the race condition, you need to [add a cleanup function](https://react.dev/learn/synchronizing-with-effects) to ignore stale responses:**
```
function SearchResults({ query }) {
const [results, setResults] = useState([]);
const [page, setPage] = useState(1);
useEffect(() => {
let ignore = false;
fetchResults(query, page).then(json => {
if (!ignore) {
setResults(json);
}
});
return () => {
ignore = true;
};
}, [query, page]);
function handleNextPageClick() {
setPage(page + 1);
}
// ...
}
```
This ensures that when your Effect fetches data, all responses except the last requested one will be ignored.
Handling race conditions is not the only difficulty with implementing data fetching. You might also want to think about caching responses (so that the user can click Back and see the previous screen instantly), how to fetch data on the server (so that the initial server-rendered HTML contains the fetched content instead of a spinner), and how to avoid network waterfalls (so that a child can fetch data without waiting for every parent).
**These issues apply to any UI library, not just React. Solving them is not trivial, which is why modern [frameworks](https://react.dev/learn/start-a-new-react-project) provide more efficient built-in data fetching mechanisms than fetching data in Effects.**
If you don’t use a framework (and don’t want to build your own) but would like to make data fetching from Effects more ergonomic, consider extracting your fetching logic into a custom Hook like in this example:
```
function SearchResults({ query }) {
const [page, setPage] = useState(1);
const params = new URLSearchParams({ query, page });
const results = useData(`/api/search?${params}`);
function handleNextPageClick() {
setPage(page + 1);
}
// ...
}
function useData(url) {
const [data, setData] = useState(null);
useEffect(() => {
let ignore = false;
fetch(url)
.then(response => response.json())
.then(json => {
if (!ignore) {
setData(json);
}
});
return () => {
ignore = true;
};
}, [url]);
return data;
}
```
You’ll likely also want to add some logic for error handling and to track whether the content is loading. You can build a Hook like this yourself or use one of the many solutions already available in the React ecosystem. **Although this alone won’t be as efficient as using a framework’s built-in data fetching mechanism, moving the data fetching logic into a custom Hook will make it easier to adopt an efficient data fetching strategy later.**
In general, whenever you have to resort to writing Effects, keep an eye out for when you can extract a piece of functionality into a custom Hook with a more declarative and purpose-built API like `useData` above. The fewer raw `useEffect` calls you have in your components, the easier you will find to maintain your application.
## Recap[Link for Recap]()
- If you can calculate something during render, you don’t need an Effect.
- To cache expensive calculations, add `useMemo` instead of `useEffect`.
- To reset the state of an entire component tree, pass a different `key` to it.
- To reset a particular bit of state in response to a prop change, set it during rendering.
- Code that runs because a component was *displayed* should be in Effects, the rest should be in events.
- If you need to update the state of several components, it’s better to do it during a single event.
- Whenever you try to synchronize state variables in different components, consider lifting state up.
- You can fetch data with Effects, but you need to implement cleanup to avoid race conditions.
## Try out some challenges[Link for Try out some challenges]()
1\. Transform data without Effects 2. Cache a calculation without Effects 3. Reset state without Effects 4. Submit a form without Effects
#### Challenge 1 of 4: Transform data without Effects[Link for this heading]()
The `TodoList` below displays a list of todos. When the “Show only active todos” checkbox is ticked, completed todos are not displayed in the list. Regardless of which todos are visible, the footer displays the count of todos that are not yet completed.
Simplify this component by removing all the unnecessary state and Effects.
App.jstodos.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import { useState, useEffect } from 'react';
import { initialTodos, createTodo } from './todos.js';
export default function TodoList() {
const [todos, setTodos] = useState(initialTodos);
const [showActive, setShowActive] = useState(false);
const [activeTodos, setActiveTodos] = useState([]);
const [visibleTodos, setVisibleTodos] = useState([]);
const [footer, setFooter] = useState(null);
useEffect(() => {
setActiveTodos(todos.filter(todo => !todo.completed));
}, [todos]);
useEffect(() => {
setVisibleTodos(showActive ? activeTodos : todos);
}, [showActive, todos, activeTodos]);
useEffect(() => {
setFooter(
<footer>
{activeTodos.length} todos left
</footer>
);
}, [activeTodos]);
return (
<>
<label>
<input
type="checkbox"
checked={showActive}
onChange={e => setShowActive(e.target.checked)}
/>
Show only active todos
</label>
<NewTodo onAdd={newTodo => setTodos([...todos, newTodo])} />
<ul>
{visibleTodos.map(todo => (
<li key={todo.id}>
{todo.completed ? <s>{todo.text}</s> : todo.text}
</li>
))}
</ul>
{footer}
</>
);
}
function NewTodo({ onAdd }) {
const [text, setText] = useState('');
function handleAddClick() {
setText('');
onAdd(createTodo(text));
}
return (
<>
<input value={text} onChange={e => setText(e.target.value)} />
<button onClick={handleAddClick}>
Add
</button>
</>
);
}
```
Show more
Show hint Show solution
Next Challenge
[PreviousSynchronizing with Effects](https://react.dev/learn/synchronizing-with-effects)
[NextLifecycle of Reactive Effects](https://react.dev/learn/lifecycle-of-reactive-effects) |
https://react.dev/learn/removing-effect-dependencies | [Learn React](https://react.dev/learn)
[Escape Hatches](https://react.dev/learn/escape-hatches)
# Removing Effect Dependencies[Link for this heading]()
When you write an Effect, the linter will verify that you’ve included every reactive value (like props and state) that the Effect reads in the list of your Effect’s dependencies. This ensures that your Effect remains synchronized with the latest props and state of your component. Unnecessary dependencies may cause your Effect to run too often, or even create an infinite loop. Follow this guide to review and remove unnecessary dependencies from your Effects.
### You will learn
- How to fix infinite Effect dependency loops
- What to do when you want to remove a dependency
- How to read a value from your Effect without “reacting” to it
- How and why to avoid object and function dependencies
- Why suppressing the dependency linter is dangerous, and what to do instead
## Dependencies should match the code[Link for Dependencies should match the code]()
When you write an Effect, you first specify how to [start and stop](https://react.dev/learn/lifecycle-of-reactive-effects) whatever you want your Effect to be doing:
```
const serverUrl = 'https://localhost:1234';
function ChatRoom({ roomId }) {
useEffect(() => {
const connection = createConnection(serverUrl, roomId);
connection.connect();
return () => connection.disconnect();
// ...
}
```
Then, if you leave the Effect dependencies empty (`[]`), the linter will suggest the correct dependencies:
App.jschat.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import { useState, useEffect } from 'react';
import { createConnection } from './chat.js';
const serverUrl = 'https://localhost:1234';
function ChatRoom({ roomId }) {
useEffect(() => {
const connection = createConnection(serverUrl, roomId);
connection.connect();
return () => connection.disconnect();
}, []); // <-- Fix the mistake here!
return <h1>Welcome to the {roomId} room!</h1>;
}
export default function App() {
const [roomId, setRoomId] = useState('general');
return (
<>
<label>
Choose the chat room:{' '}
<select
value={roomId}
onChange={e => setRoomId(e.target.value)}
>
<option value="general">general</option>
<option value="travel">travel</option>
<option value="music">music</option>
</select>
</label>
<hr />
<ChatRoom roomId={roomId} />
</>
);
}
```
Show more
Fill them in according to what the linter says:
```
function ChatRoom({ roomId }) {
useEffect(() => {
const connection = createConnection(serverUrl, roomId);
connection.connect();
return () => connection.disconnect();
}, [roomId]); // ✅ All dependencies declared
// ...
}
```
[Effects “react” to reactive values.](https://react.dev/learn/lifecycle-of-reactive-effects) Since `roomId` is a reactive value (it can change due to a re-render), the linter verifies that you’ve specified it as a dependency. If `roomId` receives a different value, React will re-synchronize your Effect. This ensures that the chat stays connected to the selected room and “reacts” to the dropdown:
App.jschat.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import { useState, useEffect } from 'react';
import { createConnection } from './chat.js';
const serverUrl = 'https://localhost:1234';
function ChatRoom({ roomId }) {
useEffect(() => {
const connection = createConnection(serverUrl, roomId);
connection.connect();
return () => connection.disconnect();
}, [roomId]);
return <h1>Welcome to the {roomId} room!</h1>;
}
export default function App() {
const [roomId, setRoomId] = useState('general');
return (
<>
<label>
Choose the chat room:{' '}
<select
value={roomId}
onChange={e => setRoomId(e.target.value)}
>
<option value="general">general</option>
<option value="travel">travel</option>
<option value="music">music</option>
</select>
</label>
<hr />
<ChatRoom roomId={roomId} />
</>
);
}
```
Show more
### To remove a dependency, prove that it’s not a dependency[Link for To remove a dependency, prove that it’s not a dependency]()
Notice that you can’t “choose” the dependencies of your Effect. Every reactive value used by your Effect’s code must be declared in your dependency list. The dependency list is determined by the surrounding code:
```
const serverUrl = 'https://localhost:1234';
function ChatRoom({ roomId }) { // This is a reactive value
useEffect(() => {
const connection = createConnection(serverUrl, roomId); // This Effect reads that reactive value
connection.connect();
return () => connection.disconnect();
}, [roomId]); // ✅ So you must specify that reactive value as a dependency of your Effect
// ...
}
```
[Reactive values](https://react.dev/learn/lifecycle-of-reactive-effects) include props and all variables and functions declared directly inside of your component. Since `roomId` is a reactive value, you can’t remove it from the dependency list. The linter wouldn’t allow it:
```
const serverUrl = 'https://localhost:1234';
function ChatRoom({ roomId }) {
useEffect(() => {
const connection = createConnection(serverUrl, roomId);
connection.connect();
return () => connection.disconnect();
}, []); // 🔴 React Hook useEffect has a missing dependency: 'roomId'
// ...
}
```
And the linter would be right! Since `roomId` may change over time, this would introduce a bug in your code.
**To remove a dependency, “prove” to the linter that it *doesn’t need* to be a dependency.** For example, you can move `roomId` out of your component to prove that it’s not reactive and won’t change on re-renders:
```
const serverUrl = 'https://localhost:1234';
const roomId = 'music'; // Not a reactive value anymore
function ChatRoom() {
useEffect(() => {
const connection = createConnection(serverUrl, roomId);
connection.connect();
return () => connection.disconnect();
}, []); // ✅ All dependencies declared
// ...
}
```
Now that `roomId` is not a reactive value (and can’t change on a re-render), it doesn’t need to be a dependency:
App.jschat.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import { useState, useEffect } from 'react';
import { createConnection } from './chat.js';
const serverUrl = 'https://localhost:1234';
const roomId = 'music';
export default function ChatRoom() {
useEffect(() => {
const connection = createConnection(serverUrl, roomId);
connection.connect();
return () => connection.disconnect();
}, []);
return <h1>Welcome to the {roomId} room!</h1>;
}
```
This is why you could now specify an [empty (`[]`) dependency list.](https://react.dev/learn/lifecycle-of-reactive-effects) Your Effect *really doesn’t* depend on any reactive value anymore, so it *really doesn’t* need to re-run when any of the component’s props or state change.
### To change the dependencies, change the code[Link for To change the dependencies, change the code]()
You might have noticed a pattern in your workflow:
1. First, you **change the code** of your Effect or how your reactive values are declared.
2. Then, you follow the linter and adjust the dependencies to **match the code you have changed.**
3. If you’re not happy with the list of dependencies, you **go back to the first step** (and change the code again).
The last part is important. **If you want to change the dependencies, change the surrounding code first.** You can think of the dependency list as [a list of all the reactive values used by your Effect’s code.](https://react.dev/learn/lifecycle-of-reactive-effects) You don’t *choose* what to put on that list. The list *describes* your code. To change the dependency list, change the code.
This might feel like solving an equation. You might start with a goal (for example, to remove a dependency), and you need to “find” the code matching that goal. Not everyone finds solving equations fun, and the same thing could be said about writing Effects! Luckily, there is a list of common recipes that you can try below.
### Pitfall
If you have an existing codebase, you might have some Effects that suppress the linter like this:
```
useEffect(() => {
// ...
// 🔴 Avoid suppressing the linter like this:
// eslint-ignore-next-line react-hooks/exhaustive-deps
}, []);
```
**When dependencies don’t match the code, there is a very high risk of introducing bugs.** By suppressing the linter, you “lie” to React about the values your Effect depends on.
Instead, use the techniques below.
##### Deep Dive
#### Why is suppressing the dependency linter so dangerous?[Link for Why is suppressing the dependency linter so dangerous?]()
Show Details
Suppressing the linter leads to very unintuitive bugs that are hard to find and fix. Here’s one example:
App.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import { useState, useEffect } from 'react';
export default function Timer() {
const [count, setCount] = useState(0);
const [increment, setIncrement] = useState(1);
function onTick() {
setCount(count + increment);
}
useEffect(() => {
const id = setInterval(onTick, 1000);
return () => clearInterval(id);
// eslint-disable-next-line react-hooks/exhaustive-deps
}, []);
return (
<>
<h1>
Counter: {count}
<button onClick={() => setCount(0)}>Reset</button>
</h1>
<hr />
<p>
Every second, increment by:
<button disabled={increment === 0} onClick={() => {
setIncrement(i => i - 1);
}}>–</button>
<b>{increment}</b>
<button onClick={() => {
setIncrement(i => i + 1);
}}>+</button>
</p>
</>
);
}
```
Show more
Let’s say that you wanted to run the Effect “only on mount”. You’ve read that [empty (`[]`) dependencies](https://react.dev/learn/lifecycle-of-reactive-effects) do that, so you’ve decided to ignore the linter, and forcefully specified `[]` as the dependencies.
This counter was supposed to increment every second by the amount configurable with the two buttons. However, since you “lied” to React that this Effect doesn’t depend on anything, React forever keeps using the `onTick` function from the initial render. [During that render,](https://react.dev/learn/state-as-a-snapshot) `count` was `0` and `increment` was `1`. This is why `onTick` from that render always calls `setCount(0 + 1)` every second, and you always see `1`. Bugs like this are harder to fix when they’re spread across multiple components.
There’s always a better solution than ignoring the linter! To fix this code, you need to add `onTick` to the dependency list. (To ensure the interval is only setup once, [make `onTick` an Effect Event.](https://react.dev/learn/separating-events-from-effects))
**We recommend treating the dependency lint error as a compilation error. If you don’t suppress it, you will never see bugs like this.** The rest of this page documents the alternatives for this and other cases.
## Removing unnecessary dependencies[Link for Removing unnecessary dependencies]()
Every time you adjust the Effect’s dependencies to reflect the code, look at the dependency list. Does it make sense for the Effect to re-run when any of these dependencies change? Sometimes, the answer is “no”:
- You might want to re-execute *different parts* of your Effect under different conditions.
- You might want to only read the *latest value* of some dependency instead of “reacting” to its changes.
- A dependency may change too often *unintentionally* because it’s an object or a function.
To find the right solution, you’ll need to answer a few questions about your Effect. Let’s walk through them.
### Should this code move to an event handler?[Link for Should this code move to an event handler?]()
The first thing you should think about is whether this code should be an Effect at all.
Imagine a form. On submit, you set the `submitted` state variable to `true`. You need to send a POST request and show a notification. You’ve put this logic inside an Effect that “reacts” to `submitted` being `true`:
```
function Form() {
const [submitted, setSubmitted] = useState(false);
useEffect(() => {
if (submitted) {
// 🔴 Avoid: Event-specific logic inside an Effect
post('/api/register');
showNotification('Successfully registered!');
}
}, [submitted]);
function handleSubmit() {
setSubmitted(true);
}
// ...
}
```
Later, you want to style the notification message according to the current theme, so you read the current theme. Since `theme` is declared in the component body, it is a reactive value, so you add it as a dependency:
```
function Form() {
const [submitted, setSubmitted] = useState(false);
const theme = useContext(ThemeContext);
useEffect(() => {
if (submitted) {
// 🔴 Avoid: Event-specific logic inside an Effect
post('/api/register');
showNotification('Successfully registered!', theme);
}
}, [submitted, theme]); // ✅ All dependencies declared
function handleSubmit() {
setSubmitted(true);
}
// ...
}
```
By doing this, you’ve introduced a bug. Imagine you submit the form first and then switch between Dark and Light themes. The `theme` will change, the Effect will re-run, and so it will display the same notification again!
**The problem here is that this shouldn’t be an Effect in the first place.** You want to send this POST request and show the notification in response to *submitting the form,* which is a particular interaction. To run some code in response to particular interaction, put that logic directly into the corresponding event handler:
```
function Form() {
const theme = useContext(ThemeContext);
function handleSubmit() {
// ✅ Good: Event-specific logic is called from event handlers
post('/api/register');
showNotification('Successfully registered!', theme);
}
// ...
}
```
Now that the code is in an event handler, it’s not reactive—so it will only run when the user submits the form. Read more about [choosing between event handlers and Effects](https://react.dev/learn/separating-events-from-effects) and [how to delete unnecessary Effects.](https://react.dev/learn/you-might-not-need-an-effect)
### Is your Effect doing several unrelated things?[Link for Is your Effect doing several unrelated things?]()
The next question you should ask yourself is whether your Effect is doing several unrelated things.
Imagine you’re creating a shipping form where the user needs to choose their city and area. You fetch the list of `cities` from the server according to the selected `country` to show them in a dropdown:
```
function ShippingForm({ country }) {
const [cities, setCities] = useState(null);
const [city, setCity] = useState(null);
useEffect(() => {
let ignore = false;
fetch(`/api/cities?country=${country}`)
.then(response => response.json())
.then(json => {
if (!ignore) {
setCities(json);
}
});
return () => {
ignore = true;
};
}, [country]); // ✅ All dependencies declared
// ...
```
This is a good example of [fetching data in an Effect.](https://react.dev/learn/you-might-not-need-an-effect) You are synchronizing the `cities` state with the network according to the `country` prop. You can’t do this in an event handler because you need to fetch as soon as `ShippingForm` is displayed and whenever the `country` changes (no matter which interaction causes it).
Now let’s say you’re adding a second select box for city areas, which should fetch the `areas` for the currently selected `city`. You might start by adding a second `fetch` call for the list of areas inside the same Effect:
```
function ShippingForm({ country }) {
const [cities, setCities] = useState(null);
const [city, setCity] = useState(null);
const [areas, setAreas] = useState(null);
useEffect(() => {
let ignore = false;
fetch(`/api/cities?country=${country}`)
.then(response => response.json())
.then(json => {
if (!ignore) {
setCities(json);
}
});
// 🔴 Avoid: A single Effect synchronizes two independent processes
if (city) {
fetch(`/api/areas?city=${city}`)
.then(response => response.json())
.then(json => {
if (!ignore) {
setAreas(json);
}
});
}
return () => {
ignore = true;
};
}, [country, city]); // ✅ All dependencies declared
// ...
```
However, since the Effect now uses the `city` state variable, you’ve had to add `city` to the list of dependencies. That, in turn, introduced a problem: when the user selects a different city, the Effect will re-run and call `fetchCities(country)`. As a result, you will be unnecessarily refetching the list of cities many times.
**The problem with this code is that you’re synchronizing two different unrelated things:**
1. You want to synchronize the `cities` state to the network based on the `country` prop.
2. You want to synchronize the `areas` state to the network based on the `city` state.
Split the logic into two Effects, each of which reacts to the prop that it needs to synchronize with:
```
function ShippingForm({ country }) {
const [cities, setCities] = useState(null);
useEffect(() => {
let ignore = false;
fetch(`/api/cities?country=${country}`)
.then(response => response.json())
.then(json => {
if (!ignore) {
setCities(json);
}
});
return () => {
ignore = true;
};
}, [country]); // ✅ All dependencies declared
const [city, setCity] = useState(null);
const [areas, setAreas] = useState(null);
useEffect(() => {
if (city) {
let ignore = false;
fetch(`/api/areas?city=${city}`)
.then(response => response.json())
.then(json => {
if (!ignore) {
setAreas(json);
}
});
return () => {
ignore = true;
};
}
}, [city]); // ✅ All dependencies declared
// ...
```
Now the first Effect only re-runs if the `country` changes, while the second Effect re-runs when the `city` changes. You’ve separated them by purpose: two different things are synchronized by two separate Effects. Two separate Effects have two separate dependency lists, so they won’t trigger each other unintentionally.
The final code is longer than the original, but splitting these Effects is still correct. [Each Effect should represent an independent synchronization process.](https://react.dev/learn/lifecycle-of-reactive-effects) In this example, deleting one Effect doesn’t break the other Effect’s logic. This means they *synchronize different things,* and it’s good to split them up. If you’re concerned about duplication, you can improve this code by [extracting repetitive logic into a custom Hook.](https://react.dev/learn/reusing-logic-with-custom-hooks)
### Are you reading some state to calculate the next state?[Link for Are you reading some state to calculate the next state?]()
This Effect updates the `messages` state variable with a newly created array every time a new message arrives:
```
function ChatRoom({ roomId }) {
const [messages, setMessages] = useState([]);
useEffect(() => {
const connection = createConnection();
connection.connect();
connection.on('message', (receivedMessage) => {
setMessages([...messages, receivedMessage]);
});
// ...
```
It uses the `messages` variable to [create a new array](https://react.dev/learn/updating-arrays-in-state) starting with all the existing messages and adds the new message at the end. However, since `messages` is a reactive value read by an Effect, it must be a dependency:
```
function ChatRoom({ roomId }) {
const [messages, setMessages] = useState([]);
useEffect(() => {
const connection = createConnection();
connection.connect();
connection.on('message', (receivedMessage) => {
setMessages([...messages, receivedMessage]);
});
return () => connection.disconnect();
}, [roomId, messages]); // ✅ All dependencies declared
// ...
```
And making `messages` a dependency introduces a problem.
Every time you receive a message, `setMessages()` causes the component to re-render with a new `messages` array that includes the received message. However, since this Effect now depends on `messages`, this will *also* re-synchronize the Effect. So every new message will make the chat re-connect. The user would not like that!
To fix the issue, don’t read `messages` inside the Effect. Instead, pass an [updater function](https://react.dev/reference/react/useState) to `setMessages`:
```
function ChatRoom({ roomId }) {
const [messages, setMessages] = useState([]);
useEffect(() => {
const connection = createConnection();
connection.connect();
connection.on('message', (receivedMessage) => {
setMessages(msgs => [...msgs, receivedMessage]);
});
return () => connection.disconnect();
}, [roomId]); // ✅ All dependencies declared
// ...
```
**Notice how your Effect does not read the `messages` variable at all now.** You only need to pass an updater function like `msgs => [...msgs, receivedMessage]`. React [puts your updater function in a queue](https://react.dev/learn/queueing-a-series-of-state-updates) and will provide the `msgs` argument to it during the next render. This is why the Effect itself doesn’t need to depend on `messages` anymore. As a result of this fix, receiving a chat message will no longer make the chat re-connect.
### Do you want to read a value without “reacting” to its changes?[Link for Do you want to read a value without “reacting” to its changes?]()
### Under Construction
This section describes an **experimental API that has not yet been released** in a stable version of React.
Suppose that you want to play a sound when the user receives a new message unless `isMuted` is `true`:
```
function ChatRoom({ roomId }) {
const [messages, setMessages] = useState([]);
const [isMuted, setIsMuted] = useState(false);
useEffect(() => {
const connection = createConnection();
connection.connect();
connection.on('message', (receivedMessage) => {
setMessages(msgs => [...msgs, receivedMessage]);
if (!isMuted) {
playSound();
}
});
// ...
```
Since your Effect now uses `isMuted` in its code, you have to add it to the dependencies:
```
function ChatRoom({ roomId }) {
const [messages, setMessages] = useState([]);
const [isMuted, setIsMuted] = useState(false);
useEffect(() => {
const connection = createConnection();
connection.connect();
connection.on('message', (receivedMessage) => {
setMessages(msgs => [...msgs, receivedMessage]);
if (!isMuted) {
playSound();
}
});
return () => connection.disconnect();
}, [roomId, isMuted]); // ✅ All dependencies declared
// ...
```
The problem is that every time `isMuted` changes (for example, when the user presses the “Muted” toggle), the Effect will re-synchronize, and reconnect to the chat. This is not the desired user experience! (In this example, even disabling the linter would not work—if you do that, `isMuted` would get “stuck” with its old value.)
To solve this problem, you need to extract the logic that shouldn’t be reactive out of the Effect. You don’t want this Effect to “react” to the changes in `isMuted`. [Move this non-reactive piece of logic into an Effect Event:](https://react.dev/learn/separating-events-from-effects)
```
import { useState, useEffect, useEffectEvent } from 'react';
function ChatRoom({ roomId }) {
const [messages, setMessages] = useState([]);
const [isMuted, setIsMuted] = useState(false);
const onMessage = useEffectEvent(receivedMessage => {
setMessages(msgs => [...msgs, receivedMessage]);
if (!isMuted) {
playSound();
}
});
useEffect(() => {
const connection = createConnection();
connection.connect();
connection.on('message', (receivedMessage) => {
onMessage(receivedMessage);
});
return () => connection.disconnect();
}, [roomId]); // ✅ All dependencies declared
// ...
```
Effect Events let you split an Effect into reactive parts (which should “react” to reactive values like `roomId` and their changes) and non-reactive parts (which only read their latest values, like `onMessage` reads `isMuted`). **Now that you read `isMuted` inside an Effect Event, it doesn’t need to be a dependency of your Effect.** As a result, the chat won’t re-connect when you toggle the “Muted” setting on and off, solving the original issue!
#### Wrapping an event handler from the props[Link for Wrapping an event handler from the props]()
You might run into a similar problem when your component receives an event handler as a prop:
```
function ChatRoom({ roomId, onReceiveMessage }) {
const [messages, setMessages] = useState([]);
useEffect(() => {
const connection = createConnection();
connection.connect();
connection.on('message', (receivedMessage) => {
onReceiveMessage(receivedMessage);
});
return () => connection.disconnect();
}, [roomId, onReceiveMessage]); // ✅ All dependencies declared
// ...
```
Suppose that the parent component passes a *different* `onReceiveMessage` function on every render:
```
<ChatRoom
roomId={roomId}
onReceiveMessage={receivedMessage => {
// ...
}}
/>
```
Since `onReceiveMessage` is a dependency, it would cause the Effect to re-synchronize after every parent re-render. This would make it re-connect to the chat. To solve this, wrap the call in an Effect Event:
```
function ChatRoom({ roomId, onReceiveMessage }) {
const [messages, setMessages] = useState([]);
const onMessage = useEffectEvent(receivedMessage => {
onReceiveMessage(receivedMessage);
});
useEffect(() => {
const connection = createConnection();
connection.connect();
connection.on('message', (receivedMessage) => {
onMessage(receivedMessage);
});
return () => connection.disconnect();
}, [roomId]); // ✅ All dependencies declared
// ...
```
Effect Events aren’t reactive, so you don’t need to specify them as dependencies. As a result, the chat will no longer re-connect even if the parent component passes a function that’s different on every re-render.
#### Separating reactive and non-reactive code[Link for Separating reactive and non-reactive code]()
In this example, you want to log a visit every time `roomId` changes. You want to include the current `notificationCount` with every log, but you *don’t* want a change to `notificationCount` to trigger a log event.
The solution is again to split out the non-reactive code into an Effect Event:
```
function Chat({ roomId, notificationCount }) {
const onVisit = useEffectEvent(visitedRoomId => {
logVisit(visitedRoomId, notificationCount);
});
useEffect(() => {
onVisit(roomId);
}, [roomId]); // ✅ All dependencies declared
// ...
}
```
You want your logic to be reactive with regards to `roomId`, so you read `roomId` inside of your Effect. However, you don’t want a change to `notificationCount` to log an extra visit, so you read `notificationCount` inside of the Effect Event. [Learn more about reading the latest props and state from Effects using Effect Events.](https://react.dev/learn/separating-events-from-effects)
### Does some reactive value change unintentionally?[Link for Does some reactive value change unintentionally?]()
Sometimes, you *do* want your Effect to “react” to a certain value, but that value changes more often than you’d like—and might not reflect any actual change from the user’s perspective. For example, let’s say that you create an `options` object in the body of your component, and then read that object from inside of your Effect:
```
function ChatRoom({ roomId }) {
// ...
const options = {
serverUrl: serverUrl,
roomId: roomId
};
useEffect(() => {
const connection = createConnection(options);
connection.connect();
// ...
```
This object is declared in the component body, so it’s a [reactive value.](https://react.dev/learn/lifecycle-of-reactive-effects) When you read a reactive value like this inside an Effect, you declare it as a dependency. This ensures your Effect “reacts” to its changes:
```
// ...
useEffect(() => {
const connection = createConnection(options);
connection.connect();
return () => connection.disconnect();
}, [options]); // ✅ All dependencies declared
// ...
```
It is important to declare it as a dependency! This ensures, for example, that if the `roomId` changes, your Effect will re-connect to the chat with the new `options`. However, there is also a problem with the code above. To see it, try typing into the input in the sandbox below, and watch what happens in the console:
App.jschat.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import { useState, useEffect } from 'react';
import { createConnection } from './chat.js';
const serverUrl = 'https://localhost:1234';
function ChatRoom({ roomId }) {
const [message, setMessage] = useState('');
// Temporarily disable the linter to demonstrate the problem
// eslint-disable-next-line react-hooks/exhaustive-deps
const options = {
serverUrl: serverUrl,
roomId: roomId
};
useEffect(() => {
const connection = createConnection(options);
connection.connect();
return () => connection.disconnect();
}, [options]);
return (
<>
<h1>Welcome to the {roomId} room!</h1>
<input value={message} onChange={e => setMessage(e.target.value)} />
</>
);
}
export default function App() {
const [roomId, setRoomId] = useState('general');
return (
<>
<label>
Choose the chat room:{' '}
<select
value={roomId}
onChange={e => setRoomId(e.target.value)}
>
<option value="general">general</option>
<option value="travel">travel</option>
<option value="music">music</option>
</select>
</label>
<hr />
<ChatRoom roomId={roomId} />
</>
);
}
```
Show more
In the sandbox above, the input only updates the `message` state variable. From the user’s perspective, this should not affect the chat connection. However, every time you update the `message`, your component re-renders. When your component re-renders, the code inside of it runs again from scratch.
A new `options` object is created from scratch on every re-render of the `ChatRoom` component. React sees that the `options` object is a *different object* from the `options` object created during the last render. This is why it re-synchronizes your Effect (which depends on `options`), and the chat re-connects as you type.
**This problem only affects objects and functions. In JavaScript, each newly created object and function is considered distinct from all the others. It doesn’t matter that the contents inside of them may be the same!**
```
// During the first render
const options1 = { serverUrl: 'https://localhost:1234', roomId: 'music' };
// During the next render
const options2 = { serverUrl: 'https://localhost:1234', roomId: 'music' };
// These are two different objects!
console.log(Object.is(options1, options2)); // false
```
**Object and function dependencies can make your Effect re-synchronize more often than you need.**
This is why, whenever possible, you should try to avoid objects and functions as your Effect’s dependencies. Instead, try moving them outside the component, inside the Effect, or extracting primitive values out of them.
#### Move static objects and functions outside your component[Link for Move static objects and functions outside your component]()
If the object does not depend on any props and state, you can move that object outside your component:
```
const options = {
serverUrl: 'https://localhost:1234',
roomId: 'music'
};
function ChatRoom() {
const [message, setMessage] = useState('');
useEffect(() => {
const connection = createConnection(options);
connection.connect();
return () => connection.disconnect();
}, []); // ✅ All dependencies declared
// ...
```
This way, you *prove* to the linter that it’s not reactive. It can’t change as a result of a re-render, so it doesn’t need to be a dependency. Now re-rendering `ChatRoom` won’t cause your Effect to re-synchronize.
This works for functions too:
```
function createOptions() {
return {
serverUrl: 'https://localhost:1234',
roomId: 'music'
};
}
function ChatRoom() {
const [message, setMessage] = useState('');
useEffect(() => {
const options = createOptions();
const connection = createConnection(options);
connection.connect();
return () => connection.disconnect();
}, []); // ✅ All dependencies declared
// ...
```
Since `createOptions` is declared outside your component, it’s not a reactive value. This is why it doesn’t need to be specified in your Effect’s dependencies, and why it won’t ever cause your Effect to re-synchronize.
#### Move dynamic objects and functions inside your Effect[Link for Move dynamic objects and functions inside your Effect]()
If your object depends on some reactive value that may change as a result of a re-render, like a `roomId` prop, you can’t pull it *outside* your component. You can, however, move its creation *inside* of your Effect’s code:
```
const serverUrl = 'https://localhost:1234';
function ChatRoom({ roomId }) {
const [message, setMessage] = useState('');
useEffect(() => {
const options = {
serverUrl: serverUrl,
roomId: roomId
};
const connection = createConnection(options);
connection.connect();
return () => connection.disconnect();
}, [roomId]); // ✅ All dependencies declared
// ...
```
Now that `options` is declared inside of your Effect, it is no longer a dependency of your Effect. Instead, the only reactive value used by your Effect is `roomId`. Since `roomId` is not an object or function, you can be sure that it won’t be *unintentionally* different. In JavaScript, numbers and strings are compared by their content:
```
// During the first render
const roomId1 = 'music';
// During the next render
const roomId2 = 'music';
// These two strings are the same!
console.log(Object.is(roomId1, roomId2)); // true
```
Thanks to this fix, the chat no longer re-connects if you edit the input:
App.jschat.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import { useState, useEffect } from 'react';
import { createConnection } from './chat.js';
const serverUrl = 'https://localhost:1234';
function ChatRoom({ roomId }) {
const [message, setMessage] = useState('');
useEffect(() => {
const options = {
serverUrl: serverUrl,
roomId: roomId
};
const connection = createConnection(options);
connection.connect();
return () => connection.disconnect();
}, [roomId]);
return (
<>
<h1>Welcome to the {roomId} room!</h1>
<input value={message} onChange={e => setMessage(e.target.value)} />
</>
);
}
export default function App() {
const [roomId, setRoomId] = useState('general');
return (
<>
<label>
Choose the chat room:{' '}
<select
value={roomId}
onChange={e => setRoomId(e.target.value)}
>
<option value="general">general</option>
<option value="travel">travel</option>
<option value="music">music</option>
</select>
</label>
<hr />
<ChatRoom roomId={roomId} />
</>
);
}
```
Show more
However, it *does* re-connect when you change the `roomId` dropdown, as you would expect.
This works for functions, too:
```
const serverUrl = 'https://localhost:1234';
function ChatRoom({ roomId }) {
const [message, setMessage] = useState('');
useEffect(() => {
function createOptions() {
return {
serverUrl: serverUrl,
roomId: roomId
};
}
const options = createOptions();
const connection = createConnection(options);
connection.connect();
return () => connection.disconnect();
}, [roomId]); // ✅ All dependencies declared
// ...
```
You can write your own functions to group pieces of logic inside your Effect. As long as you also declare them *inside* your Effect, they’re not reactive values, and so they don’t need to be dependencies of your Effect.
#### Read primitive values from objects[Link for Read primitive values from objects]()
Sometimes, you may receive an object from props:
```
function ChatRoom({ options }) {
const [message, setMessage] = useState('');
useEffect(() => {
const connection = createConnection(options);
connection.connect();
return () => connection.disconnect();
}, [options]); // ✅ All dependencies declared
// ...
```
The risk here is that the parent component will create the object during rendering:
```
<ChatRoom
roomId={roomId}
options={{
serverUrl: serverUrl,
roomId: roomId
}}
/>
```
This would cause your Effect to re-connect every time the parent component re-renders. To fix this, read information from the object *outside* the Effect, and avoid having object and function dependencies:
```
function ChatRoom({ options }) {
const [message, setMessage] = useState('');
const { roomId, serverUrl } = options;
useEffect(() => {
const connection = createConnection({
roomId: roomId,
serverUrl: serverUrl
});
connection.connect();
return () => connection.disconnect();
}, [roomId, serverUrl]); // ✅ All dependencies declared
// ...
```
The logic gets a little repetitive (you read some values from an object outside an Effect, and then create an object with the same values inside the Effect). But it makes it very explicit what information your Effect *actually* depends on. If an object is re-created unintentionally by the parent component, the chat would not re-connect. However, if `options.roomId` or `options.serverUrl` really are different, the chat would re-connect.
#### Calculate primitive values from functions[Link for Calculate primitive values from functions]()
The same approach can work for functions. For example, suppose the parent component passes a function:
```
<ChatRoom
roomId={roomId}
getOptions={() => {
return {
serverUrl: serverUrl,
roomId: roomId
};
}}
/>
```
To avoid making it a dependency (and causing it to re-connect on re-renders), call it outside the Effect. This gives you the `roomId` and `serverUrl` values that aren’t objects, and that you can read from inside your Effect:
```
function ChatRoom({ getOptions }) {
const [message, setMessage] = useState('');
const { roomId, serverUrl } = getOptions();
useEffect(() => {
const connection = createConnection({
roomId: roomId,
serverUrl: serverUrl
});
connection.connect();
return () => connection.disconnect();
}, [roomId, serverUrl]); // ✅ All dependencies declared
// ...
```
This only works for [pure](https://react.dev/learn/keeping-components-pure) functions because they are safe to call during rendering. If your function is an event handler, but you don’t want its changes to re-synchronize your Effect, [wrap it into an Effect Event instead.]()
## Recap[Link for Recap]()
- Dependencies should always match the code.
- When you’re not happy with your dependencies, what you need to edit is the code.
- Suppressing the linter leads to very confusing bugs, and you should always avoid it.
- To remove a dependency, you need to “prove” to the linter that it’s not necessary.
- If some code should run in response to a specific interaction, move that code to an event handler.
- If different parts of your Effect should re-run for different reasons, split it into several Effects.
- If you want to update some state based on the previous state, pass an updater function.
- If you want to read the latest value without “reacting” it, extract an Effect Event from your Effect.
- In JavaScript, objects and functions are considered different if they were created at different times.
- Try to avoid object and function dependencies. Move them outside the component or inside the Effect.
## Try out some challenges[Link for Try out some challenges]()
1\. Fix a resetting interval 2. Fix a retriggering animation 3. Fix a reconnecting chat 4. Fix a reconnecting chat, again
#### Challenge 1 of 4: Fix a resetting interval[Link for this heading]()
This Effect sets up an interval that ticks every second. You’ve noticed something strange happening: it seems like the interval gets destroyed and re-created every time it ticks. Fix the code so that the interval doesn’t get constantly re-created.
App.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import { useState, useEffect } from 'react';
export default function Timer() {
const [count, setCount] = useState(0);
useEffect(() => {
console.log('✅ Creating an interval');
const id = setInterval(() => {
console.log('⏰ Interval tick');
setCount(count + 1);
}, 1000);
return () => {
console.log('❌ Clearing an interval');
clearInterval(id);
};
}, [count]);
return <h1>Counter: {count}</h1>
}
```
Show more
Show hint Show solution
Next Challenge
[PreviousSeparating Events from Effects](https://react.dev/learn/separating-events-from-effects)
[NextReusing Logic with Custom Hooks](https://react.dev/learn/reusing-logic-with-custom-hooks) |
https://react.dev/learn/updating-arrays-in-state | [Learn React](https://react.dev/learn)
[Adding Interactivity](https://react.dev/learn/adding-interactivity)
# Updating Arrays in State[Link for this heading]()
Arrays are mutable in JavaScript, but you should treat them as immutable when you store them in state. Just like with objects, when you want to update an array stored in state, you need to create a new one (or make a copy of an existing one), and then set state to use the new array.
### You will learn
- How to add, remove, or change items in an array in React state
- How to update an object inside of an array
- How to make array copying less repetitive with Immer
## Updating arrays without mutation[Link for Updating arrays without mutation]()
In JavaScript, arrays are just another kind of object. [Like with objects](https://react.dev/learn/updating-objects-in-state), **you should treat arrays in React state as read-only.** This means that you shouldn’t reassign items inside an array like `arr[0] = 'bird'`, and you also shouldn’t use methods that mutate the array, such as `push()` and `pop()`.
Instead, every time you want to update an array, you’ll want to pass a *new* array to your state setting function. To do that, you can create a new array from the original array in your state by calling its non-mutating methods like `filter()` and `map()`. Then you can set your state to the resulting new array.
Here is a reference table of common array operations. When dealing with arrays inside React state, you will need to avoid the methods in the left column, and instead prefer the methods in the right column:
avoid (mutates the array)prefer (returns a new array)adding`push`, `unshift``concat`, `[...arr]` spread syntax ([example]())removing`pop`, `shift`, `splice``filter`, `slice` ([example]())replacing`splice`, `arr[i] = ...` assignment`map` ([example]())sorting`reverse`, `sort`copy the array first ([example]())
Alternatively, you can [use Immer]() which lets you use methods from both columns.
### Pitfall
Unfortunately, [`slice`](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Array/slice) and [`splice`](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Array/splice) are named similarly but are very different:
- `slice` lets you copy an array or a part of it.
- `splice` **mutates** the array (to insert or delete items).
In React, you will be using `slice` (no `p`!) a lot more often because you don’t want to mutate objects or arrays in state. [Updating Objects](https://react.dev/learn/updating-objects-in-state) explains what mutation is and why it’s not recommended for state.
### Adding to an array[Link for Adding to an array]()
`push()` will mutate an array, which you don’t want:
App.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import { useState } from 'react';
let nextId = 0;
export default function List() {
const [name, setName] = useState('');
const [artists, setArtists] = useState([]);
return (
<>
<h1>Inspiring sculptors:</h1>
<input
value={name}
onChange={e => setName(e.target.value)}
/>
<button onClick={() => {
artists.push({
id: nextId++,
name: name,
});
}}>Add</button>
<ul>
{artists.map(artist => (
<li key={artist.id}>{artist.name}</li>
))}
</ul>
</>
);
}
```
Show more
Instead, create a *new* array which contains the existing items *and* a new item at the end. There are multiple ways to do this, but the easiest one is to use the `...` [array spread](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Operators/Spread_syntax) syntax:
```
setArtists( // Replace the state
[ // with a new array
...artists, // that contains all the old items
{ id: nextId++, name: name } // and one new item at the end
]
);
```
Now it works correctly:
App.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import { useState } from 'react';
let nextId = 0;
export default function List() {
const [name, setName] = useState('');
const [artists, setArtists] = useState([]);
return (
<>
<h1>Inspiring sculptors:</h1>
<input
value={name}
onChange={e => setName(e.target.value)}
/>
<button onClick={() => {
setArtists([
...artists,
{ id: nextId++, name: name }
]);
}}>Add</button>
<ul>
{artists.map(artist => (
<li key={artist.id}>{artist.name}</li>
))}
</ul>
</>
);
}
```
Show more
The array spread syntax also lets you prepend an item by placing it *before* the original `...artists`:
```
setArtists([
{ id: nextId++, name: name },
...artists // Put old items at the end
]);
```
In this way, spread can do the job of both `push()` by adding to the end of an array and `unshift()` by adding to the beginning of an array. Try it in the sandbox above!
### Removing from an array[Link for Removing from an array]()
The easiest way to remove an item from an array is to *filter it out*. In other words, you will produce a new array that will not contain that item. To do this, use the `filter` method, for example:
App.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import { useState } from 'react';
let initialArtists = [
{ id: 0, name: 'Marta Colvin Andrade' },
{ id: 1, name: 'Lamidi Olonade Fakeye'},
{ id: 2, name: 'Louise Nevelson'},
];
export default function List() {
const [artists, setArtists] = useState(
initialArtists
);
return (
<>
<h1>Inspiring sculptors:</h1>
<ul>
{artists.map(artist => (
<li key={artist.id}>
{artist.name}{' '}
<button onClick={() => {
setArtists(
artists.filter(a =>
a.id !== artist.id
)
);
}}>
Delete
</button>
</li>
))}
</ul>
</>
);
}
```
Show more
Click the “Delete” button a few times, and look at its click handler.
```
setArtists(
artists.filter(a => a.id !== artist.id)
);
```
Here, `artists.filter(a => a.id !== artist.id)` means “create an array that consists of those `artists` whose IDs are different from `artist.id`”. In other words, each artist’s “Delete” button will filter *that* artist out of the array, and then request a re-render with the resulting array. Note that `filter` does not modify the original array.
### Transforming an array[Link for Transforming an array]()
If you want to change some or all items of the array, you can use `map()` to create a **new** array. The function you will pass to `map` can decide what to do with each item, based on its data or its index (or both).
In this example, an array holds coordinates of two circles and a square. When you press the button, it moves only the circles down by 50 pixels. It does this by producing a new array of data using `map()`:
App.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import { useState } from 'react';
let initialShapes = [
{ id: 0, type: 'circle', x: 50, y: 100 },
{ id: 1, type: 'square', x: 150, y: 100 },
{ id: 2, type: 'circle', x: 250, y: 100 },
];
export default function ShapeEditor() {
const [shapes, setShapes] = useState(
initialShapes
);
function handleClick() {
const nextShapes = shapes.map(shape => {
if (shape.type === 'square') {
// No change
return shape;
} else {
// Return a new circle 50px below
return {
...shape,
y: shape.y + 50,
};
}
});
// Re-render with the new array
setShapes(nextShapes);
}
return (
<>
<button onClick={handleClick}>
Move circles down!
</button>
{shapes.map(shape => (
<div
key={shape.id}
style={{
background: 'purple',
position: 'absolute',
left: shape.x,
top: shape.y,
borderRadius:
shape.type === 'circle'
? '50%' : '',
width: 20,
height: 20,
}} />
))}
</>
);
}
```
Show more
### Replacing items in an array[Link for Replacing items in an array]()
It is particularly common to want to replace one or more items in an array. Assignments like `arr[0] = 'bird'` are mutating the original array, so instead you’ll want to use `map` for this as well.
