description
stringlengths 38
154k
| category
stringclasses 5
values | solutions
stringlengths 13
289k
| name
stringlengths 3
179
| id
stringlengths 24
24
| tags
sequencelengths 0
13
| url
stringlengths 54
54
| rank_name
stringclasses 8
values |
---|---|---|---|---|---|---|---|
A [perfect power](https://en.wikipedia.org/wiki/Perfect_power) is a classification of positive integers:
> In mathematics, a **perfect power** is a positive integer that can be expressed as an integer power of another positive integer. More formally, n is a perfect power if there exist natural numbers m > 1, and k > 1 such that m<sup>k</sup> = n.
Your task is to check wheter a given integer is a perfect power. If it is a perfect power, return a pair `m` and `k` with m<sup>k</sup> = n as a proof. Otherwise return `Nothing`, `Nil`, `null`, `NULL`, `None` or your language's equivalent.
**Note:** For a perfect power, there might be several pairs. For example `81 = 3^4 = 9^2`, so `(3,4)` and `(9,2)` are valid solutions. However, the tests take care of this, so if a number is a perfect power, return any pair that proves it.
### Examples
```javascript
Test.describe("perfect powers", function(){
Test.it("should work for some examples",function(){
Test.assertSimilar(isPP(4), [2,2], "4 = 2^2");
Test.assertSimilar(isPP(9), [3,2], "9 = 3^2");
Test.assertEquals( isPP(5), null, "5 isn't a perfect number");
});
});
```
```coffeescript
Test.describe "perfect powers", ->
Test.it "should work for some examples", ->
Test.assertSimilar isPP(4), [ 2, 2 ], "4 = 2^2"
Test.assertSimilar isPP(9), [ 3, 2 ], "9 = 3^2"
Test.assertEquals isPP(5), null, "5 isn't a perfect number"
```
```haskell
isPP 4 `shouldBe` Just (2,2)
isPP 9 `shouldBe` Just (3,2)
isPP 5 `shouldBe` Nothing
```
```python
isPP(4) => [2,2]
isPP(9) => [3,2]
isPP(5) => None
```
```ruby
isPP(4) => [2,2]
isPP(9) => [3,2]
isPP(5) => nil
```
```java
isPerfectPower(4) => new int[]{2,2}
isPerfectPower(5) => null
isPerfectPower(8) => new int[]{2,3}
isPerfectPower(9) => new int[]{3,2}
```
```r
isPP(4) => 2 2
isPP(9) => 3 2
isPP(5) => NULL
```
```csharp
IsPerfectPower(4) => (2,2)
IsPerfectPower(5) => null
IsPerfectPower(8) => (2,3)
IsPerfectPower(9) => (3,2)
```
| reference | def isPP(n):
for i in range(2, n + 1):
for j in range(2, n + 1):
if i * * j > n:
break
elif i * * j == n:
return [i, j]
return None
| What's a Perfect Power anyway? | 54d4c8b08776e4ad92000835 | [
"Mathematics",
"Fundamentals"
] | https://www.codewars.com/kata/54d4c8b08776e4ad92000835 | 5 kyu |
The input is a string `str` of digits. Cut the string into chunks (a chunk here is a substring of the initial string) of size `sz` (ignore the last chunk if its size is less than `sz`).
If the sum of a chunk's digits is divisible by `2`, reverse that chunk;
otherwise rotate it to the left by one position.
Put together these modified chunks and return the result as a string.
If
- `sz` is `<= 0` or if `str == ""` return ""
- `sz` is greater `(>)` than the length of `str` it is impossible to take a chunk of size `sz` hence return "".
#### Examples:
```
("123456987654", 6) --> "234561876549"
("123456987653", 6) --> "234561356789"
("66443875", 4) --> "44668753"
("66443875", 8) --> "64438756"
("664438769", 8) --> "67834466"
("123456779", 8) --> "23456771"
("", 8) --> ""
("123456779", 0) --> ""
("563000655734469485", 4) --> "0365065073456944"
```
```
Example of a string rotated to the left by one position:
s = "123456" gives "234561".
``` | reference | def func(s):
result = sum(int(x) * * 3 for x in s)
if result % 2 == 0:
return s[:: - 1]
else:
return s[1:] + s[0]
def revrot(s, sz):
if not sz:
return ''
return '' . join(func('' . join(x)) for x in zip(* [iter(list(s))] * sz))
| Reverse or rotate? | 56b5afb4ed1f6d5fb0000991 | [
"Algorithms",
"Strings"
] | https://www.codewars.com/kata/56b5afb4ed1f6d5fb0000991 | 6 kyu |
Write a function that accepts a string, and returns the same string with all even indexed characters in each word upper cased, and all odd indexed characters in each word lower cased. The indexing just explained is zero based, so the zero-ith index is even, therefore that character should be upper cased and you need to start over for each word.
The passed in string will only consist of alphabetical characters and spaces(`' '`). Spaces will only be present if there are multiple words. Words will be separated by a single space(`' '`).
### Examples:
```
"String" => "StRiNg"
"Weird string case" => "WeIrD StRiNg CaSe"
``` | algorithms | def to_weird_case_word(string):
return "" . join(c . upper() if i % 2 == 0 else c for i, c in enumerate(string . lower()))
def to_weird_case(string):
return " " . join(to_weird_case_word(str) for str in string . split())
| WeIrD StRiNg CaSe | 52b757663a95b11b3d00062d | [
"Strings",
"Algorithms"
] | https://www.codewars.com/kata/52b757663a95b11b3d00062d | 6 kyu |
In this kata, you should calculate the type of triangle with three given sides ``a``, ``b`` and ``c`` (given in any order).
If each angle is less than ``90°``, this triangle is ``acute`` and the function should return ``1``.
If one angle is strictly ``90°``, this triangle is ``right`` and the function should return ``2``.
If one angle is more than ``90°``, this triangle is ``obtuse`` and the function should return ``3``.
If three sides cannot form a triangle, or one angle is ``180°`` (which turns the triangle into a segment) - the function should return ``0``.
Three input parameters are ``sides`` of given triangle. All input values are non-negative floating point or integer numbers (or both, depending on the language).
<table style="background: white; color: black;">
<tr>
<td>
<img src='http://upload.wikimedia.org/wikipedia/commons/thumb/e/ed/Triangle.Acute.svg/181px-Triangle.Acute.svg.png'><center>Acute
</td>
<td>
<img src='http://upload.wikimedia.org/wikipedia/commons/thumb/7/72/Triangle.Right.svg/150px-Triangle.Right.svg.png'><center>Right
</td>
<td>
<img src='http://upload.wikimedia.org/wikipedia/commons/thumb/0/05/Triangle.Obtuse.svg/113px-Triangle.Obtuse.svg.png'><center>Obtuse
</td>
</tr>
</table>
### Examples:
```
(2, 4, 6) ---> return 0 (Not triangle)
(8, 5, 7) ---> return 1 (Acute, angles are approx. 82°, 38° and 60°)
(3, 4, 5) ---> return 2 (Right, angles are approx. 37°, 53° and exactly 90°)
(7, 12, 8) ---> return 3 (Obtuse, angles are approx. 34°, 106° and 40°)
```
If you stuck, this can help you: http://en.wikipedia.org/wiki/Law_of_cosines. But you can solve this kata even without angle calculation.
There is a very small chance of random test to fail due to round-off error, in such case resubmit your solution.
| algorithms | def triangle_type(a, b, c):
x, y, z = sorted([a, b, c])
if z >= x + y:
return 0
if z * z == x * x + y * y:
return 2
return 1 if z * z < x * x + y * y else 3
| Triangle type | 53907ac3cd51b69f790006c5 | [
"Geometry",
"Mathematics",
"Algorithms"
] | https://www.codewars.com/kata/53907ac3cd51b69f790006c5 | 6 kyu |
There is a secret string which is unknown to you. Given a collection of random triplets from the string, recover the original string.
A triplet here is defined as a sequence of three letters such that each letter occurs somewhere before the next in the given string. "whi" is a triplet for the string "whatisup".
As a simplification, you may assume that no letter occurs more than once in the secret string.
You can assume nothing about the triplets given to you other than that they are valid triplets and that they contain sufficient information to deduce the original string. In particular, this means that the secret string will never contain letters that do not occur in one of the triplets given to you. | algorithms | def recoverSecret(triplets):
r = list(set([i for l in triplets for i in l]))
for l in triplets:
fix(r, l[1], l[2])
fix(r, l[0], l[1])
return '' . join(r)
def fix(l, a, b):
"""let l.index(a) < l.index(b)"""
if l . index(a) > l . index(b):
l . remove(a)
l . insert(l . index(b), a)
| Recover a secret string from random triplets | 53f40dff5f9d31b813000774 | [
"Algorithms"
] | https://www.codewars.com/kata/53f40dff5f9d31b813000774 | 4 kyu |
We want to create a function that will add numbers together when called in succession.
```javascript
add(1)(2); // == 3
```
```ruby
add(1).(2); # equals 3
```
```python
add(1)(2) # equals 3
```
We also want to be able to continue to add numbers to our chain.
```javascript
add(1)(2)(3); // == 6
add(1)(2)(3)(4); // == 10
add(1)(2)(3)(4)(5); // == 15
```
```ruby
add(1).(2).(3); # 6
add(1).(2).(3).(4); # 10
add(1).(2).(3).(4).(5); # 15
```
```python
add(1)(2)(3) # 6
add(1)(2)(3)(4); # 10
add(1)(2)(3)(4)(5) # 15
```
and so on.
A single call should be equal to the number passed in.
```javascript
add(1); // == 1
```
```ruby
add(1); # 1
```
```python
add(1) # 1
```
We should be able to store the returned values and reuse them.
```javascript
var addTwo = add(2);
addTwo; // == 2
addTwo + 5; // == 7
addTwo(3); // == 5
addTwo(3)(5); // == 10
```
```ruby
var addTwo = add(2);
addTwo; # 2
addTwo + 5; # 7
addTwo(3); # 5
addTwo(3).(5); # 10
```
```python
addTwo = add(2)
addTwo # 2
addTwo + 5 # 7
addTwo(3) # 5
addTwo(3)(5) # 10
```
We can assume any number being passed in will be valid whole number. | games | class add (int):
def __call__(self, n):
return add(self + n)
| A Chain adding function | 539a0e4d85e3425cb0000a88 | [
"Mathematics",
"Functional Programming",
"Puzzles"
] | https://www.codewars.com/kata/539a0e4d85e3425cb0000a88 | 5 kyu |
# Introduction
<pre style="white-space: pre-wrap;white-space: -moz-pre-wrap;white-space: -pre-wrap;white-space: -o-pre-wrap;word-wrap: break-word;">
Guess Who? is a two-player guessing game created by Ora and Theo Coster, also known as Theora Design, that was first manufactured by Milton Bradley in 1979. It was first brought to the UK by Jack Barr Sr in 1982 (Source <a href="https://en.wikipedia.org/wiki/Guess_Who%3F">Wikipedia</a>)
</pre>
<center><img src="https://raw.githubusercontent.com/adrianeyre/codewars/master/Ruby/Authored/guesswho_banner.jpg" alt="Connect 4"></center>
# Task
<pre style="white-space: pre-wrap;white-space: -moz-pre-wrap;white-space: -pre-wrap;white-space: -o-pre-wrap;word-wrap: break-word;">
Your task is to make a simple class called <font color="#A1A85E">GuessWho</font>. The computer will try and guess your character, your job is to return back to the computer a list of possible characters so that it can guess successfully. You will need at least one method in the class called <font color="#A1A85E">guess</font>, this is where the computer posts the guess.
</pre>
# Characters
<img src="https://raw.githubusercontent.com/adrianeyre/codewars/master/Ruby/Authored/guesswho.png">
# Rules
<pre style="white-space: pre-wrap;white-space: -moz-pre-wrap;white-space: -pre-wrap;white-space: -o-pre-wrap;word-wrap: break-word;">
1. The computer willl give you the character in the initialization of the class
2. The computer will post the guess to the method <font color="#A1A85E">guess</font>
3. The computers guess will either be a name of a <font color="#A1A85E">character</font> or one of these characteristics <font color="#A1A85E">["Male","Female","Glasses","Brown eyes","Bald","White hair","Small mouth","Mustache","Brown hair","Big mouth","Small nose","Blue eyes","Hat","Long hair","Black hair","Earrings","Blonde hair","Ginger hair","Beard","Big nose"]</font>
4. If the computer passes a characteristic that your character has then return all the characters that have that characteristic.
5. If the computer passes a characteristic that your character does not have then return all characters that do not have that characteristic.
6. Update your character list
7. Keep a tally of the amount of turns the computer has had.
8. All characters and characteristic are capitalized
</pre>
# Character Characteristics - pre-loaded into initial solution
```
characteristic = [["Jean-Claude",["Male","Glasses","Brown eyes","Bald","White hair","Small mouth","Small nose"]],
["Pierre",["Male","Mustache","Brown eyes","Brown hair","Big mouth","Small nose"]],
["Jean",["Male","White hair","Big nose","Big mouth","Blue eyes"]],
["Amelie",["Female","Hat","Brown hair","Small mouth","Long hair","Brown eyes","Small nose"]],
["Mirabelle",["Female","Black hair","Earrings","Small mouth","Brown eyes","Big nose"]],
["Isabelle",["Female","Blonde hair","Glasses","Hat","Small mouth","Small nose","Brown eyes"]],
["Antonin",["Male","Brown eyes","Black hair","Small nose","Big mouth"]],
["Bernard",["Male","Brown eyes","Brown hair","Small nose","Hat"]],
["Owen",["Male","Blue eyes","Blonde hair","Small nose","Small mouth"]],
["Dylan",["Male","Brown eyes","Blonde hair","Small nose","Small mouth","Bald","Beard"]],
["Herbert",["Male","Brown eyes","Blonde hair","Big nose","Small mouth","Bald"]],
["Christine",["Female","Blue eyes","Blonde hair","Small nose","Small mouth","Long hair"]],
["Luc",["Male","Brown eyes","White hair","Small nose","Small mouth","Glasses"]],
["Cecilian",["Male","Brown eyes","Ginger hair","Small nose","Small mouth"]],
["Lionel",["Male","Brown eyes","Brown hair","Big nose","Big mouth","Mustache"]],
["Benoit",["Male","Brown eyes","Brown hair","Small mouth","Small nose","Mustache","Beard"]],
["Robert",["Male","Blue eyes","Brown hair","Big nose","Big mouth"]],
["Charline",["Female","Brown hair","White hair","Small nose","Big mouth"]],
["Renaud",["Male","Brown eyes","Blonde hair","Small nose","Big mouth","Mustache"]],
["Michel",["Male","Brown eyes","Blonde hair","Small nose","Big mouth","Beard"]],
["Pierre-Louis",["Male","Blue eyes","Brown hair","Small nose","Small mouth","Bald","Glasses"]],
["Etienne",["Male","Brown eyes","Blonde hair","Small nose","Small mouth","Glasses"]],
["Henri",["Male","Brown eyes","White hair","Small nose","Big mouth","Hat"]],
["Damien",["Male","Brown eyes","Blonde hair","Small nose","Big mouth","Hat"]]]
```
# Returns
<pre style="white-space: pre-wrap;white-space: -moz-pre-wrap;white-space: -pre-wrap;white-space: -o-pre-wrap;word-wrap: break-word;">
Return <font color="#A1A85E">["Correct! in n turns"]</font>. Where n is the amount of turns the computer has taken, if the computer guesses the correct character.
Return <font color="#A1A85E">array of possible characters</font> if a computer doesn't guess the correct character or characteristic.
Return <font color="#A1A85E">array of possible characters</font> if a computer doesn't guess the correct characteristic.
</pre>
# Example
Game set up with the character `Amelie`
```ruby
game = GuessWho.new("Amelie")
```
```python
game = GuessWho("Amelie")
```
```javascript
game = new GuessWho("Amelie")
```
The computer guesses the characteristic `Female`
```
game.guess("Female")
```
`Amelia` is female so you should return all the characters that are female.
```
["Amelie", "Mirabelle", "Isabelle", "Christine", "Charline"]
```
Good luck and enjoy!
# Kata Series
If you enjoyed this, then please try one of my other Katas. Any feedback, translations and grading of beta Katas are greatly appreciated. Thank you.
<span style="display: flex !important;"><img style="margin:0px;" src="https://raw.githubusercontent.com/adrianeyre/codewars/master/Ruby/Authored/6KYU.png" alt="Rank"/> <a href="https://www.codewars.com/kata/58663693b359c4a6560001d6" target="_blank">Maze Runner</a></span>
<span style="display: flex !important;"><img style="margin:0px;" src="https://raw.githubusercontent.com/adrianeyre/codewars/master/Ruby/Authored/6KYU.png" alt="Rank"/> <a href="https://www.codewars.com/kata/58693bbfd7da144164000d05" target="_blank">Scooby Doo Puzzle</a></span>
<span style="display: flex !important;"><img style="margin:0px;" src="https://raw.githubusercontent.com/adrianeyre/codewars/master/Ruby/Authored/7KYU.png" alt="Rank"/> <a href="https://www.codewars.com/kata/586a1af1c66d18ad81000134" target="_blank">Driving License</a></span>
<span style="display: flex !important;"><img style="margin:0px;" src="https://raw.githubusercontent.com/adrianeyre/codewars/master/Ruby/Authored/6KYU.png" alt="Rank"/> <a href="https://www.codewars.com/kata/586c0909c1923fdb89002031" target="_blank">Connect 4</a></span>
<span style="display: flex !important;"><img style="margin:0px;" src="https://raw.githubusercontent.com/adrianeyre/codewars/master/Ruby/Authored/6KYU.png" alt="Rank"/> <a href="https://www.codewars.com/kata/586e6d4cb98de09e3800014f" target="_blank">Vending Machine</a></span>
<span style="display: flex !important;"><img style="margin:0px;" src="https://raw.githubusercontent.com/adrianeyre/codewars/master/Ruby/Authored/6KYU.png" alt="Rank"/> <a href="https://www.codewars.com/kata/587136ba2eefcb92a9000027" target="_blank">Snakes and Ladders</a></span>
<span style="display: flex !important;"><img style="margin:0px;" src="https://raw.githubusercontent.com/adrianeyre/codewars/master/Ruby/Authored/6KYU.png" alt="Rank"/> <a href="https://www.codewars.com/kata/58a848258a6909dd35000003" target="_blank">Mastermind</a></span>
<span style="display: flex !important;"><img style="margin:0px;" src="https://raw.githubusercontent.com/adrianeyre/codewars/master/Ruby/Authored/6KYU.png" alt="Rank"/> <a href="https://www.codewars.com/kata/58b2c5de4cf8b90723000051" target="_blank">Guess Who?</a></span>
<span style="display: flex !important;"><img style="margin:0px;" src="https://raw.githubusercontent.com/adrianeyre/codewars/master/Ruby/Authored/6KYU.png" alt="Rank"/> <a href="https://www.codewars.com/kata/58f5c63f1e26ecda7e000029" target="_blank">Am I safe to drive?</a></span>
<span style="display: flex !important;"><img style="margin:0px;" src="https://raw.githubusercontent.com/adrianeyre/codewars/master/Ruby/Authored/6KYU.png" alt="Rank"/> <a href="https://www.codewars.com/kata/58f5c63f1e26ecda7e000029" target="_blank">Mexican Wave</a></span>
<span style="display: flex !important;"><img style="margin:0px;" src="https://raw.githubusercontent.com/adrianeyre/codewars/master/Ruby/Authored/6KYU.png" alt="Rank"/> <a href="https://www.codewars.com/kata/58fdcc51b4f81a0b1e00003e" target="_blank">Pigs in a Pen</a></span>
<span style="display: flex !important;"><img style="margin:0px;" src="https://raw.githubusercontent.com/adrianeyre/codewars/master/Ruby/Authored/6KYU.png" alt="Rank"/> <a href="https://www.codewars.com/kata/590300eb378a9282ba000095" target="_blank">Hungry Hippos</a></span>
<span style="display: flex !important;"><img style="margin:0px;" src="https://raw.githubusercontent.com/adrianeyre/codewars/master/Ruby/Authored/6KYU.png" alt="Rank"/> <a href="https://www.codewars.com/kata/5904be220881cb68be00007d" target="_blank">Plenty of Fish in the Pond</a></span>
<span style="display: flex !important;"><img style="margin:0px;" src="https://raw.githubusercontent.com/adrianeyre/codewars/master/Ruby/Authored/6KYU.png" alt="Rank"/> <a href="https://www.codewars.com/kata/590adadea658017d90000039" target="_blank">Fruit Machine</a></span>
<span style="display: flex !important;"><img style="margin:0px;" src="https://raw.githubusercontent.com/adrianeyre/codewars/master/Ruby/Authored/6KYU.png" alt="Rank"/> <a href="https://www.codewars.com/kata/591eab1d192fe0435e000014" target="_blank">Car Park Escape</a></span> | reference | class GuessWho (object):
def __init__(self, character):
self . char = character
self . possibles = set(characters)
self . attempt = 0
def guess(self, guess):
self . attempt += 1
if guess == self . char:
return [f"Correct! in { self . attempt } turns"]
if guess in characters:
self . possibles -= {guess}
else:
self . possibles &= {char for char in characters if (
guess in characters[char]) == (guess in characters[self . char])}
return sorted(self . possibles)
characters = {
"Jean-Claude": ("Male", "Glasses", "Brown eyes", "Bald", "White hair", "Small mouth", "Small nose"),
"Pierre": ("Male", "Mustache", "Brown eyes", "Brown hair", "Big mouth", "Small nose"),
"Jean": ("Male", "White hair", "Big nose", "Big mouth", "Blue eyes"),
"Amelie": ("Female", "Hat", "Brown hair", "Small mouth", "Long hair", "Brown eyes", "Small nose"),
"Mirabelle": ("Female", "Black hair", "Earrings", "Small mouth", "Brown eyes", "Big nose"),
"Isabelle": ("Female", "Blonde hair", "Glasses", "Hat", "Small mouth", "Small nose", "Brown eyes"),
"Antonin": ("Male", "Brown eyes", "Black hair", "Small nose", "Big mouth"),
"Bernard": ("Male", "Brown eyes", "Brown hair", "Small nose", "Hat"),
"Owen": ("Male", "Blue eyes", "Blonde hair", "Small nose", "Small mouth"),
"Dylan": ("Male", "Brown eyes", "Blonde hair", "Small nose", "Small mouth", "Bald", "Beard"),
"Herbert": ("Male", "Brown eyes", "Blonde hair", "Big nose", "Small mouth", "Bald"),
"Christine": ("Female", "Blue eyes", "Blonde hair", "Small nose", "Small mouth", "Long hair"),
"Luc": ("Male", "Brown eyes", "White hair", "Small nose", "Small mouth", "Glasses"),
"Cecilian": ("Male", "Brown eyes", "Ginger hair", "Small nose", "Small mouth"),
"Lionel": ("Male", "Brown eyes", "Brown hair", "Big nose", "Big mouth", "Mustache"),
"Benoit": ("Male", "Brown eyes", "Brown hair", "Small mouth", "Small nose", "Mustache", "Beard"),
"Robert": ("Male", "Blue eyes", "Brown hair", "Big nose", "Big mouth"),
"Charline": ("Female", "Brown hair", "White hair", "Small nose", "Big mouth"),
"Renaud": ("Male", "Brown eyes", "Blonde hair", "Small nose", "Big mouth", "Mustache"),
"Michel": ("Male", "Brown eyes", "Blonde hair", "Small nose", "Big mouth", "Beard"),
"Pierre-Louis": ("Male", "Blue eyes", "Brown hair", "Small nose", "Small mouth", "Bald", "Glasses"),
"Etienne": ("Male", "Brown eyes", "Blonde hair", "Small nose", "Small mouth", "Glasses"),
"Henri": ("Male", "Brown eyes", "White hair", "Small nose", "Big mouth", "Hat"),
"Damien": ("Male", "Brown eyes", "Blonde hair", "Small nose", "Big mouth", "Hat"),
}
| Guess Who? | 58b2c5de4cf8b90723000051 | [
"Games",
"Fundamentals"
] | https://www.codewars.com/kata/58b2c5de4cf8b90723000051 | 6 kyu |
A squared string is a string of `n` lines, each substring being `n` characters long.
We are given two n-squared strings.
For example:
`s1 = "abcd\nefgh\nijkl\nmnop"`
`s2 = "qrst\nuvwx\nyz12\n3456"`
Let us build a new string `strng` of size `(n + 1) x n` in the following way:
- The first line of `strng` has the first char of the first line of s1 plus the chars of the last line of s2.
- The second line of `strng` has the first two chars of the second line of s1 plus the chars of the penultimate
line of s2 except the last char
- and so on until the nth line of `strng` has the n chars of the nth line
of s1 plus the first char of the first line of s2.
Calling this function `compose(s1, s2)` we have:
```
compose(s1, s2) -> "a3456\nefyz1\nijkuv\nmnopq"
or printed:
abcd qrst --> a3456
efgh uvwx efyz1
ijkl yz12 ijkuv
mnop 3456 mnopq
``` | reference | def compose(s1, s2):
s1 = s1 . split("\n")
s2 = s2 . split("\n")[:: - 1]
n = len(s1)
out = []
for i in range(n):
out . append(s1[i][: i + 1] + s2[i][:(n - i)])
return "\n" . join(out)
| Composing squared strings | 56f253dd75e340ff670002ac | [
"Fundamentals"
] | https://www.codewars.com/kata/56f253dd75e340ff670002ac | 7 kyu |
A number system with moduli is defined by a vector of k moduli, `[m1,m2, ···,mk]`.
The moduli must be `pairwise co-prime`, which means that, for any pair of moduli, the only common factor is `1`.
In such a system each number `n` is represented by a string `"-x1--x2-- ... --xk-"` of its residues, one for each modulus.
The product `m1 * ... * mk` must be greater than the given number `n` which is to be converted in the moduli number system.
For example, if we use the system `[2, 3, 5]` the number `n = 11` is represented by `"-1--2--1-"`,
the number `n = 23` by `"-1--2--3-"`.
If we use the system `[8, 7, 5, 3]` the number `n = 187` becomes
`"-3--5--2--1-"`.
You will be given a number `n (n >= 0)` and a system `S = [m1,m2, ···,mk]` and you will return a string
`"-x1--x2-- ...--xk-"` representing the number `n` in the system `S`.
If the moduli are not `pairwise co-prime` or if the product `m1 * ... * mk` is not greater than `n`, return `"Not applicable"`.
#### Examples:
(you can add them in the "Sample tests")
```
fromNb2Str(11, [2,3,5]) -> "-1--2--1-"
fromNb2Str(6, [2, 3, 4]) -> "Not applicable", since 2 and 4 are not coprime
fromNb2Str(7, [2, 3]) -> "Not applicable" since 2 * 3 < 7
```
| reference | from math import gcd, prod
from itertools import combinations
def from_nb_2_str(n, modsys):
if prod(modsys) < n or next((True for a, b in combinations(modsys, 2) if gcd(a, b) != 1), False):
return "Not applicable"
return '-' + '--' . join(str(n % m) for m in modsys) + '-'
| Moduli number system | 54db15b003e88a6a480000b9 | [
"Fundamentals"
] | https://www.codewars.com/kata/54db15b003e88a6a480000b9 | 6 kyu |
In the drawing below we have a part of the Pascal's triangle, horizontal lines are numbered from **zero** (top).
