description
stringlengths 38
154k
| category
stringclasses 5
values | solutions
stringlengths 13
289k
| name
stringlengths 3
179
| id
stringlengths 24
24
| tags
sequencelengths 0
13
| url
stringlengths 54
54
| rank_name
stringclasses 8
values |
---|---|---|---|---|---|---|---|
You will be given an array of objects representing data about developers who have signed up to attend the next web development meetup that you are organising. Three programming languages will be represented: Python, Ruby and JavaScript.
Your task is to return either:
- `true` if the number of meetup participants representing any of the three programming languages is ** at most 2 times higher than the number of developers representing any of the remaining programming languages**; or
- `false` otherwise.
For example, given the following input array:
```javascript
var list1 = [
{ firstName: 'Daniel', lastName: 'J.', country: 'Aruba', continent: 'Americas', age: 42, language: 'Python' },
{ firstName: 'Kseniya', lastName: 'T.', country: 'Belarus', continent: 'Europe', age: 22, language: 'Ruby' },
{ firstName: 'Sou', lastName: 'B.', country: 'Japan', continent: 'Asia', age: 43, language: 'Ruby' },
{ firstName: 'Hanna', lastName: 'L.', country: 'Hungary', continent: 'Europe', age: 95, language: 'JavaScript' },
{ firstName: 'Jayden', lastName: 'P.', country: 'Jamaica', continent: 'Americas', age: 18, language: 'JavaScript' },
{ firstName: 'Joao', lastName: 'D.', country: 'Portugal', continent: 'Europe', age: 25, language: 'JavaScript' }
];
```
```python
list1 = [
{ 'firstName': 'Daniel', 'lastName': 'J.', 'country': 'Aruba', 'continent': 'Americas', 'age': 42, 'language': 'Python' },
{ 'firstName': 'Kseniya', 'lastName': 'T.', 'country': 'Belarus', 'continent': 'Europe', 'age': 22, 'language': 'Ruby' },
{ 'firstName': 'Sou', 'lastName': 'B.', 'country': 'Japan', 'continent': 'Asia', 'age': 43, 'language': 'Ruby' },
{ 'firstName': 'Hanna', 'lastName': 'L.', 'country': 'Hungary', 'continent': 'Europe', 'age': 95, 'language': 'JavaScript' },
{ 'firstName': 'Jayden', 'lastName': 'P.', 'country': 'Jamaica', 'continent': 'Americas', 'age': 18, 'language': 'JavaScript' },
{ 'firstName': 'Joao', 'lastName': 'D.', 'country': 'Portugal', 'continent': 'Europe', 'age': 25, 'language': 'JavaScript' }
]
```
```cobol
01 List.
03 ListLength pic 9(2) value 6.
03 dev1.
05 FirstName pic a(9) value 'Daniel'.
05 LastName pic x(2) value 'J.'.
05 Country pic a(24) value 'Aruba'.
05 Continent pic a(8) value 'Americas'.
05 Age pic 9(3) value 42.
05 Language pic a(10) value 'Python'.
03 dev2.
05 FirstName pic a(9) value 'Kseniya'.
05 LastName pic x(2) value 'T.'.
05 Country pic a(24) value 'Belarus'.
05 Continent pic a(8) value 'Europe'.
05 Age pic 9(3) value 29.
05 Language pic a(10) value 'Ruby'.
03 dev3.
05 FirstName pic a(9) value 'Sou'.
05 LastName pic x(2) value 'B.'.
05 Country pic a(24) value 'Japan'.
05 Continent pic a(8) value 'Asia'.
05 Age pic 9(3) value 43.
05 Language pic a(10) value 'Ruby'.
03 dev4.
05 FirstName pic a(9) value 'Hanna'.
05 LastName pic x(2) value 'L.'.
05 Country pic a(24) value 'Hungary'.
05 Continent pic a(8) value 'Europe'.
05 Age pic 9(3) value 29.
05 Language pic a(10) value 'JavaScript'.
03 dev5.
05 FirstName pic a(9) value 'Jayden'.
05 LastName pic x(2) value 'P.'.
05 Country pic a(24) value 'Jamaica'.
05 Continent pic a(8) value 'Americas'.
05 Age pic 9(3) value 42.
05 Language pic a(10) value 'JavaScript'.
03 dev6.
05 FirstName pic a(9) value 'Joao'.
05 LastName pic x(2) value 'D.'.
05 Country pic a(24) value 'Portugal'.
05 Continent pic a(8) value 'Europe'.
05 Age pic 9(3) value 65.
05 Language pic a(10) value 'JavaScript'.
```
your function should return `false` as the number of JavaScript developers (`3`) is **3 times higher** than the number of Python developers (`1`). It can't be more than 2 times higher to be regarded as language-diverse.
Notes:
- The strings representing all three programming languages will always be formatted in the same way (e.g. 'JavaScript' will always be formatted with upper-case 'J' and 'S'.
- The input array will always be valid and formatted as in the example above.
- Each of the 3 programming languages will always be represented.
<br>
<br>
<br>
<br>
<br>
This kata is part of the **Coding Meetup** series which includes a number of short and easy to follow katas which have been designed to allow mastering the use of higher-order functions. In JavaScript this includes methods like: `forEach, filter, map, reduce, some, every, find, findIndex`. Other approaches to solving the katas are of course possible.
Here is the full list of the katas in the **Coding Meetup** series:
<a href="http://www.codewars.com/kata/coding-meetup-number-1-higher-order-functions-series-count-the-number-of-javascript-developers-coming-from-europe">Coding Meetup #1 - Higher-Order Functions Series - Count the number of JavaScript developers coming from Europe</a>
<a href="https://www.codewars.com/kata/coding-meetup-number-2-higher-order-functions-series-greet-developers">Coding Meetup #2 - Higher-Order Functions Series - Greet developers</a>
<a href="https://www.codewars.com/kata/coding-meetup-number-3-higher-order-functions-series-is-ruby-coming">Coding Meetup #3 - Higher-Order Functions Series - Is Ruby coming?</a>
<a href="https://www.codewars.com/kata/coding-meetup-number-4-higher-order-functions-series-find-the-first-python-developer">Coding Meetup #4 - Higher-Order Functions Series - Find the first Python developer</a>
<a href="https://www.codewars.com/kata/coding-meetup-number-5-higher-order-functions-series-prepare-the-count-of-languages">Coding Meetup #5 - Higher-Order Functions Series - Prepare the count of languages</a>
<a href="https://www.codewars.com/kata/coding-meetup-number-6-higher-order-functions-series-can-they-code-in-the-same-language">Coding Meetup #6 - Higher-Order Functions Series - Can they code in the same language?</a>
<a href="http://www.codewars.com/kata/coding-meetup-number-7-higher-order-functions-series-find-the-most-senior-developer">Coding Meetup #7 - Higher-Order Functions Series - Find the most senior developer</a>
<a href="https://www.codewars.com/kata/coding-meetup-number-8-higher-order-functions-series-will-all-continents-be-represented">Coding Meetup #8 - Higher-Order Functions Series - Will all continents be represented?</a>
<a href="https://www.codewars.com/kata/coding-meetup-number-9-higher-order-functions-series-is-the-meetup-age-diverse">Coding Meetup #9 - Higher-Order Functions Series - Is the meetup age-diverse?</a>
<a href="https://www.codewars.com/kata/coding-meetup-number-10-higher-order-functions-series-create-usernames">Coding Meetup #10 - Higher-Order Functions Series - Create usernames</a>
<a href="https://www.codewars.com/kata/coding-meetup-number-11-higher-order-functions-series-find-the-average-age">Coding Meetup #11 - Higher-Order Functions Series - Find the average age</a>
<a href="https://www.codewars.com/kata/coding-meetup-number-12-higher-order-functions-series-find-github-admins">Coding Meetup #12 - Higher-Order Functions Series - Find GitHub admins</a>
<a href="https://www.codewars.com/kata/coding-meetup-number-13-higher-order-functions-series-is-the-meetup-language-diverse">Coding Meetup #13 - Higher-Order Functions Series - Is the meetup language-diverse?</a>
<a href="https://www.codewars.com/kata/coding-meetup-number-14-higher-order-functions-series-order-the-food">Coding Meetup #14 - Higher-Order Functions Series - Order the food</a>
<a href="https://www.codewars.com/kata/coding-meetup-number-15-higher-order-functions-series-find-the-odd-names">Coding Meetup #15 - Higher-Order Functions Series - Find the odd names</a>
<a href="https://www.codewars.com/kata/coding-meetup-number-16-higher-order-functions-series-ask-for-missing-details">Coding Meetup #16 - Higher-Order Functions Series - Ask for missing details</a> | algorithms | from collections import Counter
def is_language_diverse(lst):
c = Counter(map(lambda x: x["language"], lst)). values()
return max(c) <= 2 * min(c)
| Coding Meetup #13 - Higher-Order Functions Series - Is the meetup language-diverse? | 58381907f8ac48ae070000de | [
"Functional Programming",
"Data Structures",
"Arrays",
"Fundamentals",
"Algorithms",
"Strings"
] | https://www.codewars.com/kata/58381907f8ac48ae070000de | 6 kyu |
Create a finite automaton that has three states. Finite automatons are the same as finite state machines for our purposes.
Our simple automaton, accepts the language of `A`, defined as `{0, 1}` and should have three states: `q1`, `q2`, and `q3`. Here is the description of the states:
* `q1` is our start state, we begin reading commands from here
* `q2` is our accept state, we return `true` if this is our last state
And the transitions:
* `q1` moves to `q2` when given a `1`, and stays at `q1` when given a `0`
* `q2` moves to `q3` when given a `0`, and stays at `q2` when given a `1`
* `q3` moves to `q2` when given a `0` or `1`
The automaton should return whether we end in our accepted state (`q2`), or not (`true`/`false`).
## Your task
You will have to design your state objects, and how your Automaton handles transitions. Also make sure you set up the three states, `q1`, `q2`, and `q3` for the myAutomaton instance. The test fixtures will be calling against myAutomaton.
As an aside, the automaton accepts an array of strings, rather than just numbers, or a number represented as a string, because the language an automaton can accept isn't confined to just numbers. An automaton should be able to accept any 'symbol.'
Here are some resources on DFAs (the automaton this Kata asks you to create):
* http://en.wikipedia.org/wiki/Deterministic_finite_automaton
* http://www.cs.odu.edu/~toida/nerzic/390teched/regular/fa/dfa-definitions.html
* http://www.cse.chalmers.se/~coquand/AUTOMATA/o2.pdf
## Example
```javascript
var a = new Automaton();
a.readCommands(["1", "0", "0", "1", "0"]); ==> false
```
```python
a = Automaton()
a.read_commands(["1", "0", "0", "1", "0"]) ==> False
```
```ruby
a = Automaton.new
a.read_commands(["1", "0", "0", "1", "0"]) ==> false
```
```coffeescript
a = new Automaton()
a.readCommands ["1", "0", "0", "1", "0"] ==> false
```
```c++
a = Automaton()
a.read_commands({'1', '0', '0', '1', '0'}); ==> false
```
```c
read_commands("10010"); ==> false
```
```haskell
readCommands "10010" --> False
```
We make these transitions:
```
input: ["1", "0", "0", "1", "0"]
1: q1 -> q2
0: q2 -> q3
0: q3 -> q2
1: q2 -> q2
0: q2 -> q3
```
We end in `q3` which is **not** our accept state, so we return `false`
| algorithms | class Automaton (object):
def __init__(self):
self . automata = {('q1', '1'): 'q2', ('q1', '0'): 'q1',
('q2', '0'): 'q3', ('q2', '1'): 'q2',
('q3', '0'): 'q2', ('q3', '1'): 'q2'}
self . state = "q1"
def read_commands(self, commands):
for c in commands:
self . state = self . automata[(self . state, c)]
return self . state == "q2"
my_automaton = Automaton()
# Do anything necessary to set up your automaton's states, q1, q2, and q3.
| Design a Simple Automaton (Finite State Machine) | 5268acac0d3f019add000203 | [
"State Machines",
"Artificial Intelligence",
"Algorithms",
"Object-oriented Programming"
] | https://www.codewars.com/kata/5268acac0d3f019add000203 | 6 kyu |
There is a house with 4 levels.
In that house there is an elevator.
You can program this elevator to go up or down,
depending on what button the user touches inside the elevator.
Valid levels must be only these numbers: `0,1,2,3`
Valid buttons must be only these strings: `'0','1','2','3'`
Possible return values are these numbers: `-3,-2,-1,0,1,2,3`
If the elevator is on the ground floor(0th level)
and the user touches button '2'
the elevator must go 2 levels up,
so our function must return 2.
If the elevator is on the 3rd level
and the user touches button '0'
the elevator must go 3 levels down, so our function must return -3.
If the elevator is on the 2nd level,
and the user touches button '2'
the elevator must remain on the same level,
so we return 0.
We cannot endanger the lives of our passengers,
so if we get erronous inputs,
our elevator must remain on the same level.
So for example:
- `goto(2,'4')` must return 0, because there is no button '4' in the elevator.
- `goto(4,'0')` must return 0, because there is no level 4.
- `goto(3,undefined)` must return 0.
- `goto(undefined,'2')` must return 0.
- `goto([],'2')` must return 0 because the type of the input level is array instead of a number.
- `goto(3,{})` must return 0 because the type of the input button is object instead of a string. | reference | levels = [0, 1, 2, 3]
buttons = ['0', '1', '2', '3']
def goto(level, button):
if level not in levels or button not in buttons:
return 0
else:
return int(button) - level
| Simple elevator | 52ed326b8df6540e06000029 | [
"State Machines",
"Fundamentals"
] | https://www.codewars.com/kata/52ed326b8df6540e06000029 | 7 kyu |
In this Kata, you have to design a simple routing class for a web framework.
The router should accept bindings for a given url, http method and an action.
Then, when a request with a bound url and method comes in, it should return the result of the action.
Example usage:
```python
router = Router()
router.bind('/hello', 'GET', lambda: 'hello world')
router.runRequest('/hello', 'GET') // returns 'hello world'
```
```javascript
var router = new Router;
router.bind('/hello', 'GET', function(){ return 'hello world'; });
router.runRequest('/hello', 'GET') // returns 'hello world';
```
```coffeescript
router = new Router
router.bind '/hello', 'GET', -> 'hello world'
router.runRequest '/hello', 'GET' # returns 'hello world'
```
```groovy
router = new Router()
router.bind("/hello", "GET", { -> "hello world" })
router.runRequest("/hello", "GET") // returns "hello world"
```
```scala
router = new Router()
router.bind("/hello", "GET", () => "hello world")
router.runRequest("/hello", "GET") // returns "hello world"
```
When asked for a route that doesn't exist, router should return:
```javascript
'Error 404: Not Found'
```
```python
'Error 404: Not Found'
```
```groovy
"Error 404: Not Found"
```
```scala
"Error 404: Not Found"
```
The router should also handle modifying existing routes. See the example tests for more details.
| reference | class Router:
def __init__(self):
self . _routes = {}
def bind(self, url, method, action):
self . _routes[(url, method)] = action
def runRequest(self, url, method):
return self . _routes . get((url, method), lambda: "Error 404: Not Found")()
| Simple Web Framework #1: Create a basic router | 588a00ad70720f2cd9000005 | [
"Object-oriented Programming",
"Arrays",
"Fundamentals"
] | https://www.codewars.com/kata/588a00ad70720f2cd9000005 | 6 kyu |
The dragon's curve is a self-similar fractal which can be obtained by a recursive method.
Starting with the string `D0 = 'Fa'`, at each step simultaneously perform the following operations:
```
replace 'a' with: 'aRbFR'
replace 'b' with: 'LFaLb'
```
For example (spaces added for more visibility) :
```
1st iteration: Fa -> F aRbF R
2nd iteration: FaRbFR -> F aRbFR R LFaLb FR
```
After `n` iteration, remove `'a'` and `'b'`. You will have a string with `'R'`,`'L'`, and `'F'`. This is a set of instruction. Starting at the origin of a grid looking in the `(0,1)` direction, `'F'` means a step forward, `'L'` and `'R'` mean respectively turn left and right. After executing all instructions, the trajectory will give a beautifull self-replicating pattern called 'Dragon Curve'
The goal of this kata is to code a function wich takes one parameter `n`, the number of iterations needed and return the string of instruction as defined above. For example:
```
n=0, should return: 'F'
n=1, should return: 'FRFR'
n=2, should return: 'FRFRRLFLFR'
```
`n` should be a number and non-negative integer. All other case should return the empty string: `''`.
| algorithms | def Dragon(n):
if type(n) != int or n < 0:
return ""
stg, a, b = "F{a}", "{a}R{b}FR", "LF{a}L{b}"
for _ in range(n):
stg = stg . format(a=a, b=b)
return stg . replace("{a}", ""). replace("{b}", "")
| Dragon's Curve | 53ad7224454985e4e8000eaa | [
"Mathematics",
"Recursion",
"Algorithms"
] | https://www.codewars.com/kata/53ad7224454985e4e8000eaa | 6 kyu |
The Ackermann function is a famous function that played a big role in computability theory as the first example of a total computable function that is not primitive recursive.
Since then the function has been a bit simplified but is still of good use. Due to its definition in terms of extremely deep recursion it can be used as a benchmark of a compiler's ability to optimize recursion.
The goal of this kata is to code a function which will be given two inputs, m and n, and will return the Ackermann number A(m,n) defined by:
```
A(m,n) = n+1 if m=0
A(m,n) = A(m-1,1) if m>0 , n=0
A(m,n) = A(m-1,A(m,n-1)) if m,n > 0
```
~~~if-not:c
`m`,`n` should be non-negative integers, the function should return null (Javascript), None (Python), or nil (Ruby) for other type, non-integer and negative numbers. In C, input is restricted to integer type.
~~~
~~~if:c
If m or n is negative, return -1.
~~~
| algorithms | from numbers import Number
def Ackermann(m, n):
if isinstance(n, Number) and isinstance(m, Number):
if m >= 0 and n >= 0:
return Ackermann_Aux(m, n)
return None
def Ackermann_Aux(m, n):
if m == 0:
return n + 1
if m > 0:
if n == 0:
return Ackermann_Aux(m - 1, 1)
if n > 0:
return Ackermann_Aux(m - 1, Ackermann_Aux(m, n - 1))
| Ackermann Function | 53ad69892a27079b34000bd9 | [
"Mathematics",
"Algorithms",
"Recursion"
] | https://www.codewars.com/kata/53ad69892a27079b34000bd9 | 6 kyu |
Mutual Recursion allows us to take the fun of regular recursion (where a function calls itself until a terminating condition) and apply it to multiple functions calling each other!
Let's use the Hofstadter Female and Male sequences to demonstrate this technique. You'll want to create two functions `F` and `M` such that the following equations are true:
```
F(0) = 1
M(0) = 0
F(n) = n - M(F(n - 1))
M(n) = n - F(M(n - 1))
```
Don't worry about negative numbers, `n` will always be greater than or equal to zero.
~~~if:php,csharp
You *do* have to worry about performance though, mutual recursion uses up a lot of stack space (and is highly inefficient) so you may have to find a way to make your solution consume less stack space (and time). Good luck :)
~~~
~~~if:lambdacalc
- Purity: `Let`
- Number Encoding: `Scott`
~~~
Hofstadter Wikipedia Reference http://en.wikipedia.org/wiki/Hofstadter_sequence#Hofstadter_Female_and_Male_sequences
| algorithms | def f(n): return n - m(f(n - 1)) if n else 1
def m(n): return n - f(m(n - 1)) if n else 0
| Mutual Recursion | 53a1eac7e0afd3ad3300008b | [
"Mathematics",
"Algorithms",
"Recursion"
] | https://www.codewars.com/kata/53a1eac7e0afd3ad3300008b | 6 kyu |
You are given a string of `n` lines, each substring being `n` characters long: For example:
`s = "abcd\nefgh\nijkl\nmnop"`
We will study some transformations of this square of strings.
- Symmetry with respect to the main cross diagonal: `diag_2_sym` (or `diag2Sym` or `diag-2-sym`)
```
diag_2_sym(s) => "plhd\nokgc\nnjfb\nmiea"
```
- Counterclockwise rotation 90 degrees: `rot_90_counter` (or `rot90Counter` or `rot-90-counter`)
```
rot_90_counter(s)=> "dhlp\ncgko\nbfjn\naeim"
```
- `selfie_diag2_counterclock` (or `selfieDiag2Counterclock` or `selfie-diag2-counterclock`)
It is initial string + string obtained by symmetry with respect to the main cross
diagonal + counterclockwise rotation 90 degrees .
```
s = "abcd\nefgh\nijkl\nmnop" -->
"abcd|plhd|dhlp\nefgh|okgc|cgko\nijkl|njfb|bfjn\nmnop|miea|aeim"
```
or printed for the last:
```
selfie_diag2_counterclock
abcd|plhd|dhlp
efgh|okgc|cgko
ijkl|njfb|bfjn
mnop|miea|aeim
```
#### Task
- Write these functions `diag_2_sym`, `rot_90_counter`, `selfie_diag2_counterclock`
and
- high-order function `oper(fct, s)` where `fct` is the function of one variable `f` to apply to the string `s` (`fct` will be one of `diag_2_sym`, `rot_90_counter`, `selfie_diag2_counterclock`)
#### Examples
```
s = "abcd\nefgh\nijkl\nmnop"
oper(diag_2_sym, s) => "plhd\nokgc\nnjfb\nmiea"
oper(rot_90_counter, s) => "dhlp\ncgko\nbfjn\naeim"
oper(selfie_diag2_counterclock, s) => "abcd|plhd|dhlp\nefgh|okgc|cgko\nijkl|njfb|bfjn\nmnop|miea|aeim"
```
#### Notes
- The form of the parameter `fct` in oper
changes according to the language. You can see each form according to the language in "Your test cases".
- It could be easier to take these katas from number (I) to number (IV)
- Bash Note: The ouput strings should be separated by \r instead of \n. See "Sample Tests".
| reference | def rot_90_counter(s): return list(zip(* s))[:: - 1]
def diag_2_sym(s): return list(zip(* s[:: - 1]))[:: - 1]
def selfie_diag2_counterclock(s): return (tuple(l) + ('|',) + m + ('|',) + r
for l, m, r in zip(s, diag_2_sym(s), rot_90_counter(s)))
def oper(func, s): return '\n' . join(map('' . join, func(s . split('\n'))))
| Moves in squared strings (IV) | 56dbf59b0a10feb08c000227 | [
"Algorithms",
"Strings"
] | https://www.codewars.com/kata/56dbf59b0a10feb08c000227 | 6 kyu |
You are given a string of `n` lines, each substring being `n` characters long: For example:
`s = "abcd\nefgh\nijkl\nmnop"`
We will study some transformations of this square of strings.
Let's now transform this string!
- Symmetry with respect to the main diagonal: diag_1_sym (or diag1Sym or diag-1-sym)
```
diag_1_sym(s) => "aeim\nbfjn\ncgko\ndhlp"
```
- Clockwise rotation 90 degrees: rot_90_clock (or rot90Clock or rot-90-clock)
```
rot_90_clock(s) => "miea\nnjfb\nokgc\nplhd"
```
- selfie_and_diag1(s) (or selfieAndDiag1 or selfie-and-diag1)
It is initial string + string obtained by symmetry with respect to the main diagonal.
```
s = "abcd\nefgh\nijkl\nmnop" -->
"abcd|aeim\nefgh|bfjn\nijkl|cgko\nmnop|dhlp"
```
or printed for the last:
```
selfie_and_diag1
abcd|aeim
efgh|bfjn
ijkl|cgko
mnop|dhlp
```
#### Task:
- Write these functions `diag_1_sym`, `rot_90_clock`, `selfie_and_diag1`
and
- high-order function `oper(fct, s)` where
- fct is the function of one variable f to apply to the string `s`
(fct will be one of `diag_1_sym`, `rot_90_clock`, `selfie_and_diag1`)
#### Examples:
```
s = "abcd\nefgh\nijkl\nmnop"
oper(diag_1_sym, s) => "aeim\nbfjn\ncgko\ndhlp"
oper(rot_90_clock, s) => "miea\nnjfb\nokgc\nplhd"
oper(selfie_and_diag1, s) => "abcd|aeim\nefgh|bfjn\nijkl|cgko\nmnop|dhlp"
```
#### Notes:
- The form of the parameter `fct` in oper
changes according to the language. You can see each form according to the language in "Your test cases".
- It could be easier to take these katas from number (I) to number (IV)
- Bash Note:
The output strings should be separated by \r instead of \n. See "Sample Tests".
| reference | def rot_90_clock(strng):
return '\n' . join('' . join(x) for x in zip(* strng . split('\n')[:: - 1]))
def diag_1_sym(strng):
return '\n' . join('' . join(x) for x in zip(* strng . split('\n')))
def selfie_and_diag1(strng):
return '\n' . join('|' . join(x) for x in zip(strng . split('\n'), diag_1_sym(strng). split('\n')))
def oper(fct, s):
return fct(s)
| Moves in squared strings (III) | 56dbeec613c2f63be4000be6 | [
"Algorithms",
"Strings"
] | https://www.codewars.com/kata/56dbeec613c2f63be4000be6 | 6 kyu |
# Be Concise I - The Ternary Operator
You are given a function ```describeAge``` / ```describe_age``` that takes a parameter ```age``` (which will always be a **positive integer**) and does the following:
1. If the age is ```12``` or lower, it ```return "You're a(n) kid"```
2. If the age is anything between ```13``` and ```17``` (inclusive), it ```return "You're a(n) teenager"```
3. If the age is anything between ```18``` and ```64``` (inclusive), it ```return "You're a(n) adult"```
4. If the age is ```65``` or above, it ```return "You're a(n) elderly"```
~~~if-not:python
Your task is to shorten the code as much as possible. Note that submitting the given code will not work because there is a character limit of **137**.
~~~
~~~if:python
Your task is to shorten the code as much as possible. Note that submitting the given code will not work because there is a character limit of **145**.
~~~
I'll give you a few hints:
1. The title itself is a hint - if you're not sure what to do, **always** research any terminology in this description that you have not heard of!
2. Don't you think the whole ```"You're a(n) <insert_something_here>"``` is very repetitive? ;) Perhaps we can shorten it?
3. Write everything in one line, `\n` and other whitespaces counts.
**Whatever you do, do not change what the function does.** Good luck :) | refactoring | def describe_age(a):
return f"You're a(n) { a < 13 and 'kid' or a < 18 and 'teenager' or a < 65 and 'adult' or 'elderly' } "
| Be Concise I - The Ternary Operator | 56f3f6a82010832b02000f38 | [
"Fundamentals",
"Refactoring"
] | https://www.codewars.com/kata/56f3f6a82010832b02000f38 | 8 kyu |
You are given a string of `n` lines, each substring being `n` characters long: For example:
`s = "abcd\nefgh\nijkl\nmnop"`
We will study some transformations of this square of strings.
- Clock rotation 180 degrees: rot
```
rot(s) => "ponm\nlkji\nhgfe\ndcba"
```
- selfie_and_rot(s) (or selfieAndRot or selfie-and-rot)
It is initial string + string obtained by clock rotation 180 degrees with dots interspersed in order (hopefully) to better show the rotation when printed.
```
s = "abcd\nefgh\nijkl\nmnop" -->
"abcd....\nefgh....\nijkl....\nmnop....\n....ponm\n....lkji\n....hgfe\n....dcba"
```
or printed:
```
|rotation |selfie_and_rot
|abcd --> ponm |abcd --> abcd....
|efgh lkji |efgh efgh....
|ijkl hgfe |ijkl ijkl....
|mnop dcba |mnop mnop....
....ponm
....lkji
....hgfe
....dcba
```
Notice that the *number of dots* is the *common length* of "abcd", "efgh", "ijkl", "mnop".