To replace an item, create a new array with `map`. Inside your `map` call, you will receive the item index as the second argument. Use it to decide whether to return the original item (the first argument) or something else:
App.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import { useState } from 'react';
let initialCounters = [
0, 0, 0
];
export default function CounterList() {
const [counters, setCounters] = useState(
initialCounters
);
function handleIncrementClick(index) {
const nextCounters = counters.map((c, i) => {
if (i === index) {
// Increment the clicked counter
return c + 1;
} else {
// The rest haven't changed
return c;
}
});
setCounters(nextCounters);
}
return (
<ul>
{counters.map((counter, i) => (
<li key={i}>
{counter}
<button onClick={() => {
handleIncrementClick(i);
}}>+1</button>
</li>
))}
</ul>
);
}
```
Show more
### Inserting into an array[Link for Inserting into an array]()
Sometimes, you may want to insert an item at a particular position that’s neither at the beginning nor at the end. To do this, you can use the `...` array spread syntax together with the `slice()` method. The `slice()` method lets you cut a “slice” of the array. To insert an item, you will create an array that spreads the slice *before* the insertion point, then the new item, and then the rest of the original array.
In this example, the Insert button always inserts at the index `1`:
App.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import { useState } from 'react';
let nextId = 3;
const initialArtists = [
{ id: 0, name: 'Marta Colvin Andrade' },
{ id: 1, name: 'Lamidi Olonade Fakeye'},
{ id: 2, name: 'Louise Nevelson'},
];
export default function List() {
const [name, setName] = useState('');
const [artists, setArtists] = useState(
initialArtists
);
function handleClick() {
const insertAt = 1; // Could be any index
const nextArtists = [
// Items before the insertion point:
...artists.slice(0, insertAt),
// New item:
{ id: nextId++, name: name },
// Items after the insertion point:
...artists.slice(insertAt)
];
setArtists(nextArtists);
setName('');
}
return (
<>
<h1>Inspiring sculptors:</h1>
<input
value={name}
onChange={e => setName(e.target.value)}
/>
<button onClick={handleClick}>
Insert
</button>
<ul>
{artists.map(artist => (
<li key={artist.id}>{artist.name}</li>
))}
</ul>
</>
);
}
```
Show more
### Making other changes to an array[Link for Making other changes to an array]()
There are some things you can’t do with the spread syntax and non-mutating methods like `map()` and `filter()` alone. For example, you may want to reverse or sort an array. The JavaScript `reverse()` and `sort()` methods are mutating the original array, so you can’t use them directly.
**However, you can copy the array first, and then make changes to it.**
For example:
App.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import { useState } from 'react';
const initialList = [
{ id: 0, title: 'Big Bellies' },
{ id: 1, title: 'Lunar Landscape' },
{ id: 2, title: 'Terracotta Army' },
];
export default function List() {
const [list, setList] = useState(initialList);
function handleClick() {
const nextList = [...list];
nextList.reverse();
setList(nextList);
}
return (
<>
<button onClick={handleClick}>
Reverse
</button>
<ul>
{list.map(artwork => (
<li key={artwork.id}>{artwork.title}</li>
))}
</ul>
</>
);
}
```
Show more
Here, you use the `[...list]` spread syntax to create a copy of the original array first. Now that you have a copy, you can use mutating methods like `nextList.reverse()` or `nextList.sort()`, or even assign individual items with `nextList[0] = "something"`.
However, **even if you copy an array, you can’t mutate existing items *inside* of it directly.** This is because copying is shallow—the new array will contain the same items as the original one. So if you modify an object inside the copied array, you are mutating the existing state. For example, code like this is a problem.
```
const nextList = [...list];
nextList[0].seen = true; // Problem: mutates list[0]
setList(nextList);
```
Although `nextList` and `list` are two different arrays, **`nextList[0]` and `list[0]` point to the same object.** So by changing `nextList[0].seen`, you are also changing `list[0].seen`. This is a state mutation, which you should avoid! You can solve this issue in a similar way to [updating nested JavaScript objects](https://react.dev/learn/updating-objects-in-state)—by copying individual items you want to change instead of mutating them. Here’s how.
## Updating objects inside arrays[Link for Updating objects inside arrays]()
Objects are not *really* located “inside” arrays. They might appear to be “inside” in code, but each object in an array is a separate value, to which the array “points”. This is why you need to be careful when changing nested fields like `list[0]`. Another person’s artwork list may point to the same element of the array!
**When updating nested state, you need to create copies from the point where you want to update, and all the way up to the top level.** Let’s see how this works.
In this example, two separate artwork lists have the same initial state. They are supposed to be isolated, but because of a mutation, their state is accidentally shared, and checking a box in one list affects the other list:
App.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import { useState } from 'react';
let nextId = 3;
const initialList = [
{ id: 0, title: 'Big Bellies', seen: false },
{ id: 1, title: 'Lunar Landscape', seen: false },
{ id: 2, title: 'Terracotta Army', seen: true },
];
export default function BucketList() {
const [myList, setMyList] = useState(initialList);
const [yourList, setYourList] = useState(
initialList
);
function handleToggleMyList(artworkId, nextSeen) {
const myNextList = [...myList];
const artwork = myNextList.find(
a => a.id === artworkId
);
artwork.seen = nextSeen;
setMyList(myNextList);
}
function handleToggleYourList(artworkId, nextSeen) {
const yourNextList = [...yourList];
const artwork = yourNextList.find(
a => a.id === artworkId
);
artwork.seen = nextSeen;
setYourList(yourNextList);
}
return (
<>
<h1>Art Bucket List</h1>
<h2>My list of art to see:</h2>
<ItemList
artworks={myList}
onToggle={handleToggleMyList} />
<h2>Your list of art to see:</h2>
<ItemList
artworks={yourList}
onToggle={handleToggleYourList} />
</>
);
}
function ItemList({ artworks, onToggle }) {
return (
<ul>
{artworks.map(artwork => (
<li key={artwork.id}>
<label>
<input
type="checkbox"
checked={artwork.seen}
onChange={e => {
onToggle(
artwork.id,
e.target.checked
);
}}
/>
{artwork.title}
</label>
</li>
))}
</ul>
);
}
```
Show more
The problem is in code like this:
```
const myNextList = [...myList];
const artwork = myNextList.find(a => a.id === artworkId);
artwork.seen = nextSeen; // Problem: mutates an existing item
setMyList(myNextList);
```
Although the `myNextList` array itself is new, the *items themselves* are the same as in the original `myList` array. So changing `artwork.seen` changes the *original* artwork item. That artwork item is also in `yourList`, which causes the bug. Bugs like this can be difficult to think about, but thankfully they disappear if you avoid mutating state.
**You can use `map` to substitute an old item with its updated version without mutation.**
```
setMyList(myList.map(artwork => {
if (artwork.id === artworkId) {
// Create a *new* object with changes
return { ...artwork, seen: nextSeen };
} else {
// No changes
return artwork;
}
}));
```
Here, `...` is the object spread syntax used to [create a copy of an object.](https://react.dev/learn/updating-objects-in-state)
With this approach, none of the existing state items are being mutated, and the bug is fixed:
App.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import { useState } from 'react';
let nextId = 3;
const initialList = [
{ id: 0, title: 'Big Bellies', seen: false },
{ id: 1, title: 'Lunar Landscape', seen: false },
{ id: 2, title: 'Terracotta Army', seen: true },
];
export default function BucketList() {
const [myList, setMyList] = useState(initialList);
const [yourList, setYourList] = useState(
initialList
);
function handleToggleMyList(artworkId, nextSeen) {
setMyList(myList.map(artwork => {
if (artwork.id === artworkId) {
// Create a *new* object with changes
return { ...artwork, seen: nextSeen };
} else {
// No changes
return artwork;
}
}));
}
function handleToggleYourList(artworkId, nextSeen) {
setYourList(yourList.map(artwork => {
if (artwork.id === artworkId) {
// Create a *new* object with changes
return { ...artwork, seen: nextSeen };
} else {
// No changes
return artwork;
}
}));
}
return (
<>
<h1>Art Bucket List</h1>
<h2>My list of art to see:</h2>
<ItemList
artworks={myList}
onToggle={handleToggleMyList} />
<h2>Your list of art to see:</h2>
<ItemList
artworks={yourList}
onToggle={handleToggleYourList} />
</>
);
}
function ItemList({ artworks, onToggle }) {
return (
<ul>
{artworks.map(artwork => (
<li key={artwork.id}>
<label>
<input
type="checkbox"
checked={artwork.seen}
onChange={e => {
onToggle(
artwork.id,
e.target.checked
);
}}
/>
{artwork.title}
</label>
</li>
))}
</ul>
);
}
```
Show more
In general, **you should only mutate objects that you have just created.** If you were inserting a *new* artwork, you could mutate it, but if you’re dealing with something that’s already in state, you need to make a copy.
### Write concise update logic with Immer[Link for Write concise update logic with Immer]()
Updating nested arrays without mutation can get a little bit repetitive. [Just as with objects](https://react.dev/learn/updating-objects-in-state):
- Generally, you shouldn’t need to update state more than a couple of levels deep. If your state objects are very deep, you might want to [restructure them differently](https://react.dev/learn/choosing-the-state-structure) so that they are flat.
- If you don’t want to change your state structure, you might prefer to use [Immer](https://github.com/immerjs/use-immer), which lets you write using the convenient but mutating syntax and takes care of producing the copies for you.
Here is the Art Bucket List example rewritten with Immer:
package.jsonApp.js
package.json
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
{
"dependencies": {
"immer": "1.7.3",
"react": "latest",
"react-dom": "latest",
"react-scripts": "latest",
"use-immer": "0.5.1"
},
"scripts": {
"start": "react-scripts start",
"build": "react-scripts build",
"test": "react-scripts test --env=jsdom",
"eject": "react-scripts eject"
},
"devDependencies": {}
}
```
Note how with Immer, **mutation like `artwork.seen = nextSeen` is now okay:**
```
updateMyTodos(draft => {
const artwork = draft.find(a => a.id === artworkId);
artwork.seen = nextSeen;
});
```
This is because you’re not mutating the *original* state, but you’re mutating a special `draft` object provided by Immer. Similarly, you can apply mutating methods like `push()` and `pop()` to the content of the `draft`.
Behind the scenes, Immer always constructs the next state from scratch according to the changes that you’ve done to the `draft`. This keeps your event handlers very concise without ever mutating state.
## Recap[Link for Recap]()
- You can put arrays into state, but you can’t change them.
- Instead of mutating an array, create a *new* version of it, and update the state to it.
- You can use the `[...arr, newItem]` array spread syntax to create arrays with new items.
- You can use `filter()` and `map()` to create new arrays with filtered or transformed items.
- You can use Immer to keep your code concise.
## Try out some challenges[Link for Try out some challenges]()
1\. Update an item in the shopping cart 2. Remove an item from the shopping cart 3. Fix the mutations using non-mutative methods 4. Fix the mutations using Immer
#### Challenge 1 of 4: Update an item in the shopping cart[Link for this heading]()
Fill in the `handleIncreaseClick` logic so that pressing ”+” increases the corresponding number:
App.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import { useState } from 'react';
const initialProducts = [{
id: 0,
name: 'Baklava',
count: 1,
}, {
id: 1,
name: 'Cheese',
count: 5,
}, {
id: 2,
name: 'Spaghetti',
count: 2,
}];
export default function ShoppingCart() {
const [
products,
setProducts
] = useState(initialProducts)
function handleIncreaseClick(productId) {
}
return (
<ul>
{products.map(product => (
<li key={product.id}>
{product.name}
{' '}
(<b>{product.count}</b>)
<button onClick={() => {
handleIncreaseClick(product.id);
}}>
+
</button>
</li>
))}
</ul>
);
}
```
Show more
Show solutionNext Challenge
[PreviousUpdating Objects in State](https://react.dev/learn/updating-objects-in-state)
[NextManaging State](https://react.dev/learn/managing-state) |
https://react.dev/learn/separating-events-from-effects | [Learn React](https://react.dev/learn)
[Escape Hatches](https://react.dev/learn/escape-hatches)
# Separating Events from Effects[Link for this heading]()
Event handlers only re-run when you perform the same interaction again. Unlike event handlers, Effects re-synchronize if some value they read, like a prop or a state variable, is different from what it was during the last render. Sometimes, you also want a mix of both behaviors: an Effect that re-runs in response to some values but not others. This page will teach you how to do that.
### You will learn
- How to choose between an event handler and an Effect
- Why Effects are reactive, and event handlers are not
- What to do when you want a part of your Effect’s code to not be reactive
- What Effect Events are, and how to extract them from your Effects
- How to read the latest props and state from Effects using Effect Events
## Choosing between event handlers and Effects[Link for Choosing between event handlers and Effects]()
First, let’s recap the difference between event handlers and Effects.
Imagine you’re implementing a chat room component. Your requirements look like this:
1. Your component should automatically connect to the selected chat room.
2. When you click the “Send” button, it should send a message to the chat.
Let’s say you’ve already implemented the code for them, but you’re not sure where to put it. Should you use event handlers or Effects? Every time you need to answer this question, consider [*why* the code needs to run.](https://react.dev/learn/synchronizing-with-effects)
### Event handlers run in response to specific interactions[Link for Event handlers run in response to specific interactions]()
From the user’s perspective, sending a message should happen *because* the particular “Send” button was clicked. The user will get rather upset if you send their message at any other time or for any other reason. This is why sending a message should be an event handler. Event handlers let you handle specific interactions:
```
function ChatRoom({ roomId }) {
const [message, setMessage] = useState('');
// ...
function handleSendClick() {
sendMessage(message);
}
// ...
return (
<>
<input value={message} onChange={e => setMessage(e.target.value)} />
<button onClick={handleSendClick}>Send</button>
</>
);
}
```
With an event handler, you can be sure that `sendMessage(message)` will *only* run if the user presses the button.
### Effects run whenever synchronization is needed[Link for Effects run whenever synchronization is needed]()
Recall that you also need to keep the component connected to the chat room. Where does that code go?
The *reason* to run this code is not some particular interaction. It doesn’t matter why or how the user navigated to the chat room screen. Now that they’re looking at it and could interact with it, the component needs to stay connected to the selected chat server. Even if the chat room component was the initial screen of your app, and the user has not performed any interactions at all, you would *still* need to connect. This is why it’s an Effect:
```
function ChatRoom({ roomId }) {
// ...
useEffect(() => {
const connection = createConnection(serverUrl, roomId);
connection.connect();
return () => {
connection.disconnect();
};
}, [roomId]);
// ...
}
```
With this code, you can be sure that there is always an active connection to the currently selected chat server, *regardless* of the specific interactions performed by the user. Whether the user has only opened your app, selected a different room, or navigated to another screen and back, your Effect ensures that the component will *remain synchronized* with the currently selected room, and will [re-connect whenever it’s necessary.](https://react.dev/learn/lifecycle-of-reactive-effects)
App.jschat.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import { useState, useEffect } from 'react';
import { createConnection, sendMessage } from './chat.js';
const serverUrl = 'https://localhost:1234';
function ChatRoom({ roomId }) {
const [message, setMessage] = useState('');
useEffect(() => {
const connection = createConnection(serverUrl, roomId);
connection.connect();
return () => connection.disconnect();
}, [roomId]);
function handleSendClick() {
sendMessage(message);
}
return (
<>
<h1>Welcome to the {roomId} room!</h1>
<input value={message} onChange={e => setMessage(e.target.value)} />
<button onClick={handleSendClick}>Send</button>
</>
);
}
export default function App() {
const [roomId, setRoomId] = useState('general');
const [show, setShow] = useState(false);
return (
<>
<label>
Choose the chat room:{' '}
<select
value={roomId}
onChange={e => setRoomId(e.target.value)}
>
<option value="general">general</option>
<option value="travel">travel</option>
<option value="music">music</option>
</select>
</label>
<button onClick={() => setShow(!show)}>
{show ? 'Close chat' : 'Open chat'}
</button>
{show && <hr />}
{show && <ChatRoom roomId={roomId} />}
</>
);
}
```
Show more
## Reactive values and reactive logic[Link for Reactive values and reactive logic]()
Intuitively, you could say that event handlers are always triggered “manually”, for example by clicking a button. Effects, on the other hand, are “automatic”: they run and re-run as often as it’s needed to stay synchronized.
There is a more precise way to think about this.
Props, state, and variables declared inside your component’s body are called reactive values. In this example, `serverUrl` is not a reactive value, but `roomId` and `message` are. They participate in the rendering data flow:
```
const serverUrl = 'https://localhost:1234';
function ChatRoom({ roomId }) {
const [message, setMessage] = useState('');
// ...
}
```
Reactive values like these can change due to a re-render. For example, the user may edit the `message` or choose a different `roomId` in a dropdown. Event handlers and Effects respond to changes differently:
- **Logic inside event handlers is *not reactive.*** It will not run again unless the user performs the same interaction (e.g. a click) again. Event handlers can read reactive values without “reacting” to their changes.
- **Logic inside Effects is *reactive.*** If your Effect reads a reactive value, [you have to specify it as a dependency.](https://react.dev/learn/lifecycle-of-reactive-effects) Then, if a re-render causes that value to change, React will re-run your Effect’s logic with the new value.
Let’s revisit the previous example to illustrate this difference.
### Logic inside event handlers is not reactive[Link for Logic inside event handlers is not reactive]()
Take a look at this line of code. Should this logic be reactive or not?
```
// ...
sendMessage(message);
// ...
```
From the user’s perspective, **a change to the `message` does *not* mean that they want to send a message.** It only means that the user is typing. In other words, the logic that sends a message should not be reactive. It should not run again only because the reactive value has changed. That’s why it belongs in the event handler:
```
function handleSendClick() {
sendMessage(message);
}
```
Event handlers aren’t reactive, so `sendMessage(message)` will only run when the user clicks the Send button.
### Logic inside Effects is reactive[Link for Logic inside Effects is reactive]()
Now let’s return to these lines:
```
// ...
const connection = createConnection(serverUrl, roomId);
connection.connect();
// ...
```
From the user’s perspective, **a change to the `roomId` *does* mean that they want to connect to a different room.** In other words, the logic for connecting to the room should be reactive. You *want* these lines of code to “keep up” with the reactive value, and to run again if that value is different. That’s why it belongs in an Effect:
```
useEffect(() => {
const connection = createConnection(serverUrl, roomId);
connection.connect();
return () => {
connection.disconnect()
};
}, [roomId]);
```
Effects are reactive, so `createConnection(serverUrl, roomId)` and `connection.connect()` will run for every distinct value of `roomId`. Your Effect keeps the chat connection synchronized to the currently selected room.
## Extracting non-reactive logic out of Effects[Link for Extracting non-reactive logic out of Effects]()
Things get more tricky when you want to mix reactive logic with non-reactive logic.
For example, imagine that you want to show a notification when the user connects to the chat. You read the current theme (dark or light) from the props so that you can show the notification in the correct color:
```
function ChatRoom({ roomId, theme }) {
useEffect(() => {
const connection = createConnection(serverUrl, roomId);
connection.on('connected', () => {
showNotification('Connected!', theme);
});
connection.connect();
// ...
```
However, `theme` is a reactive value (it can change as a result of re-rendering), and [every reactive value read by an Effect must be declared as its dependency.](https://react.dev/learn/lifecycle-of-reactive-effects) Now you have to specify `theme` as a dependency of your Effect:
```
function ChatRoom({ roomId, theme }) {
useEffect(() => {
const connection = createConnection(serverUrl, roomId);
connection.on('connected', () => {
showNotification('Connected!', theme);
});
connection.connect();
return () => {
connection.disconnect()
};
}, [roomId, theme]); // ✅ All dependencies declared
// ...
```
Play with this example and see if you can spot the problem with this user experience:
App.jschat.jsnotifications.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import { useState, useEffect } from 'react';
import { createConnection, sendMessage } from './chat.js';
import { showNotification } from './notifications.js';
const serverUrl = 'https://localhost:1234';
function ChatRoom({ roomId, theme }) {
useEffect(() => {
const connection = createConnection(serverUrl, roomId);
connection.on('connected', () => {
showNotification('Connected!', theme);
});
connection.connect();
return () => connection.disconnect();
}, [roomId, theme]);
return <h1>Welcome to the {roomId} room!</h1>
}
export default function App() {
const [roomId, setRoomId] = useState('general');
const [isDark, setIsDark] = useState(false);
return (
<>
<label>
Choose the chat room:{' '}
<select
value={roomId}
onChange={e => setRoomId(e.target.value)}
>
<option value="general">general</option>
<option value="travel">travel</option>
<option value="music">music</option>
</select>
</label>
<label>
<input
type="checkbox"
checked={isDark}
onChange={e => setIsDark(e.target.checked)}
/>
Use dark theme
</label>
<hr />
<ChatRoom
roomId={roomId}
theme={isDark ? 'dark' : 'light'}
/>
</>
);
}
```
Show more
When the `roomId` changes, the chat re-connects as you would expect. But since `theme` is also a dependency, the chat *also* re-connects every time you switch between the dark and the light theme. That’s not great!
In other words, you *don’t* want this line to be reactive, even though it is inside an Effect (which is reactive):
```
// ...
showNotification('Connected!', theme);
// ...
```
You need a way to separate this non-reactive logic from the reactive Effect around it.
### Declaring an Effect Event[Link for Declaring an Effect Event]()
### Under Construction
This section describes an **experimental API that has not yet been released** in a stable version of React.
Use a special Hook called [`useEffectEvent`](https://react.dev/reference/react/experimental_useEffectEvent) to extract this non-reactive logic out of your Effect:
```
import { useEffect, useEffectEvent } from 'react';
function ChatRoom({ roomId, theme }) {
const onConnected = useEffectEvent(() => {
showNotification('Connected!', theme);
});
// ...
```
Here, `onConnected` is called an *Effect Event.* It’s a part of your Effect logic, but it behaves a lot more like an event handler. The logic inside it is not reactive, and it always “sees” the latest values of your props and state.
Now you can call the `onConnected` Effect Event from inside your Effect:
```
function ChatRoom({ roomId, theme }) {
const onConnected = useEffectEvent(() => {
showNotification('Connected!', theme);
});
useEffect(() => {
const connection = createConnection(serverUrl, roomId);
connection.on('connected', () => {
onConnected();
});
connection.connect();
return () => connection.disconnect();
}, [roomId]); // ✅ All dependencies declared
// ...
```
This solves the problem. Note that you had to *remove* `onConnected` from the list of your Effect’s dependencies. **Effect Events are not reactive and must be omitted from dependencies.**
Verify that the new behavior works as you would expect:
App.jschat.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import { useState, useEffect } from 'react';
import { experimental_useEffectEvent as useEffectEvent } from 'react';
import { createConnection, sendMessage } from './chat.js';
import { showNotification } from './notifications.js';
const serverUrl = 'https://localhost:1234';
function ChatRoom({ roomId, theme }) {
const onConnected = useEffectEvent(() => {
showNotification('Connected!', theme);
});
useEffect(() => {
const connection = createConnection(serverUrl, roomId);
connection.on('connected', () => {
onConnected();
});
connection.connect();
return () => connection.disconnect();
}, [roomId]);
return <h1>Welcome to the {roomId} room!</h1>
}
export default function App() {
const [roomId, setRoomId] = useState('general');
const [isDark, setIsDark] = useState(false);
return (
<>
<label>
Choose the chat room:{' '}
<select
value={roomId}
onChange={e => setRoomId(e.target.value)}
>
<option value="general">general</option>
<option value="travel">travel</option>
<option value="music">music</option>
</select>
</label>
<label>
<input
type="checkbox"
checked={isDark}
onChange={e => setIsDark(e.target.checked)}
/>
Use dark theme
</label>
<hr />
<ChatRoom
roomId={roomId}
theme={isDark ? 'dark' : 'light'}
/>
</>
);
}
```
Show more
You can think of Effect Events as being very similar to event handlers. The main difference is that event handlers run in response to a user interactions, whereas Effect Events are triggered by you from Effects. Effect Events let you “break the chain” between the reactivity of Effects and code that should not be reactive.
### Reading latest props and state with Effect Events[Link for Reading latest props and state with Effect Events]()
### Under Construction
This section describes an **experimental API that has not yet been released** in a stable version of React.
Effect Events let you fix many patterns where you might be tempted to suppress the dependency linter.
For example, say you have an Effect to log the page visits:
```
function Page() {
useEffect(() => {
logVisit();
}, []);
// ...
}
```
Later, you add multiple routes to your site. Now your `Page` component receives a `url` prop with the current path. You want to pass the `url` as a part of your `logVisit` call, but the dependency linter complains:
```
function Page({ url }) {
useEffect(() => {
logVisit(url);
}, []); // 🔴 React Hook useEffect has a missing dependency: 'url'
// ...
}
```
Think about what you want the code to do. You *want* to log a separate visit for different URLs since each URL represents a different page. In other words, this `logVisit` call *should* be reactive with respect to the `url`. This is why, in this case, it makes sense to follow the dependency linter, and add `url` as a dependency:
```
function Page({ url }) {
useEffect(() => {
logVisit(url);
}, [url]); // ✅ All dependencies declared
// ...
}
```
Now let’s say you want to include the number of items in the shopping cart together with every page visit:
```
function Page({ url }) {
const { items } = useContext(ShoppingCartContext);
const numberOfItems = items.length;
useEffect(() => {
logVisit(url, numberOfItems);
}, [url]); // 🔴 React Hook useEffect has a missing dependency: 'numberOfItems'
// ...
}
```
You used `numberOfItems` inside the Effect, so the linter asks you to add it as a dependency. However, you *don’t* want the `logVisit` call to be reactive with respect to `numberOfItems`. If the user puts something into the shopping cart, and the `numberOfItems` changes, this *does not mean* that the user visited the page again. In other words, *visiting the page* is, in some sense, an “event”. It happens at a precise moment in time.
Split the code in two parts:
```
function Page({ url }) {
const { items } = useContext(ShoppingCartContext);
const numberOfItems = items.length;
const onVisit = useEffectEvent(visitedUrl => {
logVisit(visitedUrl, numberOfItems);
});
useEffect(() => {
onVisit(url);
}, [url]); // ✅ All dependencies declared
// ...
}
```
Here, `onVisit` is an Effect Event. The code inside it isn’t reactive. This is why you can use `numberOfItems` (or any other reactive value!) without worrying that it will cause the surrounding code to re-execute on changes.
On the other hand, the Effect itself remains reactive. Code inside the Effect uses the `url` prop, so the Effect will re-run after every re-render with a different `url`. This, in turn, will call the `onVisit` Effect Event.
As a result, you will call `logVisit` for every change to the `url`, and always read the latest `numberOfItems`. However, if `numberOfItems` changes on its own, this will not cause any of the code to re-run.
### Note
You might be wondering if you could call `onVisit()` with no arguments, and read the `url` inside it:
```
const onVisit = useEffectEvent(() => {
logVisit(url, numberOfItems);
});
useEffect(() => {
onVisit();
}, [url]);
```
This would work, but it’s better to pass this `url` to the Effect Event explicitly. **By passing `url` as an argument to your Effect Event, you are saying that visiting a page with a different `url` constitutes a separate “event” from the user’s perspective.** The `visitedUrl` is a *part* of the “event” that happened:
```
const onVisit = useEffectEvent(visitedUrl => {
logVisit(visitedUrl, numberOfItems);
});
useEffect(() => {
onVisit(url);
}, [url]);
```
Since your Effect Event explicitly “asks” for the `visitedUrl`, now you can’t accidentally remove `url` from the Effect’s dependencies. If you remove the `url` dependency (causing distinct page visits to be counted as one), the linter will warn you about it. You want `onVisit` to be reactive with regards to the `url`, so instead of reading the `url` inside (where it wouldn’t be reactive), you pass it *from* your Effect.
This becomes especially important if there is some asynchronous logic inside the Effect:
```
const onVisit = useEffectEvent(visitedUrl => {
logVisit(visitedUrl, numberOfItems);
});
useEffect(() => {
setTimeout(() => {
onVisit(url);
}, 5000); // Delay logging visits
}, [url]);
```
Here, `url` inside `onVisit` corresponds to the *latest* `url` (which could have already changed), but `visitedUrl` corresponds to the `url` that originally caused this Effect (and this `onVisit` call) to run.
##### Deep Dive
#### Is it okay to suppress the dependency linter instead?[Link for Is it okay to suppress the dependency linter instead?]()
Show Details
In the existing codebases, you may sometimes see the lint rule suppressed like this:
```
function Page({ url }) {
const { items } = useContext(ShoppingCartContext);
const numberOfItems = items.length;
useEffect(() => {
logVisit(url, numberOfItems);
// 🔴 Avoid suppressing the linter like this:
// eslint-disable-next-line react-hooks/exhaustive-deps
}, [url]);
// ...
}
```
After `useEffectEvent` becomes a stable part of React, we recommend **never suppressing the linter**.
The first downside of suppressing the rule is that React will no longer warn you when your Effect needs to “react” to a new reactive dependency you’ve introduced to your code. In the earlier example, you added `url` to the dependencies *because* React reminded you to do it. You will no longer get such reminders for any future edits to that Effect if you disable the linter. This leads to bugs.
Here is an example of a confusing bug caused by suppressing the linter. In this example, the `handleMove` function is supposed to read the current `canMove` state variable value in order to decide whether the dot should follow the cursor. However, `canMove` is always `true` inside `handleMove`.
Can you see why?
App.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import { useState, useEffect } from 'react';
export default function App() {
const [position, setPosition] = useState({ x: 0, y: 0 });
const [canMove, setCanMove] = useState(true);
function handleMove(e) {
if (canMove) {
setPosition({ x: e.clientX, y: e.clientY });
}
}
useEffect(() => {
window.addEventListener('pointermove', handleMove);
return () => window.removeEventListener('pointermove', handleMove);
// eslint-disable-next-line react-hooks/exhaustive-deps
}, []);
return (
<>
<label>
<input type="checkbox"
checked={canMove}
onChange={e => setCanMove(e.target.checked)}
/>
The dot is allowed to move
</label>
<hr />
<div style={{
position: 'absolute',
backgroundColor: 'pink',
borderRadius: '50%',
opacity: 0.6,
transform: `translate(${position.x}px, ${position.y}px)`,
pointerEvents: 'none',
left: -20,
top: -20,
width: 40,
height: 40,
}} />
</>
);
}
```
Show more
The problem with this code is in suppressing the dependency linter. If you remove the suppression, you’ll see that this Effect should depend on the `handleMove` function. This makes sense: `handleMove` is declared inside the component body, which makes it a reactive value. Every reactive value must be specified as a dependency, or it can potentially get stale over time!
The author of the original code has “lied” to React by saying that the Effect does not depend (`[]`) on any reactive values. This is why React did not re-synchronize the Effect after `canMove` has changed (and `handleMove` with it). Because React did not re-synchronize the Effect, the `handleMove` attached as a listener is the `handleMove` function created during the initial render. During the initial render, `canMove` was `true`, which is why `handleMove` from the initial render will forever see that value.
**If you never suppress the linter, you will never see problems with stale values.**
With `useEffectEvent`, there is no need to “lie” to the linter, and the code works as you would expect:
App.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import { useState, useEffect } from 'react';
import { experimental_useEffectEvent as useEffectEvent } from 'react';
export default function App() {
const [position, setPosition] = useState({ x: 0, y: 0 });
const [canMove, setCanMove] = useState(true);
const onMove = useEffectEvent(e => {
if (canMove) {
setPosition({ x: e.clientX, y: e.clientY });
}
});
useEffect(() => {
window.addEventListener('pointermove', onMove);
return () => window.removeEventListener('pointermove', onMove);
}, []);
return (
<>
<label>
<input type="checkbox"
checked={canMove}
onChange={e => setCanMove(e.target.checked)}
/>
The dot is allowed to move
</label>
<hr />
<div style={{
position: 'absolute',
backgroundColor: 'pink',
borderRadius: '50%',
opacity: 0.6,
transform: `translate(${position.x}px, ${position.y}px)`,
pointerEvents: 'none',
left: -20,
top: -20,
width: 40,
height: 40,
}} />
</>
);
}
```
Show more
This doesn’t mean that `useEffectEvent` is *always* the correct solution. You should only apply it to the lines of code that you don’t want to be reactive. In the above sandbox, you didn’t want the Effect’s code to be reactive with regards to `canMove`. That’s why it made sense to extract an Effect Event.
Read [Removing Effect Dependencies](https://react.dev/learn/removing-effect-dependencies) for other correct alternatives to suppressing the linter.
### Limitations of Effect Events[Link for Limitations of Effect Events]()
### Under Construction
This section describes an **experimental API that has not yet been released** in a stable version of React.
Effect Events are very limited in how you can use them:
- **Only call them from inside Effects.**
- **Never pass them to other components or Hooks.**
For example, don’t declare and pass an Effect Event like this:
```
function Timer() {
const [count, setCount] = useState(0);
const onTick = useEffectEvent(() => {
setCount(count + 1);
});
useTimer(onTick, 1000); // 🔴 Avoid: Passing Effect Events
return <h1>{count}</h1>
}
function useTimer(callback, delay) {
useEffect(() => {
const id = setInterval(() => {
callback();
}, delay);
return () => {
clearInterval(id);
};
}, [delay, callback]); // Need to specify "callback" in dependencies
}
```
Instead, always declare Effect Events directly next to the Effects that use them:
```
function Timer() {
const [count, setCount] = useState(0);
useTimer(() => {
setCount(count + 1);
}, 1000);
return <h1>{count}</h1>
}
function useTimer(callback, delay) {
const onTick = useEffectEvent(() => {
callback();
});
useEffect(() => {
const id = setInterval(() => {
onTick(); // ✅ Good: Only called locally inside an Effect
}, delay);
return () => {
clearInterval(id);
};
}, [delay]); // No need to specify "onTick" (an Effect Event) as a dependency
}
```
Effect Events are non-reactive “pieces” of your Effect code. They should be next to the Effect using them.
## Recap[Link for Recap]()
- Event handlers run in response to specific interactions.
- Effects run whenever synchronization is needed.
- Logic inside event handlers is not reactive.
- Logic inside Effects is reactive.
- You can move non-reactive logic from Effects into Effect Events.
- Only call Effect Events from inside Effects.
- Don’t pass Effect Events to other components or Hooks.
## Try out some challenges[Link for Try out some challenges]()
1\. Fix a variable that doesn’t update 2. Fix a freezing counter 3. Fix a non-adjustable delay 4. Fix a delayed notification
#### Challenge 1 of 4: Fix a variable that doesn’t update[Link for this heading]()
This `Timer` component keeps a `count` state variable which increases every second. The value by which it’s increasing is stored in the `increment` state variable. You can control the `increment` variable with the plus and minus buttons.
However, no matter how many times you click the plus button, the counter is still incremented by one every second. What’s wrong with this code? Why is `increment` always equal to `1` inside the Effect’s code? Find the mistake and fix it.
App.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import { useState, useEffect } from 'react';
export default function Timer() {
const [count, setCount] = useState(0);
const [increment, setIncrement] = useState(1);
useEffect(() => {
const id = setInterval(() => {
setCount(c => c + increment);
}, 1000);
return () => {
clearInterval(id);
};
// eslint-disable-next-line react-hooks/exhaustive-deps
}, []);
return (
<>
<h1>
Counter: {count}
<button onClick={() => setCount(0)}>Reset</button>
</h1>
<hr />
<p>
Every second, increment by:
<button disabled={increment === 0} onClick={() => {
setIncrement(i => i - 1);
}}>–</button>
<b>{increment}</b>
<button onClick={() => {
setIncrement(i => i + 1);
}}>+</button>
</p>
</>
);
}
```
Show more
Show hint Show solution
Next Challenge
[PreviousLifecycle of Reactive Effects](https://react.dev/learn/lifecycle-of-reactive-effects)
[NextRemoving Effect Dependencies](https://react.dev/learn/removing-effect-dependencies) |
https://react.dev/learn/synchronizing-with-effects | [Learn React](https://react.dev/learn)
[Escape Hatches](https://react.dev/learn/escape-hatches)
# Synchronizing with Effects[Link for this heading]()
Some components need to synchronize with external systems. For example, you might want to control a non-React component based on the React state, set up a server connection, or send an analytics log when a component appears on the screen. *Effects* let you run some code after rendering so that you can synchronize your component with some system outside of React.
### You will learn
- What Effects are
- How Effects are different from events
- How to declare an Effect in your component
- How to skip re-running an Effect unnecessarily
- Why Effects run twice in development and how to fix them
## What are Effects and how are they different from events?[Link for What are Effects and how are they different from events?]()
Before getting to Effects, you need to be familiar with two types of logic inside React components:
- **Rendering code** (introduced in [Describing the UI](https://react.dev/learn/describing-the-ui)) lives at the top level of your component. This is where you take the props and state, transform them, and return the JSX you want to see on the screen. [Rendering code must be pure.](https://react.dev/learn/keeping-components-pure) Like a math formula, it should only *calculate* the result, but not do anything else.
- **Event handlers** (introduced in [Adding Interactivity](https://react.dev/learn/adding-interactivity)) are nested functions inside your components that *do* things rather than just calculate them. An event handler might update an input field, submit an HTTP POST request to buy a product, or navigate the user to another screen. Event handlers contain [“side effects”](https://en.wikipedia.org/wiki/Side_effect_%28computer_science%29) (they change the program’s state) caused by a specific user action (for example, a button click or typing).
Sometimes this isn’t enough. Consider a `ChatRoom` component that must connect to the chat server whenever it’s visible on the screen. Connecting to a server is not a pure calculation (it’s a side effect) so it can’t happen during rendering. However, there is no single particular event like a click that causes `ChatRoom` to be displayed.
***Effects* let you specify side effects that are caused by rendering itself, rather than by a particular event.** Sending a message in the chat is an *event* because it is directly caused by the user clicking a specific button. However, setting up a server connection is an *Effect* because it should happen no matter which interaction caused the component to appear. Effects run at the end of a [commit](https://react.dev/learn/render-and-commit) after the screen updates. This is a good time to synchronize the React components with some external system (like network or a third-party library).
### Note
Here and later in this text, capitalized “Effect” refers to the React-specific definition above, i.e. a side effect caused by rendering. To refer to the broader programming concept, we’ll say “side effect”.
## You might not need an Effect[Link for You might not need an Effect]()
**Don’t rush to add Effects to your components.** Keep in mind that Effects are typically used to “step out” of your React code and synchronize with some *external* system. This includes browser APIs, third-party widgets, network, and so on. If your Effect only adjusts some state based on other state, [you might not need an Effect.](https://react.dev/learn/you-might-not-need-an-effect)
## How to write an Effect[Link for How to write an Effect]()
To write an Effect, follow these three steps:
1. **Declare an Effect.** By default, your Effect will run after every [commit](https://react.dev/learn/render-and-commit).
2. **Specify the Effect dependencies.** Most Effects should only re-run *when needed* rather than after every render. For example, a fade-in animation should only trigger when a component appears. Connecting and disconnecting to a chat room should only happen when the component appears and disappears, or when the chat room changes. You will learn how to control this by specifying *dependencies.*
3. **Add cleanup if needed.** Some Effects need to specify how to stop, undo, or clean up whatever they were doing. For example, “connect” needs “disconnect”, “subscribe” needs “unsubscribe”, and “fetch” needs either “cancel” or “ignore”. You will learn how to do this by returning a *cleanup function*.
Let’s look at each of these steps in detail.
### Step 1: Declare an Effect[Link for Step 1: Declare an Effect]()
To declare an Effect in your component, import the [`useEffect` Hook](https://react.dev/reference/react/useEffect) from React:
```
import { useEffect } from 'react';
```
Then, call it at the top level of your component and put some code inside your Effect:
```
function MyComponent() {
useEffect(() => {
// Code here will run after *every* render
});
return <div />;
}
```
Every time your component renders, React will update the screen *and then* run the code inside `useEffect`. In other words, **`useEffect` “delays” a piece of code from running until that render is reflected on the screen.**
Let’s see how you can use an Effect to synchronize with an external system. Consider a `<VideoPlayer>` React component. It would be nice to control whether it’s playing or paused by passing an `isPlaying` prop to it:
```
<VideoPlayer isPlaying={isPlaying} />;
```
Your custom `VideoPlayer` component renders the built-in browser [`<video>`](https://developer.mozilla.org/en-US/docs/Web/HTML/Element/video) tag:
```
function VideoPlayer({ src, isPlaying }) {
// TODO: do something with isPlaying
return <video src={src} />;
}
```
However, the browser `<video>` tag does not have an `isPlaying` prop. The only way to control it is to manually call the [`play()`](https://developer.mozilla.org/en-US/docs/Web/API/HTMLMediaElement/play) and [`pause()`](https://developer.mozilla.org/en-US/docs/Web/API/HTMLMediaElement/pause) methods on the DOM element. **You need to synchronize the value of `isPlaying` prop, which tells whether the video *should* currently be playing, with calls like `play()` and `pause()`.**
We’ll need to first [get a ref](https://react.dev/learn/manipulating-the-dom-with-refs) to the `<video>` DOM node.
You might be tempted to try to call `play()` or `pause()` during rendering, but that isn’t correct:
App.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import { useState, useRef, useEffect } from 'react';
function VideoPlayer({ src, isPlaying }) {
const ref = useRef(null);
if (isPlaying) {
ref.current.play(); // Calling these while rendering isn't allowed.
} else {
ref.current.pause(); // Also, this crashes.
}
return <video ref={ref} src={src} loop playsInline />;
}
export default function App() {
const [isPlaying, setIsPlaying] = useState(false);
return (
<>
<button onClick={() => setIsPlaying(!isPlaying)}>
{isPlaying ? 'Pause' : 'Play'}
</button>
<VideoPlayer
isPlaying={isPlaying}
src="https://interactive-examples.mdn.mozilla.net/media/cc0-videos/flower.mp4"
/>
</>
);
}
```
Show more
The reason this code isn’t correct is that it tries to do something with the DOM node during rendering. In React, [rendering should be a pure calculation](https://react.dev/learn/keeping-components-pure) of JSX and should not contain side effects like modifying the DOM.
Moreover, when `VideoPlayer` is called for the first time, its DOM does not exist yet! There isn’t a DOM node yet to call `play()` or `pause()` on, because React doesn’t know what DOM to create until you return the JSX.
The solution here is to **wrap the side effect with `useEffect` to move it out of the rendering calculation:**
```
import { useEffect, useRef } from 'react';
function VideoPlayer({ src, isPlaying }) {
const ref = useRef(null);
useEffect(() => {
if (isPlaying) {
ref.current.play();
} else {
ref.current.pause();
}
});
return <video ref={ref} src={src} loop playsInline />;
}
```
By wrapping the DOM update in an Effect, you let React update the screen first. Then your Effect runs.
When your `VideoPlayer` component renders (either the first time or if it re-renders), a few things will happen. First, React will update the screen, ensuring the `<video>` tag is in the DOM with the right props. Then React will run your Effect. Finally, your Effect will call `play()` or `pause()` depending on the value of `isPlaying`.
Press Play/Pause multiple times and see how the video player stays synchronized to the `isPlaying` value:
App.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import { useState, useRef, useEffect } from 'react';
function VideoPlayer({ src, isPlaying }) {
const ref = useRef(null);
useEffect(() => {
if (isPlaying) {
ref.current.play();
} else {
ref.current.pause();
}
});
return <video ref={ref} src={src} loop playsInline />;
}
export default function App() {
const [isPlaying, setIsPlaying] = useState(false);
return (
<>
<button onClick={() => setIsPlaying(!isPlaying)}>
{isPlaying ? 'Pause' : 'Play'}
</button>
<VideoPlayer
isPlaying={isPlaying}
src="https://interactive-examples.mdn.mozilla.net/media/cc0-videos/flower.mp4"
/>
</>
);
}
```
Show more
In this example, the “external system” you synchronized to React state was the browser media API. You can use a similar approach to wrap legacy non-React code (like jQuery plugins) into declarative React components.
Note that controlling a video player is much more complex in practice. Calling `play()` may fail, the user might play or pause using the built-in browser controls, and so on. This example is very simplified and incomplete.
### Pitfall
By default, Effects run after *every* render. This is why code like this will **produce an infinite loop:**
```
const [count, setCount] = useState(0);
useEffect(() => {
setCount(count + 1);
});
```
Effects run as a *result* of rendering. Setting state *triggers* rendering. Setting state immediately in an Effect is like plugging a power outlet into itself. The Effect runs, it sets the state, which causes a re-render, which causes the Effect to run, it sets the state again, this causes another re-render, and so on.
Effects should usually synchronize your components with an *external* system. If there’s no external system and you only want to adjust some state based on other state, [you might not need an Effect.](https://react.dev/learn/you-might-not-need-an-effect)
### Step 2: Specify the Effect dependencies[Link for Step 2: Specify the Effect dependencies]()
By default, Effects run after *every* render. Often, this is **not what you want:**
- Sometimes, it’s slow. Synchronizing with an external system is not always instant, so you might want to skip doing it unless it’s necessary. For example, you don’t want to reconnect to the chat server on every keystroke.
- Sometimes, it’s wrong. For example, you don’t want to trigger a component fade-in animation on every keystroke. The animation should only play once when the component appears for the first time.
To demonstrate the issue, here is the previous example with a few `console.log` calls and a text input that updates the parent component’s state. Notice how typing causes the Effect to re-run:
App.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import { useState, useRef, useEffect } from 'react';
function VideoPlayer({ src, isPlaying }) {
const ref = useRef(null);
useEffect(() => {
if (isPlaying) {
console.log('Calling video.play()');
ref.current.play();
} else {
console.log('Calling video.pause()');
ref.current.pause();
}
});
return <video ref={ref} src={src} loop playsInline />;
}
export default function App() {
const [isPlaying, setIsPlaying] = useState(false);
const [text, setText] = useState('');
return (
<>
<input value={text} onChange={e => setText(e.target.value)} />
<button onClick={() => setIsPlaying(!isPlaying)}>
{isPlaying ? 'Pause' : 'Play'}
</button>
<VideoPlayer
isPlaying={isPlaying}
src="https://interactive-examples.mdn.mozilla.net/media/cc0-videos/flower.mp4"
/>
</>
);
}
```
Show more
You can tell React to **skip unnecessarily re-running the Effect** by specifying an array of *dependencies* as the second argument to the `useEffect` call. Start by adding an empty `[]` array to the above example on line 14:
```
useEffect(() => {
// ...