We want to calculate the sum of the squares of the binomial coefficients on a given horizontal line with a function called `easyline` (or easyLine or easy-line).
Can you write a program which calculate `easyline(n)` where `n` is the horizontal line number?
The function will take n (with: `n>= 0`) as parameter and will return the sum
of the squares of the binomial coefficients on line n.
#### Examples:
```
easyline(0) => 1
easyline(1) => 2
easyline(4) => 70
easyline(50) => 100891344545564193334812497256
```
#### Ref:
http://mathworld.wolfram.com/BinomialCoefficient.html

#### Note:
In Javascript, Coffeescript, Typescript, C++, PHP, C, R, Nim, Fortran to get around the fact that we have no big integers the function `easyLine(n)` will in fact return
`round(log(easyline(n)))`
and not the `easyline(n)` of the other languages.
So, in Javascript, Coffeescript, Typescript, C++, PHP, R, Nim, C, Fortran:
```
easyLine(0) => 0
easyLine(1) => 1
easyLine(4) => 4
easyLine(50) => 67
``` | reference | def easyline(n):
return easyline(n - 1) * (4 * n - 2) / / n if n else 1
| Easy Line | 56e7d40129035aed6c000632 | [
"Fundamentals",
"Mathematics"
] | https://www.codewars.com/kata/56e7d40129035aed6c000632 | 7 kyu |
# RoboScript #2 - Implement the RS1 Specification
## Disclaimer
The story presented in this Kata Series is purely fictional; any resemblance to actual programming languages, products, organisations or people should be treated as purely coincidental.
## About this Kata Series
This Kata Series is based on a fictional story about a computer scientist and engineer who owns a firm that sells a toy robot called MyRobot which can interpret its own (esoteric) programming language called RoboScript. Naturally, this Kata Series deals with the software side of things (I'm afraid Codewars cannot test your ability to build a physical robot!).
## Story
Now that you've built your own code editor for RoboScript with appropriate syntax highlighting to make it look like serious code, it's time to properly implement RoboScript so that our MyRobots can execute any RoboScript provided and move according to the will of our customers. Since this is the first version of RoboScript, let's call our specification RS1 (like how the newest specification for JavaScript is called ES6 :p)
## Task
Write an interpreter for RS1 called `execute()` which accepts 1 required argument `code`, the RS1 program to be executed. The interpreter should return a string representation of the smallest 2D grid containing the full path that the MyRobot has walked on (explained in more detail later).
Initially, the robot starts at the middle of a 1x1 grid. Everywhere the robot walks it will leave a path `"*"`. If the robot has not been at a particular point on the grid then that point will be represented by a whitespace character `" "`. So if the RS1 program passed in to `execute()` is empty, then:
```
"" --> "*"
```
The robot understands 3 major commands:
- `F` - Move forward by 1 step in the direction that it is currently pointing. Initially, the robot faces to the right.
- `L` - Turn "left" (i.e. **rotate** 90 degrees **anticlockwise**)
- `R` - Turn "right" (i.e. **rotate** 90 degrees **clockwise**)
As the robot moves forward, if there is not enough space in the grid, the grid should expand accordingly. So:
```
"FFFFF" --> "******"
```
As you will notice, 5 `F` commands in a row should cause your interpreter to return a string containing 6 `"*"`s in a row. This is because initially, your robot is standing at the middle of the 1x1 grid facing right. It leaves a mark on the spot it is standing on, hence the first `"*"`. Upon the first command, the robot moves 1 unit to the right. Since the 1x1 grid is not large enough, your interpreter should expand the grid 1 unit to the right. The robot then leaves a mark on its newly arrived destination hence the second `"*"`. As this process is repeated 4 more times, the grid expands 4 more units to the right and the robot keeps leaving a mark on its newly arrived destination so by the time the entire program is executed, 6 "squares" have been marked `"*"` from left to right.
Each row in your grid must be separated from the next by a CRLF (`\r\n`). Let's look at another example:
```
"FFFFFLFFFFFLFFFFFLFFFFFL" --> "******\r\n* *\r\n* *\r\n* *\r\n* *\r\n******"
```
So the grid will look like this:
```
******
* *
* *
* *
* *
******
```
The robot moves 5 units to the right, then turns left, then moves 5 units upwards, then turns left again, then moves 5 units to the left, then turns left again and moves 5 units downwards, returning to the starting point before turning left one final time. Note that the marks do **not** disappear no matter how many times the robot steps on them, e.g. the starting point is still marked `"*"` despite the robot having stepped on it twice (initially and on the last step).
Another example:
```
"LFFFFFRFFFRFFFRFFFFFFF" --> " ****\r\n * *\r\n * *\r\n********\r\n * \r\n * "
```
So the grid will look like this:
```
****
* *
* *
********
*
*
```
Initially the robot turns left to face upwards, then moves upwards 5 squares, then turns right and moves 3 squares, then turns right again (to face downwards) and move 3 squares, then finally turns right again and moves 7 squares.
Since you've realised that it is probably quite inefficient to repeat certain commands over and over again by repeating the characters (especially the `F` command - what if you want to move forwards 20 steps?), you decide to allow a shorthand notation in the RS1 specification which allows your customers to postfix a non-negative integer onto a command to specify how many times an instruction is to be executed:
- `Fn` - Execute the `F` command `n` times (NOTE: `n` *may* be more than 1 digit long!)
- `Ln` - Execute `L` n times
- `Rn` - Execute `R` n times
So the example directly above can also be written as:
```
"LF5RF3RF3RF7"
```
These 5 example test cases have been included for you :)
## Kata in this Series
1. [RoboScript #1 - Implement Syntax Highlighting](https://www.codewars.com/kata/roboscript-number-1-implement-syntax-highlighting)
2. **RoboScript #2 - Implement the RS1 Specification**
3. [RoboScript #3 - Implement the RS2 Specification](https://www.codewars.com/kata/58738d518ec3b4bf95000192)
4. [RoboScript #4 - RS3 Patterns to the Rescue](https://www.codewars.com/kata/594b898169c1d644f900002e)
5. [RoboScript #5 - The Final Obstacle (Implement RSU)](https://www.codewars.com/kata/5a12755832b8b956a9000133) | algorithms | from collections import deque
import re
TOKENIZER = re . compile(r'(R+|F+|L+)(\d*)')
def execute(code):
pos, dirs = (0, 0), deque([(0, 1), (1, 0), (0, - 1), (- 1, 0)])
seens = {pos}
for act, n in TOKENIZER . findall(code):
s, r = act[0], int(n or '1') + len(act) - 1
if s == 'F':
for _ in range(r):
pos = tuple(z + dz for z, dz in zip(pos, dirs[0]))
seens . add(pos)
else:
dirs . rotate((r % 4) * (- 1) * * (s == 'R'))
miX, maX = min(x for x, y in seens), max(x for x, y in seens)
miY, maY = min(y for x, y in seens), max(y for x, y in seens)
return '\r\n' . join('' . join('*' if (x, y) in seens else ' ' for y in range(miY, maY + 1))
for x in range(miX, maX + 1))
| RoboScript #2 - Implement the RS1 Specification | 5870fa11aa0428da750000da | [
"Esoteric Languages",
"Algorithms"
] | https://www.codewars.com/kata/5870fa11aa0428da750000da | 5 kyu |
# RoboScript #1 - Implement Syntax Highlighting
## Disclaimer
The story presented in this Kata Series is purely fictional; any resemblance to actual programming languages, products, organisations or people should be treated as purely coincidental.
## About this Kata Series
This Kata Series is based on a fictional story about a computer scientist and engineer who owns a firm that sells a toy robot called MyRobot which can interpret its own (esoteric) programming language called RoboScript. Naturally, this Kata Series deals with the software side of things (I'm afraid Codewars cannot test your ability to build a physical robot!).
## Story
You are a computer scientist and engineer who has recently founded a firm which sells a toy product called MyRobot which can move by receiving a set of instructions by reading a file containing a script. Initially you have planned the robot to be able to interpret JavaScript files for its movement instructions but you later decided that it would make MyRobot too hard to operate for most customers out there who aren't even computer programmers in the first place. For this reason, you have decided to invent a new (esoteric) scripting language called RoboScript which has a much simpler syntax so non-computer programmers can easily learn how to write scripts in this language which would enable them to properly operate MyRobot. However, you are currently at the initial stage of inventing this new Esolang. The first step to popularize this (esoteric) scripting language is naturally to invent a new editor for it which provides syntax highlighting for this language so your customers feel like they are writing a proper program when they are writing scripts for MyRobot.
## Task
Your MyRobot-specific (esoteric) scripting language called RoboScript only ever contains the following characters: `F`, `L`, `R`, the digits `0-9` and brackets (`(` and `)`). Your goal is to write a function `highlight` which accepts 1 required argument `code` which is the RoboScript program passed in as a string and returns the script with syntax highlighting. The following commands/characters should have the following colors:
- `F` - Wrap this command around `<span style="color: pink">` and `</span>` tags so that it is highlighted pink in our editor
- `L` - Wrap this command around `<span style="color: red">` and `</span>` tags so that it is highlighted red in our editor
- `R` - Wrap this command around `<span style="color: green">` and `</span>` tags so that it is highlighted green in our editor
- Digits from `0` through `9` - Wrap these around `<span style="color: orange">` and `</span>` tags so that they are highlighted orange in our editor
- Round Brackets - Do not apply any syntax highlighting to these characters
For example:
```javascript
highlight("F3RF5LF7"); // => "<span style=\"color: pink\">F</span><span style=\"color: orange\">3</span><span style=\"color: green\">R</span><span style=\"color: pink\">F</span><span style=\"color: orange\">5</span><span style=\"color: red\">L</span><span style=\"color: pink\">F</span><span style=\"color: orange\">7</span>"
```
```coffeescript
highlight("F3RF5LF7"); # => "<span style=\"color: pink\">F</span><span style=\"color: orange\">3</span><span style=\"color: green\">R</span><span style=\"color: pink\">F</span><span style=\"color: orange\">5</span><span style=\"color: red\">L</span><span style=\"color: pink\">F</span><span style=\"color: orange\">7</span>"
```
```php
highlight("F3RF5LF7"); // => "<span style=\"color: pink\">F</span><span style=\"color: orange\">3</span><span style=\"color: green\">R</span><span style=\"color: pink\">F</span><span style=\"color: orange\">5</span><span style=\"color: red\">L</span><span style=\"color: pink\">F</span><span style=\"color: orange\">7</span>"
```
```ruby
highlight("F3RF5LF7") # => "<span style=\"color: pink\">F</span><span style=\"color: orange\">3</span><span style=\"color: green\">R</span><span style=\"color: pink\">F</span><span style=\"color: orange\">5</span><span style=\"color: red\">L</span><span style=\"color: pink\">F</span><span style=\"color: orange\">7</span>"
```
```java
RoboScript.highlight("F3RF5LF7"); // => "<span style=\"color: pink\">F</span><span style=\"color: orange\">3</span><span style=\"color: green\">R</span><span style=\"color: pink\">F</span><span style=\"color: orange\">5</span><span style=\"color: red\">L</span><span style=\"color: pink\">F</span><span style=\"color: orange\">7</span>"
```
```elixir
assert highlight("F3RF5LF7") == "<span style=\"color: pink\">F</span><span style=\"color: orange\">3</span><span style=\"color: green\">R</span><span style=\"color: pink\">F</span><span style=\"color: orange\">5</span><span style=\"color: red\">L</span><span style=\"color: pink\">F</span><span style=\"color: orange\">7</span>"
```
```haskell
highlight "F3RF5LF7" -- => "<span style=\"color: pink\">F</span><span style=\"color: orange\">3</span><span style=\"color: green\">R</span><span style=\"color: pink\">F</span><span style=\"color: orange\">5</span><span style=\"color: red\">L</span><span style=\"color: pink\">F</span><span style=\"color: orange\">7</span>"
```
And for multiple characters with the same color, simply wrap them with a **single** `<span>` tag of the correct color:
```javascript
highlight("FFFR345F2LL"); // => "<span style=\"color: pink\">FFF</span><span style=\"color: green\">R</span><span style=\"color: orange\">345</span><span style=\"color: pink\">F</span><span style=\"color: orange\">2</span><span style=\"color: red\">LL</span>"
```
```coffeescript
highlight("FFFR345F2LL"); # => "<span style=\"color: pink\">FFF</span><span style=\"color: green\">R</span><span style=\"color: orange\">345</span><span style=\"color: pink\">F</span><span style=\"color: orange\">2</span><span style=\"color: red\">LL</span>"
```
```php
highlight("FFFR345F2LL"); // => "<span style=\"color: pink\">FFF</span><span style=\"color: green\">R</span><span style=\"color: orange\">345</span><span style=\"color: pink\">F</span><span style=\"color: orange\">2</span><span style=\"color: red\">LL</span>"
```
```ruby
highlight("FFFR345F2LL") # => "<span style=\"color: pink\">FFF</span><span style=\"color: green\">R</span><span style=\"color: orange\">345</span><span style=\"color: pink\">F</span><span style=\"color: orange\">2</span><span style=\"color: red\">LL</span>"
```
```java
RoboScript.highlight("FFFR345F2LL"); // => "<span style=\"color: pink\">FFF</span><span style=\"color: green\">R</span><span style=\"color: orange\">345</span><span style=\"color: pink\">F</span><span style=\"color: orange\">2</span><span style=\"color: red\">LL</span>"
```
```elixir
assert highlight("FFFR345F2LL") == "<span style=\"color: pink\">FFF</span><span style=\"color: green\">R</span><span style=\"color: orange\">345</span><span style=\"color: pink\">F</span><span style=\"color: orange\">2</span><span style=\"color: red\">LL</span>"
```
```haskell
highlight "FFFR345F2LL" -- => "<span style=\"color: pink\">FFF</span><span style=\"color: green\">R</span><span style=\"color: orange\">345</span><span style=\"color: pink\">F</span><span style=\"color: orange\">2</span><span style=\"color: red\">LL</span>"
```
Note that the use of `<span>` tags must be **exactly** the same format as demonstrated above. Even if your solution produces the same visual result as the expected answers, if you miss a space betwen `"color:"` and `"green"`, for example, you will fail the tests.
## Kata in this Series
1. **RoboScript #1 - Implement Syntax Highlighting**
2. [RoboScript #2 - Implement the RS1 Specification](https://www.codewars.com/kata/5870fa11aa0428da750000da)
3. [RoboScript #3 - Implement the RS2 Specification](https://www.codewars.com/kata/58738d518ec3b4bf95000192)
4. [RoboScript #4 - RS3 Patterns to the Rescue](https://www.codewars.com/kata/594b898169c1d644f900002e)
5. [RoboScript #5 - The Final Obstacle (Implement RSU)](https://www.codewars.com/kata/5a12755832b8b956a9000133) | reference | import re
def highlight(code):
code = re . sub(r"(F+)", '<span style="color: pink">\g<1></span>', code)
code = re . sub(r"(L+)", '<span style="color: red">\g<1></span>', code)
code = re . sub(r"(R+)", '<span style="color: green">\g<1></span>', code)
code = re . sub(r"(\d+)", '<span style="color: orange">\g<1></span>', code)
return code
| RoboScript #1 - Implement Syntax Highlighting | 58708934a44cfccca60000c4 | [
"Fundamentals"
] | https://www.codewars.com/kata/58708934a44cfccca60000c4 | 6 kyu |
Given u<sub>0</sub> = 1, u<sub>1</sub> = 2 and the relation
6u<sub>n</sub>u<sub>n+1</sub>-5u<sub>n</sub>u<sub>n+2</sub>+u<sub>n+1</sub>u<sub>n+2</sub> = 0</code>
calculate u<sub>n</sub> for any integer n >= 0.
#### Examples:
Call `fcn` the function such as fcn(n) = u<sub>n</sub>.
`fcn(17) -> 131072; fcn(21) -> 2097152`
#### Remark:
You can take two points of view to do this kata:
- the first one purely algorithmic from the definition of u<sub>n</sub>
- the second one - not at all mandatory, but as a complement - is to get a bit your head around and find which sequence is hidden behind u<sub>n</sub>.
| algorithms | def fcn(n):
return 2 * * n
| A disguised sequence (I) | 563f0c54a22b9345bf000053 | [
"Algorithms",
"Mathematics"
] | https://www.codewars.com/kata/563f0c54a22b9345bf000053 | 6 kyu |
Agent 47, you have a new task!
Among citizens of the city X are hidden 2 dangerous criminal twins.
Your task is to identify them and eliminate!
Given an array of integers, your task is to find two same numbers and return one of them, for example in array ``[2, 3, 6, 34, 7, 8, 2]`` answer is ``2``.
```if-not:c
If there are no twins in the city - return `None` or the equivalent in the language that you are using.
```
```if:c
If you find two identical numbers, store one of them in the output parameter
and return ``true``. If there are no twins in the city, return ``false``.
``` | algorithms | def elimination(arr):
for x in arr:
if arr . count(x) == 2:
return x
| Find twins | 5834315e06f227a6ac000099 | [
"Algorithms"
] | https://www.codewars.com/kata/5834315e06f227a6ac000099 | 7 kyu |
<a href="http://imgur.com/h75nn5H"><img src="http://i.imgur.com/h75nn5H.png?1" title="source: imgur.com" /></a>
This kata was thaught to continue with the topic of ```Tracking Hits for Different Sum Values for Different Kinds of Dice``` see at:http://www.codewars.com/kata/56f852635d7c12fb610013d7
In that kata we show the different sum values obtained rolling ```3``` tetrahedral dice. There is a table that shows how values ```7``` and ```8``` are the most probable sum values to obtain, having the highest probability of all, ```0.1875```.
Looking the image, if we take dice from a row(up to 7 elements) we will have the same type dice (different colours)
The probability is obtained doing the quotient between the total different combinations and total of cases.
```
probability(tetraedral_dice, 3, sum) = different_combinations_for_sum / total cases = 12 /(4*4*4) = 0.1875
```
The function ```most_prob_sum()``` will receive two arguments:
- a string with the type dice, one the following ones:
```
'tetrahedral', 'cubic', 'octahedral', 'ninesides', 'tensides', 'dodecahedral', 'icosahedral'.
```
- a number ```n```, that is the amount of rolled dice.
The corresponding range for each die are :
```
'tetrahedral' [1, 4]
'cubic' [1, 6]
'octahedral' [1, 8]
'ninesides' [1, 9]
'tensides' [1, 10]
'dodecahedral' [1, 12]
'icosahedral' [1, 20]
```
Let's see the output of the function with the given example:
```python
most_prob_sum('tetrahedral', 3) == [[7, 8], 0.1875]
```
```javascript
mostProbSum('tetrahedral', 3) ---> [[7, 8], 0.1875]
```
We need a list with two terms, the first one: a sorted list with the most probable sum value(s) and the second one: the maximum probability value.
For this kata we will have more challenging tests than the previous one,so we will need an optimized version for the solution.
More example cases are given in the Example Test Cases box.
Do not round the results for the probability value.
Enjoy it! | reference | from itertools import product
from collections import Counter
def most_prob_sum(dice, n):
c = Counter(sum(p) for p in product(
* [[i for i in range(1, 1 + {'tet': 4, 'cub': 6, 'oct': 8, 'nin': 9, 'ten': 10, 'dod': 12, 'ico': 20}[dice[: 3]])] for _ in range(n)]))
mx = max(c . values())
return [[k for k in c if c[k] == mx], mx / float(sum(c . values()))]
| Find the Most Probable Sum Value or Values, in Rolling N-dice of n Sides | 56fb9da2fca8b9d7de00083f | [
"Algorithms",
"Mathematics",
"Statistics"
] | https://www.codewars.com/kata/56fb9da2fca8b9d7de00083f | 5 kyu |
<a href="http://imgur.com/zlXr2na"><img src="http://i.imgur.com/zlXr2na.jpg?1" title="source: imgur.com" /></a>
Perhaps it would be convenient to solve first ```Probabilities for Sums in Rolling Cubic Dice``` see at: http://www.codewars.com/kata/probabilities-for-sums-in-rolling-cubic-dice
Suppose that we roll dice that we never used, a tetrahedral die that has only 4 sides(values 1 to 4), or perhaps better the dodecahedral one with 12 sides and 12 values (from 1 to 12), or look! an icosahedral die with 20 sides and 20 values (from 1 to 20).
Let's roll dice of same type and try to see the distribution of the probability for all the possible sum values.
Suppose that we roll 3 tetrahedral dice:
```
Sum Prob Amount of hits Combinations
3 0.015625 1 {1,1,1}
4 0.046875 3 {1,2,1},{2,1,1},{1,1,2}
5 0.09375 6 {3,1,1},{1,1,3},{1,2,2},{1,3,1},{2,2,1},{2,1,2}
6 0.15625 10 {2,2,2},{1,3,2},{3,2,1},{1,4,1},{2,1,3},{1,1,4},{4,1,1},{3,1,2},{1,2,3},{2,3,1}
7 0.1875 12 {2,3,2},{1,2,4},{2,1,4},{1,4,2},{3,2,2}, {4,2,1},{3,3,1},{3,1,3},{2,2,3},{4,1,2},{2,4,1},{1,3,3}
8 0.1875 12 {2,3,3},{3,3,2},{2,2,4},{4,3,1},{2,4,2},{4,1,3},{4,2,2},{3,4,1},{1,3,4},{3,2,3},{3,1,4},{1,4,3}
9 0.15625 10 {3,3,3},{4,4,1},{2,4,3},{4,2,3},{4,3,2},{1,4,4},{4,1,4},{3,4,2},{2,3,4},{3,2,4}
10 0.09375 6 {3,4,3},{4,4,2},{3,3,4},{4,2,4},(2,4,4),{4,3,3}
11 0.046875 3 {4,3,4},{3,4,4},{4,4,3}
12 0.015625 1 {4,4,4}
tot: 1.0000
```
Note that the total sum of all the probabilities for each end has to be ```1.0000```
The register of hits per each sum value will be as follows:
```
[[3, 1], [4, 3], [5, 6], [6, 10], [7, 12], [8, 12], [9, 10], [10, 6], [11, 3], [12, 1]]
```
Create the function ```reg_sum_hits()``` that receive two arguments:
- number of dice, ```n```
- number of sides of the die, ```s```
The function will output a list of pairs with the sum value and the corresponding hits for it.
For the example given above:
```python
reg_sum_hits(3, 4) == [[3, 1], [4, 3], [5, 6], [6, 10], [7, 12], [8, 12], [9, 10], [10, 6], [11, 3], [12, 1]]
```
You will find more examples in the Example Test Cases.
Assumption: If the die has n sides, the values of it are from ```1``` to ```n``` | reference | def reg_sum_hits(dices, sides=6):
d, s = sides * [1], sides - 1
for i in range(dices - 1):
t = s * [0] + d + s * [0]
d = [sum(t[i: i + sides]) for i in range(len(t) - s)]
return [[i + dices, prob] for (i, prob) in enumerate(d)]
| Tracking Hits for Different Sum Values for Different Kinds of Dice | 56f852635d7c12fb610013d7 | [
"Mathematics",
"Statistics",
"Probability",
"Permutations",
"Data Science"
] | https://www.codewars.com/kata/56f852635d7c12fb610013d7 | 5 kyu |
<a href="http://imgur.com/E4b42Sm"><img src="http://i.imgur.com/E4b42Sm.jpg?1" title="source: imgur.com" /></a>
When we throw 2 classical dice (values on each side from 1 to 6) we have 36 (6 * 6) different results.
We want to know the probability of having the sum of the results equals to ```11```. For that result we have only the combination of ```6``` and ```5```. So we will have two events: {5, 6} and {6, 5}
So the probability for that result will be:
```
P(11, 2) = 2/(6*6) = 1/18 (The two is because we have 2 dice)
```
Now, we want to calculate the probability of having the sum equals to ```8```. The combinations for that result will be the following:
```{4,4}, {3,5}, {5,3}, {2,6}, {6,2}``` with a total of five combinations.
```
P(8, 2) = 5/36
```
Things may be more complicated if we have more dices and sum values higher.
We want to know the probability of having the sum equals to ```8``` but having ```3``` dice.
Now the combinations and corresponding events are:
```
{2,3,3}, {3,2,3}, {3,3,2}
{2,2,4}, {2,4,2}, {4,2,2}
{1,3,4}, {1,4,3}, {3,1,4}, {4,1,3}, {3,4,1}, {4,3,1}
{1,2,5}, {1,5,2}, {2,1,5}, {5,1,2}, {2,5,1}, {5,2,1}
{1,1,6}, {1,6,1}, {6,1,1}
A total amount of 21 different combinations
So the probability is:
P(8, 3) = 21/(6*6*6) = 0.09722222222222222
```
Summarizing the cases we have seen with a function that receives the two arguments
```python
rolldice_sum_prob(11, 2) == 0.0555555555 # or 1/18
rolldice_sum_prob(8, 2) == 0.13888888889# or 5/36
rolldice_sum_prob(8, 3) == 0.0972222222222 # or 7/72
```
And think why we have this result:
```python
rolldice_sum_prob(22, 3) == 0
```
Create the function ```rolldice_sum_prob()``` for this calculation.
Have a nice time!
(You do not have to round the results)
| reference | def rolldice_sum_prob(sum_, dice_amount):
import itertools
import collections
sums = [sum(list(i)) for i in itertools . product(
[1, 2, 3, 4, 5, 6], repeat=dice_amount)]
count = collections . Counter(sums)
prob = count[sum_] * 1.0 / (len(sums))
return prob
| Probabilities for Sums in Rolling Cubic Dice | 56f78a42f749ba513b00037f | [
"Mathematics",
"Statistics",
"Probability",
"Logic"
] | https://www.codewars.com/kata/56f78a42f749ba513b00037f | 5 kyu |
Check if given chord is minor or major.
### Rules
1. Basic minor/major chord have three elements.
2. A chord is *minor* when the interval between first and second element = 3 and between second and third = 4
3. A chord is *major* when the interval between first and second element = 4 and between second and third = 3
4. In a minor/major chord the interval between first and third element is always = 7
_______________________________________________________________
There is a preloaded list of the 12 notes of a chromatic scale built on C. This means that there are (almost) all allowed note' s names in music:
```python
['C', ['C#', 'Db'], 'D', ['D#', 'Eb'], 'E', 'F', ['F#', 'Gb'], 'G', ['G#', 'Ab'], 'A', ['A#', 'Bb'], 'B']
```
Note that e.g. 'C#' - 'C' = 1, 'C' - 'C#' = 1, 'Db' - 'C' = 1 and 'B' - 'C' = 1.
Input:
String of notes separated by whitespace, e. g. `'A C# E'`
Output:
String message: `'Minor'`, `'Major'` or `'Not a chord'` | reference | from itertools import product
NOTES = [['C'], ['C#', 'Db'], ['D'], ['D#', 'Eb'], ['E'], ['F'], [
'F#', 'Gb'], ['G'], ['G#', 'Ab'], ['A'], ['A#', 'Bb'], ['B']] * 2
config = [('Major', 4), ('Minor', 3)]
DCT_CHORDS = {c: mode for mode, offset in config
for i in range(len(NOTES) / / 2)
for c in product(NOTES[i], NOTES[i + offset], NOTES[i + 7])}
def minor_or_major(chord):
chord = tuple(chord . split()) if isinstance(chord, str) else ""
return DCT_CHORDS . get(chord, "Not a chord")
| #01 - Music theory - Minor/Major chords | 57052ac958b58fbede001616 | [
"Strings",
"Data Structures",
"Logic",
"Fundamentals"
] | https://www.codewars.com/kata/57052ac958b58fbede001616 | 6 kyu |
<img src="https://i.imgur.com/ta6gv1i.png?1" title="cw weekly challenge" />
Have you ever noticed that cows in a field are always facing in the same direction?