#### Task:
- Write these two functions `rot`and `selfie_and_rot`
and
- high-order function `oper(fct, s)` where
- fct is the function of one variable f to apply to the string `s`
(fct will be one of `rot, selfie_and_rot`)
#### Examples:
```
s = "abcd\nefgh\nijkl\nmnop"
oper(rot, s) => "ponm\nlkji\nhgfe\ndcba"
oper(selfie_and_rot, s) => "abcd....\nefgh....\nijkl....\nmnop....\n....ponm\n....lkji\n....hgfe\n....dcba"
```
#### Notes:
- The form of the parameter `fct` in oper
changes according to the language. You can see each form according to the language in "Your test cases".
- It could be easier to take these katas from number (I) to number (IV)
Forthcoming katas will study other tranformations.
#### Bash Note:
The input strings are separated by `,` instead of `\n`. The ouput strings should be separated by `\r` instead of `\n`. See "Sample Tests". | reference | def rot(string):
return string[:: - 1]
def selfie_and_rot(string):
s_dot = '\n' . join([s + '.' * len(s) for s in string . split('\n')])
return s_dot + '\n' + rot(s_dot)
def oper(fct, s):
return fct(s)
| Moves in squared strings (II) | 56dbe7f113c2f63570000b86 | [
"Algorithms",
"Strings"
] | https://www.codewars.com/kata/56dbe7f113c2f63570000b86 | 6 kyu |
There exists a sequence of numbers that follows the pattern
```
1
11
21
1211
111221
312211
13112221
1113213211
.
.
.
```
Starting with "1" the following lines are produced by "saying what you see", so that line two is "one one", line three is "two one(s)", line four is "one two one one".
Write a function that given a starting value as a string, returns the appropriate sequence as a list. The starting value can have any number of digits. The termination condition is a defined by the maximum number of iterations, also supplied as an argument. | algorithms | from itertools import groupby
def look_and_say(data='1', maxlen=5):
L = []
for i in range(maxlen):
data = "" . join(str(len(list(g))) + str(n) for n, g in groupby(data))
L . append(data)
return L
| Look and say numbers | 53ea07c9247bc3fcaa00084d | [
"Recursion",
"Algorithms"
] | https://www.codewars.com/kata/53ea07c9247bc3fcaa00084d | 6 kyu |
# The die is cast!
Your task in this kata is to write a "dice roller" that interprets a subset of [dice notation](http://en.wikipedia.org/wiki/Dice_notation).
# Description
In most role-playing games, die rolls required by the system are given in the form `AdX`. `A` and `X` are variables, separated by the letter **d**, which stands for *die* or *dice*.
- `A` is the number of dice to be rolled (usually omitted if 1).
- `X` is the number of faces of each die.
Here are some examples of input:
```javascript
'd6' => // One 6-sided die
'2d4' => // Two 4-sided dice
```
# Modifiers
As an addition to the above rules the input may also contain modifiers in the form `+N` or `-N` where `N` is an integer.
Here are some examples of input containing modifiers:
```javascript
'd3+4' => // One 3-sided die plus 4 (will be in range 5 -> 7)
'd3+4-1' => // One 3-sided die plus 4 minus 1 (will be in range 4 -> 6)
```
*Modifiers must be applied **after** all dice has been summed up.*
# Output
Your function must support two types of output depending on the second argument; *verbose* and *summed*.
## Summed output
If the verbose flag isn't set your function should sum up all the dice and modifiers and return the result as an integer.
## Verbose output
With the verbose flag your function should return an object/hash containing an array (`dice`) with all the dice rolls, and a integer (`modifier`) containing the sum of the modifiers which defaults to zero.
Example of verbose output:
```javascript
roll('3d7 +3 -2', true) // => { dice: [ 6, 4, 5 ], modifier: 1 }
```
# Invalid input
Here are some examples of invalid inputs:
```javascript
'' // Empty
{} // Not a string
'abc' // Not dice notation
'2d6+3 abc' // Contains extra bytes
'abc 2d6+3' // Contains extra bytes
'2d6++4' // Invalid modifiers
```
# Additional information
- Your solution should ignore all whitespace.
- `roll` should return `false` for invalid input. | algorithms | import re
import random
def roll(desc, verbose=False):
if not isinstance(desc, str):
return False
ans = re . findall(r'^(\d*)d(\d+)(([+\-]\d+)*)$', desc . replace(' ', ''))
if len(ans) == 0:
return False
dct = {i: eval(v) for i, v in enumerate(ans[0]) if v}
dices = {'dice': [1 + random . randrange(dct[1]) for i in range(dct . get(0, 1))],
'modifier': dct . get(2, 0)}
return dices if verbose else sum(dices['dice']) + dices['modifier']
| RPG dice roller | 549cb9c0c36a02ce2e000156 | [
"Regular Expressions",
"Arrays",
"Algorithms"
] | https://www.codewars.com/kata/549cb9c0c36a02ce2e000156 | 5 kyu |
This time we want to write calculations using functions and get the results. Let's have a look at some examples:
```javascript
seven(times(five())); // must return 35
four(plus(nine())); // must return 13
eight(minus(three())); // must return 5
six(dividedBy(two())); // must return 3
```
```haskell
seven $ times $ five -> 35 :: Int
four $ plus $ nine -> 13 :: Int
eight $ minus $ three -> 5 :: Int
six $ dividedBy $ two -> 3 :: Int
```
```ruby
seven(times(five)) # must return 35
four(plus(nine)) # must return 13
eight(minus(three)) # must return 5
six(divided_by(two)) # must return 3
```
```python
seven(times(five())) # must return 35
four(plus(nine())) # must return 13
eight(minus(three())) # must return 5
six(divided_by(two())) # must return 3
```
```factor
seven multiplied-by five ! must evaluate to 35
four plus nine ! must evaluate to 13
eight minus three ! must evaluate to 5
six divided-by two ! must evaluate to 3
```
Requirements:
~~~if:ruby,python
* There must be a function for each number from 0 ("zero") to 9 ("nine")
* There must be a function for each of the following mathematical operations: plus, minus, times, divided_by
* Each calculation consist of exactly one operation and two numbers
* The most outer function represents the left operand, the most inner function represents the right operand
* Division should be **integer division**. For example, this should return `2`, not `2.666666...`:
~~~
~~~if:factor
* There must be a word for each number from 0 ("zero") to 9 ("nine")
* There must be a word for each of the following mathematical operations: plus, minus, multiplied-by, divided-by
* Each calculation consist of exactly one operation and two numbers
* The leftmost word represents the left operand, the rightmost word represents the right operand
* Division should be **integer division**. For example, this should return `2`, not `2.666666...`:
~~~
~~~if-not:ruby,python,factor
* There must be a function for each number from 0 ("zero") to 9 ("nine")
* There must be a function for each of the following mathematical operations: plus, minus, times, dividedBy
* Each calculation consist of exactly one operation and two numbers
* The most outer function represents the left operand, the most inner function represents the right operand
* Division should be **integer division**. For example, this should return `2`, not `2.666666...`:
~~~
```javascript
eight(dividedBy(three()));
```
```haskell
eight $ dividedBy $ three
```
```ruby
eight(divided_by(three))
```
```python
eight(divided_by(three()))
```
```factor
eight divided-by three
``` | reference | def identity(a): return a
def zero(f=identity): return f(0)
def one(f=identity): return f(1)
def two(f=identity): return f(2)
def three(f=identity): return f(3)
def four(f=identity): return f(4)
def five(f=identity): return f(5)
def six(f=identity): return f(6)
def seven(f=identity): return f(7)
def eight(f=identity): return f(8)
def nine(f=identity): return f(9)
def plus(b): return lambda a: a + b
def minus(b): return lambda a: a - b
def times(b): return lambda a: a * b
def divided_by(b): return lambda a: a / / b
| Calculating with Functions | 525f3eda17c7cd9f9e000b39 | [
"Functional Programming"
] | https://www.codewars.com/kata/525f3eda17c7cd9f9e000b39 | 5 kyu |
You need to create a function that will validate if given parameters are valid geographical coordinates.
Valid coordinates look like the following: __"23.32353342, -32.543534534"__.
The return value should be either __true__ or __false__.
Latitude (which is first float) can be between 0 and 90, positive or negative.
Longitude (which is second float) can be between 0 and 180, positive or negative.
Coordinates can only contain digits, or one of the following symbols (including space after comma) __ -, . __
There should be no space between the minus "-" sign and the digit after it.
Here are some valid coordinates:
* -23, 25
* 24.53525235, 23.45235
* 04, -23.234235
* 43.91343345, 143
* 4, -3
And some invalid ones:
* 23.234, - 23.4234
* 2342.43536, 34.324236
* N23.43345, E32.6457
* 99.234, 12.324
* 6.325624, 43.34345.345
* 0, 1,2
* 0.342q0832, 1.2324 | algorithms | def is_valid_coordinates(coordinates):
try:
lat, lng = [abs(float(c))
for c in coordinates . split(',') if 'e' not in c]
except ValueError:
return False
return lat <= 90 and lng <= 180
| Coordinates Validator | 5269452810342858ec000951 | [
"Regular Expressions",
"Algorithms"
] | https://www.codewars.com/kata/5269452810342858ec000951 | 6 kyu |
I started this as a joke among friends, telling that converting numbers to other integer bases is for n00bs, while an actual coder at least converts numbers to more complex bases like [pi (or π or however you wish to spell it in your language)](http://en.wikipedia.org/wiki/Pi), so they dared me proving I was better.
And I did it in few hours, discovering that what I started as a joke actually has [some math ground and application (particularly the conversion to base pi, it seems)](http://en.wikipedia.org/wiki/Non-integer_representation).
That said, now I am daring you to do the same, that is to build a function so that it takes a **number** (any number, you are warned!) and optionally the **number of decimals** (default: 0) and a **base** (default: pi), returning the proper conversion **as a string**:
#Note
In Java, Rust and Go there is no easy way with optional parameters so all three parameters will be given; the same in C# because, as of now, the used version is not known.
```python
converter(13) #returns '103'
converter(13,3) #returns '103.010'
converter(-13,0,2) #returns '-1101'
```
```javascript
converter(13) //returns '103'
converter(13,3) //returns '103.010'
converter(-13,0,2) //returns '-1101'
```
```ruby
converter(13) #returns '103'
converter(13,3) #returns '103.010'
converter(-13,0,2) #returns '-1101'
```
```java
Converter.converter(13, 0, Math.PI) #returns '103'
Converter.converter(13, 3, Math.PI) #returns '103.010'
Converter.converter(-13, 0, 2) #returns '-1101'
```
```csharp
Converter.Convert(13, 0, Math.PI) #returns '103'
Converter.Convert(13, 3, Math.PI) #returns '103.010'
Converter.Convert(-13, 0, 2) #returns '-1101'
```
```rust
converter(13.0, 0, std::f64::consts::PI) => 103.0
converter(13.0, 3, std::f64::consts::PI) => 103.010
converter(-13.0, 0, 2.0) => -1101.0
```
```go
converter(13.0, 0, math.Pi) return 103.0
converter(13.0, 3, math.Pi) return 103.010
converter(-13.0, 0, 2.0) return -1101.0
```
```d
converter(13.0, 0, PI) => 103.0
converter(13.0, 3, PI) => 103.010
converter(-13.0, 0, 2.0) => -1101.0
```
I know most of the world uses a comma as a [decimal mark](http://en.wikipedia.org/wiki/Decimal_mark), but as English language and culture are *de facto* the Esperanto of us coders, we will stick to our common glorious traditions and uses, adopting the trivial dot (".") as decimal separator; if the absolute value of the result is <1, you have of course to put one (and only one) leading 0 before the decimal separator.
Finally, you may assume that decimals if provided will always be >= 0 and that no test base will be smaller than 2 (because, you know, converting to base 1 is pretty lame) or greater than 36; as usual, for digits greater than 9 you can use uppercase alphabet letter, so your base of numeration is going to be: '0123456789ABCDEFGHIJKLMNOPQRSTUVWXYZ'.
That is my first 3-languages-kata, so I count on you all to give me extensive feedback, no matter how harsh it may sound, so to improve myself even further :)
| algorithms | from math import pi, log
char = '0123456789ABCDEFGHIJKLMNOPQRSTUVWXYZ'
def converter(n, decimals=0, base=pi):
if n == 0:
return "0" if not decimals else "0." + "0" * decimals
res = "" if n > 0 else "-"
n = abs(n)
for q in xrange(int(log(n, base)), - decimals - 1, - 1):
if q == - 1:
res += "."
res += char[int(n / base * * q)]
n %= base * * q
return res
| Decimal to any Rational or Irrational Base Converter | 5509609d1dbf20a324000714 | [
"Mathematics",
"Fundamentals",
"Algorithms"
] | https://www.codewars.com/kata/5509609d1dbf20a324000714 | 4 kyu |
Write a function that will solve a 9x9 Sudoku puzzle. The function will take one argument consisting of the 2D puzzle array, with the value ```0``` representing an unknown square.
The Sudokus tested against your function will be "easy" (i.e. determinable; there will be no need to assume and test possibilities on unknowns) and can be solved with a brute-force approach.
For Sudoku rules, see [the Wikipedia article](http://en.wikipedia.org/wiki/Sudoku).
```python
puzzle = [[5,3,0,0,7,0,0,0,0],
[6,0,0,1,9,5,0,0,0],
[0,9,8,0,0,0,0,6,0],
[8,0,0,0,6,0,0,0,3],
[4,0,0,8,0,3,0,0,1],
[7,0,0,0,2,0,0,0,6],
[0,6,0,0,0,0,2,8,0],
[0,0,0,4,1,9,0,0,5],
[0,0,0,0,8,0,0,7,9]]
sudoku(puzzle)
# Should return
[[5,3,4,6,7,8,9,1,2],
[6,7,2,1,9,5,3,4,8],
[1,9,8,3,4,2,5,6,7],
[8,5,9,7,6,1,4,2,3],
[4,2,6,8,5,3,7,9,1],
[7,1,3,9,2,4,8,5,6],
[9,6,1,5,3,7,2,8,4],
[2,8,7,4,1,9,6,3,5],
[3,4,5,2,8,6,1,7,9]]
```
```javascript
var puzzle = [
[5,3,0,0,7,0,0,0,0],
[6,0,0,1,9,5,0,0,0],
[0,9,8,0,0,0,0,6,0],
[8,0,0,0,6,0,0,0,3],
[4,0,0,8,0,3,0,0,1],
[7,0,0,0,2,0,0,0,6],
[0,6,0,0,0,0,2,8,0],
[0,0,0,4,1,9,0,0,5],
[0,0,0,0,8,0,0,7,9]];
sudoku(puzzle);
/* Should return
[[5,3,4,6,7,8,9,1,2],
[6,7,2,1,9,5,3,4,8],
[1,9,8,3,4,2,5,6,7],
[8,5,9,7,6,1,4,2,3],
[4,2,6,8,5,3,7,9,1],
[7,1,3,9,2,4,8,5,6],
[9,6,1,5,3,7,2,8,4],
[2,8,7,4,1,9,6,3,5],
[3,4,5,2,8,6,1,7,9]]
```
```php
sudoku([
[5,3,0,0,7,0,0,0,0],
[6,0,0,1,9,5,0,0,0],
[0,9,8,0,0,0,0,6,0],
[8,0,0,0,6,0,0,0,3],
[4,0,0,8,0,3,0,0,1],
[7,0,0,0,2,0,0,0,6],
[0,6,0,0,0,0,2,8,0],
[0,0,0,4,1,9,0,0,5],
[0,0,0,0,8,0,0,7,9]
]); /* => [
[5,3,4,6,7,8,9,1,2],
[6,7,2,1,9,5,3,4,8],
[1,9,8,3,4,2,5,6,7],
[8,5,9,7,6,1,4,2,3],
[4,2,6,8,5,3,7,9,1],
[7,1,3,9,2,4,8,5,6],
[9,6,1,5,3,7,2,8,4],
[2,8,7,4,1,9,6,3,5],
[3,4,5,2,8,6,1,7,9]
] */
```
```haskell
puzzle = [[5,3,0,0,7,0,0,0,0],
[6,0,0,1,9,5,0,0,0],
[0,9,8,0,0,0,0,6,0],
[8,0,0,0,6,0,0,0,3],
[4,0,0,8,0,3,0,0,1],
[7,0,0,0,2,0,0,0,6],
[0,6,0,0,0,0,2,8,0],
[0,0,0,4,1,9,0,0,5],
[0,0,0,0,8,0,0,7,9]]
sudoku puzzle
{- Should return
[[5,3,4,6,7,8,9,1,2],
[6,7,2,1,9,5,3,4,8],
[1,9,8,3,4,2,5,6,7],
[8,5,9,7,6,1,4,2,3],
[4,2,6,8,5,3,7,9,1],
[7,1,3,9,2,4,8,5,6],
[9,6,1,5,3,7,2,8,4],
[2,8,7,4,1,9,6,3,5],
[3,4,5,2,8,6,1,7,9]]
-}
```
```rust
// puzzle before
puzzle = [
[5,3,0,0,7,0,0,0,0],
[6,0,0,1,9,5,0,0,0],
[0,9,8,0,0,0,0,6,0],
[8,0,0,0,6,0,0,0,3],
[4,0,0,8,0,3,0,0,1],
[7,0,0,0,2,0,0,0,6],
[0,6,0,0,0,0,2,8,0],
[0,0,0,4,1,9,0,0,5],
[0,0,0,0,8,0,0,7,9]
]
sudoku(&mut puzzle);
// puzzle after
puzzle == [
[5,3,4,6,7,8,9,1,2],
[6,7,2,1,9,5,3,4,8],
[1,9,8,3,4,2,5,6,7],
[8,5,9,7,6,1,4,2,3],
[4,2,6,8,5,3,7,9,1],
[7,1,3,9,2,4,8,5,6],
[9,6,1,5,3,7,2,8,4],
[2,8,7,4,1,9,6,3,5],
[3,4,5,2,8,6,1,7,9]
]
``` | algorithms | def sudoku(P):
for row, col in [(r, c) for r in range(9) for c in range(9) if not P[r][c]]:
rr, cc = (row / / 3) * 3, (col / / 3) * 3
use = {1, 2, 3, 4, 5, 6, 7, 8, 9} - ({P[row][c] for c in range(9)} | {P[r][col]
for r in range(9)} | {P[rr + r][cc + c] for r in range(3) for c in range(3)})
if len(use) == 1:
P[row][col] = use . pop()
return sudoku(P)
return P
| Sudoku Solver | 5296bc77afba8baa690002d7 | [
"Games",
"Game Solvers",
"Algorithms"
] | https://www.codewars.com/kata/5296bc77afba8baa690002d7 | 3 kyu |
# Task
Consider the following ciphering algorithm:
```
For each character replace it with its code.
Concatenate all of the obtained numbers.
```
Given a ciphered string, return the initial one if it is known that it consists only of lowercase letters.
Note: here the character's code means its `decimal ASCII code`, the numerical representation of a character used by most modern programming languages.
# Example
For `cipher = "10197115121"`, the output should be `"easy"`.
Explanation:
```
charCode('e') = 101,
charCode('a') = 97,
charCode('s') = 115
charCode('y') = 121.
```
# Input/Output
- `[input]` string `cipher`
A non-empty string which is guaranteed to be a cipher for some other string of lowercase letters.
- `[output]` a string | games | import re
def decipher(cipher):
return re . sub(r'1?\d\d', lambda m: chr(int(m . group())), cipher)
| Simple Fun #49: Decipher | 5888514674b58e929a000036 | [
"Puzzles"
] | https://www.codewars.com/kata/5888514674b58e929a000036 | 7 kyu |
# Kata Impossible I - The Impossible Lottery
## Overview and Background Story
One day, someone tells you that there exists a lottery very close to your home in which every participant is only required to pay ```5 British pounds``` per round but the winner of the lottery receives ```1000 trillion``` British pounds! Not believing this to be true, you decide to investigate the matter. After a period of careful investigation and confirmation (because you don't want to be tricked and fall into a trap), you come to the **depressing** conclusion that this lottery is indeed legitimate.
Now, why is this **depressing**? After all, ```1000 trillion British pounds``` is more money than you can ever wish for!
## Lottery Details
The lottery consists of exactly ```10``` numbers in an array. You only win the lottery **if all 10 numbers on your ```lotteryTicket``` match up exactly with the lottery results in the correct order**. For example:
```javascript
var lotteryTicket = [1,2,3,4,5,6,7,8,9,10]; // Your lottery ticket
var results = [11,12,13,14,15,16,17,18,19,20]; // Numbers do not match. Lottery lost
lotteryTicket = [1,2,3,4,5,6,7,8,9,10];
results = [10,9,8,7,6,5,4,3,2,1]; // Numbers not in the correct order. Lottery lost
lotteryTicket = [1,2,3,4,5,6,7,8,9,10];
results = [1,2,3,4,5,99,7,8,9,10]; // One number out of 10 does not match. Lottery lost
lotteryTicket = [1,2,3,4,5,6,7,8,9,10];
results = [1,2,3,4,5,6,7,8,9,10]; // All 10 numbers match up perfectly. Lottery won! You get 1000 trillion British pounds worth of money
```
Furthermore, each number in the lottery can vary from ```0``` to ```1000000``` (inclusive) and *numbers can be repeated*, although that is highly unlikely. Therefore the chances of winning this lottery is ```1 / ((10 ** 6) ** 10) === 1 / (10 ** 60)```. **No wonder no one has ever won the lottery.**
**However, with the power of code, nothing is impossible.** Good luck on the lottery! :D | games | class Ticket:
def __eq__(self, _):
return True
lottery_ticket = Ticket()
| Kata Impossible I - The Impossible Lottery | 56caf4a1145912a5c4000b76 | [
"Puzzles"
] | https://www.codewars.com/kata/56caf4a1145912a5c4000b76 | 6 kyu |
There's a new security company in Paris, and they decided to give their employees an algorithm to make first name recognition faster. In the blink of an eye, they can now detect if a string is a first name, no matter if it is a one-word name or an hyphenated name. They're given this documentation with the algorithm:
*In France, you'll often find people with hyphenated first names. They're called "prénoms composés".
There could be two, or even more words linked to form a new name, quite like jQuery function chaining ;).
They're linked using the - symbol, like Marie-Joelle, Jean-Michel, Jean-Mouloud.
Thanks to this algorithm, you can now recognize hyphenated names quicker than Flash !*
(yeah, their employees know how to use jQuery. Don't ask me why)
Your mission if you accept it, recreate the algorithm.
Using the function **showMe**, which takes a **yourID** argument, you will check if the given argument is a name or not, by returning true or false.
*Note that*
- String will either be a one-word first name, or an hyphenated first name , its words being linked by "-".
- Words can only start with an uppercase letter, and then lowercase letters (from a to z)
Now is your time to help the guards ! | reference | import re
def show_me(name):
return bool(re . match(r'(-[A-Z][a-z]+)+$', '-' + name))
| Sir , showMe yourID | 574c51aa3e4ea6de22001363 | [
"Regular Expressions",
"Strings",
"Fundamentals"
] | https://www.codewars.com/kata/574c51aa3e4ea6de22001363 | 7 kyu |
Linked Lists - Merge Sort
Write a MergeSort() function which recursively sorts a list in ascending order. Note that this problem requires recursion. Given FrontBackSplit() and SortedMerge(), you can write a classic recursive MergeSort(). Split the list into two smaller lists, recursively sort those lists, and finally merge the two sorted lists together into a single sorted list. Return the list.
```javascript
var list = 4 -> 2 -> 1 -> 3 -> 8 -> 9 -> null
mergeSort(list) === 1 -> 2 -> 3 -> 4 -> 8 -> 9 -> null
```
<a href="http://www.codewars.com/kata/linked-lists-front-back-split">FrontBackSplit()</a> and <a href="http://www.codewars.com/kata/linked-lists-sorted-merge">SortedMerge()</a> need not be redefined. You may call these functions in your solution.
These function names will depend on the accepted naming conventions of language you are using. In Python, FrontBackSplit() is actually front_back_split(). In JavaScript, it is frontBackSplit(), etc.
Related Kata in order of expected completion (increasing difficulty):<br>
<a href="http://www.codewars.com/kata/linked-lists-push-and-buildonetwothree">Linked Lists - Push & BuildOneTwoThree</a><br>
<a href="http://www.codewars.com/kata/linked-lists-length-and-count">Linked Lists - Length & Count</a><br>
<a href="http://www.codewars.com/kata/linked-lists-get-nth-node">Linked Lists - Get Nth Node</a><br>
<a href="http://www.codewars.com/kata/linked-lists-insert-nth-node">Linked Lists - Insert Nth Node</a><br>
<a href="http://www.codewars.com/kata/linked-lists-sorted-insert">Linked Lists - Sorted Insert</a><br>
<a href="http://www.codewars.com/kata/linked-lists-insert-sort">Linked Lists - Insert Sort</a><br>
<a href="http://www.codewars.com/kata/linked-lists-append">Linked Lists - Append</a><br>
<a href="http://www.codewars.com/kata/linked-lists-remove-duplicates">Linked Lists - Remove Duplicates</a><br>
<a href="http://www.codewars.com/kata/linked-lists-move-node">Linked Lists - Move Node</a><br>
<a href="http://www.codewars.com/kata/linked-lists-move-node-in-place">Linked Lists - Move Node In-place</a><br>
<a href="http://www.codewars.com/kata/linked-lists-alternating-split">Linked Lists - Alternating Split</a><br>
<a href="http://www.codewars.com/kata/linked-lists-front-back-split">Linked Lists - Front Back Split</a><br>
<a href="http://www.codewars.com/kata/linked-lists-shuffle-merge">Linked Lists - Shuffle Merge</a><br>
<a href="http://www.codewars.com/kata/linked-lists-sorted-merge">Linked Lists - Sorted Merge</a><br>
<a href="http://www.codewars.com/kata/linked-lists-merge-sort">Linked Lists - Merge Sort</a><br>
<a href="http://www.codewars.com/kata/linked-lists-sorted-intersect">Linked Lists - Sorted Intersect</a><br>
<a href="http://www.codewars.com/kata/linked-lists-iterative-reverse">Linked Lists - Iterative Reverse</a><br>
<a href="http://www.codewars.com/kata/linked-lists-recursive-reverse">Linked Lists - Recursive Reverse</a><br>
Inspired by Stanford Professor Nick Parlante's excellent [Linked List teachings.](http://cslibrary.stanford.edu/103/LinkedListBasics.pdf) | algorithms | class Node (object):
def __init__(self, data=None):
self . data = data
self . next = None
def merge_sort(list):
if not list or not list . next:
return list
front, back = Node(), Node()
front_back_split(list, front, back)
return sorted_merge(merge_sort(front), merge_sort(back))
| Linked Lists - Merge Sort | 55e5fa3501fd9c3f4d000050 | [
"Linked Lists",
"Data Structures",
"Algorithms",
"Sorting",
"Recursion"
] | https://www.codewars.com/kata/55e5fa3501fd9c3f4d000050 | 6 kyu |
Linked Lists - Shuffle Merge
Write a ShuffleMerge() function that takes two lists and merges their nodes together to make one list, taking nodes alternately between the two lists. So ShuffleMerge() with `1 -> 2 -> 3 -> null` and `7 -> 13 -> 1 -> null` should yield `1 -> 7 -> 2 -> 13 -> 3 -> 1 -> null`. If either list runs out, all the nodes should be taken from the other list. ShuffleMerge() should return the new list. The solution depends on being able to move nodes to the end of a list.
```javascript
var first = 3 -> 2 -> 8 -> null
var second = 5 -> 6 -> 1 -> 9 -> 11 -> null
shuffleMerge(first, second) === 3 -> 5 -> 2 -> 6 -> 8 -> 1 -> 9 -> 11 -> null
```
If one of the argument lists is null, the returned list should be the other linked list (even if it is also null). No errors need to be thrown in ShuffleMerge().