}, []);
```
You should see an error saying `React Hook useEffect has a missing dependency: 'isPlaying'`:
App.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import { useState, useRef, useEffect } from 'react';
function VideoPlayer({ src, isPlaying }) {
const ref = useRef(null);
useEffect(() => {
if (isPlaying) {
console.log('Calling video.play()');
ref.current.play();
} else {
console.log('Calling video.pause()');
ref.current.pause();
}
}, []); // This causes an error
return <video ref={ref} src={src} loop playsInline />;
}
export default function App() {
const [isPlaying, setIsPlaying] = useState(false);
const [text, setText] = useState('');
return (
<>
<input value={text} onChange={e => setText(e.target.value)} />
<button onClick={() => setIsPlaying(!isPlaying)}>
{isPlaying ? 'Pause' : 'Play'}
</button>
<VideoPlayer
isPlaying={isPlaying}
src="https://interactive-examples.mdn.mozilla.net/media/cc0-videos/flower.mp4"
/>
</>
);
}
```
Show more
The problem is that the code inside of your Effect *depends on* the `isPlaying` prop to decide what to do, but this dependency was not explicitly declared. To fix this issue, add `isPlaying` to the dependency array:
```
useEffect(() => {
if (isPlaying) { // It's used here...
// ...
} else {
// ...
}
}, [isPlaying]); // ...so it must be declared here!
```
Now all dependencies are declared, so there is no error. Specifying `[isPlaying]` as the dependency array tells React that it should skip re-running your Effect if `isPlaying` is the same as it was during the previous render. With this change, typing into the input doesn’t cause the Effect to re-run, but pressing Play/Pause does:
App.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import { useState, useRef, useEffect } from 'react';
function VideoPlayer({ src, isPlaying }) {
const ref = useRef(null);
useEffect(() => {
if (isPlaying) {
console.log('Calling video.play()');
ref.current.play();
} else {
console.log('Calling video.pause()');
ref.current.pause();
}
}, [isPlaying]);
return <video ref={ref} src={src} loop playsInline />;
}
export default function App() {
const [isPlaying, setIsPlaying] = useState(false);
const [text, setText] = useState('');
return (
<>
<input value={text} onChange={e => setText(e.target.value)} />
<button onClick={() => setIsPlaying(!isPlaying)}>
{isPlaying ? 'Pause' : 'Play'}
</button>
<VideoPlayer
isPlaying={isPlaying}
src="https://interactive-examples.mdn.mozilla.net/media/cc0-videos/flower.mp4"
/>
</>
);
}
```
Show more
The dependency array can contain multiple dependencies. React will only skip re-running the Effect if *all* of the dependencies you specify have exactly the same values as they had during the previous render. React compares the dependency values using the [`Object.is`](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Object/is) comparison. See the [`useEffect` reference](https://react.dev/reference/react/useEffect) for details.
**Notice that you can’t “choose” your dependencies.** You will get a lint error if the dependencies you specified don’t match what React expects based on the code inside your Effect. This helps catch many bugs in your code. If you don’t want some code to re-run, [*edit the Effect code itself* to not “need” that dependency.](https://react.dev/learn/lifecycle-of-reactive-effects)
### Pitfall
The behaviors without the dependency array and with an *empty* `[]` dependency array are different:
```
useEffect(() => {
// This runs after every render
});
useEffect(() => {
// This runs only on mount (when the component appears)
}, []);
useEffect(() => {
// This runs on mount *and also* if either a or b have changed since the last render
}, [a, b]);
```
We’ll take a close look at what “mount” means in the next step.
##### Deep Dive
#### Why was the ref omitted from the dependency array?[Link for Why was the ref omitted from the dependency array?]()
Show Details
This Effect uses *both* `ref` and `isPlaying`, but only `isPlaying` is declared as a dependency:
```
function VideoPlayer({ src, isPlaying }) {
const ref = useRef(null);
useEffect(() => {
if (isPlaying) {
ref.current.play();
} else {
ref.current.pause();
}
}, [isPlaying]);
```
This is because the `ref` object has a *stable identity:* React guarantees [you’ll always get the same object](https://react.dev/reference/react/useRef) from the same `useRef` call on every render. It never changes, so it will never by itself cause the Effect to re-run. Therefore, it does not matter whether you include it or not. Including it is fine too:
```
function VideoPlayer({ src, isPlaying }) {
const ref = useRef(null);
useEffect(() => {
if (isPlaying) {
ref.current.play();
} else {
ref.current.pause();
}
}, [isPlaying, ref]);
```
The [`set` functions](https://react.dev/reference/react/useState) returned by `useState` also have stable identity, so you will often see them omitted from the dependencies too. If the linter lets you omit a dependency without errors, it is safe to do.
Omitting always-stable dependencies only works when the linter can “see” that the object is stable. For example, if `ref` was passed from a parent component, you would have to specify it in the dependency array. However, this is good because you can’t know whether the parent component always passes the same ref, or passes one of several refs conditionally. So your Effect *would* depend on which ref is passed.
### Step 3: Add cleanup if needed[Link for Step 3: Add cleanup if needed]()
Consider a different example. You’re writing a `ChatRoom` component that needs to connect to the chat server when it appears. You are given a `createConnection()` API that returns an object with `connect()` and `disconnect()` methods. How do you keep the component connected while it is displayed to the user?
Start by writing the Effect logic:
```
useEffect(() => {
const connection = createConnection();
connection.connect();
});
```
It would be slow to connect to the chat after every re-render, so you add the dependency array:
```
useEffect(() => {
const connection = createConnection();
connection.connect();
}, []);
```
**The code inside the Effect does not use any props or state, so your dependency array is `[]` (empty). This tells React to only run this code when the component “mounts”, i.e. appears on the screen for the first time.**
Let’s try running this code:
App.jschat.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import { useEffect } from 'react';
import { createConnection } from './chat.js';
export default function ChatRoom() {
useEffect(() => {
const connection = createConnection();
connection.connect();
}, []);
return <h1>Welcome to the chat!</h1>;
}
```
This Effect only runs on mount, so you might expect `"✅ Connecting..."` to be printed once in the console. **However, if you check the console, `"✅ Connecting..."` gets printed twice. Why does it happen?**
Imagine the `ChatRoom` component is a part of a larger app with many different screens. The user starts their journey on the `ChatRoom` page. The component mounts and calls `connection.connect()`. Then imagine the user navigates to another screen—for example, to the Settings page. The `ChatRoom` component unmounts. Finally, the user clicks Back and `ChatRoom` mounts again. This would set up a second connection—but the first connection was never destroyed! As the user navigates across the app, the connections would keep piling up.
Bugs like this are easy to miss without extensive manual testing. To help you spot them quickly, in development React remounts every component once immediately after its initial mount.
Seeing the `"✅ Connecting..."` log twice helps you notice the real issue: your code doesn’t close the connection when the component unmounts.
To fix the issue, return a *cleanup function* from your Effect:
```
useEffect(() => {
const connection = createConnection();
connection.connect();
return () => {
connection.disconnect();
};
}, []);
```
React will call your cleanup function each time before the Effect runs again, and one final time when the component unmounts (gets removed). Let’s see what happens when the cleanup function is implemented:
App.jschat.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import { useState, useEffect } from 'react';
import { createConnection } from './chat.js';
export default function ChatRoom() {
useEffect(() => {
const connection = createConnection();
connection.connect();
return () => connection.disconnect();
}, []);
return <h1>Welcome to the chat!</h1>;
}
```
Now you get three console logs in development:
1. `"✅ Connecting..."`
2. `"❌ Disconnected."`
3. `"✅ Connecting..."`
**This is the correct behavior in development.** By remounting your component, React verifies that navigating away and back would not break your code. Disconnecting and then connecting again is exactly what should happen! When you implement the cleanup well, there should be no user-visible difference between running the Effect once vs running it, cleaning it up, and running it again. There’s an extra connect/disconnect call pair because React is probing your code for bugs in development. This is normal—don’t try to make it go away!
**In production, you would only see `"✅ Connecting..."` printed once.** Remounting components only happens in development to help you find Effects that need cleanup. You can turn off [Strict Mode](https://react.dev/reference/react/StrictMode) to opt out of the development behavior, but we recommend keeping it on. This lets you find many bugs like the one above.
## How to handle the Effect firing twice in development?[Link for How to handle the Effect firing twice in development?]()
React intentionally remounts your components in development to find bugs like in the last example. **The right question isn’t “how to run an Effect once”, but “how to fix my Effect so that it works after remounting”.**
Usually, the answer is to implement the cleanup function. The cleanup function should stop or undo whatever the Effect was doing. The rule of thumb is that the user shouldn’t be able to distinguish between the Effect running once (as in production) and a *setup → cleanup → setup* sequence (as you’d see in development).
Most of the Effects you’ll write will fit into one of the common patterns below.
### Pitfall
#### Don’t use refs to prevent Effects from firing[Link for Don’t use refs to prevent Effects from firing]()
A common pitfall for preventing Effects firing twice in development is to use a `ref` to prevent the Effect from running more than once. For example, you could “fix” the above bug with a `useRef`:
```
const connectionRef = useRef(null);
useEffect(() => {
// 🚩 This wont fix the bug!!!
if (!connectionRef.current) {
connectionRef.current = createConnection();
connectionRef.current.connect();
}
}, []);
```
This makes it so you only see `"✅ Connecting..."` once in development, but it doesn’t fix the bug.
When the user navigates away, the connection still isn’t closed and when they navigate back, a new connection is created. As the user navigates across the app, the connections would keep piling up, the same as it would before the “fix”.
To fix the bug, it is not enough to just make the Effect run once. The effect needs to work after re-mounting, which means the connection needs to be cleaned up like in the solution above.
See the examples below for how to handle common patterns.
### Controlling non-React widgets[Link for Controlling non-React widgets]()
Sometimes you need to add UI widgets that aren’t written in React. For example, let’s say you’re adding a map component to your page. It has a `setZoomLevel()` method, and you’d like to keep the zoom level in sync with a `zoomLevel` state variable in your React code. Your Effect would look similar to this:
```
useEffect(() => {
const map = mapRef.current;
map.setZoomLevel(zoomLevel);
}, [zoomLevel]);
```
Note that there is no cleanup needed in this case. In development, React will call the Effect twice, but this is not a problem because calling `setZoomLevel` twice with the same value does not do anything. It may be slightly slower, but this doesn’t matter because it won’t remount needlessly in production.
Some APIs may not allow you to call them twice in a row. For example, the [`showModal`](https://developer.mozilla.org/en-US/docs/Web/API/HTMLDialogElement/showModal) method of the built-in [`<dialog>`](https://developer.mozilla.org/en-US/docs/Web/API/HTMLDialogElement) element throws if you call it twice. Implement the cleanup function and make it close the dialog:
```
useEffect(() => {
const dialog = dialogRef.current;
dialog.showModal();
return () => dialog.close();
}, []);
```
In development, your Effect will call `showModal()`, then immediately `close()`, and then `showModal()` again. This has the same user-visible behavior as calling `showModal()` once, as you would see in production.
### Subscribing to events[Link for Subscribing to events]()
If your Effect subscribes to something, the cleanup function should unsubscribe:
```
useEffect(() => {
function handleScroll(e) {
console.log(window.scrollX, window.scrollY);
}
window.addEventListener('scroll', handleScroll);
return () => window.removeEventListener('scroll', handleScroll);
}, []);
```
In development, your Effect will call `addEventListener()`, then immediately `removeEventListener()`, and then `addEventListener()` again with the same handler. So there would be only one active subscription at a time. This has the same user-visible behavior as calling `addEventListener()` once, as in production.
### Triggering animations[Link for Triggering animations]()
If your Effect animates something in, the cleanup function should reset the animation to the initial values:
```
useEffect(() => {
const node = ref.current;
node.style.opacity = 1; // Trigger the animation
return () => {
node.style.opacity = 0; // Reset to the initial value
};
}, []);
```
In development, opacity will be set to `1`, then to `0`, and then to `1` again. This should have the same user-visible behavior as setting it to `1` directly, which is what would happen in production. If you use a third-party animation library with support for tweening, your cleanup function should reset the timeline to its initial state.
### Fetching data[Link for Fetching data]()
If your Effect fetches something, the cleanup function should either [abort the fetch](https://developer.mozilla.org/en-US/docs/Web/API/AbortController) or ignore its result:
```
useEffect(() => {
let ignore = false;
async function startFetching() {
const json = await fetchTodos(userId);
if (!ignore) {
setTodos(json);
}
}
startFetching();
return () => {
ignore = true;
};
}, [userId]);
```
You can’t “undo” a network request that already happened, but your cleanup function should ensure that the fetch that’s *not relevant anymore* does not keep affecting your application. If the `userId` changes from `'Alice'` to `'Bob'`, cleanup ensures that the `'Alice'` response is ignored even if it arrives after `'Bob'`.
**In development, you will see two fetches in the Network tab.** There is nothing wrong with that. With the approach above, the first Effect will immediately get cleaned up so its copy of the `ignore` variable will be set to `true`. So even though there is an extra request, it won’t affect the state thanks to the `if (!ignore)` check.
**In production, there will only be one request.** If the second request in development is bothering you, the best approach is to use a solution that deduplicates requests and caches their responses between components:
```
function TodoList() {
const todos = useSomeDataLibrary(`/api/user/${userId}/todos`);
// ...
```
This will not only improve the development experience, but also make your application feel faster. For example, the user pressing the Back button won’t have to wait for some data to load again because it will be cached. You can either build such a cache yourself or use one of the many alternatives to manual fetching in Effects.
##### Deep Dive
#### What are good alternatives to data fetching in Effects?[Link for What are good alternatives to data fetching in Effects?]()
Show Details
Writing `fetch` calls inside Effects is a [popular way to fetch data](https://www.robinwieruch.de/react-hooks-fetch-data/), especially in fully client-side apps. This is, however, a very manual approach and it has significant downsides:
- **Effects don’t run on the server.** This means that the initial server-rendered HTML will only include a loading state with no data. The client computer will have to download all JavaScript and render your app only to discover that now it needs to load the data. This is not very efficient.
- **Fetching directly in Effects makes it easy to create “network waterfalls”.** You render the parent component, it fetches some data, renders the child components, and then they start fetching their data. If the network is not very fast, this is significantly slower than fetching all data in parallel.
- **Fetching directly in Effects usually means you don’t preload or cache data.** For example, if the component unmounts and then mounts again, it would have to fetch the data again.
- **It’s not very ergonomic.** There’s quite a bit of boilerplate code involved when writing `fetch` calls in a way that doesn’t suffer from bugs like [race conditions.](https://maxrozen.com/race-conditions-fetching-data-react-with-useeffect)
This list of downsides is not specific to React. It applies to fetching data on mount with any library. Like with routing, data fetching is not trivial to do well, so we recommend the following approaches:
- **If you use a [framework](https://react.dev/learn/start-a-new-react-project), use its built-in data fetching mechanism.** Modern React frameworks have integrated data fetching mechanisms that are efficient and don’t suffer from the above pitfalls.
- **Otherwise, consider using or building a client-side cache.** Popular open source solutions include [React Query](https://tanstack.com/query/latest), [useSWR](https://swr.vercel.app/), and [React Router 6.4+.](https://beta.reactrouter.com/en/main/start/overview) You can build your own solution too, in which case you would use Effects under the hood, but add logic for deduplicating requests, caching responses, and avoiding network waterfalls (by preloading data or hoisting data requirements to routes).
You can continue fetching data directly in Effects if neither of these approaches suit you.
### Sending analytics[Link for Sending analytics]()
Consider this code that sends an analytics event on the page visit:
```
useEffect(() => {
logVisit(url); // Sends a POST request
}, [url]);
```
In development, `logVisit` will be called twice for every URL, so you might be tempted to try to fix that. **We recommend keeping this code as is.** Like with earlier examples, there is no *user-visible* behavior difference between running it once and running it twice. From a practical point of view, `logVisit` should not do anything in development because you don’t want the logs from the development machines to skew the production metrics. Your component remounts every time you save its file, so it logs extra visits in development anyway.
**In production, there will be no duplicate visit logs.**
To debug the analytics events you’re sending, you can deploy your app to a staging environment (which runs in production mode) or temporarily opt out of [Strict Mode](https://react.dev/reference/react/StrictMode) and its development-only remounting checks. You may also send analytics from the route change event handlers instead of Effects. For more precise analytics, [intersection observers](https://developer.mozilla.org/en-US/docs/Web/API/Intersection_Observer_API) can help track which components are in the viewport and how long they remain visible.
### Not an Effect: Initializing the application[Link for Not an Effect: Initializing the application]()
Some logic should only run once when the application starts. You can put it outside your components:
```
if (typeof window !== 'undefined') { // Check if we're running in the browser.
checkAuthToken();
loadDataFromLocalStorage();
}
function App() {
// ...
}
```
This guarantees that such logic only runs once after the browser loads the page.
### Not an Effect: Buying a product[Link for Not an Effect: Buying a product]()
Sometimes, even if you write a cleanup function, there’s no way to prevent user-visible consequences of running the Effect twice. For example, maybe your Effect sends a POST request like buying a product:
```
useEffect(() => {
// 🔴 Wrong: This Effect fires twice in development, exposing a problem in the code.
fetch('/api/buy', { method: 'POST' });
}, []);
```
You wouldn’t want to buy the product twice. However, this is also why you shouldn’t put this logic in an Effect. What if the user goes to another page and then presses Back? Your Effect would run again. You don’t want to buy the product when the user *visits* a page; you want to buy it when the user *clicks* the Buy button.
Buying is not caused by rendering; it’s caused by a specific interaction. It should run only when the user presses the button. **Delete the Effect and move your `/api/buy` request into the Buy button event handler:**
```
function handleClick() {
// ✅ Buying is an event because it is caused by a particular interaction.
fetch('/api/buy', { method: 'POST' });
}
```
**This illustrates that if remounting breaks the logic of your application, this usually uncovers existing bugs.** From a user’s perspective, visiting a page shouldn’t be different from visiting it, clicking a link, then pressing Back to view the page again. React verifies that your components abide by this principle by remounting them once in development.
## Putting it all together[Link for Putting it all together]()
This playground can help you “get a feel” for how Effects work in practice.
This example uses [`setTimeout`](https://developer.mozilla.org/en-US/docs/Web/API/setTimeout) to schedule a console log with the input text to appear three seconds after the Effect runs. The cleanup function cancels the pending timeout. Start by pressing “Mount the component”:
App.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import { useState, useEffect } from 'react';
function Playground() {
const [text, setText] = useState('a');
useEffect(() => {
function onTimeout() {
console.log('⏰ ' + text);
}
console.log('🔵 Schedule "' + text + '" log');
const timeoutId = setTimeout(onTimeout, 3000);
return () => {
console.log('🟡 Cancel "' + text + '" log');
clearTimeout(timeoutId);
};
}, [text]);
return (
<>
<label>
What to log:{' '}
<input
value={text}
onChange={e => setText(e.target.value)}
/>
</label>
<h1>{text}</h1>
</>
);
}
export default function App() {
const [show, setShow] = useState(false);
return (
<>
<button onClick={() => setShow(!show)}>
{show ? 'Unmount' : 'Mount'} the component
</button>
{show && <hr />}
{show && <Playground />}
</>
);
}
```
Show more
You will see three logs at first: `Schedule "a" log`, `Cancel "a" log`, and `Schedule "a" log` again. Three second later there will also be a log saying `a`. As you learned earlier, the extra schedule/cancel pair is because React remounts the component once in development to verify that you’ve implemented cleanup well.
Now edit the input to say `abc`. If you do it fast enough, you’ll see `Schedule "ab" log` immediately followed by `Cancel "ab" log` and `Schedule "abc" log`. **React always cleans up the previous render’s Effect before the next render’s Effect.** This is why even if you type into the input fast, there is at most one timeout scheduled at a time. Edit the input a few times and watch the console to get a feel for how Effects get cleaned up.
Type something into the input and then immediately press “Unmount the component”. Notice how unmounting cleans up the last render’s Effect. Here, it clears the last timeout before it has a chance to fire.
Finally, edit the component above and comment out the cleanup function so that the timeouts don’t get cancelled. Try typing `abcde` fast. What do you expect to happen in three seconds? Will `console.log(text)` inside the timeout print the *latest* `text` and produce five `abcde` logs? Give it a try to check your intuition!
Three seconds later, you should see a sequence of logs (`a`, `ab`, `abc`, `abcd`, and `abcde`) rather than five `abcde` logs. **Each Effect “captures” the `text` value from its corresponding render.** It doesn’t matter that the `text` state changed: an Effect from the render with `text = 'ab'` will always see `'ab'`. In other words, Effects from each render are isolated from each other. If you’re curious how this works, you can read about [closures](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Closures).
##### Deep Dive
#### Each render has its own Effects[Link for Each render has its own Effects]()
Show Details
You can think of `useEffect` as “attaching” a piece of behavior to the render output. Consider this Effect:
```
export default function ChatRoom({ roomId }) {
useEffect(() => {
const connection = createConnection(roomId);
connection.connect();
return () => connection.disconnect();
}, [roomId]);
return <h1>Welcome to {roomId}!</h1>;
}
```
Let’s see what exactly happens as the user navigates around the app.
#### Initial render[Link for Initial render]()
The user visits `<ChatRoom roomId="general" />`. Let’s [mentally substitute](https://react.dev/learn/state-as-a-snapshot) `roomId` with `'general'`:
```
// JSX for the first render (roomId = "general")
return <h1>Welcome to general!</h1>;
```
**The Effect is *also* a part of the rendering output.** The first render’s Effect becomes:
```
// Effect for the first render (roomId = "general")
() => {
const connection = createConnection('general');
connection.connect();
return () => connection.disconnect();
},
// Dependencies for the first render (roomId = "general")
['general']
```
React runs this Effect, which connects to the `'general'` chat room.
#### Re-render with same dependencies[Link for Re-render with same dependencies]()
Let’s say `<ChatRoom roomId="general" />` re-renders. The JSX output is the same:
```
// JSX for the second render (roomId = "general")
return <h1>Welcome to general!</h1>;
```
React sees that the rendering output has not changed, so it doesn’t update the DOM.
The Effect from the second render looks like this:
```
// Effect for the second render (roomId = "general")
() => {
const connection = createConnection('general');
connection.connect();
return () => connection.disconnect();
},
// Dependencies for the second render (roomId = "general")
['general']
```
React compares `['general']` from the second render with `['general']` from the first render. **Because all dependencies are the same, React *ignores* the Effect from the second render.** It never gets called.
#### Re-render with different dependencies[Link for Re-render with different dependencies]()
Then, the user visits `<ChatRoom roomId="travel" />`. This time, the component returns different JSX:
```
// JSX for the third render (roomId = "travel")
return <h1>Welcome to travel!</h1>;
```
React updates the DOM to change `"Welcome to general"` into `"Welcome to travel"`.
The Effect from the third render looks like this:
```
// Effect for the third render (roomId = "travel")
() => {
const connection = createConnection('travel');
connection.connect();
return () => connection.disconnect();
},
// Dependencies for the third render (roomId = "travel")
['travel']
```
React compares `['travel']` from the third render with `['general']` from the second render. One dependency is different: `Object.is('travel', 'general')` is `false`. The Effect can’t be skipped.
**Before React can apply the Effect from the third render, it needs to clean up the last Effect that *did* run.** The second render’s Effect was skipped, so React needs to clean up the first render’s Effect. If you scroll up to the first render, you’ll see that its cleanup calls `disconnect()` on the connection that was created with `createConnection('general')`. This disconnects the app from the `'general'` chat room.
After that, React runs the third render’s Effect. It connects to the `'travel'` chat room.
#### Unmount[Link for Unmount]()
Finally, let’s say the user navigates away, and the `ChatRoom` component unmounts. React runs the last Effect’s cleanup function. The last Effect was from the third render. The third render’s cleanup destroys the `createConnection('travel')` connection. So the app disconnects from the `'travel'` room.
#### Development-only behaviors[Link for Development-only behaviors]()
When [Strict Mode](https://react.dev/reference/react/StrictMode) is on, React remounts every component once after mount (state and DOM are preserved). This [helps you find Effects that need cleanup]() and exposes bugs like race conditions early. Additionally, React will remount the Effects whenever you save a file in development. Both of these behaviors are development-only.
## Recap[Link for Recap]()
- Unlike events, Effects are caused by rendering itself rather than a particular interaction.
- Effects let you synchronize a component with some external system (third-party API, network, etc).
- By default, Effects run after every render (including the initial one).
- React will skip the Effect if all of its dependencies have the same values as during the last render.
- You can’t “choose” your dependencies. They are determined by the code inside the Effect.
- Empty dependency array (`[]`) corresponds to the component “mounting”, i.e. being added to the screen.
- In Strict Mode, React mounts components twice (in development only!) to stress-test your Effects.
- If your Effect breaks because of remounting, you need to implement a cleanup function.
- React will call your cleanup function before the Effect runs next time, and during the unmount.
## Try out some challenges[Link for Try out some challenges]()
1\. Focus a field on mount 2. Focus a field conditionally 3. Fix an interval that fires twice 4. Fix fetching inside an Effect
#### Challenge 1 of 4: Focus a field on mount[Link for this heading]()
In this example, the form renders a `<MyInput />` component.
Use the input’s [`focus()`](https://developer.mozilla.org/en-US/docs/Web/API/HTMLElement/focus) method to make `MyInput` automatically focus when it appears on the screen. There is already a commented out implementation, but it doesn’t quite work. Figure out why it doesn’t work, and fix it. (If you’re familiar with the `autoFocus` attribute, pretend that it does not exist: we are reimplementing the same functionality from scratch.)
MyInput.js
MyInput.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import { useEffect, useRef } from 'react';
export default function MyInput({ value, onChange }) {
const ref = useRef(null);
// TODO: This doesn't quite work. Fix it.
// ref.current.focus()
return (
<input
ref={ref}
value={value}
onChange={onChange}
/>
);
}
```
Show more
To verify that your solution works, press “Show form” and verify that the input receives focus (becomes highlighted and the cursor is placed inside). Press “Hide form” and “Show form” again. Verify the input is highlighted again.
`MyInput` should only focus *on mount* rather than after every render. To verify that the behavior is right, press “Show form” and then repeatedly press the “Make it uppercase” checkbox. Clicking the checkbox should *not* focus the input above it.
Show solutionNext Challenge
[PreviousManipulating the DOM with Refs](https://react.dev/learn/manipulating-the-dom-with-refs)
[NextYou Might Not Need an Effect](https://react.dev/learn/you-might-not-need-an-effect) |
https://react.dev/learn/manipulating-the-dom-with-refs | [Learn React](https://react.dev/learn)
[Escape Hatches](https://react.dev/learn/escape-hatches)
# Manipulating the DOM with Refs[Link for this heading]()
React automatically updates the [DOM](https://developer.mozilla.org/docs/Web/API/Document_Object_Model/Introduction) to match your render output, so your components won’t often need to manipulate it. However, sometimes you might need access to the DOM elements managed by React—for example, to focus a node, scroll to it, or measure its size and position. There is no built-in way to do those things in React, so you will need a *ref* to the DOM node.
### You will learn
- How to access a DOM node managed by React with the `ref` attribute
- How the `ref` JSX attribute relates to the `useRef` Hook
- How to access another component’s DOM node
- In which cases it’s safe to modify the DOM managed by React
## Getting a ref to the node[Link for Getting a ref to the node]()
To access a DOM node managed by React, first, import the `useRef` Hook:
```
import { useRef } from 'react';
```
Then, use it to declare a ref inside your component:
```
const myRef = useRef(null);
```
Finally, pass your ref as the `ref` attribute to the JSX tag for which you want to get the DOM node:
```
<div ref={myRef}>
```
The `useRef` Hook returns an object with a single property called `current`. Initially, `myRef.current` will be `null`. When React creates a DOM node for this `<div>`, React will put a reference to this node into `myRef.current`. You can then access this DOM node from your [event handlers](https://react.dev/learn/responding-to-events) and use the built-in [browser APIs](https://developer.mozilla.org/docs/Web/API/Element) defined on it.
```
// You can use any browser APIs, for example:
myRef.current.scrollIntoView();
```
### Example: Focusing a text input[Link for Example: Focusing a text input]()
In this example, clicking the button will focus the input:
App.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import { useRef } from 'react';
export default function Form() {
const inputRef = useRef(null);
function handleClick() {
inputRef.current.focus();
}
return (
<>
<input ref={inputRef} />
<button onClick={handleClick}>
Focus the input
</button>
</>
);
}
```
Show more
To implement this:
1. Declare `inputRef` with the `useRef` Hook.
2. Pass it as `<input ref={inputRef}>`. This tells React to **put this `<input>`’s DOM node into `inputRef.current`.**
3. In the `handleClick` function, read the input DOM node from `inputRef.current` and call [`focus()`](https://developer.mozilla.org/en-US/docs/Web/API/HTMLElement/focus) on it with `inputRef.current.focus()`.
4. Pass the `handleClick` event handler to `<button>` with `onClick`.
While DOM manipulation is the most common use case for refs, the `useRef` Hook can be used for storing other things outside React, like timer IDs. Similarly to state, refs remain between renders. Refs are like state variables that don’t trigger re-renders when you set them. Read about refs in [Referencing Values with Refs.](https://react.dev/learn/referencing-values-with-refs)
### Example: Scrolling to an element[Link for Example: Scrolling to an element]()
You can have more than a single ref in a component. In this example, there is a carousel of three images. Each button centers an image by calling the browser [`scrollIntoView()`](https://developer.mozilla.org/en-US/docs/Web/API/Element/scrollIntoView) method on the corresponding DOM node:
App.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import { useRef } from 'react';
export default function CatFriends() {
const firstCatRef = useRef(null);
const secondCatRef = useRef(null);
const thirdCatRef = useRef(null);
function handleScrollToFirstCat() {
firstCatRef.current.scrollIntoView({
behavior: 'smooth',
block: 'nearest',
inline: 'center'
});
}
function handleScrollToSecondCat() {
secondCatRef.current.scrollIntoView({
behavior: 'smooth',
block: 'nearest',
inline: 'center'
});
}
function handleScrollToThirdCat() {
thirdCatRef.current.scrollIntoView({
behavior: 'smooth',
block: 'nearest',
inline: 'center'
});
}
return (
<>
<nav>
<button onClick={handleScrollToFirstCat}>
Neo
</button>
<button onClick={handleScrollToSecondCat}>
Millie
</button>
<button onClick={handleScrollToThirdCat}>
Bella
</button>
</nav>
<div>
<ul>
<li>
<img
src="https://placecats.com/neo/300/200"
alt="Neo"
ref={firstCatRef}
/>
</li>
<li>
<img
src="https://placecats.com/millie/200/200"
alt="Millie"
ref={secondCatRef}
/>
</li>
<li>
<img
src="https://placecats.com/bella/199/200"
alt="Bella"
ref={thirdCatRef}
/>
</li>
</ul>
</div>
</>
);
}
```
Show more
##### Deep Dive
#### How to manage a list of refs using a ref callback[Link for How to manage a list of refs using a ref callback]()
Show Details
In the above examples, there is a predefined number of refs. However, sometimes you might need a ref to each item in the list, and you don’t know how many you will have. Something like this **wouldn’t work**:
```
<ul>
{items.map((item) => {
// Doesn't work!
const ref = useRef(null);
return <li ref={ref} />;
})}
</ul>
```
This is because **Hooks must only be called at the top-level of your component.** You can’t call `useRef` in a loop, in a condition, or inside a `map()` call.
One possible way around this is to get a single ref to their parent element, and then use DOM manipulation methods like [`querySelectorAll`](https://developer.mozilla.org/en-US/docs/Web/API/Document/querySelectorAll) to “find” the individual child nodes from it. However, this is brittle and can break if your DOM structure changes.
Another solution is to **pass a function to the `ref` attribute.** This is called a [`ref` callback.](https://react.dev/reference/react-dom/components/common) React will call your ref callback with the DOM node when it’s time to set the ref, and with `null` when it’s time to clear it. This lets you maintain your own array or a [Map](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Map), and access any ref by its index or some kind of ID.
This example shows how you can use this approach to scroll to an arbitrary node in a long list:
App.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import { useRef, useState } from "react";
export default function CatFriends() {
const itemsRef = useRef(null);
const [catList, setCatList] = useState(setupCatList);
function scrollToCat(cat) {
const map = getMap();
const node = map.get(cat);
node.scrollIntoView({
behavior: "smooth",
block: "nearest",
inline: "center",
});
}
function getMap() {
if (!itemsRef.current) {
// Initialize the Map on first usage.
itemsRef.current = new Map();
}
return itemsRef.current;
}
return (
<>
<nav>
<button onClick={() => scrollToCat(catList[0])}>Neo</button>
<button onClick={() => scrollToCat(catList[5])}>Millie</button>
<button onClick={() => scrollToCat(catList[9])}>Bella</button>
</nav>
<div>
<ul>
{catList.map((cat) => (
<li
key={cat}
ref={(node) => {
const map = getMap();
map.set(cat, node);
return () => {
map.delete(cat);
};
}}
>
<img src={cat} />
</li>
))}
</ul>
</div>
</>
);
}
function setupCatList() {
const catList = [];
for (let i = 0; i < 10; i++) {
catList.push("https://loremflickr.com/320/240/cat?lock=" + i);
}
return catList;
}
```
Show more
In this example, `itemsRef` doesn’t hold a single DOM node. Instead, it holds a [Map](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Map) from item ID to a DOM node. ([Refs can hold any values!](https://react.dev/learn/referencing-values-with-refs)) The [`ref` callback](https://react.dev/reference/react-dom/components/common) on every list item takes care to update the Map:
```
<li
key={cat.id}
ref={node => {
const map = getMap();
// Add to the Map
map.set(cat, node);
return () => {
// Remove from the Map
map.delete(cat);
};
}}
>
```
This lets you read individual DOM nodes from the Map later.
### Note
When Strict Mode is enabled, ref callbacks will run twice in development.
Read more about [how this helps find bugs](https://react.dev/reference/react/StrictMode) in callback refs.
## Accessing another component’s DOM nodes[Link for Accessing another component’s DOM nodes]()
### Pitfall
Refs are an escape hatch. Manually manipulating *another* component’s DOM nodes can make your code fragile.
You can pass refs from parent component to child components [just like any other prop](https://react.dev/learn/passing-props-to-a-component).
```
import { useRef } from 'react';
function MyInput({ ref }) {
return <input ref={ref} />;
}
function MyForm() {
const inputRef = useRef(null);
return <MyInput ref={inputRef} />
}
```
In the above example, a ref is created in the parent component, `MyForm`, and is passed to the child component, `MyInput`. `MyInput` then passes the ref to `<input>`. Because `<input>` is a [built-in component](https://react.dev/reference/react-dom/components/common) React sets the `.current` property of the ref to the `<input>` DOM element.
The `inputRef` created in `MyForm` now points to the `<input>` DOM element returned by `MyInput`. A click handler created in `MyForm` can access `inputRef` and call `focus()` to set the focus on `<input>`.
App.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import { useRef } from 'react';
function MyInput({ ref }) {
return <input ref={ref} />;
}
export default function MyForm() {
const inputRef = useRef(null);
function handleClick() {
inputRef.current.focus();
}
return (
<>
<MyInput ref={inputRef} />
<button onClick={handleClick}>
Focus the input
</button>
</>
);
}
```
Show more
##### Deep Dive
#### Exposing a subset of the API with an imperative handle[Link for Exposing a subset of the API with an imperative handle]()
Show Details
In the above example, the ref passed to `MyInput` is passed on to the original DOM input element. This lets the parent component call `focus()` on it. However, this also lets the parent component do something else—for example, change its CSS styles. In uncommon cases, you may want to restrict the exposed functionality. You can do that with [`useImperativeHandle`](https://react.dev/reference/react/useImperativeHandle):
App.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import { useRef, useImperativeHandle } from "react";
function MyInput({ ref }) {
const realInputRef = useRef(null);
useImperativeHandle(ref, () => ({
// Only expose focus and nothing else
focus() {
realInputRef.current.focus();
},
}));
return <input ref={realInputRef} />;
};
export default function Form() {
const inputRef = useRef(null);
function handleClick() {
inputRef.current.focus();
}
return (
<>
<MyInput ref={inputRef} />
<button onClick={handleClick}>Focus the input</button>
</>
);
}
```
Show more
Here, `realInputRef` inside `MyInput` holds the actual input DOM node. However, [`useImperativeHandle`](https://react.dev/reference/react/useImperativeHandle) instructs React to provide your own special object as the value of a ref to the parent component. So `inputRef.current` inside the `Form` component will only have the `focus` method. In this case, the ref “handle” is not the DOM node, but the custom object you create inside [`useImperativeHandle`](https://react.dev/reference/react/useImperativeHandle) call.
## When React attaches the refs[Link for When React attaches the refs]()
In React, every update is split in [two phases](https://react.dev/learn/render-and-commit):
- During **render,** React calls your components to figure out what should be on the screen.
- During **commit,** React applies changes to the DOM.
In general, you [don’t want](https://react.dev/learn/referencing-values-with-refs) to access refs during rendering. That goes for refs holding DOM nodes as well. During the first render, the DOM nodes have not yet been created, so `ref.current` will be `null`. And during the rendering of updates, the DOM nodes haven’t been updated yet. So it’s too early to read them.
React sets `ref.current` during the commit. Before updating the DOM, React sets the affected `ref.current` values to `null`. After updating the DOM, React immediately sets them to the corresponding DOM nodes.
**Usually, you will access refs from event handlers.** If you want to do something with a ref, but there is no particular event to do it in, you might need an Effect. We will discuss Effects on the next pages.
##### Deep Dive
#### Flushing state updates synchronously with flushSync[Link for Flushing state updates synchronously with flushSync]()
Show Details
Consider code like this, which adds a new todo and scrolls the screen down to the last child of the list. Notice how, for some reason, it always scrolls to the todo that was *just before* the last added one:
App.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import { useState, useRef } from 'react';
export default function TodoList() {
const listRef = useRef(null);
const [text, setText] = useState('');
const [todos, setTodos] = useState(
initialTodos
);
function handleAdd() {
const newTodo = { id: nextId++, text: text };
setText('');
setTodos([ ...todos, newTodo]);
listRef.current.lastChild.scrollIntoView({
behavior: 'smooth',
block: 'nearest'
});
}
return (
<>
<button onClick={handleAdd}>
Add
</button>
<input
value={text}
onChange={e => setText(e.target.value)}
/>
<ul ref={listRef}>
{todos.map(todo => (
<li key={todo.id}>{todo.text}</li>
))}
</ul>
</>
);
}
let nextId = 0;
let initialTodos = [];
for (let i = 0; i < 20; i++) {
initialTodos.push({
id: nextId++,
text: 'Todo #' + (i + 1)
});
}
```
Show more
The issue is with these two lines:
```
setTodos([ ...todos, newTodo]);
listRef.current.lastChild.scrollIntoView();
```
In React, [state updates are queued.](https://react.dev/learn/queueing-a-series-of-state-updates) Usually, this is what you want. However, here it causes a problem because `setTodos` does not immediately update the DOM. So the time you scroll the list to its last element, the todo has not yet been added. This is why scrolling always “lags behind” by one item.
To fix this issue, you can force React to update (“flush”) the DOM synchronously. To do this, import `flushSync` from `react-dom` and **wrap the state update** into a `flushSync` call:
```
flushSync(() => {
setTodos([ ...todos, newTodo]);
});
listRef.current.lastChild.scrollIntoView();
```
This will instruct React to update the DOM synchronously right after the code wrapped in `flushSync` executes. As a result, the last todo will already be in the DOM by the time you try to scroll to it:
App.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import { useState, useRef } from 'react';
import { flushSync } from 'react-dom';
export default function TodoList() {
const listRef = useRef(null);
const [text, setText] = useState('');
const [todos, setTodos] = useState(
initialTodos
);
function handleAdd() {
const newTodo = { id: nextId++, text: text };
flushSync(() => {
setText('');
setTodos([ ...todos, newTodo]);
});
listRef.current.lastChild.scrollIntoView({
behavior: 'smooth',
block: 'nearest'
});
}
return (
<>
<button onClick={handleAdd}>
Add
</button>
<input
value={text}
onChange={e => setText(e.target.value)}
/>
<ul ref={listRef}>
{todos.map(todo => (
<li key={todo.id}>{todo.text}</li>
))}
</ul>
</>
);
}
let nextId = 0;
let initialTodos = [];
for (let i = 0; i < 20; i++) {
initialTodos.push({
id: nextId++,
text: 'Todo #' + (i + 1)
});
}
```
Show more
## Best practices for DOM manipulation with refs[Link for Best practices for DOM manipulation with refs]()
Refs are an escape hatch. You should only use them when you have to “step outside React”. Common examples of this include managing focus, scroll position, or calling browser APIs that React does not expose.
If you stick to non-destructive actions like focusing and scrolling, you shouldn’t encounter any problems. However, if you try to **modify** the DOM manually, you can risk conflicting with the changes React is making.
To illustrate this problem, this example includes a welcome message and two buttons. The first button toggles its presence using [conditional rendering](https://react.dev/learn/conditional-rendering) and [state](https://react.dev/learn/state-a-components-memory), as you would usually do in React. The second button uses the [`remove()` DOM API](https://developer.mozilla.org/en-US/docs/Web/API/Element/remove) to forcefully remove it from the DOM outside of React’s control.
Try pressing “Toggle with setState” a few times. The message should disappear and appear again. Then press “Remove from the DOM”. This will forcefully remove it. Finally, press “Toggle with setState”:
App.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import { useState, useRef } from 'react';
export default function Counter() {
const [show, setShow] = useState(true);
const ref = useRef(null);
return (
<div>
<button
onClick={() => {
setShow(!show);
}}>
Toggle with setState
</button>
<button
onClick={() => {
ref.current.remove();
}}>
Remove from the DOM
</button>
{show && <p ref={ref}>Hello world</p>}
</div>
);
}
```
Show more
After you’ve manually removed the DOM element, trying to use `setState` to show it again will lead to a crash. This is because you’ve changed the DOM, and React doesn’t know how to continue managing it correctly.
**Avoid changing DOM nodes managed by React.** Modifying, adding children to, or removing children from elements that are managed by React can lead to inconsistent visual results or crashes like above.
However, this doesn’t mean that you can’t do it at all. It requires caution. **You can safely modify parts of the DOM that React has *no reason* to update.** For example, if some `<div>` is always empty in the JSX, React won’t have a reason to touch its children list. Therefore, it is safe to manually add or remove elements there.
## Recap[Link for Recap]()
- Refs are a generic concept, but most often you’ll use them to hold DOM elements.
- You instruct React to put a DOM node into `myRef.current` by passing `<div ref={myRef}>`.
- Usually, you will use refs for non-destructive actions like focusing, scrolling, or measuring DOM elements.
- A component doesn’t expose its DOM nodes by default. You can opt into exposing a DOM node by using `forwardRef` and passing the second `ref` argument down to a specific node.
- Avoid changing DOM nodes managed by React.
- If you do modify DOM nodes managed by React, modify parts that React has no reason to update.
## Try out some challenges[Link for Try out some challenges]()
1\. Play and pause the video 2. Focus the search field 3. Scrolling an image carousel 4. Focus the search field with separate components
#### Challenge 1 of 4: Play and pause the video[Link for this heading]()
In this example, the button toggles a state variable to switch between a playing and a paused state. However, in order to actually play or pause the video, toggling state is not enough. You also need to call [`play()`](https://developer.mozilla.org/en-US/docs/Web/API/HTMLMediaElement/play) and [`pause()`](https://developer.mozilla.org/en-US/docs/Web/API/HTMLMediaElement/pause) on the DOM element for the `<video>`. Add a ref to it, and make the button work.
App.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import { useState, useRef } from 'react';
export default function VideoPlayer() {
const [isPlaying, setIsPlaying] = useState(false);
function handleClick() {
const nextIsPlaying = !isPlaying;
setIsPlaying(nextIsPlaying);
}
return (
<>
<button onClick={handleClick}>
{isPlaying ? 'Pause' : 'Play'}
</button>
<video width="250">
<source
src="https://interactive-examples.mdn.mozilla.net/media/cc0-videos/flower.mp4"
type="video/mp4"
/>
</video>
</>
)
}
```
Show more
For an extra challenge, keep the “Play” button in sync with whether the video is playing even if the user right-clicks the video and plays it using the built-in browser media controls. You might want to listen to `onPlay` and `onPause` on the video to do that.
Show solutionNext Challenge
[PreviousReferencing Values with Refs](https://react.dev/learn/referencing-values-with-refs)
[NextSynchronizing with Effects](https://react.dev/learn/synchronizing-with-effects) |
https://react.dev/reference/react/experimental_useEffectEvent | [API Reference](https://react.dev/reference/react)
# experimental\_useEffectEvent[Link for this heading]()
### Under Construction
**This API is experimental and is not available in a stable version of React yet.**
You can try it by upgrading React packages to the most recent experimental version:
- `react@experimental`
- `react-dom@experimental`
- `eslint-plugin-react-hooks@experimental`
Experimental versions of React may contain bugs. Don’t use them in production.
`useEffectEvent` is a React Hook that lets you extract non-reactive logic into an [Effect Event.](https://react.dev/learn/separating-events-from-effects)
```
const onSomething = useEffectEvent(callback)
``` |
https://react.dev/reference/rsc/server-functions | [API Reference](https://react.dev/reference/react)
# Server Functions[Link for this heading]()
### React Server Components
Server Functions are for use in [React Server Components](https://react.dev/learn/start-a-new-react-project).
**Note:** Until September 2024, we referred to all Server Functions as “Server Actions”. If a Server Function is passed to an action prop or called from inside an action then it is a Server Action, but not all Server Functions are Server Actions. The naming in this documentation has been updated to reflect that Server Functions can be used for multiple purposes.