Reference: http://bfy.tw/7fgf
<hr>
Well.... not quite always.
One stubborn cow wants to be different from the rest of the herd - it's that damn *Wrong-Way Cow!*
# Task
Given a field of cows find which one is the Wrong-Way Cow and return her position.
Notes:
* There are always at least 3 cows in a herd
* There is only 1 Wrong-Way Cow!
* Fields are rectangular
* The cow position is zero-based [x,y] of her head (i.e. the letter c)
* There are no _diagonal_ cows -- they only face North/South/East/West (i.e. up/down/right/left)
# Examples
Ex1
<pre style="margin-bottom:20px">
cow.cow.cow.cow.cow
cow.cow.cow.cow.cow
cow.<span style="background:red">woc</span>.cow.cow.cow
cow.cow.cow.cow.cow
</pre>
*Answer: [6,2]*
Ex2
<pre style="margin-bottom:20px">
c..........
o...c......
w...o.c....
....w.o....
......w.<span style="background:red">cow</span>
</pre>
*Answer: [8,4]*
# Notes
*The test cases will NOT test any situations where there are "imaginary" cows, so your solution does not need to worry about such things!*
To explain - Yes, I recognise that there are certain configurations where an "imaginary" cow may appear that in fact is just made of three other "real" cows. In the following field you can see there are 4 real cows (3 are facing south and 1 is facing north). There are also 2 imaginary cows (facing east and west).
But such a field will never be tested by this Kata.
<pre style="margin-bottom:20px">
...w...
..cow..
.woco..
.ow.c..
.c.....
</pre>
| algorithms | SEARCH_COWES = {"cow", "woc"}
def find_wrong_way_cow(field):
for i, f in enumerate([field, list(zip(* field))]):
length, strField = len(f[0]), '\n' . join('' . join(line) for line in f)
for sCow in SEARCH_COWES:
pos = strField . find(sCow)
if pos != - 1 and pos == strField . rfind(sCow):
n = pos + 2 * (sCow == "woc")
ans = [n % (length + 1), n / / (length + 1)]
return ans[:: - 1] if i else ans
| The Wrong-Way Cow | 57d7536d950d8474f6000a06 | [
"Arrays",
"Algorithms"
] | https://www.codewars.com/kata/57d7536d950d8474f6000a06 | 5 kyu |
The Arara are an isolated tribe found in the Amazon who count in pairs. For example one to eight is as follows:
1 = anane </br>
2 = adak </br>
3 = adak anane </br>
4 = adak adak </br>
5 = adak adak anane </br>
6 = adak adak adak</br>
7 = adak adak adak anane</br>
8 = adak adak adak adak </br>
Take a given number and return the Arara's equivalent.
e.g.
```javascript
countArara(3) 'adak anane'
countArara(8) 'adak adak adak adak'
```
```python
count_arara(3) # -> 'adak anane'
count_arara(8) # -> 'adak adak adak adak'
```
```ruby
count_arara(3) # -> 'adak anane'
count_arara(8) # -> 'adak adak adak adak'
```
```
countArara 3 // -> "adak anane"
countArara 8 // -> "adak adak adak adak"
```
```swift
countArara(3) // "adak anane"
countArara(8) // "adak adak adak adak"
```
```csharp
Kata.CountArara(3) // "adak anane"
Kata.CountArara(8) // "adak adak adak adak"
```
```haskell
countArara(3) -- "adak anane"
countArara(8) -- "adak adak adak adak"
```
https://en.wikipedia.org/wiki/Arara_people
| games | def count_arara(n):
return " " . join(['adak'] * (n / / 2) + ['anane'] * (n % 2))
| Counting in the Amazon | 55b95c76e08bd5eef100001e | [
"Puzzles",
"Fundamentals"
] | https://www.codewars.com/kata/55b95c76e08bd5eef100001e | 7 kyu |
## Task
Implement a function which receives a list of values `lst` and a function `fn` as its arguments, and returns a new list where the `i`-th element is the result of left-reducing the first `i+1` elements of `lst` using `fn`.
Assuming `lst[:n]` syntax represents taking the first `n` elements of `lst`, the function which you should implement must produce the following result:
```
[
reduce(lst[:1], fn),
reduce(lst[:2], fn),
reduce(lst[:3], fn),
...,
reduce(lst, fn)
]
```
### Reduction
Reduction - the process of combining a list of values into a single value using a function. Assuming `lst[-1]` represents accessing the last element of a list, the left-reduction is equivalent to the following pseudo-code:
```
reduce(lst, fn) {
result = lst[0]
result = fn(result, lst[1])
result = fn(result, lst[2])
result = fn(result, lst[3])
...
result = fn(result, lst[-1])
return result
}
```
### Examples
```
running([1,1,1,1], add) = [1,2,3,4]
running([10,3,4,1], sub) = [10,7,3,2]
running([1,9,2,10], max) = [1,9,9,10]
running([1,9,2,10], min) = [1,1,1,1]
``` | reference | from itertools import accumulate
def running(lst, fn):
return list(accumulate(lst, fn))
| Running functions | 58b42c98f4cdd62f45000c6e | [
"Lists",
"Fundamentals"
] | https://www.codewars.com/kata/58b42c98f4cdd62f45000c6e | 7 kyu |
Data: an array of integers, a function f of two variables and an init value.
`Example: a = [2, 4, 6, 8, 10, 20], f(x, y) = x + y; init = 0`
Output: an array of integers, say r, such that
`r = [r[0] = f(init, a[0]), r[1] = f(r[0], a[1]), r[2] = f(r[1], a[2]), ...]`
With our example: `r = [2, 6, 12, 20, 30, 50]`
#### Task:
Write the following functions of two variables
- som : (x, y) -> x + y
- mini : (x, y) -> min(x, y)
- maxi : (x, y) -> max(x, y)
- lcmu : (x, y) -> lcm(abs(x), abs(y) (see note for lcm)
- gcdi : (x, y) -> gcd(abs(x), abs(y) (see note for gcd)
and
- function `oper_array(fct, arr, init)` (or operArray or oper-array) where
- `fct` is the function of two variables to apply to the array `arr`
(fct will be one of `som, mini, maxi, lcmu or gcdi`)
- `init` is the initial value
#### Examples:
```
a = [18, 69, -90, -78, 65, 40]
oper_array(gcd, a, a[0]) => [18, 3, 3, 3, 1, 1]
oper_array(lcm, a, a[0]) => [18, 414, 2070, 26910, 26910, 107640]
oper_array(sum, a, 0) => [18, 87, -3, -81, -16, 24]
oper_array(min, a, a[0]) => [18, 18, -90, -90, -90, -90]
oper_array(max, a, a[0]) => [18, 69, 69, 69, 69, 69]
```
#### Notes:
- The form of the parameter `fct` in oper_array (or operArray or oper-array)
changes according to the language. You can see each form according to the language in "Your test cases".
- AFAIK there are no corner cases, everything is as nice as possible.
- lcm and gcd see:
<https://en.wikipedia.org/wiki/Least_common_multiple>
<https://en.wikipedia.org/wiki/Greatest_common_divisor>
- you could google "reduce function (your language)" to have a general view about the reduce functions.
- In Shell bash, arrays are replaced by strings.
- In OCaml arrays are replaced by lists.
| reference | def gcdi(a, b):
from fractions import gcd
return abs(gcd(a, b))
def lcmu(a, b):
from fractions import gcd
return abs(a * b / / gcd(a, b))
def som(a, b):
return a + b
def maxi(a, b):
return max(a, b)
def mini(a, b):
return min(a, b)
def oper_array(fct, arr, init):
out = [init]
for i in range(len(arr)):
out . append(fct(out[- 1], arr[i]))
return out[1:]
| Reducing by steps | 56efab15740d301ab40002ee | [
"Mathematics",
"Arrays",
"Functional Programming",
"Lists",
"Data Structures"
] | https://www.codewars.com/kata/56efab15740d301ab40002ee | 6 kyu |
*There is a secret message in the first six sentences of this kata description. Have you ever felt like there was something more being said? Was it hard to figure out that unspoken meaning? Never again! Never will a secret go undiscovered. Find all duplicates from our message!*
Your job is to write a function that will find the secret words of the message and return them in order. The words in the secret message should be ordered in the order in which they are found as a duplicate, for example:
```javascript
'This is a test. this test is fun.' // --> 'this test is'
```
```ruby
'This is a test. this test is fun.' // --> 'this test is'
```
```python
'This is a test. this test is fun.' # --> 'this test is'
```
## Notes
The input will always be a string.
If the random tests repeat a word multiple times, it should show up in the secret message only once, based on the position of the first time it was duplicated.
The punctuation and casing of words (uppercase, lowercase) should not matter for the purpose of this kata. We are only concerned with word duplication. | algorithms | def find_secret_message(paragraph):
s = set()
ret = []
for w in (word . strip('.,:!?'). lower() for word in paragraph . split()):
if w in s and not w in ret:
ret . append(w)
else:
s . add(w)
return ' ' . join(ret)
| Secret Message | 54808e45ab03a2c8330009fb | [
"Algorithms"
] | https://www.codewars.com/kata/54808e45ab03a2c8330009fb | 6 kyu |
## Objective
Given a number `n` we will define its scORe to be `0 | 1 | 2 | 3 | ... | n`, where `|` is the [bitwise OR operator](https://en.wikipedia.org/wiki/Bitwise_operation#OR).
Write a function that takes `n` and finds it's scORe.
---------------------
| n | scORe n |
|---------|-------- |
| 0 | 0 |
| 1 | 1 |
| 49 | 63 |
| 1000000 | 1048575 |
Any feedback would be much appreciated
| algorithms | def score(n): return 2 * * n . bit_length() - 1
| Binary scORe | 56cafdabc8cfcc3ad4000a2b | [
"Binary",
"Algorithms"
] | https://www.codewars.com/kata/56cafdabc8cfcc3ad4000a2b | 7 kyu |
In this kata you need to write a function that will receive two strings (```n1``` and ```n2```), each representing an integer as a binary number. A third parameter will be provided (```o```) as a string representing one of the following operators: add, subtract, multiply.
Your task is to write the calculate function so that it will perform the arithmetic and the result returned should be a string representing the binary result.
Examples:
```
1 + 1 === 10
10 + 10 === 100
```
Negative binary numbers are usually preceded by several 1's. For this kata, negative numbers can be represented with the negative symbol at the beginning of the string.
Examples of negatives:
```
1 - 10 === -1
10 - 100 === -10
``` | algorithms | def calculate(n1, n2, o):
operators = {
"add": (lambda x, y: x + y),
"subtract": (lambda x, y: x - y),
"multiply": (lambda x, y: x * y),
}
return "{:b}" . format(operators[o](int(n1, 2), int(n2, 2)))
| Binary Calculator | 546ba103f0cf8f7982000df4 | [
"Binary",
"Algorithms"
] | https://www.codewars.com/kata/546ba103f0cf8f7982000df4 | 7 kyu |
Define n!! as
n!! = 1 \* 3 \* 5 \* ... \* n if n is odd,
n!! = 2 \* 4 \* 6 \* ... \* n if n is even.
Hence 8!! = 2 \* 4 \* 6 \* 8 = 384, there is no zero at the end.
30!! has 3 zeros at the end.
For a positive integer n, please count how many zeros are there at
the end of n!!.
Example:
```
30!! = 2 * 4 * 6 * 8 * 10 * ... * 22 * 24 * 26 * 28 * 30
30!! = 42849873690624000 (3 zeros at the end)
```
| games | def count_zeros_n_double_fact(n):
if n % 2 != 0:
return 0
k = 0
while n >= 10:
k += n / / 10
n / /= 5
return k
| Jungerstein's Math Training Room: 1. How many zeros are at the end of n!! ? | 58cbfe2516341cce1e000001 | [
"Puzzles",
"Mathematics",
"Number Theory",
"Discrete Mathematics"
] | https://www.codewars.com/kata/58cbfe2516341cce1e000001 | 6 kyu |
No Story
No Description
Only by Thinking and Testing
Look at result of testcase, guess the code!
## Series:
* [01: A and B?](https://www.codewars.com/kata/56d904db9963e9cf5000037d)
* [02: Incomplete string](https://www.codewars.com/kata/56d9292cc11bcc3629000533)
* [03: True or False](https://www.codewars.com/kata/56d931ecc443d475d5000003)
* [04: Something capitalized](https://www.codewars.com/kata/56d93f249c844788bc000002)
* [05: Uniq or not Uniq](https://www.codewars.com/kata/56d949281b5fdc7666000004)
* [06: Spatiotemporal index](https://www.codewars.com/kata/56d98b555492513acf00077d)
* [07: Math of Primary School](https://www.codewars.com/kata/56d9b46113f38864b8000c5a)
* [08: Math of Middle school](https://www.codewars.com/kata/56d9c274c550b4a5c2000d92)
* [09: From nothingness To nothingness](https://www.codewars.com/kata/56d9cfd3f3928b4edd000021)
* [10: Not perfect? Throw away!](https://www.codewars.com/kata/56dae2913cb6f5d428000f77)
* [11: Welcome to take the bus](https://www.codewars.com/kata/56db19703cb6f5ec3e001393)
* [12: A happy day will come](https://www.codewars.com/kata/56dc41173e5dd65179001167)
* [13: Sum of 15(Hetu Luosliu)](https://www.codewars.com/kata/56dc5a773e5dd6dcf7001356)
* [14: Nebula or Vortex](https://www.codewars.com/kata/56dd3dd94c9055a413000b22)
* [15: Sport Star](https://www.codewars.com/kata/56dd927e4c9055f8470013a5)
* [16: Falsetto Rap Concert](https://www.codewars.com/kata/56de38c1c54a9248dd0006e4)
* [17: Wind whispers](https://www.codewars.com/kata/56de4d58301c1156170008ff)
* [18: Mobile phone simulator](https://www.codewars.com/kata/56de82fb9905a1c3e6000b52)
* [19: Join but not join](https://www.codewars.com/kata/56dfce76b832927775000027)
* [20: I hate big and small](https://www.codewars.com/kata/56dfd5dfd28ffd52c6000bb7)
* [21: I want to become diabetic ;-)](https://www.codewars.com/kata/56e0e065ef93568edb000731)
* [22: How many blocks?](https://www.codewars.com/kata/56e0f1dc09eb083b07000028)
* [23: Operator hidden in a string](https://www.codewars.com/kata/56e1161fef93568228000aad)
* [24: Substring Magic](https://www.codewars.com/kata/56e127d4ef93568228000be2)
* [25: Report about something](https://www.codewars.com/kata/56eccc08b9d9274c300019b9)
* [26: Retention and discard I](https://www.codewars.com/kata/56ee0448588cbb60740013b9)
* [27: Retention and discard II](https://www.codewars.com/kata/56eee006ff32e1b5b0000c32)
* [28: How many "word"?](https://www.codewars.com/kata/56eff1e64794404a720002d2)
* [29: Hail and Waterfall](https://www.codewars.com/kata/56f167455b913928a8000c49)
* [30: Love Forever](https://www.codewars.com/kata/56f214580cd8bc66a5001a0f)
* [31: Digital swimming pool](https://www.codewars.com/kata/56f25b17e40b7014170002bd)
* [32: Archery contest](https://www.codewars.com/kata/56f4202199b3861b880013e0)
* [33: The repair of parchment](https://www.codewars.com/kata/56f606236b88de2103000267)
* [34: Who are you?](https://www.codewars.com/kata/56f6b4369400f51c8e000d64)
* [35: Safe position](https://www.codewars.com/kata/56f7eb14f749ba513b0009c3)
## Special recommendation
Another series, innovative and interesting, medium difficulty. People who like to challenge, can try these kata:
* [Play Tetris : Shape anastomosis](https://www.codewars.com/kata/56c85eebfd8fc02551000281)
* [Play FlappyBird : Advance Bravely](https://www.codewars.com/kata/56cd5d09aa4ac772e3000323)
| games | def testit(a, b):
return a | b
| Thinking & Testing: A and B? | 56d904db9963e9cf5000037d | [
"Puzzles"
] | https://www.codewars.com/kata/56d904db9963e9cf5000037d | 7 kyu |
Let us define two sums `v(n, p)` and `u(n, p)`:
```math
v(n,p) = \sum_{k=0}^n (-1)^k \times p \times 4^{n-k} \times {2n - k \choose k}
```
```math
u(n,p) = \sum_{k=0}^n (-1)^k \times p \times 4^{n-k} \times {2n - k + 1 \choose k}
```
#### Task:
- 1) Calculate `v(n, p)` and `u(n, p)` with two brute-force functions `v1(n, p)` and `u1(n, p)`.
- 2) Try `v1(n, p)` and `u1(n, p)` for small values of `n` and `p` and guess the results of `u(n, p)` and `v(n, p)`
- 3) Using 2) write `v_eff(n, p)` and `u_eff(n, p)` (or vEff(n, p) and uEff(n, p) or v-eff(n, p) and u-eff(n, p)) to efficiently calculate `v` and `u` for bigger values of `n` and `p`.
-- (This third part is not tested in
JS, CS, TS, C++, C, PHP, Crystal, Rust, Swift, R, Nim, Fortran, NASM, Haxe, Pascal, Lua since there you don't have big integers to control your guess in part 2. See note below for "Shell").
#### Examples:
```
v1(12, 70) --> 1750
u1(13, 18) --> 252
```
#### Extra points:-)
For the mathy ones: find a relation between `v(n, p)`, `v(n-1, p)` and `u(n-1, p)` :-)
#### Notes
- Erlang, Prolog: only uEff(u_eff) and vEff(v_eff) are tested.
- Factor: only ueff and veff are tested.
- Forth: only ueff and veff are tested with small numbers.
- Shell: only `v1(n, p)`is tested (use the solution you find for `v_eff(n, p)`.
- If you have found `u_eff(n, p)` and `v_eff(n, p)` you can use them to calculate `u(n, p)` and `v(n, p)`.
- You could see: <https://en.wikipedia.org/wiki/Binomial_coefficient> for a refresh about `binomial coefficients`. | reference | '''
from math import factorial
def nCr(n, r):
return factorial(n) / factorial(n-r) / factorial(r)
def u1(n, p):
return sum((-1)**k * p * 4 ** (n-k) * nCr(2*n-k+1, k) for k in range(n + 1))
def v1(n, p):
return sum((-1)**k * p * 4 ** (n-k) * nCr(2*n-k, k) for k in range(n + 1))
'''
u1 = u_eff = lambda n, p: p * (n + 1)
v1 = v_eff = lambda n, p: p * (2 * n + 1)
# v(n) = u(2n)
| Disguised sequences (II) | 56fe17fcc25bf3e19a000292 | [
"Puzzles",
"Discrete Mathematics",
"Mathematics"
] | https://www.codewars.com/kata/56fe17fcc25bf3e19a000292 | 6 kyu |
Braking distance `d1` is the distance a vehicle will go from the point when it brakes to when it comes to a complete stop.
It depends on the original speed `v` and on the coefficient of friction `mu` between the tires and the road surface.
The braking distance is one of two principal components of the total stopping distance.
The other component is the reaction distance, which is the product of the speed and the perception-reaction time of the driver.
The kinetic energy E is `0.5*m*v**2`, the work W given by braking is `mu*m*g*d1`. Equalling E and W gives the braking distance:
`d1 = v*v / 2*mu*g` where `g` is the gravity of Earth and `m` the vehicle's mass.
We have `v` in km per hour, `g` as `9.81 m/s/s` and in the following we suppose that the reaction time
is constant and equal to `1 s`. The coefficient `mu` is dimensionless.
#### Tasks.
- The first one is to calculate the **total** stopping distance in meters given `v`, `mu`
(and the reaction time `t = 1`).
`dist(v, mu) -> d = total stopping distance`
- The second task is to calculate `v` in km per hour knowing `d` in meters and `mu`
with the supposition that the reaction time is still `t = 1`.
`speed(d, mu) -> v such that dist(v, mu) = d`.
#### Examples:
dist(100, 0.7) -> 83.9598760937531
speed(83.9598760937531, 0.7) -> 100.0
#### Notes:
- Remember to convert the velocity from km/h to m/s or from m/s in km/h when necessary.
- Don't forget the reaction time t: `t = 1`
- Don't truncate or round your results. See in "RUN SAMPLE TESTS" the function `assertFuzzyEquals` or `dotest or ...`.
- Shell: only `dist` is tested. | reference | def dist(v, mu): # suppose reaction time is 1
v /= 3.6
return v + v * v / (2 * mu * 9.81)
def speed(d, mu): # suppose reaction time is 1
b = - 2 * mu * 9.81
return round(3.6 * (b + (b * b - 4 * b * d) * * 0.5) / 2, 2)
| Braking well | 565c0fa6e3a7d39dee000125 | [
"Fundamentals",
"Mathematics"
] | https://www.codewars.com/kata/565c0fa6e3a7d39dee000125 | 6 kyu |
When working with color values it can sometimes be useful to extract the individual red, green, and blue (RGB) component values for a color. Implement a function that meets these requirements:
+ Accepts a case-insensitive hexadecimal color string as its parameter (ex. `"#FF9933"` or `"#ff9933"`)
+ Returns a Map<String, int> with the structure `{r: 255, g: 153, b: 51}` where *r*, *g*, and *b* range from 0 through 255
**Note:** your implementation does not need to support the shorthand form of hexadecimal notation (ie `"#FFF"`)
## Example
```
"#FF9933" --> {r: 255, g: 153, b: 51}
```
| algorithms | def hex_string_to_RGB(hex):
return {'r': int(hex[1: 3], 16), 'g': int(hex[3: 5], 16), 'b': int(hex[5: 7], 16)}
| Convert A Hex String To RGB | 5282b48bb70058e4c4000fa7 | [
"Parsing",
"Strings",
"Algorithms"
] | https://www.codewars.com/kata/5282b48bb70058e4c4000fa7 | 5 kyu |
**Description:**
We want to generate a function that computes the series starting from 0 and ending until the given number.
Example:
----
**Input:**
> 6
**Output:**
> 0+1+2+3+4+5+6 = 21
**Input:**
> -15
**Output:**
> -15<0
**Input:**
> 0
**Output:**
> 0=0
| reference | def show_sequence(n):
if n == 0:
return "0=0"
elif n < 0:
return str(n) + "<0"
else:
counter = sum(range(n + 1))
return '+' . join(map(str, range(n + 1))) + " = " + str(counter)
| Sum of numbers from 0 to N | 56e9e4f516bcaa8d4f001763 | [
"Fundamentals"
] | https://www.codewars.com/kata/56e9e4f516bcaa8d4f001763 | 7 kyu |
The maximum sum subarray problem consists in finding the maximum sum of a contiguous subsequence in an array or list of integers:
```haskell
maxSequence [-2, 1, -3, 4, -1, 2, 1, -5, 4]
-- should be 6: [4, -1, 2, 1]
```
```javascript
maxSequence([-2, 1, -3, 4, -1, 2, 1, -5, 4])
// should be 6: [4, -1, 2, 1]
```
```python
max_sequence([-2, 1, -3, 4, -1, 2, 1, -5, 4])
# should be 6: [4, -1, 2, 1]
```
```clojure
(max-sequence [-2, 1, -3, 4, -1, 2, 1, -5, 4])
;; should be 6: [4, -1, 2, 1]
```
```java
Max.sequence(new int[]{-2, 1, -3, 4, -1, 2, 1, -5, 4});
// should be 6: {4, -1, 2, 1}
```
```scala
Max.sequence(Array(-2, 1, -3, 4, -1, 2, 1, -5, 4));
// should be 6: Array(4, -1, 2, 1)
```
```kotlin
maxSequence(listOf(-2, 1, -3, 4, -1, 2, 1, -5, 4));
// should be 6: listOf(4, -1, 2, 1)
```
```c
maxSequence({-2, 1, -3, 4, -1, 2, 1, -5, 4}, 9)
// should return 6, from sub-array: {4, -1, 2, 1}
```
```cpp
maxSequence({-2, 1, -3, 4, -1, 2, 1, -5, 4});
//should be 6: {4, -1, 2, 1}
```
```rust
max_sequence(&[-2, 1, -3, 4, -1, 2, 1, -5, 4]);
//should be 6: [4, -1, 2, 1]
```
```cobol
maxSequence [-2, 1, -3, 4, -1, 2, 1, -5, 4]
* should be 6: [4, -1, 2, 1]
```
Easy case is when the list is made up of only positive numbers and the maximum sum is the sum of the whole array. If the list is made up of only negative numbers, return 0 instead.
Empty list is considered to have zero greatest sum. Note that the empty list or array is also a valid sublist/subarray.
| reference | def maxSequence(arr):
max, curr = 0, 0
for x in arr:
curr += x
if curr < 0:
curr = 0
if curr > max:
max = curr
return max
| Maximum subarray sum | 54521e9ec8e60bc4de000d6c | [
"Algorithms",
"Lists",
"Dynamic Programming",
"Fundamentals",
"Performance"
] | https://www.codewars.com/kata/54521e9ec8e60bc4de000d6c | 5 kyu |
*Don't be afraid, the description is rather long but - hopefully - it is in order that the process be well understood*.
You are given a string `s` made up of substring `s(1), s(2), ..., s(n)` separated by whitespaces.
Example:
`"after be arrived two My so"`
#### Task
Return a string `t` having the following property:
`length t(O) <= length t(1) >= length t(2) <= length t(3) >= length t(4) .... (P)`
where the `t(i)` are the substring of `s`;
you must respect the following rule:
at each step from left to right, you can only move either already consecutive strings
or strings that became consecutive after a previous move. The number of moves should be minimum.
#### Let us go with our example:
The length of "after" is greater than the length of "be". Let us move them ->`"be after arrived two My so"`
The length of "after" is smaller than the length of "arrived". Let us move them -> `"be arrived after two My so"`
The length of "after" is greater than the length of "two" ->`"be arrived two after My so"`
The length of "after" is greater than the length of "My". Good!
Finally the length of "My" and "so" are the same, nothing to do.
At the end of the process, the substrings s(i) verify:
`length s(0) <= length s(1) >= length s(2) <= length s(3) >= length (s4) <= length (s5)`
Hence given a string s of substrings `s(i)` the function `arrange` **with the previous process**
should return a *unique* string `t` having the property (P).
It is kind of roller coaster or up and down.
When you have property (P), to make the result more "up and down" visible t(0), t(2), ...
will be lower cases and the others upper cases.
```
arrange("after be arrived two My so") should return "be ARRIVED two AFTER my SO"
```
#### Notes:
- The string `"My after be arrived so two"` has the property (P) but can't be obtained by
the **described process** so it won't be accepted as a result. The property (P)
doesn't give unicity by itself.
- Process: go from left to right, move only consecutive strings when needed.