Related Kata in order of expected completion (increasing difficulty):<br>
<a href="http://www.codewars.com/kata/linked-lists-push-and-buildonetwothree">Linked Lists - Push & BuildOneTwoThree</a><br>
<a href="http://www.codewars.com/kata/linked-lists-length-and-count">Linked Lists - Length & Count</a><br>
<a href="http://www.codewars.com/kata/linked-lists-get-nth-node">Linked Lists - Get Nth Node</a><br>
<a href="http://www.codewars.com/kata/linked-lists-insert-nth-node">Linked Lists - Insert Nth Node</a><br>
<a href="http://www.codewars.com/kata/linked-lists-sorted-insert">Linked Lists - Sorted Insert</a><br>
<a href="http://www.codewars.com/kata/linked-lists-insert-sort">Linked Lists - Insert Sort</a><br>
<a href="http://www.codewars.com/kata/linked-lists-append">Linked Lists - Append</a><br>
<a href="http://www.codewars.com/kata/linked-lists-remove-duplicates">Linked Lists - Remove Duplicates</a><br>
<a href="http://www.codewars.com/kata/linked-lists-move-node">Linked Lists - Move Node</a><br>
<a href="http://www.codewars.com/kata/linked-lists-move-node-in-place">Linked Lists - Move Node In-place</a><br>
<a href="http://www.codewars.com/kata/linked-lists-alternating-split">Linked Lists - Alternating Split</a><br>
<a href="http://www.codewars.com/kata/linked-lists-front-back-split">Linked Lists - Front Back Split</a><br>
<a href="http://www.codewars.com/kata/linked-lists-shuffle-merge">Linked Lists - Shuffle Merge</a><br>
<a href="http://www.codewars.com/kata/linked-lists-sorted-merge">Linked Lists - Sorted Merge</a><br>
<a href="http://www.codewars.com/kata/linked-lists-merge-sort">Linked Lists - Merge Sort</a><br>
<a href="http://www.codewars.com/kata/linked-lists-sorted-intersect">Linked Lists - Sorted Intersect</a><br>
<a href="http://www.codewars.com/kata/linked-lists-iterative-reverse">Linked Lists - Iterative Reverse</a><br>
<a href="http://www.codewars.com/kata/linked-lists-recursive-reverse">Linked Lists - Recursive Reverse</a><br>
Inspired by Stanford Professor Nick Parlante's excellent [Linked List teachings.](http://cslibrary.stanford.edu/103/LinkedListBasics.pdf) | algorithms | class Node (object):
def __init__(self, data=None):
self . data = data
self . next = None
def shuffle_merge(first, second):
if not first:
return second
head = first
while second:
first . next, first, second = second, second, first . next
return head
| Linked Lists - Shuffle Merge | 55e5253dcd20f821c400008e | [
"Linked Lists",
"Data Structures",
"Algorithms"
] | https://www.codewars.com/kata/55e5253dcd20f821c400008e | 6 kyu |
# Generate user links
Your task is to create userlinks for the url, you will be given a username and must return a valid link.
## Example
```
generate_link('matt c')
http://www.codewars.com/users/matt%20c
```
### reference
use this as a reference [encoding](http://www.w3schools.com/tags/ref_urlencode.asp) | games | from urllib . parse import quote
def generate_link(user: str) - > str:
return f"http://www.codewars.com/users/ { quote ( user )} "
| Generate user links | 57037ed25a7263ac35000c80 | [
"Puzzles"
] | https://www.codewars.com/kata/57037ed25a7263ac35000c80 | 8 kyu |
For this exercise you will be strengthening your page-fu mastery. You will complete the PaginationHelper class, which is a utility class helpful for querying paging information related to an array.
The class is designed to take in an array of values and an integer indicating how many items will be allowed per each page. The types of values contained within the collection/array are not relevant.
The following are some examples of how this class is used:
```javascript
var helper = new PaginationHelper(['a','b','c','d','e','f'], 4);
helper.pageCount(); // should == 2
helper.itemCount(); // should == 6
helper.pageItemCount(0); // should == 4
helper.pageItemCount(1); // last page - should == 2
helper.pageItemCount(2); // should == -1 since the page is invalid
// pageIndex takes an item index and returns the page that it belongs on
helper.pageIndex(5); // should == 1 (zero based index)
helper.pageIndex(2); // should == 0
helper.pageIndex(20); // should == -1
helper.pageIndex(-10); // should == -1
```
```crystal
helper = PaginationHelper(Char).new(['a','b','c','d','e','f'], 4);
helper.page_count() # should == 2
helper.item_count() # should == 6
helper.page_item_count(0) # should == 4
helper.page_item_count(1) # last page - should == 2
helper.page_item_count(2) # should == -1 since the page is invalid
# pageIndex takes an item index and returns the page that it belongs on
helper.page_index(5) # should == 1 (zero based index)
helper.page_index(2) # should == 0
helper.page_index(20) # should == -1
helper.page_index(-10) # should == -1
```
```java
PaginationHelper<Character> helper = new PaginationHelper(Arrays.asList('a', 'b', 'c', 'd', 'e', 'f'), 4);
helper.pageCount(); // should == 2
helper.itemCount(); // should == 6
helper.pageItemCount(0); // should == 4
helper.pageItemCount(1); // last page - should == 2
helper.pageItemCount(2); // should == -1 since the page is invalid
// pageIndex takes an item index and returns the page that it belongs on
helper.pageIndex(5); // should == 1 (zero based index)
helper.pageIndex(2); // should == 0
helper.pageIndex(20); // should == -1
helper.pageIndex(-10); // should == -1
```
```coffeescript
helper = new PaginationHelper(['a','b','c','d','e','f'], 4)
helper.pageCount() # should == 2
helper.itemCount() # should == 6
helper.pageItemCount(0) # should == 4
helper.pageItemCount(1) # last page - should == 2
helper.pageItemCount(2) # should == -1 since the page is invalid
# pageIndex takes an item index and returns the page that it belongs on
helper.pageIndex(5) # should == 1 (zero based index)
helper.pageIndex(2) # should == 0
helper.pageIndex(20) # should == -1
helper.pageIndex(-10) # should == -1
```
```ruby
helper = PaginationHelper.new(['a','b','c','d','e','f'], 4)
helper.page_count() # should == 2
helper.item_count() # should == 6
helper.page_item_count(0) # should == 4
helper.page_item_count(1) # last page - should == 2
helper.page_item_count(2) # should == -1 since the page is invalid
# page_index takes an item index and returns the page that it belongs on
helper.page_index(5) # should == 1 (zero based index)
helper.page_index(2) # should == 0
helper.page_index(20) # should == -1
helper.page_index(-10) # should == -1 because negative indexes are invalid
```
```haskell
collection = ['a','b','c','d','e','f']
itemsPerPage = 4
pageCount collection itemsPerPage `shouldBe` 2
itemCount collection itemsPerPage `shouldBe` 6
pageItemCount collection itemsPerPage 0 `shouldBe` Just 4 -- four of six items
pageItemCount collection itemsPerPage 1 `shouldBe` Just 2 -- the last two items
pageItemCount collection itemsPerPage 3 `shouldBe` Nothing -- page doesn't exist
pageIndex collection itemsPerPage 0 `shouldBe` Just 0 -- zero based index
pageIndex collection itemsPerPage 5 `shouldBe` Just 1
pageIndex collection itemsPerPage 20 `shouldBe` Nothing
pageIndex collection itemsPerPage (-20) `shouldBe` Nothing
```
```python
helper = PaginationHelper(['a','b','c','d','e','f'], 4)
helper.page_count() # should == 2
helper.item_count() # should == 6
helper.page_item_count(0) # should == 4
helper.page_item_count(1) # last page - should == 2
helper.page_item_count(2) # should == -1 since the page is invalid
# page_index takes an item index and returns the page that it belongs on
helper.page_index(5) # should == 1 (zero based index)
helper.page_index(2) # should == 0
helper.page_index(20) # should == -1
helper.page_index(-10) # should == -1 because negative indexes are invalid
```
```csharp
var helper = new PaginationHelper<char>(new List<char>{'a', 'b', 'c', 'd', 'e', 'f'}, 4);
helper.PageCount; // should == 2
helper.ItemCount; // should == 6
helper.PageItemCount(0); // should == 4
helper.PageItemCount(1); // last page - should == 2
helper.PageItemCount(2); // should == -1 since the page is invalid
// pageIndex takes an item index and returns the page that it belongs on
helper.PageIndex(5); // should == 1 (zero based index)
helper.PageIndex(2); // should == 0
helper.PageIndex(20); // should == -1
helper.PageIndex(-10); // should == -1
```
```kotlin
val helper = PaginationHelper<Char>(listOf('a', 'b', 'c', 'd', 'e', 'f'), 4)
helper.pageCount // should == 2
helper.itemCount // should == 6
helper.pageItemCount(0) // should == 4
helper.pageItemCount(1) // last page - should == 2
helper.pageItemCount(2) // should == -1 since the page is invalid
// pageIndex takes an item index and returns the page that it belongs on
helper.pageIndex(5) // should == 1 (zero based index)
helper.pageIndex(2) // should == 0
helper.pageIndex(20) // should == -1
helper.pageIndex(-10) // should == -1
```
```typescript
let helper = new PaginationHelper(["a", "b", "c", "d", "e", "f"], 4)
helper.pageCount() // should == 2
helper.itemCount() // should == 6
helper.pageItemCount(0) // should == 4
helper.pageItemCount(1) // last page - should == 2
helper.pageItemCount(2) // should == -1 since the page is invalid
// pageIndex takes an item index and returns the page that it belongs on
helper.pageIndex(5) // should == 1 (zero based index)
helper.pageIndex(2) // should == 0
helper.pageIndex(20) // should == -1
helper.pageIndex(-10) // should == -1
```
```rust
let helper = PaginationHelper::new(vec!['a', 'b', 'c', 'd', 'e', 'f'], 4);
helper.page_count() // should be 2
helper.item_count() // should be 6
helper.page_item_count(0) // should be Some(4)
helper.page_item_count(1) // should be Some(2) (last page)
helper.page_item_count(2) // should be None (since the page is invalid)
// page_index takes an item index and returns the page that it belongs on
helper.page_index(5) // should be Some(1) (zero based index)
helper.page_index(2) // should be Some(0)
helper.page_index(20) // should be None (since the item is invalid)
```
| algorithms | class PaginationHelper:
def __init__(self, collection, items_per_page):
self . _item_count = len(collection)
self . items_per_page = items_per_page
def item_count(self):
return self . _item_count
def page_count(self):
return - (self . _item_count / / - self . items_per_page)
def page_item_count(self, page_index):
return min(self . items_per_page, self . _item_count - page_index * self . items_per_page) \
if 0 <= page_index < self . page_count() else - 1
def page_index(self, item_index):
return item_index / / self . items_per_page \
if 0 <= item_index < self . _item_count else - 1
| PaginationHelper | 515bb423de843ea99400000a | [
"Object-oriented Programming",
"Arrays",
"Algorithms"
] | https://www.codewars.com/kata/515bb423de843ea99400000a | 5 kyu |
Linked Lists - Front Back Split
Write a FrontBackSplit() function that takes one list and splits it into two sublists — one for the front half, and one for the back half. If the number of elements is odd, the extra element should go in the front list. For example, FrontBackSplit() on the list `2 -> 3 -> 5 -> 7 -> 11 -> null` should yield the two lists `2 -> 3 -> 5 -> null` and `7 -> 11 -> null`. Getting this right for all the cases is harder than it looks. You will probably need special case code to deal with lists of length < 2 cases.
```javascript
var source = 1 -> 3 -> 7 -> 8 -> 11 -> 12 -> 14 -> null
var front = new Node()
var back = new Node()
frontBackSplit(source, front, back)
front === 1 -> 3 -> 7 -> 8 -> null
back === 11 -> 12 -> 14 -> null
```
You should throw an error if any of the arguments to FrontBackSplit are null or if the source list has < 2 nodes.
Hint. Probably the simplest strategy is to compute the length of the list, then use a for loop to hop over the right number of nodes to find the last node of the front half, and then cut the list at that point. There is a trick technique that uses two pointers to traverse the list. A "slow" pointer advances one nodes at a time, while the "fast" pointer goes two nodes at a time. When the fast pointer reaches the end, the slow pointer will be about half way. For either strategy, care is required to split the list at the right point.
Related Kata in order of expected completion (increasing difficulty):<br>
<a href="http://www.codewars.com/kata/linked-lists-push-and-buildonetwothree">Linked Lists - Push & BuildOneTwoThree</a><br>
<a href="http://www.codewars.com/kata/linked-lists-length-and-count">Linked Lists - Length & Count</a><br>
<a href="http://www.codewars.com/kata/linked-lists-get-nth-node">Linked Lists - Get Nth Node</a><br>
<a href="http://www.codewars.com/kata/linked-lists-insert-nth-node">Linked Lists - Insert Nth Node</a><br>
<a href="http://www.codewars.com/kata/linked-lists-sorted-insert">Linked Lists - Sorted Insert</a><br>
<a href="http://www.codewars.com/kata/linked-lists-insert-sort">Linked Lists - Insert Sort</a><br>
<a href="http://www.codewars.com/kata/linked-lists-append">Linked Lists - Append</a><br>
<a href="http://www.codewars.com/kata/linked-lists-remove-duplicates">Linked Lists - Remove Duplicates</a><br>
<a href="http://www.codewars.com/kata/linked-lists-move-node">Linked Lists - Move Node</a><br>
<a href="http://www.codewars.com/kata/linked-lists-move-node-in-place">Linked Lists - Move Node In-place</a><br>
<a href="http://www.codewars.com/kata/linked-lists-alternating-split">Linked Lists - Alternating Split</a><br>
<a href="http://www.codewars.com/kata/linked-lists-front-back-split">Linked Lists - Front Back Split</a><br>
<a href="http://www.codewars.com/kata/linked-lists-shuffle-merge">Linked Lists - Shuffle Merge</a><br>
<a href="http://www.codewars.com/kata/linked-lists-sorted-merge">Linked Lists - Sorted Merge</a><br>
<a href="http://www.codewars.com/kata/linked-lists-merge-sort">Linked Lists - Merge Sort</a><br>
<a href="http://www.codewars.com/kata/linked-lists-sorted-intersect">Linked Lists - Sorted Intersect</a><br>
<a href="http://www.codewars.com/kata/linked-lists-iterative-reverse">Linked Lists - Iterative Reverse</a><br>
<a href="http://www.codewars.com/kata/linked-lists-recursive-reverse">Linked Lists - Recursive Reverse</a><br>
Inspired by Stanford Professor Nick Parlante's excellent [Linked List teachings.](http://cslibrary.stanford.edu/103/LinkedListBasics.pdf) | reference | class Node (object):
def __init__(self, data=None):
self . data = data
self . next = None
def front_back_split(source, front, back):
if not source or not source . next:
raise ValueError
if not source . next . next:
front . data, front . next = source . data, None
back . data, back . next = source . next . data, None
return
slowhead = fasthead = source
front . data, front . next = source . data, source . next
while fasthead . next and fasthead . next . next:
slowhead = slowhead . next
fasthead = fasthead . next . next
back . data = slowhead . next . data
back . next = slowhead . next . next
slowhead . next = None
| Linked Lists - Front Back Split | 55e1d2ba1a3229674d000037 | [
"Linked Lists",
"Data Structures",
"Fundamentals"
] | https://www.codewars.com/kata/55e1d2ba1a3229674d000037 | 5 kyu |
Linked Lists - Recursive Reverse
Write a **Recursive** Reverse() function that recursively reverses a linked list. You may want to use a nested function for the recursive calls.
```javascript
var list = 2 -> 1 -> 3 -> 6 -> 5 -> null
reverse(list) === 5 -> 6 -> 3 -> 1 -> 2 -> null
```
Related Kata in order of expected completion (increasing difficulty):<br>
<a href="http://www.codewars.com/kata/linked-lists-push-and-buildonetwothree">Linked Lists - Push & BuildOneTwoThree</a><br>
<a href="http://www.codewars.com/kata/linked-lists-length-and-count">Linked Lists - Length & Count</a><br>
<a href="http://www.codewars.com/kata/linked-lists-get-nth-node">Linked Lists - Get Nth Node</a><br>
<a href="http://www.codewars.com/kata/linked-lists-insert-nth-node">Linked Lists - Insert Nth Node</a><br>
<a href="http://www.codewars.com/kata/linked-lists-sorted-insert">Linked Lists - Sorted Insert</a><br>
<a href="http://www.codewars.com/kata/linked-lists-insert-sort">Linked Lists - Insert Sort</a><br>
<a href="http://www.codewars.com/kata/linked-lists-append">Linked Lists - Append</a><br>
<a href="http://www.codewars.com/kata/linked-lists-remove-duplicates">Linked Lists - Remove Duplicates</a><br>
<a href="http://www.codewars.com/kata/linked-lists-move-node">Linked Lists - Move Node</a><br>
<a href="http://www.codewars.com/kata/linked-lists-move-node-in-place">Linked Lists - Move Node In-place</a><br>
<a href="http://www.codewars.com/kata/linked-lists-alternating-split">Linked Lists - Alternating Split</a><br>
<a href="http://www.codewars.com/kata/linked-lists-front-back-split">Linked Lists - Front Back Split</a><br>
<a href="http://www.codewars.com/kata/linked-lists-shuffle-merge">Linked Lists - Shuffle Merge</a><br>
<a href="http://www.codewars.com/kata/linked-lists-sorted-merge">Linked Lists - Sorted Merge</a><br>
<a href="http://www.codewars.com/kata/linked-lists-merge-sort">Linked Lists - Merge Sort</a><br>
<a href="http://www.codewars.com/kata/linked-lists-sorted-intersect">Linked Lists - Sorted Intersect</a><br>
<a href="http://www.codewars.com/kata/linked-lists-iterative-reverse">Linked Lists - Iterative Reverse</a><br>
<a href="http://www.codewars.com/kata/linked-lists-recursive-reverse">Linked Lists - Recursive Reverse</a><br>
Inspired by Stanford Professor Nick Parlante's excellent [Linked List teachings.](http://cslibrary.stanford.edu/103/LinkedListBasics.pdf) | algorithms | class Node:
def __init__(self, data=None, next=None):
self . data = data
self . next = next
def reverse(head, tail=None):
return reverse(head . next, Node(head . data, tail)) if head else tail
| Linked Lists - Recursive Reverse | 55e725b930957a038a000042 | [
"Linked Lists",
"Data Structures",
"Algorithms"
] | https://www.codewars.com/kata/55e725b930957a038a000042 | 6 kyu |
Linked Lists - Iterative Reverse
Write an **iterative** Reverse() function that reverses a linked list. Ideally, Reverse() should only need to make one pass of the list.
```javascript
var list = 2 -> 1 -> 3 -> 6 -> 5 -> null
reverse(list)
list === 5 -> 6 -> 3 -> 1 -> 2 -> null
```
The push() and buildOneTwoThree() functions need not be redefined.
Related Kata in order of expected completion (increasing difficulty):<br>
<a href="http://www.codewars.com/kata/linked-lists-push-and-buildonetwothree">Linked Lists - Push & BuildOneTwoThree</a><br>
<a href="http://www.codewars.com/kata/linked-lists-length-and-count">Linked Lists - Length & Count</a><br>
<a href="http://www.codewars.com/kata/linked-lists-get-nth-node">Linked Lists - Get Nth Node</a><br>
<a href="http://www.codewars.com/kata/linked-lists-insert-nth-node">Linked Lists - Insert Nth Node</a><br>
<a href="http://www.codewars.com/kata/linked-lists-sorted-insert">Linked Lists - Sorted Insert</a><br>
<a href="http://www.codewars.com/kata/linked-lists-insert-sort">Linked Lists - Insert Sort</a><br>
<a href="http://www.codewars.com/kata/linked-lists-append">Linked Lists - Append</a><br>
<a href="http://www.codewars.com/kata/linked-lists-remove-duplicates">Linked Lists - Remove Duplicates</a><br>
<a href="http://www.codewars.com/kata/linked-lists-move-node">Linked Lists - Move Node</a><br>
<a href="http://www.codewars.com/kata/linked-lists-move-node-in-place">Linked Lists - Move Node In-place</a><br>
<a href="http://www.codewars.com/kata/linked-lists-alternating-split">Linked Lists - Alternating Split</a><br>
<a href="http://www.codewars.com/kata/linked-lists-front-back-split">Linked Lists - Front Back Split</a><br>
<a href="http://www.codewars.com/kata/linked-lists-shuffle-merge">Linked Lists - Shuffle Merge</a><br>
<a href="http://www.codewars.com/kata/linked-lists-sorted-merge">Linked Lists - Sorted Merge</a><br>
<a href="http://www.codewars.com/kata/linked-lists-merge-sort">Linked Lists - Merge Sort</a><br>
<a href="http://www.codewars.com/kata/linked-lists-sorted-intersect">Linked Lists - Sorted Intersect</a><br>
<a href="http://www.codewars.com/kata/linked-lists-iterative-reverse">Linked Lists - Iterative Reverse</a><br>
<a href="http://www.codewars.com/kata/linked-lists-recursive-reverse">Linked Lists - Recursive Reverse</a><br>
Inspired by Stanford Professor Nick Parlante's excellent [Linked List teachings.](http://cslibrary.stanford.edu/103/LinkedListBasics.pdf) | algorithms | class Node (object):
def __init__(self, data=None):
self . data = data
self . next = None
def reverse(head):
rev = None
current = head
while current:
rev = push(rev, current . data)
current = current . next
if head:
head . data = rev . data
head . next = rev . next
| Linked Lists - Iterative Reverse | 55e72695870aae78c4000026 | [
"Linked Lists",
"Data Structures",
"Algorithms"
] | https://www.codewars.com/kata/55e72695870aae78c4000026 | 6 kyu |
Linked Lists - Remove Duplicates
Write a RemoveDuplicates() function which takes a list sorted in increasing order and
deletes any duplicate nodes from the list. Ideally, the list should only be traversed once. The head of the resulting list should be returned.
```javascript
var list = 1 -> 2 -> 3 -> 3 -> 4 -> 4 -> 5 -> null
removeDuplicates(list) === 1 -> 2 -> 3 -> 4 -> 5 -> null
```
If the passed in list is null/None/nil, simply return null.
Note: Your solution is expected to work on long lists. Recursive solutions may fail due to stack size limitations.
The push() and buildOneTwoThree() functions need not be redefined.
Related Kata in order of expected completion (increasing difficulty):<br>
<a href="http://www.codewars.com/kata/linked-lists-push-and-buildonetwothree">Linked Lists - Push & BuildOneTwoThree</a><br>
<a href="http://www.codewars.com/kata/linked-lists-length-and-count">Linked Lists - Length & Count</a><br>
<a href="http://www.codewars.com/kata/linked-lists-get-nth-node">Linked Lists - Get Nth Node</a><br>
<a href="http://www.codewars.com/kata/linked-lists-insert-nth-node">Linked Lists - Insert Nth Node</a><br>
<a href="http://www.codewars.com/kata/linked-lists-sorted-insert">Linked Lists - Sorted Insert</a><br>
<a href="http://www.codewars.com/kata/linked-lists-insert-sort">Linked Lists - Insert Sort</a><br>
<a href="http://www.codewars.com/kata/linked-lists-append">Linked Lists - Append</a><br>
<a href="http://www.codewars.com/kata/linked-lists-remove-duplicates">Linked Lists - Remove Duplicates</a><br>
<a href="http://www.codewars.com/kata/linked-lists-move-node">Linked Lists - Move Node</a><br>
<a href="http://www.codewars.com/kata/linked-lists-move-node-in-place">Linked Lists - Move Node In-place</a><br>
<a href="http://www.codewars.com/kata/linked-lists-alternating-split">Linked Lists - Alternating Split</a><br>
<a href="http://www.codewars.com/kata/linked-lists-front-back-split">Linked Lists - Front Back Split</a><br>
<a href="http://www.codewars.com/kata/linked-lists-shuffle-merge">Linked Lists - Shuffle Merge</a><br>
<a href="http://www.codewars.com/kata/linked-lists-sorted-merge">Linked Lists - Sorted Merge</a><br>
<a href="http://www.codewars.com/kata/linked-lists-merge-sort">Linked Lists - Merge Sort</a><br>
<a href="http://www.codewars.com/kata/linked-lists-sorted-intersect">Linked Lists - Sorted Intersect</a><br>
<a href="http://www.codewars.com/kata/linked-lists-iterative-reverse">Linked Lists - Iterative Reverse</a><br>
<a href="http://www.codewars.com/kata/linked-lists-recursive-reverse">Linked Lists - Recursive Reverse</a><br>
Inspired by Stanford Professor Nick Parlante's excellent [Linked List teachings.](http://cslibrary.stanford.edu/103/LinkedListBasics.pdf) | reference | class Node (object):
def __init__(self, data):
self . data = data
self . next = None
def remove_duplicates(head):
if head == None:
return head
current = head
next = current . next
while next:
if current . data == next . data:
current . next = current . next . next
next = current . next
else:
current = next
next = current . next
return head
| Linked Lists - Remove Duplicates | 55d9f257d60c5fd98d00001b | [
"Linked Lists",
"Data Structures",
"Fundamentals"
] | https://www.codewars.com/kata/55d9f257d60c5fd98d00001b | 6 kyu |
Linked Lists - Insert Sort
Write an InsertSort() function which rearranges nodes in a linked list so they are sorted in increasing order. You can use the SortedInsert() function that you created in the "Linked Lists - Sorted Insert" kata below. The InsertSort() function takes the head of a linked list as an argument and must return the head of the linked list.
```javascript
var list = 4 -> 3 -> 1 -> 2 -> null
insertSort(list) === 1 -> 2 -> 3 -> 4 -> null
```
If the passed in head node is null or a single node, return null or the single node, respectively. You can assume that the head node will always be either null, a single node, or a linked list consisting of multiple nodes.
The push(), buildOneTwoThree(), and sortedInsert() functions need not be redefined.
Related Kata in order of expected completion (increasing difficulty):<br>
<a href="http://www.codewars.com/kata/linked-lists-push-and-buildonetwothree">Linked Lists - Push & BuildOneTwoThree</a><br>
<a href="http://www.codewars.com/kata/linked-lists-length-and-count">Linked Lists - Length & Count</a><br>
<a href="http://www.codewars.com/kata/linked-lists-get-nth-node">Linked Lists - Get Nth Node</a><br>
<a href="http://www.codewars.com/kata/linked-lists-insert-nth-node">Linked Lists - Insert Nth Node</a><br>
<a href="http://www.codewars.com/kata/linked-lists-sorted-insert">Linked Lists - Sorted Insert</a><br>
<a href="http://www.codewars.com/kata/linked-lists-insert-sort">Linked Lists - Insert Sort</a><br>
<a href="http://www.codewars.com/kata/linked-lists-append">Linked Lists - Append</a><br>
<a href="http://www.codewars.com/kata/linked-lists-remove-duplicates">Linked Lists - Remove Duplicates</a><br>
<a href="http://www.codewars.com/kata/linked-lists-move-node">Linked Lists - Move Node</a><br>
<a href="http://www.codewars.com/kata/linked-lists-move-node-in-place">Linked Lists - Move Node In-place</a><br>
<a href="http://www.codewars.com/kata/linked-lists-alternating-split">Linked Lists - Alternating Split</a><br>
<a href="http://www.codewars.com/kata/linked-lists-front-back-split">Linked Lists - Front Back Split</a><br>
<a href="http://www.codewars.com/kata/linked-lists-shuffle-merge">Linked Lists - Shuffle Merge</a><br>
<a href="http://www.codewars.com/kata/linked-lists-sorted-merge">Linked Lists - Sorted Merge</a><br>
<a href="http://www.codewars.com/kata/linked-lists-merge-sort">Linked Lists - Merge Sort</a><br>
<a href="http://www.codewars.com/kata/linked-lists-sorted-intersect">Linked Lists - Sorted Intersect</a><br>
<a href="http://www.codewars.com/kata/linked-lists-iterative-reverse">Linked Lists - Iterative Reverse</a><br>
<a href="http://www.codewars.com/kata/linked-lists-recursive-reverse">Linked Lists - Recursive Reverse</a><br>
Inspired by Stanford Professor Nick Parlante's excellent [Linked List teachings.](http://cslibrary.stanford.edu/103/LinkedListBasics.pdf) | reference | class Node (object):
def __init__(self, data, next=None):
self . data = data
self . next = next
def insert_sort(head):
n, r = head, None
while n:
r = sorted_insert(r, n . data)
n = n . next
return r
| Linked Lists - Insert Sort | 55d0c7ee7c0d30a12b000045 | [
"Linked Lists",
"Data Structures",
"Fundamentals",
"Sorting"
] | https://www.codewars.com/kata/55d0c7ee7c0d30a12b000045 | 6 kyu |
Linked Lists - Move Node
Write a MoveNode() function which takes the node from the front of the source list and moves it to the front of the destintation list. You should throw an error when the source list is empty. For simplicity, we use a Context object to store and return the state of the two linked lists. A Context object containing the two mutated lists should be returned by moveNode.