Server Functions allow Client Components to call async functions executed on the server.
### Note
#### How do I build support for Server Functions?[Link for How do I build support for Server Functions?]()
While Server Functions in React 19 are stable and will not break between minor versions, the underlying APIs used to implement Server Functions in a React Server Components bundler or framework do not follow semver and may break between minors in React 19.x.
To support Server Functions as a bundler or framework, we recommend pinning to a specific React version, or using the Canary release. We will continue working with bundlers and frameworks to stabilize the APIs used to implement Server Functions in the future.
When a Server Functions is defined with the [`"use server"`](https://react.dev/reference/rsc/use-server) directive, your framework will automatically create a reference to the server function, and pass that reference to the Client Component. When that function is called on the client, React will send a request to the server to execute the function, and return the result.
Server Functions can be created in Server Components and passed as props to Client Components, or they can be imported and used in Client Components.
## Usage[Link for Usage]()
### Creating a Server Function from a Server Component[Link for Creating a Server Function from a Server Component]()
Server Components can define Server Functions with the `"use server"` directive:
```
// Server Component
import Button from './Button';
function EmptyNote () {
async function createNoteAction() {
// Server Function
'use server';
await db.notes.create();
}
return <Button onClick={createNoteAction}/>;
}
```
When React renders the `EmptyNote` Server Function, it will create a reference to the `createNoteAction` function, and pass that reference to the `Button` Client Component. When the button is clicked, React will send a request to the server to execute the `createNoteAction` function with the reference provided:
```
"use client";
export default function Button({onClick}) {
console.log(onClick);
// {$$typeof: Symbol.for("react.server.reference"), $$id: 'createNoteAction'}
return <button onClick={() => onClick()}>Create Empty Note</button>
}
```
For more, see the docs for [`"use server"`](https://react.dev/reference/rsc/use-server).
### Importing Server Functions from Client Components[Link for Importing Server Functions from Client Components]()
Client Components can import Server Functions from files that use the `"use server"` directive:
```
"use server";
export async function createNote() {
await db.notes.create();
}
```
When the bundler builds the `EmptyNote` Client Component, it will create a reference to the `createNoteAction` function in the bundle. When the `button` is clicked, React will send a request to the server to execute the `createNoteAction` function using the reference provided:
```
"use client";
import {createNote} from './actions';
function EmptyNote() {
console.log(createNote);
// {$$typeof: Symbol.for("react.server.reference"), $$id: 'createNoteAction'}
<button onClick={() => createNote()} />
}
```
For more, see the docs for [`"use server"`](https://react.dev/reference/rsc/use-server).
### Server Functions with Actions[Link for Server Functions with Actions]()
Server Functions can be called from Actions on the client:
```
"use server";
export async function updateName(name) {
if (!name) {
return {error: 'Name is required'};
}
await db.users.updateName(name);
}
```
```
"use client";
import {updateName} from './actions';
function UpdateName() {
const [name, setName] = useState('');
const [error, setError] = useState(null);
const [isPending, startTransition] = useTransition();
const submitAction = async () => {
startTransition(async () => {
const {error} = await updateName(name);
if (!error) {
setError(error);
} else {
setName('');
}
})
}
return (
<form action={submitAction}>
<input type="text" name="name" disabled={isPending}/>
{state.error && <span>Failed: {state.error}</span>}
</form>
)
}
```
This allows you to access the `isPending` state of the Server Function by wrapping it in an Action on the client.
For more, see the docs for [Calling a Server Function outside of `<form>`](https://react.dev/reference/rsc/use-server)
### Server Functions with Form Actions[Link for Server Functions with Form Actions]()
Server Functions work with the new Form features in React 19.
You can pass a Server Function to a Form to automatically submit the form to the server:
```
"use client";
import {updateName} from './actions';
function UpdateName() {
return (
<form action={updateName}>
<input type="text" name="name" />
</form>
)
}
```
When the Form submission succeeds, React will automatically reset the form. You can add `useActionState` to access the pending state, last response, or to support progressive enhancement.
For more, see the docs for [Server Functions in Forms](https://react.dev/reference/rsc/use-server).
### Server Functions with `useActionState`[Link for this heading]()
You can call Server Functions with `useActionState` for the common case where you just need access to the action pending state and last returned response:
```
"use client";
import {updateName} from './actions';
function UpdateName() {
const [state, submitAction, isPending] = useActionState(updateName, {error: null});
return (
<form action={submitAction}>
<input type="text" name="name" disabled={isPending}/>
{state.error && <span>Failed: {state.error}</span>}
</form>
);
}
```
When using `useActionState` with Server Functions, React will also automatically replay form submissions entered before hydration finishes. This means users can interact with your app even before the app has hydrated.
For more, see the docs for [`useActionState`](https://react.dev/reference/react-dom/hooks/useFormState).
### Progressive enhancement with `useActionState`[Link for this heading]()
Server Functions also support progressive enhancement with the third argument of `useActionState`.
```
"use client";
import {updateName} from './actions';
function UpdateName() {
const [, submitAction] = useActionState(updateName, null, `/name/update`);
return (
<form action={submitAction}>
...
</form>
);
}
```
When the permalink is provided to `useActionState`, React will redirect to the provided URL if the form is submitted before the JavaScript bundle loads.
For more, see the docs for [`useActionState`](https://react.dev/reference/react-dom/hooks/useFormState).
[PreviousServer Components](https://react.dev/reference/rsc/server-components)
[NextDirectives](https://react.dev/reference/rsc/directives) |
https://react.dev/learn/reusing-logic-with-custom-hooks | [Learn React](https://react.dev/learn)
[Escape Hatches](https://react.dev/learn/escape-hatches)
# Reusing Logic with Custom Hooks[Link for this heading]()
React comes with several built-in Hooks like `useState`, `useContext`, and `useEffect`. Sometimes, you’ll wish that there was a Hook for some more specific purpose: for example, to fetch data, to keep track of whether the user is online, or to connect to a chat room. You might not find these Hooks in React, but you can create your own Hooks for your application’s needs.
### You will learn
- What custom Hooks are, and how to write your own
- How to reuse logic between components
- How to name and structure your custom Hooks
- When and why to extract custom Hooks
## Custom Hooks: Sharing logic between components[Link for Custom Hooks: Sharing logic between components]()
Imagine you’re developing an app that heavily relies on the network (as most apps do). You want to warn the user if their network connection has accidentally gone off while they were using your app. How would you go about it? It seems like you’ll need two things in your component:
1. A piece of state that tracks whether the network is online.
2. An Effect that subscribes to the global [`online`](https://developer.mozilla.org/en-US/docs/Web/API/Window/online_event) and [`offline`](https://developer.mozilla.org/en-US/docs/Web/API/Window/offline_event) events, and updates that state.
This will keep your component [synchronized](https://react.dev/learn/synchronizing-with-effects) with the network status. You might start with something like this:
App.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import { useState, useEffect } from 'react';
export default function StatusBar() {
const [isOnline, setIsOnline] = useState(true);
useEffect(() => {
function handleOnline() {
setIsOnline(true);
}
function handleOffline() {
setIsOnline(false);
}
window.addEventListener('online', handleOnline);
window.addEventListener('offline', handleOffline);
return () => {
window.removeEventListener('online', handleOnline);
window.removeEventListener('offline', handleOffline);
};
}, []);
return <h1>{isOnline ? '✅ Online' : '❌ Disconnected'}</h1>;
}
```
Show more
Try turning your network on and off, and notice how this `StatusBar` updates in response to your actions.
Now imagine you *also* want to use the same logic in a different component. You want to implement a Save button that will become disabled and show “Reconnecting…” instead of “Save” while the network is off.
To start, you can copy and paste the `isOnline` state and the Effect into `SaveButton`:
App.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import { useState, useEffect } from 'react';
export default function SaveButton() {
const [isOnline, setIsOnline] = useState(true);
useEffect(() => {
function handleOnline() {
setIsOnline(true);
}
function handleOffline() {
setIsOnline(false);
}
window.addEventListener('online', handleOnline);
window.addEventListener('offline', handleOffline);
return () => {
window.removeEventListener('online', handleOnline);
window.removeEventListener('offline', handleOffline);
};
}, []);
function handleSaveClick() {
console.log('✅ Progress saved');
}
return (
<button disabled={!isOnline} onClick={handleSaveClick}>
{isOnline ? 'Save progress' : 'Reconnecting...'}
</button>
);
}
```
Show more
Verify that, if you turn off the network, the button will change its appearance.
These two components work fine, but the duplication in logic between them is unfortunate. It seems like even though they have different *visual appearance,* you want to reuse the logic between them.
### Extracting your own custom Hook from a component[Link for Extracting your own custom Hook from a component]()
Imagine for a moment that, similar to [`useState`](https://react.dev/reference/react/useState) and [`useEffect`](https://react.dev/reference/react/useEffect), there was a built-in `useOnlineStatus` Hook. Then both of these components could be simplified and you could remove the duplication between them:
```
function StatusBar() {
const isOnline = useOnlineStatus();
return <h1>{isOnline ? '✅ Online' : '❌ Disconnected'}</h1>;
}
function SaveButton() {
const isOnline = useOnlineStatus();
function handleSaveClick() {
console.log('✅ Progress saved');
}
return (
<button disabled={!isOnline} onClick={handleSaveClick}>
{isOnline ? 'Save progress' : 'Reconnecting...'}
</button>
);
}
```
Although there is no such built-in Hook, you can write it yourself. Declare a function called `useOnlineStatus` and move all the duplicated code into it from the components you wrote earlier:
```
function useOnlineStatus() {
const [isOnline, setIsOnline] = useState(true);
useEffect(() => {
function handleOnline() {
setIsOnline(true);
}
function handleOffline() {
setIsOnline(false);
}
window.addEventListener('online', handleOnline);
window.addEventListener('offline', handleOffline);
return () => {
window.removeEventListener('online', handleOnline);
window.removeEventListener('offline', handleOffline);
};
}, []);
return isOnline;
}
```
At the end of the function, return `isOnline`. This lets your components read that value:
App.jsuseOnlineStatus.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import { useOnlineStatus } from './useOnlineStatus.js';
function StatusBar() {
const isOnline = useOnlineStatus();
return <h1>{isOnline ? '✅ Online' : '❌ Disconnected'}</h1>;
}
function SaveButton() {
const isOnline = useOnlineStatus();
function handleSaveClick() {
console.log('✅ Progress saved');
}
return (
<button disabled={!isOnline} onClick={handleSaveClick}>
{isOnline ? 'Save progress' : 'Reconnecting...'}
</button>
);
}
export default function App() {
return (
<>
<SaveButton />
<StatusBar />
</>
);
}
```
Show more
Verify that switching the network on and off updates both components.
Now your components don’t have as much repetitive logic. **More importantly, the code inside them describes *what they want to do* (use the online status!) rather than *how to do it* (by subscribing to the browser events).**
When you extract logic into custom Hooks, you can hide the gnarly details of how you deal with some external system or a browser API. The code of your components expresses your intent, not the implementation.
### Hook names always start with `use`[Link for this heading]()
React applications are built from components. Components are built from Hooks, whether built-in or custom. You’ll likely often use custom Hooks created by others, but occasionally you might write one yourself!
You must follow these naming conventions:
1. **React component names must start with a capital letter,** like `StatusBar` and `SaveButton`. React components also need to return something that React knows how to display, like a piece of JSX.
2. **Hook names must start with `use` followed by a capital letter,** like [`useState`](https://react.dev/reference/react/useState) (built-in) or `useOnlineStatus` (custom, like earlier on the page). Hooks may return arbitrary values.
This convention guarantees that you can always look at a component and know where its state, Effects, and other React features might “hide”. For example, if you see a `getColor()` function call inside your component, you can be sure that it can’t possibly contain React state inside because its name doesn’t start with `use`. However, a function call like `useOnlineStatus()` will most likely contain calls to other Hooks inside!
### Note
If your linter is [configured for React,](https://react.dev/learn/editor-setup) it will enforce this naming convention. Scroll up to the sandbox above and rename `useOnlineStatus` to `getOnlineStatus`. Notice that the linter won’t allow you to call `useState` or `useEffect` inside of it anymore. Only Hooks and components can call other Hooks!
##### Deep Dive
#### Should all functions called during rendering start with the use prefix?[Link for Should all functions called during rendering start with the use prefix?]()
Show Details
No. Functions that don’t *call* Hooks don’t need to *be* Hooks.
If your function doesn’t call any Hooks, avoid the `use` prefix. Instead, write it as a regular function *without* the `use` prefix. For example, `useSorted` below doesn’t call Hooks, so call it `getSorted` instead:
```
// 🔴 Avoid: A Hook that doesn't use Hooks
function useSorted(items) {
return items.slice().sort();
}
// ✅ Good: A regular function that doesn't use Hooks
function getSorted(items) {
return items.slice().sort();
}
```
This ensures that your code can call this regular function anywhere, including conditions:
```
function List({ items, shouldSort }) {
let displayedItems = items;
if (shouldSort) {
// ✅ It's ok to call getSorted() conditionally because it's not a Hook
displayedItems = getSorted(items);
}
// ...
}
```
You should give `use` prefix to a function (and thus make it a Hook) if it uses at least one Hook inside of it:
```
// ✅ Good: A Hook that uses other Hooks
function useAuth() {
return useContext(Auth);
}
```
Technically, this isn’t enforced by React. In principle, you could make a Hook that doesn’t call other Hooks. This is often confusing and limiting so it’s best to avoid that pattern. However, there may be rare cases where it is helpful. For example, maybe your function doesn’t use any Hooks right now, but you plan to add some Hook calls to it in the future. Then it makes sense to name it with the `use` prefix:
```
// ✅ Good: A Hook that will likely use some other Hooks later
function useAuth() {
// TODO: Replace with this line when authentication is implemented:
// return useContext(Auth);
return TEST_USER;
}
```
Then components won’t be able to call it conditionally. This will become important when you actually add Hook calls inside. If you don’t plan to use Hooks inside it (now or later), don’t make it a Hook.
### Custom Hooks let you share stateful logic, not state itself[Link for Custom Hooks let you share stateful logic, not state itself]()
In the earlier example, when you turned the network on and off, both components updated together. However, it’s wrong to think that a single `isOnline` state variable is shared between them. Look at this code:
```
function StatusBar() {
const isOnline = useOnlineStatus();
// ...
}
function SaveButton() {
const isOnline = useOnlineStatus();
// ...
}
```
It works the same way as before you extracted the duplication:
```
function StatusBar() {
const [isOnline, setIsOnline] = useState(true);
useEffect(() => {
// ...
}, []);
// ...
}
function SaveButton() {
const [isOnline, setIsOnline] = useState(true);
useEffect(() => {
// ...
}, []);
// ...
}
```
These are two completely independent state variables and Effects! They happened to have the same value at the same time because you synchronized them with the same external value (whether the network is on).
To better illustrate this, we’ll need a different example. Consider this `Form` component:
App.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import { useState } from 'react';
export default function Form() {
const [firstName, setFirstName] = useState('Mary');
const [lastName, setLastName] = useState('Poppins');
function handleFirstNameChange(e) {
setFirstName(e.target.value);
}
function handleLastNameChange(e) {
setLastName(e.target.value);
}
return (
<>
<label>
First name:
<input value={firstName} onChange={handleFirstNameChange} />
</label>
<label>
Last name:
<input value={lastName} onChange={handleLastNameChange} />
</label>
<p><b>Good morning, {firstName} {lastName}.</b></p>
</>
);
}
```
Show more
There’s some repetitive logic for each form field:
1. There’s a piece of state (`firstName` and `lastName`).
2. There’s a change handler (`handleFirstNameChange` and `handleLastNameChange`).
3. There’s a piece of JSX that specifies the `value` and `onChange` attributes for that input.
You can extract the repetitive logic into this `useFormInput` custom Hook:
App.jsuseFormInput.js
useFormInput.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import { useState } from 'react';
export function useFormInput(initialValue) {
const [value, setValue] = useState(initialValue);
function handleChange(e) {
setValue(e.target.value);
}
const inputProps = {
value: value,
onChange: handleChange
};
return inputProps;
}
```
Show more
Notice that it only declares *one* state variable called `value`.
However, the `Form` component calls `useFormInput` *two times:*
```
function Form() {
const firstNameProps = useFormInput('Mary');
const lastNameProps = useFormInput('Poppins');
// ...
```
This is why it works like declaring two separate state variables!
**Custom Hooks let you share *stateful logic* but not *state itself.* Each call to a Hook is completely independent from every other call to the same Hook.** This is why the two sandboxes above are completely equivalent. If you’d like, scroll back up and compare them. The behavior before and after extracting a custom Hook is identical.
When you need to share the state itself between multiple components, [lift it up and pass it down](https://react.dev/learn/sharing-state-between-components) instead.
## Passing reactive values between Hooks[Link for Passing reactive values between Hooks]()
The code inside your custom Hooks will re-run during every re-render of your component. This is why, like components, custom Hooks [need to be pure.](https://react.dev/learn/keeping-components-pure) Think of custom Hooks’ code as part of your component’s body!
Because custom Hooks re-render together with your component, they always receive the latest props and state. To see what this means, consider this chat room example. Change the server URL or the chat room:
App.jsChatRoom.jschat.jsnotifications.js
ChatRoom.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import { useState, useEffect } from 'react';
import { createConnection } from './chat.js';
import { showNotification } from './notifications.js';
export default function ChatRoom({ roomId }) {
const [serverUrl, setServerUrl] = useState('https://localhost:1234');
useEffect(() => {
const options = {
serverUrl: serverUrl,
roomId: roomId
};
const connection = createConnection(options);
connection.on('message', (msg) => {
showNotification('New message: ' + msg);
});
connection.connect();
return () => connection.disconnect();
}, [roomId, serverUrl]);
return (
<>
<label>
Server URL:
<input value={serverUrl} onChange={e => setServerUrl(e.target.value)} />
</label>
<h1>Welcome to the {roomId} room!</h1>
</>
);
}
```
Show more
When you change `serverUrl` or `roomId`, the Effect [“reacts” to your changes](https://react.dev/learn/lifecycle-of-reactive-effects) and re-synchronizes. You can tell by the console messages that the chat re-connects every time that you change your Effect’s dependencies.
Now move the Effect’s code into a custom Hook:
```
export function useChatRoom({ serverUrl, roomId }) {
useEffect(() => {
const options = {
serverUrl: serverUrl,
roomId: roomId
};
const connection = createConnection(options);
connection.connect();
connection.on('message', (msg) => {
showNotification('New message: ' + msg);
});
return () => connection.disconnect();
}, [roomId, serverUrl]);
}
```
This lets your `ChatRoom` component call your custom Hook without worrying about how it works inside:
```
export default function ChatRoom({ roomId }) {
const [serverUrl, setServerUrl] = useState('https://localhost:1234');
useChatRoom({
roomId: roomId,
serverUrl: serverUrl
});
return (
<>
<label>
Server URL:
<input value={serverUrl} onChange={e => setServerUrl(e.target.value)} />
</label>
<h1>Welcome to the {roomId} room!</h1>
</>
);
}
```
This looks much simpler! (But it does the same thing.)
Notice that the logic *still responds* to prop and state changes. Try editing the server URL or the selected room:
App.jsChatRoom.jsuseChatRoom.jschat.jsnotifications.js
ChatRoom.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import { useState } from 'react';
import { useChatRoom } from './useChatRoom.js';
export default function ChatRoom({ roomId }) {
const [serverUrl, setServerUrl] = useState('https://localhost:1234');
useChatRoom({
roomId: roomId,
serverUrl: serverUrl
});
return (
<>
<label>
Server URL:
<input value={serverUrl} onChange={e => setServerUrl(e.target.value)} />
</label>
<h1>Welcome to the {roomId} room!</h1>
</>
);
}
```
Show more
Notice how you’re taking the return value of one Hook:
```
export default function ChatRoom({ roomId }) {
const [serverUrl, setServerUrl] = useState('https://localhost:1234');
useChatRoom({
roomId: roomId,
serverUrl: serverUrl
});
// ...
```
and pass it as an input to another Hook:
```
export default function ChatRoom({ roomId }) {
const [serverUrl, setServerUrl] = useState('https://localhost:1234');
useChatRoom({
roomId: roomId,
serverUrl: serverUrl
});
// ...
```
Every time your `ChatRoom` component re-renders, it passes the latest `roomId` and `serverUrl` to your Hook. This is why your Effect re-connects to the chat whenever their values are different after a re-render. (If you ever worked with audio or video processing software, chaining Hooks like this might remind you of chaining visual or audio effects. It’s as if the output of `useState` “feeds into” the input of the `useChatRoom`.)
### Passing event handlers to custom Hooks[Link for Passing event handlers to custom Hooks]()
### Under Construction
This section describes an **experimental API that has not yet been released** in a stable version of React.
As you start using `useChatRoom` in more components, you might want to let components customize its behavior. For example, currently, the logic for what to do when a message arrives is hardcoded inside the Hook:
```
export function useChatRoom({ serverUrl, roomId }) {
useEffect(() => {
const options = {
serverUrl: serverUrl,
roomId: roomId
};
const connection = createConnection(options);
connection.connect();
connection.on('message', (msg) => {
showNotification('New message: ' + msg);
});
return () => connection.disconnect();
}, [roomId, serverUrl]);
}
```
Let’s say you want to move this logic back to your component:
```
export default function ChatRoom({ roomId }) {
const [serverUrl, setServerUrl] = useState('https://localhost:1234');
useChatRoom({
roomId: roomId,
serverUrl: serverUrl,
onReceiveMessage(msg) {
showNotification('New message: ' + msg);
}
});
// ...
```
To make this work, change your custom Hook to take `onReceiveMessage` as one of its named options:
```
export function useChatRoom({ serverUrl, roomId, onReceiveMessage }) {
useEffect(() => {
const options = {
serverUrl: serverUrl,
roomId: roomId
};
const connection = createConnection(options);
connection.connect();
connection.on('message', (msg) => {
onReceiveMessage(msg);
});
return () => connection.disconnect();
}, [roomId, serverUrl, onReceiveMessage]); // ✅ All dependencies declared
}
```
This will work, but there’s one more improvement you can do when your custom Hook accepts event handlers.
Adding a dependency on `onReceiveMessage` is not ideal because it will cause the chat to re-connect every time the component re-renders. [Wrap this event handler into an Effect Event to remove it from the dependencies:](https://react.dev/learn/removing-effect-dependencies)
```
import { useEffect, useEffectEvent } from 'react';
// ...
export function useChatRoom({ serverUrl, roomId, onReceiveMessage }) {
const onMessage = useEffectEvent(onReceiveMessage);
useEffect(() => {
const options = {
serverUrl: serverUrl,
roomId: roomId
};
const connection = createConnection(options);
connection.connect();
connection.on('message', (msg) => {
onMessage(msg);
});
return () => connection.disconnect();
}, [roomId, serverUrl]); // ✅ All dependencies declared
}
```
Now the chat won’t re-connect every time that the `ChatRoom` component re-renders. Here is a fully working demo of passing an event handler to a custom Hook that you can play with:
App.jsChatRoom.jsuseChatRoom.jschat.jsnotifications.js
ChatRoom.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import { useState } from 'react';
import { useChatRoom } from './useChatRoom.js';
import { showNotification } from './notifications.js';
export default function ChatRoom({ roomId }) {
const [serverUrl, setServerUrl] = useState('https://localhost:1234');
useChatRoom({
roomId: roomId,
serverUrl: serverUrl,
onReceiveMessage(msg) {
showNotification('New message: ' + msg);
}
});
return (
<>
<label>
Server URL:
<input value={serverUrl} onChange={e => setServerUrl(e.target.value)} />
</label>
<h1>Welcome to the {roomId} room!</h1>
</>
);
}
```
Show more
Notice how you no longer need to know *how* `useChatRoom` works in order to use it. You could add it to any other component, pass any other options, and it would work the same way. That’s the power of custom Hooks.
## When to use custom Hooks[Link for When to use custom Hooks]()
You don’t need to extract a custom Hook for every little duplicated bit of code. Some duplication is fine. For example, extracting a `useFormInput` Hook to wrap a single `useState` call like earlier is probably unnecessary.
However, whenever you write an Effect, consider whether it would be clearer to also wrap it in a custom Hook. [You shouldn’t need Effects very often,](https://react.dev/learn/you-might-not-need-an-effect) so if you’re writing one, it means that you need to “step outside React” to synchronize with some external system or to do something that React doesn’t have a built-in API for. Wrapping it into a custom Hook lets you precisely communicate your intent and how the data flows through it.
For example, consider a `ShippingForm` component that displays two dropdowns: one shows the list of cities, and another shows the list of areas in the selected city. You might start with some code that looks like this:
```
function ShippingForm({ country }) {
const [cities, setCities] = useState(null);
// This Effect fetches cities for a country
useEffect(() => {
let ignore = false;
fetch(`/api/cities?country=${country}`)
.then(response => response.json())
.then(json => {
if (!ignore) {
setCities(json);
}
});
return () => {
ignore = true;
};
}, [country]);
const [city, setCity] = useState(null);
const [areas, setAreas] = useState(null);
// This Effect fetches areas for the selected city
useEffect(() => {
if (city) {
let ignore = false;
fetch(`/api/areas?city=${city}`)
.then(response => response.json())
.then(json => {
if (!ignore) {
setAreas(json);
}
});
return () => {
ignore = true;
};
}
}, [city]);
// ...
```
Although this code is quite repetitive, [it’s correct to keep these Effects separate from each other.](https://react.dev/learn/removing-effect-dependencies) They synchronize two different things, so you shouldn’t merge them into one Effect. Instead, you can simplify the `ShippingForm` component above by extracting the common logic between them into your own `useData` Hook:
```
function useData(url) {
const [data, setData] = useState(null);
useEffect(() => {
if (url) {
let ignore = false;
fetch(url)
.then(response => response.json())
.then(json => {
if (!ignore) {
setData(json);
}
});
return () => {
ignore = true;
};
}
}, [url]);
return data;
}
```
Now you can replace both Effects in the `ShippingForm` components with calls to `useData`:
```
function ShippingForm({ country }) {
const cities = useData(`/api/cities?country=${country}`);
const [city, setCity] = useState(null);
const areas = useData(city ? `/api/areas?city=${city}` : null);
// ...
```
Extracting a custom Hook makes the data flow explicit. You feed the `url` in and you get the `data` out. By “hiding” your Effect inside `useData`, you also prevent someone working on the `ShippingForm` component from adding [unnecessary dependencies](https://react.dev/learn/removing-effect-dependencies) to it. With time, most of your app’s Effects will be in custom Hooks.
##### Deep Dive
#### Keep your custom Hooks focused on concrete high-level use cases[Link for Keep your custom Hooks focused on concrete high-level use cases]()
Show Details
Start by choosing your custom Hook’s name. If you struggle to pick a clear name, it might mean that your Effect is too coupled to the rest of your component’s logic, and is not yet ready to be extracted.
Ideally, your custom Hook’s name should be clear enough that even a person who doesn’t write code often could have a good guess about what your custom Hook does, what it takes, and what it returns:
- ✅ `useData(url)`
- ✅ `useImpressionLog(eventName, extraData)`
- ✅ `useChatRoom(options)`
When you synchronize with an external system, your custom Hook name may be more technical and use jargon specific to that system. It’s good as long as it would be clear to a person familiar with that system:
- ✅ `useMediaQuery(query)`
- ✅ `useSocket(url)`
- ✅ `useIntersectionObserver(ref, options)`
**Keep custom Hooks focused on concrete high-level use cases.** Avoid creating and using custom “lifecycle” Hooks that act as alternatives and convenience wrappers for the `useEffect` API itself:
- 🔴 `useMount(fn)`
- 🔴 `useEffectOnce(fn)`
- 🔴 `useUpdateEffect(fn)`
For example, this `useMount` Hook tries to ensure some code only runs “on mount”:
```
function ChatRoom({ roomId }) {
const [serverUrl, setServerUrl] = useState('https://localhost:1234');
// 🔴 Avoid: using custom "lifecycle" Hooks
useMount(() => {
const connection = createConnection({ roomId, serverUrl });
connection.connect();
post('/analytics/event', { eventName: 'visit_chat' });
});
// ...
}
// 🔴 Avoid: creating custom "lifecycle" Hooks
function useMount(fn) {
useEffect(() => {
fn();
}, []); // 🔴 React Hook useEffect has a missing dependency: 'fn'
}
```
**Custom “lifecycle” Hooks like `useMount` don’t fit well into the React paradigm.** For example, this code example has a mistake (it doesn’t “react” to `roomId` or `serverUrl` changes), but the linter won’t warn you about it because the linter only checks direct `useEffect` calls. It won’t know about your Hook.
If you’re writing an Effect, start by using the React API directly:
```
function ChatRoom({ roomId }) {
const [serverUrl, setServerUrl] = useState('https://localhost:1234');
// ✅ Good: two raw Effects separated by purpose
useEffect(() => {
const connection = createConnection({ serverUrl, roomId });
connection.connect();
return () => connection.disconnect();
}, [serverUrl, roomId]);
useEffect(() => {
post('/analytics/event', { eventName: 'visit_chat', roomId });
}, [roomId]);
// ...
}
```
Then, you can (but don’t have to) extract custom Hooks for different high-level use cases:
```
function ChatRoom({ roomId }) {
const [serverUrl, setServerUrl] = useState('https://localhost:1234');
// ✅ Great: custom Hooks named after their purpose
useChatRoom({ serverUrl, roomId });
useImpressionLog('visit_chat', { roomId });
// ...
}
```
**A good custom Hook makes the calling code more declarative by constraining what it does.** For example, `useChatRoom(options)` can only connect to the chat room, while `useImpressionLog(eventName, extraData)` can only send an impression log to the analytics. If your custom Hook API doesn’t constrain the use cases and is very abstract, in the long run it’s likely to introduce more problems than it solves.
### Custom Hooks help you migrate to better patterns[Link for Custom Hooks help you migrate to better patterns]()
Effects are an [“escape hatch”](https://react.dev/learn/escape-hatches): you use them when you need to “step outside React” and when there is no better built-in solution for your use case. With time, the React team’s goal is to reduce the number of the Effects in your app to the minimum by providing more specific solutions to more specific problems. Wrapping your Effects in custom Hooks makes it easier to upgrade your code when these solutions become available.
Let’s return to this example:
App.jsuseOnlineStatus.js
useOnlineStatus.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import { useState, useEffect } from 'react';
export function useOnlineStatus() {
const [isOnline, setIsOnline] = useState(true);
useEffect(() => {
function handleOnline() {
setIsOnline(true);
}
function handleOffline() {
setIsOnline(false);
}
window.addEventListener('online', handleOnline);
window.addEventListener('offline', handleOffline);
return () => {
window.removeEventListener('online', handleOnline);
window.removeEventListener('offline', handleOffline);
};
}, []);
return isOnline;
}
```
Show more
In the above example, `useOnlineStatus` is implemented with a pair of [`useState`](https://react.dev/reference/react/useState) and [`useEffect`.](https://react.dev/reference/react/useEffect) However, this isn’t the best possible solution. There is a number of edge cases it doesn’t consider. For example, it assumes that when the component mounts, `isOnline` is already `true`, but this may be wrong if the network already went offline. You can use the browser [`navigator.onLine`](https://developer.mozilla.org/en-US/docs/Web/API/Navigator/onLine) API to check for that, but using it directly would not work on the server for generating the initial HTML. In short, this code could be improved.
Luckily, React 18 includes a dedicated API called [`useSyncExternalStore`](https://react.dev/reference/react/useSyncExternalStore) which takes care of all of these problems for you. Here is how your `useOnlineStatus` Hook, rewritten to take advantage of this new API:
App.jsuseOnlineStatus.js
useOnlineStatus.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import { useSyncExternalStore } from 'react';
function subscribe(callback) {
window.addEventListener('online', callback);
window.addEventListener('offline', callback);
return () => {
window.removeEventListener('online', callback);
window.removeEventListener('offline', callback);
};
}
export function useOnlineStatus() {
return useSyncExternalStore(
subscribe,
() => navigator.onLine, // How to get the value on the client
() => true // How to get the value on the server
);
}
```
Show more
Notice how **you didn’t need to change any of the components** to make this migration:
```
function StatusBar() {
const isOnline = useOnlineStatus();
// ...
}
function SaveButton() {
const isOnline = useOnlineStatus();
// ...
}
```
This is another reason for why wrapping Effects in custom Hooks is often beneficial:
1. You make the data flow to and from your Effects very explicit.
2. You let your components focus on the intent rather than on the exact implementation of your Effects.
3. When React adds new features, you can remove those Effects without changing any of your components.
Similar to a [design system,](https://uxdesign.cc/everything-you-need-to-know-about-design-systems-54b109851969) you might find it helpful to start extracting common idioms from your app’s components into custom Hooks. This will keep your components’ code focused on the intent, and let you avoid writing raw Effects very often. Many excellent custom Hooks are maintained by the React community.
##### Deep Dive
#### Will React provide any built-in solution for data fetching?[Link for Will React provide any built-in solution for data fetching?]()
Show Details
We’re still working out the details, but we expect that in the future, you’ll write data fetching like this:
```
import { use } from 'react'; // Not available yet!
function ShippingForm({ country }) {
const cities = use(fetch(`/api/cities?country=${country}`));
const [city, setCity] = useState(null);
const areas = city ? use(fetch(`/api/areas?city=${city}`)) : null;
// ...
```
If you use custom Hooks like `useData` above in your app, it will require fewer changes to migrate to the eventually recommended approach than if you write raw Effects in every component manually. However, the old approach will still work fine, so if you feel happy writing raw Effects, you can continue to do that.
### There is more than one way to do it[Link for There is more than one way to do it]()
Let’s say you want to implement a fade-in animation *from scratch* using the browser [`requestAnimationFrame`](https://developer.mozilla.org/en-US/docs/Web/API/window/requestAnimationFrame) API. You might start with an Effect that sets up an animation loop. During each frame of the animation, you could change the opacity of the DOM node you [hold in a ref](https://react.dev/learn/manipulating-the-dom-with-refs) until it reaches `1`. Your code might start like this:
App.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import { useState, useEffect, useRef } from 'react';
function Welcome() {
const ref = useRef(null);
useEffect(() => {
const duration = 1000;
const node = ref.current;
let startTime = performance.now();
let frameId = null;
function onFrame(now) {
const timePassed = now - startTime;
const progress = Math.min(timePassed / duration, 1);
onProgress(progress);
if (progress < 1) {
// We still have more frames to paint
frameId = requestAnimationFrame(onFrame);
}
}
function onProgress(progress) {
node.style.opacity = progress;
}
function start() {
onProgress(0);
startTime = performance.now();
frameId = requestAnimationFrame(onFrame);
}
function stop() {
cancelAnimationFrame(frameId);
startTime = null;
frameId = null;
}
start();
return () => stop();
}, []);
return (
<h1 className="welcome" ref={ref}>
Welcome
</h1>
);
}
export default function App() {
const [show, setShow] = useState(false);
return (
<>
<button onClick={() => setShow(!show)}>
{show ? 'Remove' : 'Show'}
</button>
<hr />
{show && <Welcome />}
</>
);
}
```
Show more
To make the component more readable, you might extract the logic into a `useFadeIn` custom Hook:
App.jsuseFadeIn.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import { useState, useEffect, useRef } from 'react';
import { useFadeIn } from './useFadeIn.js';
function Welcome() {
const ref = useRef(null);
useFadeIn(ref, 1000);
return (
<h1 className="welcome" ref={ref}>
Welcome
</h1>
);
}
export default function App() {
const [show, setShow] = useState(false);
return (
<>
<button onClick={() => setShow(!show)}>
{show ? 'Remove' : 'Show'}
</button>
<hr />
{show && <Welcome />}
</>
);
}
```
Show more
You could keep the `useFadeIn` code as is, but you could also refactor it more. For example, you could extract the logic for setting up the animation loop out of `useFadeIn` into a custom `useAnimationLoop` Hook:
App.jsuseFadeIn.js
useFadeIn.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import { useState, useEffect } from 'react';
import { experimental_useEffectEvent as useEffectEvent } from 'react';
export function useFadeIn(ref, duration) {
const [isRunning, setIsRunning] = useState(true);
useAnimationLoop(isRunning, (timePassed) => {
const progress = Math.min(timePassed / duration, 1);
ref.current.style.opacity = progress;
if (progress === 1) {
setIsRunning(false);
}
});
}
function useAnimationLoop(isRunning, drawFrame) {
const onFrame = useEffectEvent(drawFrame);
useEffect(() => {
if (!isRunning) {
return;
}
const startTime = performance.now();
let frameId = null;
function tick(now) {
const timePassed = now - startTime;
onFrame(timePassed);
frameId = requestAnimationFrame(tick);
}
tick();
return () => cancelAnimationFrame(frameId);
}, [isRunning]);
}
```
Show more
However, you didn’t *have to* do that. As with regular functions, ultimately you decide where to draw the boundaries between different parts of your code. You could also take a very different approach. Instead of keeping the logic in the Effect, you could move most of the imperative logic inside a JavaScript [class:](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Classes)
App.jsuseFadeIn.jsanimation.js
useFadeIn.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import { useState, useEffect } from 'react';
import { FadeInAnimation } from './animation.js';
export function useFadeIn(ref, duration) {
useEffect(() => {
const animation = new FadeInAnimation(ref.current);
animation.start(duration);
return () => {
animation.stop();
};
}, [ref, duration]);
}
```
Effects let you connect React to external systems. The more coordination between Effects is needed (for example, to chain multiple animations), the more it makes sense to extract that logic out of Effects and Hooks *completely* like in the sandbox above. Then, the code you extracted *becomes* the “external system”. This lets your Effects stay simple because they only need to send messages to the system you’ve moved outside React.
The examples above assume that the fade-in logic needs to be written in JavaScript. However, this particular fade-in animation is both simpler and much more efficient to implement with a plain [CSS Animation:](https://developer.mozilla.org/en-US/docs/Web/CSS/CSS_Animations/Using_CSS_animations)
App.jswelcome.css
welcome.css
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
.welcome {
color: white;
padding: 50px;
text-align: center;
font-size: 50px;
background-image: radial-gradient(circle, rgba(63,94,251,1) 0%, rgba(252,70,107,1) 100%);
animation: fadeIn 1000ms;
}
@keyframes fadeIn {
0% { opacity: 0; }
100% { opacity: 1; }
}
```
Sometimes, you don’t even need a Hook!
## Recap[Link for Recap]()
- Custom Hooks let you share logic between components.
- Custom Hooks must be named starting with `use` followed by a capital letter.
- Custom Hooks only share stateful logic, not state itself.
- You can pass reactive values from one Hook to another, and they stay up-to-date.
- All Hooks re-run every time your component re-renders.
- The code of your custom Hooks should be pure, like your component’s code.
- Wrap event handlers received by custom Hooks into Effect Events.
- Don’t create custom Hooks like `useMount`. Keep their purpose specific.
- It’s up to you how and where to choose the boundaries of your code.
## Try out some challenges[Link for Try out some challenges]()
1\. Extract a `useCounter` Hook 2. Make the counter delay configurable 3. Extract `useInterval` out of `useCounter` 4. Fix a resetting interval 5. Implement a staggering movement
#### Challenge 1 of 5: Extract a `useCounter` Hook[Link for this heading]()
This component uses a state variable and an Effect to display a number that increments every second. Extract this logic into a custom Hook called `useCounter`. Your goal is to make the `Counter` component implementation look exactly like this:
```
export default function Counter() {
const count = useCounter();
return <h1>Seconds passed: {count}</h1>;
}
```
You’ll need to write your custom Hook in `useCounter.js` and import it into the `App.js` file.
App.jsuseCounter.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import { useState, useEffect } from 'react';
export default function Counter() {
const [count, setCount] = useState(0);
useEffect(() => {
const id = setInterval(() => {
setCount(c => c + 1);
}, 1000);
return () => clearInterval(id);
}, []);
return <h1>Seconds passed: {count}</h1>;
}
```
Show solutionNext Challenge
[PreviousRemoving Effect Dependencies](https://react.dev/learn/removing-effect-dependencies) |
https://react.dev/learn/lifecycle-of-reactive-effects | [Learn React](https://react.dev/learn)
[Escape Hatches](https://react.dev/learn/escape-hatches)
# Lifecycle of Reactive Effects[Link for this heading]()
Effects have a different lifecycle from components. Components may mount, update, or unmount. An Effect can only do two things: to start synchronizing something, and later to stop synchronizing it. This cycle can happen multiple times if your Effect depends on props and state that change over time. React provides a linter rule to check that you’ve specified your Effect’s dependencies correctly. This keeps your Effect synchronized to the latest props and state.
### You will learn
- How an Effect’s lifecycle is different from a component’s lifecycle
- How to think about each individual Effect in isolation
- When your Effect needs to re-synchronize, and why
- How your Effect’s dependencies are determined
- What it means for a value to be reactive
- What an empty dependency array means
- How React verifies your dependencies are correct with a linter
- What to do when you disagree with the linter
## The lifecycle of an Effect[Link for The lifecycle of an Effect]()
Every React component goes through the same lifecycle:
- A component *mounts* when it’s added to the screen.
- A component *updates* when it receives new props or state, usually in response to an interaction.
- A component *unmounts* when it’s removed from the screen.
**It’s a good way to think about components, but *not* about Effects.** Instead, try to think about each Effect independently from your component’s lifecycle. An Effect describes how to [synchronize an external system](https://react.dev/learn/synchronizing-with-effects) to the current props and state. As your code changes, synchronization will need to happen more or less often.
To illustrate this point, consider this Effect connecting your component to a chat server:
```
const serverUrl = 'https://localhost:1234';
function ChatRoom({ roomId }) {
useEffect(() => {
const connection = createConnection(serverUrl, roomId);
connection.connect();
return () => {
connection.disconnect();
};
}, [roomId]);
// ...
}
```
Your Effect’s body specifies how to **start synchronizing:**
```
// ...
const connection = createConnection(serverUrl, roomId);
connection.connect();
return () => {
connection.disconnect();
};
// ...
```
The cleanup function returned by your Effect specifies how to **stop synchronizing:**
```
// ...
const connection = createConnection(serverUrl, roomId);
connection.connect();
return () => {
connection.disconnect();
};
// ...
```
Intuitively, you might think that React would **start synchronizing** when your component mounts and **stop synchronizing** when your component unmounts. However, this is not the end of the story! Sometimes, it may also be necessary to **start and stop synchronizing multiple times** while the component remains mounted.
Let’s look at *why* this is necessary, *when* it happens, and *how* you can control this behavior.
### Note
Some Effects don’t return a cleanup function at all. [More often than not,](https://react.dev/learn/synchronizing-with-effects) you’ll want to return one—but if you don’t, React will behave as if you returned an empty cleanup function.
### Why synchronization may need to happen more than once[Link for Why synchronization may need to happen more than once]()
Imagine this `ChatRoom` component receives a `roomId` prop that the user picks in a dropdown. Let’s say that initially the user picks the `"general"` room as the `roomId`. Your app displays the `"general"` chat room:
```
const serverUrl = 'https://localhost:1234';
function ChatRoom({ roomId /* "general" */ }) {
// ...
return <h1>Welcome to the {roomId} room!</h1>;
}
```
After the UI is displayed, React will run your Effect to **start synchronizing.** It connects to the `"general"` room:
```
function ChatRoom({ roomId /* "general" */ }) {
useEffect(() => {
const connection = createConnection(serverUrl, roomId); // Connects to the "general" room
connection.connect();
return () => {
connection.disconnect(); // Disconnects from the "general" room
};
}, [roomId]);
// ...
```
So far, so good.
Later, the user picks a different room in the dropdown (for example, `"travel"`). First, React will update the UI:
```
function ChatRoom({ roomId /* "travel" */ }) {
// ...
return <h1>Welcome to the {roomId} room!</h1>;
}
```
Think about what should happen next. The user sees that `"travel"` is the selected chat room in the UI. However, the Effect that ran the last time is still connected to the `"general"` room. **The `roomId` prop has changed, so what your Effect did back then (connecting to the `"general"` room) no longer matches the UI.**
At this point, you want React to do two things:
1. Stop synchronizing with the old `roomId` (disconnect from the `"general"` room)
2. Start synchronizing with the new `roomId` (connect to the `"travel"` room)
**Luckily, you’ve already taught React how to do both of these things!** Your Effect’s body specifies how to start synchronizing, and your cleanup function specifies how to stop synchronizing. All that React needs to do now is to call them in the correct order and with the correct props and state. Let’s see how exactly that happens.
### How React re-synchronizes your Effect[Link for How React re-synchronizes your Effect]()
Recall that your `ChatRoom` component has received a new value for its `roomId` prop. It used to be `"general"`, and now it is `"travel"`. React needs to re-synchronize your Effect to re-connect you to a different room.
To **stop synchronizing,** React will call the cleanup function that your Effect returned after connecting to the `"general"` room. Since `roomId` was `"general"`, the cleanup function disconnects from the `"general"` room:
```
function ChatRoom({ roomId /* "general" */ }) {
useEffect(() => {
const connection = createConnection(serverUrl, roomId); // Connects to the "general" room
connection.connect();
return () => {
connection.disconnect(); // Disconnects from the "general" room
};
// ...
```
Then React will run the Effect that you’ve provided during this render. This time, `roomId` is `"travel"` so it will **start synchronizing** to the `"travel"` chat room (until its cleanup function is eventually called too):
```
function ChatRoom({ roomId /* "travel" */ }) {
useEffect(() => {
const connection = createConnection(serverUrl, roomId); // Connects to the "travel" room
connection.connect();
// ...
```
Thanks to this, you’re now connected to the same room that the user chose in the UI. Disaster averted!
Every time after your component re-renders with a different `roomId`, your Effect will re-synchronize. For example, let’s say the user changes `roomId` from `"travel"` to `"music"`. React will again **stop synchronizing** your Effect by calling its cleanup function (disconnecting you from the `"travel"` room). Then it will **start synchronizing** again by running its body with the new `roomId` prop (connecting you to the `"music"` room).