- For the first fixed tests the needed number of moves to get property (P)
is given as a comment so that you can know if your process follows the rule.
| reference | def arrange(strng):
words = strng . split()
for i in range(len(words)):
words[i: i + 2] = sorted(words[i: i + 2], key=len, reverse=i % 2)
words[i] = words[i]. upper() if i % 2 else words[i]. lower()
return ' ' . join(words)
| up AND down | 56cac350145912e68b0006f0 | [
"Fundamentals",
"Strings"
] | https://www.codewars.com/kata/56cac350145912e68b0006f0 | 6 kyu |
Congratulations! That Special Someone has given you their phone number.
But WAIT, is it a valid number?
Your task is to write a function that verifies whether a given string contains a valid British mobile (cell) phone number or not.
If valid, return 'In with a chance'.
If invalid, or if you're given an empty string, return 'Plenty more fish in the sea'.
A number can be valid in the following ways:
Here in the UK mobile numbers begin with '07' followed by 9 other digits, e.g. '07454876120'.
Sometimes the number is preceded by the country code, the prefix '+44', which replaces the '0' in ‘07’, e.g. '+447454876120'.
And sometimes you will find numbers with dashes in-between digits or on either side, e.g. '+44--745---487-6120' or '-074-54-87-61-20-'. As you can see, dashes may be consecutive.
Good Luck Romeo/Juliette!
| algorithms | import re
yes = "In with a chance"
no = "Plenty more fish in the sea"
def validate_number(string):
return yes if re . match(r'^(\+44|0)7[\d]{9}$', re . sub('-', '', string)) else no
| Is that a real phone number? (British version) | 581a52d305fe7756720002eb | [
"Regular Expressions",
"Strings",
"Algorithms"
] | https://www.codewars.com/kata/581a52d305fe7756720002eb | 7 kyu |
** SHORT INTRO **
"Tombola" is an Italian raffle/bingo-like game, mostly played during Christmas holidays; you have a sheet with 15 numbers and win increasing prizes while you complete it.
[Wikipedia link](https://en.wikipedia.org/wiki/Tombola_(raffle).
** SHEET SAMPLES **
<img src="http://i46.servimg.com/u/f46/13/76/83/45/cartel11.jpg" alt="Tombola sheet samples" style="width: 550px;"/>
** THE KATA **
In this kata you have to validate the correctness of a tombola sheet. More specifically:
- check if the sheet is made of 3 x 9 squares;
- check if there is a total of 15 unique numbers (> 0) over the squares (empty spaces will be represented with zeros);
- check if each of the 3 rows has 5 of the 15 numbers;
- check if each column has from 1 to 3 numbers in increasing order from top to bottom row;
- check if each column is divided in this way (inclusive, from first column to last):
1-9, 10-19, 20-29, 30-39, 40-49, 50-59, 60-69, 70-79, 80-90 (<- careful here! 90 is included in the last range!).
** TESTS **
To make the kata more challenging, I will not tell you why the input sheet is not valid. | reference | from itertools import chain
def checkCols(i, col, sortedCol): return (len(set(col)) != 0 # 1 to 3 numbers (cannot be more if shapeGridOK is True so need only to check not zero)
and col == sortedCol # In increasing order
# Values in the same thenth...
and i * 10 <= sortedCol[0]
and sortedCol[- 1] < (i + 1) * 10 + (i == 8)) # ... including 90 for the last column
def check_tombola(sheet):
filteredCols = [list(filter(lambda n: n != 0, col))
for i, col in enumerate(zip(* sheet))]
shapeGridOk = list(map(len, sheet)) == [9, 9, 9] # 3x9 grid
uniqueNums15_Ok = len(set(chain(* sheet))) == 16 # 16 with the 0s
Are5NumsByRow = all(len(set(row)) == 6 for row in sheet) # 6 with the 0s
validCols = all(checkCols(i, col, sorted(col))
for i, col in enumerate(filteredCols))
return all((shapeGridOk, uniqueNums15_Ok, Are5NumsByRow, validCols))
| Tombola - validation | 5898a751b2edc082f60005f4 | [
"Arrays",
"Fundamentals"
] | https://www.codewars.com/kata/5898a751b2edc082f60005f4 | 6 kyu |
The company you work for has just been awarded a contract to build a payment gateway. In order to help move things along, you have volunteered to create a function that will take a float and return the amount formatting in dollars and cents.
`39.99 becomes $39.99`
The rest of your team will make sure that the argument is sanitized before being passed to your function although you will need to account for adding trailing zeros if they are missing (though you won't have to worry about a dangling period).
Examples:
```
3 needs to become $3.00
3.1 needs to become $3.10
```
Good luck! Your team knows they can count on you! | algorithms | def format_money(amount):
return '${:.2f}' . format(amount)
| Dollars and Cents | 55902c5eaa8069a5b4000083 | [
"Functional Programming",
"Strings",
"Algorithms"
] | https://www.codewars.com/kata/55902c5eaa8069a5b4000083 | 8 kyu |
Create a function `add(n)`/`Add(n)` which returns a function that always adds n to any number
Note for Java: the return type and methods have not been provided to make it a bit more challenging.
```javascript
var addOne = add(1);
addOne(3); // 4
var addThree = add(3);
addThree(3); // 6
```
```python
add_one = add(1)
add_one(3) # 4
add_three = add(3)
add_three(3) # 6
```
```haskell
addOne = add 1
addOne 3 `shouldBe` 4
```
```fsharp
addOne = add 1
addOne 3 # 4
```
```swift
addOne = add(1)
addOne(3) // 4
```
```csharp
Func<double, double> AddOne = Kata.Add(1);
AddOne(3) => 4
```
```java
...returnType addOne = Kata.add(1);
addOne.method(3) => 4
```
```go
var addOne = Add(1)
addOne(3) // 4
```
```elixir
add_one = Kata.add(1)
add_one.(3) # 4
```
| reference | def add(n):
return lambda x: x + n
| Functional Addition | 538835ae443aae6e03000547 | [
"Functional Programming",
"Fundamentals"
] | https://www.codewars.com/kata/538835ae443aae6e03000547 | 7 kyu |
# Write Number in Expanded Form - Part 2
This is version 2 of my ['Write Number in Exanded Form' Kata](https://www.codewars.com/kata/write-number-in-expanded-form).
You will be given a number and you will need to return it as a string in expanded form :
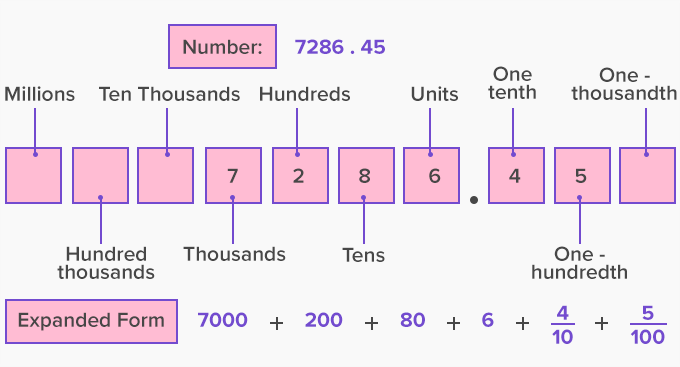
For example:
```rust
expanded_from(807.304); // Should return "800 + 7 + 3/10 + 4/1000"
expanded_from(1.24); // should return "1 + 2/10 + 4/100"
expanded_from(7.304); // should return "7 + 3/10 + 4/1000"
expanded_from(0.04); // should return "4/100"
``` | reference | def expanded_form(num):
integer_part, fractional_part = str(num). split('.')
result = [str(int(num) * (10 * * i)) for i, num in enumerate(integer_part[:: - 1]) if num != '0'][:: - 1]
result += [str(num) + '/' + str(10 * * (i + 1)) for i, num in enumerate(fractional_part) if num != '0']
return ' + ' . join(result)
| Write Number in Expanded Form - Part 2 | 58cda88814e65627c5000045 | [
"Mathematics",
"Algorithms",
"Fundamentals"
] | https://www.codewars.com/kata/58cda88814e65627c5000045 | 6 kyu |
# Write Number in Expanded Form
You will be given a number and you will need to return it as a string in [Expanded Form](https://www.mathsisfun.com/definitions/expanded-notation.html). For example:
```haskell
expandedForm 12 -- Should return '10 + 2'
expandedForm 42 -- Should return '40 + 2'
expandedForm 70304 -- Should return '70000 + 300 + 4'
```
```javascript
expandedForm(12); // Should return '10 + 2'
expandedForm(42); // Should return '40 + 2'
expandedForm(70304); // Should return '70000 + 300 + 4'
```
```python
expanded_form(12) # Should return '10 + 2'
expanded_form(42) # Should return '40 + 2'
expanded_form(70304) # Should return '70000 + 300 + 4'
```
```php
expanded_form(12); // Should return "10 + 2"
expanded_form(42); // Should return "40 + 2"
expanded_form(70304); // Should return "70000 + 300 + 4"
```
```ruby
expanded_form(12); # Should return '10 + 2'
expanded_form(42); # Should return '40 + 2'
expanded_form(70304); # Should return '70000 + 300 + 4'
```
```coffeescript
expandedForm(12); # Should return '10 + 2'
expandedForm(42); # Should return '40 + 2'
expandedForm(70304); # Should return '70000 + 300 + 4'
```
```java
Kata.expandedForm(12); # Should return "10 + 2"
Kata.expandedForm(42); # Should return "40 + 2"
Kata.expandedForm(70304); # Should return "70000 + 300 + 4"
```
```csharp
Kata.ExpandedForm(12); # Should return "10 + 2"
Kata.ExpandedForm(42); # Should return "40 + 2"
Kata.ExpandedForm(70304); # Should return "70000 + 300 + 4"
```
```fsharp
expandedForm 12L // Should return "10 + 2"
expandedForm 42L // Should return "40 + 2"
expandedForm 70304L // Should return "70000 + 300 + 4"
```
```rust
expanded_form(12); // Should return "10 + 2"
expanded_form(42); // Should return "40 + 2"
expanded_form(70304); // Should return "70000 + 300 + 4"
```
NOTE: All numbers will be whole numbers greater than 0.
If you liked this kata, check out [part 2](https://www.codewars.com/kata/write-number-in-expanded-form-part-2)!!
| reference | def expanded_form(num):
num = list(str(num))
return ' + ' . join(x + '0' * (len(num) - y - 1) for y, x in enumerate(num) if x != '0')
| Write Number in Expanded Form | 5842df8ccbd22792a4000245 | [
"Strings",
"Mathematics",
"Algorithms",
"Fundamentals"
] | https://www.codewars.com/kata/5842df8ccbd22792a4000245 | 6 kyu |
NOTE: It is recommended you complete [Introduction to Esolangs](https://www.codewars.com/kata/esolang-interpreters-number-1-introduction-to-esolangs-and-my-first-interpreter-ministringfuck/) or [MiniBitFlip](https://www.codewars.com/kata/esolang-minibitflip/) before solving this one.
<h3>Task:</h3>
Make an interpreter for an esoteric language called Ticker. Ticker is a descendant of [Tick](https://www.codewars.com/kata/esolang-tick). Your language has the following commands:
`>`: increment the selector by 1
`<`: decrement the selector by 1
`*`: add the ascii value of selected cell to the output tape
`+`: increment selected cell data by 1. If 256, then it is 0
`-`: increment selected cell data by -1. If less than 0, then 255
`/`: set selected cell data to 0
`!`: add new data cell to the end of the array
You start with selector at `0` and one cell with a value of `0`. If selector goes out of bounds, assume 0 for that cell <b>but do not add it to the memory. If a + or - is being made do not change the value of the assumed cell. It will always stay 0 unless it is added to the memory</b>
In other words:
```
data: start 0 end
selector: ^
data start 1 2 4 end
selector: ^
Assume that cell is zero.
```
<h3>Examples:</h3>
Consider the following program:
```python
'++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++*/+++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++*/++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++*/++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++*/+++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++*/++++++++++++++++++++++++++++++++*/+++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++*/+++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++*/++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++*/++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++*/++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++*/+++++++++++++++++++++++++++++++++*/'
```
It's output is this:
```python
'Hello World!'
```
This is made just by using 1 data cell.
Example using multiple data cells:
```python
'++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++*!>+++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++*!>++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++*!>++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++*!>+++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++*!>++++++++++++++++++++++++++++++++*!>+++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++*!>+++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++*!>++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++*!>++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++*!>++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++*!>+++++++++++++++++++++++++++++++++*!>'
```
And it's output is still:
```python
'Hello World!'
```
A more efficient example:
```python
'++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++*!>+++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++*!>++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++**!>+++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++*!>++++++++++++++++++++++++++++++++*!>+++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++*<<*>>!>++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++*<<<<*!>>>>>++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++*!>+++++++++++++++++++++++++++++++++*'
```
Which still returns the classic:
```python
'Hello World!'
```
Other characters are ingnored and therefore can serve as comments.
After you're done, fell free to make translations and to discuss this kata. | reference | def interpreter(tape):
memory, ptr, output = {0: 0}, 0, ""
for command in tape:
if command == ">":
ptr += 1
elif command == "<":
ptr -= 1
elif command == "!":
memory[len(memory)] = 0
elif command == "*":
output += chr(memory . get(ptr, 0) % 256)
elif ptr in memory:
if command == "+":
memory[ptr] += 1
elif command == "-":
memory[ptr] -= 1
elif command == "/":
memory[ptr] = 0
return output
| Esolang: Ticker | 5876e24130b45aaa0c00001d | [
"Interpreters",
"Strings",
"Arrays",
"Esoteric Languages"
] | https://www.codewars.com/kata/5876e24130b45aaa0c00001d | 5 kyu |
Tired of those repetitive javascript challenges? Here's a unique hackish one that should keep you busy for a while ;)
There's a mystery function which is already available for you to use. It's a simple function called `mystery`. It accepts a string as a parameter and outputs a string. The exercise depends on guessing what this function actually does.
You can call the mystery function like this:
```javascript
let myOutput = mystery('myInput');
```
```python
my_output = mystery("my_input")
```
Using your own test cases, try to call the mystery function with different input strings and try to analyze its output in order to guess what is does. You are free to call the mystery function in your own test cases however you want.
When you think you've understood how my mystery function works, prove it by reimplementing its logic in a function that you should call 'solved(x)'. To validate your code, your function 'solved' should return the same output as my function 'mystery' given the same inputs.
Beware! Passing your own test cases doesn't imply you'll pass mine.
Cheaters are welcome :)
Have fun!
---
Too easy? Then this kata will totally blow your mind:
http://www.codewars.com/kata/mystery-function-number-2 | games | def solved(s):
x = len(s) / / 2
return '' . join(sorted(s[: x] + s[x + (len(s) % 2):]))
| Mystery function #1 | 531963f82dde6fc8c800048a | [
"Puzzles"
] | https://www.codewars.com/kata/531963f82dde6fc8c800048a | 5 kyu |
# Task
You are given an array of integers `arr` that representing coordinates of obstacles situated on a straight line.
Assume that you are jumping from the point with coordinate 0 to the right. You are allowed only to make jumps of the same length represented by some integer.
Find the minimal length of the jump enough to avoid all the obstacles.
# Example
For `arr = [5, 3, 6, 7, 9]`, the output should be `4`.
Check out the image below for better understanding:
<img src = "https://codefightsuserpics.s3.amazonaws.com/tasks/avoidObstacles/img/example.png?_tm=1476261772863" />
# Input/Output
- `[input]` integer array `arr`
Non-empty array of positive integers.
Constraints: `1 ≤ inputArray[i] ≤ 100.`
- `[output]` an integer
The desired length. | games | def avoid_obstacles(arr):
n = 2
while 1:
if all([x % n for x in arr]):
return n
n += 1
| Simple Fun #70: Avoid Obstacles | 5894045b8a8a230d0c000077 | [
"Puzzles"
] | https://www.codewars.com/kata/5894045b8a8a230d0c000077 | 6 kyu |
We have the numbers with different colours with the sequence: [<span style="color:red">'red'</span>, <span style="color:#EEBB00">'yellow'</span>, <span style="color:DodgerBlue">'blue'</span>].
That sequence colours the numbers in the following way:
<span style="color:red"> 1 </span> <span style="color:#EEBB00"> 2 </span><span style="color:DodgerBlue"> 3 </span> <span style="color:red"> 4 </span> <span style="color:#EEBB00"> 5 </span> <span style="color:DodgerBlue"> 6 </span><span style="color:red"> 7</span> <span style="color:#EEBB00"> 8</span> <span style="color:DodgerBlue"> 9</span> <span style="color:red"> 10</span> <span style="color:#EEBB00"> 11</span> <span style="color:DodgerBlue"> 12 </span><span style="color:red"> 13</span> .....
We have got the following recursive function:
```
f(1) = 1
f(n) = f(n - 1) + n
```
Some terms of this sequence with their corresponding colour are:
```
term value colour
1 1 "red"
2 3 "blue"
3 6 "blue"
4 10 "red"
5 15 "blue"
6 21 "blue"
7 28 "red"
```
The three terms of the same colour "blue", higher than 3, are: `[6, 15, 21]`
We need a function `same_col_seq(), that may receive three arguments:
- `val`, an integer number
- `k`, an integer number
- `colour`, the name of one of the three colours(red, yellow or blue), as a string.
The function will output a sorted array with the smallest `k` terms, having the same marked colour, but higher than `val`.
Let's see some examples:
```python
same_col_seq(3, 3, 'blue') == [6, 15, 21]
same_col_seq(100, 4, 'red') == [136, 190, 253, 325]
```
The function may output an empty list if it does not find terms of the sequence with the wanted colour in the range [val, 2* k * val]
```python
same_col_seq(250, 6, 'yellow') == []
```
That means that the function did not find any "yellow" term in the range `[250, 3000]`
Tests will be with the following features:
* Nmber of tests: `100`
* `100 < val < 1000000`
* `3 < k < 20`
| reference | D, R = {}, [[], [], []]
for i in range(10000):
D[i] = D . get(i - 1, 0) + i
R[D[i] % 3]. append(D[i])
def same_col_seq(val, k, col):
r = ['blue', 'red', 'yellow']. index(col)
return [e for e in R[r] if e > val][: k]
| Working With Coloured Numbers | 57f891255cae44b2e10000c5 | [
"Fundamentals",
"Data Structures",
"Algorithms",
"Mathematics",
"Logic",
"Strings",
"Recursion",
"Dynamic Programming"
] | https://www.codewars.com/kata/57f891255cae44b2e10000c5 | 5 kyu |
# Parse a linked list from a string
## Related Kata
Although this Kata is not part of an official Series, you may want to complete [this Kata](https://www.codewars.com/kata/convert-a-linked-list-to-a-string) before attempting this one as these two Kata are deeply related.
## Preloaded
Preloaded for you is a class, struct or derived data type `Node` ( depending on the language ) used to construct linked lists in this Kata:
```php
class Node {
public $data, $next;
public function __construct($data, $next = NULL) {
$this->data = $data;
$this->next = $next;
}
}
```
```javascript
class Node {
constructor(data, next = null) {
this.data = data;
this.next = next;
}
}
```
```csharp
public class Node : Object
{
public int Data;
public Node Next;
public Node(int data, Node next = null)
{
this.Data = data;
this.Next = next;
}
public override bool Equals(Object obj)
{
// Check for null values and compare run-time types.
if (obj == null || GetType() != obj.GetType()) { return false; }
return this.ToString() == obj.ToString();
}
public override string ToString()
{
List<int> result = new List<int>();
Node curr = this;
while (curr != null)
{
result.Add(curr.Data);
curr = curr.Next;
}
return String.Join(" -> ", result) + " -> null";
}
}
```
```swift
class Node {
var data : Int
var next : Node?
init(_ data:Int) {
self.data = data
self.next = nil
}
init(_ data:Int, _ next: Node?) {
self.data = data
self.next = next
}
}
```
```c
typedef struct node {
int data;
struct node *next;
} Node;
```
```cpp
class Node {
public:
int data;
Node *next;
Node(int data, Node *next = nullptr): data(data), next(next) {}
};
```
```objc
typedef struct node {
int data;
struct node *next;
} Node;
```
```fortran
type Node
integer :: data
type(Node), pointer :: next
end type Node
```
```scala
case class Node(data: Int, next: Node = null)
```
```haskell
-- use regular lists, which are already singly-linked
data [a] = [] | a : [a]
```
```python
class Node:
def __init__(self, data, next=None):
self.data = data
self.next = next
```
```cobol
01 node.
05 val pic 9(4).
05 nxt usage pointer.
```
~~~if:c
*NOTE: In C, the* `Node` *struct is placed on top of your main solution because otherwise the compiler complains about not recognizing the struct (even if it is defined in the Preloaded section). Attempts to modify it (e.g. to cheat the tests in some way) will likely result in a test crash so it is not recommended for you to modify that section ;)*
~~~
~~~if:objc
*NOTE: In Objective-C, the* `Node` *struct is placed on top of your main solution because there is a "double-import" bug in the Preloaded section at the time of writing that cannot be fixed on my end. Attempts to modify it (e.g. to cheat the tests in some way) will likely result in a test crash so it is not recommended for you to modify that section ;)*
~~~
~~~if:cobol
Use the preallocated table `nodesTable` in linkage-section to store the nodes of the linked list.
~~~
## Prerequisites
This Kata assumes that you are already familiar with the idea of a linked list. If you do not know what that is, you may want to read up on [this article on Wikipedia](https://en.wikipedia.org/wiki/Linked_list). Specifically, the linked lists this Kata is referring to are **singly linked lists**, where the value of a specific node is stored in its `data` / `$data`/`Data` property, the reference to the next node is stored in its `next` / `$next` / `Next` property and the terminator for a list is `null` / `NULL` / `nil` / `nullptr` / `null()` / `[]`.
Additionally, this Kata assumes that you have basic knowledge of Object-Oriented Programming ( or a similar concept ) in the programming language you are undertaking. If you have not come across Object-Oriented Programming in your selected language, you may want to try out an online course or read up on some code examples of OOP in your selected language up to ( but not necessarily including ) Classical Inheritance.
*Specifically, if you are attempting this Kata in PHP and haven't come across OOP, you may want to try out the first 4 Kata in [this Series](https://www.codewars.com/collections/object-oriented-php).*
## Task
Create a function `parse` which accepts exactly one argument `string` / `$string` / `s` / `strrep` ( or similar, depending on the language ) which is a string representation of a linked list. Your function must return the corresponding linked list, constructed from instances of the `Node` class/struct/type. The string representation of a list has the following format: the value of the node, followed by a whitespace, an arrow and another whitespace (`" -> "`), followed by the rest of the linked list. Each string representation of a linked list will end in `"null"` / `"NULL"` / `"nil"` / `"nullptr"` / `"null()"` depending on the language you are undertaking this Kata in. For example, given the following string representation of a linked list:
```javascript
"1 -> 2 -> 3 -> null"
```
```php
"1 -> 2 -> 3 -> NULL"
```
```c
"1 -> 2 -> 3 -> NULL"
```
```swift
"1 -> 2 -> 3 -> nil"
```
```cpp
"1 -> 2 -> 3 -> nullptr"
```
```objc
@"1 -> 2 -> 3 -> NULL"
```
```fortran
"1 -> 2 -> 3 -> null()"
```
```cobol
"1 -> 2 -> 3 -> null"
```
... your function should return:
<!-- unlisted languages use the top block -- please keep javascript up top -->
```javascript
new Node(1, new Node(2, new Node(3)))
```
```swift
Node(1, Node(2, Node(3)))
```
```c
// Code example not applicable to C - the Node struct does not have a constructor function
```
```objc
// Code example not applicable to Objective-C - the Node struct does not have a constructor function
```
```fortran
type(Node), pointer :: list
! Where:
! list%data == 1
! list%next%data == 2
! list%next%next%data == 3
! list%next%next%next => null()
```
```scala
Node(1, Node(2, Node(3)))
```
```haskell
[ 1, 2, 3 ]
```
Note that due to the way the constructor for `Node` is defined, if a second argument is not provided, the `next` / `$next` / `Next` field is automatically set to `null` / `NULL` / `nil` / `nullptr` ( or equivalent in your language ). That means your function could also return the following ( if it helps you better visualise what is actually going on ):
<!-- unlisted languages use the top block -- please keep javascript up top -->
```javascript
new Node(1, new Node(2, new Node(3, null)))
```
```php
new Node(1, new Node(2, new Node(3, NULL)))
```
```swift
Node(1, Node(2, Node(3, nil)))
```
```c
// In C the Node struct does not have a constructor function - please return a dynamically allocated version of the list displayed below:
&((Node){
.data = 1,
.next = &((Node){
.data = 2,
.next = &((Node){
.data = 3,
.next = NULL
})
})
})
```
```cpp
new Node(1, new Node(2, new Node(3, nullptr)))
```
```objc
// In Objective-C the Node struct does not have a constructor function - please return a dynamically allocated version of the list displayed below:
&((Node){
.data = 1,
.next = &((Node){
.data = 2,
.next = &((Node){
.data = 3,
.next = NULL
})
})
})
```
```fortran
! Code example not applicable to Fortran - there is no constructor for `Node`
```
```haskell
this section is not applicable to Haskell
```
```cobol
* Code example not applicable to COBOL
```
Another example: given the following string input:
<!-- unlisted languages use the top block -- please keep javascript up top -->
```javascript
"0 -> 1 -> 4 -> 9 -> 16 -> null"
```
```php
"0 -> 1 -> 4 -> 9 -> 16 -> NULL"
```
```c
"0 -> 1 -> 4 -> 9 -> 16 -> NULL"
```
```swift
"0 -> 1 -> 4 -> 9 -> 16 -> nil"
```
```cpp
"0 -> 1 -> 4 -> 9 -> 16 -> nullptr"
```
```objc
@"0 -> 1 -> 4 -> 9 -> 16 -> NULL"
```
```fortran
"0 -> 1 -> 4 -> 9 -> 16 -> null()"
```
... your function should return:
<!-- unlisted languages use the top block -- please keep javascript up top -->
```javascript
new Node(0, new Node(1, new Node(4, new Node(9, new Node(16)))))
```
```swift
Node(0, Node(1, Node(4, Node(9, Node(16)))))
```
```c
&((Node){
.data = 0,
.next = &((Node){
.data = 1,
.next = &((Node){
.data = 4,
.next = &((Node){
.data = 9,
.next = &((Node){
.data = 16,
.next = NULL
})
})
})
})
})
```
```objc
&((Node){
.data = 0,
.next = &((Node){
.data = 1,
.next = &((Node){
.data = 4,
.next = &((Node){
.data = 9,
.next = &((Node){
.data = 16,
.next = NULL
})
})
})
})
})
```
```fortran
type(Node), pointer :: list
! Where:
! list%data == 0
! list%next%data == 1
! list%next%next%data == 4
! list%next%next%next%data == 9
! list%next%next%next%next%data == 16
! list%next%next%next%next%next => null()
```
```haskell
[ 0, 1, 4, 9, 16 ]
```
If the input string is just `"null"` / `"NULL"` / `"nil"` / `"nullptr"` / `"null()"`, return `null` / `NULL` / `nil` / `nullptr` / `null()` / `[]` ( or equivalent ).
For the simplicity of this Kata, the values of the nodes in the string representation will always ever be **non-negative integers**, so the following would **not** occur: `"Hello World -> Goodbye World -> 123 -> null"` / `"Hello World -> Goodbye World -> 123 -> NULL"` / `"Hello World -> Goodbye World -> 123 -> nil"` / `"Hello World -> Goodbye World -> 123 -> nullptr"` ( depending on the language ). This also means that the values of each `Node` must also be **non-negative integers** so keep that in mind when you are parsing the list from the string.