MoveNode() is a handy utility function to have for later problems.
### JavaScript
```javascript
var source = 1 -> 2 -> 3 -> null
var dest = 4 -> 5 -> 6 -> null
moveNode(source, dest).source === 2 -> 3 -> null
moveNode(source, dest).dest === 1 -> 4 -> 5 -> 6 -> null
```
### Python
```python
source = 1 -> 2 -> 3 -> None
dest = 4 -> 5 -> 6 -> None
move_node(source, dest).source == 2 -> 3 -> None
move_node(source, dest).dest == 1 -> 4 -> 5 -> 6 -> None
```
### Ruby
```ruby
source = 1 -> 2 -> 3 -> nil
dest = 4 -> 5 -> 6 -> nil
move_node(source, dest).source == 2 -> 3 -> nil
move_node(source, dest).dest == 1 -> 4 -> 5 -> 6 -> nil
```
The push() and buildOneTwoThree() functions need not be redefined.
There is another kata called <a href="http://www.codewars.com/kata/linked-lists-move-node-in-place">Linked Lists - Move Node In-place</a> that is related but more difficult.
Related Kata in order of expected completion (increasing difficulty):<br>
<a href="http://www.codewars.com/kata/linked-lists-push-and-buildonetwothree">Linked Lists - Push & BuildOneTwoThree</a><br>
<a href="http://www.codewars.com/kata/linked-lists-length-and-count">Linked Lists - Length & Count</a><br>
<a href="http://www.codewars.com/kata/linked-lists-get-nth-node">Linked Lists - Get Nth Node</a><br>
<a href="http://www.codewars.com/kata/linked-lists-insert-nth-node">Linked Lists - Insert Nth Node</a><br>
<a href="http://www.codewars.com/kata/linked-lists-sorted-insert">Linked Lists - Sorted Insert</a><br>
<a href="http://www.codewars.com/kata/linked-lists-insert-sort">Linked Lists - Insert Sort</a><br>
<a href="http://www.codewars.com/kata/linked-lists-append">Linked Lists - Append</a><br>
<a href="http://www.codewars.com/kata/linked-lists-remove-duplicates">Linked Lists - Remove Duplicates</a><br>
<a href="http://www.codewars.com/kata/linked-lists-move-node">Linked Lists - Move Node</a><br>
<a href="http://www.codewars.com/kata/linked-lists-move-node-in-place">Linked Lists - Move Node In-place</a><br>
<a href="http://www.codewars.com/kata/linked-lists-alternating-split">Linked Lists - Alternating Split</a><br>
<a href="http://www.codewars.com/kata/linked-lists-front-back-split">Linked Lists - Front Back Split</a><br>
<a href="http://www.codewars.com/kata/linked-lists-shuffle-merge">Linked Lists - Shuffle Merge</a><br>
<a href="http://www.codewars.com/kata/linked-lists-sorted-merge">Linked Lists - Sorted Merge</a><br>
<a href="http://www.codewars.com/kata/linked-lists-merge-sort">Linked Lists - Merge Sort</a><br>
<a href="http://www.codewars.com/kata/linked-lists-sorted-intersect">Linked Lists - Sorted Intersect</a><br>
<a href="http://www.codewars.com/kata/linked-lists-iterative-reverse">Linked Lists - Iterative Reverse</a><br>
<a href="http://www.codewars.com/kata/linked-lists-recursive-reverse">Linked Lists - Recursive Reverse</a><br>
Inspired by Stanford Professor Nick Parlante's excellent [Linked List teachings.](http://cslibrary.stanford.edu/103/LinkedListBasics.pdf) | reference | class Node (object):
def __init__(self, data, nxt=None):
self . data, self . next = data, nxt
class Context (object):
def __init__(self, source, dest):
self . source, self . dest = source, dest
def move_node(source, dest):
if source is None:
raise ValueError
return Context(source . next, Node(source . data, dest))
| Linked Lists - Move Node | 55da347204760ba494000038 | [
"Linked Lists",
"Data Structures",
"Fundamentals"
] | https://www.codewars.com/kata/55da347204760ba494000038 | 7 kyu |
Linked Lists - Alternating Split
Write an AlternatingSplit() function that takes one list and divides up its nodes to make two smaller lists. The sublists should be made from alternating elements in the original list. So if the original list is `a -> b -> a -> b -> a -> null` then one sublist should be `a -> a -> a -> null` and the other should be `b -> b -> null`.
```javascript
var list = 1 -> 2 -> 3 -> 4 -> 5 -> null
alternatingSplit(list).first === 1 -> 3 -> 5 -> null
alternatingSplit(list).second === 2 -> 4 -> null
```
```python
list = 1 -> 2 -> 3 -> 4 -> 5 -> None
alternating_split(list).first == 1 -> 3 -> 5 -> None
alternating_split(list).second == 2 -> 4 -> None
```
```ruby
list = 1 -> 2 -> 3 -> 4 -> 5 -> nil
alternating_split(list).first == 1 -> 3 -> 5 -> nil
alternating_split(list).second == 2 -> 4 -> nil
```
For simplicity, we use a Context object to store and return the state of the two linked lists. A Context object containing the two mutated lists should be returned by AlternatingSplit().
If the passed in head node is null/None/nil or a single node, throw an error.
Related Kata in order of expected completion (increasing difficulty):<br>
<a href="http://www.codewars.com/kata/linked-lists-push-and-buildonetwothree">Linked Lists - Push & BuildOneTwoThree</a><br>
<a href="http://www.codewars.com/kata/linked-lists-length-and-count">Linked Lists - Length & Count</a><br>
<a href="http://www.codewars.com/kata/linked-lists-get-nth-node">Linked Lists - Get Nth Node</a><br>
<a href="http://www.codewars.com/kata/linked-lists-insert-nth-node">Linked Lists - Insert Nth Node</a><br>
<a href="http://www.codewars.com/kata/linked-lists-sorted-insert">Linked Lists - Sorted Insert</a><br>
<a href="http://www.codewars.com/kata/linked-lists-insert-sort">Linked Lists - Insert Sort</a><br>
<a href="http://www.codewars.com/kata/linked-lists-append">Linked Lists - Append</a><br>
<a href="http://www.codewars.com/kata/linked-lists-remove-duplicates">Linked Lists - Remove Duplicates</a><br>
<a href="http://www.codewars.com/kata/linked-lists-move-node">Linked Lists - Move Node</a><br>
<a href="http://www.codewars.com/kata/linked-lists-move-node-in-place">Linked Lists - Move Node In-place</a><br>
<a href="http://www.codewars.com/kata/linked-lists-alternating-split">Linked Lists - Alternating Split</a><br>
<a href="http://www.codewars.com/kata/linked-lists-front-back-split">Linked Lists - Front Back Split</a><br>
<a href="http://www.codewars.com/kata/linked-lists-shuffle-merge">Linked Lists - Shuffle Merge</a><br>
<a href="http://www.codewars.com/kata/linked-lists-sorted-merge">Linked Lists - Sorted Merge</a><br>
<a href="http://www.codewars.com/kata/linked-lists-merge-sort">Linked Lists - Merge Sort</a><br>
<a href="http://www.codewars.com/kata/linked-lists-sorted-intersect">Linked Lists - Sorted Intersect</a><br>
<a href="http://www.codewars.com/kata/linked-lists-iterative-reverse">Linked Lists - Iterative Reverse</a><br>
<a href="http://www.codewars.com/kata/linked-lists-recursive-reverse">Linked Lists - Recursive Reverse</a><br>
Inspired by Stanford Professor Nick Parlante's excellent [Linked List teachings.](http://cslibrary.stanford.edu/103/LinkedListBasics.pdf) | reference | class Node (object):
def __init__(self, data=None):
self . data = data
self . next = None
class Context (object):
def __init__(self, first, second):
self . first = first
self . second = second
def alternating_split(head):
if head is None or head . next is None:
raise ValueError('Bad input')
orig_a, orig_b = a, b = Node(), Node()
while head:
a . next = Node(head . data)
a = a . next
a, b = b, a
head = head . next
return Context(orig_a . next, orig_b . next)
| Linked Lists - Alternating Split | 55dd5386575839a74f0000a9 | [
"Data Structures",
"Linked Lists",
"Fundamentals"
] | https://www.codewars.com/kata/55dd5386575839a74f0000a9 | 5 kyu |
Linked Lists - Append
Write an Append() function which appends one linked list to another. The head of the resulting list should be returned.
```javascript
var listA = 1 -> 2 -> 3 -> null
var listB = 4 -> 5 -> 6 -> null
append(listA, listB) === 1 -> 2 -> 3 -> 4 -> 5 -> 6 -> null
```
```coffeescript
listA = 1 -> 2 -> 3 -> null
listB = 4 -> 5 -> 6 -> null
append(listA, listB) == 1 -> 2 -> 3 -> 4 -> 5 -> 6 -> null
```
```php
$list_a = 1 -> 2 -> 3 -> NULL;
$list_b = 4 -> 5 -> 6 -> NULL;
append($list_a, $list_b) // returns 1 -> 2 -> 3 -> 4 -> 5 -> 6 -> NULL
```
```ruby
list_a = 1 -> 2 -> 3 -> nil
list_b = 4 -> 5 -> 6 -> nil
append(list_a, list_b) == 1 -> 2 -> 3 -> 4 -> 5 -> 6 -> nil
```
If both listA and listB are null/NULL/None/nil, return null/NULL/None/nil. If one list is null/NULL/None/nil and the other is not, simply return the non-null/NULL/None/nil list.
The push() and buildOneTwoThree() (`build_one_two_three()` in PHP and ruby) functions need not be redefined. The `Node` class is also predefined for you in PHP.
```php
/* PHP Only */
class Node {
public $data, $next;
public function __construct($data, $next = NULL) {
$this->data = $data;
$this->next = $next;
}
}
```
Related Kata in order of expected completion (increasing difficulty):<br>
<a href="http://www.codewars.com/kata/linked-lists-push-and-buildonetwothree">Linked Lists - Push & BuildOneTwoThree</a><br>
<a href="http://www.codewars.com/kata/linked-lists-length-and-count">Linked Lists - Length & Count</a><br>
<a href="http://www.codewars.com/kata/linked-lists-get-nth-node">Linked Lists - Get Nth Node</a><br>
<a href="http://www.codewars.com/kata/linked-lists-insert-nth-node">Linked Lists - Insert Nth Node</a><br>
<a href="http://www.codewars.com/kata/linked-lists-sorted-insert">Linked Lists - Sorted Insert</a><br>
<a href="http://www.codewars.com/kata/linked-lists-insert-sort">Linked Lists - Insert Sort</a><br>
<a href="http://www.codewars.com/kata/linked-lists-append">Linked Lists - Append</a><br>
<a href="http://www.codewars.com/kata/linked-lists-remove-duplicates">Linked Lists - Remove Duplicates</a><br>
<a href="http://www.codewars.com/kata/linked-lists-move-node">Linked Lists - Move Node</a><br>
<a href="http://www.codewars.com/kata/linked-lists-move-node-in-place">Linked Lists - Move Node In-place</a><br>
<a href="http://www.codewars.com/kata/linked-lists-alternating-split">Linked Lists - Alternating Split</a><br>
<a href="http://www.codewars.com/kata/linked-lists-front-back-split">Linked Lists - Front Back Split</a><br>
<a href="http://www.codewars.com/kata/linked-lists-shuffle-merge">Linked Lists - Shuffle Merge</a><br>
<a href="http://www.codewars.com/kata/linked-lists-sorted-merge">Linked Lists - Sorted Merge</a><br>
<a href="http://www.codewars.com/kata/linked-lists-merge-sort">Linked Lists - Merge Sort</a><br>
<a href="http://www.codewars.com/kata/linked-lists-sorted-intersect">Linked Lists - Sorted Intersect</a><br>
<a href="http://www.codewars.com/kata/linked-lists-iterative-reverse">Linked Lists - Iterative Reverse</a><br>
<a href="http://www.codewars.com/kata/linked-lists-recursive-reverse">Linked Lists - Recursive Reverse</a><br>
Inspired by Stanford Professor Nick Parlante's excellent [Linked List teachings.](http://cslibrary.stanford.edu/103/LinkedListBasics.pdf) | reference | class Node (object):
def __init__(self, data):
self . data = data
self . next = None
def last(head):
n = head
while n and n . next:
n = n . next
return n
def append(listA, listB):
if not listA:
return listB
last(listA). next = listB
return listA
| Linked Lists - Append | 55d17ddd6d7868493e000074 | [
"Linked Lists",
"Data Structures",
"Fundamentals"
] | https://www.codewars.com/kata/55d17ddd6d7868493e000074 | 7 kyu |
Implement the method **length**, which accepts a linked list (head), and returns the length of the list.
For example:
Given the list: `1 -> 2 -> 3 -> 4`, **length** should return 4.
The linked list is defined as follows:
```javascript
function Node(data, next = null) {
this.data = data;
this.next = next;
}
```
```java
class Node {
public Object data;
public Node next;
Node(T data, Node next) {
this.data = data;
this.next = next;
}
Node(T data) {
this(data, null);
}
}
```
```php
class Node {
public $data, $next;
public function __construct($data, $next = NULL) {
$this->data = $data;
$this->next = $next;
}
}
```
```python
class Node:
def __init__(self, data, next=None):
self.data = data
self.next = next
```
```cpp
template <class T>
class Node
{
public:
T data;
Node *next;
Node(T x, Node *p = NULL) : data(x), next(p) {}
Node() : next(NULL) {}
};
```
```c
typedef struct node_t {
void *data;
struct node_t *next;
} Node;
```
```nasm
typedef struct node_t {
void *data;
struct node_t *next;
} Node;
```
Note: the list may be null and can hold any type of value.
Good luck!
This kata is part of [fun with lists](https://www.codewars.com/collections/fun-with-lists) series:
* [Fun with lists: length](https://www.codewars.com/kata/581e476d5f59408553000a4b)
* [Fun with lists: indexOf](https://www.codewars.com/kata/581c6b075cfa83852700021f)
* [Fun with lists: lastIndexOf](https://www.codewars.com/kata/581c867a33b9fe732e000076)
* [Fun with lists: countIf](https://www.codewars.com/kata/5819081d056d4bdd410004f8)
* [Fun with lists: anyMatch + allMatch](https://www.codewars.com/kata/581e50555f59405743001813)
* [Fun with lists: filter](https://www.codewars.com/kata/582041237df353e01d000084)
* [Fun with lists: map](https://www.codewars.com/kata/58259d9062cfb45e1a00006b)
* [Fun with lists: reduce](https://www.codewars.com/kata/58319f37aeb69a89a00000c7) | reference | def length(head):
count = 0
while head != None:
count += 1
head = head . next
return count
| Fun with lists: length | 581e476d5f59408553000a4b | [
"Lists",
"Fundamentals"
] | https://www.codewars.com/kata/581e476d5f59408553000a4b | 7 kyu |
# Task
Given a string `str`, reverse it and omit all non-alphabetic characters.
# Example
For `str = "krishan"`, the output should be `"nahsirk"`.
For `str = "ultr53o?n"`, the output should be `"nortlu"`.
# Input/Output
- `[input]` string `str`
A string consists of lowercase latin letters, digits and symbols.
- `[output]` a string | reference | def reverse_letter(s):
return '' . join([i for i in s if i . isalpha()])[:: - 1]
| Simple Fun #176: Reverse Letter | 58b8c94b7df3f116eb00005b | [
"Fundamentals"
] | https://www.codewars.com/kata/58b8c94b7df3f116eb00005b | 7 kyu |
Linked Lists - Sorted Insert
Write a SortedInsert() function which inserts a node into the correct location of a pre-sorted linked list which is sorted in ascending order. SortedInsert takes the head of a linked list and data used to create a node as arguments. SortedInsert() should also return the head of the list.
```javascript
sortedInsert(1 -> 2 -> 3 -> null, 4) === 1 -> 2 -> 3 -> 4 -> null)
sortedInsert(1 -> 7 -> 8 -> null, 5) === 1 -> 5 -> 7 -> 8 -> null)
sortedInsert(3 -> 5 -> 9 -> null, 7) === 3 -> 5 -> 7 -> 9 -> null)
```
```coffeescript
sortedInsert(1 -> 2 -> 3 -> null, 4) === 1 -> 2 -> 3 -> 4 -> null)
sortedInsert(1 -> 7 -> 8 -> null, 5) === 1 -> 5 -> 7 -> 8 -> null)
sortedInsert(3 -> 5 -> 9 -> null, 7) === 3 -> 5 -> 7 -> 9 -> null)
```
The push() and buildOneTwoThree() functions do not need to be redefined.
Related Kata in order of expected completion (increasing difficulty):<br>
<a href="http://www.codewars.com/kata/linked-lists-push-and-buildonetwothree">Linked Lists - Push & BuildOneTwoThree</a><br>
<a href="http://www.codewars.com/kata/linked-lists-length-and-count">Linked Lists - Length & Count</a><br>
<a href="http://www.codewars.com/kata/linked-lists-get-nth-node">Linked Lists - Get Nth Node</a><br>
<a href="http://www.codewars.com/kata/linked-lists-insert-nth-node">Linked Lists - Insert Nth Node</a><br>
<a href="http://www.codewars.com/kata/linked-lists-sorted-insert">Linked Lists - Sorted Insert</a><br>
<a href="http://www.codewars.com/kata/linked-lists-insert-sort">Linked Lists - Insert Sort</a><br>
<a href="http://www.codewars.com/kata/linked-lists-append">Linked Lists - Append</a><br>
<a href="http://www.codewars.com/kata/linked-lists-remove-duplicates">Linked Lists - Remove Duplicates</a><br>
<a href="http://www.codewars.com/kata/linked-lists-move-node">Linked Lists - Move Node</a><br>
<a href="http://www.codewars.com/kata/linked-lists-move-node-in-place">Linked Lists - Move Node In-place</a><br>
<a href="http://www.codewars.com/kata/linked-lists-alternating-split">Linked Lists - Alternating Split</a><br>
<a href="http://www.codewars.com/kata/linked-lists-front-back-split">Linked Lists - Front Back Split</a><br>
<a href="http://www.codewars.com/kata/linked-lists-shuffle-merge">Linked Lists - Shuffle Merge</a><br>
<a href="http://www.codewars.com/kata/linked-lists-sorted-merge">Linked Lists - Sorted Merge</a><br>
<a href="http://www.codewars.com/kata/linked-lists-merge-sort">Linked Lists - Merge Sort</a><br>
<a href="http://www.codewars.com/kata/linked-lists-sorted-intersect">Linked Lists - Sorted Intersect</a><br>
<a href="http://www.codewars.com/kata/linked-lists-iterative-reverse">Linked Lists - Iterative Reverse</a><br>
<a href="http://www.codewars.com/kata/linked-lists-recursive-reverse">Linked Lists - Recursive Reverse</a><br>
Inspired by Stanford Professor Nick Parlante's excellent [Linked List teachings.](http://cslibrary.stanford.edu/103/LinkedListBasics.pdf) | reference | class Node (object):
def __init__(self, data, nxt=None):
self . data = data
self . next = nxt
def sorted_insert(head, data):
if not head or data < head . data:
return Node(data, head)
else:
head . next = sorted_insert(head . next, data)
return head
| Linked Lists - Sorted Insert | 55cc33e97259667a08000044 | [
"Linked Lists",
"Data Structures",
"Fundamentals"
] | https://www.codewars.com/kata/55cc33e97259667a08000044 | 6 kyu |
Linked Lists - Sorted Intersect
Write a SortedIntersect() function which creates and returns a list representing the intersection of two lists that are sorted in increasing order. Ideally, each list should only be traversed once. The resulting list should not contain duplicates.
```javascript
var first = 1 -> 2 -> 2 -> 3 -> 3 -> 6 -> null
var second = 1 -> 3 -> 4 -> 5 -> -> 6 -> null
sortedIntersect(first, second) === 1 -> 3 -> 6 -> null
```
Related Kata in order of expected completion (increasing difficulty):<br>
<a href="http://www.codewars.com/kata/linked-lists-push-and-buildonetwothree">Linked Lists - Push & BuildOneTwoThree</a><br>
<a href="http://www.codewars.com/kata/linked-lists-length-and-count">Linked Lists - Length & Count</a><br>
<a href="http://www.codewars.com/kata/linked-lists-get-nth-node">Linked Lists - Get Nth Node</a><br>
<a href="http://www.codewars.com/kata/linked-lists-insert-nth-node">Linked Lists - Insert Nth Node</a><br>
<a href="http://www.codewars.com/kata/linked-lists-sorted-insert">Linked Lists - Sorted Insert</a><br>
<a href="http://www.codewars.com/kata/linked-lists-insert-sort">Linked Lists - Insert Sort</a><br>
<a href="http://www.codewars.com/kata/linked-lists-append">Linked Lists - Append</a><br>
<a href="http://www.codewars.com/kata/linked-lists-remove-duplicates">Linked Lists - Remove Duplicates</a><br>
<a href="http://www.codewars.com/kata/linked-lists-move-node">Linked Lists - Move Node</a><br>
<a href="http://www.codewars.com/kata/linked-lists-move-node-in-place">Linked Lists - Move Node In-place</a><br>
<a href="http://www.codewars.com/kata/linked-lists-alternating-split">Linked Lists - Alternating Split</a><br>
<a href="http://www.codewars.com/kata/linked-lists-front-back-split">Linked Lists - Front Back Split</a><br>
<a href="http://www.codewars.com/kata/linked-lists-shuffle-merge">Linked Lists - Shuffle Merge</a><br>
<a href="http://www.codewars.com/kata/linked-lists-sorted-merge">Linked Lists - Sorted Merge</a><br>
<a href="http://www.codewars.com/kata/linked-lists-merge-sort">Linked Lists - Merge Sort</a><br>
<a href="http://www.codewars.com/kata/linked-lists-sorted-intersect">Linked Lists - Sorted Intersect</a><br>
<a href="http://www.codewars.com/kata/linked-lists-iterative-reverse">Linked Lists - Iterative Reverse</a><br>
<a href="http://www.codewars.com/kata/linked-lists-recursive-reverse">Linked Lists - Recursive Reverse</a><br>
Inspired by Stanford Professor Nick Parlante's excellent [Linked List teachings.](http://cslibrary.stanford.edu/103/LinkedListBasics.pdf) | algorithms | class Node (object):
def __init__(self, data=None, nxt=None):
self . data, self . next = data, nxt
# For so little text, the cyclomatic complexity here is pretty staggering
def sorted_intersect(first, second):
if not first or not second:
return None
if first . next and first . data == first . next . data:
return sorted_intersect(first . next, second)
if second . next and second . data == second . next . data:
return sorted_intersect(first, second . next)
if first . data == second . data:
return Node(first . data, sorted_intersect(first . next, second . next))
return sorted_intersect(first . next, second) if first . data < second . data else sorted_intersect(first, second . next)
| Linked Lists - Sorted Intersect | 55e67e44bf97fa66900000a0 | [
"Linked Lists",
"Data Structures",
"Algorithms"
] | https://www.codewars.com/kata/55e67e44bf97fa66900000a0 | 6 kyu |
Linked Lists - Sorted Merge
Write a SortedMerge() function that takes two lists, each of which is sorted in increasing order, and merges the two together into one list which is in increasing order. SortedMerge() should return the new list. The new list should be made by splicing together the nodes of the first two lists. Ideally, SortedMerge() should only make one pass through each list. SortedMerge() is tricky to get right and it may be solved iteratively or recursively.
```javascript
var first = 2 -> 4 -> 6 -> 7 -> null
var second = 1 -> 3 -> 5 -> 6 -> 8 -> null
sortedMerge(first, second) === 1 -> 2 -> 3 -> 4 -> 5 -> 6 -> 6 -> 7 -> 8 -> null
```
There are many cases to deal with: either 'first' or 'second' may be null/None/nil, during processing either 'first' or 'second' may run out first, and finally there's the problem of starting the result list empty, and building it up while going through 'first' and 'second'.
If one of the argument lists is null, the returned list should be the other linked list (even if it is also null). No errors need to be thrown in SortedMerge().
Try doing <a href="http://www.codewars.com/kata/linked-lists-shuffle-merge">Linked Lists - Shuffle Merge</a> before attempting this problem.
Related Kata in order of expected completion (increasing difficulty):<br>
<a href="http://www.codewars.com/kata/linked-lists-push-and-buildonetwothree">Linked Lists - Push & BuildOneTwoThree</a><br>
<a href="http://www.codewars.com/kata/linked-lists-length-and-count">Linked Lists - Length & Count</a><br>
<a href="http://www.codewars.com/kata/linked-lists-get-nth-node">Linked Lists - Get Nth Node</a><br>
<a href="http://www.codewars.com/kata/linked-lists-insert-nth-node">Linked Lists - Insert Nth Node</a><br>
<a href="http://www.codewars.com/kata/linked-lists-sorted-insert">Linked Lists - Sorted Insert</a><br>
<a href="http://www.codewars.com/kata/linked-lists-insert-sort">Linked Lists - Insert Sort</a><br>
<a href="http://www.codewars.com/kata/linked-lists-append">Linked Lists - Append</a><br>
<a href="http://www.codewars.com/kata/linked-lists-remove-duplicates">Linked Lists - Remove Duplicates</a><br>
<a href="http://www.codewars.com/kata/linked-lists-move-node">Linked Lists - Move Node</a><br>
<a href="http://www.codewars.com/kata/linked-lists-move-node-in-place">Linked Lists - Move Node In-place</a><br>
<a href="http://www.codewars.com/kata/linked-lists-alternating-split">Linked Lists - Alternating Split</a><br>
<a href="http://www.codewars.com/kata/linked-lists-front-back-split">Linked Lists - Front Back Split</a><br>
<a href="http://www.codewars.com/kata/linked-lists-shuffle-merge">Linked Lists - Shuffle Merge</a><br>
<a href="http://www.codewars.com/kata/linked-lists-sorted-merge">Linked Lists - Sorted Merge</a><br>
<a href="http://www.codewars.com/kata/linked-lists-merge-sort">Linked Lists - Merge Sort</a><br>
<a href="http://www.codewars.com/kata/linked-lists-sorted-intersect">Linked Lists - Sorted Intersect</a><br>
<a href="http://www.codewars.com/kata/linked-lists-iterative-reverse">Linked Lists - Iterative Reverse</a><br>
<a href="http://www.codewars.com/kata/linked-lists-recursive-reverse">Linked Lists - Recursive Reverse</a><br>
Inspired by Stanford Professor Nick Parlante's excellent [Linked List teachings.](http://cslibrary.stanford.edu/103/LinkedListBasics.pdf) | algorithms | class Node (object):
def __init__(self, data=None, nxt=None):
self . data, self . next = data, nxt
def sorted_merge(first, second):
if not first:
return second
if not second:
return first
return Node(first . data, sorted_merge(first . next, second)) if first . data < second . data else Node(second . data, sorted_merge(first, second . next))
| Linked Lists - Sorted Merge | 55e5d31bf7ca1e44980000a7 | [
"Linked Lists",
"Data Structures",
"Algorithms"
] | https://www.codewars.com/kata/55e5d31bf7ca1e44980000a7 | 6 kyu |
John and Mary want to travel between a few towns A, B, C ... Mary has on a sheet of paper a list of distances between these towns.