Finally, when the user goes to a different screen, `ChatRoom` unmounts. Now there is no need to stay connected at all. React will **stop synchronizing** your Effect one last time and disconnect you from the `"music"` chat room.
### Thinking from the Effect’s perspective[Link for Thinking from the Effect’s perspective]()
Let’s recap everything that’s happened from the `ChatRoom` component’s perspective:
1. `ChatRoom` mounted with `roomId` set to `"general"`
2. `ChatRoom` updated with `roomId` set to `"travel"`
3. `ChatRoom` updated with `roomId` set to `"music"`
4. `ChatRoom` unmounted
During each of these points in the component’s lifecycle, your Effect did different things:
1. Your Effect connected to the `"general"` room
2. Your Effect disconnected from the `"general"` room and connected to the `"travel"` room
3. Your Effect disconnected from the `"travel"` room and connected to the `"music"` room
4. Your Effect disconnected from the `"music"` room
Now let’s think about what happened from the perspective of the Effect itself:
```
useEffect(() => {
// Your Effect connected to the room specified with roomId...
const connection = createConnection(serverUrl, roomId);
connection.connect();
return () => {
// ...until it disconnected
connection.disconnect();
};
}, [roomId]);
```
This code’s structure might inspire you to see what happened as a sequence of non-overlapping time periods:
1. Your Effect connected to the `"general"` room (until it disconnected)
2. Your Effect connected to the `"travel"` room (until it disconnected)
3. Your Effect connected to the `"music"` room (until it disconnected)
Previously, you were thinking from the component’s perspective. When you looked from the component’s perspective, it was tempting to think of Effects as “callbacks” or “lifecycle events” that fire at a specific time like “after a render” or “before unmount”. This way of thinking gets complicated very fast, so it’s best to avoid.
**Instead, always focus on a single start/stop cycle at a time. It shouldn’t matter whether a component is mounting, updating, or unmounting. All you need to do is to describe how to start synchronization and how to stop it. If you do it well, your Effect will be resilient to being started and stopped as many times as it’s needed.**
This might remind you how you don’t think whether a component is mounting or updating when you write the rendering logic that creates JSX. You describe what should be on the screen, and React [figures out the rest.](https://react.dev/learn/reacting-to-input-with-state)
### How React verifies that your Effect can re-synchronize[Link for How React verifies that your Effect can re-synchronize]()
Here is a live example that you can play with. Press “Open chat” to mount the `ChatRoom` component:
App.jschat.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import { useState, useEffect } from 'react';
import { createConnection } from './chat.js';
const serverUrl = 'https://localhost:1234';
function ChatRoom({ roomId }) {
useEffect(() => {
const connection = createConnection(serverUrl, roomId);
connection.connect();
return () => connection.disconnect();
}, [roomId]);
return <h1>Welcome to the {roomId} room!</h1>;
}
export default function App() {
const [roomId, setRoomId] = useState('general');
const [show, setShow] = useState(false);
return (
<>
<label>
Choose the chat room:{' '}
<select
value={roomId}
onChange={e => setRoomId(e.target.value)}
>
<option value="general">general</option>
<option value="travel">travel</option>
<option value="music">music</option>
</select>
</label>
<button onClick={() => setShow(!show)}>
{show ? 'Close chat' : 'Open chat'}
</button>
{show && <hr />}
{show && <ChatRoom roomId={roomId} />}
</>
);
}
```
Show more
Notice that when the component mounts for the first time, you see three logs:
1. `✅ Connecting to "general" room at https://localhost:1234...` *(development-only)*
2. `❌ Disconnected from "general" room at https://localhost:1234.` *(development-only)*
3. `✅ Connecting to "general" room at https://localhost:1234...`
The first two logs are development-only. In development, React always remounts each component once.
**React verifies that your Effect can re-synchronize by forcing it to do that immediately in development.** This might remind you of opening a door and closing it an extra time to check if the door lock works. React starts and stops your Effect one extra time in development to check [you’ve implemented its cleanup well.](https://react.dev/learn/synchronizing-with-effects)
The main reason your Effect will re-synchronize in practice is if some data it uses has changed. In the sandbox above, change the selected chat room. Notice how, when the `roomId` changes, your Effect re-synchronizes.
However, there are also more unusual cases in which re-synchronization is necessary. For example, try editing the `serverUrl` in the sandbox above while the chat is open. Notice how the Effect re-synchronizes in response to your edits to the code. In the future, React may add more features that rely on re-synchronization.
### How React knows that it needs to re-synchronize the Effect[Link for How React knows that it needs to re-synchronize the Effect]()
You might be wondering how React knew that your Effect needed to re-synchronize after `roomId` changes. It’s because *you told React* that its code depends on `roomId` by including it in the [list of dependencies:](https://react.dev/learn/synchronizing-with-effects)
```
function ChatRoom({ roomId }) { // The roomId prop may change over time
useEffect(() => {
const connection = createConnection(serverUrl, roomId); // This Effect reads roomId
connection.connect();
return () => {
connection.disconnect();
};
}, [roomId]); // So you tell React that this Effect "depends on" roomId
// ...
```
Here’s how this works:
1. You knew `roomId` is a prop, which means it can change over time.
2. You knew that your Effect reads `roomId` (so its logic depends on a value that may change later).
3. This is why you specified it as your Effect’s dependency (so that it re-synchronizes when `roomId` changes).
Every time after your component re-renders, React will look at the array of dependencies that you have passed. If any of the values in the array is different from the value at the same spot that you passed during the previous render, React will re-synchronize your Effect.
For example, if you passed `["general"]` during the initial render, and later you passed `["travel"]` during the next render, React will compare `"general"` and `"travel"`. These are different values (compared with [`Object.is`](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Object/is)), so React will re-synchronize your Effect. On the other hand, if your component re-renders but `roomId` has not changed, your Effect will remain connected to the same room.
### Each Effect represents a separate synchronization process[Link for Each Effect represents a separate synchronization process]()
Resist adding unrelated logic to your Effect only because this logic needs to run at the same time as an Effect you already wrote. For example, let’s say you want to send an analytics event when the user visits the room. You already have an Effect that depends on `roomId`, so you might feel tempted to add the analytics call there:
```
function ChatRoom({ roomId }) {
useEffect(() => {
logVisit(roomId);
const connection = createConnection(serverUrl, roomId);
connection.connect();
return () => {
connection.disconnect();
};
}, [roomId]);
// ...
}
```
But imagine you later add another dependency to this Effect that needs to re-establish the connection. If this Effect re-synchronizes, it will also call `logVisit(roomId)` for the same room, which you did not intend. Logging the visit **is a separate process** from connecting. Write them as two separate Effects:
```
function ChatRoom({ roomId }) {
useEffect(() => {
logVisit(roomId);
}, [roomId]);
useEffect(() => {
const connection = createConnection(serverUrl, roomId);
// ...
}, [roomId]);
// ...
}
```
**Each Effect in your code should represent a separate and independent synchronization process.**
In the above example, deleting one Effect wouldn’t break the other Effect’s logic. This is a good indication that they synchronize different things, and so it made sense to split them up. On the other hand, if you split up a cohesive piece of logic into separate Effects, the code may look “cleaner” but will be [more difficult to maintain.](https://react.dev/learn/you-might-not-need-an-effect) This is why you should think whether the processes are same or separate, not whether the code looks cleaner.
## Effects “react” to reactive values[Link for Effects “react” to reactive values]()
Your Effect reads two variables (`serverUrl` and `roomId`), but you only specified `roomId` as a dependency:
```
const serverUrl = 'https://localhost:1234';
function ChatRoom({ roomId }) {
useEffect(() => {
const connection = createConnection(serverUrl, roomId);
connection.connect();
return () => {
connection.disconnect();
};
}, [roomId]);
// ...
}
```
Why doesn’t `serverUrl` need to be a dependency?
This is because the `serverUrl` never changes due to a re-render. It’s always the same no matter how many times the component re-renders and why. Since `serverUrl` never changes, it wouldn’t make sense to specify it as a dependency. After all, dependencies only do something when they change over time!
On the other hand, `roomId` may be different on a re-render. **Props, state, and other values declared inside the component are *reactive* because they’re calculated during rendering and participate in the React data flow.**
If `serverUrl` was a state variable, it would be reactive. Reactive values must be included in dependencies:
```
function ChatRoom({ roomId }) { // Props change over time
const [serverUrl, setServerUrl] = useState('https://localhost:1234'); // State may change over time
useEffect(() => {
const connection = createConnection(serverUrl, roomId); // Your Effect reads props and state
connection.connect();
return () => {
connection.disconnect();
};
}, [roomId, serverUrl]); // So you tell React that this Effect "depends on" on props and state
// ...
}
```
By including `serverUrl` as a dependency, you ensure that the Effect re-synchronizes after it changes.
Try changing the selected chat room or edit the server URL in this sandbox:
App.jschat.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import { useState, useEffect } from 'react';
import { createConnection } from './chat.js';
function ChatRoom({ roomId }) {
const [serverUrl, setServerUrl] = useState('https://localhost:1234');
useEffect(() => {
const connection = createConnection(serverUrl, roomId);
connection.connect();
return () => connection.disconnect();
}, [roomId, serverUrl]);
return (
<>
<label>
Server URL:{' '}
<input
value={serverUrl}
onChange={e => setServerUrl(e.target.value)}
/>
</label>
<h1>Welcome to the {roomId} room!</h1>
</>
);
}
export default function App() {
const [roomId, setRoomId] = useState('general');
return (
<>
<label>
Choose the chat room:{' '}
<select
value={roomId}
onChange={e => setRoomId(e.target.value)}
>
<option value="general">general</option>
<option value="travel">travel</option>
<option value="music">music</option>
</select>
</label>
<hr />
<ChatRoom roomId={roomId} />
</>
);
}
```
Show more
Whenever you change a reactive value like `roomId` or `serverUrl`, the Effect re-connects to the chat server.
### What an Effect with empty dependencies means[Link for What an Effect with empty dependencies means]()
What happens if you move both `serverUrl` and `roomId` outside the component?
```
const serverUrl = 'https://localhost:1234';
const roomId = 'general';
function ChatRoom() {
useEffect(() => {
const connection = createConnection(serverUrl, roomId);
connection.connect();
return () => {
connection.disconnect();
};
}, []); // ✅ All dependencies declared
// ...
}
```
Now your Effect’s code does not use *any* reactive values, so its dependencies can be empty (`[]`).
Thinking from the component’s perspective, the empty `[]` dependency array means this Effect connects to the chat room only when the component mounts, and disconnects only when the component unmounts. (Keep in mind that React would still [re-synchronize it an extra time]() in development to stress-test your logic.)
App.jschat.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import { useState, useEffect } from 'react';
import { createConnection } from './chat.js';
const serverUrl = 'https://localhost:1234';
const roomId = 'general';
function ChatRoom() {
useEffect(() => {
const connection = createConnection(serverUrl, roomId);
connection.connect();
return () => connection.disconnect();
}, []);
return <h1>Welcome to the {roomId} room!</h1>;
}
export default function App() {
const [show, setShow] = useState(false);
return (
<>
<button onClick={() => setShow(!show)}>
{show ? 'Close chat' : 'Open chat'}
</button>
{show && <hr />}
{show && <ChatRoom />}
</>
);
}
```
Show more
However, if you [think from the Effect’s perspective,]() you don’t need to think about mounting and unmounting at all. What’s important is you’ve specified what your Effect does to start and stop synchronizing. Today, it has no reactive dependencies. But if you ever want the user to change `roomId` or `serverUrl` over time (and they would become reactive), your Effect’s code won’t change. You will only need to add them to the dependencies.
### All variables declared in the component body are reactive[Link for All variables declared in the component body are reactive]()
Props and state aren’t the only reactive values. Values that you calculate from them are also reactive. If the props or state change, your component will re-render, and the values calculated from them will also change. This is why all variables from the component body used by the Effect should be in the Effect dependency list.
Let’s say that the user can pick a chat server in the dropdown, but they can also configure a default server in settings. Suppose you’ve already put the settings state in a [context](https://react.dev/learn/scaling-up-with-reducer-and-context) so you read the `settings` from that context. Now you calculate the `serverUrl` based on the selected server from props and the default server:
```
function ChatRoom({ roomId, selectedServerUrl }) { // roomId is reactive
const settings = useContext(SettingsContext); // settings is reactive
const serverUrl = selectedServerUrl ?? settings.defaultServerUrl; // serverUrl is reactive
useEffect(() => {
const connection = createConnection(serverUrl, roomId); // Your Effect reads roomId and serverUrl
connection.connect();
return () => {
connection.disconnect();
};
}, [roomId, serverUrl]); // So it needs to re-synchronize when either of them changes!
// ...
}
```
In this example, `serverUrl` is not a prop or a state variable. It’s a regular variable that you calculate during rendering. But it’s calculated during rendering, so it can change due to a re-render. This is why it’s reactive.
**All values inside the component (including props, state, and variables in your component’s body) are reactive. Any reactive value can change on a re-render, so you need to include reactive values as Effect’s dependencies.**
In other words, Effects “react” to all values from the component body.
##### Deep Dive
#### Can global or mutable values be dependencies?[Link for Can global or mutable values be dependencies?]()
Show Details
Mutable values (including global variables) aren’t reactive.
**A mutable value like [`location.pathname`](https://developer.mozilla.org/en-US/docs/Web/API/Location/pathname) can’t be a dependency.** It’s mutable, so it can change at any time completely outside of the React rendering data flow. Changing it wouldn’t trigger a re-render of your component. Therefore, even if you specified it in the dependencies, React *wouldn’t know* to re-synchronize the Effect when it changes. This also breaks the rules of React because reading mutable data during rendering (which is when you calculate the dependencies) breaks [purity of rendering.](https://react.dev/learn/keeping-components-pure) Instead, you should read and subscribe to an external mutable value with [`useSyncExternalStore`.](https://react.dev/learn/you-might-not-need-an-effect)
**A mutable value like [`ref.current`](https://react.dev/reference/react/useRef) or things you read from it also can’t be a dependency.** The ref object returned by `useRef` itself can be a dependency, but its `current` property is intentionally mutable. It lets you [keep track of something without triggering a re-render.](https://react.dev/learn/referencing-values-with-refs) But since changing it doesn’t trigger a re-render, it’s not a reactive value, and React won’t know to re-run your Effect when it changes.
As you’ll learn below on this page, a linter will check for these issues automatically.
### React verifies that you specified every reactive value as a dependency[Link for React verifies that you specified every reactive value as a dependency]()
If your linter is [configured for React,](https://react.dev/learn/editor-setup) it will check that every reactive value used by your Effect’s code is declared as its dependency. For example, this is a lint error because both `roomId` and `serverUrl` are reactive:
App.jschat.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import { useState, useEffect } from 'react';
import { createConnection } from './chat.js';
function ChatRoom({ roomId }) { // roomId is reactive
const [serverUrl, setServerUrl] = useState('https://localhost:1234'); // serverUrl is reactive
useEffect(() => {
const connection = createConnection(serverUrl, roomId);
connection.connect();
return () => connection.disconnect();
}, []); // <-- Something's wrong here!
return (
<>
<label>
Server URL:{' '}
<input
value={serverUrl}
onChange={e => setServerUrl(e.target.value)}
/>
</label>
<h1>Welcome to the {roomId} room!</h1>
</>
);
}
export default function App() {
const [roomId, setRoomId] = useState('general');
return (
<>
<label>
Choose the chat room:{' '}
<select
value={roomId}
onChange={e => setRoomId(e.target.value)}
>
<option value="general">general</option>
<option value="travel">travel</option>
<option value="music">music</option>
</select>
</label>
<hr />
<ChatRoom roomId={roomId} />
</>
);
}
```
Show more
This may look like a React error, but really React is pointing out a bug in your code. Both `roomId` and `serverUrl` may change over time, but you’re forgetting to re-synchronize your Effect when they change. You will remain connected to the initial `roomId` and `serverUrl` even after the user picks different values in the UI.
To fix the bug, follow the linter’s suggestion to specify `roomId` and `serverUrl` as dependencies of your Effect:
```
function ChatRoom({ roomId }) { // roomId is reactive
const [serverUrl, setServerUrl] = useState('https://localhost:1234'); // serverUrl is reactive
useEffect(() => {
const connection = createConnection(serverUrl, roomId);
connection.connect();
return () => {
connection.disconnect();
};
}, [serverUrl, roomId]); // ✅ All dependencies declared
// ...
}
```
Try this fix in the sandbox above. Verify that the linter error is gone, and the chat re-connects when needed.
### Note
In some cases, React *knows* that a value never changes even though it’s declared inside the component. For example, the [`set` function](https://react.dev/reference/react/useState) returned from `useState` and the ref object returned by [`useRef`](https://react.dev/reference/react/useRef) are *stable*—they are guaranteed to not change on a re-render. Stable values aren’t reactive, so you may omit them from the list. Including them is allowed: they won’t change, so it doesn’t matter.
### What to do when you don’t want to re-synchronize[Link for What to do when you don’t want to re-synchronize]()
In the previous example, you’ve fixed the lint error by listing `roomId` and `serverUrl` as dependencies.
**However, you could instead “prove” to the linter that these values aren’t reactive values,** i.e. that they *can’t* change as a result of a re-render. For example, if `serverUrl` and `roomId` don’t depend on rendering and always have the same values, you can move them outside the component. Now they don’t need to be dependencies:
```
const serverUrl = 'https://localhost:1234'; // serverUrl is not reactive
const roomId = 'general'; // roomId is not reactive
function ChatRoom() {
useEffect(() => {
const connection = createConnection(serverUrl, roomId);
connection.connect();
return () => {
connection.disconnect();
};
}, []); // ✅ All dependencies declared
// ...
}
```
You can also move them *inside the Effect.* They aren’t calculated during rendering, so they’re not reactive:
```
function ChatRoom() {
useEffect(() => {
const serverUrl = 'https://localhost:1234'; // serverUrl is not reactive
const roomId = 'general'; // roomId is not reactive
const connection = createConnection(serverUrl, roomId);
connection.connect();
return () => {
connection.disconnect();
};
}, []); // ✅ All dependencies declared
// ...
}
```
**Effects are reactive blocks of code.** They re-synchronize when the values you read inside of them change. Unlike event handlers, which only run once per interaction, Effects run whenever synchronization is necessary.
**You can’t “choose” your dependencies.** Your dependencies must include every [reactive value]() you read in the Effect. The linter enforces this. Sometimes this may lead to problems like infinite loops and to your Effect re-synchronizing too often. Don’t fix these problems by suppressing the linter! Here’s what to try instead:
- **Check that your Effect represents an independent synchronization process.** If your Effect doesn’t synchronize anything, [it might be unnecessary.](https://react.dev/learn/you-might-not-need-an-effect) If it synchronizes several independent things, [split it up.]()
- **If you want to read the latest value of props or state without “reacting” to it and re-synchronizing the Effect,** you can split your Effect into a reactive part (which you’ll keep in the Effect) and a non-reactive part (which you’ll extract into something called an *Effect Event*). [Read about separating Events from Effects.](https://react.dev/learn/separating-events-from-effects)
- **Avoid relying on objects and functions as dependencies.** If you create objects and functions during rendering and then read them from an Effect, they will be different on every render. This will cause your Effect to re-synchronize every time. [Read more about removing unnecessary dependencies from Effects.](https://react.dev/learn/removing-effect-dependencies)
### Pitfall
The linter is your friend, but its powers are limited. The linter only knows when the dependencies are *wrong*. It doesn’t know *the best* way to solve each case. If the linter suggests a dependency, but adding it causes a loop, it doesn’t mean the linter should be ignored. You need to change the code inside (or outside) the Effect so that that value isn’t reactive and doesn’t *need* to be a dependency.
If you have an existing codebase, you might have some Effects that suppress the linter like this:
```
useEffect(() => {
// ...
// 🔴 Avoid suppressing the linter like this:
// eslint-ignore-next-line react-hooks/exhaustive-deps
}, []);
```
On the [next](https://react.dev/learn/separating-events-from-effects) [pages](https://react.dev/learn/removing-effect-dependencies), you’ll learn how to fix this code without breaking the rules. It’s always worth fixing!
## Recap[Link for Recap]()
- Components can mount, update, and unmount.
- Each Effect has a separate lifecycle from the surrounding component.
- Each Effect describes a separate synchronization process that can *start* and *stop*.
- When you write and read Effects, think from each individual Effect’s perspective (how to start and stop synchronization) rather than from the component’s perspective (how it mounts, updates, or unmounts).
- Values declared inside the component body are “reactive”.
- Reactive values should re-synchronize the Effect because they can change over time.
- The linter verifies that all reactive values used inside the Effect are specified as dependencies.
- All errors flagged by the linter are legitimate. There’s always a way to fix the code to not break the rules.
## Try out some challenges[Link for Try out some challenges]()
1\. Fix reconnecting on every keystroke 2. Switch synchronization on and off 3. Investigate a stale value bug 4. Fix a connection switch 5. Populate a chain of select boxes
#### Challenge 1 of 5: Fix reconnecting on every keystroke[Link for this heading]()
In this example, the `ChatRoom` component connects to the chat room when the component mounts, disconnects when it unmounts, and reconnects when you select a different chat room. This behavior is correct, so you need to keep it working.
However, there is a problem. Whenever you type into the message box input at the bottom, `ChatRoom` *also* reconnects to the chat. (You can notice this by clearing the console and typing into the input.) Fix the issue so that this doesn’t happen.
App.jschat.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import { useState, useEffect } from 'react';
import { createConnection } from './chat.js';
const serverUrl = 'https://localhost:1234';
function ChatRoom({ roomId }) {
const [message, setMessage] = useState('');
useEffect(() => {
const connection = createConnection(serverUrl, roomId);
connection.connect();
return () => connection.disconnect();
});
return (
<>
<h1>Welcome to the {roomId} room!</h1>
<input
value={message}
onChange={e => setMessage(e.target.value)}
/>
</>
);
}
export default function App() {
const [roomId, setRoomId] = useState('general');
return (
<>
<label>
Choose the chat room:{' '}
<select
value={roomId}
onChange={e => setRoomId(e.target.value)}
>
<option value="general">general</option>
<option value="travel">travel</option>
<option value="music">music</option>
</select>
</label>
<hr />
<ChatRoom roomId={roomId} />
</>
);
}
```
Show more
Show hint Show solution
Next Challenge
[PreviousYou Might Not Need an Effect](https://react.dev/learn/you-might-not-need-an-effect)
[NextSeparating Events from Effects](https://react.dev/learn/separating-events-from-effects) |
https://react.dev/reference/react-dom/client/hydrateRoot#hydrating-server-rendered-html | [API Reference](https://react.dev/reference/react)
[Client APIs](https://react.dev/reference/react-dom/client)
# hydrateRoot[Link for this heading]()
`hydrateRoot` lets you display React components inside a browser DOM node whose HTML content was previously generated by [`react-dom/server`.](https://react.dev/reference/react-dom/server)
```
const root = hydrateRoot(domNode, reactNode, options?)
```
- [Reference]()
- [`hydrateRoot(domNode, reactNode, options?)`]()
- [`root.render(reactNode)`]()
- [`root.unmount()`]()
- [Usage]()
- [Hydrating server-rendered HTML]()
- [Hydrating an entire document]()
- [Suppressing unavoidable hydration mismatch errors]()
- [Handling different client and server content]()
- [Updating a hydrated root component]()
- [Show a dialog for uncaught errors]()
- [Displaying Error Boundary errors]()
- [Show a dialog for recoverable hydration mismatch errors]()
- [Troubleshooting]()
- [I’m getting an error: “You passed a second argument to root.render”]()
* * *
## Reference[Link for Reference]()
### `hydrateRoot(domNode, reactNode, options?)`[Link for this heading]()
Call `hydrateRoot` to “attach” React to existing HTML that was already rendered by React in a server environment.
```
import { hydrateRoot } from 'react-dom/client';
const domNode = document.getElementById('root');
const root = hydrateRoot(domNode, reactNode);
```
React will attach to the HTML that exists inside the `domNode`, and take over managing the DOM inside it. An app fully built with React will usually only have one `hydrateRoot` call with its root component.
[See more examples below.]()
#### Parameters[Link for Parameters]()
- `domNode`: A [DOM element](https://developer.mozilla.org/en-US/docs/Web/API/Element) that was rendered as the root element on the server.
- `reactNode`: The “React node” used to render the existing HTML. This will usually be a piece of JSX like `<App />` which was rendered with a `ReactDOM Server` method such as `renderToPipeableStream(<App />)`.
- **optional** `options`: An object with options for this React root.
- **optional** `onCaughtError`: Callback called when React catches an error in an Error Boundary. Called with the `error` caught by the Error Boundary, and an `errorInfo` object containing the `componentStack`.
- **optional** `onUncaughtError`: Callback called when an error is thrown and not caught by an Error Boundary. Called with the `error` that was thrown and an `errorInfo` object containing the `componentStack`.
- **optional** `onRecoverableError`: Callback called when React automatically recovers from errors. Called with the `error` React throws, and an `errorInfo` object containing the `componentStack`. Some recoverable errors may include the original error cause as `error.cause`.
- **optional** `identifierPrefix`: A string prefix React uses for IDs generated by [`useId`.](https://react.dev/reference/react/useId) Useful to avoid conflicts when using multiple roots on the same page. Must be the same prefix as used on the server.
#### Returns[Link for Returns]()
`hydrateRoot` returns an object with two methods: [`render`]() and [`unmount`.]()
#### Caveats[Link for Caveats]()
- `hydrateRoot()` expects the rendered content to be identical with the server-rendered content. You should treat mismatches as bugs and fix them.
- In development mode, React warns about mismatches during hydration. There are no guarantees that attribute differences will be patched up in case of mismatches. This is important for performance reasons because in most apps, mismatches are rare, and so validating all markup would be prohibitively expensive.
- You’ll likely have only one `hydrateRoot` call in your app. If you use a framework, it might do this call for you.
- If your app is client-rendered with no HTML rendered already, using `hydrateRoot()` is not supported. Use [`createRoot()`](https://react.dev/reference/react-dom/client/createRoot) instead.
* * *
### `root.render(reactNode)`[Link for this heading]()
Call `root.render` to update a React component inside a hydrated React root for a browser DOM element.
```
root.render(<App />);
```
React will update `<App />` in the hydrated `root`.
[See more examples below.]()
#### Parameters[Link for Parameters]()
- `reactNode`: A “React node” that you want to update. This will usually be a piece of JSX like `<App />`, but you can also pass a React element constructed with [`createElement()`](https://react.dev/reference/react/createElement), a string, a number, `null`, or `undefined`.
#### Returns[Link for Returns]()
`root.render` returns `undefined`.
#### Caveats[Link for Caveats]()
- If you call `root.render` before the root has finished hydrating, React will clear the existing server-rendered HTML content and switch the entire root to client rendering.
* * *
### `root.unmount()`[Link for this heading]()
Call `root.unmount` to destroy a rendered tree inside a React root.
```
root.unmount();
```
An app fully built with React will usually not have any calls to `root.unmount`.
This is mostly useful if your React root’s DOM node (or any of its ancestors) may get removed from the DOM by some other code. For example, imagine a jQuery tab panel that removes inactive tabs from the DOM. If a tab gets removed, everything inside it (including the React roots inside) would get removed from the DOM as well. You need to tell React to “stop” managing the removed root’s content by calling `root.unmount`. Otherwise, the components inside the removed root won’t clean up and free up resources like subscriptions.
Calling `root.unmount` will unmount all the components in the root and “detach” React from the root DOM node, including removing any event handlers or state in the tree.
#### Parameters[Link for Parameters]()
`root.unmount` does not accept any parameters.
#### Returns[Link for Returns]()
`root.unmount` returns `undefined`.
#### Caveats[Link for Caveats]()
- Calling `root.unmount` will unmount all the components in the tree and “detach” React from the root DOM node.
- Once you call `root.unmount` you cannot call `root.render` again on the root. Attempting to call `root.render` on an unmounted root will throw a “Cannot update an unmounted root” error.
* * *
## Usage[Link for Usage]()
### Hydrating server-rendered HTML[Link for Hydrating server-rendered HTML]()
If your app’s HTML was generated by [`react-dom/server`](https://react.dev/reference/react-dom/client/createRoot), you need to *hydrate* it on the client.
```
import { hydrateRoot } from 'react-dom/client';
hydrateRoot(document.getElementById('root'), <App />);
```
This will hydrate the server HTML inside the browser DOM node with the React component for your app. Usually, you will do it once at startup. If you use a framework, it might do this behind the scenes for you.
To hydrate your app, React will “attach” your components’ logic to the initial generated HTML from the server. Hydration turns the initial HTML snapshot from the server into a fully interactive app that runs in the browser.
index.jsindex.htmlApp.js
index.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import './styles.css';
import { hydrateRoot } from 'react-dom/client';
import App from './App.js';
hydrateRoot(
document.getElementById('root'),
<App />
);
```
You shouldn’t need to call `hydrateRoot` again or to call it in more places. From this point on, React will be managing the DOM of your application. To update the UI, your components will [use state](https://react.dev/reference/react/useState) instead.
### Pitfall
The React tree you pass to `hydrateRoot` needs to produce **the same output** as it did on the server.
This is important for the user experience. The user will spend some time looking at the server-generated HTML before your JavaScript code loads. Server rendering creates an illusion that the app loads faster by showing the HTML snapshot of its output. Suddenly showing different content breaks that illusion. This is why the server render output must match the initial render output on the client.
The most common causes leading to hydration errors include:
- Extra whitespace (like newlines) around the React-generated HTML inside the root node.
- Using checks like `typeof window !== 'undefined'` in your rendering logic.
- Using browser-only APIs like [`window.matchMedia`](https://developer.mozilla.org/en-US/docs/Web/API/Window/matchMedia) in your rendering logic.
- Rendering different data on the server and the client.
React recovers from some hydration errors, but **you must fix them like other bugs.** In the best case, they’ll lead to a slowdown; in the worst case, event handlers can get attached to the wrong elements.
* * *
### Hydrating an entire document[Link for Hydrating an entire document]()
Apps fully built with React can render the entire document as JSX, including the [`<html>`](https://developer.mozilla.org/en-US/docs/Web/HTML/Element/html) tag:
```
function App() {
return (
<html>
<head>
<meta charSet="utf-8" />
<meta name="viewport" content="width=device-width, initial-scale=1" />
<link rel="stylesheet" href="/styles.css"></link>
<title>My app</title>
</head>
<body>
<Router />
</body>
</html>
);
}
```
To hydrate the entire document, pass the [`document`](https://developer.mozilla.org/en-US/docs/Web/API/Window/document) global as the first argument to `hydrateRoot`:
```
import { hydrateRoot } from 'react-dom/client';
import App from './App.js';
hydrateRoot(document, <App />);
```
* * *
### Suppressing unavoidable hydration mismatch errors[Link for Suppressing unavoidable hydration mismatch errors]()
If a single element’s attribute or text content is unavoidably different between the server and the client (for example, a timestamp), you may silence the hydration mismatch warning.
To silence hydration warnings on an element, add `suppressHydrationWarning={true}`:
index.jsindex.htmlApp.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
export default function App() {
return (
<h1 suppressHydrationWarning={true}>
Current Date: {new Date().toLocaleDateString()}
</h1>
);
}
```
This only works one level deep, and is intended to be an escape hatch. Don’t overuse it. Unless it’s text content, React still won’t attempt to patch it up, so it may remain inconsistent until future updates.
* * *
### Handling different client and server content[Link for Handling different client and server content]()
If you intentionally need to render something different on the server and the client, you can do a two-pass rendering. Components that render something different on the client can read a [state variable](https://react.dev/reference/react/useState) like `isClient`, which you can set to `true` in an [Effect](https://react.dev/reference/react/useEffect):
index.jsindex.htmlApp.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import { useState, useEffect } from "react";
export default function App() {
const [isClient, setIsClient] = useState(false);
useEffect(() => {
setIsClient(true);
}, []);
return (
<h1>
{isClient ? 'Is Client' : 'Is Server'}
</h1>
);
}
```
This way the initial render pass will render the same content as the server, avoiding mismatches, but an additional pass will happen synchronously right after hydration.
### Pitfall
This approach makes hydration slower because your components have to render twice. Be mindful of the user experience on slow connections. The JavaScript code may load significantly later than the initial HTML render, so rendering a different UI immediately after hydration may also feel jarring to the user.
* * *
### Updating a hydrated root component[Link for Updating a hydrated root component]()
After the root has finished hydrating, you can call [`root.render`]() to update the root React component. **Unlike with [`createRoot`](https://react.dev/reference/react-dom/client/createRoot), you don’t usually need to do this because the initial content was already rendered as HTML.**
If you call `root.render` at some point after hydration, and the component tree structure matches up with what was previously rendered, React will [preserve the state.](https://react.dev/learn/preserving-and-resetting-state) Notice how you can type in the input, which means that the updates from repeated `render` calls every second in this example are not destructive:
index.jsindex.htmlApp.js
index.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import { hydrateRoot } from 'react-dom/client';
import './styles.css';
import App from './App.js';
const root = hydrateRoot(
document.getElementById('root'),
<App counter={0} />
);
let i = 0;
setInterval(() => {
root.render(<App counter={i} />);
i++;
}, 1000);
```
It is uncommon to call [`root.render`]() on a hydrated root. Usually, you’ll [update state](https://react.dev/reference/react/useState) inside one of the components instead.
### Show a dialog for uncaught errors[Link for Show a dialog for uncaught errors]()
By default, React will log all uncaught errors to the console. To implement your own error reporting, you can provide the optional `onUncaughtError` root option:
```
import { hydrateRoot } from 'react-dom/client';
const root = hydrateRoot(
document.getElementById('root'),
<App />,
{
onUncaughtError: (error, errorInfo) => {
console.error(
'Uncaught error',
error,
errorInfo.componentStack
);
}
}
);
root.render(<App />);
```
The onUncaughtError option is a function called with two arguments:
1. The error that was thrown.
2. An errorInfo object that contains the componentStack of the error.
You can use the `onUncaughtError` root option to display error dialogs:
index.jsApp.js
index.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import { hydrateRoot } from "react-dom/client";
import App from "./App.js";
import {reportUncaughtError} from "./reportError";
import "./styles.css";
import {renderToString} from 'react-dom/server';
const container = document.getElementById("root");
const root = hydrateRoot(container, <App />, {
onUncaughtError: (error, errorInfo) => {
if (error.message !== 'Known error') {
reportUncaughtError({
error,
componentStack: errorInfo.componentStack
});
}
}
});
```
Show more
### Displaying Error Boundary errors[Link for Displaying Error Boundary errors]()
By default, React will log all errors caught by an Error Boundary to `console.error`. To override this behavior, you can provide the optional `onCaughtError` root option for errors caught by an [Error Boundary](https://react.dev/reference/react/Component):
```
import { hydrateRoot } from 'react-dom/client';
const root = hydrateRoot(
document.getElementById('root'),
<App />,
{
onCaughtError: (error, errorInfo) => {
console.error(
'Caught error',
error,
errorInfo.componentStack
);
}
}
);
root.render(<App />);
```
The onCaughtError option is a function called with two arguments:
1. The error that was caught by the boundary.
2. An errorInfo object that contains the componentStack of the error.
You can use the `onCaughtError` root option to display error dialogs or filter known errors from logging:
index.jsApp.js
index.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import { hydrateRoot } from "react-dom/client";
import App from "./App.js";
import {reportCaughtError} from "./reportError";
import "./styles.css";
const container = document.getElementById("root");
const root = hydrateRoot(container, <App />, {
onCaughtError: (error, errorInfo) => {
if (error.message !== 'Known error') {
reportCaughtError({
error,
componentStack: errorInfo.componentStack
});
}
}
});
```
Show more
### Show a dialog for recoverable hydration mismatch errors[Link for Show a dialog for recoverable hydration mismatch errors]()
When React encounters a hydration mismatch, it will automatically attempt to recover by rendering on the client. By default, React will log hydration mismatch errors to `console.error`. To override this behavior, you can provide the optional `onRecoverableError` root option:
```
import { hydrateRoot } from 'react-dom/client';
const root = hydrateRoot(
document.getElementById('root'),
<App />,
{
onRecoverableError: (error, errorInfo) => {
console.error(
'Caught error',
error,
error.cause,
errorInfo.componentStack
);
}
}
);
```
The onRecoverableError option is a function called with two arguments:
1. The error React throws. Some errors may include the original cause as error.cause.
2. An errorInfo object that contains the componentStack of the error.
You can use the `onRecoverableError` root option to display error dialogs for hydration mismatches:
index.jsApp.js
index.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import { hydrateRoot } from "react-dom/client";
import App from "./App.js";
import {reportRecoverableError} from "./reportError";
import "./styles.css";
const container = document.getElementById("root");
const root = hydrateRoot(container, <App />, {
onRecoverableError: (error, errorInfo) => {
reportRecoverableError({
error,
cause: error.cause,
componentStack: errorInfo.componentStack
});
}
});
```
## Troubleshooting[Link for Troubleshooting]()
### I’m getting an error: “You passed a second argument to root.render”[Link for I’m getting an error: “You passed a second argument to root.render”]()
A common mistake is to pass the options for `hydrateRoot` to `root.render(...)`:
Console
Warning: You passed a second argument to root.render(…) but it only accepts one argument.
To fix, pass the root options to `hydrateRoot(...)`, not `root.render(...)`:
```
// 🚩 Wrong: root.render only takes one argument.
root.render(App, {onUncaughtError});
// ✅ Correct: pass options to createRoot.
const root = hydrateRoot(container, <App />, {onUncaughtError});
```
[PreviouscreateRoot](https://react.dev/reference/react-dom/client/createRoot)
[NextServer APIs](https://react.dev/reference/react-dom/server) |
https://react.dev/warnings/react-dom-test-utils | [React Docs](https://react.dev/)
# react-dom/test-utils Deprecation Warnings[Link for this heading]()
TODO: update for 19?
## ReactDOMTestUtils.act() warning[Link for ReactDOMTestUtils.act() warning]()
`act` from `react-dom/test-utils` has been deprecated in favor of `act` from `react`.
Before:
```
import {act} from 'react-dom/test-utils';
```
After:
```
import {act} from 'react';
```
## Rest of ReactDOMTestUtils APIS[Link for Rest of ReactDOMTestUtils APIS]()
All APIs except `act` have been removed.
The React Team recommends migrating your tests to [@testing-library/react](https://testing-library.com/docs/react-testing-library/intro/) for a modern and well supported testing experience.
### ReactDOMTestUtils.renderIntoDocument[Link for ReactDOMTestUtils.renderIntoDocument]()
`renderIntoDocument` can be replaced with `render` from `@testing-library/react`.
Before:
```
import {renderIntoDocument} from 'react-dom/test-utils';
renderIntoDocument(<Component />);
```
After:
```
import {render} from '@testing-library/react';
render(<Component />);
```
### ReactDOMTestUtils.Simulate[Link for ReactDOMTestUtils.Simulate]()
`Simulate` can be replaced with `fireEvent` from `@testing-library/react`.
Before:
```
import {Simulate} from 'react-dom/test-utils';
const element = document.querySelector('button');
Simulate.click(element);
```
After:
```
import {fireEvent} from '@testing-library/react';
const element = document.querySelector('button');
fireEvent.click(element);
```
Be aware that `fireEvent` dispatches an actual event on the element and doesn’t just synthetically call the event handler.
### List of all removed APIs[Link for List of all removed APIs]()
- `mockComponent()`
- `isElement()`
- `isElementOfType()`
- `isDOMComponent()`
- `isCompositeComponent()`
- `isCompositeComponentWithType()`
- `findAllInRenderedTree()`
- `scryRenderedDOMComponentsWithClass()`
- `findRenderedDOMComponentWithClass()`
- `scryRenderedDOMComponentsWithTag()`
- `findRenderedDOMComponentWithTag()`
- `scryRenderedComponentsWithType()`
- `findRenderedComponentWithType()`
- `renderIntoDocument`
- `Simulate` |
https://react.dev/reference/react | [API Reference](https://react.dev/reference/react)
# React Reference Overview[Link for this heading]()
This section provides detailed reference documentation for working with React. For an introduction to React, please visit the [Learn](https://react.dev/learn) section.
The React reference documentation is broken down into functional subsections:
## React[Link for React]()
Programmatic React features:
- [Hooks](https://react.dev/reference/react/hooks) - Use different React features from your components.
- [Components](https://react.dev/reference/react/components) - Built-in components that you can use in your JSX.
- [APIs](https://react.dev/reference/react/apis) - APIs that are useful for defining components.
- [Directives](https://react.dev/reference/rsc/directives) - Provide instructions to bundlers compatible with React Server Components.
## React DOM[Link for React DOM]()
React-dom contains features that are only supported for web applications (which run in the browser DOM environment). This section is broken into the following:
- [Hooks](https://react.dev/reference/react-dom/hooks) - Hooks for web applications which run in the browser DOM environment.
- [Components](https://react.dev/reference/react-dom/components) - React supports all of the browser built-in HTML and SVG components.
- [APIs](https://react.dev/reference/react-dom) - The `react-dom` package contains methods supported only in web applications.
- [Client APIs](https://react.dev/reference/react-dom/client) - The `react-dom/client` APIs let you render React components on the client (in the browser).
- [Server APIs](https://react.dev/reference/react-dom/server) - The `react-dom/server` APIs let you render React components to HTML on the server.
## Rules of React[Link for Rules of React]()
React has idioms — or rules — for how to express patterns in a way that is easy to understand and yields high-quality applications:
- [Components and Hooks must be pure](https://react.dev/reference/rules/components-and-hooks-must-be-pure) – Purity makes your code easier to understand, debug, and allows React to automatically optimize your components and hooks correctly.
- [React calls Components and Hooks](https://react.dev/reference/rules/react-calls-components-and-hooks) – React is responsible for rendering components and hooks when necessary to optimize the user experience.
- [Rules of Hooks](https://react.dev/reference/rules/rules-of-hooks) – Hooks are defined using JavaScript functions, but they represent a special type of reusable UI logic with restrictions on where they can be called.
## Legacy APIs[Link for Legacy APIs]()
- [Legacy APIs](https://react.dev/reference/react/legacy) - Exported from the `react` package, but not recommended for use in newly written code.
[NextHooks](https://react.dev/reference/react/hooks) |
https://react.dev/reference/react/useActionState | [API Reference](https://react.dev/reference/react)
[Hooks](https://react.dev/reference/react/hooks)
# useActionState[Link for this heading]()
`useActionState` is a Hook that allows you to update state based on the result of a form action.
```
const [state, formAction, isPending] = useActionState(fn, initialState, permalink?);
```
### Note
In earlier React Canary versions, this API was part of React DOM and called `useFormState`.
- [Reference]()
- [`useActionState(action, initialState, permalink?)`]()
- [Usage]()
- [Using information returned by a form action]()
- [Troubleshooting]()
- [My action can no longer read the submitted form data]()
* * *
## Reference[Link for Reference]()
### `useActionState(action, initialState, permalink?)`[Link for this heading]()
Call `useActionState` at the top level of your component to create component state that is updated [when a form action is invoked](https://react.dev/reference/react-dom/components/form). You pass `useActionState` an existing form action function as well as an initial state, and it returns a new action that you use in your form, along with the latest form state and whether the Action is still pending. The latest form state is also passed to the function that you provided.
```
import { useActionState } from "react";
async function increment(previousState, formData) {
return previousState + 1;
}
function StatefulForm({}) {
const [state, formAction] = useActionState(increment, 0);
return (
<form>
{state}
<button formAction={formAction}>Increment</button>
</form>
)
}
```
The form state is the value returned by the action when the form was last submitted. If the form has not yet been submitted, it is the initial state that you pass.
If used with a Server Function, `useActionState` allows the server’s response from submitting the form to be shown even before hydration has completed.
[See more examples below.]()
#### Parameters[Link for Parameters]()
- `fn`: The function to be called when the form is submitted or button pressed. When the function is called, it will receive the previous state of the form (initially the `initialState` that you pass, subsequently its previous return value) as its initial argument, followed by the arguments that a form action normally receives.
- `initialState`: The value you want the state to be initially. It can be any serializable value. This argument is ignored after the action is first invoked.
- **optional** `permalink`: A string containing the unique page URL that this form modifies. For use on pages with dynamic content (eg: feeds) in conjunction with progressive enhancement: if `fn` is a [server function](https://react.dev/reference/rsc/server-functions) and the form is submitted before the JavaScript bundle loads, the browser will navigate to the specified permalink URL, rather than the current page’s URL. Ensure that the same form component is rendered on the destination page (including the same action `fn` and `permalink`) so that React knows how to pass the state through. Once the form has been hydrated, this parameter has no effect.
#### Returns[Link for Returns]()
`useActionState` returns an array with the following values:
1. The current state. During the first render, it will match the `initialState` you have passed. After the action is invoked, it will match the value returned by the action.
2. A new action that you can pass as the `action` prop to your `form` component or `formAction` prop to any `button` component within the form.
3. The `isPending` flag that tells you whether there is a pending Transition.
#### Caveats[Link for Caveats]()
- When used with a framework that supports React Server Components, `useActionState` lets you make forms interactive before JavaScript has executed on the client. When used without Server Components, it is equivalent to component local state.
- The function passed to `useActionState` receives an extra argument, the previous or initial state, as its first argument. This makes its signature different than if it were used directly as a form action without using `useActionState`.
* * *
## Usage[Link for Usage]()
### Using information returned by a form action[Link for Using information returned by a form action]()
Call `useActionState` at the top level of your component to access the return value of an action from the last time a form was submitted.
```
import { useActionState } from 'react';
import { action } from './actions.js';
function MyComponent() {
const [state, formAction] = useActionState(action, null);
// ...
return (
<form action={formAction}>
{/* ... */}
</form>
);
}
```
`useActionState` returns an array with the following items:
1. The current state of the form, which is initially set to the initial state you provided, and after the form is submitted is set to the return value of the action you provided.