~~~if:fortran
Furthermore, if you are attempting this Kata in Fortran, you may assume that the string input will never contains leading and/or trailing whitespace.
~~~
Enjoy, and don't forget to check out my other Kata Series :D
| algorithms | def linked_list_from_string(s):
head = None
for i in s . split('->')[- 2:: - 1]:
head = Node(int(i), head)
return head
| Parse a linked list from a string | 582c5382f000e535100001a7 | [
"Linked Lists",
"Recursion",
"Algorithms"
] | https://www.codewars.com/kata/582c5382f000e535100001a7 | 6 kyu |
Professor Chambouliard hast just discovered a new type of magnet material. He put particles of this material in a box made of small boxes arranged
in K rows and N columns as a kind of **2D matrix** `K x N` where `K` and `N` are postive integers.
He thinks that his calculations show that the force exerted by the particle in the small box `(k, n)` is:
```math
\displaystyle v(k,n) = \dfrac{1}{k(n+1)^{2k}}
```
The total force exerted by the first row with `k = 1` is:
```math
\displaystyle u(1, N) = \sum_{n=1}^{n=N}v(1, n) = \dfrac{1}{1 \cdot 2^2}+\dfrac{1}{1\cdot 3^2} + \dots + \frac{1}{1 \cdot (N+1)^2}
```
We can go on with `k = 2` and then `k = 3` etc ... and consider:
```math
\displaystyle S(K, N) = \sum_{k=1}^{k=K} u(k, N) = \sum_{k=1}^{k=K} \bigg(\sum_{n=1}^{n=N} v(k, n) \bigg) \to(double(max_k, max_n))
```
#### Task:
To help Professor Chambouliard can we calculate the function `doubles` that will take as parameter `maxk` and `maxn` such that `doubles(maxk, maxn) = S(maxk, maxn)`?
Experiences seems to show that this could be something around `0.7` when `maxk` and `maxn` are big enough.
#### Examples:
```
doubles(1, 3) => 0.4236111111111111
doubles(1, 10) => 0.5580321939764581
doubles(10, 100) => 0.6832948559787737
```
#### Notes:
- In `u(1, N)` the dot is the *multiplication operator*.
- Don't truncate or round: Have a look at the testing function in "Sample Tests".
- [link to symbol Sigma](https://en.wikipedia.org/wiki/Summation)
| algorithms | from scipy . special import zeta, zetac
def doubles(maxk, maxn):
return sum((zetac(2 * k) - zeta(2 * k, 2 + maxn)) / k for k in range(1, maxk + 1))
| Magnet particules in boxes | 56c04261c3fcf33f2d000534 | [
"Mathematics",
"Matrix",
"Algorithms"
] | https://www.codewars.com/kata/56c04261c3fcf33f2d000534 | 4 kyu |
Teemo is not really excited about the new year's eve, but he has to celebrate it with his friends anyway.
<br>
<br>
He has a really big passion about programming and he wants to be productive till midnight. He wants to know how many minutes he has left to work on his new project.<br> He doesn't want to look on the clock all the time, so he thought about a function, which returns him the number of minutes.<br>
<br>
<code>Can you write him a function, so he can stay productive?</code>
<br>
<br>
<b>The function <code>minutesToMidnight(d)</code> will take a date object as parameter. Return the number of minutes in the following format:</b> <br><br>
<code><b>"x minute(s)"</b></code>
<br>
<br>
You will always get a date object with of today with a random timestamp. <br>
You have to round the number of minutes. <br>
Milliseconds doesn't matter!
<code>
Some examples:<br>
<br>
10.00 am => "840 minutes"<br>
23.59 pm => "1 minute"
</code>
<br>
<br>
<b>This kata is part of the Collection "Date fundamentals":</b>
<ul>
<li><a href="https://www.codewars.com/kata/count-the-days/javascript">#1 Count the Days!</a></li>
<li>#2 Minutes to Midnight</li>
<li><a href="https://www.codewars.com/kata/can-santa-save-christmas">#3 Can Santa save Christmas?</a></li>
<li><a href="https://www.codewars.com/kata/christmas-present-calculator">#4 Christmas Present Calculator</a></li>
</ul> | reference | from datetime import datetime, time, timedelta
def minutes_to_midnight(d):
next_day = datetime . combine(d + timedelta(days=1), time . min)
remain = next_day - d
minutes = round(remain . seconds / 60)
return f' { minutes } minutes'
| Minutes to Midnight | 58528e9e22555d8d33000163 | [
"Date Time",
"Fundamentals"
] | https://www.codewars.com/kata/58528e9e22555d8d33000163 | 6 kyu |
Little Annie is very excited for upcoming events. She wants to know how many days she has to wait for a specific event.
Your job is to help her out.
Task:
Write a function which returns the number of days from today till the given date. The function will take a Date object as parameter.
You have to round the amount of days.
If the event is in the past, return <b>"The day is in the past!"</b> <br>
If the event is today, return <b>"Today is the day!"</b> <br>
Else, return <b>"x days"</b>
PS: This is my first kata. I hope you have fun^^
<br>
<br>
<b>This kata is part of the Collection "Date fundamentals":</b>
<ul>
<li>#1 Count the Days!</li>
<li><a href="https://www.codewars.com/kata/minutes-to-midnight">#2 Minutes to Midnight</a></li>
<li><a href="https://www.codewars.com/kata/can-santa-save-christmas">#3 Can Santa save Christmas?</a></li>
<li><a href="https://www.codewars.com/kata/christmas-present-calculator">#4 Christmas Present Calculator</a></li>
</ul> | reference | from datetime import datetime as dt
def count_days(d):
days = round((d - dt . now()). total_seconds() / 86400)
return ['Today is the day!', '%d days' % days, 'The day is in the past!'][(days > 0) - (days < 0)]
| Count the days! | 5837fd7d44ff282acd000157 | [
"Date Time",
"Fundamentals"
] | https://www.codewars.com/kata/5837fd7d44ff282acd000157 | 6 kyu |
# Can Santa save Christmas?
Oh no! Santa's little elves are sick this year. He has to distribute the presents on his own.
But he has only 24 hours left. Can he do it?
## Your Task:
You will get an array as input with time durations as string in the following format: `HH:MM:SS`. Each duration represents the time taken by Santa to deliver a present. Determine whether he can do it in 24 hours or not.
In case the time required to deliver all of the presents is exactly 24 hours, Santa can complete the delivery ;-) .
___
If this kata was to easy for you. Try the [harder one](https://www.codewars.com/kata/christmas-present-calculator).
### This kata is part of the Collection "Date fundamentals":
* [#1 Count the Days!](https://www.codewars.com/kata/count-the-days/javascript)
* [#2 Minutes to Midnight](https://www.codewars.com/kata/minutes-to-midnight)
* #3 Can Santa save Christmas?
* [#4 Christmas Present Calculator](https://www.codewars.com/kata/christmas-present-calculator)
| reference | def determine_time(arr):
total = 0
for time in arr:
h, m, s = map(int, time . split(":"))
total += h * 60 * 60 + m * 60 + s
return total <= 24 * 60 * 60
| Can Santa save Christmas? | 5857e8bb9948644aa1000246 | [
"Date Time",
"Fundamentals"
] | https://www.codewars.com/kata/5857e8bb9948644aa1000246 | 7 kyu |
The student needs to get on a train that leaves from the station `D` kilometres away in `T` minutes.
She can get a taxi that drives at `V1` km/h for the price of `R` €/km or she can walk at `V2` km/h for free.
A correct solution will be a function that returns the minimum price she needs to pay the taxi driver or the string `"Won't make it!"`.
All the inputs will be positive integers, the output has to be a string containing a number with two decimal places - or `"Won't make it!"` if that is the case.
It won't take her any time to get on the taxi or the train.
In non-trivial cases you need to combine walking and riding the taxi so that she makes it, but pays as little as possible. | games | def calculate_optimal_fare(d, t, taxi, r, walk):
h = t / 60.0
if walk * h >= d:
return "0.00"
if taxi * h < d:
return "Won't make it!"
return "%.2f" % (r * taxi * (d - walk * h) / (taxi - walk))
| Optimal Taxi Fare | 52f51502053125863c0009d7 | [
"Mathematics",
"Algebra",
"Puzzles"
] | https://www.codewars.com/kata/52f51502053125863c0009d7 | 6 kyu |
# Task
You are given a `moment` in time and space. What you must do is break it down into time and space, to determine if that moment is from the past, present or future.
`Time` is the sum of characters that increase time (i.e. numbers in range `['1'..'9']`.
`Space` in the number of characters which do not increase time (i.e. all characters but those that increase time).
The moment of time is determined as follows:
```
If time is greater than space, then the moment is from the future.
If time is less than space, then the moment is from the past.
Otherwise, it is the present moment.```
You should return an array of three elements, two of which are false, and one is true. The true value should be at the `1st, 2nd or 3rd` place for `past, present and future` respectively.
# Examples
For `moment = "01:00 pm"`, the output should be `[true, false, false]`.
time equals 1, and space equals 7, so the moment is from the past.
For `moment = "12:02 pm"`, the output should be `[false, true, false]`.
time equals 5, and space equals 5, which means that it's a present moment.
For `moment = "12:30 pm"`, the output should be `[false, false, true]`.
time equals 6, space equals 5, so the moment is from the future.
# Input/Output
- `[input]` string `moment`
The moment of time and space that the input time came from.
- `[output]` a boolean array
Array of three elements, two of which are false, and one is true. The true value should be at the 1st, 2nd or 3rd place for past, present and future respectively. | reference | def moment_of_time_in_space(moment):
d = sum(int(c) if c in '123456789' else - 1 for c in moment)
return [d < 0, d == 0, d > 0]
| Simple Fun #193: Moment Of Time In Space | 58c2158ec7df54a39d00015c | [
"Fundamentals"
] | https://www.codewars.com/kata/58c2158ec7df54a39d00015c | 7 kyu |
Given two numbers and an arithmetic operator (the name of it, as a string), return the result of the two numbers having that operator used on them.
```a``` and ```b``` will both be positive integers, and ```a``` will always be the first number in the operation, and ```b``` always the second.
The four operators are "add", "subtract", "divide", "multiply".
~~~if-not:haskell
A few **examples:(Input1, Input2, Input3 --> Output)**
```
5, 2, "add" --> 7
5, 2, "subtract" --> 3
5, 2, "multiply" --> 10
5, 2, "divide" --> 2.5
```
~~~
~~~if:haskell
In **Haskell**:
1. The operation is defined as
```haskell
data Operation = Add | Divide | Multiply | Subtract deriving (Eq, Show, Enum, Bounded)
```
2. The arithmetic function as
```haskell
arithmetic :: Double -> Double -> Operation -> Double
arithmetic :: Fractional a => a -> a -> Operation -> a
```
~~~
Try to do it without using if statements!
| reference | def arithmetic(a, b, operator):
return {
'add': a + b,
'subtract': a - b,
'multiply': a * b,
'divide': a / b,
}[operator]
| Make a function that does arithmetic! | 583f158ea20cfcbeb400000a | [
"Fundamentals"
] | https://www.codewars.com/kata/583f158ea20cfcbeb400000a | 7 kyu |
Given: an array containing hashes of names
Return: a string formatted as a list of names separated by commas except for the last two names, which should be separated by an ampersand.
Example:
``` ruby
list([ {name: 'Bart'}, {name: 'Lisa'}, {name: 'Maggie'} ])
# returns 'Bart, Lisa & Maggie'
list([ {name: 'Bart'}, {name: 'Lisa'} ])
# returns 'Bart & Lisa'
list([ {name: 'Bart'} ])
# returns 'Bart'
list([])
# returns ''
```
``` elixir
list([ %{name: "Bart"}, %{name: "Lisa"}, %{name: "Maggie"} ])
# returns 'Bart, Lisa & Maggie'
list([ %{name: "Bart"}, %{name: "Lisa"} ])
# returns 'Bart & Lisa'
list([ %{name: "Bart"} ])
# returns 'Bart'
list([])
# returns ''
```
``` javascript
list([ {name: 'Bart'}, {name: 'Lisa'}, {name: 'Maggie'} ])
// returns 'Bart, Lisa & Maggie'
list([ {name: 'Bart'}, {name: 'Lisa'} ])
// returns 'Bart & Lisa'
list([ {name: 'Bart'} ])
// returns 'Bart'
list([])
// returns ''
```
```python
namelist([ {'name': 'Bart'}, {'name': 'Lisa'}, {'name': 'Maggie'} ])
# returns 'Bart, Lisa & Maggie'
namelist([ {'name': 'Bart'}, {'name': 'Lisa'} ])
# returns 'Bart & Lisa'
namelist([ {'name': 'Bart'} ])
# returns 'Bart'
namelist([])
# returns ''
```
Note: all the hashes are pre-validated and will only contain A-Z, a-z, '-' and '.'.
| reference | def namelist(names):
if len(names) > 1:
return '{} & {}' . format(', ' . join(name['name'] for name in names[: - 1]),
names[- 1]['name'])
elif names:
return names[0]['name']
else:
return ''
| Format a string of names like 'Bart, Lisa & Maggie'. | 53368a47e38700bd8300030d | [
"Fundamentals",
"Strings",
"Data Types",
"Formatting",
"Algorithms",
"Logic"
] | https://www.codewars.com/kata/53368a47e38700bd8300030d | 6 kyu |
The number six has this interesting property, and is the smallest number in having it (after the integer ```1``` that obviously fulfills this condition):
Its cube, is divisible by the sum of its divisors.
Let's see it:
```
6 ^ 3 = 216
divisors of 6: 1, 2, 3, 6
sum of its divisors= 1 + 2 + 3 + 6 = 12
And 216 / 12 = 18 (integer) and 18.12 = 216
```
The first terms of this sequence are:
```
n a(n)
1 6
2 28
3 30
4 84
5 102
```
Your challenge is to create a function that receives an ordinal number `n` of the term of the sequence as an argument, and returns the `nth` term of that sequece of integers.
Let's see some cases (input ------> output):
```
4 ------> 84
/// divisors of 84 : 1, 2, 3, 4, 6, 7, 12, 14, 21, 28, 42, 84
sum of divisors = 1 + 2 + 3 + 4 + 6 + 7 + 12 + 14 + 21 + 28 + 42 + 84 = 224
cube of 84 = 84^3 = 592704
592704 is a multiple of 224///
5 ------> 102
```
Your code should use memoization in order to have higher speed for testing. Does your code reach up to the 120-th term? We can get this upper limit in the tests.
Enjoy it and happy coding!! | algorithms | # The global variable holding the matching numbers.
# It will increase in size as more numbers are found while the program is running continuously.
NUMS = [6, 28, 30, 84, 102]
def divisors(n):
d = {1, n}
for k in range(2, int(n * * 0.5) + 1):
if n % k == 0:
d . add(k)
d . add(n / / k)
return d
def int_cube_sum_div(n):
global NUMS
while len(NUMS) < n:
a = NUMS[- 1] + 1
while pow(a, 3) % sum(divisors(a)):
a += 1
NUMS . append(a)
return NUMS[n - 1]
| Raise Me to The Third Power, Search My Divisors... .....Could You Believe that? | 56060ba7b02b967eb1000013 | [
"Algorithms",
"Mathematics",
"Memoization",
"Dynamic Programming"
] | https://www.codewars.com/kata/56060ba7b02b967eb1000013 | 6 kyu |
The number 45 is the first integer in having this interesting property:
the sum of the number with its reversed is divisible by the difference between them(absolute Value).
```
45 + 54 = 99
abs(45 - 54) = 9
99 is divisible by 9.
```
The first terms of this special sequence are :
```
n a(n)
1 45
2 54
3 495
4 594
```
Make the function that receives ```n```, the ordinal number of the term and may give us, the value of the term of the sequence.
Let's see some cases (input ----> output):
```
1 -----> 45
3 -----> 495
```
"Important: Do not include numbers which, when reversed, have a leading zero, e.g.: 1890 reversed is 0981, so 1890 should not be included."
Your code will be tested up to the first 65 terms, so you should think to optimize it as much as you can.
(Hint: I memoize, you memoize, he memoizes, ......they (of course) memoize)
Happy coding!!
| reference | MEMO = []
def sum_dif_rev(n):
i = MEMO[- 1] if MEMO else 0
while len(MEMO) < n:
i += 1
r = int(str(i)[:: - 1])
if i % 10 and r != i and (i + r) % abs(r - i) == 0:
MEMO . append(i)
return MEMO[n - 1]
| Sum and Rest the Number with its Reversed and See What Happens | 5603a9585480c94bd5000073 | [
"Fundamentals",
"Mathematics",
"Algorithms",
"Memoization",
"Dynamic Programming"
] | https://www.codewars.com/kata/5603a9585480c94bd5000073 | 5 kyu |
The number `12` is the first number in having six divisors, they are: `1, 2, 3, 4, 6 and 12.`
Your challenge for this kata is to find the minimum number that has a certain number of divisors.
For this purpose we have to create the function
`find_min_num() or findMinNum() or similar in the other languages`
that receives the wanted number of divisors `num_div`, and outputs the smallest number having an amount of divisors equals to `num_div`.
Let's see some cases:
```
find_min_num(10) = 48 # divisors are: 1, 2, 3, 4, 6, 8, 12, 16, 24 and 48
find_min_num(12) = 60
```
In this kata all the tests will be with `numDiv < 80`
(There will be a next kata with numDiv < 10000, Find the First Number Having a Certain Number of Divisors II, should have the help of number theory)
Enjoy it and happy coding!
(Memoization is advisable) | reference | # key - number of divisors: value - the smallest number with the given number of divisors.
# 'nr' indicates the number that the search was performed to
# When the program is running continuously, the dictionary grows with new values.
CACHE = {'nr': 2, 2: 2}
def divisors(n):
d = {1, n}
for k in range(2, int(n * * 0.5) + 1):
if n % k == 0:
d . add(k)
d . add(n / / k)
return d
def find_min_num(num_div):
global CACHE
# If the number of divisors you are looking for has not yet been found.
while num_div not in CACHE:
# Start searching from this number as the previous numbers have already been checked.
nr = CACHE['nr'] + 1
while True:
# The number of divisors of the currently checked number.
d = len(divisors(nr))
if d == num_div: # If the required number of divisors is found
# add it to CACHE so you don't have to search for it next time.
CACHE[d] = nr
break
if d not in CACHE: # If the number of divisors does not yet exist in CACHE
# add it to CACHE so you don't have to search for it next time.
CACHE[d] = nr
nr += 1 # Check the next number.
# Update the number from which the next search will start.
CACHE['nr'] = nr
return CACHE[num_div]
| Find the First Number in Having a Certain Number of Divisors I | 5612ab201830eb000f0000c0 | [
"Fundamentals",
"Mathematics",
"Data Structures",
"Dynamic Programming",
"Memoization"
] | https://www.codewars.com/kata/5612ab201830eb000f0000c0 | 6 kyu |
You receive some random elements as a space-delimited string. Check if the elements are part of an ascending sequence of integers starting with 1, with an increment of 1 (e.g. 1, 2, 3, 4).
Return:
* `0` if the elements can form such a sequence, and no number is missing ("not broken", e.g. `"1 2 4 3"`)
* `1` if there are any non-numeric elements in the input ("invalid", e.g. `"1 2 a"`)
* `n` if the elements are part of such a sequence, but some numbers are missing, and `n` is the lowest of them ("broken", e.g. `"1 2 4"` or `"1 5"`)
## Examples
```
"1 2 3 4" ==> return 0, because the sequence is complete
"1 2 4 3" ==> return 0, because the sequence is complete (order doesn't matter)
"2 1 3 a" ==> return 1, because it contains a non numerical character
"1 3 2 5" ==> return 4, because 4 is missing from the sequence
"1 5" ==> return 2, because the sequence is missing 2, 3, 4 and 2 is the lowest
``` | reference | def find_missing_number(sequence):
try:
numbers = sorted([int(x) for x in sequence . split()])
for i in range(1, len(numbers) + 1):
if i not in numbers:
return i
except ValueError:
return 1
return 0
| Broken sequence | 5512e5662b34d88e44000060 | [
"Strings",
"Fundamentals"
] | https://www.codewars.com/kata/5512e5662b34d88e44000060 | 7 kyu |
##Do you know how to write a recursive function? Let's test it!
---
*
Definition: Recursive function is a function that calls itself during its execution
*
```
Classic factorial counting on Javascript
function factorial(n) {
return n <= 1 ? 1 : n * factorial(n-1)
}
```
---
Your objective is to complete a recursive function `reverse()` that receives `str` as String and returns the same string in reverse order
Rules:
- reverse function should be executed exactly N times. `N = length of the input string`
- helper functions are not allowed
- changing the signature of the function is not allowed
Examples:
```
reverse("hello world") = "dlrow olleh" (N = 11)
reverse("abcd") = "dcba" (N = 4)
reverse("12345") = "54321" (N = 5)
```
<i>All tests for this Kata are randomly generated, besides checking the reverse logic they will count how many times the reverse() function has been executed. <i> | reference | def reverse(str):
return "" if not str else str[- 1] + reverse(str[: - 1])
| Recursive reverse string | 536a9f94021a76ef0f00052f | [
"Fundamentals",
"Strings",
"Data Types"
] | https://www.codewars.com/kata/536a9f94021a76ef0f00052f | 7 kyu |
We are interested in collecting the sets of six prime numbers, that having a starting prime p, the following values are also primes forming the sextuplet ```[p, p + 4, p + 6, p + 10, p + 12, p + 16]```
The first sextuplet that we find is ```[7, 11, 13, 17, 19, 23]```
The second one is ```[97, 101, 103, 107, 109, 113]```
Given a number ```sum_limit```, you should give the first sextuplet which sum (of its six primes) surpasses the sum_limit value.
```python
find_primes_sextuplet(70) == [7, 11, 13, 17, 19, 23]
find_primes_sextuplet(600) == [97, 101, 103, 107, 109, 113]
```
Features of the tests:
```
Number Of Tests = 18
10000 < sum_limit < 29700000
```
If you have solved this kata perhaps you will find easy to solve this one:
https://www.codewars.com/kata/primes-with-two-even-and-double-even-jumps/
Enjoy it!! | reference | def find_primes_sextuplet(limit):
for p in [7, 97, 16057, 19417, 43777, 1091257, 1615837, 1954357, 2822707, 2839927, 3243337, 3400207, 6005887]:
if p * 6 + 48 > limit:
return [p, p + 4, p + 6, p + 10, p + 12, p + 16]
| Prime Sextuplets | 57bf7fae3b3164dcac000352 | [
"Algorithms",
"Mathematics",
"Sorting",
"Data Structures",
"Memoization"
] | https://www.codewars.com/kata/57bf7fae3b3164dcac000352 | 5 kyu |
Your task is to generate the Fibonacci sequence to `n` places, with each alternating value as `"skip"`. For example:
`"1 skip 2 skip 5 skip 13 skip 34"`
Return the result as a string
You can presume that `n` is always a positive integer between (and including) 1 and 64. | games | def skiponacci(n):
fib = [1, 1][: n]
for _ in range(n - 2):
fib . append(sum(fib[- 2:]))
return " " . join(str(n) if i % 2 else "skip" for i, n in enumerate(fib, 1))
| The Skiponacci Sequence | 580777ee2e14accd9f000165 | [
"Puzzles",
"Algorithms"
] | https://www.codewars.com/kata/580777ee2e14accd9f000165 | 7 kyu |
# SpeedCode #2 - Array Madness
## Objective
Given two **integer arrays** ```a, b```, both of ```length >= 1```, create a program that returns ```true``` if the **sum of the squares** of each element in ```a``` is **strictly greater than** the **sum of the cubes** of each element in ```b```.
E.g.
```c
array_madness(3, {4, 5, 6}, 3, {1, 2, 3}) == true;
// because 4 ** 2 + 5 ** 2 + 6 ** 2 > 1 ** 3 + 2 ** 3 + 3 ** 3
```
```javascript
arrayMadness([4, 5, 6], [1, 2, 3]); // returns true since 4 ** 2 + 5 ** 2 + 6 ** 2 > 1 ** 3 + 2 ** 3 + 3 ** 3
```
```csharp
Kata.ArrayMadness(new int[] {4, 5, 6}, new int[] {1, 2, 3}) => true // because 4 ** 2 + 5 ** 2 + 6 ** 2 > 1 ** 3 + 2 ** 3 + 3 ** 3
```
```python
array_madness([4, 5, 6], [1, 2, 3]) => True #because 4 ** 2 + 5 ** 2 + 6 ** 2 > 1 ** 3 + 2 ** 3 + 3 ** 3
```
```factor
{ 4 5 6 } { 1 2 3 } array-madness ! returns t since 4 2 ^ 5 2 ^ + 6 2 ^ + is greater than 1 3 ^ 2 3 ^ + 3 3 ^ +
```
Get your timer out. Are you ready? Ready, get set, GO!!! | games | def array_madness(a, b):
return sum(x * * 2 for x in a) > sum(x * * 3 for x in b)
| SpeedCode #2 - Array Madness | 56ff6a70e1a63ccdfa0001b1 | [
"Arrays",
"Puzzles"
] | https://www.codewars.com/kata/56ff6a70e1a63ccdfa0001b1 | 8 kyu |
# Task
Timed Reading is an educational tool used in many schools to improve and advance reading skills. A young elementary student has just finished his very first timed reading exercise. Unfortunately he's not a very good reader yet, so whenever he encountered a word longer than maxLength, he simply skipped it and read on.
Help the teacher figure out how many words the boy has read by calculating the number of words in the text he has read, no longer than maxLength.
Formally, a word is a substring consisting of English letters, such that characters to the left of the leftmost letter and to the right of the rightmost letter are not letters.
# Example
For `maxLength = 4` and `text = "The Fox asked the stork, 'How is the soup?'"`, the output should be `7`
The boy has read the following words: `"The", "Fox", "the", "How", "is", "the", "soup".`
# Input/Output
- `[input]` integer `maxLength`
A positive integer, the maximum length of the word the boy can read.
Constraints: `1 ≤ maxLength ≤ 10.`
- `[input]` string `text`
A non-empty string of English letters and punctuation marks.
- `[output]` an integer
The number of words the boy has read. | games | import re
def timed_reading(max_length, text):
return sum(len(i) <= max_length for i in re . findall('\w+', text))
| Simple Fun #40: Timed Reading | 588817db5fb13af14a000020 | [
"Puzzles"
] | https://www.codewars.com/kata/588817db5fb13af14a000020 | 7 kyu |
Given a Sudoku data structure with size `NxN, N > 0 and √N == integer`, write a method to validate if it has been filled out correctly.