`ls = [50, 55, 57, 58, 60]`.
John is tired of driving and he says to Mary that he doesn't want to drive more than `t = 174 miles` and he
will visit only `3` towns.
Which distances, hence which towns, they will choose so that the sum of the distances is the biggest possible to please Mary and John?
#### Example:
With list `ls` and 3 towns to visit they can make a choice between:
`[50,55,57],[50,55,58],[50,55,60],[50,57,58],[50,57,60],[50,58,60],[55,57,58],[55,57,60],[55,58,60],[57,58,60]`.
The sums of distances are then:
`162, 163, 165, 165, 167, 168, 170, 172, 173, 175`.
The biggest possible sum taking a limit of `174` into account is then `173` and the distances of the `3`
corresponding towns is `[55, 58, 60]`.
The function `chooseBestSum` (or `choose_best_sum` or ... depending on the language) will take as parameters `t` (maximum sum of distances, integer >= 0), `k` (number of towns to visit, k >= 1)
and `ls` (list of distances, all distances are positive or zero integers and this list has at least one element).
The function returns the "best" sum ie the biggest possible sum of `k` distances less than or equal to the given limit `t`, if that sum exists,
or otherwise nil, null, None, Nothing, depending on the language. In that case with C, C++, D, Dart, Fortran, F#, Go, Julia, Kotlin, Nim, OCaml, Pascal, Perl, PowerShell, Reason, Rust, Scala, Shell, Swift return `-1`.
#### Examples:
`ts = [50, 55, 56, 57, 58]`
`choose_best_sum(163, 3, ts) -> 163`
`xs = [50]`
`choose_best_sum(163, 3, xs) -> nil (or null or ... or -1 (C++, C, D, Rust, Swift, Go, ...)`
`ys = [91, 74, 73, 85, 73, 81, 87]`
`choose_best_sum(230, 3, ys) -> 228`
#### Notes:
- try not to modify the input list of distances `ls`
- in some languages this "list" is in fact a *string* (see the Sample Tests).
| reference | import itertools
def choose_best_sum(t, k, ls):
try:
return max(sum(i) for i in itertools . combinations(ls, k) if sum(i) <= t)
except:
return None
| Best travel | 55e7280b40e1c4a06d0000aa | [
"Fundamentals"
] | https://www.codewars.com/kata/55e7280b40e1c4a06d0000aa | 5 kyu |
## Introduction
<pre style="white-space: pre-wrap;white-space: -moz-pre-wrap;white-space: -pre-wrap;white-space: -o-pre-wrap;word-wrap: break-word;">
We all love to play games especially as children. I have fond memories playing Connect 4 with my brother so decided to create this Kata based on the classic game. Connect 4 is known as several names such as “Four in a Row” and “Captain’s Mistress" but was made popular by Hasbro MB Games
</pre>
<center><img src="https://raw.githubusercontent.com/adrianeyre/codewars/master/Ruby/Authored/connect4.jpg" alt="Connect 4"></center>
## Task
<pre style="white-space: pre-wrap;white-space: -moz-pre-wrap;white-space: -pre-wrap;white-space: -o-pre-wrap;word-wrap: break-word;">
The game consists of a grid (7 columns and 6 rows) and two players that take turns to drop their discs. The pieces fall straight down, occupying the next available space within the column. The objective of the game is to be the first to form a horizontal, vertical, or diagonal line of four of one's own discs.
Your task is to create a Class called <span style="color:#A1A85E">Connect4</span> that has a method called <span style="color:#A1A85E">play</span> which takes one argument for the column where the player is going to place their disc.
</pre>
## Rules
<pre style="white-space: pre-wrap;white-space: -moz-pre-wrap;white-space: -pre-wrap;white-space: -o-pre-wrap;word-wrap: break-word;">
If a player successfully has 4 discs horizontally, vertically or diagonally then you should return "<span style="color:#A1A85E">Player n wins!</span>” where n is the current player either 1 or 2.
If a player attempts to place a disc in a column that is full then you should return ”<span style="color:#A1A85E">Column full!</span>” and the next move must be taken by the same player.
If the game has been won by a player, any following moves should return ”<span style="color:#A1A85E">Game has finished!</span>”.
Any other move should return ”<span style="color:#A1A85E">Player n has a turn</span>” where n is the current player either 1 or 2.
Player 1 starts the game every time and alternates with player 2.
The columns are numbered <span style="color:#A1A85E">0-6</span> left to right.
</pre>
Good luck and enjoy!
## Kata Series
If you enjoyed this, then please try one of my other Katas. Any feedback, translations and grading of beta Katas are greatly appreciated. Thank you.
<span style="display: flex !important;"><img style="margin:0px;" src="https://raw.githubusercontent.com/adrianeyre/codewars/master/Ruby/Authored/6KYU.png" alt="Rank"/> <a href="https://www.codewars.com/kata/58663693b359c4a6560001d6" target="_blank">Maze Runner</a></span>
<span style="display: flex !important;"><img style="margin:0px;" src="https://raw.githubusercontent.com/adrianeyre/codewars/master/Ruby/Authored/6KYU.png" alt="Rank"/> <a href="https://www.codewars.com/kata/58693bbfd7da144164000d05" target="_blank">Scooby Doo Puzzle</a></span>
<span style="display: flex !important;"><img style="margin:0px;" src="https://raw.githubusercontent.com/adrianeyre/codewars/master/Ruby/Authored/7KYU.png" alt="Rank"/> <a href="https://www.codewars.com/kata/586a1af1c66d18ad81000134" target="_blank">Driving License</a></span>
<span style="display: flex !important;"><img style="margin:0px;" src="https://raw.githubusercontent.com/adrianeyre/codewars/master/Ruby/Authored/6KYU.png" alt="Rank"/> <a href="https://www.codewars.com/kata/586c0909c1923fdb89002031" target="_blank">Connect 4</a></span>
<span style="display: flex !important;"><img style="margin:0px;" src="https://raw.githubusercontent.com/adrianeyre/codewars/master/Ruby/Authored/6KYU.png" alt="Rank"/> <a href="https://www.codewars.com/kata/586e6d4cb98de09e3800014f" target="_blank">Vending Machine</a></span>
<span style="display: flex !important;"><img style="margin:0px;" src="https://raw.githubusercontent.com/adrianeyre/codewars/master/Ruby/Authored/6KYU.png" alt="Rank"/> <a href="https://www.codewars.com/kata/587136ba2eefcb92a9000027" target="_blank">Snakes and Ladders</a></span>
<span style="display: flex !important;"><img style="margin:0px;" src="https://raw.githubusercontent.com/adrianeyre/codewars/master/Ruby/Authored/6KYU.png" alt="Rank"/> <a href="https://www.codewars.com/kata/58a848258a6909dd35000003" target="_blank">Mastermind</a></span>
<span style="display: flex !important;"><img style="margin:0px;" src="https://raw.githubusercontent.com/adrianeyre/codewars/master/Ruby/Authored/6KYU.png" alt="Rank"/> <a href="https://www.codewars.com/kata/58b2c5de4cf8b90723000051" target="_blank">Guess Who?</a></span>
<span style="display: flex !important;"><img style="margin:0px;" src="https://raw.githubusercontent.com/adrianeyre/codewars/master/Ruby/Authored/6KYU.png" alt="Rank"/> <a href="https://www.codewars.com/kata/58f5c63f1e26ecda7e000029" target="_blank">Am I safe to drive?</a></span>
<span style="display: flex !important;"><img style="margin:0px;" src="https://raw.githubusercontent.com/adrianeyre/codewars/master/Ruby/Authored/6KYU.png" alt="Rank"/> <a href="https://www.codewars.com/kata/58f5c63f1e26ecda7e000029" target="_blank">Mexican Wave</a></span>
<span style="display: flex !important;"><img style="margin:0px;" src="https://raw.githubusercontent.com/adrianeyre/codewars/master/Ruby/Authored/6KYU.png" alt="Rank"/> <a href="https://www.codewars.com/kata/58fdcc51b4f81a0b1e00003e" target="_blank">Pigs in a Pen</a></span>
<span style="display: flex !important;"><img style="margin:0px;" src="https://raw.githubusercontent.com/adrianeyre/codewars/master/Ruby/Authored/6KYU.png" alt="Rank"/> <a href="https://www.codewars.com/kata/590300eb378a9282ba000095" target="_blank">Hungry Hippos</a></span>
<span style="display: flex !important;"><img style="margin:0px;" src="https://raw.githubusercontent.com/adrianeyre/codewars/master/Ruby/Authored/6KYU.png" alt="Rank"/> <a href="https://www.codewars.com/kata/5904be220881cb68be00007d" target="_blank">Plenty of Fish in the Pond</a></span>
<span style="display: flex !important;"><img style="margin:0px;" src="https://raw.githubusercontent.com/adrianeyre/codewars/master/Ruby/Authored/6KYU.png" alt="Rank"/> <a href="https://www.codewars.com/kata/590adadea658017d90000039" target="_blank">Fruit Machine</a></span>
<span style="display: flex !important;"><img style="margin:0px;" src="https://raw.githubusercontent.com/adrianeyre/codewars/master/Ruby/Authored/6KYU.png" alt="Rank"/> <a href="https://www.codewars.com/kata/591eab1d192fe0435e000014" target="_blank">Car Park Escape</a></span> | reference | class Connect4 ():
def __init__(self):
self . grid = [[0] * 7 for _ in range(6)]
self . player = 1
self . finish = False
# We only check the possibles moves from the last play, no need to check the whole board
def check(self, row, col):
# Horizontal
for j in range(max(0, col - 3), min(4, col + 1)):
if self . grid[row][j] == self . grid[row][j + 1] == self . grid[row][j + 2] == self . grid[row][j + 3]:
return True
# Vertical
for i in range(max(0, row - 3), min(3, row + 1)):
if self . grid[i][col] == self . grid[i + 1][col] == self . grid[i + 2][col] == self . grid[i + 3][col]:
return True
# First diagonal
for i in range(- min(3, row, col), min(1, 3 - row, 4 - col)):
if self . grid[row + i][col + i] == self . grid[row + i + 1][col + i + 1] == self . grid[row + i + 2][col + i + 2] == self . grid[row + i + 3][col + i + 3]:
return True
# Second diagonal
for i in range(- min(3, row, 6 - col), min(1, 3 - row, col - 2)):
if self . grid[row + i][col - i] == self . grid[row + i + 1][col - i - 1] == self . grid[row + i + 2][col - i - 2] == self . grid[row + i + 3][col - i - 3]:
return True
return False
def play(self, col):
if self . finish:
return "Game has finished!"
row = next((i for i in range(6) if not self . grid[i][col]), None)
if row is None:
return "Column full!"
self . grid[row][col] = self . player
if self . check(row, col):
res = f"Player { self . player } wins!"
self . finish = True
else:
res = f"Player { self . player } has a turn"
self . player = 3 - self . player
return res
| Connect 4 | 586c0909c1923fdb89002031 | [
"Arrays",
"Fundamentals"
] | https://www.codewars.com/kata/586c0909c1923fdb89002031 | 5 kyu |
# Introduction
<pre style="white-space: pre-wrap;white-space: -moz-pre-wrap;white-space: -pre-wrap;white-space: -o-pre-wrap;word-wrap: break-word;">
Welcome Adventurer. Your aim is to navigate the maze and reach the finish point without touching any walls. Doing so will kill you instantly!
</pre>
# Task
<pre style="white-space: pre-wrap;white-space: -moz-pre-wrap;white-space: -pre-wrap;white-space: -o-pre-wrap;word-wrap: break-word;">
You will be given a 2D array of the maze and an array of directions. Your task is to follow the directions given. If you reach the end point before all your moves have gone, you should return <span style="color:#A1A85E">Finish</span>. If you hit any walls or go outside the maze border, you should return <span style="color:#A1A85E">Dead</span>. If you find yourself still in the maze after using all the moves, you should return <span style="color:#A1A85E">Lost</span>.
</pre>
The Maze array will look like
```
maze = [[1,1,1,1,1,1,1],
[1,0,0,0,0,0,3],
[1,0,1,0,1,0,1],
[0,0,1,0,0,0,1],
[1,0,1,0,1,0,1],
[1,0,0,0,0,0,1],
[1,2,1,0,1,0,1]]
```
..with the following key
<pre style="white-space: pre-wrap;white-space: -moz-pre-wrap;white-space: -pre-wrap;white-space: -o-pre-wrap;word-wrap: break-word;">
0 = Safe place to walk
1 = Wall
2 = Start Point
3 = Finish Point
</pre>
```lua
direction = {"N","N","N","N","N","E","E","E","E","E"} == "Finish"
```
```c
directions = "NNNNNEEEEE" == "Finish" // (directions passed as a string)
```
```ruby
direction = ["N","N","N","N","N","E","E","E","E","E"] == "Finish"
```
```python
direction = ["N","N","N","N","N","E","E","E","E","E"] == "Finish"
```
```javascript
direction = ["N","N","N","N","N","E","E","E","E","E"] == "Finish"
```
```php
direction = ["N","N","N","N","N","E","E","E","E","E"] == "Finish"
```
```csharp
direction = ["N","N","N","N","N","E","E","E","E","E"] == "Finish"
```
```haskell
direction = ["N","N","N","N","N","E","E","E","E","E"] == "Finish"
```
# Rules
<pre style="white-space: pre-wrap;white-space: -moz-pre-wrap;white-space: -pre-wrap;white-space: -o-pre-wrap;word-wrap: break-word;">
1. The Maze array will always be square i.e. <span style="color:#A1A85E">N x N</span> but its size and content will alter from test to test.
2. The <span style="color:#A1A85E">start</span> and <span style="color:#A1A85E">finish</span> positions will change for the final tests.
3. The directions array will always be in upper case and will be in the format of <span style="color:#A1A85E">N = North, E = East, W = West and S = South</span>.
4. If you reach the end point before all your moves have gone, you should return <span style="color:#A1A85E">Finish</span>.
5. If you hit any walls or go outside the maze border, you should return <span style="color:#A1A85E">Dead</span>.
6. If you find yourself still in the maze after using all the moves, you should return <span style="color:#A1A85E">Lost</span>.
</pre>
Good luck, and stay safe!
# Kata Series
If you enjoyed this, then please try one of my other Katas. Any feedback, translations and grading of beta Katas are greatly appreciated. Thank you.
<span style="display: flex !important;"><img style="margin:0px;" src="https://raw.githubusercontent.com/adrianeyre/codewars/master/Ruby/Authored/6KYU.png" alt="Rank"/> <a href="https://www.codewars.com/kata/58663693b359c4a6560001d6" target="_blank">Maze Runner</a></span>
<span style="display: flex !important;"><img style="margin:0px;" src="https://raw.githubusercontent.com/adrianeyre/codewars/master/Ruby/Authored/6KYU.png" alt="Rank"/> <a href="https://www.codewars.com/kata/58693bbfd7da144164000d05" target="_blank">Scooby Doo Puzzle</a></span>
<span style="display: flex !important;"><img style="margin:0px;" src="https://raw.githubusercontent.com/adrianeyre/codewars/master/Ruby/Authored/7KYU.png" alt="Rank"/> <a href="https://www.codewars.com/kata/586a1af1c66d18ad81000134" target="_blank">Driving License</a></span>
<span style="display: flex !important;"><img style="margin:0px;" src="https://raw.githubusercontent.com/adrianeyre/codewars/master/Ruby/Authored/6KYU.png" alt="Rank"/> <a href="https://www.codewars.com/kata/586c0909c1923fdb89002031" target="_blank">Connect 4</a></span>
<span style="display: flex !important;"><img style="margin:0px;" src="https://raw.githubusercontent.com/adrianeyre/codewars/master/Ruby/Authored/6KYU.png" alt="Rank"/> <a href="https://www.codewars.com/kata/586e6d4cb98de09e3800014f" target="_blank">Vending Machine</a></span>
<span style="display: flex !important;"><img style="margin:0px;" src="https://raw.githubusercontent.com/adrianeyre/codewars/master/Ruby/Authored/6KYU.png" alt="Rank"/> <a href="https://www.codewars.com/kata/587136ba2eefcb92a9000027" target="_blank">Snakes and Ladders</a></span>
<span style="display: flex !important;"><img style="margin:0px;" src="https://raw.githubusercontent.com/adrianeyre/codewars/master/Ruby/Authored/6KYU.png" alt="Rank"/> <a href="https://www.codewars.com/kata/58a848258a6909dd35000003" target="_blank">Mastermind</a></span>
<span style="display: flex !important;"><img style="margin:0px;" src="https://raw.githubusercontent.com/adrianeyre/codewars/master/Ruby/Authored/6KYU.png" alt="Rank"/> <a href="https://www.codewars.com/kata/58b2c5de4cf8b90723000051" target="_blank">Guess Who?</a></span>
<span style="display: flex !important;"><img style="margin:0px;" src="https://raw.githubusercontent.com/adrianeyre/codewars/master/Ruby/Authored/6KYU.png" alt="Rank"/> <a href="https://www.codewars.com/kata/58f5c63f1e26ecda7e000029" target="_blank">Am I safe to drive?</a></span>
<span style="display: flex !important;"><img style="margin:0px;" src="https://raw.githubusercontent.com/adrianeyre/codewars/master/Ruby/Authored/6KYU.png" alt="Rank"/> <a href="https://www.codewars.com/kata/58f5c63f1e26ecda7e000029" target="_blank">Mexican Wave</a></span>
<span style="display: flex !important;"><img style="margin:0px;" src="https://raw.githubusercontent.com/adrianeyre/codewars/master/Ruby/Authored/6KYU.png" alt="Rank"/> <a href="https://www.codewars.com/kata/58fdcc51b4f81a0b1e00003e" target="_blank">Pigs in a Pen</a></span>
<span style="display: flex !important;"><img style="margin:0px;" src="https://raw.githubusercontent.com/adrianeyre/codewars/master/Ruby/Authored/6KYU.png" alt="Rank"/> <a href="https://www.codewars.com/kata/590300eb378a9282ba000095" target="_blank">Hungry Hippos</a></span>
<span style="display: flex !important;"><img style="margin:0px;" src="https://raw.githubusercontent.com/adrianeyre/codewars/master/Ruby/Authored/6KYU.png" alt="Rank"/> <a href="https://www.codewars.com/kata/5904be220881cb68be00007d" target="_blank">Plenty of Fish in the Pond</a></span>
<span style="display: flex !important;"><img style="margin:0px;" src="https://raw.githubusercontent.com/adrianeyre/codewars/master/Ruby/Authored/6KYU.png" alt="Rank"/> <a href="https://www.codewars.com/kata/590adadea658017d90000039" target="_blank">Fruit Machine</a></span>
<span style="display: flex !important;"><img style="margin:0px;" src="https://raw.githubusercontent.com/adrianeyre/codewars/master/Ruby/Authored/6KYU.png" alt="Rank"/> <a href="https://www.codewars.com/kata/591eab1d192fe0435e000014" target="_blank">Car Park Escape</a></span> | reference | def maze_runner(maze, directions):
n = len(maze)
# find start point
for i in range(n):
if 2 in maze[i]:
row = i
col = maze[row]. index(2)
break
# follow directions
for step in directions:
if step == "N":
row -= 1
elif step == "S":
row += 1
elif step == "E":
col += 1
elif step == "W":
col -= 1
# check the result
if row < 0 or col < 0 or row == n or col == n or maze[row][col] == 1:
return "Dead"
elif maze[row][col] == 3:
return "Finish"
return "Lost"
| Maze Runner | 58663693b359c4a6560001d6 | [
"Arrays",
"Fundamentals"
] | https://www.codewars.com/kata/58663693b359c4a6560001d6 | 6 kyu |
Your task, is to create a NxN spiral with a given `size`.
For example, spiral with size 5 should look like this:
```
00000
....0
000.0
0...0
00000
```
and with the size 10:
```
0000000000
.........0
00000000.0
0......0.0
0.0000.0.0
0.0..0.0.0
0.0....0.0
0.000000.0
0........0
0000000000
```
Return value should contain array of arrays, of `0` and `1`, with the first row being composed of `1`s.
For example for given size `5` result should be:
```javascript
[[1,1,1,1,1],[0,0,0,0,1],[1,1,1,0,1],[1,0,0,0,1],[1,1,1,1,1]]
```
```lua
{{1,1,1,1,1},{0,0,0,0,1},{1,1,1,0,1},{1,0,0,0,1},{1,1,1,1,1}}
```
```rust
[[1,1,1,1,1],[0,0,0,0,1],[1,1,1,0,1],[1,0,0,0,1],[1,1,1,1,1]]
```
```julia
[1 1 1 1 1; 0 0 0 0 1; 1 1 1 0 1; 1 0 0 0 1; 1 1 1 1 1]
```
```go
[[1,1,1,1,1],[0,0,0,0,1],[1,1,1,0,1],[1,0,0,0,1],[1,1,1,1,1]]
```
Because of the edge-cases for tiny spirals, the size will be at least 5.
General rule-of-a-thumb is, that the snake made with '1' cannot touch to itself.
| algorithms | def spiralize(size):
def on_board(x, y):
return 0 <= x < size and 0 <= y < size
def is_one(x, y):
return on_board(x, y) and spiral[y][x] == 1
def can_move():
return on_board(x + dx, y + dy) and not (is_one(x + 2 * dx, y + 2 * dy) or is_one(x + dx - dy, y + dy + dx) or is_one(x + dx + dy, y + dy - dx))
spiral = [[0 for x in range(size)] for y in range(size)]
x, y = - 1, 0
dx, dy = 1, 0
turns = 0
while (turns < 2):
if can_move():
x += dx
y += dy
spiral[y][x] = 1
turns = 0
else:
dx, dy = - dy, dx
turns += 1
return spiral
| Make a spiral | 534e01fbbb17187c7e0000c6 | [
"Algorithms",
"Arrays",
"Logic"
] | https://www.codewars.com/kata/534e01fbbb17187c7e0000c6 | 3 kyu |
You need to write a password generator that meets the following criteria:
* 6 - 20 characters long
* contains at least one lowercase letter
* contains at least one uppercase letter
* contains at least one number
* contains only alphanumeric characters (no special characters)
Return the random password as a string.
**Note**: "randomness" is checked by counting the characters used in the generated passwords - all characters should have less than 50% occurance. Based on extensive tests, the normal rate is around 35%.
| reference | from string import ascii_lowercase as LOWER, ascii_uppercase as UPPER, digits as DIGITS
from random import choice, shuffle, randint
def password_gen():
pw = [choice(UPPER), choice(LOWER), choice(DIGITS)] + \
[choice(UPPER + LOWER + DIGITS) for i in range(randint(3, 17))]
shuffle(pw)
return "" . join(pw)
| Password generator | 58ade2233c9736b01d0000b3 | [
"Regular Expressions",
"Security",
"Fundamentals"
] | https://www.codewars.com/kata/58ade2233c9736b01d0000b3 | 6 kyu |
Cara is applying for several different jobs.
The online application forms ask her to respond within a specific character count.
Cara needs to check that her answers fit into the character limit.
Annoyingly, some application forms count spaces as a character, and some don't.
Your challenge:
Write Cara a function `charCheck()` with the arguments:
- `"text"`: a string containing Cara's answer for the question
- `"max"`: a number equal to the maximum number of characters allowed in the answer
- `"spaces"`: a boolean which is `True` if spaces are included in the character count and `False` if they are not
The function `charCheck()` should return an array: `[True, "Answer"]` , where `"Answer"` is equal to the original text, if Cara's answer is short enough.
If her answer `"text"` is too long, return an array: `[False, "Answer"]`.
The second element should be the original `"text"` string truncated to the length of the limit.
When the `"spaces"` argument is `False`, you should remove the spaces from the `"Answer"`.
For example:
- `charCheck("Cara Hertz", 10, True)` should return `[ True, "Cara Hertz" ]`
- `charCheck("Cara Hertz", 9, False)` should return `[ True, "CaraHertz" ]`
- `charCheck("Cara Hertz", 5, True)` should return `[ False, "Cara " ]`
- `charCheck("Cara Hertz", 5, False)` should return `[ False, "CaraH" ]`
| reference | def charCheck(text, mx, spaces):
text = text if spaces else text . replace(' ', '')
return [len(text) <= mx, text[: mx]]
| Character limits: How long is your piece of string? | 58af4ed8673e88a719000116 | [
"Fundamentals",
"Strings"
] | https://www.codewars.com/kata/58af4ed8673e88a719000116 | 6 kyu |
Write function alternateCase which switch every letter in string from upper to lower and from lower to upper.
E.g: Hello World -> hELLO wORLD | reference | def alternateCase(s):
return s . swapcase()
| Alternate case | 57a62154cf1fa5b25200031e | [
"Fundamentals"
] | https://www.codewars.com/kata/57a62154cf1fa5b25200031e | 7 kyu |
Write function `replaceAll` (Python: `replace_all`) that will replace all occurrences of an item with another.
__Python / JavaScript__: The function has to work for strings and lists.
Example: replaceAll [1,2,2] 1 2 -> in list [1,2,2] we replace 1 with 2 to get new list [2,2,2]
```swift
replaceAll(replaceAll(array: [1,2,2], old: 1, new: 2) // [2,2,2]
```
```if:c
In C, replace the elements directly inside the array given as argument and return that array.
``` | reference | def replace_all(obj, find, replace):
if isinstance(obj, str):
return obj . replace(find, replace)
elif isinstance(obj, list):
return [x if x != find else replace for x in obj]
| Replace all items | 57ae18c6e298a7a6d5000c7a | [
"Fundamentals",
"Arrays",
"Lists",
"Regular Expressions"
] | https://www.codewars.com/kata/57ae18c6e298a7a6d5000c7a | 7 kyu |
In this kata you parse RGB colors represented by strings. The formats are primarily used in HTML and CSS. Your task is to implement a function which takes a color as a string and returns the parsed color as a map (see Examples).
## Input:
The input string represents one of the following:
- **6-digit hexadecimal** - "#RRGGBB"
e.g. "#012345", "#789abc", "#FFA077"
Each pair of digits represents a value of the channel in hexadecimal: 00 to FF
- **3-digit hexadecimal** - "#RGB"
e.g. "#012", "#aaa", "#F5A"
Each digit represents a value 0 to F which translates to 2-digit hexadecimal: 0->00, 1->11, 2->22, and so on.
- **Preset color name**
e.g. "red", "BLUE", "LimeGreen"
You have to use the predefined map `PRESET_COLORS` (JavaScript, Python, Ruby), `presetColors` (Java, C#, Haskell), `PresetColors` (Go) or `preset-colors` (Clojure). The keys are the names of preset colors *in lower-case* and the values are the corresponding colors in 6-digit hexadecimal (same as 1. "#RRGGBB").