2. A new action that you pass to `<form>` as its `action` prop.
3. A pending state that you can utilise whilst your action is processing.
When the form is submitted, the action function that you provided will be called. Its return value will become the new current state of the form.
The action that you provide will also receive a new first argument, namely the current state of the form. The first time the form is submitted, this will be the initial state you provided, while with subsequent submissions, it will be the return value from the last time the action was called. The rest of the arguments are the same as if `useActionState` had not been used.
```
function action(currentState, formData) {
// ...
return 'next state';
}
```
#### Display information after submitting a form[Link for Display information after submitting a form]()
1\. Display form errors 2. Display structured information after submitting a form
#### Example 1 of 2: Display form errors[Link for this heading]()
To display messages such as an error message or toast that’s returned by a Server Function, wrap the action in a call to `useActionState`.
App.jsactions.js
App.js
Reset[Fork](https://codesandbox.io/api/v1/sandboxes/define?undefined&environment=create-react-app "Open in CodeSandbox")
```
import { useActionState, useState } from "react";
import { addToCart } from "./actions.js";
function AddToCartForm({itemID, itemTitle}) {
const [message, formAction, isPending] = useActionState(addToCart, null);
return (
<form action={formAction}>
<h2>{itemTitle}</h2>
<input type="hidden" name="itemID" value={itemID} />
<button type="submit">Add to Cart</button>
{isPending ? "Loading..." : message}
</form>
);
}
export default function App() {
return (
<>
<AddToCartForm itemID="1" itemTitle="JavaScript: The Definitive Guide" />
<AddToCartForm itemID="2" itemTitle="JavaScript: The Good Parts" />
</>
)
}
```
Show more
Next Example
## Troubleshooting[Link for Troubleshooting]()
### My action can no longer read the submitted form data[Link for My action can no longer read the submitted form data]()
When you wrap an action with `useActionState`, it gets an extra argument *as its first argument*. The submitted form data is therefore its *second* argument instead of its first as it would usually be. The new first argument that gets added is the current state of the form.
```
function action(currentState, formData) {
// ...
}
```
[PreviousHooks](https://react.dev/reference/react/hooks)
[NextuseCallback](https://react.dev/reference/react/useCallback) |
https://www.chakra-ui.com | Build faster with Premium Chakra UI Components 💎
[Learn more](https://pro.chakra-ui.com?utm_source=chakra-ui.com)
[](https://www.chakra-ui.com/)
[Docs](https://www.chakra-ui.com/docs/get-started/installation)[Playground](https://www.chakra-ui.com/playground)[Guides](https://www.chakra-ui.com/guides)[Blog](https://www.chakra-ui.com/blog)
[](https://github.com/chakra-ui/chakra-ui)
[Celebrating the launch of Chakra 3.0](https://www.chakra-ui.com/blog/00-announcing-v3)
# Chakra UI is a component system for building products with speed
Accessible React components for building high-quality web apps and design systems. Works with Next.js RSC
[Start Building](https://www.chakra-ui.com/docs/get-started/installation)
```
npm i @chakra-ui/react
```
Slider
Pin Input
ChakraArkZag
Chakra UI is a component library for building web applications.
Ark UI is a headless library for building reusable, scalable design systems
Zag.js provides a set of UI components powered by Finite State Machines
Tabs
Open Menu
New Text File
New File...
New Window
Menu
Switch
## Built for modern product teams. From next-gen startups to established enterprises.
Design System
## Build your design system on top of Chakra UI
Spend less time writing UI code and more time building a great experience for your customers.
- Tokens. Streamline design decisions with semantic tokens
- Typography. Customise your font related properties in one place
- Recipes. Design components variants with ease
TokensTypographyRecipes
```
import { defineTokens } from "@chakra-ui/react"
export const tokens = defineTokens({
colors: {
primary: { value: "#0FEE0F" },
secondary: { value: "#EE0F0F" },
},
fonts: {
body: { value: "system-ui, sans-serif" },
},
animations: {
"slide-in-right": { value: "slide-in-right 0.5s ease-in-out" },
},
})
```
```
import { defineTextStyles } from "@chakra-ui/react"
export const textStyles = defineTextStyles({
headline: {
value: {
fontSize: "4xl",
fontWeight: "bold",
},
},
subheadline: {
value: {
fontSize: "2xl",
fontWeight: "semibold",
},
},
})
```
```
import { defineRecipe } from "@chakra-ui/react"
export const cardRecipe = defineRecipe({
base: {
display: "flex",
flexDirection: "column",
},
variants: {
variant: {
primary: {
bg: "teal.600",
color: "white",
},
},
},
})
```
## Built for developers By developers
## Built for modern product teams. From next-gen startups to established enterprises.
2.5M
downloads / month
38.2K
github stars
8.9K
discord members
## Top-tier teams use and love Chakra
[Chakra UI is glorious. Dark mode support looks amazing and it is 100% built-in. I love the consistent use of focus styling and the subtle animation. Great care for accessibility throughout. It is a guiding principle of the design system.
\
Guillermo Rauch
\
CEO / Vercel
\
](https://twitter.com/rauchg/status/1169632334389248000)
[Chakra is a fantastic component library that helps shape and accelerate the work we're doing with Twilio Paste. Thank you @thesegunadebayo!
\
Aayush Iyer
\
Engineer / Twillio
\
](https://twitter.com/aayush/status/1264251538735632384)
[Awesome new open-source component library from @thesegunadebayo. Really impressive stuff!
\
Colm Tuite
\
CEO / Modulz
\
](https://twitter.com/colmtuite/status/1169622886052782081)
[Chakra UI has become part of our default stack for React apps, Chakra saves our team tons of time, is well designed and documented, has solid accessibility defaults, and looks great out of the box.
\
Echobind Engineering
\
Echobind
\
](https://twitter.com/echobind/status/1272895730299154438)
Sponsors
## Sponsored by these amazing companies
Our maintainers devote their time, effort, and heart to ensure Chakra UI keeps getting better. Support us by donating to our collective 🙏
[Sponsor](https://opencollective.com/chakra-ui)[Patreon](https://www.patreon.com/segunadebayo)
Gold + Platinum Sponsors
[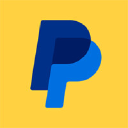](https://www.paypal.me/eliasaadeh)[](https://goread.io/buy-instagram-followers)[](https://www.bairesdev.com/sponsoring-open-source-projects/)[](https://veepn.com/vpn-apps/download-vpn-for-pc/)[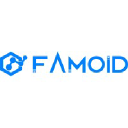](https://famoid.com/)[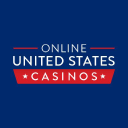](https://www.onlineunitedstatescasinos.com/)[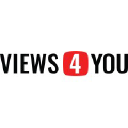](https://views4you.com/buy-youtube-views/)[](https://lyssna.com/)[](https://get.scribe.ai/)[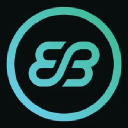](https://echobind.com)[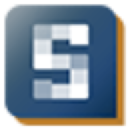](https://sidesmedia.com/buy-youtube-views)[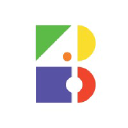](https://www.brikl.com/)[](https://twicsy.com/buy-instagram-followers)[](https://buyreviewz.com/buy-google-reviews)[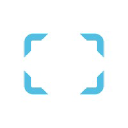](https://www.shootproof.com/)[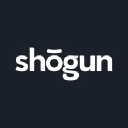](https://getshogun.com)[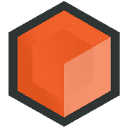](https://buzzoid.com/buy-instagram-followers/)[](https://opencollective.com/touchless)[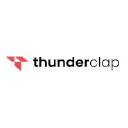](https://thunderclap.it/buy-instagram-followers)[](https://ssmarket.net)[](https://livecycle.io)[](https://www.fbpostlikes.com/buy-tiktok-views.html)[](https://www.famety.com/buy-tiktok-likes)[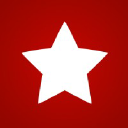](https://techreviewer.co/top-web-development-companies)[](https://www.vpsserver.com)[](https://xata.io/)[](https://insfollowpro.com)[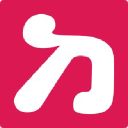](https://meeshkan.com)
Silver + Bronze Sponsors
[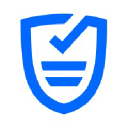](https://trustpage.com)[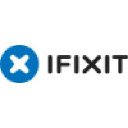](https://www.ifixit.com)[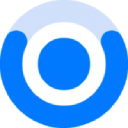](https://fitmymoney.com/)[](https://fantasyrsps.com/)[](https://onlinecasinohex.ph/)[](https://casinohex.org/)[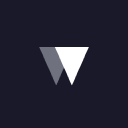](https://xyflow.com/)[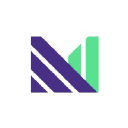](https://materialize.com)[](https://www.socialwick.com/youtube/views)[](https://legitgamblingsites.com)[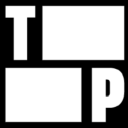](https://www.tightpoker.com/)[](https://casinohex.gr/)[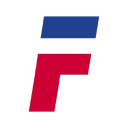](https://freebets.ltd.uk/)[](https://777.ua/games/)[](https://www.upgrow.com/)[](https://mochalabs.com)[](https://www.creditcaptain.com/)[](https://thunderclap.com/)[](https://buyyoutubviews.com/)[](https://writingmetier.com)[](https://solcellsforetag.se/)[](https://www.nongamstopbets.com/casinos-not-on-gamstop/)[](https://rsps-servers.com/)[](https://opencollective.com/buy-youtube-views-paypal)
## Works with your favorite application framework
Chakra provides a consistent developer experience for most modern frameworks
Ready made templates
## Build even faster with Chakra Pro 💎
Premade components and pages for application, marketing and ecommerce. Beautiful and responsive.
[Explore Templates](https://pro.chakra-ui.com/?utm_source=chakra-ui.com&utm_medium=homepage-ad)
 |
https://www.chakra-ui.com/ | Build faster with Premium Chakra UI Components 💎
[Learn more](https://pro.chakra-ui.com?utm_source=chakra-ui.com)
[](https://www.chakra-ui.com/)
[Docs](https://www.chakra-ui.com/docs/get-started/installation)[Playground](https://www.chakra-ui.com/playground)[Guides](https://www.chakra-ui.com/guides)[Blog](https://www.chakra-ui.com/blog)
[](https://github.com/chakra-ui/chakra-ui)
[Celebrating the launch of Chakra 3.0](https://www.chakra-ui.com/blog/00-announcing-v3)
# Chakra UI is a component system for building products with speed
Accessible React components for building high-quality web apps and design systems. Works with Next.js RSC
[Start Building](https://www.chakra-ui.com/docs/get-started/installation)
```
npm i @chakra-ui/react
```
Slider
Pin Input
ChakraArkZag
Chakra UI is a component library for building web applications.
Ark UI is a headless library for building reusable, scalable design systems
Zag.js provides a set of UI components powered by Finite State Machines
Tabs
Open Menu
New Text File
New File...
New Window
Menu
Switch
## Built for modern product teams. From next-gen startups to established enterprises.
Design System
## Build your design system on top of Chakra UI
Spend less time writing UI code and more time building a great experience for your customers.
- Tokens. Streamline design decisions with semantic tokens
- Typography. Customise your font related properties in one place
- Recipes. Design components variants with ease
TokensTypographyRecipes
```
import { defineTokens } from "@chakra-ui/react"
export const tokens = defineTokens({
colors: {
primary: { value: "#0FEE0F" },
secondary: { value: "#EE0F0F" },
},
fonts: {
body: { value: "system-ui, sans-serif" },
},
animations: {
"slide-in-right": { value: "slide-in-right 0.5s ease-in-out" },
},
})
```
```
import { defineTextStyles } from "@chakra-ui/react"
export const textStyles = defineTextStyles({
headline: {
value: {
fontSize: "4xl",
fontWeight: "bold",
},
},
subheadline: {
value: {
fontSize: "2xl",
fontWeight: "semibold",
},
},
})
```
```
import { defineRecipe } from "@chakra-ui/react"
export const cardRecipe = defineRecipe({
base: {
display: "flex",
flexDirection: "column",
},
variants: {
variant: {
primary: {
bg: "teal.600",
color: "white",
},
},
},
})
```
## Built for developers By developers
## Built for modern product teams. From next-gen startups to established enterprises.
2.5M
downloads / month
38.2K
github stars
8.9K
discord members
## Top-tier teams use and love Chakra
[Chakra UI is glorious. Dark mode support looks amazing and it is 100% built-in. I love the consistent use of focus styling and the subtle animation. Great care for accessibility throughout. It is a guiding principle of the design system.
\
Guillermo Rauch
\
CEO / Vercel
\
](https://twitter.com/rauchg/status/1169632334389248000)
[Chakra is a fantastic component library that helps shape and accelerate the work we're doing with Twilio Paste. Thank you @thesegunadebayo!
\
Aayush Iyer
\
Engineer / Twillio
\
](https://twitter.com/aayush/status/1264251538735632384)
[Awesome new open-source component library from @thesegunadebayo. Really impressive stuff!
\
Colm Tuite
\
CEO / Modulz
\
](https://twitter.com/colmtuite/status/1169622886052782081)
[Chakra UI has become part of our default stack for React apps, Chakra saves our team tons of time, is well designed and documented, has solid accessibility defaults, and looks great out of the box.
\
Echobind Engineering
\
Echobind
\
](https://twitter.com/echobind/status/1272895730299154438)
Sponsors
## Sponsored by these amazing companies
Our maintainers devote their time, effort, and heart to ensure Chakra UI keeps getting better. Support us by donating to our collective 🙏
[Sponsor](https://opencollective.com/chakra-ui)[Patreon](https://www.patreon.com/segunadebayo)
Gold + Platinum Sponsors
[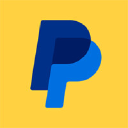](https://www.paypal.me/eliasaadeh)[](https://goread.io/buy-instagram-followers)[](https://www.bairesdev.com/sponsoring-open-source-projects/)[](https://veepn.com/vpn-apps/download-vpn-for-pc/)[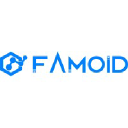](https://famoid.com/)[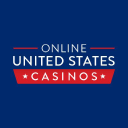](https://www.onlineunitedstatescasinos.com/)[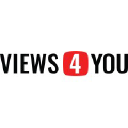](https://views4you.com/buy-youtube-views/)[](https://lyssna.com/)[](https://get.scribe.ai/)[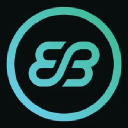](https://echobind.com)[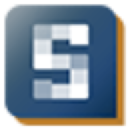](https://sidesmedia.com/buy-youtube-views)[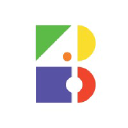](https://www.brikl.com/)[](https://twicsy.com/buy-instagram-followers)[](https://buyreviewz.com/buy-google-reviews)[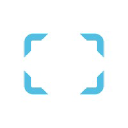](https://www.shootproof.com/)[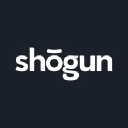](https://getshogun.com)[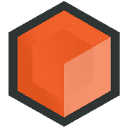](https://buzzoid.com/buy-instagram-followers/)[](https://opencollective.com/touchless)[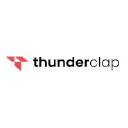](https://thunderclap.it/buy-instagram-followers)[](https://ssmarket.net)[](https://livecycle.io)[](https://www.fbpostlikes.com/buy-tiktok-views.html)[](https://www.famety.com/buy-tiktok-likes)[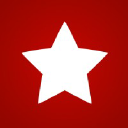](https://techreviewer.co/top-web-development-companies)[](https://www.vpsserver.com)[](https://xata.io/)[](https://insfollowpro.com)[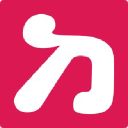](https://meeshkan.com)
Silver + Bronze Sponsors
[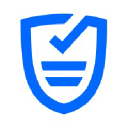](https://trustpage.com)[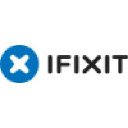](https://www.ifixit.com)[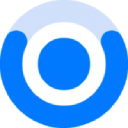](https://fitmymoney.com/)[](https://fantasyrsps.com/)[](https://onlinecasinohex.ph/)[](https://casinohex.org/)[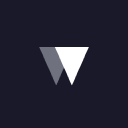](https://xyflow.com/)[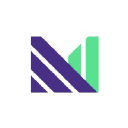](https://materialize.com)[](https://www.socialwick.com/youtube/views)[](https://legitgamblingsites.com)[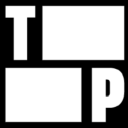](https://www.tightpoker.com/)[](https://casinohex.gr/)[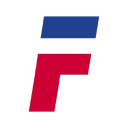](https://freebets.ltd.uk/)[](https://777.ua/games/)[](https://www.upgrow.com/)[](https://mochalabs.com)[](https://www.creditcaptain.com/)[](https://thunderclap.com/)[](https://buyyoutubviews.com/)[](https://writingmetier.com)[](https://solcellsforetag.se/)[](https://www.nongamstopbets.com/casinos-not-on-gamstop/)[](https://rsps-servers.com/)[](https://opencollective.com/buy-youtube-views-paypal)
## Works with your favorite application framework
Chakra provides a consistent developer experience for most modern frameworks
Ready made templates
## Build even faster with Chakra Pro 💎
Premade components and pages for application, marketing and ecommerce. Beautiful and responsive.
[Explore Templates](https://pro.chakra-ui.com/?utm_source=chakra-ui.com&utm_medium=homepage-ad)
 |
https://www.chakra-ui.com/docs/get-started/installation | 1. Overview
2. Installation
# Installation
How to install and set up Chakra UI in your project
## [Framework Guide]()
Chakra UI works in your favorite framework. We've put together step-by-step guides for these frameworks
[Next.js
\
Easily add Chakra UI with Next.js app](https://www.chakra-ui.com/docs/get-started/frameworks/next-app)
[Vite
\
Use Chakra UI in with Vite](https://www.chakra-ui.com/docs/get-started/frameworks/vite)
[Stackblitz
\
Try Chakra UI in Stackblitz sandbox](https://stackblitz.com/edit/chakra-ui-v3)
The minimum node version required is Node.20.x
## [Installation]()
To manually set up Chakra UI in your project, follow the steps below.
1
### [Install `@chakra-ui/react`]()
```
npm i @chakra-ui/react @emotion/react
```
2
### [Add snippets]()
Snippets are pre-built components that you can use to build your UI faster. Using the `@chakra-ui/cli` you can add snippets to your project.
```
npx @chakra-ui/cli snippet add
```
3
### [Setup provider]()
Wrap your application with the `Provider` component generated in the `components/ui/provider` component at the root of your application.
This provider composes the following:
- `ChakraProvider` from `@chakra-ui/react` for the styling system
- `ThemeProvider` from `next-themes` for color mode
```
import { Provider } from "@/components/ui/provider"
function App({ Component, pageProps }) {
return (
<Provider>
<Component {...pageProps} />
</Provider>
)
}
```
4
### [Update tsconfig]()
If you're using TypeScript, you need to update the `compilerOptions` in the tsconfig file to include the following options:
```
{
"compilerOptions": {
"module": "ESNext",
"moduleResolution": "Bundler",
"skipLibCheck": true,
"paths": {
"@/*": ["./src/*"]
}
}
}
```
If you're using JavaScript, create a `jsconfig.json` file and add the above code to the file.
5
### [Enjoy!]()
With the power of the snippets and the primitive components from Chakra UI, you can build your UI faster.
```
import { Button } from "@/components/ui/button"
import { HStack } from "@chakra-ui/react"
const Demo = () => {
return (
<HStack>
<Button>Click me</Button>
<Button>Click me</Button>
</HStack>
)
}
```
## [Learn]()
Watch our official courses and dive into dozens of videos that will teach you everything you need to know about Chakra UI, from basics to advanced concepts.
## [Contribute]()
Whether you're a beginner or advanced Chakra UI user, joining our community is the best way to connect with like-minded people who build great products with the library.
[Next
\
Migration](https://www.chakra-ui.com/docs/get-started/migration) |
https://www.chakra-ui.com/guides | Build faster with Premium Chakra UI Components 💎
[Learn more](https://pro.chakra-ui.com?utm_source=chakra-ui.com)
[](https://www.chakra-ui.com/)
[Docs](https://www.chakra-ui.com/docs/get-started/installation)[Playground](https://www.chakra-ui.com/playground)[Guides](https://www.chakra-ui.com/guides)[Blog](https://www.chakra-ui.com/blog)
[](https://github.com/chakra-ui/chakra-ui)
# How can we help?
Find answers to common questions related to Chakra UI v3.0
### Theming
6 articles
Configuring colors, fonts, breakpoints, and more
[Add custom fonts to a Next.js project](https://www.chakra-ui.com/guides/theming-add-custom-font-to-nextjs) [Add custom fonts to a Vite project](https://www.chakra-ui.com/guides/theming-add-custom-font-to-vite) [Change the default color palette](https://www.chakra-ui.com/guides/theming-change-default-color-palette) [Creating custom breakpoints](https://www.chakra-ui.com/guides/theming-custom-breakpoints) [Creating custom colors](https://www.chakra-ui.com/guides/theming-custom-colors) [Customize dark mode colors](https://www.chakra-ui.com/guides/theming-customize-dark-mode-colors)
### Components
2 articles
Tips and recommendations for components
[Add a Floating Label to an Input](https://www.chakra-ui.com/guides/component-floating-input-label) [Implement a Context Menu](https://www.chakra-ui.com/guides/component-implement-context-menu)
### Snippets
2 articles
Questions about component snippets
[How to manage updates](https://www.chakra-ui.com/guides/snippet-how-to-manage-updates) [Specifying custom directory](https://www.chakra-ui.com/guides/snippet-specify-custom-directory) |
https://www.chakra-ui.com/blog | Build faster with Premium Chakra UI Components 💎
[Learn more](https://pro.chakra-ui.com?utm_source=chakra-ui.com)
[](https://www.chakra-ui.com/)
[Docs](https://www.chakra-ui.com/docs/get-started/installation)[Playground](https://www.chakra-ui.com/playground)[Guides](https://www.chakra-ui.com/guides)[Blog](https://www.chakra-ui.com/blog)
[](https://github.com/chakra-ui/chakra-ui)
# Blog and Updates
Catch up on the latest updates and releases
December 3, 2024
EA
### [Chakra UI London Meetup 2024 Recap](https://www.chakra-ui.com/blog/04-chakra-ui-london-meetup-2024)
Last Thursday's meetup was nothing short of amazing.
[Read more](https://www.chakra-ui.com/blog/04-chakra-ui-london-meetup-2024)
November 24, 2024
EA
### [Building Consistent UIs with Design Tokens](https://www.chakra-ui.com/blog/03-building-consistent-uis-with-design-tokens)
Design tokens allow developers to create maintainable UIs.
[Read more](https://www.chakra-ui.com/blog/03-building-consistent-uis-with-design-tokens)
v3.2
November 21, 2024
SA
### [Chakra 3.2](https://www.chakra-ui.com/blog/02-chakra-3.2)
Introducing new color components, input masking example, and CLI improvements
[Read more](https://www.chakra-ui.com/blog/02-chakra-3.2)
October 28, 2024
EA
### [Chakra v2 vs v3 - A Detailed Comparison](https://www.chakra-ui.com/blog/01-chakra-v2-vs-v3-a-detailed-comparison)
Discover new features in Chakra UI v3.
[Read more](https://www.chakra-ui.com/blog/01-chakra-v2-vs-v3-a-detailed-comparison)
v3.0
October 22, 2024
SA
### [Announcing v3](https://www.chakra-ui.com/blog/00-announcing-v3)
Today, we're excited to announce the release of Chakra UI v3.0
[Read more](https://www.chakra-ui.com/blog/00-announcing-v3) |
https://www.chakra-ui.com/docs/theming/overview | 1. Concepts
2. Overview
# Overview
A guide for configuring the Chakra UI theming system.
## [Architecture]()
The Chakra UI theming system is built around the API of [Panda CSS](https://panda-css.com/).
Here's a quick overview of how the system is structured to provide a performant and extensible styling system:
- Define the styling system configuration using the `defineConfig` function
- Create the styling engine using the `createSystem` function
- Pass the styling engine to the `ChakraProvider` component
```
import { ChakraProvider, createSystem } from "@chakra-ui/react"
const config = defineConfig({
theme: {
tokens: {
colors: {},
},
},
})
const system = createSystem(config)
export default function App() {
return (
<ChakraProvider value={system}>
<Box>Hello World</Box>
</ChakraProvider>
)
}
```
## [Config]()
The Chakra UI system is configured using the `defineConfig` function. This function accepts a configuration object that allows you to customize the styling system's behavior.
After a config is defined, it is passed to the `createSystem` function to create the styling engine.
### [cssVarsRoot]()
`cssVarsRoot` is the root element where the token CSS variables will be applied.
theme.ts
```
const config = defineConfig({
cssVarsRoot: ":where(:root, :host)",
})
export default createSystem(config)
```
### [cssVarsPrefix]()
`cssVarsPrefix` is the prefix used for the token CSS variables.
theme.ts
```
const config = defineConfig({
cssVarsPrefix: "ck",
})
export default createSystem(config)
```
### [globalCss]()
`globalCss` is used to apply global styles to the system.
theme.ts
```
const config = defineConfig({
globalCss: {
"html, body": {
margin: 0,
padding: 0,
},
},
})
export default createSystem(config)
```
### [theme]()
Use the `theme` config property to define the system theme. This property accepts the following properties:
- `breakpoints`: for defining breakpoints
- `keyframes`: for defining css keyframes animations
- `tokens`: for defining tokens
- `semanticTokens`: for defining semantic tokens
- `textStyles`: for defining typography styles
- `layerStyles`: for defining layer styles
- `animationStyles`: for defining animation styles
- `recipes`: for defining component recipes
- `slotRecipes`: for defining component slot recipes
theme.ts
```
const config = defineConfig({
theme: {
breakpoints: {
sm: "320px",
md: "768px",
lg: "960px",
xl: "1200px",
},
tokens: {
colors: {
red: "#EE0F0F",
},
},
semanticTokens: {
colors: {
danger: { value: "{colors.red}" },
},
},
keyframes: {
spin: {
from: { transform: "rotate(0deg)" },
to: { transform: "rotate(360deg)" },
},
},
},
})
export default createSystem(config)
```
### [conditions]()
Use the `conditions` config property to define custom selectors and media query conditions for use in the system.
theme.ts
```
const config = defineConfig({
conditions: {
cqSm: "@container(min-width: 320px)",
child: "& > *",
},
})
export default createSystem(config)
```
Sample usage:
```
<Box mt="40px" _cqSm={{ mt: "0px" }}>
<Text>Hello World</Text>
</Box>
```
### [strictTokens]()
Use the `strictTokens` config property to enforce the usage of only design tokens. This will throw a TS error if you try to use a token that is not defined in the theme.
theme.ts
```
const config = defineConfig({
strictTokens: true,
})
export default createSystem(config)
```
```
// ❌ This will throw a TS error
<Box color="#4f343e">Hello World</Box>
// ✅ This will work
<Box color="red.400">Hello World</Box>
```
## [TypeScript]()
When you configure the system properties (like `colors`, `space`, `fonts`, etc.), the CLI can be used to generate type definitions for them.
```
npx @chakra-ui/cli typegen ./theme.ts
```
This will update the internal types in the `@chakra-ui/react` package, and make sure they are in sync with the theme. Providing a type-safe API and delightful experience for developers.
## [System]()
After a config is defined, it is passed to the `createSystem` function to create the styling engine. The returned `system` is framework-agnostic JavaScript styling engine that can be used to style components.
```
const system = createSystem(config)
```
The system includes the following properties:
### [token]()
The token function is used to get a raw token value, or css variable.
```
const system = createSystem(config)
// raw token
system.token("colors.red.200")
// => "#EE0F0F"
// token with fallback
system.token("colors.pink.240", "#000")
// => "#000"
```
Use the `token.var` function to get the css variable:
```
// css variable
system.token.var("colors.red.200")
// => "var(--chakra-colors-red-200)"
// token with fallback
system.token.var("colors.pink.240", "colors.red.200")
// => "var(--chakra-colors-red-200)"
```
It's important to note that `semanticTokens` always return a css variable, regardless of whether you use `token` or `token.var`. This is because semantic tokens change based on the theme.
```
// semantic token
system.token("colors.danger")
// => "var(--chakra-colors-danger)"
system.token.var("colors.danger")
// => "var(--chakra-colors-danger)"
```
### [tokens]()
```
const system = createSystem(config)
system.tokens.getVar("colors.red.200")
// => "var(--chakra-colors-red-200)"
system.tokens.expandReferenceInValue("3px solid {colors.red.200}")
// => "3px solid var(--chakra-colors-red-200)"
system.tokens.cssVarMap
// => Map { "colors": Map { "red.200": "var(--chakra-colors-red-200)" } }
system.tokens.flatMap
// => Map { "colors.red.200": "var(--chakra-colors-red-200)" }
```
### [css]()
The `css` function is used to convert chakra style objects to CSS style object that can be passed to `emotion` or `styled-components` or any other styling library.
```
const system = createSystem(config)
system.css({
color: "red.200",
bg: "blue.200",
})
// => { color: "var(--chakra-colors-red-200)", background: "var(--chakra-colors-blue-200)" }
```
### [cva]()
The `cva` function is used to create component recipes. It returns a function that, when called with a set of props, returns a style object.
```
const system = createSystem(config)
const button = system.cva({
base: {
color: "white",
bg: "blue.500",
},
variants: {
outline: {
color: "blue.500",
bg: "transparent",
border: "1px solid",
},
},
})
button({ variant: "outline" })
// => { color: "blue.500", bg: "transparent", border: "1px solid" }
```
### [sva]()
The `sva` function is used to create component slot recipes. It returns a function that, when called with a set of props, returns a style object for each slot.
```
const system = createSystem(config)
const alert = system.sva({
slots: ["title", "description", "icon"],
base: {
title: { color: "white" },
description: { color: "white" },
icon: { color: "white" },
},
variants: {
status: {
info: {
title: { color: "blue.500" },
description: { color: "blue.500" },
icon: { color: "blue.500" },
},
},
},
})
alert({ status: "info" })
// => { title: { color: "blue.500" }, description: { color: "blue.500" }, icon: { color: "blue.500" } }
```
### [isValidProperty]()
The `isValidProperty` function is used to check if a property is valid.
```
const system = createSystem(config)
system.isValidProperty("color")
// => true
system.isValidProperty("background")
// => true
system.isValidProperty("invalid")
// => false
```
### [splitCssProps]()
The `splitCssProps` function is used to split the props into css props and non-css props.
```
const system = createSystem(config)
system.splitCssProps({
color: "red.200",
bg: "blue.200",
"aria-label": "Hello World",
})
// => [{ color: "red.200", bg: "blue.200" }, { "aria-label": "Hello World" }]
```
### [breakpoints]()
The `breakpoints` property is used to query breakpoints.
```
const system = createSystem(config)
system.breakpoints.up("sm")
// => "@media (min-width: 320px)"
system.breakpoints.down("sm")
// => "@media (max-width: 319px)"
system.breakpoints.only("md")
// => "@media (min-width: 320px) and (max-width: 768px)"
system.breakpoints.keys()
// => ["sm", "md", "lg", "xl"]
```
## [Tokens]()
To learn more about tokens, please refer to the [tokens](https://www.chakra-ui.com/docs/theming/tokens) section.
## [Recipes]()
To learn more about recipes, please refer to the [recipes](https://www.chakra-ui.com/docs/theming/recipes) section.
[Previous
\
Typography](https://www.chakra-ui.com/docs/styling/style-props/typography)
[Next
\
Tokens](https://www.chakra-ui.com/docs/theming/tokens) |
https://www.chakra-ui.com/blog/00-announcing-v3 | Build faster with Premium Chakra UI Components 💎
[Learn more](https://pro.chakra-ui.com?utm_source=chakra-ui.com)
[](https://www.chakra-ui.com/)
[Docs](https://www.chakra-ui.com/docs/get-started/installation)[Playground](https://www.chakra-ui.com/playground)[Guides](https://www.chakra-ui.com/guides)[Blog](https://www.chakra-ui.com/blog)
[](https://github.com/chakra-ui/chakra-ui)
release·
October 22, 2024
## Announcing v3
SA
Segun Adebayo
@thesegunadebayo
Today, we're excited to announce the long-awaited release of Chakra UI v3. The feedback for Chakra v3 has been incredible and we appreciate those who took the time to test and catch bugs.
Chakra v3 is a complete rewrite of Chakra to enhance it's performance, speed and consistency across components. We've also added over 25 new components, and that's just the beginning.
## [Credits]()
Before giving you a quick overview, I'd like to start by acknowledging those whose ideas and efforts contributed to the redesign of Chakra v3.
- [**Park UI**](https://park-ui.com/): The groundwork by Christian and Phil laid the foundation of the design philiosophy in Chakra v3. Consistent sizing, colors, shadows, and naming convention is the heart and soul of a design system.
- [**Panda**](https://panda-css.com/): The theming and styling APIs in Panda CSS created paved the way for Chakra v3's styling engine. The end goal was to make it almost effortless to switch between Park UI and Chakra UI.
- [**Radix Themes**](https://www.radix-ui.com/themes): For inspiring the playground, composable animations and accent color system.
- [**Shadcn**](https://ui.shadcn.com/): For inspiring the CLI and driving the idea of copy-paste snippets which Chakra now embraces.
We also want to appreciate these individuals have contributed consistently and helped to shape v3.
[Christian Schröter](https://x.com/grizzly_codes), [Esther Adebayo](https://x.com/_estheradebayo), [Eelco](https://x.com/pagebakers), [Alex Stahmer](https://x.com/astahmer_dev), [Tolulope Oyewumi](https://x.com/Delighteebrands), [Abraham Anuoluwapo](https://x.com/anubra266), [Ivica Batinić](https://x.com/_isBatak)
## [Design Architecture]()
In Chakra v3, we're unifying our ecosystem of tools by combining the headless library, Ark UI with the styling APIs in Panda CSS, then using Park UI as the design system.

We've redesigned most of the components from ground-up to ensure they are all consistent and use design tokens in most cases.
## [Semantic Tokens]()
Semantic tokens make it easy to personalize your token without having to restyle every component by hand. Chakra v3 provides 7 semantic tokens for each color palette, giving you ultimate flexibility without having to think about dark mode.
- **solid:** The solid color of the palette
- **muted:** A muted version of the palette
- **subtle:** A subtle version of the palette, lower then the muted one.
- **emphasized:** A more pronounced version of the palette
- **contrast:** A color that goes on the solid background (also called "on-solid")
- **fg:** The foreground color of the palette
- **focusRing:** The focus ring color of the palette
Here's an example of using the red color in a semantic way that automatically adapts to dark mode.
```
// A subtle version of red
<Box bg="red.subtle" color="red.fg">
Welcome
</Box>
// A solid version of red
<Box bg="red.solid" color="red.contrast">
Welcome
</Box>
```
To take this to the next level, you can leverage the new `colorPalette` feature. It allows you create a color placeholder that be swapped to any color at any depth on the DOM tree using CSS variables.
```
<Box colorPalette="red">
<Box bg="colorPalette.subtle" color="colorPalette.fg">
Welcome
</Box>
<Box bg="colorPalette.solid" color="colorPalette.contrast">
Welcome
</Box>
</Box>
```
## [Open by default]()
We moved away from closed components to open, compound components by default. This makes it easier for you compose your own components and reduce the maintenance on our end.
To illustrate the difference, here's how you'd create a checkbox in v2.
```
<Checkbox>Click me</Checkbox>
```
Here's a contrived example of the checkbox component in Chakra v2.
```
export const Checkbox = forwardRef(function Checkbox(props, ref) {
const { children, iconColor, iconSize, icon, inputProps, ...checkboxProps } =
props
const checkbox = useCheckbox(checkboxProps)
return (
<chakra.label {...checkbox.getRootProps()}>
<input {...checkbox.getInputProps(inputProps, ref)} />
<chakra.span {...getCheckboxProps()}>
<CheckIcon as={icon} color={iconColor} size={iconSize} />
</chakra.span>
{children && (
<chakra.span {...checkbox.getLabelProps()}>{children}</chakra.span>
)}
</chakra.label>
)
})
```
While the snippet above looks easy to use, you get to the point where customization becomes a challenge. Questions like these often arise:
- how to pass props to the underlying icon?
- how to pass props to the label that renders the children?
- how to change the underlying animation of the checkbox?
This often leads to a component with many props that can be confusing to learn.
We've now made Chakra UI composable by default, this means you no longer get closed components by default.
Here's how you'd create a checkbox with the new approach.
```
<Checkbox.Root>
<Checkbox.HiddenInput />
<Checkbox.Control>
<Checkbox.Indicator />
</Checkbox.Control>
<Checkbox.Label>Click me</Checkbox.Label>
</Checkbox.Root>
```
It's a lot more code and can be overwhelming to write. To account for this slight tweak in DX, we've created the concept of "snippets" which can help you wrap the composable checkbox and get you back to the initial v2 style.
Let me explain how it works:
## [Snippets]()
By running the snippets CLI command, Chakra composes the components in Chakra and puts it in your project. Giving you maximum control of every aspect.
```
npx @chakra-ui/cli@init snippets add
```
After running this you should see primitive components add to the `components/ui/*` directory in your project.
```
// components/ui/checkbox.tsx
export const Checkbox = forwardRef(function Checkbox(props, ref) {
const { children, icon, inputProps, ...restProps } = props
return (
<Checkbox.Root {...restProps}>
<Checkbox.HiddenInput {...inputProps} ref={ref} />
<Checkbox.Control>
<Checkbox.Indicator>{icon}</Checkbox.Indicator>
</Checkbox.Control>
{children && <Checkbox.Label>{children}</Checkbox.Label>}
</Checkbox.Root>
)
})
```
This makes it easy to achieve the same DX as v2 in v3.
```
import { Checkbox } from "@/components/ui/checkbox"
const Demo = () => <Checkbox>Click me</Checkbox>
```
## [Improve runtime performance]()
- **Remove runtime functions in theme:** Previously we allowed the use of functions in the theming system which could negatively impact runtime performance. We've now switched to a variant-based system called "recipes", largely inspired by Panda CSS.
- **Externalize styling engine:** The styling engine is initialized outside of the React tree and consumed by the components, providing a faster style resolution and rendering times in every component.
- **Migration to CSS animations:** We removed the `framer-motion` dependency in favor of using the platform's animation features. This gives us a performance boost and reduces the bundle size of your application.
## [New components]()
We've added new components from Ark UI. These headless components are powered by statecharts and work consistently across major frameworks. We truly believe the future of UI libraries is framework-agnostic.

We also added new presentational components to save you time when building UIs.
## [Embracing the ecosystem]()
We don't want to re-invent the wheel for common needs. In the spirit of this, we removed a good number of modules from Chakra to keep us focused on delivering best-in-class components.
- removed our icons in favor of a more robust icon library like `lucide-react` or `react-icons`
- removed our internal color mode management in favor of `next-themes` or `remix-themes`
- removed most hooks in favor of robust hook libraries like `react-use` or `use-hooks-ts`
## [What's next]()
- Picker components from Ark: ColorPicker, DatePicker
- Redesigned Chakra UI Pro
- Redesigned Figma UI Kit
- Redesigned FigPilot: Our code generation tool for Figma that converts any design to Chakra compatible code.
- Explore the [React 19 style tag](https://react.dev/reference/react-dom/components/style)
## [FAQs]()
### [Does Chakra v3 use Panda internally?]()
No. To reduce the breaking change surface, we've decided to keep emotion (and runtime css-in-js) to preserve the dynamic styling benefits. There's already a lot of changes in v3, so we'll handle this progressively.
We might not even have to use Panda at all. The progress of the [style tag](https://react.dev/reference/react-dom/components/style) in React 19 is very promising and we give Chakra even more performance boost without any migration cost.
### [Are we going to have all components from Ark UI?]()
Yes, Ark UI features a lot of useful components. We've included some of them already in Chakra, but we'll bring in more interesting components like `ColorPicker`, `DatePicker` over time.
### [Do we really need to use the snippets?]()
No, you don't have to use the snippets if you're not a fan. We recommend doing this though, it helps simplify your development experience since you'll anyway have to do the same work on your end.
If you find the number of snippets overwhelming, remove the snippets you don't need.
### [Why the new logo?]()
The launch of v3 marks a new era for Chakra UI. The new era synchronizes Zag.js, Ark UI and Panda CSS in a very unique way. This alone warrants a new story and new brand.
### [Why did it take so long to launch v3?]()
I ask myself the same question as well. It's an Herculean task to design the foundations of Chakra UI in a way that is framework agnostic and entirely built on statecharts. This hadn't been done before and took a lot of time to get that stable. In the end, it was worth the effort and we appreciate your patience.
## [Get started]()
We invite you to try Chakra v3 today and experience the delight of building user interface with speed. **Get started with Chakra UI v3 now**
```
npm i @chakra-ui/react @emotion/react
```
Explore the [migration guide](https://www.chakra-ui.com/docs/get-started/migration) to upgrade your project to Chakra UI v3. |
https://www.chakra-ui.com/docs/styling/overview | 1. Concepts
2. Overview
# Styling
All components are designed to be styled using props.
## [Concepts]()
After installing Chakra UI, follow these guidelines to learn the key concepts:
- [Chakra Factory](https://www.chakra-ui.com/docs/styling/chakra-factory)
- [Responsive Design](https://www.chakra-ui.com/docs/styling/responsive-design)
- [CSS Variables](https://www.chakra-ui.com/docs/styling/css-variables)
- [Dark Mode](https://www.chakra-ui.com/docs/styling/dark-mode)
- [Color Opacity Modifier](https://www.chakra-ui.com/docs/styling/color-opacity-modifier)
- [Conditional Styles](https://www.chakra-ui.com/docs/styling/conditional-styles)
- [Virtual Color](https://www.chakra-ui.com/docs/styling/virtual-color)
## [Compositions]()
After understanding the concepts, learn how to use these compositions to avoid repeating styles:
- [Text Styles](https://www.chakra-ui.com/docs/styling/text-styles)
- [Layer Styles](https://www.chakra-ui.com/docs/styling/layer-styles)
- [Animation Styles](https://www.chakra-ui.com/docs/styling/animation-styles)
- [Focus Ring](https://www.chakra-ui.com/docs/styling/focus-ring)
## [Style Props]()
Style props are the most fundamental way to style your components in Chakra UI. They are basically css styles as props. [Learn more about style props](https://www.chakra-ui.com/docs/styling/style-props/background)
[Previous
\
Visually Hidden](https://www.chakra-ui.com/docs/components/visually-hidden)
[Next
\
Chakra Factory](https://www.chakra-ui.com/docs/styling/chakra-factory) |
https://www.chakra-ui.com/docs/get-started/cli | 1. Overview
2. CLI
# CLI
Learn more to use the Chakra CLI command to generate snippets and typings
The Chakra UI CLI can be used to generate typings for your custom theme tokens, like colors, semantic tokens, recipe variants, etc.
## [Installation]()
In order to use the CLI, you need to install it as a dev dependency in your project:
npmpnpmbun
```
npm i -D @chakra-ui/cli
```
```
pnpm add -D @chakra-ui/cli
```
```
bun add -d @chakra-ui/cli
```
warning
To use the CLI tool, please ensure that the version of Node.js is `>= 20.6.0`.
## [Usage]()
Use the Chakra CLI to run any of the commands listed below with your preferred package manager.
```
Usage: chakra-ui [options] [command]
The official CLI for Chakra UI projects
Options:
-V, --version output the version number
-h, --help display help for command
Commands:
typegen [options] <source> Generate theme and recipe typings
snippet Add snippets to your project for better DX
eject [options] Take control over the default theme tokens and recipes
help [command] display help for command
```
## [`chakra typegen`]()
Generates theme and recipe typings for your custom theme. This helps to provide autocompletion and type safety in your project.
```
# Generate typings
chakra typegen src/theme.ts
# Watch for changes and rebuild
chakra typegen src/theme.ts --watch
# Generate strict types for props variant and size
chakra typegen src/theme.ts --strict
```
## [`chakra snippet`]()
Generates useful component compositions that boost your development speed.
```
# Add all snippets
chakra snippet add --all
# Add a specific snippet
chakra snippet add button
# List all available snippets
chakra snippet list
# Specify a custom directory
chakra snippet --outdir ./components/custom
```
## [`chakra eject`]()
Generated the file(s) that contain the default theme tokens and recipes so you can have full control over them.
```
# Copy the tokens and recipes to your project
chakra eject --outdir src/theme
```
## [FAQ]()
### [Autocomplete for custom tokens not working?]()
After generating the typings, you need to "Restart TS Server" for the autocomplete to show up.
Alternatively, you can install the `@chakra-ui/cli` package locally as a dev dependency and run the `chakra typegen` command to generate the typings.
[Previous
\
Migration](https://www.chakra-ui.com/docs/get-started/migration)
[Next
\
Contributing](https://www.chakra-ui.com/docs/get-started/contributing) |
https://www.chakra-ui.com/docs/components/concepts/overview | 1. Concepts
2. Overview
# Components
Accessible, modern and easy to style UI components.
Here's a list of all the components available in the library.