The data structure is a multi-dimensional Array, i.e:
```
[
[7,8,4, 1,5,9, 3,2,6],
[5,3,9, 6,7,2, 8,4,1],
[6,1,2, 4,3,8, 7,5,9],
[9,2,8, 7,1,5, 4,6,3],
[3,5,7, 8,4,6, 1,9,2],
[4,6,1, 9,2,3, 5,8,7],
[8,7,6, 3,9,4, 2,1,5],
[2,4,3, 5,6,1, 9,7,8],
[1,9,5, 2,8,7, 6,3,4]
]
```
**Rules for validation**
- Data structure dimension: `NxN` where `N > 0` and `√N == integer`
- Rows may only contain integers: `1..N (N included)`
- Columns may only contain integers: `1..N (N included)`
- *'Little squares'* (`3x3` in example above) may also only contain integers: `1..N (N included)`
| games | import math
class Sudoku (object):
def __init__(self, board):
self . board = board
def is_valid(self):
if not isinstance(self . board, list):
return False
n = len(self . board)
rootN = int(round(math . sqrt(n)))
if rootN * rootN != n:
return False
def isValidRow(r): return (isinstance(r, list) and
len(r) == n and
all(map(lambda x: type(x) == int, r)))
if not all(map(isValidRow, self . board)):
return False
oneToN = set(range(1, n + 1))
def isOneToN(l): return set(l) == oneToN
tranpose = [[self . board[j][i] for i in range(n)] for j in range(n)]
squares = [[self . board[p + x][q + y] for x in range(rootN)
for y in range(rootN)]
for p in range(0, n, rootN)
for q in range(0, n, rootN)]
return (all(map(isOneToN, self . board)) and
all(map(isOneToN, tranpose)) and
all(map(isOneToN, squares)))
| Validate Sudoku with size `NxN` | 540afbe2dc9f615d5e000425 | [
"Arrays",
"Puzzles",
"Algorithms",
"Object-oriented Programming"
] | https://www.codewars.com/kata/540afbe2dc9f615d5e000425 | 4 kyu |
There are no explanations. You have to create the code that gives the following results in Python, Ruby, and Haskell:
```javascript
one_two_three(0) == [0, 0]
one_two_three(1) == [1, 1]
one_two_three(2) == [2, 11]
one_two_three(3) == [3, 111]
one_two_three(19) == [991, 1111111111111111111]
```
And it should give the following results in Javascript, Scala, D, Go, Rust, C, and Java:
```javascript
oneTwoThree(0) == ['0', '0']
oneTwoThree(1) == ['1', '1']
oneTwoThree(3) == ['3', '111']
oneTwoThree(19) == ['991', '1111111111111111111']
```
In C, the results are to be assigned to seperate pointers.
| games | def one_two_three(n):
x, y = divmod(n, 9)
return [* map(int, ("9" * x + str(y) * (y > 0), "1" * n))] if n else [0] * 2
| Begin your day with a challenge, but an easy one. | 5873b2010565844b9100026d | [
"Puzzles"
] | https://www.codewars.com/kata/5873b2010565844b9100026d | 6 kyu |
# Task
A rectangle with sides equal to even integers a and b is drawn on the Cartesian plane. Its center (the intersection point of its diagonals) coincides with the point (0, 0), but the sides of the rectangle are not parallel to the axes; instead, they are forming `45 degree` angles with the axes.
How many points with integer coordinates are located inside the given rectangle (including on its sides)?
# Example
For `a = 6 and b = 4`, the output should be `23`
The following picture illustrates the example, and the 23 points are marked green.
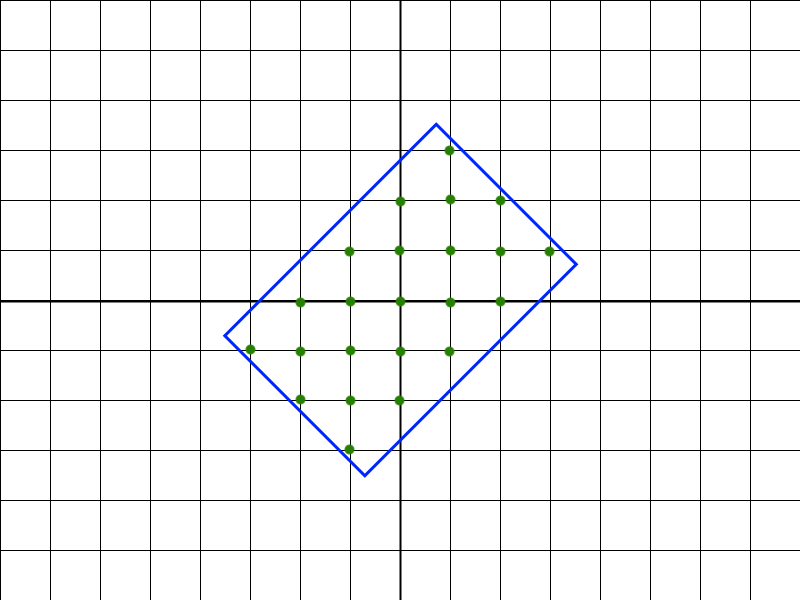
# Input/Output
- `[input]` integer `a`
A positive `even` integer.
Constraints: `2 ≤ a ≤ 10000`.
- `[input]` integer `b`
A positive `even` integer.
Constraints: `2 ≤ b ≤ 10000`.
- `[output]` an integer
The number of inner points with integer coordinates. | games | def rectangle_rotation(a, b):
a / /= 2 * * 0.5
b / /= 2 * * 0.5
r = (a + 1) * (b + 1) + a * b
return r + r % 2 - 1
| Simple Fun #27: Rectangle Rotation | 5886e082a836a691340000c3 | [
"Puzzles"
] | https://www.codewars.com/kata/5886e082a836a691340000c3 | 4 kyu |
Write a function done_or_not/`DoneOrNot` passing a board (list[list_lines]) as parameter. If the board is valid return 'Finished!', otherwise return 'Try again!'
Sudoku rules:
Complete the Sudoku puzzle so that each and every row, column, and region contains the numbers one through nine only once.
Rows:
<img src="http://www.sudokuessentials.com/images/Row.gif">
There are 9 rows in a traditional Sudoku puzzle. Every row must contain the numbers 1, 2, 3, 4, 5, 6, 7, 8, and 9. There may not be any duplicate numbers in any row. In other words, there can not be any rows that are identical.
In the illustration the numbers 5, 3, 1, and 2 are the "givens". They can not be changed. The remaining numbers in black are the numbers that you fill in to complete the row.
Columns:
<img src="http://www.sudokuessentials.com/images/Column.gif">
There are 9 columns in a traditional Sudoku puzzle. Like the Sudoku rule for rows, every column must also contain the numbers 1, 2, 3, 4, 5, 6, 7, 8, and 9. Again, there may not be any duplicate numbers in any column. Each column will be unique as a result.
In the illustration the numbers 7, 2, and 6 are the "givens". They can not be changed. You fill in the remaining numbers as shown in black to complete the column.
Regions
<img src="http://www.sudokuessentials.com/images/Region.gif">
A region is a 3x3 box like the one shown to the left. There are 9 regions in a traditional Sudoku puzzle.
Like the Sudoku requirements for rows and columns, every region must also contain the numbers 1, 2, 3, 4, 5, 6, 7, 8, and 9. Duplicate numbers are not permitted in any region. Each region will differ from the other regions.
In the illustration the numbers 1, 2, and 8 are the "givens". They can not be changed. Fill in the remaining numbers as shown in black to complete the region.
Valid board example:
<img src="http://upload.wikimedia.org/wikipedia/commons/thumb/3/31/Sudoku-by-L2G-20050714_solution.svg/364px-Sudoku-by-L2G-20050714_solution.svg.png">
For those who don't know the game, here are some information about rules and how to play Sudoku: http://en.wikipedia.org/wiki/Sudoku and http://www.sudokuessentials.com/ | games | import numpy as np
def done_or_not(aboard): # board[i][j]
board = np . array(aboard)
rows = [board[i, :] for i in range(9)]
cols = [board[:, j] for j in range(9)]
sqrs = [board[i: i + 3, j: j + 3]. flatten() for i in [0, 3, 6]
for j in [0, 3, 6]]
for view in np . vstack((rows, cols, sqrs)):
if len(np . unique(view)) != 9:
return 'Try again!'
return 'Finished!'
| Did I Finish my Sudoku? | 53db96041f1a7d32dc0004d2 | [
"Mathematics",
"Puzzles",
"Games",
"Game Solvers"
] | https://www.codewars.com/kata/53db96041f1a7d32dc0004d2 | 5 kyu |
Implement a function that adds two numbers together and returns their sum in binary. The conversion can be done before, or after the addition.
The binary number returned should be a string.
**Examples:(Input1, Input2 --> Output (explanation)))**
```
1, 1 --> "10" (1 + 1 = 2 in decimal or 10 in binary)
5, 9 --> "1110" (5 + 9 = 14 in decimal or 1110 in binary)
```
| reference | def add_binary(a, b):
return bin(a + b)[2:]
| Binary Addition | 551f37452ff852b7bd000139 | [
"Binary",
"Fundamentals"
] | https://www.codewars.com/kata/551f37452ff852b7bd000139 | 7 kyu |
I always thought that my old friend John was rather richer than he looked, but I never knew exactly how much money he actually had. One day (as I was plying him with questions) he said:
* "Imagine I have between `m` and `n` Zloty..." (or did he say Quetzal? I can't remember!)
* "If I were to buy **9** cars costing `c` each, I'd only have 1 Zloty (or was it Meticals?) left."
* "And if I were to buy **7** boats at `b` each, I'd only have 2 Ringglets (or was it Zloty?) left."
Could you tell me in each possible case:
1. how much money `f` he could possibly have ?
2. the cost `c` of a car?
3. the cost `b` of a boat?
So, I will have a better idea about his fortune. Note that if `m-n` is big enough, you might have a lot of possible answers.
Each answer should be given as `["M: f", "B: b", "C: c"]` and all the answers as `[ ["M: f", "B: b", "C: c"], ... ]`. "M" stands for money, "B" for boats, "C" for cars.
**Note:** `m, n, f, b, c` are positive integers, where `0 <= m <= n` or `m >= n >= 0`. `m` and `n` are inclusive.
#### Examples:
```
howmuch(1, 100) => [["M: 37", "B: 5", "C: 4"], ["M: 100", "B: 14", "C: 11"]]
howmuch(1000, 1100) => [["M: 1045", "B: 149", "C: 116"]]
howmuch(10000, 9950) => [["M: 9991", "B: 1427", "C: 1110"]]
howmuch(0, 200) => [["M: 37", "B: 5", "C: 4"], ["M: 100", "B: 14", "C: 11"], ["M: 163", "B: 23", "C: 18"]]
```
Explanation of the results for `howmuch(1, 100)`:
* In the first answer his possible fortune is **37**:
* so he can buy 7 boats each worth 5: `37 - 7 * 5 = 2`
* or he can buy 9 cars worth 4 each: `37 - 9 * 4 = 1`
* The second possible answer is **100**:
* he can buy 7 boats each worth 14: `100 - 7 * 14 = 2`
* or he can buy 9 cars worth 11: `100 - 9 * 11 = 1`
#### Note
See "Sample Tests" to know the format of the return.
| reference | def howmuch(m, n):
return [['M: %d' % i, 'B: %d' % (i / 7), 'C: %d' % (i / 9)] for i in range(min(m, n), max(m, n) + 1) if i % 7 == 2 and i % 9 == 1]
| How Much? | 55b4d87a3766d9873a0000d4 | [
"Fundamentals"
] | https://www.codewars.com/kata/55b4d87a3766d9873a0000d4 | 6 kyu |
----
Sum of Pairs
----
Given a list of integers and a single sum value, return the first two values (parse from the left please) in order of appearance that add up to form the sum.
If there are two or more pairs with the required sum, the pair whose second element has the smallest index is the solution.
```python
sum_pairs([11, 3, 7, 5], 10)
# ^--^ 3 + 7 = 10
== [3, 7]
sum_pairs([4, 3, 2, 3, 4], 6)
# ^-----^ 4 + 2 = 6, indices: 0, 2 *
# ^-----^ 3 + 3 = 6, indices: 1, 3
# ^-----^ 2 + 4 = 6, indices: 2, 4
# * the correct answer is the pair whose second value has the smallest index
== [4, 2]
sum_pairs([0, 0, -2, 3], 2)
# there are no pairs of values that can be added to produce 2.
== None/nil/undefined (Based on the language)
sum_pairs([10, 5, 2, 3, 7, 5], 10)
# ^-----------^ 5 + 5 = 10, indices: 1, 5
# ^--^ 3 + 7 = 10, indices: 3, 4 *
# * the correct answer is the pair whose second value has the smallest index
== [3, 7]
```
Negative numbers and duplicate numbers can and will appear.
__NOTE:__ There will also be lists tested of lengths upwards of 10,000,000 elements. Be sure your code doesn't time out. | reference | def sum_pairs(lst, s):
cache = set()
for i in lst:
if s - i in cache:
return [s - i, i]
cache . add(i)
| Sum of Pairs | 54d81488b981293527000c8f | [
"Memoization",
"Fundamentals",
"Performance"
] | https://www.codewars.com/kata/54d81488b981293527000c8f | 5 kyu |
Check to see if a string has the same amount of 'x's and 'o's. The method must return a boolean and be case insensitive. The string can contain any char.
Examples input/output:
```
XO("ooxx") => true
XO("xooxx") => false
XO("ooxXm") => true
XO("zpzpzpp") => true // when no 'x' and 'o' is present should return true
XO("zzoo") => false
``` | reference | def xo(s):
s = s . lower()
return s . count('x') == s . count('o')
| Exes and Ohs | 55908aad6620c066bc00002a | [
"Fundamentals"
] | https://www.codewars.com/kata/55908aad6620c066bc00002a | 7 kyu |
# Task
A little boy is studying arithmetics. He has just learned how to add two integers, written one below another, column by column. But he always forgets about the important part - carrying.
Given two integers, find the result which the little boy will get.
# Example
For param1 = 456 and param2 = 1734, the output should be `1180`
```
456
1734
+ ____
1180
```
The little boy goes from right to left:
6 + 4 = 10 but the little boy forgets about 1 and just writes down 0 in the last column
5 + 3 = 8
4 + 7 = 11 but the little boy forgets about the leading 1 and just writes down 1 under 4 and 7.
There is no digit in the first number corresponding to the leading digit of the second one, so the little boy imagines that 0 is written before 456. Thus, he gets 0 + 1 = 1.
# Input/Output
- `[input]` integer `a`
A non-negative integer.
Constraints: 0 ≤ a ≤ 99999.
- `[input]` integer `b`
A non-negative integer.
Constraints: 0 ≤ b ≤ 59999.
- `[output]` an integer
The result that the little boy will get. | games | def addition_without_carrying(a, b):
r = 0
m = 1
while a + b:
r += (a + b) % 10 * m
m *= 10
a / /= 10
b / /= 10
return r
| Simple Fun #15: Addition without Carrying | 588468f3b3d02cf67b0005cd | [
"Puzzles"
] | https://www.codewars.com/kata/588468f3b3d02cf67b0005cd | 6 kyu |
Implement the function unique_in_order which takes as argument a sequence and returns a list of items without any elements with the same value next to each other and preserving the original order of elements.
For example:
```cpp
uniqueInOrder("AAAABBBCCDAABBB") == {'A', 'B', 'C', 'D', 'A', 'B'}
uniqueInOrder("ABBCcAD") == {'A', 'B', 'C', 'c', 'A', 'D'}
uniqueInOrder([1,2,2,3,3]) == {1,2,3}
```
```javascript
uniqueInOrder('AAAABBBCCDAABBB') == ['A', 'B', 'C', 'D', 'A', 'B']
uniqueInOrder('ABBCcAD') == ['A', 'B', 'C', 'c', 'A', 'D']
uniqueInOrder([1,2,2,3,3]) == [1,2,3]
```
```python
unique_in_order('AAAABBBCCDAABBB') == ['A', 'B', 'C', 'D', 'A', 'B']
unique_in_order('ABBCcAD') == ['A', 'B', 'C', 'c', 'A', 'D']
unique_in_order([1, 2, 2, 3, 3]) == [1, 2, 3]
unique_in_order((1, 2, 2, 3, 3)) == [1, 2, 3]
```
```ruby
unique_in_order('AAAABBBCCDAABBB') == ['A', 'B', 'C', 'D', 'A', 'B']
unique_in_order('ABBCcAD') == ['A', 'B', 'C', 'c', 'A', 'D']
unique_in_order([1,2,2,3,3]) == [1,2,3]
```
```haskell
uniqueInOrder "AAAABBBCCDAABBB" == "ABCDAB"
uniqueInOrder "ABBCcAD" == "ABCcAD"
uniqueInOrder [1,2,2,3,3] == [1,2,3]
```
```crystal
unique_in_order("AAAABBBCCDAABBB") == ['A', 'B', 'C', 'D', 'A', 'B']
unique_in_order("ABBCcAD") == ['A', 'B', 'C', 'c', 'A', 'D']
unique_in_order([1,2,2,3,3]) == [1,2,3]
```
```scala
uniqueInOrder("AAAABBBCCDAABBB") == List('A', 'B', 'C', 'D', 'A', 'B')
uniqueInOrder("ABBCcAD") == List('A', 'B', 'C', 'c', 'A', 'D')
uniqueInOrder(List(1, 2, 2, 3, 3)) == List(1, 2, 3)
```
| reference | def unique_in_order(iterable):
result = []
prev = None
for char in iterable[0:]:
if char != prev:
result . append(char)
prev = char
return result
| Unique In Order | 54e6533c92449cc251001667 | [
"Algorithms",
"Fundamentals"
] | https://www.codewars.com/kata/54e6533c92449cc251001667 | 6 kyu |
# Task
A media access control address (MAC address) is a unique identifier assigned to network interfaces for communications on the physical network segment.
The standard (IEEE 802) format for printing MAC-48 addresses in human-friendly form is six groups of two hexadecimal digits (0 to 9 or A to F), separated by hyphens (e.g. 01-23-45-67-89-AB).
# Example
For `inputString = "00-1B-63-84-45-E6"`, the output should be `true`;
For `inputString = "Z1-1B-63-84-45-E6"`, the output should be `false`;
For `inputString = "not a MAC-48 address"`, the output should be `false`.
# Input/Output
- `[input]` string `inputString`
- `[output]` a boolean value
`true` if inputString corresponds to MAC-48 address naming rules, `false` otherwise. | games | import re
def is_mac_48_address(address):
return bool(re . match("^([0-9A-F]{2}[-]){5}([0-9A-F]{2})$", address . upper()))
| Simple Fun #29: Is MAC48 Address? | 5886faac54a7111c21000072 | [
"Puzzles"
] | https://www.codewars.com/kata/5886faac54a7111c21000072 | 6 kyu |
# MOD 256 without the MOD operator
The MOD-operator % (aka mod/modulus/remainder):
```
Returns the remainder of a division operation.
The sign of the result is the same as the sign of the first operand.
(Different behavior in Python!)
```
The short unbelievable mad story for this kata:<br>
I wrote a program and needed the remainder of the division by 256. And then it happened: The "5"/"%"-Key did not react. It must be broken! So I needed a way to:
```
Calculate the remainder of the division by 256 without the %-operator.
```
Also here some examples:
```
Input 254 -> Result 254
Input 256 -> Result 0
Input 258 -> Result 2
Input -258 -> Result -2 (in Python: Result: 254!)
```
It is always expected the behavior of the MOD-Operator of the language!
The input number will always between -10000 and 10000.
For some languages the %-operator will be blocked. If it is not blocked and you know how to block it, tell me and I will include it.
For all, who say, this would be a duplicate: No, this is no duplicate! There are two katas, in that you have to write a general method for MOD without %. But this kata is only for MOD 256. And so you can create also other specialized solutions. ;-)
Of course you can use the digit "5" in your solution. :-)
I'm very curious for your solutions and the way you solve it. I found several interesting "funny" ways.
Have fun coding it and please don't forget to vote and rank this kata! :-)
I have also created other katas. Take a look if you enjoyed this kata! | games | def mod256_without_mod(number):
return number & 255
| MOD 256 without the MOD operator | 581e1d083a4820eb4f00004f | [
"Algorithms",
"Fundamentals",
"Logic",
"Mathematics",
"Restricted",
"Puzzles"
] | https://www.codewars.com/kata/581e1d083a4820eb4f00004f | 7 kyu |
<font>You've been going to the gym for some time now and recently you started taking care of your nutrition as well. You want to gain some weight but who wants to bother counting calories every day. It said somewhere that protein is the foundation of building muscle, so let's try to calculate the total amount of calories and proteins we take in.</font>
<h2>Task:</h2>
<font>Given an array of meals where each element is a string in the form <code>'300g turkey, 300g potatoes, 100g cucumber'</code> find out how many proteins and calories you consumed. You like to keep things simple so all values will be expressed in grams. In case you didn't know every gram of protein and carbohydrate has 4 calories, while 1 gram of fat provides 9 calories. <br>An object <b><i>food</i></b> (in Ruby <b><i>$food</i></b> ) is preloaded for you that contains the information about the given food <b>per 100 grams</b>:</font>
```javascript
var food = {
chicken: [20, 5, 10], //per 100g chicken has 20g of protein, 5 grams of carbohydrates and 10 grams of fat.
eggs: [10, 5, 15], //protein:10g , carbs:5g , fats: 15g
salmon: [27, 0, 10],
beans: [8, 25, 0],
bananas: [1, 23, 0],
...
...
}
```
```ruby
$food = {
"chicken" => [20, 5, 10], # per 100g chicken has 20g of protein, 5 grams of carbohydrates and 10 grams of fat.
"eggs" => [10, 5, 15], # protein:10g , carbs:5g , fats: 15g
"salmon" => [27, 0, 10],
"beans" => [8, 25, 0],
"bananas" => [1, 23, 0],
...
...
}
```
```python
food = {
"chicken": [20, 5, 10], # per 100g chicken has 20g of protein, 5 grams of carbohydrates and 10 grams of fat.
"eggs": [10, 5, 15], # protein:10g , carbs:5g , fats: 15g
"salmon": [27, 0, 10],
"beans": [8, 25, 0],
"bananas": [1, 23, 0],
...
...
}
```
Round your results to 2 decimal places and return a string in the form <code>"Total proteins: n grams, Total calories: n"</code>.<br>
Delete all trailing zeros on every float and remove trailing point if the result is an integer.
<font>Note: <b>No invalid input testing.</b></font>
<h3><font color="#25B6CC">Have fun!</font></h2>
```javascript
_....----"""----...._
.-' o o o o '-.
/ o o o o \
/ o o o o o \
_| o o o o o o |_
/ `''-----.................-----''` \
\___________________________________/
\~`-`.__.`-~`._.~`-`~.-~.__.~`-`/
\ /
`-._______________________.-'
//lonely burger passing by
``` | games | import re
PATTERN = re . compile(r'(\d+)g (\w+)')
CALORIES_PER_100g = {typ: sum(
m * cal for m, cal in zip(masses, [4, 4, 9])) for typ, masses in food . items()}
def formatAns(n): return str(round(n, 2)) if n % 1 else str(int(n))
def bulk(arr):
prots, cals = 0, 0
for plate in arr:
for mass, typ in PATTERN . findall(plate):
prots += int(mass) * food[typ][0] / 100
cals += int(mass) * CALORIES_PER_100g[typ] / 100
return 'Total proteins: {} grams, Total calories: {}' . format(formatAns(prots), formatAns(cals))
| Bulk up! | 5863f1c8b359c4dd4e000001 | [
"Puzzles"
] | https://www.codewars.com/kata/5863f1c8b359c4dd4e000001 | 5 kyu |
# Task
You have a rectangular white board with some black cells. The black cells create a connected black figure, i.e. it is possible to get from any black cell to any other one through connected adjacent (sharing a common side) black cells.
Find the perimeter of the black figure assuming that a single cell has unit length.
# Example
For
```
matrix = [[false, true, true ],
[true, true, false],
[true, false, false]]```
the output should be `12`.
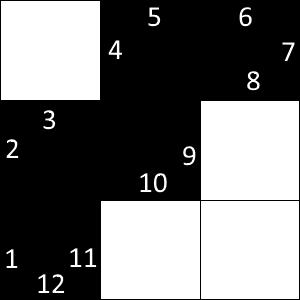
For
```
matrix = [[true, true, true],
[true, false, true],
[true, true, true]]```
the output should be `16`.
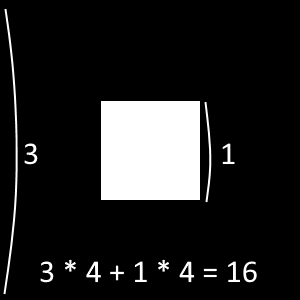
# Input/Output
- `[input]` 2D boolean array `matrix`
A matrix of booleans representing the rectangular board where true means a black cell and false means a white one.
Constraints:
`2 ≤ matrix.length ≤ 10,`
`2 ≤ matrix[0].length ≤ 10.`
- `[output]` an integer
| games | import numpy as np
from scipy import signal
k = np . array([[0, - 1, 0], [- 1, 4, - 1], [0, - 1, 0]])
def polygon_perimeter(m):
return signal . convolve2d(np . array(m, int), k, mode='same'). clip(0). sum()
| Simple Fun #85: Polygon Perimeter | 58953a5a41c97914d7000070 | [
"Puzzles"
] | https://www.codewars.com/kata/58953a5a41c97914d7000070 | 6 kyu |
A new task for you!
* You have to create a method, that corrects a given time string.
* There was a problem in addition, so many of the time strings are broken.
* Time is formatted using the 24-hour clock, so from `00:00:00` to `23:59:59`.
## Examples
```
"09:10:01" -> "09:10:01"
"11:70:10" -> "12:10:10"
"19:99:99" -> "20:40:39"
"24:01:01" -> "00:01:01"
```
~~~if-not:rust
If the input-string is null or empty return exactly this value! (empty string for C++)
If the time-string-format is invalid, return null. (empty string for C++)
~~~
~~~if:rust
* If the input string is empty or invalid, return `None`.
~~~
Have fun coding it and please don't forget to vote and rank this kata! :-)
I have created other katas. Have a look if you like coding and challenges. | reference | def time_correct(t):
if not t:
return t
try:
h, m, s = map(int, t . split(':'))
if s >= 60:
s -= 60
m += 1
if m >= 60:
m -= 60
h += 1
return '%02d:%02d:%02d' % (h % 24, m, s)
except:
pass
| Correct the time-string | 57873ab5e55533a2890000c7 | [
"Parsing",
"Strings",
"Fundamentals"
] | https://www.codewars.com/kata/57873ab5e55533a2890000c7 | 7 kyu |
#### Task:
Your job here is to implement a method, `approx_root` in Ruby/Python/Crystal and `approxRoot` in JavaScript/CoffeeScript, that takes one argument, `n`, and returns the approximate square root of that number, rounded to the nearest hundredth and computed in the following manner.
1. Start with `n = 213` (as an example).
2. To approximate the square root of n, we will first find the greatest perfect square that is below or equal to `n`. (In this example, that would be 196, or 14 squared.) We will call the square root of this number (which means sqrt 196, or 14) `base`.
3. Then, we will take the lowest perfect square that is greater than or equal to `n`. (In this example, that would be 225, or 15 squared.)
4. Next, subtract 196 (greatest perfect square less than or equal to `n`) from `n`. (213 - 196 = **17**) We will call this value `diff_gn`.
5. Find the difference between the lowest perfect square greater than or equal to `n` and the greatest perfect square less than or equal to `n`. (225 – 196 = **29**) We will call this value `diff_lg`.
6. Your final answer is `base` + (`diff_gn` / `diff_lg`). In this example: 14 + (17 / 29) which is 14.59, rounded to the nearest hundredth.
Just to clarify, if the input is a perfect square itself, you should return the exact square of the input.
In case you are curious, the approximation (computed like above) for 213 rounded to four decimal places is 14.5862. The actual square root of 213 is 14.5945.