## Examples:
```ruby
parse_html_color('#80FFA0') # => { r: 128, g: 255, b: 160 }
parse_html_color('#3B7') # => { r: 51, g: 187, b: 119 }
parse_html_color('LimeGreen') # => { r: 50, g: 205, b: 50 }
```
```python
parse_html_color('#80FFA0') # => {'r': 128, 'g': 255, 'b': 160}
parse_html_color('#3B7') # => {'r': 51, 'g': 187, 'b': 119}
parse_html_color('LimeGreen') # => {'r': 50, 'g': 205, 'b': 50 }
```
```javascript
parseHTMLColor('#80FFA0'); // => { r: 128, g: 255, b: 160 }
parseHTMLColor('#3B7'); // => { r: 51, g: 187, b: 119 }
parseHTMLColor('LimeGreen'); // => { r: 50, g: 205, b: 50 }
```
```clojure
(parse-html-color "#80FFA0") ; => {:r 128 :g 255 :b 160}
(parse-html-color "#3B7") ; => {:r 51 :g 187 :b 119}
(parse-html-color "LimeGreen") ; => {:r 50 :g 205 :b 50 }
```
```haskell
-- You can get a value from the map like this:
presetColors ! "blue"
--
parseHtmlColor "#80FFA0" === fromList [('r',128), ('g',255), ('b',160)]
parseHtmlColor "#3B7" === fromList [('r',51), ('g',187), ('b',119)]
parseHtmlColor "LimeGreen" === fromList [('r',50), ('g',205), ('b',50)]
```
```java
parse("#80FFA0") === new RGB(128, 255, 160))
parse("#3B7") === new RGB( 51, 187, 119))
parse("LimeGreen") === new RGB( 50, 205, 50))
// RGB class is defined as follows:
final class RGB {
public int r, g, b;
public RGB();
public RGB(int r, int g, int b);
}
```
```csharp
Parse("#80FFA0") === new RGB(128, 255, 160))
Parse("#3B7") === new RGB( 51, 187, 119))
Parse("LimeGreen") === new RGB( 50, 205, 50))
// RGB struct is defined as follows:
struct RGB
{
public byte r, g, b;
public RGB(byte r, byte g, byte b);
}
```
```go
Parse("#80FFA0") == Color{128, 255, 160}
Parse("#3B7") == Color{51, 187, 119}
Parse("LimeGreen") == Color{50, 205, 50}
// Color struct is defined as follows:
type Color struct{ R, G, B byte }
```
| reference | def parse_html_color(color):
color = PRESET_COLORS . get(color . lower(), color)
if len(color) == 7:
r, g, b = (int(color[i: i + 2], 16) for i in range(1, 7, 2))
else:
r, g, b = (int(color[i + 1] * 2, 16) for i in range(3))
return dict(zip("rgb", (r, g, b)))
| Parse HTML/CSS Colors | 58b57ae2724e3c63df000006 | [
"Fundamentals",
"Algorithms",
"Strings",
"Parsing"
] | https://www.codewars.com/kata/58b57ae2724e3c63df000006 | 6 kyu |
You are writing an encoder/decoder to convert between javascript strings and a binary representation of Morse code.
Each Morse code character is represented by a series of "dots" and "dashes". In binary, a dot is a single bit (`1`) and a dash is three bits (`111`). Between each dot or dash within a single character, we place a single zero bit. (I.e. "dot dash" would become `10111`.) Separate characters are separated by three zero bits (`000`). Words are spearated by a single space, which is represented by 7 zero bits (`0000000`).
<br>
<h3>Formal Syntax</h3>
Binary Morse code has the following syntax: (Where '1' and '0' are literal bits.)
<pre>
message ::= word
| word 0000000 message
word ::= character
| character 000 word
character ::= mark
| mark 0 character
mark ::= dot
| dash
dot (·) ::= 1
dash (–) ::= 111
</pre>
<br>
<h3>Morse Code</h3>
Chracters in Morse code are traditionally represented by a series of dots and dashes. Below is the full list of characters supported by our Binary Morse code:
<pre>
A ·–
B –···
C –·–·
D –··
E ·
F ··–·
G ––·
H ····
I ··
J ·–––
K –·–
L ·–··
M ––
N –·
O –––
P ·––·
Q ––·–
R ·–·
S ···
T –
U ··–
V ···–
W ·––
X –··–
Y –·––
Z ––··
0 –––––
1 ·––––
2 ··–––
3 ···––
4 ····–
5 ·····
6 –····
7 ––···
8 –––··
9 ––––·
. ·–·–·–
, ––··––
? ··––··
' ·––––·
! –·–·––
/ –··–·
( –·––·
) –·––·–
& ·–···
: –––···
; –·–·–·
= –···–
+ ·–·–·
- –····–
_ ··––·–
" ·–··–·
$ ···–··–
@ ·––·–·
</pre>
<br>
Note that space itself is not a character but is interpreted as the separater between words.
<br>
<br>
The first method `Morse.encode` will take a String representing the message and will return an array of signed 32-bit integers in big-endian order and in two's complement format. (Note: This is the standard format for numbers returned by JavaScript bitwise operators.) Since it is possible that the Morse encoded message will not align perfectly with the binary 32-bit numbers, all unused bits are to be padded with 0's.
<br>
<br>
The second method `Morse.decode` will take an array of numbers and return the String representation of the message.
<h3>Example</h3>
<pre>
Text content H E L L O [space] W O R L D
Morse Code ···· · ·−·· ·−·· −−− ·−− −−− ·−· ·−·· −··
Bit pattern 1010101 000 1 000 101110101 000 101110101 000 11101110111 0000000 101110111 000 11101110111 000 1011101 000 10111010 1000 1110101 00000000000000000
32-bit Value -1,440,552,402 | -1,547,992,901 | -1,896,993,141 | -1,461,059,584
Hex Value AA22 EA2E | A3BB 80BB | 8EEE 2E8B | A8EA 0000
^ ^ ^ ^ ^ ^ ^ ^ ^ ^ ^ ^ ^ ^ ^ ^
| | | | | | | | | | | | | | | |
0 8 16 24 0 8 16 24 0 8 16 24 0 8 16 24
</pre> | algorithms | import re
class Morse:
@ classmethod
def encode(self, message):
bits = "0000000" . join(["000" . join([Morse . alpha[char] for char in word])
for word in message . split(' ')])
return [int((int("{0:0<32s}" . format(bit32), base=2) + 0x80000000) % 0x100000000 - 0x80000000)
for bit32 in re . findall(r'.{1,32}', bits)]
@ classmethod
def decode(self, array):
code = '' . join(["{0:032b}" . format((i + 0x100000000) %
0x100000000) for i in array]). rstrip('0')
return ' ' . join(['' . join([Morse . alpha_re[char] for char in word . split("000")])
for word in code . split("0000000")])
alpha = {
'A': '10111',
'B': '111010101',
'C': '11101011101',
'D': '1110101',
'E': '1',
'F': '101011101',
'G': '111011101',
'H': '1010101',
'I': '101',
'J': '1011101110111',
'K': '111010111',
'L': '101110101',
'M': '1110111',
'N': '11101',
'O': '11101110111',
'P': '10111011101',
'Q': '1110111010111',
'R': '1011101',
'S': '10101',
'T': '111',
'U': '1010111',
'V': '101010111',
'W': '101110111',
'X': '11101010111',
'Y': '1110101110111',
'Z': '11101110101',
'0': '1110111011101110111',
'1': '10111011101110111',
'2': '101011101110111',
'3': '1010101110111',
'4': '10101010111',
'5': '101010101',
'6': '11101010101',
'7': '1110111010101',
'8': '111011101110101',
'9': '11101110111011101',
'.': '10111010111010111',
',': '1110111010101110111',
'?': '101011101110101',
"'": '1011101110111011101',
'!': '1110101110101110111',
'/': '1110101011101',
'(': '111010111011101',
')': '1110101110111010111',
'&': '10111010101',
':': '11101110111010101',
';': '11101011101011101',
'=': '1110101010111',
'+': '1011101011101',
'-': '111010101010111',
'_': '10101110111010111',
'"': '101110101011101',
'$': '10101011101010111',
'@': '10111011101011101',
' ': '0'}
alpha_re = dict([(value, key) for key, value in alpha . items()])
| Morse Encoding | 536602df5d0266e7b0000d31 | [
"Bits",
"Strings",
"Binary",
"Parsing",
"Algorithms"
] | https://www.codewars.com/kata/536602df5d0266e7b0000d31 | 5 kyu |
Write function `makeParts` or `make_parts` (depending on your language) that will take an array as argument and the size of the chunk.
Example: if an array of size 123 is given and chunk size is 10 there will be 13 parts, 12 of size 10 and 1 of size 3. | reference | def makeParts(arr, csize):
return [arr[i: i + csize] for i in range(0, len(arr), csize)]
| Cut array into smaller parts | 58ac59d21c9e1d7dc5000150 | [
"Fundamentals"
] | https://www.codewars.com/kata/58ac59d21c9e1d7dc5000150 | 7 kyu |
Batman & Robin have gotten into quite a pickle this time. The Joker has mixed up their iconic quotes and also replaced one of the characters in their names, with a number. They need help getting things back in order.
Implement the getQuote method which takes in an array of quotes, and a string comprised of letters and a single number (e.g. "Rob1n") where the number corresponds to their quote indexed in the passed in array.
Return the quote along with the character who said it:
```java
BatmanQuotes.getQuote(["I am vengeance. I am the night. I am Batman!", "Holy haberdashery, Batman!", "Let's put a smile on that faaaceee!"], "Rob1n") should output => "Robin: Holy haberdashery, Batman!
```
```javascript
getQuote(["I am vengeance. I am the night. I am Batman!", "Holy haberdashery, Batman!", "Let's put a smile on that faaaceee!"], "Rob1n")
// => "Robin: Holy haberdashery, Batman!
```
```clojure
(getQuote ["I am vengeance. I am the night. I am Batman!" "Holy haberdashery, Batman!" "Let's put a smile on that faaaceee!"] "Rob1n")
// => "Robin: Holy haberdashery, Batman!"
```
Hint: You are guaranteed that the number in the passed in string is placed somewhere after the first character of the string. The quotes either belong to "Batman", "Robin", or "Joker". | reference | import re
class BatmanQuotes (object):
@ staticmethod
def get_quote(quotes, hero):
return {'B': 'Batman', 'R': 'Robin', 'J': 'Joker'}[hero[0]] + ": " + quotes[int(re . search('\d+', hero). group())]
| Batman Quotes | 551614eb77dd9ee37100003e | [
"Arrays",
"Fundamentals"
] | https://www.codewars.com/kata/551614eb77dd9ee37100003e | 7 kyu |
# The problem
```if:javascript,coffeescript,haskell,rust
In this kata, you're going write a function called `pointInPoly` to test if a point is inside a polygon.
```
```if:python
In this kata, you're going write a function called `point_in_polygon` to test if a point is inside a polygon.
```
```if:javascript,coffeescript,haskell,rust
Points will be represented as `[x,y]` arrays.
```
```if:python
Points will be represented as tuples `(x,y)`.
```
The polygon will be an array of points which are the polygon's vertices. The last point in the array connects back to the first point.
You can assume:
1. The polygon will be a valid [simple polygon](https://en.wikipedia.org/wiki/Simple_polygon). That is, it will have at least three points, none of its edges will cross each other, and exactly two edges will meet at each vertex.
2. In the tests, the point will never fall exactly on an edge of the polygon.
# Testing
```if:javascript,coffeescript,haskell,rust
To help you visualize your test cases, the `showAndTest(poly,point,inside)` function is preloaded. It draws the polygon and point and then calls `Test.expect` automatically.
So if you call:
showAndTest([[-5, -5], [5, -5], [0, 5]], [0,0], true)
then you'll see:
<div style="background:white; width:140px"><svg width="140" ,="" height="140"><polygon points="20.5,20.5 120.5,20.5 70.5,120.5" stroke="blue" fill="white"></polygon><circle cx="70" cy="70" r="2" fill="green"></circle></svg></div>
The drawing window is 14x14 units wide and centered at the origin.
```
```if:python
To help you visualize your test cases, the `visualize_polygon(polygon, point)` function is preloaded. It draws the polygon and point.
``` | algorithms | from typing import List, Tuple
# Input parameters for visualize_polygon are the same, as for point_in_polygon
from preloaded import visualize_polygon
from matplotlib . path import Path
def point_in_polygon(polygon: List[Tuple[float, float]], point: Tuple[float, float]) - > bool:
return Path(polygon). contains_point(point)
| Point in Polygon | 530265044b7e23379d00076a | [
"Geometry",
"Mathematics",
"Algorithms"
] | https://www.codewars.com/kata/530265044b7e23379d00076a | 6 kyu |
#Unflatten a list (Easy)
There are several katas like "Flatten a list".
These katas are done by so many warriors, that the count of available list to flattin goes down!
So you have to build a method, that creates new arrays, that can be flattened!
#Shorter: You have to unflatten a list/an array.
You get an array of integers and have to unflatten it by these rules:
```
- You start at the first number.
- If this number x is smaller than 3, take this number x direct
for the new array and continue with the next number.
- If this number x is greater than 2, take the next x numbers (inclusive this number) as a
sub-array in the new array. Continue with the next number AFTER this taken numbers.
- If there are too few numbers to take by number, take the last available numbers.
```
The given array will always contain numbers. There will only be numbers > 0.
Example:
```
[1,4,5,2,1,2,4,5,2,6,2,3,3] -> [1,[4,5,2,1],2,[4,5,2,6],2,[3,3]]
Steps:
1. The 1 is added directly to the new array.
2. The next number is 4. So the next 4 numbers (4,5,2,1) are added as sub-array in the new array.
3. The 2 is added directly to the new array.
4. The next number is 4. So the next 4 numbers (4,5,2,6) are added as sub-array in the new array.
5. The 2 is added directly to the new array.
6. The next number is 3. So the next 3 numbers would be taken. There are only 2,
so take these (3,3) as sub-array in the new array.
```
There is a harder version of this kata!<br>
<a href="https://www.codewars.com/kata/57e5aa1d7fbcc988800001ae"taget=_blank>Unflatten a list (Harder than easy)</a>
Have fun coding it and please don't forget to vote and rank this kata! :-)
I have created other katas. Have a look if you like coding and challenges. | algorithms | def unflatten(flat_array):
arr = flat_array[:]
for i, v in enumerate(arr):
if v > 2:
arr[i], arr[i + 1: i + v] = arr[i: i + v], []
return arr
| Unflatten a list (Easy) | 57e2dd0bec7d247e5600013a | [
"Mathematics",
"Arrays",
"Algorithms"
] | https://www.codewars.com/kata/57e2dd0bec7d247e5600013a | 7 kyu |
After yet another dispute on their game the Bingo Association decides to change course and automate the game.
Can you help the association by writing a method to create a random Bingo card?
## Bingo Cards
A Bingo card contains 24 unique and random numbers according to this scheme:
* 5 numbers from the B column in the range 1 to 15
* 5 numbers from the I column in the range 16 to 30
* 4 numbers from the N column in the range 31 to 45
* 5 numbers from the G column in the range 46 to 60
* 5 numbers from the O column in the range 61 to 75
## Task
Write the function `get_card()`/`getCard()`.
The card must be returned as an array of Bingo style numbers:
```
[ 'B14', 'B12', 'B5', 'B6', 'B3', 'I28', 'I27', ... ]
```
The numbers must be in the order of their column: B, I, N, G, O. Within the columns the order of the numbers is random.
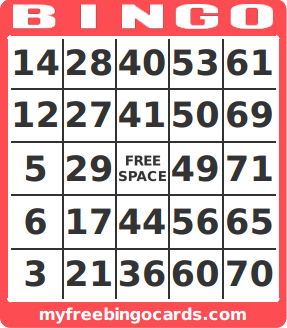 | algorithms | import random
def get_bingo_card():
b = ['B' + str(n) for n in random . sample(range(1, 16), 5)]
i = ['I' + str(n) for n in random . sample(range(16, 31), 5)]
n = ['N' + str(n) for n in random . sample(range(31, 46), 4)]
g = ['G' + str(n) for n in random . sample(range(46, 61), 5)]
o = ['O' + str(n) for n in random . sample(range(61, 76), 5)]
return b + i + n + g + o
| Bingo Card | 566d5e2e57d8fae53c00000c | [
"Games",
"Algorithms"
] | https://www.codewars.com/kata/566d5e2e57d8fae53c00000c | 6 kyu |
This is the advanced version of the [Vigenère Cipher Helper](https://www.codewars.com/kata/vigenere-cipher-helper) kata. The following assumes that you have already completed that kata -- if you haven't done it yet, you should start there.
The basic concept is the same as in the previous kata (see the detailed explanation there). However, there is a major difference:
With the basic Vigenère Cipher, we assume the key is repeated for the length of the text. **In this kata, the key is only used once, and then complemented by the decoded text.** Thus every encoding and decoding is independent (still using the same key to begin with). Furthermore, **the key index is only incremented if the current letter is in the provided alphabet.**
Visual representation:
```javascript
"password" // original key
"my secret code i want to secure" // message
"pa ssword myse c retc od eiwant" // full key used
```
Write a class that, when given a key and an alphabet, can be used to encode and decode from the cipher.
## Examples
```javascript
var alphabet = 'abcdefghijklmnopqrstuvwxyz';
var key = 'password';
// creates a cipher helper with each letter substituted
// by the corresponding character in the key
var c = new VigenèreCipher(key, alphabet);
c.encode('codewars'); // returns 'rovwsoiv'
c.decode('laxxhsj'); // returns 'waffles'
c.encode('amazingly few discotheques provide jukeboxes')
// returns 'pmsrebxoy rev lvynmylatcwu dkvzyxi bjbswwaib'
c.decode('pmsrebxoy rev lvynmylatcwu dkvzyxi bjbswwaib')
// returns 'amazingly few discotheques provide jukeboxes'
```
```python
alphabet = 'abcdefghijklmnopqrstuvwxyz'
key = 'password'
# creates a cipher helper with each letter substituted
# by the corresponding character in the key
c = VigenereCipher(key, alphabet)
c.encode('codewars') # returns 'rovwsoiv'
c.decode('laxxhsj') # returns 'waffles'
c.encode('amazingly few discotheques provide jukeboxes')
# returns 'pmsrebxoy rev lvynmylatcwu dkvzyxi bjbswwaib'
c.decode('pmsrebxoy rev lvynmylatcwu dkvzyxi bjbswwaib')
# returns 'amazingly few discotheques provide jukeboxes'
```
```coffeescript
alphabet = 'abcdefghijklmnopqrstuvwxyz'
key = 'password'
# creates a cipher helper with each letter substituted
# by the corresponding character in the key
c = new VigenereCipher(key, alphabet)
c.encode('codewars') # returns 'rovwsoiv'
c.decode('laxxhsj') # returns 'waffles'
c.encode('amazingly few discotheques provide jukeboxes')
# returns 'pmsrebxoy rev lvynmylatcwu dkvzyxi bjbswwaib'
c.decode('pmsrebxoy rev lvynmylatcwu dkvzyxi bjbswwaib')
# returns 'amazingly few discotheques provide jukeboxes'
```
Any character not in the alphabet should be left alone. For example (following from above):
```javascript
c.encode('CODEWARS'); // returns 'CODEWARS'
```
```python
c.encode('CODEWARS') # returns 'CODEWARS'
```
```coffeescript
c.encode('CODEWARS') # returns 'CODEWARS'
``` | algorithms | class VigenereAutokeyCipher:
def __init__(self, key, abc):
self . key = key
self . abc = abc
self . alle = len(abc)
def cipher(self, s, m):
output, keyarr = '', list(self . key)
for char in s:
try:
output += self . abc[(self . abc . index(char) +
self . abc . index(keyarr . pop(0)) * (- 1, 1)[m]) % self . alle]
keyarr . append((output[- 1], char)[m])
except ValueError:
output += char
return output
def encode(self, s):
return self . cipher(s, 1)
def decode(self, s):
return self . cipher(s, 0)
| Vigenère Autokey Cipher Helper | 52d2e2be94d26fc622000735 | [
"Algorithms",
"Ciphers",
"Security",
"Object-oriented Programming",
"Strings"
] | https://www.codewars.com/kata/52d2e2be94d26fc622000735 | 5 kyu |
Extend the String object (JS) or create a function (Python, C#) that converts the value of the String **to and from Base64** using the ASCII (UTF-8 for C#) character set.
### Example (input -> output):
```
'this is a string!!' -> 'dGhpcyBpcyBhIHN0cmluZyEh'
```
You can learn more about Base64 encoding and decoding <a href="http://en.wikipedia.org/wiki/Base64">here</a>.
Note: This kata uses the non-padding version ("=" is not added to the end).
| algorithms | CODES = "ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz0123456789+/"
def to_base_64(string):
padding = 3 - len(string) % 3 if len(string) % 3 else 0
binary = '' . join(format(ord(i), '08b') for i in string) + '00' * padding
return '' . join(CODES[int(binary[i: i + 6], 2)] for i in range(0, len(binary), 6))
def from_base_64(string):
binary = '' . join(format(CODES . find(i), '06b') for i in string)
return '' . join(chr(int(binary[i: i + 8], 2)) for i in range(0, len(binary), 8)). rstrip('\x00')
| Base64 Encoding | 5270f22f862516c686000161 | [
"Binary",
"Strings",
"Algorithms"
] | https://www.codewars.com/kata/5270f22f862516c686000161 | 5 kyu |
Esoteric languages are pretty hard to program, but it's fairly interesting to write interpreters for them!
Your task is to write a method which will interpret Befunge-93 code! Befunge-93 is a language in which the code is presented not as a series of instructions, but as instructions scattered on a 2D plane; your pointer **starts at the top-left corner** and defaults to **moving right** through the code. Note that the instruction pointer **wraps** around the screen! There is a singular stack which we will assume is unbounded and only contain integers. While Befunge-93 code is supposed to be restricted to 80x25, you need not be concerned with code size. Befunge-93 supports the following instructions (from [Wikipedia](https://en.wikipedia.org/wiki/Befunge)):
- `0-9` Push this number onto the stack.
- `+` Addition: Pop `a` and `b`, then push `a+b`.
- `-` Subtraction: Pop `a` and `b`, then push `b-a`.
- `*` Multiplication: Pop `a` and `b`, then push `a*b`.
- `/` Integer division: Pop `a` and `b`, then push `b/a`, rounded down. If `a` is zero, push zero.
- `%` Modulo: Pop `a` and `b`, then push the `b%a`. If `a` is zero, push zero.
- `!` Logical NOT: Pop a value. If the value is zero, push `1`; otherwise, push zero.
- ``` ` ``` (backtick) Greater than: Pop `a` and `b`, then push `1` if `b>a`, otherwise push zero.
- `>` Start moving right.
- `<` Start moving left.
- `^` Start moving up.
- `v` Start moving down.
- `?` Start moving in a random cardinal direction.
- `_` Pop a value; move right if `value = 0`, left otherwise.
- `|` Pop a value; move down if `value = 0`, up otherwise.
- `"` Start string mode: push each character's ASCII value all the way up to the next `"`.
- `:` Duplicate value on top of the stack. If there is nothing on top of the stack, push a `0`.
- `\` Swap two values on top of the stack. If there is only one value, pretend there is an extra `0` on bottom of the stack.
- `$` Pop value from the stack and discard it.
- `.` Pop value and output as an integer.
- `,` Pop value and output the ASCII character represented by the integer code that is stored in the value.
- `#` Trampoline: Skip next cell.
- `p` A "put" call (a way to store a value for later use). Pop `y`, `x` and `v`, then change the character at the position `(x,y)` in the program to the character with ASCII value `v`.
- `g` A "get" call (a way to retrieve data in storage). Pop `y` and `x`, then push ASCII value of the character at that position in the program.
- `@` End program.
- ` ` (i.e. a space) No-op. Does nothing.
The above list is slightly modified: you'll notice if you look at the Wikipedia page that we do not use the user input instructions and dividing by zero simply yields zero.
Here's an example:
```
>987v>.v
v456< :
>321 ^ _@
```
will create the output `123456789`.
So what you must do is create a function such that when you pass in the Befunge code, the function returns the output that would be generated by the code. So, for example:
```
"123456789".equals(new BefungeInterpreter().interpret(">987v>.v\nv456< :\n>321 ^ _@")
```
This test case will be added for you. | algorithms | from random import choice
def interpret(code):
code = [list(l) for l in code . split('\n')]
x, y = 0, 0
dx, dy = 1, 0
output = ''
stack = []
string_mode = False
while True:
move = 1
i = code[y][x]
if string_mode:
if i == '"':
string_mode = False
else:
stack . append(ord(i))
else:
if i . isdigit():
stack . append(int(i))
elif i == '+':
stack[- 2:] = [stack[- 2] + stack[- 1]]
elif i == '-':
stack[- 2:] = [stack[- 2] - stack[- 1]]
elif i == '*':
stack[- 2:] = [stack[- 2] * stack[- 1]]
elif i == '/':
stack[- 2:] = [stack[- 2] and stack[- 2] / stack[- 1]]
elif i == '%':
stack[- 2:] = [stack[- 2] and stack[- 2] % stack[- 1]]
elif i == '!':
stack[- 1] = not stack[- 1]
elif i == '`':
stack[- 2:] = [stack[- 2] > stack[- 1]]
elif i in '><^v?':
if i == '?':
i = choice('><^v')
if i == '>':
dx, dy = 1, 0
elif i == '<':
dx, dy = - 1, 0
elif i == '^':
dx, dy = 0, - 1
elif i == 'v':
dx, dy = 0, 1
elif i == '_':
dx, dy = (- 1 if stack . pop() else 1), 0
elif i == '|':
dx, dy = 0, (- 1 if stack . pop() else 1)
elif i == '"':
string_mode = True
elif i == ':':
stack . append(stack[- 1] if stack else 0)
elif i == '\\':
stack[- 2:] = stack[- 2:][:: - 1]
elif i == '$':
stack . pop()
elif i == '.':
output += str(stack . pop())
elif i == ',':
output += chr(stack . pop())
elif i == '#':
move += 1
elif i == 'p':
ty, tx, tv = stack . pop(), stack . pop(), stack . pop()
code[ty][tx] = chr(tv)
elif i == 'g':
ty, tx = stack . pop(), stack . pop()
stack . append(ord(code[ty][tx]))
elif i == '@':
return output
for _ in range(move):
x = (x + dx) % len(code[y])
y = (y + dy) % len(code)
| Befunge Interpreter | 526c7b931666d07889000a3c | [
"Interpreters",
"Algorithms"
] | https://www.codewars.com/kata/526c7b931666d07889000a3c | 4 kyu |
# Introduction
<pre style="white-space: pre-wrap;white-space: -moz-pre-wrap;white-space: -pre-wrap;white-space: -o-pre-wrap;word-wrap: break-word;">
In the United Kingdom, the driving licence is the official document which authorises its holder to operate various types of motor vehicle on highways and some other roads to which the public have access. In England, Scotland and Wales they are administered by the Driver and Vehicle Licensing Agency (DVLA) and in Northern Ireland by the Driver & Vehicle Agency (DVA). A driving licence is required in the UK by any person driving a vehicle on any highway or other road defined in s.192 Road Traffic Act 1988[1] irrespective of ownership of the land over which the road passes, thus including many which allow the public to pass over private land; similar requirements apply in Northern Ireland under the Road Traffic (Northern Ireland) Order 1981. (Source <a href="https://en.wikipedia.org/wiki/Driving_licence_in_the_United_Kingdom">Wikipedia</a>)
</pre>
<center><img src="https://raw.githubusercontent.com/adrianeyre/codewars/master/Ruby/Authored/drivinglicense.jpg" alt="Driving"></center>
# Task
<pre style="white-space: pre-wrap;white-space: -moz-pre-wrap;white-space: -pre-wrap;white-space: -o-pre-wrap;word-wrap: break-word;">
The UK driving number is made up from the personal details of the driver. The individual letters and digits can be code using the below information
</pre>
# Rules
<pre style="white-space: pre-wrap;white-space: -moz-pre-wrap;white-space: -pre-wrap;white-space: -o-pre-wrap;word-wrap: break-word;">
1–5: The first five characters of the surname (padded with 9s if less than 5 characters)
6: The decade digit from the year of birth (e.g. for 1987 it would be 8)
7–8: The month of birth (7th character incremented by 5 if driver is female i.e. 51–62 instead of 01–12)
9–10: The date within the month of birth
11: The year digit from the year of birth (e.g. for 1987 it would be 7)
12–13: The first two initials of the first name and middle name, padded with a 9 if no middle name
14: Arbitrary digit – usually 9, but decremented to differentiate drivers with the first 13 characters in common. We will always use 9
15–16: Two computer check digits. We will always use "AA"
</pre>
Your task is to code a UK driving license number using an Array of data. The Array will look like
```ruby
data = ["John","James","Smith","01-Jan-2000","M"]
```
```python
data = ["John","James","Smith","01-Jan-2000","M"]
```
```coffeescript
data = ["John","James","Smith","01-Jan-2000","M"]
```
```crystal
data = ["John","James","Smith","01-Jan-2000","M"]
```
```javascript
data = ["John","James","Smith","01-Jan-2000","M"];
```
```csharp
data = ["John","James","Smith","01-Jan-2000","M"];
```
```c
data = {"John","James","Smith","01-Jan-2000","M"};
```
```cpp
data = {"John","James","Smith","01-Jan-2000","M"};
```
```java
data = {"John","James","Smith","01-Jan-2000","M"};
```
Where the elements are as follows
<pre style="white-space: pre-wrap;white-space: -moz-pre-wrap;white-space: -pre-wrap;white-space: -o-pre-wrap;word-wrap: break-word;">
0 = Forename
1 = Middle Name (if any)
2 = Surname
3 = Date of Birth (In the format Day Month Year, this could include the full Month name or just shorthand ie September or Sep)
4 = M-Male or F-Female
</pre>
You will need to output the full 16 digit driving license number.