[Accordion
\
Used to show and hide sections of related content on a page](https://www.chakra-ui.com/docs/components/accordion)
[Action Bar
\
Used to display a bottom action bar with a set of actions](https://www.chakra-ui.com/docs/components/action-bar)
[Alert
\
Used to communicate a state that affects a system, feature or page.](https://www.chakra-ui.com/docs/components/alert)
[Aspect Ratio
\
Used to embed responsive videos and maps, etc](https://www.chakra-ui.com/docs/components/aspect-ratio)
[Avatar
\
Used to represent user profile picture or initials](https://www.chakra-ui.com/docs/components/avatar)
[Badge
\
Used to highlight an item's status for quick recognition.](https://www.chakra-ui.com/docs/components/badge)
[Bleed
\
Used to break an element from the boundaries of its container](https://www.chakra-ui.com/docs/components/bleed)
[Blockquote
\
Used to quote text content from an external source](https://www.chakra-ui.com/docs/components/blockquote)
[Box
\
The most abstract styling component in Chakra UI on top of which all other Chakra UI components are built.](https://www.chakra-ui.com/docs/components/box)
[Breadcrumb
\
Used to display a page's location within a site's hierarchical structure](https://www.chakra-ui.com/docs/components/breadcrumb)
[Button
\
Used to trigger an action or event](https://www.chakra-ui.com/docs/components/button)
[Card
\
Used to display content related to a single subject.](https://www.chakra-ui.com/docs/components/card)
[Center
\
Used to center its child within itself.](https://www.chakra-ui.com/docs/components/center)
[Checkbox Card
\
Used to select or deselect options displayed within cards.](https://www.chakra-ui.com/docs/components/checkbox-card)
[Checkbox
\
Used in forms when a user needs to select multiple values from several options](https://www.chakra-ui.com/docs/components/checkbox)
[Client Only
\
Used to render content only on the client side.](https://www.chakra-ui.com/docs/components/client-only)
[Clipboard
\
Used to copy text to the clipboard](https://www.chakra-ui.com/docs/components/clipboard)
[Close Button
\
Used to trigger close functionality](https://www.chakra-ui.com/docs/components/close-button)
[Code
\
Used to display inline code](https://www.chakra-ui.com/docs/components/code)
[Collapsible
\
Used to expand and collapse additional content.](https://www.chakra-ui.com/docs/components/collapsible)
[Color Picker
\
Used to select colors from a color area or a set of defined swatches](https://www.chakra-ui.com/docs/components/color-picker)
[Color Swatch
\
Used to preview a color](https://www.chakra-ui.com/docs/components/color-swatch)
[Container
\
Used to constrain a content's width to the current breakpoint, while keeping it fluid.](https://www.chakra-ui.com/docs/components/container)
[DataList
\
Used to display a list of similar data items.](https://www.chakra-ui.com/docs/components/data-list)
[Dialog
\
Used to display a dialog prompt](https://www.chakra-ui.com/docs/components/dialog)
[Drawer
\
Used to render a content that slides in from the side of the screen.](https://www.chakra-ui.com/docs/components/drawer)
[Editable
\
Used for inline renaming of some text.](https://www.chakra-ui.com/docs/components/editable)
[Em
\
Used to mark text for emphasis.](https://www.chakra-ui.com/docs/components/em)
[Empty State
\
Used to indicate when a resource is empty or unavailable.](https://www.chakra-ui.com/docs/components/empty-state)
[Environment Provider
\
Used to render components in iframes, Shadow DOM, or Electron.](https://www.chakra-ui.com/docs/components/environment-provider)
[Field
\
Used to add labels, help text, and error messages to form fields.](https://www.chakra-ui.com/docs/components/field)
[Fieldset
\
A set of form controls optionally grouped under a common name.](https://www.chakra-ui.com/docs/components/fieldset)
[File Upload
\
Used to select and upload files from their device.](https://www.chakra-ui.com/docs/components/file-upload)
[Flex
\
Used to manage flex layouts](https://www.chakra-ui.com/docs/components/flex)
[Float
\
Used to anchor an element to the edge of a container.](https://www.chakra-ui.com/docs/components/float)
[For
\
Used to loop over an array and render a component for each item.](https://www.chakra-ui.com/docs/components/for)
[Format Byte
\
Used to format bytes to a human-readable format](https://www.chakra-ui.com/docs/components/format-byte)
[Format Number
\
Used to format numbers to a specific locale and options](https://www.chakra-ui.com/docs/components/format-number)
[Grid
\
Used to manage grid layouts](https://www.chakra-ui.com/docs/components/grid)
[Group
\
Used to group and attach elements together](https://www.chakra-ui.com/docs/components/group)
[Heading
\
Used to render semantic HTML heading elements.](https://www.chakra-ui.com/docs/components/heading)
[Highlight
\
Used to highlight substrings of a text.](https://www.chakra-ui.com/docs/components/highlight)
[Hover Card
\
Used to display content when hovering over an element](https://www.chakra-ui.com/docs/components/hover-card)
[Icon Button
\
Used to render an icon within a button](https://www.chakra-ui.com/docs/components/icon-button)
[Icon
\
Used to display an svg icon](https://www.chakra-ui.com/docs/components/icon)
[Image
\
Used to display images](https://www.chakra-ui.com/docs/components/image)
[Input
\
Used to get user input in a text field.](https://www.chakra-ui.com/docs/components/input)
[Kbd
\
Used to show key combinations for an action](https://www.chakra-ui.com/docs/components/kbd)
[Link Overlay
\
Used to stretch a link over a container.](https://www.chakra-ui.com/docs/components/link-overlay)
[Link
\
Used to provide accessible navigation](https://www.chakra-ui.com/docs/components/link)
[List
\
Used to display a list of items](https://www.chakra-ui.com/docs/components/list)
[Locale Provider
\
Used for globally setting the locale](https://www.chakra-ui.com/docs/components/locale-provider)
[Mark
\
Used to mark text for emphasis.](https://www.chakra-ui.com/docs/components/mark)
[Menu
\
Used to create an accessible dropdown menu](https://www.chakra-ui.com/docs/components/menu)
[Select (Native)
\
Used to pick a value from predefined options.](https://www.chakra-ui.com/docs/components/native-select)
[Number Input
\
Used to enter a number, and increment or decrement the value using stepper buttons.](https://www.chakra-ui.com/docs/components/number-input)
[Pagination
\
Used to navigate through a series of pages.](https://www.chakra-ui.com/docs/components/pagination)
[Password Input
\
Used to collect passwords.](https://www.chakra-ui.com/docs/components/password-input)
[Pin Input
\
Used to capture a pin code or otp from the user](https://www.chakra-ui.com/docs/components/pin-input)
[Popover
\
Used to show detailed information inside a pop-up](https://www.chakra-ui.com/docs/components/popover)
[Portal
\
Used to render an element outside the DOM hierarchy.](https://www.chakra-ui.com/docs/components/portal)
[Progress Circle
\
Used to display a task's progress in a circular form.](https://www.chakra-ui.com/docs/components/progress-circle)
[Progress
\
Used to display the progress status for a task.](https://www.chakra-ui.com/docs/components/progress)
[Prose
\
Used to style remote HTML content](https://www.chakra-ui.com/docs/components/prose)
[Radio Card
\
Used to select an option from list](https://www.chakra-ui.com/docs/components/radio-card)
[Radio
\
Used to select one option from several options](https://www.chakra-ui.com/docs/components/radio)
[Rating
\
Used to show reviews and ratings in a visual format.](https://www.chakra-ui.com/docs/components/rating)
[Segmented Control
\
Used to pick one choice from a linear set of options](https://www.chakra-ui.com/docs/components/segmented-control)
[Select
\
Used to pick a value from predefined options.](https://www.chakra-ui.com/docs/components/select)
[Separator
\
Used to visually separate content](https://www.chakra-ui.com/docs/components/separator)
[Show
\
Used to conditional render part of the view based on a condition.](https://www.chakra-ui.com/docs/components/show)
[SimpleGrid
\
SimpleGrid provides a friendly interface to create responsive grid layouts with ease.](https://www.chakra-ui.com/docs/components/simple-grid)
[Skeleton
\
Used to render a placeholder while the content is loading.](https://www.chakra-ui.com/docs/components/skeleton)
[Slider
\
Used to allow users to make selections from a range of values.](https://www.chakra-ui.com/docs/components/slider)
[Spinner
\
Used to provide a visual cue that an action is processing](https://www.chakra-ui.com/docs/components/spinner)
[Stack
\
Used to layout its children in a vertical or horizontal stack.](https://www.chakra-ui.com/docs/components/stack)
[Stat
\
Used to display a statistic with a title and value.](https://www.chakra-ui.com/docs/components/stat)
[Status
\
Used to indicate the status of a process or state](https://www.chakra-ui.com/docs/components/status)
[Steps
\
Used to indicate progress through a multi-step process](https://www.chakra-ui.com/docs/components/steps)
[Switch
\
Used to capture a binary state](https://www.chakra-ui.com/docs/components/switch)
[Table
\
Used to display data in a tabular format.](https://www.chakra-ui.com/docs/components/table)
[Tabs
\
Used to display content in a tabbed interface](https://www.chakra-ui.com/docs/components/tabs)
[Tag
\
Used for categorizing or labeling content](https://www.chakra-ui.com/docs/components/tag)
[Text
\
Used to render text and paragraphs within an interface.](https://www.chakra-ui.com/docs/components/text)
[Textarea
\
Used to enter multiple lines of text.](https://www.chakra-ui.com/docs/components/textarea)
[Theme
\
Used to force a part of the tree to light or dark mode.](https://www.chakra-ui.com/docs/components/theme)
[Timeline
\
Used to display a list of events in chronological order](https://www.chakra-ui.com/docs/components/timeline)
[Toast
\
Used to display a temporary message to the user](https://www.chakra-ui.com/docs/components/toast)
[Toggle Tip
\
Looks like a tooltip, but works like a popover.](https://www.chakra-ui.com/docs/components/toggle-tip)
[Tooltip
\
Used to display additional information when a user hovers over an element.](https://www.chakra-ui.com/docs/components/tooltip)
[Visually Hidden
\
Used to hide content visually but keep it accessible to screen readers.](https://www.chakra-ui.com/docs/components/visually-hidden)
[Previous
\
Iframe](https://www.chakra-ui.com/docs/get-started/environments/iframe)
[Next
\
Composition](https://www.chakra-ui.com/docs/components/concepts/composition) |
https://www.chakra-ui.com/docs/get-started/migration | 1. Overview
2. Migration
# Migration to v3
How to migrate to Chakra UI v3.x from v2.x
warning
This guide is a work in progress and will be updated from time to time. If you find any point we missed, feel free to open a PR to update this guide.
## [Steps]()
The minimum node version required is Node.20.x
1
### [Update Packages]()
Remove the unused packages: `@emotion/styled` and `framer-motion`. These packages are no longer required in Chakra UI.
```
npm uninstall @emotion/styled framer-motion
```
Install updated versions of the packages: `@chakra-ui/react` and `@emotion/react`.
```
npm install @chakra-ui/react@latest @emotion/react@latest
```
Next, install component snippets using the CLI snippets. Snippets provide pre-built compositions of Chakra components to save you time and put you in charge.
```
npx @chakra-ui/cli snippet add
```
2
### [Refactor Custom Theme]()
Move your custom theme to a dedicated `theme.js` or `theme.ts` file. Use `createSystem` and `defaultConfig` to configure your theme.
**Before**
```
import { extendTheme } from "@chakra-ui/react"
export const theme = extendTheme({
fonts: {
heading: `'Figtree', sans-serif`,
body: `'Figtree', sans-serif`,
},
})
```
**After**
```
import { createSystem, defaultConfig } from "@chakra-ui/react"
export const system = createSystem(defaultConfig, {
theme: {
tokens: {
fonts: {
heading: { value: `'Figtree', sans-serif` },
body: { value: `'Figtree', sans-serif` },
},
},
},
})
```
All token values need to be wrapped in an object with a **value** key. Learn more about tokens [here](https://www.chakra-ui.com/docs/theming/tokens).
3
### [Update ChakraProvider]()
Update the ChakraProvider import from `@chakra-ui/react` to the one from the snippets. Next, rename the `theme` prop to `value` to match the new system-based theming approach.
**Before**
```
import { ChakraProvider } from "@chakra-ui/react"
export const App = ({ Component }) => (
<ChakraProvider theme={theme}>
<Component />
</ChakraProvider>
)
```
**After**
```
import { Provider } from "@/components/ui/provider"
import { defaultSystem } from "@chakra-ui/react"
export const App = ({ Component }) => (
<Provider value={defaultSystem}>
<Component />
</Provider>
)
```
If you have a custom theme, replace `defaultSystem` with the custom `system`
The Provider component compose the `ChakraProvider` from Chakra and `ThemeProvider` from `next-themes`
## [Improvements]()
- **Performance:** Improved reconciliation performance by `4x` and re-render performance by `1.6x`
- **Namespaced imports:** Import components using the dot notation for more concise imports
```
import { Accordion } from "@chakra-ui/react"
const Demo = () => {
return (
<Accordion.Root>
<Accordion.Item>
<Accordion.ItemTrigger />
<Accordion.ItemContent />
</Accordion.Item>
</Accordion.Root>
)
}
```
- **TypeScript:** Improved IntelliSense and type inference for style props and tokens.
- **Polymorphism:** Loosened the `as` prop typings in favor of using the `asChild` prop. This pattern was inspired by Radix Primitives and Ark UI.
## [Removed Features]()
### [Color Mode]()
- `ColorModeProvider` and `useColorMode` have been removed in favor of `next-themes`
- `LightMode`, `DarkMode` and `ColorModeScript` components have been removed. You now have to use `className="light"` or `className="dark"` to force themes.
- `useColorModeValue` has been removed in favor of `useTheme` from `next-themes`
note
We provide snippets for color mode via the CLI to help you set up color mode quickly using `next-themes`
### [Hooks]()
We removed the hooks package in favor of using dedicated, robust libraries like `react-use` and `usehooks-ts`
The only hooks we ship now are `useBreakpointValue`, `useCallbackRef`, `useDisclosure`, `useControllableState` and `useMediaQuery`.
### [Style Config]()
We removed the `styleConfig` and `multiStyleConfig` concept in favor of recipes and slot recipes. This pattern was inspired by Panda CSS.
### [Next.js package]()
We've removed the `@chakra-ui/next-js` package in favor of using the `asChild` prop for better flexibility.
To style the Next.js image component, use the `asChild` prop on the `Box` component.
```
<Box asChild>
<NextImage />
</Box>
```
To style the Next.js link component, use the `asChild` prop on the
```
<Link isExternal asChild>
<NextLink />
</Link>
```
### [Theme Tools]()
We've removed this package in favor using CSS color mix.
**Before**
We used JS to resolve the colors and then apply the transparency
```
defineStyle({
bg: transparentize("blue.200", 0.16)(theme),
// -> rgba(0, 0, 255, 0.16)
})
```
**After**
We now use CSS color-mix
```
defineStyle({
bg: "blue.200/16",
// -> color-mix(in srgb, var(--chakra-colors-200), transparent 16%)
})
```
### [forwardRef]()
Due to the simplification of the `as` prop, we no longer provide a custom `forwardRef`. Prefer to use `forwardRef` from React directly.
### [Icons]()
Removed `@chakra-ui/icons` package. Prefer to use `lucide-react` or `react-icons` instead.
### [Storybook Addon]()
We're removed the storybook addon in favor of using `@storybook/addon-themes` and `withThemeByClassName` helper.
```
import { ChakraProvider, defaultSystem } from "@chakra-ui/react"
import { withThemeByClassName } from "@storybook/addon-themes"
import type { Preview, ReactRenderer } from "@storybook/react"
const preview: Preview = {
decorators: [
withThemeByClassName<ReactRenderer>({
defaultTheme: "light",
themes: {
light: "",
dark: "dark",
},
}),
(Story) => (
<ChakraProvider value={defaultSystem}>
<Story />
</ChakraProvider>
),
],
}
export default preview
```
### [Removed Components]()
- **Wrap**: Replace with `HStack` and add `wrap=wrap` to it.
- **WrapItem**: Replace with `Flex` and add `align=flex-start` to it.
- **StackItem**: You don't need this anymore. Use `Box` instead.
- **FocusLock**: We no longer ship a focus lock component. Use `react-focus-lock` instead.
- **FormControl** and **FormErrorMessage**: Replace with the `Field` component
## [Prop Changes]()
### [Boolean Props]()
Changed naming convention for boolean properties from `is<X>` to `<x>`
- `isOpen` -> `open`
- `defaultIsOpen` -> `defaultOpen`
- `isDisabled` -> `disabled`
- `isInvalid` -> `invalid`
- `isRequired` -> `required`
### [ColorScheme Prop]()
The `colorScheme` prop has been changed to `colorPalette`
**Before**
- You could only use `colorScheme` in a component's theme
- `colorScheme` clashes with the native `colorScheme` prop in HTML elements
```
<Button colorScheme="blue">Click me</Button>
```
**After**
- You can now use `colorPalette` anywhere
```
<Button colorPalette="blue">Click me</Button>
```
Usage in any component, you can do something like:
```
<Box colorPalette="red">
<Box bg="colorPalette.400">Some box</Box>
<Text color="colorPalette.600">Some text</Text>
</Box>
```
If you are using custom colors, you must define two things to make `colorPalette` work:
- **tokens**: For the 50-950 color palette
- **semanticTokens**: For the `solid`, `contrast`, `fg`, `muted`, `subtle`, `emphasized`, and `focusRing` color keys
theme.ts
```
import { createSystem, defaultConfig } from "@chakra-ui/react"
export const system = createSystem(defaultConfig, {
theme: {
tokens: {
colors: {
brand: {
50: { value: "#e6f2ff" },
100: { value: "#e6f2ff" },
200: { value: "#bfdeff" },
300: { value: "#99caff" },
// ...
950: { value: "#001a33" },
},
},
},
semanticTokens: {
colors: {
brand: {
solid: { value: "{colors.brand.500}" },
contrast: { value: "{colors.brand.100}" },
fg: { value: "{colors.brand.700}" },
muted: { value: "{colors.brand.100}" },
subtle: { value: "{colors.brand.200}" },
emphasized: { value: "{colors.brand.300}" },
focusRing: { value: "{colors.brand.500}" },
},
},
},
},
})
```
Read more about it [here](https://www.chakra-ui.com/guides/theming-custom-colors).
### [Gradient Props]()
Gradient style prop simplified to `gradient` and `gradientFrom` and `gradientTo` props. This reduces the runtime performance cost of parsing the gradient string, and allows for better type inference.
**Before**
```
<Box bgGradient="linear(to-r, red.200, pink.500)" />
```
**After**
```
<Box bgGradient="to-r" gradientFrom="red.200" gradientTo="pink.500" />
```
### [Color Palette]()
- Default color palette is now `gray` for all components but you can configure this in your theme.
- Default theme color palette size has been increased to 11 shades to allow more color variations.
**Before**
```
const colors = {
// ...
gray: {
50: "#F7FAFC",
100: "#EDF2F7",
200: "#E2E8F0",
300: "#CBD5E0",
400: "#A0AEC0",
500: "#718096",
600: "#4A5568",
700: "#2D3748",
800: "#1A202C",
900: "#171923",
},
}
```
**After**
```
const colors = {
// ...
gray: {
50: { value: "#fafafa" },
100: { value: "#f4f4f5" },
200: { value: "#e4e4e7" },
300: { value: "#d4d4d8" },
400: { value: "#a1a1aa" },
500: { value: "#71717a" },
600: { value: "#52525b" },
700: { value: "#3f3f46" },
800: { value: "#27272a" },
900: { value: "#18181b" },
950: { value: "#09090b" },
},
}
```
### [Style Props]()
Changed the naming convention for some style props
- `noOfLines` -> `lineClamp`
- `truncated` -> `truncate`
- `_activeLink` -> `_currentPage`
- `_activeStep` -> `_currentStep`
- `_mediaDark` -> `_osDark`
- `_mediaLight` -> `_osLight`
We removed the `apply` prop in favor of `textStyle` or `layerStyles`
### [Nested Styles]()
We have changed the way you write nested styles in Chakra UI components.
**Before**
Write nested styles using the `sx` or `__css` prop, and you sometimes don't get auto-completion for nested styles.
```
<Box
sx={{
svg: { color: "red.500" },
}}
/>
```
**After**
Write nested styles using the `css` prop. All nested selectors **require** the use of the ampersand `&` prefix
```
<Box
css={{
"& svg": { color: "red.500" },
}}
/>
```
This was done for two reasons:
- **Faster style processing:** Before we had to check if a style key is a style prop or a selector which is quite expensive overall.
- **Better typings:** This makes it easier to type nested style props are strongly typed
## [Component Changes]()
### [ChakraProvider]()
- Removed `theme` prop in favor of passing the `system` prop instead. Import the `defaultSystem` module instead of `theme`
- Removed `resetCss` prop in favor of passing `preflight: false` to the `createSystem` function
Before
```
<ChakraProvider resetCss={false}>
<Component />
</ChakraProvider>
```
After
```
const system = createSystem(defaultConfig, { preflight: false })
<Provider value={system}>
<Component />
</Provider>
```
- Removed support for configuring toast options. Pass it to the `createToaster` function in `components/ui/toaster.tsx` file instead.
### [Modal]()
- Renamed to `Dialog`
- Remove `isCentered` prop in favor of using the `placement=center` prop
- Removed `isOpen` and `onClose` props in favor of using the `open` and `onOpenChange` props
### [Avatar]()
- Remove `max` prop in favor of userland control
- Remove excess label part
- Move image related props to `Avatar.Image` component
- Move fallback icon to `Avatar.Fallback` component
- Move `name` prop to `Avatar.Fallback` component
### [Portal]()
- Remove `appendToParentPortal` prop in favor of using the `containerRef`
- Remove `PortalManager` component
### [Stack]()
- Changed `spacing` to `gap`
- Removed `StackItem` in favor of using the `Box` component directly
### [Collapse]()
- Rename `Collapse` to `Collapsible` namespace
- Rename `in` to `open`
- `animateOpacity` has been removed, use keyframes animations `expand-height` and `collapse-height` instead
Before
```
<Collapse in={isOpen} animateOpacity>
Some content
</Collapse>
```
After
```
<Collapsible.Root open={isOpen}>
<Collapsible.Content>Some content</Collapsible.Content>
</Collapsible.Root>
```
### [Image]()
- Now renders a native `img` without any fallback
- Remove `fallbackSrc` due to the SSR issues it causes
- Remove `useImage` hook
- Remove `Img` in favor of using the `Image` component directly
### [PinInput]()
- Changed `value`, `defaultValue` and `onChange` to use `string[]` instead of `string`
- Add new `PinInput.Control` and `PinInput.Label` component parts
- `PinInput.Root` now renders a `div` element by default. Consider combining with `Stack` or `Group` for better layout control
### [NumberInput]()
- Rename `NumberInputStepper` to `NumberInput.Control`
- Rename `NumberInputStepperIncrement` to `NumberInput.IncrementTrigger`
- Rename `NumberInputStepperDecrement` to `NumberInput.DecrementTrigger`
- Remove `focusBorderColor` and `errorBorderColor`, consider setting the `--focus-color` and `--error-color` css variables instead
Before
```
<NumberInput>
<NumberInputField />
<NumberInputStepper>
<NumberIncrementStepper />
<NumberDecrementStepper />
</NumberInputStepper>
</NumberInput>
```
After
```
<NumberInput.Root>
<NumberInput.Field />
<NumberInput.Control>
<NumberInput.IncrementTrigger />
<NumberInput.DecrementTrigger />
</NumberInput.Control>
</NumberInput.Root>
```
### [Divider]()
- Rename to `Separator`
- Switch to `div` element for better layout control
- Simplify component to rely on `borderTopWidth` and `borderInlineStartWidth`
- To change the thickness reliably, set the `--divider-border-width` css variable
### [Input, Select, Textarea]()
- Removed `invalid` prop in favor of wrapping the component in a `Field` component. This allows for adding a label, error text and asterisk easily.
Before
```
<Input invalid />
```
After
```
<Field invalid label="Email" errorText="This field is required">
<Input />
</Field>
```
### [Link]()
- Removed `isExternal` prop in favor of explicitly setting the `target` and `rel` props
Before
```
<Link isExternal>Click me</Link>
```
After
```
<Link target="_blank" rel="noopener noreferrer">
Click me
</Link>
```
### [IconButton]()
- Removed `icon` prop in favor of rendering the `children` prop directly
- Removed `isRounded` in favor of using the `borderRadius=full` prop
[Previous
\
Installation](https://www.chakra-ui.com/docs/get-started/installation)
[Next
\
CLI](https://www.chakra-ui.com/docs/get-started/cli) |
https://www.chakra-ui.com/showcase | # Showcase
Beautiful websites built with Chakra UI
[
\
**Chakra UI Pro**
\
Featured](https://pro.chakra-ui.com)
[
\
**Udacity**
\
EdTech platform](https://www.udacity.com/)
[
\
**Ethereum**
\
Blockchain community](https://ethereum.org/)
[
\
**Suno**
\
Music platform SaaS](https://suno.com/)
[
\
**Planet.com**
\
Travel platform](https://planet.com/)
[
\
**Solidity**
\
Blockchain language](https://soliditylang.org/)
[
\
**Prepin**
\
Real-Time AI Interview](https://prepin.ai/)
[
\
**yubo**
\
Social media platform](https://yubo.live/)
[
\
**Typebot**
\
AI chatbot builder](https://typebot.io)
[
\
**snappify**
\
Code snippet SaaS](https://snappify.com/)
[
\
**thirdweb**
\
Web3 development platform](https://thirdweb.com/)
[
\
**Supa Palette**
\
Color palette SaaS](https://supa-palette.com)
[
\
**Codiga**
\
AI-powered coding assistant](https://codiga.io)
[
\
**World Economic Forum**
\
Global policy platform](https://weforum.org/)
[
\
**iFixit**
\
Repair guide platform](https://ifixit.com/)
[
\
**BrandBird**
\
Image editor SaaS](https://brandbird.app)
[
\
**StockX**
\
eCommerce for sneakers](https://stockx.com)
[
\
**MockRocket**
\
3d mock builder](https://mockrocket.io/)
[
\
**Apollo Odyssey**
\
Docs website](https://www.apollographql.com/tutorials/)
[
\
**Stately.ai**
\
Statechart builder SaaS](https://stately.ai/registry/new)
[
\
**UI Foundations**
\
Education website](https://www.uifoundations.com/)
[
\
**Tweetpik**
\
Screenshot SaaS](https://tweetpik.com)
[
\
**Bitwarden**
\
Password manager](https://bitwarden.com) |
https://www.chakra-ui.com/docs/get-started/frameworks/next-pages | 1. Frameworks
2. Next.js (Pages)
# Using Chakra UI in Next.js (Pages)
A guide for installing Chakra UI with Next.js pages directory
## [Templates]()
Use one of the following templates to get started quickly. The templates are configured correctly to use Chakra UI.
[Next.js app template](https://github.com/chakra-ui/chakra-ui/tree/main/sandbox/next-app)
[Next.js pages template](https://github.com/chakra-ui/chakra-ui/tree/main/sandbox/next-pages)
## [Installation]()
The minimum node version required is Node.20.x
1
### [Install dependencies]()
```
npm i @chakra-ui/react @emotion/react
```
2
### [Add snippets]()
Snippets are pre-built components that you can use to build your UI faster. Using the `@chakra-ui/cli` you can add snippets to your project.
```
npx @chakra-ui/cli snippet add
```
3
### [Update tsconfig]()
If you're using TypeScript, you need to update the `compilerOptions` in the tsconfig file to include the following options:
```
{
"compilerOptions": {
"target": "ESNext",
"module": "ESNext",
"moduleResolution": "Bundler",
"skipLibCheck": true,
"paths": {
"@/*": ["./src/*"]
}
}
}
```
If you're using JavaScript, create a `jsconfig.json` file and add the above code to the file.
4
### [Setup provider]()
Wrap your application with the `Provider` component at the root of your application.
This provider composes the following:
- `ChakraProvider` from `@chakra-ui/react` for the styling system
- `ThemeProvider` from `next-themes` for color mode
pages/\_app.tsx
```
import { Provider } from "@/components/ui/provider"
export default function App({ Component, pageProps }: AppProps) {
return (
<Provider>
<Component {...pageProps} />
</Provider>
)
}
```
In the `pages/_document.tsx` file, add the `suppressHydrationWarning` prop to the `html` element.
pages/\_document.tsx
```
import { Head, Html, Main, NextScript } from "next/document"
export default function Document() {
return (
<Html suppressHydrationWarning>
<Head />
<body>
<Main />
<NextScript />
</body>
</Html>
)
}
```
5
### [Optimize Bundle]()
We recommend using the `experimental.optimizePackageImports` feature in Next.js to optimize your bundle size by loading only the modules that you are actually using.
next.config.mjs
```
export default {
experimental: {
optimizePackageImports: ["@chakra-ui/react"],
},
}
```
This also helps to resolve warnings like:
```
[webpack.cache.PackFileCacheStrategy] Serializing big strings (xxxkiB)
```
6
### [Enjoy!]()
With the power of the snippets and the primitive components from Chakra UI, you can build your UI faster.
```
import { Button } from "@/components/ui/button"
import { HStack } from "@chakra-ui/react"
const Demo = () => {
return (
<HStack>
<Button>Click me</Button>
<Button>Click me</Button>
</HStack>
)
}
```
[Previous
\
Next.js (App)](https://www.chakra-ui.com/docs/get-started/frameworks/next-app)
[Next
\
Remix](https://www.chakra-ui.com/docs/get-started/frameworks/remix) |
https://www.chakra-ui.com/docs/get-started/contributing | 1. Overview
2. Contributing
# Contributing to Chakra UI
The guide to contributing to Chakra UI v3.0
Thanks for showing interest to contribute to Chakra UI 💖, you rock!
## [Overview]()
Here are a few ways you can help improve Chakra UI
- **Improve the documentation**: Add new demos, fix typos, or add missing information.
- **Add new demos**: Add new component demos to the website and storybook. Open a PR to `apps/compositions/src/examples`
- **Fix bugs**: Report bugs, fix bugs, or add missing features.
- **Contribute to the code**: Propose new features by opening a Github Discussion, or find existing bugs to work on.
- **Improve the code**: Improve the code, fix bugs, or add missing features.
info
We welcome all contributions, no matter how big or small.
## [Architecture]()
Chakra v3.x is a composition of two projects in the Chakra ecosystem, Ark UI and Zag.js. The goal is to maintain as little code as possible in Chakra UI, and delegate the heavy lifting to these projects.
[Zag.js
\
Component logic modelled as a state machine](https://github.com/chakra-ui/zag)
[Ark UI
\
State machine from Zag.js converted to headless UI components](https://github.com/chakra-ui/ark)
### [Filing Issues]()
The mindset for filing issues on Chakra v3.x works like this:
- If the issue is a logic or accessibility bug, then it's most likely a bug in Zag.js. Consider opening an issue in the Zag.js repository.
- If it's a styling issue, then you can fix it directly in the Chakra UI repo.
### [Feature Requests]()
The mindset for filing feature requests on Chakra v3.x works like this:
- If the feature is a new component without logic, then it can go in Chakra UI or Ark UI. Start a discussion on the [Chakra UI repository](https://github.com/chakra-ui/chakra-ui)
- If the feature is a new component with logic, it belongs in Zag.js. Start a discussion on the [Zag.js repository](https://github.com/chakra-ui/zag).
## [Local Setup]()
- Clone the repository
```
git clone https://github.com/chakra-ui/chakra-ui.git
```
- Install dependencies with pnpm
```
pnpm install
```
- Build local version of all packages
```
pnpm build:fast
```
- Start storybook
```
pnpm storybook
```
- Start documentation website
```
pnpm docs dev
```
- Run tests
```
pnpm test
```
## [Recommended Extensions]()
We recommend using the following extensions in your editor:
- [ESLint](https://eslint.org/)
- [Prettier](https://prettier.io/)
- [EditorConfig](https://editorconfig.org/)
- [MDX](https://mdxjs.com/)
[Previous
\
CLI](https://www.chakra-ui.com/docs/get-started/cli)
[Next
\
Showcase](https://www.chakra-ui.com/docs/get-started//showcase) |
https://www.chakra-ui.com/docs/get-started/frameworks/next-app | 1. Frameworks
2. Next.js (App)
# Using Chakra UI in Next.js (App)
A guide for installing Chakra UI with Next.js app directory
## [Templates]()
Use one of the following templates to get started quickly. The templates are configured correctly to use Chakra UI.
[Next.js app template](https://github.com/chakra-ui/chakra-ui/tree/main/sandbox/next-app)
[Next.js pages template](https://github.com/chakra-ui/chakra-ui/tree/main/sandbox/next-pages)
## [Installation]()
The minimum node version required is Node.20.x
1
### [Install dependencies]()
```
npm i @chakra-ui/react @emotion/react
```
2
### [Add snippets]()
Snippets are pre-built components that you can use to build your UI faster. Using the `@chakra-ui/cli` you can add snippets to your project.
```
npx @chakra-ui/cli snippet add
```
3
### [Update tsconfig]()
If you're using TypeScript, you need to update the `compilerOptions` in the tsconfig file to include the following options:
```
{
"compilerOptions": {
"target": "ESNext",
"module": "ESNext",
"moduleResolution": "Bundler",
"skipLibCheck": true,
"paths": {
"@/*": ["./src/*"]
}
}
}
```
If you're using JavaScript, create a `jsconfig.json` file and add the above code to the file.
4
### [Setup provider]()
Wrap your application with the `Provider` component generated in the `components/ui/provider` component at the root of your application.
This provider composes the following:
- `ChakraProvider` from `@chakra-ui/react` for the styling system
- `ThemeProvider` from `next-themes` for color mode
app/layout.tsx
```
import { Provider } from "@/components/ui/provider"
export default function RootLayout(props: { children: React.ReactNode }) {
const { children } = props
return (
<html suppressHydrationWarning>
<body>
<Provider>{children}</Provider>
</body>
</html>
)
}
```
Adding the `suppressHydrationWarning` prop to the `html` element is required to prevent the warning about the `next-themes` library.
5
### [Optimize Bundle]()
We recommend using the `experimental.optimizePackageImports` feature in Next.js to optimize your bundle size by loading only the modules that you are actually using.
next.config.mjs
```
export default {
experimental: {
optimizePackageImports: ["@chakra-ui/react"],
},
}
```
This also helps to resolve warnings like:
```
[webpack.cache.PackFileCacheStrategy] Serializing big strings (xxxkiB)
```
6
### [Enjoy!]()
With the power of the snippets and the primitive components from Chakra UI, you can build your UI faster.
```
import { Button } from "@/components/ui/button"
import { HStack } from "@chakra-ui/react"
const Demo = () => {
return (
<HStack>
<Button>Click me</Button>
<Button>Click me</Button>
</HStack>
)
}
```
[Previous
\
Showcase](https://www.chakra-ui.com/docs/get-started//showcase)
[Next
\
Next.js (Pages)](https://www.chakra-ui.com/docs/get-started/frameworks/next-pages) |
https://www.chakra-ui.com/docs/get-started/frameworks/storybook | 1. Frameworks
2. Storybook
# Using Chakra UI in Storybook
A guide for using Chakra UI with Storybook
## [Installation]()
1
### [Install dependencies]()
Install the required dependencies for Chakra UI and Storybook.
```
npm i @storybook/addon-themes @chakra-ui/react @emotion/react
```
2
### [Setup Preview]()
Edit the `.storybook/preview.tsx` file to include the Chakra UI provider.
```
import { ChakraProvider, defaultSystem } from "@chakra-ui/react"
import type { Preview } from "@storybook/react"
const preview: Preview = {
// ...
decorators: [
(Story) => (
<ChakraProvider value={defaultSystem}>
<Story />
</ChakraProvider>
),
],
}
export default preview
```
3
### [Setup dark mode toggle]()
Use the `withThemeByClassName` decorator from `@storybook/addon-themes` to add a color mode toggle to the Storybook toolbar.
```
import { withThemeByClassName } from "@storybook/addon-themes"
import type { Preview, ReactRenderer } from "@storybook/react"
const preview: Preview = {
decorators: [
// ...
withThemeByClassName({
defaultTheme: "light",
themes: { light: "", dark: "dark" },
}),
],
}
export default preview
```
4
### [Start the Storybook server]()
```
npm run storybook
```
5
### [Enjoy!]()
Use Chakra UI components in your stories.
```
import { Button } from "@chakra-ui/react"
export const SampleStory = {
render() {
return <Button>Click me</Button>
},
}
```
[Previous
\
Remix](https://www.chakra-ui.com/docs/get-started/frameworks/remix)
[Next
\
Vite](https://www.chakra-ui.com/docs/get-started/frameworks/vite) |
https://www.chakra-ui.com/docs/get-started/frameworks/remix | 1. Frameworks
2. Remix
# Using Chakra in Remix
A guide for installing Chakra UI with Remix projects
## [Templates]()
Use the remix template below to get started quickly.
[Remix template](https://github.com/chakra-ui/chakra-ui/tree/main/sandbox/remix-ts)
## [Installation]()
1
### [Install dependencies]()
```
npm i @chakra-ui/react @emotion/react
```
2
### [Add snippets]()
Snippets are pre-built components that you can use to build your UI faster. Using the `@chakra-ui/cli` you can add snippets to your project.
```
npx @chakra-ui/cli snippet add
```
3
### [Setup emotion cache]()
Using snippets from the Remix sandbox, you can add the emotion cache to your application.
[Emotion cache snippet](https://github.com/chakra-ui/chakra-ui/blob/main/sandbox/remix-ts/app/emotion)
4
### [Update tsconfig]()
If you're using TypeScript, you need to update the `compilerOptions` in the tsconfig file to include the following options:
tsconfig.json
```
{
"compilerOptions": {
"target": "ESNext",
"module": "ESNext",
"moduleResolution": "Bundler",
"skipLibCheck": true
}
}
```
If you're using JavaScript, create a `jsconfig.json` file and add the above code to the file.
5
### [Setup provider]()
Wrap your application with the `Provider` component at the root of your application.
This provider composes the following:
- `ChakraProvider` from `@chakra-ui/react` for the styling system
- `ThemeProvider` from `next-themes` for color mode
app/root.tsx
```
import React from "react"
import ReactDOM from "react-dom/client"
import App from "./App"
import { Provider } from "@/components/ui/provider"
export default function App() {
return (
<Provider>
<Outlet />
</Provider>
)
}
```
6
### [Enjoy!]()
When the power of the snippets and the primitive components from Chakra UI, you can build your UI faster.
```
import { Button } from "@/components/ui/button"
import { HStack } from "@chakra-ui/react"
const Demo = () => {
return (
<HStack>
<Button>Click me</Button>
<Button>Click me</Button>
</HStack>
)
}
```
## [Known issues]()
You may encounter the following issues when using Chakra UI with Remix:
```
Error: There was an error while hydrating. Because the error happened outside of a Suspense boundary, the entire root will switch to client rendering.
```
This is a known issue related to extension installed in your browser. We recommend testing your application in incognito mode to see if the issue persists.
We welcome contributions to fix this issue.
[Previous
\
Next.js (Pages)](https://www.chakra-ui.com/docs/get-started/frameworks/next-pages)
[Next
\
Storybook](https://www.chakra-ui.com/docs/get-started/frameworks/storybook) |
https://www.chakra-ui.com/docs/get-started/frameworks/vite | 1. Frameworks
2. Vite
# Using Chakra in Vite
A guide for installing Chakra UI with Vite.js projects
## [Templates]()
Use the vite template below to get started quickly.
[Vite template](https://github.com/chakra-ui/chakra-ui/tree/main/sandbox/vite-ts)
## [Installation]()
The minimum node version required is Node.20.x
1
### [Install dependencies]()
```
npm i @chakra-ui/react @emotion/react
```
2
### [Add snippets]()
Snippets are pre-built components that you can use to build your UI faster. Using the `@chakra-ui/cli` you can add snippets to your project.
```
npx @chakra-ui/cli snippet add
```
3
### [Update tsconfig]()
If you're using TypeScript, in the `tsconfig.app.json` file, make sure the `compilerOptions` includes the following:
tsconfig.app.json
```
{
"compilerOptions": {
"target": "ESNext",
"module": "ESNext",
"moduleResolution": "Bundler",
"skipLibCheck": true,
"paths": {
"@/*": ["./src/*"]
}
}
}
```
If you're using JavaScript, create a `jsconfig.json` file and add the above code to the file.
4
### [Setup provider]()
Wrap your application with the `Provider` component at the root of your application.
This provider composes the following:
- `ChakraProvider` from `@chakra-ui/react` for the styling system
- `ThemeProvider` from `next-themes` for color mode
src/main.tsx
```
import { Provider } from "@/components/ui/provider"
import React from "react"
import ReactDOM from "react-dom/client"
import App from "./App"
ReactDOM.createRoot(document.getElementById("root")!).render(
<React.StrictMode>
<Provider>
<App />
</Provider>
</React.StrictMode>,
)
```
5
### [Setup Vite Config Paths]()
In your project, set up a vite config path to automatically sync `tsconfig` with vite using the command:
```
npm i -D vite-tsconfig-paths
```
Update the `vite.config.ts` file:
```
import react from "@vitejs/plugin-react"
import { defineConfig } from "vite"
import tsconfigPaths from "vite-tsconfig-paths"
// https://vitejs.dev/config/
export default defineConfig({
plugins: [react(), tsconfigPaths()],
})
```
6
### [Enjoy!]()
With the power of the snippets and the primitive components from Chakra UI, you can build your UI faster.
```
import { Button } from "@/components/ui/button"
import { HStack } from "@chakra-ui/react"
const Demo = () => {
return (
<HStack>
<Button>Click me</Button>
<Button>Click me</Button>
</HStack>
)
}
```
[Previous
\
Storybook](https://www.chakra-ui.com/docs/get-started/frameworks/storybook)
[Next
\
Shadow DOM](https://www.chakra-ui.com/docs/get-started/environments/shadow-dom) |
https://www.chakra-ui.com/docs/get-started/environments/shadow-dom | 1. Environments
2. Shadow DOM
# Using Chakra UI in Shadow DOM
A guide for installing Chakra UI with Shadow DOM
When developing extensions for browsers or using Chakra as part of a large project, leveraging the Shadow DOM is useful for style and logic encapsulation.
## [Template]()
Use the following template to get started quickly
[Shadow DOM template](https://github.com/chakra-ui/chakra-ui/tree/main/sandbox/shadow-dom)
## [Installation]()
The minimum node version required is Node.20.x
1
### [Install dependencies]()
```
npm i @chakra-ui/react @emotion/react @emotion/cache react-shadow
```
The additional packages used are:
- `react-shadow` used to create a Shadow DOM easily
- `@emotion/cache` used to create a custom insertion point for styles
2
### [Add snippets]()
Snippets are pre-built components that you can use to build your UI faster. Using the `@chakra-ui/cli` you can add snippets to your project.
```
npx @chakra-ui/cli snippet add
```
3
### [Update tsconfig]()
If you're using TypeScript, you need to update the `compilerOptions` in the tsconfig file to include the following options:
```
{
"compilerOptions": {
"module": "ESNext",
"moduleResolution": "Bundler",
"skipLibCheck": true,
"paths": {
"@/*": ["./src/*"]
}
}
}
```
4
### [Configure style engine]()
Create a `system.ts` file in the root of your project and configure the style engine.
components/ui/system.ts
```
import { createSystem, defaultConfig, defineConfig } from "@chakra-ui/react"
const varRoot = ":host"
const config = defineConfig({
cssVarsRoot: varRoot,
conditions: {
light: `${varRoot} &, .light &`,
},
preflight: { scope: varRoot },
globalCss: {
[varRoot]: defaultConfig.globalCss?.html ?? {},
},
})
export const system = createSystem(defaultConfig, config)
```
**Good to know**: The main purpose of the `system.ts` file is to configure the style engine to target the Shadow DOM.
5
### [Setup provider]()
Update the generated `components/ui/provider` component with the `Provider` component.
This provider composes the following:
- `ChakraProvider` from `@chakra-ui/react` for the styling system
- `EnvironmentProvider` from `react-shadow` to ensure Chakra components query the DOM correctly
- `CacheProvider` from `@emotion/react` to provide the custom insertion point
- `ThemeProvider` from `next-themes` for color mode
components/ui/provider.tsx
```
"use client"
import { ChakraProvider, EnvironmentProvider } from "@chakra-ui/react"
import createCache from "@emotion/cache"
import { CacheProvider } from "@emotion/react"
import { ThemeProvider, type ThemeProviderProps } from "next-themes"
import { useEffect, useState } from "react"
import root from "react-shadow/emotion"
import { system } from "./system"
export function Provider(props: ThemeProviderProps) {
const [shadow, setShadow] = useState<HTMLElement | null>(null)
const [cache, setCache] = useState<ReturnType<typeof createCache> | null>(
null,
)
useEffect(() => {
if (!shadow?.shadowRoot || cache) return
const emotionCache = createCache({
key: "root",
container: shadow.shadowRoot,
})
setCache(emotionCache)
}, [shadow, cache])
return (
<root.div ref={setShadow}>
{shadow && cache && (
<EnvironmentProvider value={() => shadow.shadowRoot ?? document}>
<CacheProvider value={cache}>
<ChakraProvider value={system}>
<ThemeProvider {...props} />
</ChakraProvider>
</CacheProvider>
</EnvironmentProvider>
)}
</root.div>
)
}
```
6
### [Use the provider]()
Wrap your application with the `Provider` component generated in the `components/ui/provider` component at the root of your application.
src/main.tsx
```
import { Provider } from "@/components/ui/provider"
import { StrictMode } from "react"
import { createRoot } from "react-dom/client"
import App from "./App.tsx"
createRoot(document.getElementById("root")!).render(
<StrictMode>
<Provider>
<App />
</Provider>
</StrictMode>,
)
```
7
### [Enjoy!]()
With the power of the snippets and the primitive components from Chakra UI, you can build your UI faster.
```
import { Button } from "@/components/ui/button"
import { HStack } from "@chakra-ui/react"
export default function App() {
return (
<HStack>
<Button>Click me</Button>
<Button>Click me</Button>
</HStack>
)
}
```
[Previous
\
Vite](https://www.chakra-ui.com/docs/get-started/frameworks/vite)
[Next
\
Iframe](https://www.chakra-ui.com/docs/get-started/environments/iframe) |
https://www.chakra-ui.com/docs/get-started/environments/iframe | 1. Environments
2. Iframe
# Using Chakra UI in Iframe
A guide for installing and using Chakra UI in an Iframe
Iframes are useful for isolating styles and logic in a separate context. For example, you might want to showcase a Chakra component in dedicated sandbox.