Inputs will always be positive whole numbers. If you are having trouble understanding it, let me know with a comment, or take a look at the second group of the example cases.
#### Some examples:
```ruby
approx_root(400) #=> 20
approx_root(401) #=>
# smallest perfect square above 401 is 441 or 21 squared
# greatest perfect square below 401 is 400 or 20 squared
# difference between 441 and 400 is 41
# difference between 401 and 400 is 1
# answer is 20 + (1 / 41) which becomes 20.02, rounded to the nearest hundredth
# final answer = 20.02.
approx_root(2) #=>
# smallest perfect square above 2 is 4 or 2 squared
# greatest perfect square below 2 is 1 or 1 squared
# difference between 4 and 1 is 3
# difference between 2 and 1 is 1
# answer is 1 + (1 / 3), which becomes 1.33, rounded to the nearest hundredth
# final answer = 1.33.
# Math.sqrt() isn't disabled.
```
```crystal
approx_root(400) #=> 20
approx_root(401) #=>
# smallest perfect square above 401 is 441 or 21 squared
# greatest perfect square below 401 is 400 or 20 squared
# difference between 441 and 400 is 41
# difference between 401 and 400 is 1
# answer is 20 + (1 / 41) which becomes 20.02, rounded to the nearest hundredth
# final answer = 20.02.
approx_root(2) #=>
# smallest perfect square above 2 is 4 or 2 squared
# greatest perfect square below 2 is 1 or 1 squared
# difference between 4 and 1 is 3
# difference between 2 and 1 is 1
# answer is 1 + (1 / 3), which becomes 1.33, rounded to the nearest hundredth
# final answer = 1.33.
# Math.sqrt() isn't disabled.
```
```javascript
approx_root(400) #=> 20
approx_root(401) #=>
# smallest perfect square above 401 is 441 or 21 squared
# greatest perfect square below 401 is 400 or 20 squared
# difference between 441 and 400 is 41
# difference between 401 and 400 is 1
# answer is 20 + (1 / 41) which becomes 20.02, rounded to the nearest hundredth
# final answer = 20.02.
approx_root(2) #=>
# smallest perfect square above 2 is 4 or 2 squared
# greatest perfect square below 2 is 1 or 1 squared
# difference between 4 and 1 is 3
# difference between 2 and 1 is 1
# answer is 1 + (1 / 3), which becomes 1.33, rounded to the nearest hundredth
# final answer = 1.33.
# Math.sqrt() isn't disabled.
```
```python
approx_root(400) #=> 20
approx_root(401) #=>
# smallest perfect square above 401 is 441 or 21 squared
# greatest perfect square below 401 is 400 or 20 squared
# difference between 441 and 400 is 41
# difference between 401 and 400 is 1
# answer is 20 + (1 / 41) which becomes 20.02, rounded to the nearest hundredth
# final answer = 20.02.
approx_root(2) #=>
# smallest perfect square above 2 is 4 or 2 squared
# greatest perfect square below 2 is 1 or 1 squared
# difference between 4 and 1 is 3
# difference between 2 and 1 is 1
# answer is 1 + (1 / 3), which becomes 1.33, rounded to the nearest hundredth
# final answer = 1.33.
# math.sqrt() isn't disabled.
```
```coffeescript
approx_root(400) #=> 20
approx_root(401) #=>
# smallest perfect square above 401 is 441 or 21 squared
# greatest perfect square below 401 is 400 or 20 squared
# difference between 441 and 400 is 41
# difference between 401 and 400 is 1
# answer is 20 + (1 / 41) which becomes 20.02, rounded to the nearest hundredth
# final answer = 20.02.
approx_root(2) #=>
# smallest perfect square above 2 is 4 or 2 squared
# greatest perfect square below 2 is 1 or 1 squared
# difference between 4 and 1 is 3
# difference between 2 and 1 is 1
# answer is 1 + (1 / 3), which becomes 1.33, rounded to the nearest hundredth
# final answer = 1.33.
# Math.sqrt() isn't disabled.
```
Also check out my other creations — [Square Roots: Simplifying/Desimplifying](https://www.codewars.com/kata/square-roots-simplify-slash-desimplify/), [Square and Cubic Factors](https://www.codewars.com/kata/square-and-cubic-factors), [Keep the Order](https://www.codewars.com/kata/keep-the-order), [Naming Files](https://www.codewars.com/kata/naming-files), [Elections: Weighted Average](https://www.codewars.com/kata/elections-weighted-average), [Identify Case](https://www.codewars.com/kata/identify-case), [Split Without Loss](https://www.codewars.com/kata/split-without-loss), [Adding Fractions](https://www.codewars.com/kata/adding-fractions),
[Random Integers](https://www.codewars.com/kata/random-integers), [Implement String#transpose](https://www.codewars.com/kata/implement-string-number-transpose), [Implement Array#transpose!](https://www.codewars.com/kata/implement-array-number-transpose), [Arrays and Procs #1](https://www.codewars.com/kata/arrays-and-procs-number-1), and [Arrays and Procs #2](https://www.codewars.com/kata/arrays-and-procs-number-2).
If you notice any issues or have any suggestions/comments whatsoever, please don't hesitate to mark an issue or just comment. Thanks!
| reference | def approx_root(n):
base = int(n * * 0.5)
return round(base + (n - base * * 2) / ((base + 1) * * 2 - base * * 2), 2)
| Square Roots: Approximation | 58475cce273e5560f40000fa | [
"Fundamentals"
] | https://www.codewars.com/kata/58475cce273e5560f40000fa | 7 kyu |
A natural number is called **k-prime** if it has exactly k prime factors, counted with multiplicity. A natural number is thus prime if and only if it is 1-prime.
```
Examples:
k = 2 -> 4, 6, 9, 10, 14, 15, 21, 22, …
k = 3 -> 8, 12, 18, 20, 27, 28, 30, …
k = 5 -> 32, 48, 72, 80, 108, 112, …
```
#### Task:
Given an integer `k` and a list `arr` of positive integers the function `consec_kprimes (or its variants in other languages)` returns
how many times in the sequence `arr` numbers come up twice in a row with exactly `k` prime factors?
#### Examples:
```
arr = [10005, 10030, 10026, 10008, 10016, 10028, 10004]
consec_kprimes(4, arr) => 3 because 10005 and 10030 are consecutive 4-primes, 10030 and 10026 too as well as 10028 and 10004 but 10008 and 10016 are 6-primes.
consec_kprimes(4, [10175, 10185, 10180, 10197]) => 3 because 10175-10185 and 10185- 10180 and 10180-10197 are all consecutive 4-primes.
```
#### Note:
It could be interesting to begin with:
<https://www.codewars.com/kata/k-primes>
| reference | def primes(n):
primefac = []
d = 2
while d * d <= n:
while (n % d) == 0:
primefac . append(d)
n / /= d
d += 1
if n > 1:
primefac . append(n)
return len(primefac)
def consec_kprimes(k, arr):
return sum(primes(arr[i]) == primes(arr[i + 1]) == k for i in range(len(arr) - 1))
| Consecutive k-Primes | 573182c405d14db0da00064e | [
"Fundamentals"
] | https://www.codewars.com/kata/573182c405d14db0da00064e | 5 kyu |
For this kata, you are given three points ```(x1,y1,z1)```, ```(x2,y2,z2)```, and ```(x3,y3,z3)``` that lie on a straight line in 3-dimensional space.
You have to figure out which point lies in between the other two.
Your function should return 1, 2, or 3 to indicate which point is the in-between one. | algorithms | def middle_point(x1, y1, z1, x2, y2, z2, x3, y3, z3):
return sorted(((x1, y1, z1, 1), (x2, y2, z2, 2), (x3, y3, z3, 3)))[1][3]
| Find the in-between point | 58a672d6426bf38be4000057 | [
"Fundamentals",
"Geometry",
"Mathematics",
"Algorithms"
] | https://www.codewars.com/kata/58a672d6426bf38be4000057 | 6 kyu |
#### Task:
Your job here is to implement a function `factors`, which takes a number `n`, and outputs an array of arrays comprised of two
parts, `sq` and `cb`. The part `sq` will contain all the numbers that, when squared, yield a number which is a factor of `n`,
while the `cb` part will contain all the numbers that, when cubed, yield a number which is a factor of `n`. Discard all `1`s
from both arrays.
Both `sq` and `cb` should be sorted in ascending order.
#### What it looks like:
```ruby
factors(Integer) #=> [
sq (all the numbers that can be squared to give a factor of n) : Array,
cb (all the numbers that can be cubed to give a factor of n) : Array
]
```
```crystal
factors(Int32) #=> [
sq (all the numbers that can be squared to give a factor of n) : Array(Int32),
cb (all the numbers that can be cubed to give a factor of n) : Array(Int32)
]
```
```python
factors(int) #=> [
sq (all the numbers that can be squared to give a factor of n) : list,
cb (all the numbers that can be cubed to give a factor of n) : list
]
```
```javascript
factors(Number) #=> [
sq (all the numbers that can be squared to give a factor of n) : Array,
cb (all the numbers that can be cubed to give a factor of n) : Array
]
```
```coffeescript
factors(Number) #=> [
sq (all the numbers that can be squared to give a factor of n) : Array,
cb (all the numbers that can be cubed to give a factor of n) : Array
]
```
#### Some examples:
```ruby
factors(1) #=> [[], []]
# ones are discarded from outputs
factors(4) #=> [[2], []]
# 2 squared is 4; 4 is a factor of 4
factors(16) #=> [[2, 4], [2]]
# 2 squared is 4; 4 is a factor of 16
# 4 squared is 16; 16 is a factor of 16
# 2 cubed is 8; 8 is a factor of 16
factors(81) #=> [[3, 9], [3]]
# 3 squared is 9; 9 is a factor of 81
# 9 squared is 81; 81 is a factor of 81
# 3 cubed is 27; 27 is a factor of 81
```
Also check out my other creations — [Keep the Order](https://www.codewars.com/kata/keep-the-order), [Naming Files](https://www.codewars.com/kata/naming-files), [Elections: Weighted Average](https://www.codewars.com/kata/elections-weighted-average), [Identify Case](https://www.codewars.com/kata/identify-case), [Split Without Loss](https://www.codewars.com/kata/split-without-loss), [Adding Fractions](https://www.codewars.com/kata/adding-fractions),
[Random Integers](https://www.codewars.com/kata/random-integers), [Implement String#transpose](https://www.codewars.com/kata/implement-string-number-transpose), [Implement Array#transpose!](https://www.codewars.com/kata/implement-array-number-transpose), [Arrays and Procs #1](https://www.codewars.com/kata/arrays-and-procs-number-1), and [Arrays and Procs #2](https://www.codewars.com/kata/arrays-and-procs-number-2).
If you notice any issues or have any suggestions/comments whatsoever, please don't hesitate to mark an issue or just comment. Thanks! | reference | def factors(n):
sq = [a for a in range(2, n + 1) if not n % (a * * 2)]
cb = [b for b in range(2, n + 1) if not n % (b * * 3)]
return [sq, cb]
| Square and Cubic Factors | 582b0d73c190130d550000c6 | [
"Fundamentals"
] | https://www.codewars.com/kata/582b0d73c190130d550000c6 | 7 kyu |
### What is simplifying a square root?
If you have a number, like 80, for example, you would start by finding the greatest perfect square divisible by 80. In this case, that's 16. Find the square root of 16, and multiply it by 80 / 16. Answer = 4 √5.
##### The above example:
```math
\sqrt{80} = \sqrt{5\times 16} = \sqrt{5} \times \sqrt{16} = \sqrt{5} \times 4 = 4\sqrt{5}
```
### Task:
Your job is to write two functions, `simplify`, and `desimplify`, that simplify and desimplify square roots, respectively. (Desimplify isn't a word, but I couldn't come up with a better way to put it.) `simplify` will take an integer and return a string like "x sqrt y", and `desimplify` will take a string like "x sqrt y" and return an integer. For `simplify`, if a square root cannot be simplified, return "sqrt y".
_Do not modify the input._
### Some examples:
```ruby
simplify(1) #=> "1"
simplify(2) #=> "sqrt 2"
simplify(3) #=> "sqrt 3"
simplify(8) #=> "2 sqrt 2"
simplify(15) #=> "sqrt 15"
simplify(16) #=> "4"
simplify(18) #=> "3 sqrt 2"
simplify(20) #=> "2 sqrt 5"
simplify(24) #=> "2 sqrt 6"
simplify(32) #=> "4 sqrt 2"
desimplify("1") #=> 1
desimplify("sqrt 2") #=> 2
desimplify("sqrt 3") #=> 3
desimplify("2 sqrt 2") #=> 8
desimplify("sqrt 15") #=> 15
desimplify("4") #=> 16
desimplify("3 sqrt 2") #=> 18
desimplify("2 sqrt 5") #=> 20
desimplify("2 sqrt 6") #=> 24
desimplify("4 sqrt 2") #=> 32
```
```python
simplify(1) #=> "1"
simplify(2) #=> "sqrt 2"
simplify(3) #=> "sqrt 3"
simplify(8) #=> "2 sqrt 2"
simplify(15) #=> "sqrt 15"
simplify(16) #=> "4"
simplify(18) #=> "3 sqrt 2"
simplify(20) #=> "2 sqrt 5"
simplify(24) #=> "2 sqrt 6"
simplify(32) #=> "4 sqrt 2"
desimplify("1") #=> 1
desimplify("sqrt 2") #=> 2
desimplify("sqrt 3") #=> 3
desimplify("2 sqrt 2") #=> 8
desimplify("sqrt 15") #=> 15
desimplify("4") #=> 16
desimplify("3 sqrt 2") #=> 18
desimplify("2 sqrt 5") #=> 20
desimplify("2 sqrt 6") #=> 24
desimplify("4 sqrt 2") #=> 32
```
```javascript
simplify(1) //=> "1"
simplify(2) //=> "sqrt 2"
simplify(3) //=> "sqrt 3"
simplify(8) //=> "2 sqrt 2"
simplify(15) //=> "sqrt 15"
simplify(16) //=> "4"
simplify(18) //=> "3 sqrt 2"
simplify(20) //=> "2 sqrt 5"
simplify(24) //=> "2 sqrt 6"
simplify(32) //=> "4 sqrt 2"
desimplify("1") //=> 1
desimplify("sqrt 2") //=> 2
desimplify("sqrt 3") //=> 3
desimplify("2 sqrt 2") //=> 8
desimplify("sqrt 15") //=> 15
desimplify("4") //=> 16
desimplify("3 sqrt 2") //=> 18
desimplify("2 sqrt 5") //=> 20
desimplify("2 sqrt 6") //=> 24
desimplify("4 sqrt 2") //=> 32
```
```coffeescript
simplify(1) #=> "1"
simplify(2) #=> "sqrt 2"
simplify(3) #=> "sqrt 3"
simplify(8) #=> "2 sqrt 2"
simplify(15) #=> "sqrt 15"
simplify(16) #=> "4"
simplify(18) #=> "3 sqrt 2"
simplify(20) #=> "2 sqrt 5"
simplify(24) #=> "2 sqrt 6"
simplify(32) #=> "4 sqrt 2"
desimplify("1") #=> 1
desimplify("sqrt 2") #=> 2
desimplify("sqrt 3") #=> 3
desimplify("2 sqrt 2") #=> 8
desimplify("sqrt 15") #=> 15
desimplify("4") #=> 16
desimplify("3 sqrt 2") #=> 18
desimplify("2 sqrt 5") #=> 20
desimplify("2 sqrt 6") #=> 24
desimplify("4 sqrt 2") #=> 32
```
Also check out my other creations — [Square Roots: Approximation](https://www.codewars.com/kata/square-roots-approximation), [Square and Cubic Factors](https://www.codewars.com/kata/square-and-cubic-factors), [Keep the Order](https://www.codewars.com/kata/keep-the-order), [Naming Files](https://www.codewars.com/kata/naming-files), [Elections: Weighted Average](https://www.codewars.com/kata/elections-weighted-average), [Identify Case](https://www.codewars.com/kata/identify-case), [Split Without Loss](https://www.codewars.com/kata/split-without-loss), [Adding Fractions](https://www.codewars.com/kata/adding-fractions),
[Random Integers](https://www.codewars.com/kata/random-integers), [Implement String#transpose](https://www.codewars.com/kata/implement-string-number-transpose), [Implement Array#transpose!](https://www.codewars.com/kata/implement-array-number-transpose), [Arrays and Procs #1](https://www.codewars.com/kata/arrays-and-procs-number-1), and [Arrays and Procs #2](https://www.codewars.com/kata/arrays-and-procs-number-2). | reference | def simplify(n):
for d in range(int(n * * .5), 0, - 1):
if not n % d * * 2:
break
if d * d == n:
return '%d' % d
elif d == 1:
return 'sqrt %d' % n
else:
return '%d sqrt %d' % (d, n / / d * * 2)
def desimplify(s):
x, _, y = s . partition('sqrt')
return int(x or '1') * * 2 * int(y or '1')
| Square Roots: Simplify/Desimplify | 5850e85c6e997bddd300005d | [
"Fundamentals"
] | https://www.codewars.com/kata/5850e85c6e997bddd300005d | 6 kyu |
Say hello!
Write a function to greet a person. Function will take name as input and greet the person by saying hello.
Return null/nil/None if input is empty string or null/nil/None.
Example:
```javascript
greet("Niks") === "hello Niks!";
greet("") === null; // Return null if input is empty string
greet(null) === null; // Return null if input is null
```
```coffeescript
greet("Niks") === "hello Niks!";
greet("") === null; // Return null if input is empty string
greet(null) === null; // Return null if input is null
```
```ruby
greet("Niks") == "hello Niks!"
greet("") == nil; # Return nil if input is empty string
greet(nil) == nil; # Return nil if input is nil
```
```csharp
greet("Niks") == "hello Niks!"
greet("") == null; // Return null if input is empty string
greet(null) == null; // Return null if input is null
```
```python
greet("Niks") --> "hello Niks!"
greet("") --> None # Return None if input is empty string
greet(None) --> None # Return None if input is None
```
| reference | def greet(name):
return f"hello { name } !" if name else None
| Say hello! | 55955a48a4e9c1a77500005a | [
"Fundamentals"
] | https://www.codewars.com/kata/55955a48a4e9c1a77500005a | 7 kyu |
# Introduction
<pre style="white-space: pre-wrap;white-space: -moz-pre-wrap;white-space: -pre-wrap;white-space: -o-pre-wrap;word-wrap: break-word;">
Mastermind or Master Mind is a code-breaking game for two players. The modern game with pegs was invented in 1970 by Mordecai Meirowitz, an Israeli postmaster and telecommunications expert. It resembles an earlier pencil and paper game called Bulls and Cows that may date back a century or more. (Source <a href="https://en.wikipedia.org/wiki/Mastermind_(board_game)">Wikipedia</a>)
</pre>
<center><img src="https://raw.githubusercontent.com/adrianeyre/codewars/master/Ruby/Authored/mastermind.jpg"></center>
# Rules
<pre style="white-space: pre-wrap;white-space: -moz-pre-wrap;white-space: -pre-wrap;white-space: -o-pre-wrap;word-wrap: break-word;">
1. The Mastermind (computer) will select 4 colours. The colours are randomly selected from <font color="#A1A85E">["Red", "Blue", "Green", "Orange", "Purple", "Yellow"]</font>. Colours can be duplicated but there will always be exactly <font color="#A1A85E">4</font>.
2. The Mastermind will return an array back to you. For every correctly positioned colour in the array an element of <font color="#A1A85E">“Black”</font> is returned. For every correct colour but in the wrong position an element of <font color="#A1A85E">“White”</font> will be returned.
3. Passing the correct array will pass the Kata test and return <font color="#A1A85E">"WON!"</font>.
4. Passing an invalid colour will fail the test with the error <font color="#A1A85E">"Error: you have given an invalid colour!"</font>
5. Passing an invalid array length will fail the test with the error <font color="#A1A85E">"Error: you must pass 4 colours!"</font>
6. Guessing more than 60 times will fail the test with the error <font color="#A1A85E">"Error: you have had more than 60 tries!"</font>
7. All colours are capitalised
8. The return array will be shuffled!
</pre>
# Task
<pre style="white-space: pre-wrap;white-space: -moz-pre-wrap;white-space: -pre-wrap;white-space: -o-pre-wrap;word-wrap: break-word;">
Your task is to create a method called <font color="#A1A85E">mastermind()</font> that will take an object called <font color="#A1A85E"> game</font>. The object has already been preloaded so you do not need to worry about it.
Within your method you must pass an array into the game object method <font color="#A1A85E">.check()</font>. This will evoke the object to check your array to see if it is correct.
</pre>
# Example
If the Mastermind selected the following colours<br>
<img src="https://raw.githubusercontent.com/adrianeyre/codewars/master/Ruby/Authored/mastermind1.png"><br>
Then the array you are trying to solve is `["Red", "Blue", "Green", "Yellow"]`<br><br>
So you guess with <br>
<img src="https://raw.githubusercontent.com/adrianeyre/codewars/master/Ruby/Authored/mastermind2.png"><br>
`["Red", "Orange", "Yellow", "Orange"]`
Your method would look like this.
```ruby
def mastermind(game)
answer = game.check(["Red", "Orange", "Yellow", "Orange"])
end
```
```python
def mastermind(game):
answer = game.check(["Red", "Orange", "Yellow", "Orange"])
```
```javascript
function mastermind(game){
answer = game.check(["Red", "Orange", "Yellow", "Orange"]);
}
```
The element `0 => Red` is at the correct index so `Black` is added to the return array. Element `2 => Yellow` is in the array but at the wrong index possition so `White` is added to the return array.
The Mastermind would then return `["Black", "White"]` (But not necessarily in that order as the return array is shuffled my the Mastermind).
Keep guessing until you pass the correct solution which will pass the Kata.
# Check result
To check the Masterminds return value
```ruby
answer = game.check(["Red", "Orange", "Yellow", "Orange"])
p answer
```
```python
answer = game.check(["Red", "Orange", "Yellow", "Orange"])
print (answer)
```
```javascript
answer = game.check(["Red", "Orange", "Yellow", "Orange"]);
console.log(answer);
```
Good luck and enjoy!
# Kata Series
If you enjoyed this, then please try one of my other Katas. Any feedback, translations and grading of beta Katas are greatly appreciated. Thank you.
<span style="display: flex !important;"><img style="margin:0px;" src="https://raw.githubusercontent.com/adrianeyre/codewars/master/Ruby/Authored/6KYU.png" alt="Rank"/> <a href="https://www.codewars.com/kata/58663693b359c4a6560001d6" target="_blank">Maze Runner</a></span>
<span style="display: flex !important;"><img style="margin:0px;" src="https://raw.githubusercontent.com/adrianeyre/codewars/master/Ruby/Authored/6KYU.png" alt="Rank"/> <a href="https://www.codewars.com/kata/58693bbfd7da144164000d05" target="_blank">Scooby Doo Puzzle</a></span>
<span style="display: flex !important;"><img style="margin:0px;" src="https://raw.githubusercontent.com/adrianeyre/codewars/master/Ruby/Authored/7KYU.png" alt="Rank"/> <a href="https://www.codewars.com/kata/586a1af1c66d18ad81000134" target="_blank">Driving License</a></span>
<span style="display: flex !important;"><img style="margin:0px;" src="https://raw.githubusercontent.com/adrianeyre/codewars/master/Ruby/Authored/6KYU.png" alt="Rank"/> <a href="https://www.codewars.com/kata/586c0909c1923fdb89002031" target="_blank">Connect 4</a></span>
<span style="display: flex !important;"><img style="margin:0px;" src="https://raw.githubusercontent.com/adrianeyre/codewars/master/Ruby/Authored/6KYU.png" alt="Rank"/> <a href="https://www.codewars.com/kata/586e6d4cb98de09e3800014f" target="_blank">Vending Machine</a></span>
<span style="display: flex !important;"><img style="margin:0px;" src="https://raw.githubusercontent.com/adrianeyre/codewars/master/Ruby/Authored/6KYU.png" alt="Rank"/> <a href="https://www.codewars.com/kata/587136ba2eefcb92a9000027" target="_blank">Snakes and Ladders</a></span>
<span style="display: flex !important;"><img style="margin:0px;" src="https://raw.githubusercontent.com/adrianeyre/codewars/master/Ruby/Authored/6KYU.png" alt="Rank"/> <a href="https://www.codewars.com/kata/58a848258a6909dd35000003" target="_blank">Mastermind</a></span>
<span style="display: flex !important;"><img style="margin:0px;" src="https://raw.githubusercontent.com/adrianeyre/codewars/master/Ruby/Authored/6KYU.png" alt="Rank"/> <a href="https://www.codewars.com/kata/58b2c5de4cf8b90723000051" target="_blank">Guess Who?</a></span>
<span style="display: flex !important;"><img style="margin:0px;" src="https://raw.githubusercontent.com/adrianeyre/codewars/master/Ruby/Authored/6KYU.png" alt="Rank"/> <a href="https://www.codewars.com/kata/58f5c63f1e26ecda7e000029" target="_blank">Am I safe to drive?</a></span>
<span style="display: flex !important;"><img style="margin:0px;" src="https://raw.githubusercontent.com/adrianeyre/codewars/master/Ruby/Authored/6KYU.png" alt="Rank"/> <a href="https://www.codewars.com/kata/58f5c63f1e26ecda7e000029" target="_blank">Mexican Wave</a></span>
<span style="display: flex !important;"><img style="margin:0px;" src="https://raw.githubusercontent.com/adrianeyre/codewars/master/Ruby/Authored/6KYU.png" alt="Rank"/> <a href="https://www.codewars.com/kata/58fdcc51b4f81a0b1e00003e" target="_blank">Pigs in a Pen</a></span>
<span style="display: flex !important;"><img style="margin:0px;" src="https://raw.githubusercontent.com/adrianeyre/codewars/master/Ruby/Authored/6KYU.png" alt="Rank"/> <a href="https://www.codewars.com/kata/590300eb378a9282ba000095" target="_blank">Hungry Hippos</a></span>
<span style="display: flex !important;"><img style="margin:0px;" src="https://raw.githubusercontent.com/adrianeyre/codewars/master/Ruby/Authored/6KYU.png" alt="Rank"/> <a href="https://www.codewars.com/kata/5904be220881cb68be00007d" target="_blank">Plenty of Fish in the Pond</a></span>
<span style="display: flex !important;"><img style="margin:0px;" src="https://raw.githubusercontent.com/adrianeyre/codewars/master/Ruby/Authored/6KYU.png" alt="Rank"/> <a href="https://www.codewars.com/kata/590adadea658017d90000039" target="_blank">Fruit Machine</a></span>
<span style="display: flex !important;"><img style="margin:0px;" src="https://raw.githubusercontent.com/adrianeyre/codewars/master/Ruby/Authored/6KYU.png" alt="Rank"/> <a href="https://www.codewars.com/kata/591eab1d192fe0435e000014" target="_blank">Car Park Escape</a></span> | reference | def mastermind(game):
colors = ["Red", "Blue", "Green", "Orange", "Purple", "Yellow"]
a = random . sample(colors, 4)
return game . check(a)
| Mastermind | 58a848258a6909dd35000003 | [
"Arrays",
"Fundamentals"
] | https://www.codewars.com/kata/58a848258a6909dd35000003 | 5 kyu |
In mathematics, a [Diophantine equation](https://en.wikipedia.org/wiki/Diophantine_equation) is a polynomial equation, usually with two or more unknowns, such that only the integer solutions are sought or studied.