Good luck and enjoy!
# Kata Series
If you enjoyed this, then please try one of my other Katas. Any feedback, translations and grading of beta Katas are greatly appreciated. Thank you.
<span style="display: flex !important;"><img style="margin:0px;" src="https://raw.githubusercontent.com/adrianeyre/codewars/master/Ruby/Authored/6KYU.png" alt="Rank"/> <a href="https://www.codewars.com/kata/58663693b359c4a6560001d6" target="_blank">Maze Runner</a></span>
<span style="display: flex !important;"><img style="margin:0px;" src="https://raw.githubusercontent.com/adrianeyre/codewars/master/Ruby/Authored/6KYU.png" alt="Rank"/> <a href="https://www.codewars.com/kata/58693bbfd7da144164000d05" target="_blank">Scooby Doo Puzzle</a></span>
<span style="display: flex !important;"><img style="margin:0px;" src="https://raw.githubusercontent.com/adrianeyre/codewars/master/Ruby/Authored/7KYU.png" alt="Rank"/> <a href="https://www.codewars.com/kata/586a1af1c66d18ad81000134" target="_blank">Driving License</a></span>
<span style="display: flex !important;"><img style="margin:0px;" src="https://raw.githubusercontent.com/adrianeyre/codewars/master/Ruby/Authored/6KYU.png" alt="Rank"/> <a href="https://www.codewars.com/kata/586c0909c1923fdb89002031" target="_blank">Connect 4</a></span>
<span style="display: flex !important;"><img style="margin:0px;" src="https://raw.githubusercontent.com/adrianeyre/codewars/master/Ruby/Authored/6KYU.png" alt="Rank"/> <a href="https://www.codewars.com/kata/586e6d4cb98de09e3800014f" target="_blank">Vending Machine</a></span>
<span style="display: flex !important;"><img style="margin:0px;" src="https://raw.githubusercontent.com/adrianeyre/codewars/master/Ruby/Authored/6KYU.png" alt="Rank"/> <a href="https://www.codewars.com/kata/587136ba2eefcb92a9000027" target="_blank">Snakes and Ladders</a></span>
<span style="display: flex !important;"><img style="margin:0px;" src="https://raw.githubusercontent.com/adrianeyre/codewars/master/Ruby/Authored/6KYU.png" alt="Rank"/> <a href="https://www.codewars.com/kata/58a848258a6909dd35000003" target="_blank">Mastermind</a></span>
<span style="display: flex !important;"><img style="margin:0px;" src="https://raw.githubusercontent.com/adrianeyre/codewars/master/Ruby/Authored/6KYU.png" alt="Rank"/> <a href="https://www.codewars.com/kata/58b2c5de4cf8b90723000051" target="_blank">Guess Who?</a></span>
<span style="display: flex !important;"><img style="margin:0px;" src="https://raw.githubusercontent.com/adrianeyre/codewars/master/Ruby/Authored/6KYU.png" alt="Rank"/> <a href="https://www.codewars.com/kata/58f5c63f1e26ecda7e000029" target="_blank">Am I safe to drive?</a></span>
<span style="display: flex !important;"><img style="margin:0px;" src="https://raw.githubusercontent.com/adrianeyre/codewars/master/Ruby/Authored/6KYU.png" alt="Rank"/> <a href="https://www.codewars.com/kata/58f5c63f1e26ecda7e000029" target="_blank">Mexican Wave</a></span>
<span style="display: flex !important;"><img style="margin:0px;" src="https://raw.githubusercontent.com/adrianeyre/codewars/master/Ruby/Authored/6KYU.png" alt="Rank"/> <a href="https://www.codewars.com/kata/58fdcc51b4f81a0b1e00003e" target="_blank">Pigs in a Pen</a></span>
<span style="display: flex !important;"><img style="margin:0px;" src="https://raw.githubusercontent.com/adrianeyre/codewars/master/Ruby/Authored/6KYU.png" alt="Rank"/> <a href="https://www.codewars.com/kata/590300eb378a9282ba000095" target="_blank">Hungry Hippos</a></span>
<span style="display: flex !important;"><img style="margin:0px;" src="https://raw.githubusercontent.com/adrianeyre/codewars/master/Ruby/Authored/6KYU.png" alt="Rank"/> <a href="https://www.codewars.com/kata/5904be220881cb68be00007d" target="_blank">Plenty of Fish in the Pond</a></span>
<span style="display: flex !important;"><img style="margin:0px;" src="https://raw.githubusercontent.com/adrianeyre/codewars/master/Ruby/Authored/6KYU.png" alt="Rank"/> <a href="https://www.codewars.com/kata/590adadea658017d90000039" target="_blank">Fruit Machine</a></span>
<span style="display: flex !important;"><img style="margin:0px;" src="https://raw.githubusercontent.com/adrianeyre/codewars/master/Ruby/Authored/6KYU.png" alt="Rank"/> <a href="https://www.codewars.com/kata/591eab1d192fe0435e000014" target="_blank">Car Park Escape</a></span> | reference | from datetime import datetime
def driver(data):
first, middle, last, dob, gender = data
try:
d = datetime . strptime(dob, '%d-%b-%Y')
except ValueError:
d = datetime . strptime(dob, '%d-%B-%Y')
return '{:9<5}{[2]}{:0>2}{:0>2}{[3]}{[0]}{[0]}9AA' . format(
last[: 5]. upper(),
str(d . year),
d . month + (50 if gender == 'F' else 0),
d . day,
str(d . year),
first,
middle if middle else '9')
| Driving Licence | 586a1af1c66d18ad81000134 | [
"Strings",
"Arrays",
"Fundamentals"
] | https://www.codewars.com/kata/586a1af1c66d18ad81000134 | 7 kyu |
# Introduction
<pre style="white-space: pre-wrap;white-space: -moz-pre-wrap;white-space: -pre-wrap;white-space: -o-pre-wrap;word-wrap: break-word;">
A vending machine is a machine that dispenses items such as snacks and beverages to customers automatically, after the customer inserts currency or credit into the machine. The first modern vending machines were developed in England in the early 19th century and dispensed postcards. (Source <a href="https://en.wikipedia.org/wiki/Vending_machine">Wikipedia</a>)
</pre>
<center><img src="https://raw.githubusercontent.com/adrianeyre/codewars/master/Ruby/Authored/vendingmachine.jpg" alt="Vending Machine"></center>
# Task
<pre style="white-space: pre-wrap;white-space: -moz-pre-wrap;white-space: -pre-wrap;white-space: -o-pre-wrap;word-wrap: break-word;">
In this simple Kata aimed at beginners your task is to recreate a vending machine. You will have to make a class called <font color="#A1A85E">VendingMachine</font> with at least one method called <font color="#A1A85E">vend</font>. On creation of a new instance of <font color="#A1A85E">VendingMachine</font> the <font color="#A1A85E">items</font> Array and <font color="#A1A85E">Initial vending machine money</font> is passed. The <font color="#A1A85E">vend</font> method should take two arguments <font color="#A1A85E">1. Selection code of the item (not case sensitive)</font> and <font color="#A1A85E">2. Amount of money the user inserts into the machine.</font>
</pre>
An example call of the <font color="#ebf265">vend</font> method
```c
machine.vend("A01", 0.90)
```
where the selected item is `A01` and the money given to the machine is `90p`
An example of the items Array is below
```ruby
items = [{:name=>"Smarties", :code=>"A01", :quantity=>10, :price=>0.60},
{:name=>"Caramac Bar", :code=>"A02", :quantity=>5, :price=>0.60},
{:name=>"Dairy Milk", :code=>"A03", :quantity=>1, :price=>0.65},
{:name=>"Freddo", :code=>"A04", :quantity=>1, :price=>0.25}]
```
```javascript
var items = [{name:"Smarties", code:"A01", quantity:10, price:0.60},
{name:"Caramac Bar", code:"A02", quantity:5, price:0.60},
{name:"Dairy Milk", code:"A03", quantity:1, price:0.65},
{name:"Freddo", code:"A04", quantity:1, price:0.25}];
```
```python
items = [{'name':"Smarties", 'code':"A01", 'quantity':10, 'price':0.60},
{'name':"Caramac Bar", 'code':"A02", 'quantity':5, 'price':0.60},
{'name':"Dairy Milk", 'code':"A03", 'quantity':1, 'price':0.65},
{'name':"Freddo", 'code':"A04", 'quantity':1, 'price':0.25}];
```
# Rules
<pre style="white-space: pre-wrap;white-space: -moz-pre-wrap;white-space: -pre-wrap;white-space: -o-pre-wrap;word-wrap: break-word;">
1. If the money given to the machine is less than the item cost return "<font color="#A1A85E">Not enough money!</font>"
2. If the quantity is 0 for an item return "<font color="#A1A85E">Item Name: Out of stock!</font>". Where "Item Name" is the name of the item selected.
3. If an item is correctly selected return "<font color="#A1A85E">Vending Item Name with 9.40 change.</font>". Where "Item Name" is the name of the item and change if any given.
4. If an item is correctly selected and there is no change needed then return "<font color="#A1A85E">Vending Item Name</font>". Where "Item Name" is the name of the item.
5. If an invalid item is selected return "<font color="#A1A85E">Invalid selection! : Money in vending machine = 11.20</font>". Where 11.20 is the machines money float.
6. If a selection is successful then the quantity should be updated.
7. The vending machine never runs out of money for simplicity however you will need to keep track of the amount of money in the machine at anytime (this is not tested in any of the test cases)
8. Change is always given to 2 decimal places ie 7.00
</pre>
Good luck and Enjoy
# Kata Series
If you enjoyed this, then please try one of my other Katas. Any feedback, translations and grading of beta Katas are greatly appreciated. Thank you.
<span style="display: flex !important;"><img style="margin:0px;" src="https://raw.githubusercontent.com/adrianeyre/codewars/master/Ruby/Authored/6KYU.png" alt="Rank"/> <a href="https://www.codewars.com/kata/58663693b359c4a6560001d6" target="_blank">Maze Runner</a></span>
<span style="display: flex !important;"><img style="margin:0px;" src="https://raw.githubusercontent.com/adrianeyre/codewars/master/Ruby/Authored/6KYU.png" alt="Rank"/> <a href="https://www.codewars.com/kata/58693bbfd7da144164000d05" target="_blank">Scooby Doo Puzzle</a></span>
<span style="display: flex !important;"><img style="margin:0px;" src="https://raw.githubusercontent.com/adrianeyre/codewars/master/Ruby/Authored/7KYU.png" alt="Rank"/> <a href="https://www.codewars.com/kata/586a1af1c66d18ad81000134" target="_blank">Driving License</a></span>
<span style="display: flex !important;"><img style="margin:0px;" src="https://raw.githubusercontent.com/adrianeyre/codewars/master/Ruby/Authored/6KYU.png" alt="Rank"/> <a href="https://www.codewars.com/kata/586c0909c1923fdb89002031" target="_blank">Connect 4</a></span>
<span style="display: flex !important;"><img style="margin:0px;" src="https://raw.githubusercontent.com/adrianeyre/codewars/master/Ruby/Authored/6KYU.png" alt="Rank"/> <a href="https://www.codewars.com/kata/586e6d4cb98de09e3800014f" target="_blank">Vending Machine</a></span>
<span style="display: flex !important;"><img style="margin:0px;" src="https://raw.githubusercontent.com/adrianeyre/codewars/master/Ruby/Authored/6KYU.png" alt="Rank"/> <a href="https://www.codewars.com/kata/587136ba2eefcb92a9000027" target="_blank">Snakes and Ladders</a></span>
<span style="display: flex !important;"><img style="margin:0px;" src="https://raw.githubusercontent.com/adrianeyre/codewars/master/Ruby/Authored/6KYU.png" alt="Rank"/> <a href="https://www.codewars.com/kata/58a848258a6909dd35000003" target="_blank">Mastermind</a></span>
<span style="display: flex !important;"><img style="margin:0px;" src="https://raw.githubusercontent.com/adrianeyre/codewars/master/Ruby/Authored/6KYU.png" alt="Rank"/> <a href="https://www.codewars.com/kata/58b2c5de4cf8b90723000051" target="_blank">Guess Who?</a></span>
<span style="display: flex !important;"><img style="margin:0px;" src="https://raw.githubusercontent.com/adrianeyre/codewars/master/Ruby/Authored/6KYU.png" alt="Rank"/> <a href="https://www.codewars.com/kata/58f5c63f1e26ecda7e000029" target="_blank">Am I safe to drive?</a></span>
<span style="display: flex !important;"><img style="margin:0px;" src="https://raw.githubusercontent.com/adrianeyre/codewars/master/Ruby/Authored/6KYU.png" alt="Rank"/> <a href="https://www.codewars.com/kata/58f5c63f1e26ecda7e000029" target="_blank">Mexican Wave</a></span>
<span style="display: flex !important;"><img style="margin:0px;" src="https://raw.githubusercontent.com/adrianeyre/codewars/master/Ruby/Authored/6KYU.png" alt="Rank"/> <a href="https://www.codewars.com/kata/58fdcc51b4f81a0b1e00003e" target="_blank">Pigs in a Pen</a></span>
<span style="display: flex !important;"><img style="margin:0px;" src="https://raw.githubusercontent.com/adrianeyre/codewars/master/Ruby/Authored/6KYU.png" alt="Rank"/> <a href="https://www.codewars.com/kata/590300eb378a9282ba000095" target="_blank">Hungry Hippos</a></span>
<span style="display: flex !important;"><img style="margin:0px;" src="https://raw.githubusercontent.com/adrianeyre/codewars/master/Ruby/Authored/6KYU.png" alt="Rank"/> <a href="https://www.codewars.com/kata/5904be220881cb68be00007d" target="_blank">Plenty of Fish in the Pond</a></span>
<span style="display: flex !important;"><img style="margin:0px;" src="https://raw.githubusercontent.com/adrianeyre/codewars/master/Ruby/Authored/6KYU.png" alt="Rank"/> <a href="https://www.codewars.com/kata/590adadea658017d90000039" target="_blank">Fruit Machine</a></span>
<span style="display: flex !important;"><img style="margin:0px;" src="https://raw.githubusercontent.com/adrianeyre/codewars/master/Ruby/Authored/6KYU.png" alt="Rank"/> <a href="https://www.codewars.com/kata/591eab1d192fe0435e000014" target="_blank">Car Park Escape</a></span> | reference | class Item ():
def __init__(self, item):
self . name = item["name"]
self . quantity = item["quantity"]
self . price = item["price"]
class VendingMachine ():
def __init__(self, items, money):
self . items = {item["code"]: Item(item) for item in items}
self . money = money
def vend(self, selection, item_money):
if selection . upper() not in self . items:
return "Invalid selection! : Money in vending machine = {:.2f}" . format(self . money)
item = self . items[selection . upper()]
change = item_money - item . price
if change < 0:
return "Not enough money!"
if item . quantity == 0:
return item . name + ": Out of stock!"
item . quantity -= 1
self . money += item . price
return "Vending " + item . name + (" with {:.2f} change." . format(change)) * (change > 0)
| Vending Machine | 586e6d4cb98de09e3800014f | [
"Arrays",
"Fundamentals"
] | https://www.codewars.com/kata/586e6d4cb98de09e3800014f | 6 kyu |
# Introduction
<pre style="white-space: pre-wrap;white-space: -moz-pre-wrap;white-space: -pre-wrap;white-space: -o-pre-wrap;word-wrap: break-word;">
Snakes and Ladders is an ancient Indian board game regarded today as a worldwide classic. It is played between two or more players on a gameboard having numbered, gridded squares. A number of "ladders" and "snakes" are pictured on the board, each connecting two specific board squares. (Source <a href="https://en.wikipedia.org/wiki/Snakes_and_Ladders">Wikipedia</a>)
</pre>
<center><img src="https://raw.githubusercontent.com/adrianeyre/codewars/master/Ruby/Authored/snakesandladders.jpg"></center>
# Task
<pre style="white-space: pre-wrap;white-space: -moz-pre-wrap;white-space: -pre-wrap;white-space: -o-pre-wrap;word-wrap: break-word;">
Your task is to make a simple class called <font color="#A1A85E">SnakesLadders</font>. The test cases will call the method <font color="#A1A85E">play(die1, die2)</font> independantly of the state of the game or the player turn. The variables <font color="#A1A85E">die1</font> and <font color="#A1A85E">die2</font> are the die thrown in a turn and are both integers between 1 and 6. The player will move the sum of die1 and die2.
</pre>
# The Board
<img src="https://raw.githubusercontent.com/adrianeyre/codewars/master/Ruby/Authored/snakesandladdersboard.jpg">
# Rules
<pre style="white-space: pre-wrap;white-space: -moz-pre-wrap;white-space: -pre-wrap;white-space: -o-pre-wrap;word-wrap: break-word;">
1. There are two players and both start off the board on square 0.
2. Player 1 starts and alternates with player 2.
3. You follow the numbers up the board in order 1=>100
4. If the value of both die are the same then that player will have another go.
5. Climb up ladders. The ladders on the game board allow you to move upwards and get ahead faster. If you land exactly on a square that shows an image of the bottom of a ladder, then you may move the player all the way up to the square at the top of the ladder. (even if you roll a double).
6. Slide down snakes. Snakes move you back on the board because you have to slide down them. If you land exactly at the top of a snake, slide move the player all the way to the square at the bottom of the snake or chute. (even if you roll a double).
7. Land exactly on the last square to win. The first person to reach the highest square on the board wins. But there's a twist! If you roll too high, your player "bounces" off the last square and moves back. You can only win by rolling the exact number needed to land on the last square. For example, if you are on square 98 and roll a five, move your game piece to 100 (two moves), then "bounce" back to 99, 98, 97 (three, four then five moves.)
8. If the Player rolled a double and lands on the finish square “100” without any remaining moves then the Player wins the game and does not have to roll again.
</pre>
# Returns
<pre style="white-space: pre-wrap;white-space: -moz-pre-wrap;white-space: -pre-wrap;white-space: -o-pre-wrap;word-wrap: break-word;">
Return <font color="#A1A85E">Player n Wins!</font>. Where n is winning player that has landed on square 100 without any remainding moves left.
Return <font color="#A1A85E">Game over!</font> if a player has won and another player tries to play.
Otherwise return <font color="#A1A85E">Player n is on square x</font>. Where n is the current player and x is the sqaure they are currently on.
</pre>
Good luck and enjoy!
# Kata Series
If you enjoyed this, then please try one of my other Katas. Any feedback, translations and grading of beta Katas are greatly appreciated. Thank you.
<span style="display: flex !important;"><img style="margin:0px;" src="https://raw.githubusercontent.com/adrianeyre/codewars/master/Ruby/Authored/6KYU.png" alt="Rank"/> <a href="https://www.codewars.com/kata/58663693b359c4a6560001d6" target="_blank">Maze Runner</a></span>
<span style="display: flex !important;"><img style="margin:0px;" src="https://raw.githubusercontent.com/adrianeyre/codewars/master/Ruby/Authored/6KYU.png" alt="Rank"/> <a href="https://www.codewars.com/kata/58693bbfd7da144164000d05" target="_blank">Scooby Doo Puzzle</a></span>
<span style="display: flex !important;"><img style="margin:0px;" src="https://raw.githubusercontent.com/adrianeyre/codewars/master/Ruby/Authored/7KYU.png" alt="Rank"/> <a href="https://www.codewars.com/kata/586a1af1c66d18ad81000134" target="_blank">Driving License</a></span>
<span style="display: flex !important;"><img style="margin:0px;" src="https://raw.githubusercontent.com/adrianeyre/codewars/master/Ruby/Authored/6KYU.png" alt="Rank"/> <a href="https://www.codewars.com/kata/586c0909c1923fdb89002031" target="_blank">Connect 4</a></span>
<span style="display: flex !important;"><img style="margin:0px;" src="https://raw.githubusercontent.com/adrianeyre/codewars/master/Ruby/Authored/6KYU.png" alt="Rank"/> <a href="https://www.codewars.com/kata/586e6d4cb98de09e3800014f" target="_blank">Vending Machine</a></span>
<span style="display: flex !important;"><img style="margin:0px;" src="https://raw.githubusercontent.com/adrianeyre/codewars/master/Ruby/Authored/6KYU.png" alt="Rank"/> <a href="https://www.codewars.com/kata/587136ba2eefcb92a9000027" target="_blank">Snakes and Ladders</a></span>
<span style="display: flex !important;"><img style="margin:0px;" src="https://raw.githubusercontent.com/adrianeyre/codewars/master/Ruby/Authored/6KYU.png" alt="Rank"/> <a href="https://www.codewars.com/kata/58a848258a6909dd35000003" target="_blank">Mastermind</a></span>
<span style="display: flex !important;"><img style="margin:0px;" src="https://raw.githubusercontent.com/adrianeyre/codewars/master/Ruby/Authored/6KYU.png" alt="Rank"/> <a href="https://www.codewars.com/kata/58b2c5de4cf8b90723000051" target="_blank">Guess Who?</a></span>
<span style="display: flex !important;"><img style="margin:0px;" src="https://raw.githubusercontent.com/adrianeyre/codewars/master/Ruby/Authored/6KYU.png" alt="Rank"/> <a href="https://www.codewars.com/kata/58f5c63f1e26ecda7e000029" target="_blank">Am I safe to drive?</a></span>
<span style="display: flex !important;"><img style="margin:0px;" src="https://raw.githubusercontent.com/adrianeyre/codewars/master/Ruby/Authored/6KYU.png" alt="Rank"/> <a href="https://www.codewars.com/kata/58f5c63f1e26ecda7e000029" target="_blank">Mexican Wave</a></span>
<span style="display: flex !important;"><img style="margin:0px;" src="https://raw.githubusercontent.com/adrianeyre/codewars/master/Ruby/Authored/6KYU.png" alt="Rank"/> <a href="https://www.codewars.com/kata/58fdcc51b4f81a0b1e00003e" target="_blank">Pigs in a Pen</a></span>
<span style="display: flex !important;"><img style="margin:0px;" src="https://raw.githubusercontent.com/adrianeyre/codewars/master/Ruby/Authored/6KYU.png" alt="Rank"/> <a href="https://www.codewars.com/kata/590300eb378a9282ba000095" target="_blank">Hungry Hippos</a></span>
<span style="display: flex !important;"><img style="margin:0px;" src="https://raw.githubusercontent.com/adrianeyre/codewars/master/Ruby/Authored/6KYU.png" alt="Rank"/> <a href="https://www.codewars.com/kata/5904be220881cb68be00007d" target="_blank">Plenty of Fish in the Pond</a></span>
<span style="display: flex !important;"><img style="margin:0px;" src="https://raw.githubusercontent.com/adrianeyre/codewars/master/Ruby/Authored/6KYU.png" alt="Rank"/> <a href="https://www.codewars.com/kata/590adadea658017d90000039" target="_blank">Fruit Machine</a></span>
<span style="display: flex !important;"><img style="margin:0px;" src="https://raw.githubusercontent.com/adrianeyre/codewars/master/Ruby/Authored/6KYU.png" alt="Rank"/> <a href="https://www.codewars.com/kata/591eab1d192fe0435e000014" target="_blank">Car Park Escape</a></span> | reference | class SnakesLadders:
snakes_and_ladders = {
2: 38,
7: 14,
8: 31,
15: 26,
21: 42,
28: 84,
36: 44,
51: 67,
71: 91,
78: 98,
87: 94,
16: 6,
46: 25,
49: 11,
62: 19,
64: 60,
74: 53,
89: 68,
92: 88,
95: 75,
99: 80,
}
def __init__(self):
self . players = [(1, 0), (2, 0)]
def play(self, die1, die2):
if any(square == 100 for player, square in self . players):
return "Game over!"
new_square = self . players[0][1] + die1 + die2
if new_square > 100:
new_square = 200 - new_square
new_square = self . snakes_and_ladders . get(new_square, new_square)
self . players[0] = (self . players[0][0], new_square)
result = ("Player {0} Wins!"
if new_square == 100
else "Player {0} is on square {1}"). format(* self . players[0])
if die1 != die2:
self . players . reverse()
return result
| Snakes and Ladders | 587136ba2eefcb92a9000027 | [
"Design Patterns",
"Games",
"Fundamentals"
] | https://www.codewars.com/kata/587136ba2eefcb92a9000027 | 5 kyu |
You need to design a recursive function called ```replicate``` which will receive arguments ```times``` and ```number```.
The function should return an array containing repetitions of the ```number``` argument. For instance, ```replicate(3, 5)``` should return ```[5,5,5]```. If the ```times``` argument is negative, return an empty array.
As tempting as it may seem, do not use loops to solve this problem.
| algorithms | @ countcalls
def replicate(times, number):
if times > 0:
return [number] + replicate(times - 1, number)
return []
| Recursive Replication | 57547f9182655569ab0008c4 | [
"Recursion",
"Algorithms"
] | https://www.codewars.com/kata/57547f9182655569ab0008c4 | 7 kyu |
Write a function which reduces fractions to their simplest form! Fractions will be presented as an array/tuple (depending on the language) of strictly positive integers, and the reduced fraction must be returned as an array/tuple:
```
input: [numerator, denominator]
output: [reduced numerator, reduced denominator]
example: [45, 120] --> [3, 8]
```
All numerators and denominators will be positive integers.
Note: This is an introductory Kata for a series... coming soon!
| reference | from fractions import Fraction
def reduce_fraction(fraction):
t = Fraction(* fraction)
return (t . numerator, t . denominator)
| Reduce My Fraction | 576400f2f716ca816d001614 | [
"Fundamentals",
"Recursion",
"Algorithms"
] | https://www.codewars.com/kata/576400f2f716ca816d001614 | 7 kyu |
<h2>Write a function that sums squares of numbers in list that may contain more lists</h2>
Example:
```javascript
var l = [1,2,3]
SumSquares(l) == 14
var l = [[1,2],3]
SumSquares(l) == 14
var l = [[[[[[[[[1]]]]]]]]]
SumSquares(l) == 1
var l = [10,[[10],10],[10]]
SumSquares(l) == 400
```
Note: your solution must NOT modify the original list
Another Kata involving nested lists here (it's still in beta - more honor points for completing :D): https://www.codewars.com/kata/5786f020e55533ebb7000153 | reference | def sumsquares(l):
return sum([i * * 2 if type(i) == int else sumsquares(i) for i in l])
| Sum squares of numbers in list that may contain more lists | 57882daf90b2f375280000ad | [
"Fundamentals",
"Recursion"
] | https://www.codewars.com/kata/57882daf90b2f375280000ad | 7 kyu |
### self_converge
`Goal`: Given a number (with a minimum of 3 digits), return the number of iterations it takes to arrive at a derived number that converges on to itself, as per the following [Kaprekar][Kaprekar] routine. As a learning exercise, come up with a solution that uses recursion. The
following steps would be applicable to a number that originally had
exactly 4 digits.
0. Initialize a counter to count the number of iterations
1. Take any four-digit number `n`, using at least two different digits.
2. Arrange the digits in descending and then in ascending order to get two four-digit numbers, adding leading zeros if necessary.
- Add as many zeroes so that the width of the original number is maintained.