## [Template]()
Use the following template to get started quickly
[Iframe template](https://github.com/chakra-ui/chakra-ui/tree/main/sandbox/iframe)
## [Installation]()
The minimum node version required is Node.20.x
1
### [Install dependencies]()
```
npm i @chakra-ui/react @emotion/react @emotion/cache react-frame-component
```
The additional packages used are:
- `react-frame-component` used to create an iframe easily
- `@emotion/cache` used to create a custom insertion point for styles
2
### [Add snippets]()
Snippets are pre-built components that you can use to build your UI faster. Using the `@chakra-ui/cli` you can add snippets to your project.
```
npx @chakra-ui/cli snippet add
```
3
### [Update tsconfig]()
If you're using TypeScript, you need to update the `compilerOptions` in the tsconfig file to include the following options:
```
{
"compilerOptions": {
"module": "ESNext",
"moduleResolution": "Bundler",
"skipLibCheck": true,
"paths": {
"@/*": ["./src/*"]
}
}
}
```
4
### [Setup Iframe]()
Create a `components/ui/iframe-provider.tsx` file to setup the iframe using the `react-frame-component` package.
components/ui/iframe-provider.tsx
```
import {
ChakraProvider,
EnvironmentProvider,
defaultSystem,
} from "@chakra-ui/react"
import createCache from "@emotion/cache"
import { CacheProvider } from "@emotion/react"
import Iframe, { FrameContextConsumer } from "react-frame-component"
function memoize<T extends object, R>(func: (arg: T) => R): (arg: T) => R {
const cache = new WeakMap<T, R>()
return (arg: T) => {
if (cache.has(arg)) return cache.get(arg)!
const ret = func(arg)
cache.set(arg, ret)
return ret
}
}
const createCacheFn = memoize((container: HTMLElement) =>
createCache({ container, key: "frame" }),
)
export const IframeProvider = (props: React.PropsWithChildren) => {
const { children } = props
return (
<Iframe>
<FrameContextConsumer>
{(frame) => {
const head = frame.document?.head
if (!head) return null
return (
<CacheProvider value={createCacheFn(head)}>
<EnvironmentProvider value={() => head.ownerDocument}>
<ChakraProvider value={defaultSystem}>
{children}
</ChakraProvider>
</EnvironmentProvider>
</CacheProvider>
)
}}
</FrameContextConsumer>
</Iframe>
)
}
```
5
### [Setup provider]()
Wrap your application with the `Provider` component generated in the `components/ui/provider` component at the root of your application.
This provider composes the following:
- `ChakraProvider` from `@chakra-ui/react` for the styling system
- `ThemeProvider` from `next-themes` for color mode
```
import { Provider } from "@/components/ui/provider"
import React from "react"
import ReactDOM from "react-dom/client"
import App from "./App"
ReactDOM.createRoot(document.getElementById("root")!).render(
<React.StrictMode>
<Provider>
<App />
</Provider>
</React.StrictMode>,
)
```
6
### [Use the IframeProvider]()
At any component in your application, wrap it with the `IframeProvider` component to render it inside the iframe.
src/App.tsx
```
import { Button, Container, Heading, Stack } from "@chakra-ui/react"
import { IframeProvider } from "./components/ui/iframe-provider"
function App() {
return (
<Container py="8">
<Heading mb="5">Outside Iframe</Heading>
<IframeProvider>
<Stack p="6" align="flex-start" border="1px solid red">
<Heading>Inside Iframe</Heading>
<Button>Click me</Button>
</Stack>
</IframeProvider>
</Container>
)
}
export default App
```
## [Customization]()
If you created a custom theme using the `createSystem` function, ensure it's passed to the `IframeProvider` and `Provider` components to ensure it's used inside the iframe.
For example, let's say you created a custom theme:
```
export const system = createSystem(defaultConfig, {
theme: { colors: {} },
})
```
Then, pass it to the `IframeProvider` and `Provider` components:
```
<ChakraProvider value={system}>{/* ... */}</ChakraProvider>
```
[Previous
\
Shadow DOM](https://www.chakra-ui.com/docs/get-started/environments/shadow-dom)
[Next
\
Overview](https://www.chakra-ui.com/docs/components/concepts/overview) |
https://www.chakra-ui.com/guides/theming-change-default-color-palette | Build faster with Premium Chakra UI Components 💎
[Learn more](https://pro.chakra-ui.com?utm_source=chakra-ui.com)
[](https://www.chakra-ui.com/)
[Docs](https://www.chakra-ui.com/docs/get-started/installation)[Playground](https://www.chakra-ui.com/playground)[Guides](https://www.chakra-ui.com/guides)[Blog](https://www.chakra-ui.com/blog)
[](https://github.com/chakra-ui/chakra-ui)
1. [Guides](https://www.chakra-ui.com/guides)
2. theming
## Change the default color palette
December 4, 2024
By default, Chakra UI uses the `gray` color palette for various UI elements like selection backgrounds and hover states. You can customize this default behavior by modifying the global CSS configuration.
## [Customizing the default color palette]()
Use the `createSystem` method to override the default color palette in your theme configuration:
components/theme.ts
```
const config = defineConfig({
globalCss: {
html: {
colorPalette: "blue", // Change this to any color palette you prefer
},
},
})
export const system = createSystem(defaultConfig, config)
```
Next, add the new `system` to your `components/ui/provider.tsx` file:
```
"use client"
import { system } from "@/components/theme"
import { ChakraProvider } from "@chakra-ui/react"
export function Provider(props: ColorModeProviderProps) {
return (
<ChakraProvider value={system}>
<ColorModeProvider {...props} />
</ChakraProvider>
)
}
```
## [What this affects]()
Changing the default color palette will affect various UI elements across your application, including:
- Text selection background colors
- Default hover states
- Focus states
- Other interactive elements that use the default color palette
## [Using custom color palettes]()
You can use any of the built-in color palettes or your own custom color palette:
```
// Built-in color palettes
colorPalette: "blue"
colorPalette: "green"
colorPalette: "red"
// Custom color palette (if defined in your theme)
colorPalette: "brand"
```
warning
When using a custom color palette, make sure you've defined all the necessary color tokens (50-900) and semantic tokens in your theme configuration.
For more information about creating custom color palettes, see the guide on [creating custom colors](https://www.chakra-ui.com/docs/guides/theming-custom-colors). |
https://www.chakra-ui.com/guides/theming-add-custom-font-to-vite | Build faster with Premium Chakra UI Components 💎
[Learn more](https://pro.chakra-ui.com?utm_source=chakra-ui.com)
[](https://www.chakra-ui.com/)
[Docs](https://www.chakra-ui.com/docs/get-started/installation)[Playground](https://www.chakra-ui.com/playground)[Guides](https://www.chakra-ui.com/guides)[Blog](https://www.chakra-ui.com/blog)
[](https://github.com/chakra-ui/chakra-ui)
1. [Guides](https://www.chakra-ui.com/guides)
2. theming
## Add custom fonts to a Vite project
November 18, 2024
## [Loading the custom fonts]()
To add a custom font in your project, install the Google font you want to use from [Fontsource](https://fontsource.org/). For this guide, we'll use the ["Bricolage Grotesque"](https://fontsource.org/fonts/bricolage-grotesque) font as an example.
```
pnpm add @fontsource-variable/bricolage-grotesque
```
Next, import the font at the root of your project, referencing the `css` path:
main.tsx
```
import "@fontsource-variable/bricolage-grotesque/index.css"
```
## [Configure the custom font]()
Use the `createSystem` method to define the custom font in Chakra UI's theme configuration:
components/ui/provider.tsx
```
"use client"
import { createSystem, defaultConfig } from "@chakra-ui/react"
const system = createSystem(defaultConfig, {
theme: {
tokens: {
fonts: {
heading: { value: "Bricolage Grotesque Variable" },
body: { value: "Bricolage Grotesque Variable" },
},
},
},
})
```
info
You can customize which text elements use the font by specifying it for `heading`, `body`, or both. In this case, we are setting both the body and heading fonts to "Bricolage Grotesque".
Finally, pass the `system` into the `ChakraProvider`
components/ui/provider.tsx
```
export function Provider(props: ColorModeProviderProps) {
return (
<ChakraProvider value={system}>
<ColorModeProvider {...props} />
</ChakraProvider>
)
}
```
This ensures that the custom font is applied across your entire app. |
https://www.chakra-ui.com/guides/theming-customize-dark-mode-colors | Build faster with Premium Chakra UI Components 💎
[Learn more](https://pro.chakra-ui.com?utm_source=chakra-ui.com)
[](https://www.chakra-ui.com/)
[Docs](https://www.chakra-ui.com/docs/get-started/installation)[Playground](https://www.chakra-ui.com/playground)[Guides](https://www.chakra-ui.com/guides)[Blog](https://www.chakra-ui.com/blog)
[](https://github.com/chakra-ui/chakra-ui)
1. [Guides](https://www.chakra-ui.com/guides)
2. theming
## Customize dark mode colors
December 5, 2024
You can customize the dark mode colors in Chakra UI by modifying the `_dark` values of the semantic color tokens. This guide will show you how to override the default dark mode colors.
## [Customizing dark mode colors]()
Use the `createSystem` method to override the dark mode colors in your theme configuration:
components/theme.ts
```
const config = defineConfig({
theme: {
semanticTokens: {
colors: {
bg: {
DEFAULT: {
value: { _light: "{colors.white}", _dark: "#141414" }, // Custom dark background
},
subtle: {
value: { _light: "{colors.gray.50}", _dark: "#1a1a1a" }, // Custom dark subtle background
},
muted: {
value: { _light: "{colors.gray.100}", _dark: "#262626" }, // Custom dark muted background
},
},
fg: {
DEFAULT: {
value: { _light: "{colors.black}", _dark: "#e5e5e5" }, // Custom dark text color
},
muted: {
value: { _light: "{colors.gray.600}", _dark: "#a3a3a3" }, // Custom dark muted text
},
},
border: {
DEFAULT: {
value: { _light: "{colors.gray.200}", _dark: "#404040" }, // Custom dark border
},
},
},
},
},
})
export const system = createSystem(defaultConfig, config)
```
info
To see the full list of semantic color tokens, see the [semantic tokens source code](https://github.com/chakra-ui/chakra-ui/blob/main/packages/react/src/theme/semantic-tokens/colors.ts).
Next, add the new `system` to your `components/ui/provider.tsx` file:
```
"use client"
import { system } from "@/components/theme"
import { ChakraProvider } from "@chakra-ui/react"
export function Provider(props: ColorModeProviderProps) {
return (
<ChakraProvider value={system}>
<ColorModeProvider {...props} />
</ChakraProvider>
)
}
```
## [Understanding semantic color tokens]()
The semantic color tokens in Chakra UI follow this pattern:
```
{
[colorKey]: {
[variant]: {
value: {
_light: string, // Light mode value
_dark: string // Dark mode value
}
}
}
}
```
Common color keys include:
- `bg` - Background colors
- `fg` - Foreground/text colors
- `border` - Border colors
Each color key has variants like:
- `DEFAULT` - Base color
- `subtle` - Subtle variation
- `muted` - Muted variation
- `emphasized` - Emphasized variation
## [Customizing specific color palettes]()
You can also customize specific color palettes for dark mode:
```
const config = defineConfig({
theme: {
semanticTokens: {
colors: {
blue: {
solid: {
value: { _light: "{colors.blue.600}", _dark: "#0284c7" }, // Custom dark blue
},
muted: {
value: { _light: "{colors.blue.100}", _dark: "#082f49" }, // Custom dark muted blue
},
},
// Add more color palettes as needed
},
},
},
})
```
## [Best practices]()
1. **Color contrast**: Ensure your custom dark mode colors maintain sufficient contrast for accessibility.
2. **Consistent palette**: Keep your dark mode colors consistent across your application by using a cohesive color palette.
3. **Testing**: Always test your custom colors in both light and dark modes to ensure good readability and visual harmony.
For more information about semantic tokens, see the [semantic tokens guide](https://www.chakra-ui.com/docs/theming/semantic-tokens). |
https://www.chakra-ui.com/guides/theming-add-custom-font-to-nextjs | Build faster with Premium Chakra UI Components 💎
[Learn more](https://pro.chakra-ui.com?utm_source=chakra-ui.com)
[](https://www.chakra-ui.com/)
[Docs](https://www.chakra-ui.com/docs/get-started/installation)[Playground](https://www.chakra-ui.com/playground)[Guides](https://www.chakra-ui.com/guides)[Blog](https://www.chakra-ui.com/blog)
[](https://github.com/chakra-ui/chakra-ui)
1. [Guides](https://www.chakra-ui.com/guides)
2. theming
## Add custom fonts to a Next.js project
November 18, 2024
## [Loading the custom fonts]()
Google Fonts are available in Next.js by default. At the top of your `layout.tsx` file, import the font from `next/font/google`:
layout.tsx
```
import { Bricolage_Grotesque } from "next/font/google"
```
Configure the font by defining the variable and subsets to include:
layout.tsx
```
const bricolage = Bricolage_Grotesque({
variable: "--font-bricolage",
subsets: ["latin"],
})
```
Now, attach the font to the `<html>` element in your application. This ensures that the font is available to be used in Chakra UI.
```
<html className={bricolage.variable} lang="en" suppressHydrationWarning>
<body>
<Provider>{children}</Provider>
</body>
</html>
```
## [Configure the custom font]()
Use the `createSystem` method to define the custom font in Chakra UI's theme configuration:
components/ui/provider.tsx
```
"use client"
import { createSystem, defaultConfig } from "@chakra-ui/react"
const system = createSystem(defaultConfig, {
theme: {
tokens: {
fonts: {
heading: { value: "var(--font-bricolage)" },
body: { value: "var(--font-bricolage)" },
},
},
},
})
```
info
You can customize which text elements use the font by specifying it for `heading`, `body`, or both. In this case, we are setting both the body and heading fonts to "Bricolage Grotesque".
Finally, pass the `system` into the `ChakraProvider`
components/ui/provider.tsx
```
export function Provider(props: ColorModeProviderProps) {
return (
<ChakraProvider value={system}>
<ColorModeProvider {...props} />
</ChakraProvider>
)
}
```
This ensures that the custom font is applied across your entire app.
Remember to remove any unused styles from your `global.css` and `page.module.css` files to prevent your custom font from being overridden. |
https://www.chakra-ui.com/guides/theming-custom-colors | Build faster with Premium Chakra UI Components 💎
[Learn more](https://pro.chakra-ui.com?utm_source=chakra-ui.com)
[](https://www.chakra-ui.com/)
[Docs](https://www.chakra-ui.com/docs/get-started/installation)[Playground](https://www.chakra-ui.com/playground)[Guides](https://www.chakra-ui.com/guides)[Blog](https://www.chakra-ui.com/blog)
[](https://github.com/chakra-ui/chakra-ui)
1. [Guides](https://www.chakra-ui.com/guides)
2. theming
## Creating custom colors
November 15, 2024
To create custom colors in Chakra UI, you want to define two things:
- **tokens**: For the 50-950 color palette
- **semanticTokens**: For the `solid`, `contrast`, `fg`, `muted`, `subtle`, `emphasized`, and `focusRing` color keys
To learn more about tokens and semantic tokens, refer to the [theming guide](https://www.chakra-ui.com/docs/theming/customization/colors).
components/theme.ts
```
const config = defineConfig({
theme: {
tokens: {
colors: {
brand: {
50: { value: "#e6f2ff" },
100: { value: "#e6f2ff" },
200: { value: "#bfdeff" },
300: { value: "#99caff" },
// ...
950: { value: "#001a33" },
},
},
},
semanticTokens: {
colors: {
brand: {
solid: { value: "{colors.brand.500}" },
contrast: { value: "{colors.brand.100}" },
fg: { value: "{colors.brand.700}" },
muted: { value: "{colors.brand.100}" },
subtle: { value: "{colors.brand.200}" },
emphasized: { value: "{colors.brand.300}" },
focusRing: { value: "{colors.brand.500}" },
},
},
},
},
})
export const system = createSystem(defaultConfig, config)
```
Next, you add the new `system` to your `components/ui/provider.tsx` files
```
"use client"
import { system } from "@/components/theme"
import {
ColorModeProvider,
type ColorModeProviderProps,
} from "@/components/ui/color-mode"
import { ChakraProvider } from "@chakra-ui/react"
export function Provider(props: ColorModeProviderProps) {
return (
<ChakraProvider value={system}>
<ColorModeProvider {...props} />
</ChakraProvider>
)
}
```
Next, you run the CLI `typegen` command to generate the types.
```
npx chakra typegen ./theme.ts
```
## [Using the color]()
With that in place, you can use the color in components as well as `colorPalette` utilities.
```
<Button colorPalette="brand">Click me</Button>
```
You can also use the semantic token directly.
```
<Box color="brand.contrast" bg="brand.solid">
Hello world
</Box>
```
Alternatively, you can use the raw token value.
```
<Box color="brand.500">Hello world</Box>
``` |
https://www.chakra-ui.com/guides/component-implement-context-menu | Build faster with Premium Chakra UI Components 💎
[Learn more](https://pro.chakra-ui.com?utm_source=chakra-ui.com)
[](https://www.chakra-ui.com/)
[Docs](https://www.chakra-ui.com/docs/get-started/installation)[Playground](https://www.chakra-ui.com/playground)[Guides](https://www.chakra-ui.com/guides)[Blog](https://www.chakra-ui.com/blog)
[](https://github.com/chakra-ui/chakra-ui)
1. [Guides](https://www.chakra-ui.com/guides)
2. components
## Implement a Context Menu
October 19, 2024
A context menu provides users with a convenient way to access actions related to a specific UI element through a right-click.
Utilize the `MenuContextTrigger` component to create a context menu.
PreviewCode
Right click here
New Text File
New File...
New Window
Open File...
Export
```
import { Center } from "@chakra-ui/react"
import {
MenuContent,
MenuContextTrigger,
MenuItem,
MenuRoot,
} from "@/components/ui/menu"
const Demo = () => {
return (
<MenuRoot>
<MenuContextTrigger w="full">
<Center
width="full"
height="40"
userSelect="none"
borderWidth="2px"
borderStyle="dashed"
rounded="lg"
padding="4"
>
Right click here
</Center>
</MenuContextTrigger>
<MenuContent>
<MenuItem value="new-txt">New Text File</MenuItem>
<MenuItem value="new-file">New File...</MenuItem>
<MenuItem value="new-win">New Window</MenuItem>
<MenuItem value="open-file">Open File...</MenuItem>
<MenuItem value="export">Export</MenuItem>
</MenuContent>
</MenuRoot>
)
}
```
For more details on Chakra UI's menu components, refer to the [documentation](https://www.chakra-ui.com/docs/components/menu). |
https://www.chakra-ui.com/guides/component-floating-input-label | Build faster with Premium Chakra UI Components 💎
[Learn more](https://pro.chakra-ui.com?utm_source=chakra-ui.com)
[](https://www.chakra-ui.com/)
[Docs](https://www.chakra-ui.com/docs/get-started/installation)[Playground](https://www.chakra-ui.com/playground)[Guides](https://www.chakra-ui.com/guides)[Blog](https://www.chakra-ui.com/blog)
[](https://github.com/chakra-ui/chakra-ui)
1. [Guides](https://www.chakra-ui.com/guides)
2. components
## Add a Floating Label to an Input
October 19, 2024
Floating labels conserve space in forms, particularly on mobile devices where screen space is limited.
To add a floating label to an input component in Chakra UI, create a styled input field using the `Field`, `Input`, and `Box` components.
PreviewCode
Email
```
import { Box, Field, Input, defineStyle } from "@chakra-ui/react"
const Demo = () => {
return (
<Field.Root>
<Box pos="relative" w="full">
<Input className="peer" placeholder="" />
<Field.Label css={floatingStyles}>Email</Field.Label>
</Box>
</Field.Root>
)
}
const floatingStyles = defineStyle({
pos: "absolute",
bg: "bg",
px: "0.5",
top: "-3",
insetStart: "2",
fontWeight: "normal",
pointerEvents: "none",
transition: "position",
_peerPlaceholderShown: {
color: "fg.muted",
top: "2.5",
insetStart: "3",
},
_peerFocusVisible: {
color: "fg",
top: "-3",
insetStart: "2",
},
})
```
For more details on Chakra UI's input component, refer to the [documentation](https://www.chakra-ui.com/docs/components/input). |
https://www.chakra-ui.com/guides/snippet-how-to-manage-updates | Build faster with Premium Chakra UI Components 💎
[Learn more](https://pro.chakra-ui.com?utm_source=chakra-ui.com)
[](https://www.chakra-ui.com/)
[Docs](https://www.chakra-ui.com/docs/get-started/installation)[Playground](https://www.chakra-ui.com/playground)[Guides](https://www.chakra-ui.com/guides)[Blog](https://www.chakra-ui.com/blog)
[](https://github.com/chakra-ui/chakra-ui)
1. [Guides](https://www.chakra-ui.com/guides)
2. snippets
## How to manage updates
November 15, 2024
When snippets are updated from Chakra UI, we document them in the changelog so you're aware.
In your project, it's up to you to decide whether to update the snippets or not. In most cases, you can check the [snippet directory](https://github.com/chakra-ui/chakra-ui/tree/main/apps/compositions/src/ui) to see the changes.
When running the `npx chakra snippet add` command, the CLI will check for existing snippets in your project and skip adding them if they already exist.
If you want to overwrite the existing snippets, run the following command:
```
npx chakra snippet add --force
``` |
https://www.chakra-ui.com/guides/snippet-specify-custom-directory | Build faster with Premium Chakra UI Components 💎
[Learn more](https://pro.chakra-ui.com?utm_source=chakra-ui.com)
[](https://www.chakra-ui.com/)
[Docs](https://www.chakra-ui.com/docs/get-started/installation)[Playground](https://www.chakra-ui.com/playground)[Guides](https://www.chakra-ui.com/guides)[Blog](https://www.chakra-ui.com/blog)
[](https://github.com/chakra-ui/chakra-ui)
1. [Guides](https://www.chakra-ui.com/guides)
2. snippets
## Specifying custom directory
November 15, 2024
The generated snippets by the Chakra UI CLI are placed in the `components/ui` directory by default.
You can specify a custom directory by running the CLI with the `--outdir` flag.
```
npx chakra snippets --outdir ./components/custom
```
To learn more about the CLI snippets command, refer to the [CLI](https://www.chakra-ui.com/docs/get-started/cli) docs. |
https://www.chakra-ui.com/guides/theming-custom-breakpoints | Build faster with Premium Chakra UI Components 💎
[Learn more](https://pro.chakra-ui.com?utm_source=chakra-ui.com)
[](https://www.chakra-ui.com/)
[Docs](https://www.chakra-ui.com/docs/get-started/installation)[Playground](https://www.chakra-ui.com/playground)[Guides](https://www.chakra-ui.com/guides)[Blog](https://www.chakra-ui.com/blog)
[](https://github.com/chakra-ui/chakra-ui)
1. [Guides](https://www.chakra-ui.com/guides)
2. theming
## Creating custom breakpoints
November 15, 2024
Custom breakpoints are defined in the `breakpoints` property of your theme.
components/theme.ts
```
const config = defineConfig({
theme: {
breakpoints: {
xl: "80em" ,
"2xl": "96em" ,
"3xl": "120em" ,
"4xl": "160em" ,
},
},
})
export const system = createSystem(defaultConfig, config)
```
Next, you add the new `system` to your `components/ui/provider.tsx` files
```
"use client"
import { system } from "@/components/theme"
import {
ColorModeProvider,
type ColorModeProviderProps,
} from "@/components/ui/color-mode"
import { ChakraProvider } from "@chakra-ui/react"
export function Provider(props: ColorModeProviderProps) {
return (
<ChakraProvider value={system}>
<ColorModeProvider {...props} />
</ChakraProvider>
)
}
```
Next, you run the CLI `typegen` command to generate the types.
```
npx chakra typegen ./components/theme.ts
```
Note: You might need to restart your TypeScript server for the types to be picked up.
## [Using the breakpoint]()
With that in place, you can use the breakpoints when writing responsive styles.
```
<Box fontSize={{ base: "sm", "4xl": "lg" }}>Hello world</Box>
``` |
https://www.chakra-ui.com/blog/04-chakra-ui-london-meetup-2024 | Build faster with Premium Chakra UI Components 💎
[Learn more](https://pro.chakra-ui.com?utm_source=chakra-ui.com)
[](https://www.chakra-ui.com/)
[Docs](https://www.chakra-ui.com/docs/get-started/installation)[Playground](https://www.chakra-ui.com/playground)[Guides](https://www.chakra-ui.com/guides)[Blog](https://www.chakra-ui.com/blog)
[](https://github.com/chakra-ui/chakra-ui)
article·
December 3, 2024
## Chakra UI London Meetup 2024 Recap
EA
Esther Adebayo
@\_estheradebayo
The Chakra UI London Meetup was a special evening where we got to meet face-to-face with many community members who we had only known through online interactions in the open source world. Some of these connections spanned months or even years, making the in-person gathering even more meaningful.
The event held on Thursday, November 28th, 2024 where we talked about Chakra UI v3, and the future of Chakra UI.
The evening kicked off with light refreshments and chats. We exchanged ideas, shared our favorite Chakra components, and of course, grabbed some stickers.

## [Talks]()
We had three amazing talks that evening, each bringing unique insights into Chakra UI's evolution and new features.
### [Building Chakra UI v3]()
The opening talk was delivered by [Sage](https://x.com/thesegunadebayo), the creator of Chakra UI. His talk provided a technical deep dive into the process behind building Chakra UI v3, going from the logic layer to the presentation layer.

Key highlights from the talk:
- **Component Design**: Using the slider component, Sage showcased how components are now built in Chakra UI. Going from the headless layer to the presentation layer, and leveraging state machines to handle interactions.
- **Logic Layer** powered by [Zag.js](https://zagjs.com/)
- **Headless UI Layer** handled by [Ark UI](https://ark-ui.com/)
- **Presentation Layer** handled by Chakra's theming system
- **Component Anatomy**: He broke down the structure of Chakra UI components into separate parts so create a shared language for developers and designers.
- **Component API**: Sage emphasized how Chakra UI v3 leverages both open and closed component APIs to provide a flexible and consistent developer experience.
[View the slides](https://www.figma.com/deck/WbCGjQGQCupXNvCiSqMHsf/Chakra-Meetup-Nov.?node-id=1-25&node-type=slide&viewport=-136%2C-13%2C0.63&t=tAFo6Oa8uI2pxIW5-1&scaling=min-zoom&content-scaling=fixed&page-id=0%3A1)
### [Hidden Gems of Chakra UI v3]()
Next up, [Ivica Batinić](https://x.com/_isBatak) took the stage to highlight new features in Chakra v3, showing how the theming system works, and ways to improve runtime performance of dynamic styles.

Key highlights from the talk:
- **Multipart Component Nuances**: Understanding the finer details of multipart components.
- **Performant Dynamic Styles**: Techniques for optimizing styles dynamically.
- **Modernized Polymorphic Patterns**: Leveraging patterns that boost flexibility in component usage.
- **Advanced Styling Features**: Unlocking powerful new styling capabilities.
[View the slides](https://hidden-gems-of-chakra-ui-v3.vercel.app/1)
### [Exploring Chakra's Color Picker]()
The final talk of the night was led by [Esther Adebayo](https://x.com/_estheradebayo), who gave a stunning demo of the new Color Picker component in Chakra UI v3, showing how to build a page with real-time color customization.

Key highlights from the talk:
- **Color Picker Overview**: Why a Color Picker is an essential tool for any design system.
- **Design Considerations**: What makes a good color picker component, and how to build one.
- **Component Anatomy**: A walkthrough of the Color Picker's anatomy.
- **Live Demo**: Esther demoed the ColorPicker component, showing how it can be used to style a login screen in real time.
[Watch the talk](https://youtu.be/neesBbYuGT0)
## [Conclusion]()
We wrapped up the evening with a Q&A session where attendees got the chance to ask their questions, from technical details to what's next for Chakra UI.
A huge thank you to everyone who attended and made this meetup so special. We couldn't do this without you, and we look forward to the next one.

## [Connect with us]()
- Join our [Discord community](https://discord.gg/chakra-ui).
- Follow us on [X](https://x.com/chakra_ui).
- Keep an eye out for future events!
Until next time, keep building with Chakra UI! |
https://www.chakra-ui.com/blog/03-building-consistent-uis-with-design-tokens | Build faster with Premium Chakra UI Components 💎
[Learn more](https://pro.chakra-ui.com?utm_source=chakra-ui.com)
[](https://www.chakra-ui.com/)
[Docs](https://www.chakra-ui.com/docs/get-started/installation)[Playground](https://www.chakra-ui.com/playground)[Guides](https://www.chakra-ui.com/guides)[Blog](https://www.chakra-ui.com/blog)
[](https://github.com/chakra-ui/chakra-ui)
article·
November 24, 2024
## Building Consistent UIs with Design Tokens
EA
Esther Adebayo
@\_estheradebayo
Design tokens allow developers to create maintainable, scalable, and easy to manage user interfaces.
They create a shared language between designers and developers, allowing them to reference standardized values instead of hard-coding specific styles. This way, rather than updating styles individually across components, developers can adjust token values, which are then applied globally.
In this article, I'll cover how you can leverage Chakra UI's built-in design tokens to maintain consistency across all your applications.
## [What Are Design Tokens?]()
Design tokens are key-value pairs that represent the visual properties and UI elements of a design system, such as colors, typography, spacing and more. They play a key role in allowing you to create scalable, cohesive, and consistent user interfaces.
They serve as the foundation of a design system, allowing developers and designers to implement consistent styles across components and projects efficiently. By abstracting visual properties into tokens, design tokens create a shared language between design and development teams, ensuring cohesive designs.
In Chakra UI, these tokens fall into two main categories:
- **Core Tokens**: Fundamental properties such as colors, font sizes, spacing, and borders.
- **Semantic Tokens**: Higher-level tokens tied to themes that enable easy style updates across dark and light modes or other design contexts.
## [Using Core Tokens for Scalability]()
Chakra UI's [core design tokens](https://www.chakra-ui.com/docs/theming/tokens) include foundational properties like **colors**, **spacing**, **typography**, **radii**, **borders** and more, which help you quickly set up your application's look and feel.
Here's how to use some of these tokens to streamline development:
- **Colors**: Chakra UI provides a wide palette of color tokens, ranging from `.50` to `.950` such as `gray.50`, `gray.300`, etc.
```
<Box bg="gray.300" p={4}>
Chakra Box with Core Color Token
</Box>
```
- **Spacing and Sizing**: Tokens for margin, padding and other sizing properties allow for consistent layout dimensions.
By default, Chakra includes a comprehensive numeric spacing scale where the values are proportional. So, 1 spacing unit is equal to `0.25rem`, which translates to `4px` by default in common browsers.
Using tokens like 4, 8, 12, etc., ensures a predictable layout behavior.
```
<Box padding={4} margin={2}>
Consistent Padding and Margin
</Box>
```
This translates to a padding of `16px` and a margin of `8px`.
- **Typography**: Chakra provides font size and weight tokens, so your app's text elements maintain a uniform appearance. By specifying tokens like `lg`, `md`, or `xl` for font sizes, you reduce the likelihood of inconsistent text styling.
```
<Text fontSize="lg" fontWeight="bold">
Styled with Font Tokens
</Text>
```
## [Customizing Core Tokens]()
To start [customizing tokens](https://www.chakra-ui.com/docs/theming/customization/colors), you need to extend the Chakra theme by using Chakra's `defineConfig` function. This function allows you to override Chakra's default tokens with your own values.
Let's look at how you can start by creating a custom `theme.ts` file. A design token in Chakra UI consists of the following properties:
- value: The value of the token. This can be any valid CSS value.
- description: An optional description of what the token can be used for.
```
import { createSystem, defaultConfig, defineConfig } from "@chakra-ui/react"
const customConfig = defineConfig({
theme: {
tokens: {
colors: {
brand: {
50: { value: "#e6f2ff" },
100: { value: "#e6f2ff" },
200: { value: "#bfdeff" },
300: { value: "#99caff" },
// ...
950: { value: "#001a33" },
},
},
},
},
})
export const system = createSystem(defaultConfig, customConfig)
```
Now, you can utilize your custom token where needed, such as:
```
<Box bg="brand.100">This Box uses a custom token</Box>
```
## [Using semantic tokens for contextual styling]()
[Semantic tokens](https://www.chakra-ui.com/docs/theming/semantic-tokens) allow for context-based styling and enables you to change the appearance of your app based on the theme or mode (e.g., light or dark mode).
```
<Button color="danger">Click me</Button>
```
In most cases, the value of a semantic token references to an existing token.
To reference a value in a semantic token, use the token reference `{}` syntax.
```
import { createSystem, defineConfig } from "@chakra-ui/react"
const config = defineConfig({
theme: {
tokens: {
colors: {
red: { value: "#EE0F0F" },
},
},
semanticTokens: {
colors: {
danger: { value: "{colors.red}" },
},
},
},
})
export default createSystem(config)
```
## [Customizing the semantic tokens]()
You can [create your own semantic tokens](https://www.chakra-ui.com/docs/theming/customization/colors) by extending Chakra's theme. This flexibility enables you to customize semantic tokens based on your brand's unique color scheme.
```
import { createSystem, defaultConfig, defineConfig } from "@chakra-ui/react"
const customConfig = defineConfig({
theme: {
semanticTokens: {
colors: {
"checkbox-border": {
value: { _light: "gray.200", _dark: "gray.800" },
},
},
},
},
})
export const system = createSystem(defaultConfig, customConfig)
```
## [Boost Collaboration with Design Tokens]()
Design tokens promote a unified approach to styling, which can drastically improve collaboration between developers and designers. Here's how design tokens in Chakra UI help:
- **Creating a Shared Visual Language**: Design tokens provide a standard set of values for developers and designers, ensuring everyone uses the same color palette, spacing, and typography values.
- **Efficiently Managing Global Design Changes**: Because design tokens are centrally managed, changing a token value updates all components using that token. For example, if you adjust a `primary.500` token to a new shade, every component using this token instantly reflects the change.
- **Enhanced Reusability and Maintainability**: With Chakra's tokens, components are designed to be reusable, enhancing your code's scalability. When you need to adjust a style across multiple components, you simply update the token rather than modifying each component individually.
## [Conclusion]()
Whether you're implementing core tokens for standard styles or using semantic tokens to adapt to theme changes, Chakra UI's design tokens make your interface clean, efficient, and easier to maintain.
By using a shared set of values, you're not only speeding up your development workflow but also ensuring your designs stay cohesive. Try it out in your next project, and experience firsthand how design tokens can simplify your work and improve collaboration across your team. |
https://www.chakra-ui.com/blog/01-chakra-v2-vs-v3-a-detailed-comparison | Build faster with Premium Chakra UI Components 💎
[Learn more](https://pro.chakra-ui.com?utm_source=chakra-ui.com)
[](https://www.chakra-ui.com/)
[Docs](https://www.chakra-ui.com/docs/get-started/installation)[Playground](https://www.chakra-ui.com/playground)[Guides](https://www.chakra-ui.com/guides)[Blog](https://www.chakra-ui.com/blog)
[](https://github.com/chakra-ui/chakra-ui)
article·
October 28, 2024
## Chakra v2 vs v3 - A Detailed Comparison
EA
Esther Adebayo
@\_estheradebayo
We recently launched Chakra UI version 3, which brings a huge set of changes, improvements and new features.
With a major update like this, you may wonder, what has changed, what’s new, and is migrating really worth it?
In this article, we'll take a deep dive into the key differences between version 2 and version 3. The goal is to give you a better understanding of version 3 and help you decide if its time to migrate your projects.
## [Simplified Installation]()
The installation process is one of the first noticeable differences. Previously, you had to install multiple packages to get Chakra working in your project:
- `@chakra-ui/react`
- `@emotion/react`
- `@emotion/styled`
- `framer-motion`
But with **v3**, things are much simpler. You only need:
- `@chakra-ui/react`
- `@emotion/react`
This reduces the number of dependencies and results in a quicker setup.
**version 2:**
```
npm install @chakra-ui/react @emotion/react @emotion/styled framer-motion
```
**version 3:**
```
npm install @chakra-ui/react @emotion/react
```
## [New Provider Setup]()
In previous versions, you had to wrap your application with `ChakraProvider` to access Chakra’s default theme. However, we've now replaced `ChakraProvider` with the `Provider` component.
`Provider` composes the following:
- `ChakraProvider` from `@chakra-ui/react` for the styling system
- `ThemeProvider` from `next-themes` for color mode
**version 2:**
```
import { ChakraProvider } from "@chakra-ui/react"
function App() {
return <ChakraProvider>{/* the rest of your app */}</ChakraProvider>
}
```
**version 3:**
```
import { Provider } from "@/components/ui/provider"
function App() {
return <Provider>{/* the rest of your app */}</Provider>
}
```
## [Component Snippets]()
A common pain point for developers is the repetitive task of building common UI components. To solve this, we’ve introduced Component Snippets.
Snippets are pre-built components designed to accelerate your development process.
With the new [Chakra CLI](https://www.chakra-ui.com/docs/get-started/cli) you can instantly add ready-made components to your project with a single command.
```
# Add all snippets
chakra snippet add
# Add a specific snippet
chakra snippet add button
```
Once you run the command, you'll find the components neatly organized in a `/components/ui` folder inside the **src** directory.
You can then import your desired component snippet directly from the components folder
```
import { Button } from "@/components/ui/button"
```
## [New Components]()
We listened to you, our community, and in this version, we added 25+ new components to enhance your development workflow.
Some of the new components include:
- File Upload
- Clipboard
- Timeline
- Password Input
- Segmented Control
- Toggle Tip
- Custom Select
- And a lot more
Check out all [components here](https://www.chakra-ui.com/docs/components/concepts/overview). Each new component is built with the same focus on accessibility and design consistency that Chakra UI is known for.
## [Introduction of State Machines]()
One of the biggest updates in this version is the use of state machines for logic-based components. Chakra UI v3 now uses Ark UI as the foundation for these components.
State machines bring a new level of stability to Chakra UI components, especially for applications that require complex and interactive user interfaces.
While this isn’t something you’ll interact with directly as a user, it’s important to know that using state machines under the hood significantly improves the performance of components like modals, menus, popovers, and other complex UI elements.
Now, you can expect more consistent behaviour across Chakra UI components.
## [Streamlined Component Imports]()
In previous versions, you had to import multiple components and component parts into your project.
For example, you had to manually import both `List` and `ListItem` separately
**version 2:**
```
import { List, ListItem } from "@chakra-ui/react"
function App() {
return (
<List>
<ListItem>Item 1</ListItem>
</List>
)
}
```
Version 3 offers a more cohesive API that keeps related components together. We introduced a more organized approach to component imports, reducing the need for multiple imports and making your codebase easier to maintain.
**version 3:**
```
import { List } from "@chakra-ui/react"
function App() {
return (
<List.Root>
<List.Item>Item 1</List.Item>
</List.Root>
)
}
```
Now, you only need to import `List` and use the object notation to compose the other components.
This pattern of nesting primary components under a main “Root” container and related subcomponents streamlines Chakra’s API and keeps your imports cleaner.
## [Enhanced Theme Setup]()
We’ve reimagined the theme setup for better modularity. In previous versions, themes were typically extended using the `extendTheme` function:
**version 2:**
```
const theme = extendTheme({
colors: {
brand: {
100: "#f7fafc",
900: "#1a202c",
},
},
})
```
In **v3**, the new approach leverages the `createSystem` function, where tokens are defined under the `theme` key in your system config:
**version 3:**
```
import { createSystem } from "@chakra-ui/react"
export const system = createSystem(defaultConfig, {
theme: {
tokens: {
fonts: {
heading: { value: `'Figtree', sans-serif` },
body: { value: `'Figtree', sans-serif` },
},
},
},
})
```
This new setup makes it easier to customize and scale your design system.
## [Enhanced Design Tokens]()
In version 3, we’ve also updated our design tokens. You’ll notice changes in:
- **Colors**: The [color palette](https://www.chakra-ui.com/docs/theming/colors) has been expanded and fine-tuned for vibrancy and versatility. This ensures that your designs are more visually appealing and accessible.
- **Animations:** We’ve added more support for [animations](https://www.chakra-ui.com/docs/theming/animations) using vanilla css, making your app more perfomant.
- **Design Tokens**: Improved and more robust [tokens](https://www.chakra-ui.com/docs/theming/tokens) for better design consistency.
- **Introduction of Semantic Tokens:** We added built-in [semantic tokens](https://www.chakra-ui.com/docs/theming/semantic-tokens) to make it easier to map your design system to both light and dark color modes.
## [Updated Props]()
Props have also received a [major update](https://www.chakra-ui.com/docs/get-started/migration) in this version. A great example is the `gap` prop, which now replaces the `spacing` prop for `Stack` and similar components.
**version 2:**
```
<Stack spacing={4}>
<Box>Item 1</Box>
<Box>Item 2</Box>
</Stack>
```
**version 3:**
```
<Stack gap={4}>
<Box>Item 1</Box>
<Box>Item 2</Box>
</Stack>
```
This change is more in line with modern CSS practices and helps developers manage spacing more efficiently, especially in flex and grid layouts.
Not only that, in v2, **boolean props** were typically prefixed with `is`, such as `isDisabled`. In v3, this has been simplified by removing the `is` prefix.
**version 2:**
```
<Button isDisabled>Click Me</Button>
```
**version 3:**
```
<Button disabled>Click Me</Button>
```
This change makes prop names more intuitive and easier to read, aligning with standard HTML practices.
## [We Said Goodbye to `chakra-icons`]()
In a move towards simplicity, v3 has removed the `chakra-icons` package. Instead, we encourage developers to use libraries like `lucide-react` or `react-icons` for their icon needs.
This shift reduces dependency bloat and allows you to tap into a broader range of icon options available in the React ecosystem.
## [Go Faster With Chakra v3]()
If you’re currently using Chakra UI v2, now is a great time to consider [migrating to version 3](https://www.chakra-ui.com/docs/get-started/migration).
The new features, such as component snippets, enhanced design tokens, and state machine-powered components, are designed to streamline your development process and improve your overall developer experience.
Try out Chakra v3 and let us know what you think. |
https://www.chakra-ui.com/blog/02-chakra-3.2 | Build faster with Premium Chakra UI Components 💎
[Learn more](https://pro.chakra-ui.com?utm_source=chakra-ui.com)
[](https://www.chakra-ui.com/)
[Docs](https://www.chakra-ui.com/docs/get-started/installation)[Playground](https://www.chakra-ui.com/playground)[Guides](https://www.chakra-ui.com/guides)[Blog](https://www.chakra-ui.com/blog)
[](https://github.com/chakra-ui/chakra-ui)
release·
November 21, 2024
## Chakra 3.2
SA
Segun Adebayo
@thesegunadebayo
We're excited to announce the release of Chakra UI v3.2.0! This release brings several exciting new features and improvements to enhance your development experience.
## [ColorPicker (Preview)]()
The new `ColorPicker` component allows users to select colors in various formats including HSL, RGB, and HSB. This component is perfect for applications that need sophisticated color selection capabilities.
Color
```
"use client"
import { HStack, parseColor } from "@chakra-ui/react"
import {
ColorPickerArea,
ColorPickerContent,
ColorPickerControl,
ColorPickerEyeDropper,
ColorPickerInput,
ColorPickerLabel,
ColorPickerRoot,
ColorPickerSliders,
ColorPickerTrigger,
} from "@/components/ui/color-picker"
const Demo = () => {
return (
<ColorPickerRoot defaultValue={parseColor("#eb5e41")} maxW="200px">
<ColorPickerLabel>Color</ColorPickerLabel>
<ColorPickerControl>
<ColorPickerInput />
<ColorPickerTrigger />
</ColorPickerControl>
<ColorPickerContent>
<ColorPickerArea />
<HStack>
<ColorPickerEyeDropper />
<ColorPickerSliders />
</HStack>
</ColorPickerContent>
</ColorPickerRoot>
)
}
```
## [ColorSwatch]()
Alongside the ColorPicker, we've added the `ColorSwatch` component for previewing colors. This simple yet powerful component makes it easy to display color samples in your UI.
```
import { HStack } from "@chakra-ui/react"
import { ColorSwatch } from "@chakra-ui/react"
import { For } from "@chakra-ui/react"
const Demo = () => {
return (
<HStack>
<For each={["2xs", "xs", "sm", "md", "lg", "xl", "2xl"]}>
{(size) => <ColorSwatch key={size} value="#bada55" size={size} />}
</For>
</HStack>
)
}
```
## [New Examples]()
### [Input Masking]()
We showcase how to compose the `use-mask-input` library with the `Input` component to mask input values. This is particularly useful for formatting phone numbers and credit card numbers.
```
"use client"
import { Input } from "@chakra-ui/react"
import { withMask } from "use-mask-input"
const Demo = () => {
return (
<Input placeholder="(99) 99999-9999" ref={withMask("(99) 99999-9999")} />
)
}
```
## [CLI Improvements]()
We've enhanced our CLI with more flexible snippet installation options:
### [New --all Flag]()
By default, the CLI installs a curated set of recommended snippets including Provider, Avatar, Button, Checkbox, Radio, Input Group, Slider, Popover, Dialog, Drawer, Tooltip, and Field.
You can now use the `--all` flag to install all available snippets instead of just the recommended ones.
## [Bug Fixes]()
This release also includes important bug fixes:
- Fixed an issue where `mergeConfigs` was mutating the underlying configs
- Resolved a problem where typegen wasn't working when the CLI was installed globally or run using `npx`
## [Upgrading]()
To upgrade to the latest version, run:
```
npm install @chakra-ui/react@latest
``` |