In this kata we want to find all integers `x, y` (`x >= 0, y >= 0`) solutions of a diophantine equation of the form:
#### x<sup>2</sup> - 4 \* y<sup>2</sup> = n
(where the unknowns are `x` and `y`, and `n` is a given positive number)
in decreasing order of the positive x<sub>i</sub>.
If there is no solution return `[]` or `"[]"` or `""`. (See "RUN SAMPLE TESTS" for examples of returns).
#### Examples:
```
solEquaStr(90005) --> "[[45003, 22501], [9003, 4499], [981, 467], [309, 37]]"
solEquaStr(90002) --> "[]"
```
#### Hint:
x<sup>2</sup> - 4 \* y<sup>2</sup> = (x - 2\*y) \* (x + 2\*y) | reference | import math
def sol_equa(n):
res = []
for i in range(1, int(math . sqrt(n)) + 1):
if n % i == 0:
j = n / / i
if (i + j) % 2 == 0 and (j - i) % 4 == 0:
x = (i + j) / / 2
y = (j - i) / / 4
res . append([x, y])
return res
| Diophantine Equation | 554f76dca89983cc400000bb | [
"Fundamentals",
"Mathematics",
"Algebra"
] | https://www.codewars.com/kata/554f76dca89983cc400000bb | 5 kyu |
The prime numbers are not regularly spaced. For example from `2` to `3` the gap is `1`.
From `3` to `5` the gap is `2`. From `7` to `11` it is `4`.
Between 2 and 50 we have the following pairs of 2-gaps primes:
`3-5, 5-7, 11-13, 17-19, 29-31, 41-43`
A prime gap of length n is a run of n-1 consecutive composite numbers between two **successive** primes (see: http://mathworld.wolfram.com/PrimeGaps.html).
We will write a function gap with parameters:
- `g` (integer >= 2) which indicates the gap we are looking for
- `m` (integer > 2) which gives the start of the search (m inclusive)
- `n` (integer >= m) which gives the end of the search (n inclusive)
In the example above `gap(2, 3, 50)` will return `[3, 5] or (3, 5) or {3, 5}` which is the first pair between 3 and 50 with a 2-gap.
So this function should return the **first** pair of two prime numbers spaced with a gap of `g` between the limits `m`, `n` if these numbers exist otherwise `nil or null or None or Nothing (or ... depending on the language).
```
In such a case (no pair of prime numbers with a gap of `g`)
In C: return [0, 0]
In C++, Lua, COBOL: return `{0, 0}`.
In F#: return `[||]`.
In Kotlin, Dart and Prolog: return `[]`.
In Pascal: return Type TGap (0, 0).
```
#### Examples:
-
`gap(2, 5, 7) --> [5, 7] or (5, 7) or {5, 7}`
- `gap(2, 5, 5) --> nil. In C++ {0, 0}. In F# [||]. In Kotlin, Dart and Prolog return `[]`
- `gap(4, 130, 200) --> [163, 167] or (163, 167) or {163, 167}`
([193, 197] is also such a 4-gap primes between 130 and 200 but it's not the first pair)
- `gap(6,100,110) --> nil or {0, 0} or ...` : between 100 and 110 we have `101, 103, 107, 109` but `101-107`is not a 6-gap because there is `103`in between and `103-109`is not a 6-gap because there is `107`in between.
- You can see more examples of return in Sample Tests.
#### Note for Go
For Go: nil slice is expected when there are no gap between m and n.
Example: gap(11,30000,100000) --> nil
#### Ref
https://en.wikipedia.org/wiki/Prime_gap
| reference | def gap(g, m, n):
previous_prime = n
for i in range(m, n + 1):
if is_prime(i):
if i - previous_prime == g:
return [previous_prime, i]
previous_prime = i
return None
def is_prime(n):
for i in range(2, int(n * * .5 + 1)):
if n % i == 0:
return False
return True
| Gap in Primes | 561e9c843a2ef5a40c0000a4 | [
"Fundamentals"
] | https://www.codewars.com/kata/561e9c843a2ef5a40c0000a4 | 5 kyu |
The prime numbers are not regularly spaced. For example from `2` to `3` the step is `1`.
From `3` to `5` the step is `2`. From `7` to `11` it is `4`.
Between 2 and 50 we have the following pairs of 2-steps primes:
`3, 5 - 5, 7, - 11, 13, - 17, 19, - 29, 31, - 41, 43`
We will write a function `step` with parameters:
- `g` (integer >= 2) which indicates the step we are looking for,
- `m` (integer >= 2) which gives the start of the search (m inclusive),
- `n` (integer >= m) which gives the end of the search (n inclusive)
In the example above `step(2, 2, 50)` will return `[3, 5]` which is the first pair between 2 and 50 with a 2-steps.
So this function should return the **first** pair of the two prime numbers spaced with a step of `g`
between the limits `m`, `n` if these g-steps prime numbers exist otherwise `nil` or `null` or `None` or `Nothing` or `[]` or `"0, 0"` or `{0, 0}` or `0 0` or "" (depending on the language).
#### Examples:
`step(2, 5, 7) --> [5, 7] or (5, 7) or {5, 7} or "5 7"`
`step(2, 5, 5) --> nil or ... or [] in Ocaml or {0, 0} in C++`
`step(4, 130, 200) --> [163, 167] or (163, 167) or {163, 167}`
See more examples for your language in "TESTS"
#### Remarks:
([193, 197] is also such a 4-steps primes between 130 and 200 but it's not the first pair).
`step(6, 100, 110) --> [101, 107]` though there is a prime between 101 and 107 which is 103; the pair 101-103 is a 2-step.
#### Notes:
The idea of "step" is close to that of "gap" but it is not exactly the same. For those interested they can have a look
at <http://mathworld.wolfram.com/PrimeGaps.html>.
A "gap" is more restrictive: there must be no primes in between
(101-107 is a "step" but not a "gap". Next kata will be about "gaps":-).
For Go: nil slice is expected when there are no `step` between m and n.
Example: step(2,4900,4919) --> nil
| reference | import math
def isPrime(n):
if n <= 1:
return False
for i in range(2, int(math . sqrt(n) + 1)):
if n % i == 0:
return False
return True
def step(g, m, n):
if m >= n:
return []
else:
for i in range(m, n + 1 - g):
if isPrime(i) and isPrime(i + g):
return [i, i + g]
| Steps in Primes | 5613d06cee1e7da6d5000055 | [
"Mathematics",
"Number Theory"
] | https://www.codewars.com/kata/5613d06cee1e7da6d5000055 | 6 kyu |
# Allergies
Write a program that, given a person's allergy score, can tell them whether or not they're allergic to a given item, and their full list of allergies.
An allergy test produces a single numeric score which contains the
information about all the allergies the person has (that they were
tested for).
The list of items (and their value) that were tested are:
* eggs (1)
* peanuts (2)
* shellfish (4)
* strawberries (8)
* tomatoes (16)
* chocolate (32)
* pollen (64)
* cats (128)
So if Tom is allergic to peanuts and chocolate, he gets a score of 34.
Now, given just that score of 34, your program should be able to say:
- Whether Tom is allergic to any one of those allergens listed above.
- All the allergens Tom is allergic to., sorted alphabetically
Example:
``` python
>>> allergies = Allergies(0)
>>> allergies.is_allergic_to('peanuts')
False
```
``` python
>>> Allergies(255).allergies()
['cats', 'chocolate', 'eggs', 'peanuts', 'pollen', 'shellfish', 'strawberries', 'tomatoes']
```
``` python
>>> Allergies(259).allergies()
["eggs", "peanuts"]
```
You will be provided with a class `Allergies` which will have 2 methods
+ is_allergic_to
Checks if Tom is allergic to a particular allergen. Returns True if Tom is allergic, False otherwise
+ allergies
Returns a list of what Tom is allergic to. This list must be sorted alphabetically
Must Dos:
Ensure that your function throws a TypeError for invalid inputs such as None(Null), floats, strings, or any data type that is not an integer.
*Hint: Use Bitwise ANDing*
| games | class Allergies (object):
ALLERGY_SCORES = {
'eggs': 1,
'peanuts': 2,
'shellfish': 4,
'strawberries': 8,
'tomatoes': 16,
'chocolate': 32,
'pollen': 64,
'cats': 128
}
def __init__(self, score):
self . allergicTo = sorted(
allergen for allergen, v in self . ALLERGY_SCORES . items() if v & score)
self . score = score
def is_allergic_to(self, allergen):
return self . ALLERGY_SCORES[allergen] & self . score
def allergies(self):
return self . allergicTo
| Tom's Allergies | 58be35e9e36224a33f000023 | [
"Algorithms",
"Puzzles",
"Fundamentals"
] | https://www.codewars.com/kata/58be35e9e36224a33f000023 | 6 kyu |
Implement [the Geohashing algorithm](https://xkcd.com/426/) proposed by xkcd.
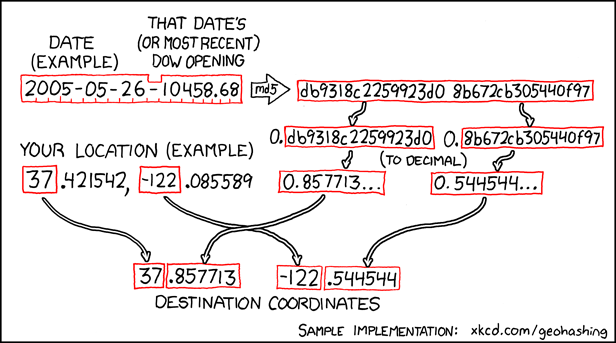
Specifically, given the the Dow opening and a date in date object (optional), return the geohashing coordinates, using the following steps (adapted from [explainxkcd](https://www.explainxkcd.com/wiki/index.php/426:_Geohashing), slightly modified):
1. Take the provided date and convert it in the format `yyyy-mm-dd-` at UTC time, then append the most recent opening value for the Dow Jones Industrial Average (supplied as an argument). Dow opening provided will always be accurate to 2 decimal places, but if it has less than 2 decimal digits, pad 0s on the right (`12345 => 12345.00`). If no dates are provided, use current time instead.
2. Pass this string through the MD5 algorithm, and output as a hexademical value.
3. Divide the hash value into two 16 character halves, treat each half as base-16 decimal expansions (by appending `0.` in the front, and convert them back to base 10, rounded to 6 decimal places.
4. Return the result coordinates as an array.
An example case straight out from the comic is provided:
<pre><code>Date: 2005-05-26, Dow opening: 10458.68
yyyy-mm-dd-dow: 2005-05-26-10458.68
MD5: db9318c2259923d08b672cb305440f97 => db9318c2259923d0 | 8b672cb305440f97
Coordinates (HEX): [0.db9318c2259923d0, 0.8b672cb305440f97]
Coordinates (DEC): [0.857713, 0.544543]</code></pre>
Note: You don't have to worry about the 30W Time Zone Rule. | reference | from datetime import datetime
import hashlib
def geohash(dow, date=datetime . utcnow()):
dow = "%.2f" % dow
s = str(date)[: 10] + "-" + str(dow)
m = hashlib . md5(s . encode('utf-8')). hexdigest()
m1, m2 = float . fromhex('0.' + m[: 16]), float . fromhex('0.' + m[- 16:])
return [round(m1, 6), round(m2, 6)]
| Geohashing | 58ca7afc92ce34dfa50001fa | [
"Algorithms",
"Fundamentals"
] | https://www.codewars.com/kata/58ca7afc92ce34dfa50001fa | 5 kyu |
# Task
You are given an array of up to four non-negative integers, each less than 256.
Your task is to pack these integers into one number M in the following way:
```
The first element of the array occupies the first 8 bits of M;
The second element occupies next 8 bits, and so on.
```
Return the obtained integer M as unsigned integer.
- Note:
the phrase "first bits of M" refers to the least significant bits of M - the right-most bits of an integer. For further clarification see the following example.
# Example
For `a = [24, 85, 0]`, the output should be `21784`
An array `[24, 85, 0]` looks like `[00011000, 01010101, 00000000]` in binary.
After packing these into one number we get `00000000 01010101 00011000` (spaces are placed for convenience), which equals to 21784.
# Input/Output
- `[input]` integer array `a`
Constraints: `1 ≤ a.length ≤ 4` and `0 ≤ a[i] < 256`
- `[output]` an unsigned integer
# More Challenge
- Are you a One-Liner? Please try to complete the kata in one line(no test for it) ;-) | games | def array_packing(arr):
return int . from_bytes(arr, 'little')
| Simple Fun #9: Array Packing | 588453ea56daa4af920000ca | [
"Puzzles"
] | https://www.codewars.com/kata/588453ea56daa4af920000ca | 7 kyu |
Complete the function that takes two numbers as input, ```num``` and ```nth``` and return the `nth` digit of `num` (counting from right to left).
## Note
- If ```num``` is negative, ignore its sign and treat it as a positive value
- If ```nth``` is not positive, return `-1`
- Keep in mind that `42 = 00042`. This means that ```findDigit(42, 5)``` would return `0`
## Examples(```num```, ```nth``` --> output)
```
5673, 4 --> 5
129, 2 --> 2
-2825, 3 --> 8
-456, 4 --> 0
0, 20 --> 0
65, 0 --> -1
24, -8 --> -1
``` | reference | def find_digit(num, nth):
if nth <= 0:
return - 1
try:
return int(str(num). lstrip('-')[- nth])
except IndexError:
return 0
| Find the nth Digit of a Number | 577b9960df78c19bca00007e | [
"Fundamentals"
] | https://www.codewars.com/kata/577b9960df78c19bca00007e | 7 kyu |
Create a function that takes a Number as its argument and returns a Chinese numeral string. You don't need to validate the input argument, it will always be a Number in the range `[-99999.999, 99999.999]`, rounded to 8 decimal places.
Simplified Chinese numerals have characters representing each number from 0 to 9 and additional numbers representing larger numbers like 10, 100, 1000, and 10000.
```
0 líng 零
1 yī 一
2 èr 二
3 sān 三
4 sì 四
5 wǔ 五
6 liù 六
7 qī 七
8 bā 八
9 jiǔ 九
10 shí 十
100 bǎi 百
1000 qiān 千
10000 wàn 万
```
Multiple-digit numbers are constructed by first the digit value (1 to 9) and then the place multiplier (such as 10, 100, 1000), starting with the most significant digit. A special case is made for 10 - 19 where the leading digit value (yī 一) is dropped. Note that this special case is only made for the actual values 10 - 19, not any larger values.
```
10 十
11 十一
18 十八
21 二十一
110 一百一十
123 一百二十三
24681 二万四千六百八十一
```
Trailing zeros are omitted, but interior zeros are grouped together and indicated by a single 零 character without giving the place multiplier.
```
10 十
20 二十
104 一百零四
1004 一千零四
10004 一万零四
10000 一万
```
Decimal numbers are constructed by first writing the whole number part, and then inserting a point (diǎn 点), followed by the decimal portion. The decimal portion is expressed using only the digits 0 to 9, without any positional characters and without grouping zeros.
```
0.1 零点一
123.45 一百二十三点四五
```
Negative numbers are the same as other numbers, but add a 负 (fù) before the number.
For more information, please see http://en.wikipedia.org/wiki/Chinese_numerals. | algorithms | import re
NEG, DOT, _, * DIGS = "负点 零一二三四五六七八九"
POWS = " 十 百 千 万" . split(' ')
NUMS = {str(i): c for i, c in enumerate(DIGS)}
for n in range(10):
NUMS[str(n + 10)] = POWS[1] + DIGS[n] * bool(n)
def to_chinese_numeral(n):
ss = str(abs(n)). split('.')
return NEG * (n < 0) + parse (ss [0 ]) + (len (ss ) > 1 and decimals (ss [1 ]) or '' )
def decimals(digs): return DOT + '' . join(NUMS [d] for d in digs)
def parse(s):
if s in NUMS:
return NUMS[s]
s = '' . join (reversed ([NUMS [d ] + POWS [i ] * (d != '0' ) for i , d in enumerate (reversed (s )) ]))
return re . sub(f'零+$|(?<=零)零+', '', s)
| Chinese Numeral Encoder | 52608f5345d4a19bed000b31 | [
"Algorithms"
] | https://www.codewars.com/kata/52608f5345d4a19bed000b31 | 4 kyu |
## Description:
Remove all exclamation marks from sentence but ensure a exclamation mark at the end of string. For a beginner kata, you can assume that the input data is always a non empty string, no need to verify it.
## Examples
```
"Hi!" ---> "Hi!"
"Hi!!!" ---> "Hi!"
"!Hi" ---> "Hi!"
"!Hi!" ---> "Hi!"
"Hi! Hi!" ---> "Hi Hi!"
"Hi" ---> "Hi!"
```
| reference | def remove(s):
return s . replace("!", "") + "!"
| Exclamation marks series #4: Remove all exclamation marks from sentence but ensure a exclamation mark at the end of string | 57faf12b21c84b5ba30001b0 | [
"Fundamentals"
] | https://www.codewars.com/kata/57faf12b21c84b5ba30001b0 | 8 kyu |
### The problem
How many zeroes are at the **end** of the [factorial](https://en.wikipedia.org/wiki/Factorial) of `10`? 10! = 36288<u>00</u>, i.e. there are `2` zeroes.
16! (or 0x10!) in [hexadecimal](https://en.wikipedia.org/wiki/Hexadecimal) would be 0x130777758<u>000</u>, which has `3` zeroes.
### Scalability
Unfortunately, machine integer numbers has not enough precision for larger values. Floating point numbers drop the tail we need. We can fall back to arbitrary-precision ones - built-ins or from a library, but calculating the full product isn't an efficient way to find just the _tail_ of a factorial. Calculating `100'000!` in compiled language takes around 10 seconds. `1'000'000!` would be around 10 minutes, even using efficient [Karatsuba algorithm](https://en.wikipedia.org/wiki/Karatsuba_algorithm)
### Your task
is to write a function, which will find the number of zeroes at the end of `(number)` factorial in arbitrary [radix](https://en.wikipedia.org/wiki/Radix) = `base` for larger numbers.
- `base` is an integer from 2 to 256
- `number` is an integer from 1 to 1'000'000
<u>**Note**</u> Second argument: number is always declared, passed and displayed as a regular _decimal_ number. If you see a test described as `42! in base 20` it's 42<sub>10</sub> **not** 42<sub>20</sub> = 82<sub>10</sub>.
| algorithms | PRIMES = [2, 3, 5, 7, 11, 13, 17, 19, 23, 29, 31, 37, 41, 43, 47, 53, 59, 61, 67, 71, 73, 79, 83,
89, 97, 101, 103, 107, 109, 113, 127, 131, 137, 139, 149, 151, 157, 163, 167, 173, 179,
181, 191, 193, 197, 199, 211, 223, 227, 229, 233, 239, 241, 251]
def find_multiplicity(n, p):
m = 0
while n > 1 and n % p == 0:
m, n = m + 1, n / / p
return m
def find_number_factors(n, p):
number, d = 0, p
while d <= n:
number, d = number + n / / d, d * p
return number
def zeroes(base, number):
def prime_decomposition(n): return [(q, m) for q, m in [(
p, find_multiplicity(n, p)) for p in PRIMES] if m > 0]
return min(find_number_factors(number, p) / / m for p, m in prime_decomposition(base))
| Factorial tail | 55c4eb777e07c13528000021 | [
"Algorithms"
] | https://www.codewars.com/kata/55c4eb777e07c13528000021 | 4 kyu |
Write a program to determine if a string contains only unique characters.
Return true if it does and false otherwise.
The string may contain any of the 128 ASCII characters.
Characters are case-sensitive, e.g. 'a' and 'A' are considered different characters.
| algorithms | def has_unique_chars(s):
return len(s) == len(set(s))
| All unique | 553e8b195b853c6db4000048 | [
"Algorithms",
"Strings"
] | https://www.codewars.com/kata/553e8b195b853c6db4000048 | 7 kyu |
Complete the function that receives as input a string, and produces outputs according to the following table:
| Input | Output
| ---- | ------
| "Jabroni" | "Patron Tequila"
| "School Counselor" | "Anything with Alcohol"
| "Programmer" | "Hipster Craft Beer"
| "Bike Gang Member" | "Moonshine"
| "Politician" | "Your tax dollars"
| "Rapper" | "Cristal"
| *anything else* | "Beer"
**Note:** *anything else* is the default case: if the input to the function is not any of the values in the table, then the return value should be `"Beer"`.
Make sure you cover the cases where certain words do not show up with correct capitalization. For example, the input `"pOLitiCIaN"` should still return `"Your tax dollars"`.
| reference | d = {
"jabroni": "Patron Tequila",
"school counselor": "Anything with Alcohol",
"programmer": "Hipster Craft Beer",
"bike gang member": "Moonshine",
"politician": "Your tax dollars",
"rapper": "Cristal"
}
def get_drink_by_profession(s):
return d . get(s . lower(), "Beer")
| L1: Bartender, drinks! | 568dc014440f03b13900001d | [
"Strings",
"Fundamentals"
] | https://www.codewars.com/kata/568dc014440f03b13900001d | 8 kyu |
# Safen User Input Part I - htmlspecialchars
You are a(n) novice/average/experienced/professional/world-famous Web Developer (choose one) who owns a(n) simple/clean/slick/beautiful/complicated/professional/business website (choose one or more) which contains form fields so visitors can send emails or leave a comment on your website with ease. However, **with ease comes danger**. Every now and then, a hacker visits your website and attempts to compromise it through the use of **XSS (Cross Site Scripting)**. This is done by injecting `script` tags into the website through form fields which may contain malicious code (e.g. a redirection to a malicious website that steals personal information).
## Mission
Your mission is to implement a function that converts the following potentially harmful characters:
1. `<` --> `<`
2. `>` --> `>`
3. `"` --> `"`
4. `&` --> `&`
Good luck :D
~~~if:riscv
RISC-V: the function signature is
```c
void htmlspecialchars(const char *str, char *out);
```
`str` is the input string. Write your result to `out`. You may assume `out` is large enough to hold the result. You do not need to return anything.
~~~ | reference | def html_special_chars(data):
symbols = {'<': '<', '>': '>', '"': '"', '&': '&'}
return "" . join(symbols . get(x, x) for x in data)
| Safen User Input Part I - htmlspecialchars | 56bcaedfcf6b7f2125001118 | [
"Fundamentals",
"Strings"
] | https://www.codewars.com/kata/56bcaedfcf6b7f2125001118 | 8 kyu |
Time to test your basic knowledge in functions!
Return the odds from a list:
```
[1, 2, 3, 4, 5] --> [1, 3, 5]
[2, 4, 6] --> []
``` | reference | def odds(values):
return [i for i in values if i % 2]
| Are arrow functions odd? | 559f80b87fa8512e3e0000f5 | [
"Fundamentals"
] | https://www.codewars.com/kata/559f80b87fa8512e3e0000f5 | 8 kyu |
Hi and welcome to team Gilded Rose.
You are asked to fix the code for our store management system.
All items have a `sell_in` value which denotes the number of days we have left to sell the item and a `quality` value which denotes how valuable the item is. (For Java specifics see ** Java Notes ** below)
At the end of each day our software should lower both values for every item.
Pretty simple, right? Well this is just the general rule with some exception:
* once the sell_in days is less then zero, quality degrades twice as fast;
* the quality of an item can never be negative or increase beyond 50;
* the _"Aged Brie"_ goods actually increases in quality each passing day;
* _"Sulfuras"_ goods, being legendary items, never change their sell_in or quality values;
* _"backstage passes"_, like aged brie, increases in quality as it's sell_in value decreases;
* not just that: for _"backstage passes"_ quality increases by 2 when there are 10 days or less and by 3 when there are 5 days or less but quality drops to 0 after the concert (sell_in 0 or lower).
Complicated enough, now? Well, there is a new item category that we would like to see added to the inventory management system:
* _"Conjured"_ items degrade in quality twice as fast as normal items.
You can change the update_quality method, add any new code, but you should NOT edit the item constructor/class: it belong to the goblin in the corner who will insta-rage and one-shot you as he doesn't believe in shared code ownership.
Just for extra clarification, an item can never have its quality increase above 50, however "Sulfuras" is a legendary item and as such its quality is 80 and it never alters.
You won't find mixed categories (like a "Conjured Sulfuras Backstage pass of Doom"), but the category name may be not in the first position (ie: expect something like "SuperUberSword, Conjured" or "Mighty Sulfuras Armour of Ultimate Awesomeness").
** Java Notes **
* `sell_in` value can be accessed using `getSellIn()` and `setSellIn()` methods
* `quality` value can be accessed using `getQuality()` and `setQuality()` methods
***************************
Personal note: I was recently asked during an interview to solve a relatively simple, yet interesting problem, original taken from [here](https://github.com/emilybache/GildedRose-Refactoring-Kata).
As I was apparently scouted thanks to CodeWars, I think the bare minimum I could do to give back to the community was to turn this idea into a bug-fixes kata, with some small twists added to the original they showed me :) | bug_fixes | def update_quality(items):
for i in items:
name = i . name . lower()
if "sulfuras" in name:
continue
i . sell_in -= 1
if "aged brie" in name:
deg = 1
elif "backstage passes" in name:
deg = 1 if i . sell_in > 10 else 2 if i . sell_in > 5 else 3 if i . sell_in >= 0 else - i . quality
else:
deg = (- 2 if i . sell_in < 0 else - 1) * (2 if "conjured" in name else 1)
i . quality = max(0, min(max(50, i . quality), i . quality + deg))
| Shop Inventory Manager | 55d1d06def244b18c100007c | [
"Debugging"
] | https://www.codewars.com/kata/55d1d06def244b18c100007c | 6 kyu |
<h3>Algorithmic predicament - Bug Fixing #9</h3>
<p>
Oh no! Timmy's algorithm has gone wrong! Help Timmy fix his algorithm!
</p>
<h2>Task</h2>
<p>
Your task is to fix Timmy's algorithm so it returns the group name with the highest total age.
</p>
<p>
You will receive two groups of `people` objects, with two properties `name` and `age`. The name property is a string, and the age property is a number.
</p>
<p>
Your goal is to calculate the total age of all people with the same name in both groups and return the name of the person with the highest total age. If two names have the same total age, return the first alphabetical name.
</p>
| algorithms | def highest_age(g1, g2):
d = {}
for p in g1 + g2:
name, age = p["name"], p["age"]
d[name] = d . setdefault(name, 0) + age
return max(sorted(d . keys()), key=d . __getitem__)
| Algorithmic predicament- Bug Fixing #9 | 55d3b1f2c1b2f0d3470000a9 | [
"Debugging",
"Algorithms"
] | https://www.codewars.com/kata/55d3b1f2c1b2f0d3470000a9 | 7 kyu |
In this example you have to validate if a user input string is alphanumeric. The given string is not `nil/null/NULL/None`, so you don't have to check that.
The string has the following conditions to be alphanumeric:
* At least one character (`""` is not valid)
* Allowed characters are uppercase / lowercase latin letters and digits from `0` to `9`
* No whitespaces / underscore
| bug_fixes | def alphanumeric(string):
return string . isalnum()
| Not very secure | 526dbd6c8c0eb53254000110 | [
"Regular Expressions",
"Strings"
] | https://www.codewars.com/kata/526dbd6c8c0eb53254000110 | 5 kyu |