3. Subtract the smaller number from the bigger number. Let us call this `nseq`.
4. Check if `nseq` (the remainder) from Step 4 equals the previous value of `n`. If not, increment the iteration counter and go back to step 2 and perform it on the `nseq`.
If the number of digits to start with was more than 4, convergence occurs on to a `cycle` of numbers. Therefore in Step 5, detect this cycle by comparing to not only the previous value, but to all previous values of `n`.
- If there is a match, then return the count of iterations
- If the sequence_number collapses to zero, then return -1
[Kaprekar]: http://mathworld.wolfram.com/KaprekarRoutine.html
### Converge values
While 3-digit numbers converge to the same unique number `k` which is also 3 digits long, all 4-digit numbers also converge to the same unique value `k1` which is 4 digits long. However, 5 digit numbers converge to any one of the following values: `53955, 59994, 61974, 62964, 63954, 71973, 74943, 75933, 82962, 83952`.
### Example
`1234` -> `4`
```
1. 4321 - 1234 = 3087 /
2. 8730 - 378 = 8352 /
3. 8532 - 2358 = 6174 /
4. 7641 - 1467 = 6174 // same as previous
```
`414` -> `5`
```
1. 441 - 144 = 297 /
2. 972 - 279 = 693 /
3. 963 - 369 = 594 /
4. 954 - 459 = 495 /
5. 954 - 459 = 495 // same as previous
```
`50000` -> `4`
```
1. 50000 - 5 = 49995 /
2. 99954 - 45999 = 53955 / # first
3. 95553 - 35559 = 59994 /
4. 99954 - 45999 = 53955 / # 2nd time
```
| algorithms | def self_converge(number):
n, cycle = str(number), set()
while n not in cycle:
cycle . add(n)
s = '' . join(sorted(n))
n = '%0*d' % (len(n), int(s[:: - 1]) - int(s))
return - 1 if not int(n) else len(cycle)
| self_converge | 54ab259e15613ce0c8001bab | [
"Recursion",
"Algorithms"
] | https://www.codewars.com/kata/54ab259e15613ce0c8001bab | 5 kyu |
# Convert a linked list to a string
## Related Kata
Although this Kata is not part of an official Series, you may also want to try out [Parse a linked list from a string](https://www.codewars.com/kata/582c5382f000e535100001a7) if you enjoyed this Kata.
## Preloaded
Preloaded for you is a class, struct or derived data type `Node` ( depending on the language ) used to construct linked lists in this Kata:
<!-- unlisted languages use the top block -- please keep c up top -->
```c
typedef struct node {
int data;
struct node *next;
} Node;
```
```cobol
* Defined in the linkage section
01 node.
05 val pic 9(4).
05 nxt usage pointer.
```
```php
class Node {
public $data, $next;
public function __construct($data, $next = NULL) {
$this->data = $data;
$this->next = $next;
}
}
```
```javascript
class Node {
constructor(data, next = null) {
this.data = data;
this.next = next;
}
}
```
```csharp
public class Node {
public int Data { get; private set; }
public Node Next { get; private set; }
public Node(int data, Node next = null) {
Data = data;
Next = next;
}
}
```
```python
class Node():
def __init__(self, data, next = None):
self.data = data
self.next = next
```
```java
class Node {
private int data;
private Node next;
public Node(int data, Node next) {
this.data = data;
this.next = next;
}
public Node(int data) {
this.data = data;
this.next = null;
}
public int getData() {
return data;
}
public Node getNext() {
return next;
}
}
```
```ruby
class Node
attr_reader :data, :next_node
def initialize(data, next_node=nil)
@data = data
@next_node = next_node
end
end
```
```cpp
class Node
{
public:
int data;
Node* next;
Node(int data, Node* next = nullptr)
{
this->data = data;
this->next = next;
}
};
```
```haskell
-- use regular lists, which are already singly-linked
data [a] = [] | a : [a]
```
```objc
typedef struct node {
int data;
struct node *next;
} Node;
```
```fortran
type Node
integer :: data
type(Node), pointer :: next
end type Node
```
```factor
SYMBOL: +nil+
TUPLE: node data next ;
C: <node> node
```
~~~if:objc
*NOTE: In Objective-C, the* `Node` *struct is placed on top of your main solution because there is a "double-import" bug in the Preloaded section at the time of writing (which cannot be fixed on my end). Attempts to modify it (e.g. to cheat the tests in some way) will likely result in a test crash so it is not recommended for you to modify that section ;)*
~~~
~~~if:c
*NOTE: In C, the* `Node` *struct is placed on top of your main solution (and the [Sample] Test Cases) because the compiler complains about not recognizing the* `Node` *datatype even after adding it to the Preloaded section. Attempts to modify it (e.g. to cheat the tests in some way) will likely result in a test crash so it is not recommended for you to modify that section ;)*
~~~
~~~if:nasm
When attempting this Kata in NASM, note that the code example shown directly above may not be relevant; please refer to the Sample Tests (written in C) for the exact definition of the `Node` structure.
~~~
## Prerequisites
This Kata assumes that you are already familiar with the idea of a linked list. If you do not know what that is, you may want to read [this article on Wikipedia](https://en.wikipedia.org/wiki/Linked_list). Specifically, the linked lists this Kata is referring to are **singly linked lists**, where the value of a specific node is stored in its `data` / `$data` / `Data` property, the reference to the next node is stored in its `next` / `$next` / `Next` / `next_node` property and the terminator for a list is `null` / `NULL` / `None` / `nil` / `nullptr` / `null()`.
## Task
~~~if:nasm
*If you are attempting this Kata in NASM, the code examples shown below may not be relevant at all - please refer to the Sample Tests (written in C) for the exact requirements.*
~~~
Create a function `stringify` which accepts an argument `list` / `$list` and returns a string representation of the list. The string representation of the list starts with the value of the current `Node`, specified by its `data` / `$data` / `Data` property, followed by a whitespace character, an arrow and another whitespace character (`" -> "`), followed by the rest of the list. The end of the string representation of a list must always end with `null` / `NULL` / `None` / `nil` / `nullptr` / `null()` ( all caps or all lowercase depending on the language you are undertaking this Kata in ). For example, given the following list:
<!-- unlisted languages use the top block -- please keep c up top -->
```c
&((Node){
.data = 1,
.next = &((Node){
.data = 2,
.next = &((Node){
.data = 3,
.next = NULL
})
})
})
```
```php
new Node(1, new Node(2, new Node(3)))
```
```javascript
new Node(1, new Node(2, new Node(3)))
```
```csharp
new Node(1, new Node(2, new Node(3)))
```
```python
Node(1, Node(2, Node(3)))
```
```java
new Node(1, new Node(2, new Node(3)))
```
```ruby
Node.new(1, Node.new(2, Node.new(3)))
```
```cpp
new Node(1, new Node(2, new Node(3)))
```
```haskell
[1,2,3]
```
```objc
&((Node){
.data = 1,
.next = &((Node){
.data = 2,
.next = &((Node){
.data = 3,
.next = NULL
})
})
})
```
```fortran
type(Node), pointer :: oneTwoThree
! Where:
! oneTwoThree%data == 1
! oneTwoThree%next%data == 2
! oneTwoThree%next%next%data == 3
! oneTwoThree%next%next%next => null()
```
```factor
1 2 3 +nil+ <node> <node> <node>
```
```cobol
01 node1.
05 val pic 9(4) value 1.
05 nxt usage pointer.
01 node2.
05 val pic 9(4) value 2.
05 nxt usage pointer.
01 node3.
05 val pic 9(4) value 3.
05 nxt usage pointer value null.
...
set nxt of node1 to address of node2
set nxt of node2 to address of node3
```
... its string representation would be:
<!-- unlisted languages use the top block -- please keep javascript up top -->
```javascript
"1 -> 2 -> 3 -> null"
```
```php
"1 -> 2 -> 3 -> NULL"
```
```c
"1 -> 2 -> 3 -> NULL"
```
```python
"1 -> 2 -> 3 -> None"
```
```ruby
"1 -> 2 -> 3 -> nil"
```
```cpp
"1 -> 2 -> 3 -> nullptr"
```
```objc
@"1 -> 2 -> 3 -> NULL"
```
```fortran
"1 -> 2 -> 3 -> null()"
```
```factor
"1 -> 2 -> 3 -> +nil+"
```
```cobol
"1 -> 2 -> 3 -> NULL"
```
And given the following linked list:
<!-- unlisted languages use the top block -- please keep javascript up top -->
```javascript
new Node(0, new Node(1, new Node(4, new Node(9, new Node(16)))))
```
```python
Node(0, Node(1, Node(4, Node(9, Node(16)))))
```
```ruby
Node.new(0, Node.new(1, Node.new(4, Node.new(9, Node.new(16)))))
```
```haskell
[ 0, 1, 4, 9, 16 ]
```
```objc
&((Node){
.data = 0,
.next = &((Node){
.data = 1,
.next = &((Node){
.data = 4,
.next = &((Node){
.data = 9,
.next = &((Node){
.data = 16,
.next = NULL
})
})
})
})
})
```
```c
&((Node){
.data = 0,
.next = &((Node){
.data = 1,
.next = &((Node){
.data = 4,
.next = &((Node){
.data = 9,
.next = &((Node){
.data = 16,
.next = NULL
})
})
})
})
})
```
```fortran
type(Node), pointer :: list
! Where:
! list%data == 0
! list%next%data == 1
! list%next%next%data == 4
! list%next%next%next%data == 9
! list%next%next%next%next%data == 16
! list%next%next%next%next%next => null()
```
```factor
0 1 4 9 16 +nil+ <node> <node> <node> <node> <node>
```
```cobol
01 node1.
05 val pic 9(4) value 0.
05 nxt usage pointer.
01 node2.
05 val pic 9(4) value 1.
05 nxt usage pointer.
01 node3.
05 val pic 9(4) value 4.
05 nxt usage pointer.
01 node4.
05 val pic 9(4) value 9.
05 nxt usage pointer.
01 node5.
05 val pic 9(4) value 16.
05 nxt usage pointer value null.
...
set nxt of node1 to address of node2
set nxt of node2 to address of node3
set nxt of node3 to address of node4
set nxt of node4 to address of node5
```
... its string representation would be:
<!-- unlisted languages use the top block -- please keep javascript up top -->
```javascript
"0 -> 1 -> 4 -> 9 -> 16 -> null"
```
```php
"0 -> 1 -> 4 -> 9 -> 16 -> NULL"
```
```c
"0 -> 1 -> 4 -> 9 -> 16 -> NULL"
```
```python
"0 -> 1 -> 4 -> 9 -> 16 -> None"
```
```ruby
"0 -> 1 -> 4 -> 9 -> 16 -> nil"
```
```cpp
"0 -> 1 -> 4 -> 9 -> 16 -> nullptr"
```
```objc
@"0 -> 1 -> 4 -> 9 -> 16 -> NULL"
```
```fortran
"0 -> 1 -> 4 -> 9 -> 16 -> null()"
```
```factor
"0 -> 1 -> 4 -> 9 -> 16 -> +nil+"
```
```cobol
"0 -> 1 -> 4 -> 9 -> 16 -> NULL"
```
Note that `null` / `NULL` / `None` / `nil` / `nullptr` / `null()` itself is also considered a valid linked list. In that case, its string representation would simply be `"null"` / `"NULL"` / `"None"` / `"nil"` / `"nullptr"` / `@"NULL"` / `"null()"` ( again, depending on the language ).
For the simplicity of this Kata, you may assume that any `Node` in this Kata may only contain **non-negative integer** values. For example, you will not encounter a `Node` whose `data` / `$data` / `Data` property is `"Hello World"`.
Enjoy, and don't forget to check out my other Kata Series :D
~~~if:fortran
_NOTE: In Fortran, your returned string is_ **not** _permitted to contain any leading and/or trailing whitespace._
~~~ | algorithms | def stringify(list):
return 'None' if list == None else str(list . data) + ' -> ' + stringify(list . next)
| Convert a linked list to a string | 582c297e56373f0426000098 | [
"Linked Lists",
"Recursion",
"Algorithms"
] | https://www.codewars.com/kata/582c297e56373f0426000098 | 7 kyu |
Task
======
Make a custom esolang interpreter for the language [InfiniTick](https://esolangs.org/wiki/InfiniTick). InfiniTick is a descendant of [Tick](https://esolangs.org/wiki/tick) but also very different. Unlike Tick, InfiniTick has 8 commands instead of 4. It also runs infinitely, stopping the program only when an error occurs. You may choose to do the [Tick](https://www.codewars.com/kata/esolang-tick) kata first.
Syntax/Info
======
InfiniTick runs in an infinite loop. This makes it both harder and easier to program in. It has an infinite memory of bytes and an infinite output amount. The program only stops when an error is reached. The program is also supposed to ignore non-commands.
Commands
======
`>`: Move data selector right.
`<`: Move data selector left.
`+`: Increment amount of memory cell. Truncate overflow: 255+1=0.
`-`: Decrement amount of memory cell. Truncate overflow: 0-1=255.
`*`: Add ascii value of memory cell to the output tape.
`&`: Raise an error, ie stop the program.
`/`: Skip next command if cell value is zero.
`\`: Skip next command if cell value is nonzero.
Examples
======
**Hello world!**
The following is a valid hello world program in InfiniTick.
```
'++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++*>+++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++*>++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++**>+++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++*>++++++++++++++++++++++++++++++++*>+++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++*<<*>>>++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++*<<<<*>>>>>++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++*>+++++++++++++++++++++++++++++++++*&'
```
Notes
======
* Please be wordy and post your likes/dislikes in the discourse area.
* If this kata is a duplicate or uses incorrect style, this is not an issue, this is a suggestion.
* Please don't edit this kata just to change the estimated rank. Thank you!
| reference | def interpreter(tape):
memory, ptr, output, iCmd = {}, 0, "", 0
while True:
cmd = tape[iCmd]
if cmd == ">":
ptr += 1
elif cmd == "<":
ptr -= 1
elif cmd == "+":
memory[ptr] = (memory . get(ptr, 0) + 1) % 256
elif cmd == "-":
memory[ptr] = (memory . get(ptr, 0) - 1) % 256
elif cmd == "*":
output += chr(memory . get(ptr, 0))
elif cmd == "&":
break
elif cmd == "/":
iCmd += memory . get(ptr, 0) == 0
elif cmd == "\\":
iCmd += memory . get(ptr, 0) != 0
iCmd = (iCmd + 1) % len(tape)
return output
| Esolang: InfiniTick | 58817056e7a31c2ceb000052 | [
"Esoteric Languages",
"Interpreters",
"Strings",
"Arrays"
] | https://www.codewars.com/kata/58817056e7a31c2ceb000052 | 5 kyu |
Task
=======
Make a custom esolang interpreter for the language Tick. Tick is a descendant of [Ticker](https://www.codewars.com/kata/esolang-ticker) but also very different data and command-wise.
Syntax/Info
========
Commands are given in character format. Non-command characters should be ignored. Tick has an potentially infinite memory as opposed to Ticker(which you have a special command to add a new cell) and only has 4 commands(as opposed to 7). Read about this esolang [here](https://esolangs.org/wiki/Tick).
Commands
========
`>`: Move data selector right
`<`: Move data selector left(infinite in both directions)
`+`: Increment memory cell by 1. 255+1=0
`*`: Add ascii value of memory cell to the output tape.
Examples
========
**Hello world!**
```
'++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++*>+++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++*>++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++**>+++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++*>++++++++++++++++++++++++++++++++*>+++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++*<<*>>>++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++*<<<<*>>>>>++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++*>+++++++++++++++++++++++++++++++++*'
``` | reference | def interpreter(tape):
memory, ptr, output = {}, 0, ""
for command in tape:
if command == ">":
ptr += 1
elif command == "<":
ptr -= 1
elif command == "+":
memory[ptr] = (memory . get(ptr, 0) + 1) % 256
elif command == "*":
output += chr(memory[ptr])
return output
| Esolang: Tick | 587edac2bdf76ea23500011a | [
"Esoteric Languages",
"Interpreters",
"Strings",
"Arrays",
"Fundamentals"
] | https://www.codewars.com/kata/587edac2bdf76ea23500011a | 6 kyu |
## Task:
Consider a sequence where the first two numbers are `0` and `1` and the next number of the sequence is the sum of the previous 2 modulo 3. Write a function that finds the nth number of the sequence.
## Constraints:
* #### 1 ≤ N ≤ 10^19
## Example:
```
sequence(1);
0
sequence(2);
1
sequence(3);
1
``` | reference | def sequence(n):
# The sequence of numers repeat after the eighth number
return [0, 1, 1, 2, 0, 2, 2, 1][(n - 1) % 8]
| The Modulo-3 Sequence | 589d33e4e0bbce5d6300061c | [
"Fundamentals"
] | https://www.codewars.com/kata/589d33e4e0bbce5d6300061c | 6 kyu |
### Task
Your friend advised you to see a new performance in the most popular theater in the city. He knows a lot about art and his advice is usually good, but not this time: the performance turned out to be awfully dull. It's so bad you want to sneak out, which is quite simple, especially since the exit is located right behind your row to the left. All you need to do is climb over your seat and make your way to the exit.
The main problem is your shyness: you're afraid that you'll end up blocking the view (even if only for a couple of seconds) of all the people who sit behind you and in your column or the columns to your left. To gain some courage, you decide to calculate the number of such people and see if you can possibly make it to the exit without disturbing too many people.
Given the total number of rows and columns in the theater (nRows and nCols, respectively), and the row and column you're sitting in, return the number of people who sit strictly behind you and in your column or to the left, assuming all seats are occupied.
### Example
For nCols = 16, nRows = 11, col = 5 and row = 3, the output should be 96.
Here is what the theater looks like:
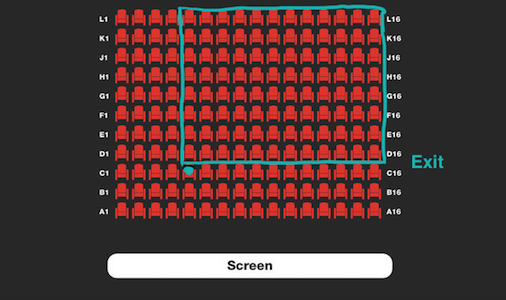
### Input/Output
- `[input]` integer `nCols`
An integer, the number of theater's columns.
Constraints: 1 ≤ `nCols` ≤ 1000.
- `[input]` integer `nRows`
An integer, the number of theater's rows.
Constraints: 1 ≤ `nRows` ≤ 1000.
- `[input]` integer `col`
An integer, the column number of your own seat (with the rightmost column having index 1).
Constraints: 1 ≤ `col` ≤ `nCols`.
- `[input]` integer `row`
An integer, the row number of your own seat (with the front row having index 1).
Constraints: 1 ≤ `row` ≤ `nRows`.
- `[output]` an integer
The number of people who sit strictly behind you and in your column or to the left.
| games | def seats_in_theater(tot_cols, tot_rows, col, row):
return (tot_cols - col + 1) * (tot_rows - row)
| Simple Fun #1: Seats in Theater | 588417e576933b0ec9000045 | [
"Puzzles",
"Mathematics"
] | https://www.codewars.com/kata/588417e576933b0ec9000045 | 8 kyu |
This kata is the first of a sequence of four about "Squared Strings".
You are given a string of `n` lines, each substring being `n` characters long: For example:
`s = "abcd\nefgh\nijkl\nmnop"`
We will study some transformations of this square of strings.
- Vertical mirror:
vert_mirror (or vertMirror or vert-mirror)
```
vert_mirror(s) => "dcba\nhgfe\nlkji\nponm"
```
- Horizontal mirror:
hor_mirror (or horMirror or hor-mirror)
```
hor_mirror(s) => "mnop\nijkl\nefgh\nabcd"
```
or printed:
```
vertical mirror |horizontal mirror
abcd --> dcba |abcd --> mnop
efgh hgfe |efgh ijkl
ijkl lkji |ijkl efgh
mnop ponm |mnop abcd
```
#### Task:
- Write these two functions
and
- high-order function `oper(fct, s)` where
- fct is the function of one variable f to apply to the string `s`
(fct will be one of `vertMirror, horMirror`)
#### Examples:
```
s = "abcd\nefgh\nijkl\nmnop"
oper(vert_mirror, s) => "dcba\nhgfe\nlkji\nponm"
oper(hor_mirror, s) => "mnop\nijkl\nefgh\nabcd"
```
#### Note:
The form of the parameter `fct` in oper
changes according to the language. You can see each form according to the language in "Sample Tests".
#### Bash Note:
The input strings are separated by `,` instead of `\n`. The output strings should be separated by `\r` instead of `\n`. See "Sample Tests".
| reference | def vert_mirror(s):
return "\n" . join(line[:: - 1] for line in s . split("\n"))
def hor_mirror(s):
return "\n" . join(s . split("\n")[:: - 1])
def oper(fct, s):
return fct(s)
| Moves in squared strings (I) | 56dbe0e313c2f63be4000b25 | [
"Fundamentals",
"Algorithms",
"Strings"
] | https://www.codewars.com/kata/56dbe0e313c2f63be4000b25 | 7 kyu |
Your goal is to implement the method **meanVsMedian** which accepts an *odd-length* array of integers and returns one of the following:
* 'mean' - in case **mean** value is **larger than** median value
* 'median' - in case **median** value is **larger than** mean value
* 'same' - in case both mean and median share the **same value**
Reminder: [Median](https://en.wikipedia.org/wiki/Median)
Array will always be valid (odd-length >= 3) | reference | from numpy import mean, median
def mean_vs_median(numbers):
if mean(numbers) > median(numbers):
return 'mean'
elif mean(numbers) < median(numbers):
return 'median'
else:
return 'same'
| Mean vs. Median | 5806445c3f1f9c2f72000031 | [
"Fundamentals",
"Arrays"
] | https://www.codewars.com/kata/5806445c3f1f9c2f72000031 | 7 kyu |
Compare two strings by comparing the sum of their values (ASCII character code).
* For comparing treat all letters as UpperCase
* `null/NULL/Nil/None` should be treated as empty strings
* If the string contains other characters than letters, treat the whole string as it would be empty
Your method should return `true`, if the strings are equal and `false` if they are not equal.
## Examples:
```
"AD", "BC" -> equal
"AD", "DD" -> not equal
"gf", "FG" -> equal
"zz1", "" -> equal (both are considered empty)
"ZzZz", "ffPFF" -> equal
"kl", "lz" -> not equal
null, "" -> equal
```
| reference | def string_cnt(s):
try:
if s . isalpha():
return sum(ord(a) for a in s . upper())
except AttributeError:
pass
return 0
def compare(s1, s2):
return string_cnt(s1) == string_cnt(s2)
| Compare Strings by Sum of Chars | 576bb3c4b1abc497ec000065 | [
"Mathematics",
"Strings",
"Fundamentals"
] | https://www.codewars.com/kata/576bb3c4b1abc497ec000065 | 7 kyu |
My friend John likes to go to the cinema. He can choose between system A and system B.
```
System A : he buys a ticket (15 dollars) every time
System B : he buys a card (500 dollars) and a first ticket for 0.90 times the ticket price,
then for each additional ticket he pays 0.90 times the price paid for the previous ticket.
```
#### Example:
If John goes to the cinema 3 times:
```
System A : 15 * 3 = 45
System B : 500 + 15 * 0.90 + (15 * 0.90) * 0.90 + (15 * 0.90 * 0.90) * 0.90 ( = 536.5849999999999, no rounding for each ticket)
```
John wants to know how many times he must go to the cinema so that the *final result* of System B, when rounded *up* to the next dollar, will be cheaper than System A.
The function `movie` has 3 parameters: `card` (price of the card), `ticket` (normal price of
a ticket), `perc` (fraction of what he paid for the previous ticket) and returns the first `n` such that
```
ceil(price of System B) < price of System A.
```
#### More examples:
```
movie(500, 15, 0.9) should return 43
(with card the total price is 634, with tickets 645)
movie(100, 10, 0.95) should return 24
(with card the total price is 235, with tickets 240)
``` | reference | import math
def movie(card, ticket, perc):
num = 0
priceA = 0
priceB = card
while math . ceil(priceB) >= priceA:
num += 1
priceA += ticket
priceB += ticket * (perc * * num)
return num
| Going to the cinema | 562f91ff6a8b77dfe900006e | [
"Fundamentals"
] | https://www.codewars.com/kata/562f91ff6a8b77dfe900006e | 7 kyu |
In this kata, your job is to create a class Dictionary which you can add words to and their entries. Example:
```python
>>> d = Dictionary()
>>> d.newentry('Apple', 'A fruit that grows on trees')
>>> print(d.look('Apple'))
A fruit that grows on trees
>>> print(d.look('Banana'))
Can't find entry for Banana
```
```java
>>> Dictionary d = new Dictionary();
>>> d.newEntry("Apple", "A fruit that grows on trees");
>>> System.out.println(d.look("Apple"));
A fruit that grows on trees
>>> System.out.println(d.look("Banana"));
Cant find entry for Banana
```
```javascript
>>> let d = new Dictionary();
>>> d.newEntry("Apple", "A fruit that grows on trees");
>>> console.log(d.look("Apple"));
A fruit that grows on trees
>>> console.log(d.look("Banana"));
Can't find entry for Banana
```
Good luck and happy coding! | reference | class Dictionary (object):
def __init__(self):
self . my_dict = {}
def look(self, key):
return self . my_dict . get(key, "Can't find entry for {}" . format(key))
def newentry(self, key, value):
""" new_entry == PEP8 (forced by Codewars) """
self . my_dict[key] = value
| Interactive Dictionary | 57a93f93bb9944516d0000c1 | [
"Fundamentals"
] | https://www.codewars.com/kata/57a93f93bb9944516d0000c1 | 7 kyu |
ASCII85 is a binary-to-ASCII encoding scheme that's used within PDF and Postscript, and which provides data-size savings over base 64. Your task is to extend the String object with two new methods, ```toAscii85``` (```to_ascii85``` in ruby) and ```fromAscii85``` (```from_ascii85``` in ruby), which handle encoding and decoding ASCII85 strings.
As Python does not allow modifying the built-in string class, for Python the task is to provide functions ```toAscii85(data)``` and ```fromAscii85(ascii85)```, which handle encoding and decoding ASCII85 strings (instead of string object methods).
As Swift strings are very picky about character encodings (and don't take kindly to storing binary data), in Swift, extend Data with a ```toAscii85``` method and String with a ```fromAscii85``` method.
The ```toAscii85``` method should take no arguments and must encode the value of the string to ASCII85, without any line breaks or other whitespace added to the native ASCII85-encoded value.
Example:
* ```'easy'.toAscii85()``` should return ```<~ARTY*~>```
* ```'moderate'.toAscii85()``` should return ```<~D/WrrEaa'$~>```
* ```'somewhat difficult'.toAscii85()``` should return ```<~F)Po,GA(E,+Co1uAnbatCif~>```
The ```fromAscii85``` method should take no arguments and must decode the value of the string (which is presumed to be ASCII85-encoded text).
Example:
* ```'<~ARTY*~>'.fromAscii85()``` should return ```easy```
* ```'<~D/WrrEaa\'$~>'.fromAscii85()``` should return ```moderate```
* ```'<~F)Po,GA(E,+Co1uAnbatCif~>'.fromAscii85()``` should return ```somewhat difficult```
You can [learn all about ASCII85 here](http://en.wikipedia.org/wiki/Ascii85). And remember, this is a binary-to-ASCII encoding scheme, so the input isn't necessarily always a readable string! A brief summary of the salient points, however, is as follows:
1. In general, four binary bytes are encoded into five ASCII85 characters.
2. The character set that ASCII85 encodes into is the 85 characters between ASCII 33 (```!```) and ASCII 117 (```u```), as well as ASCII 122 (```z```), which is used for data compression (see below).
3. In order to encode, four binary bytes are taken together as a single 32-bit number (you can envision concatenating their binary representations, which creates a 32-bit binary number). You then serially perform division by 85, and add 33 to the remainder of each division to get the ASCII character value for the encoded value; the first division and addition of 33 is the rightmost character in the encoded five-character block, etc. (This is all represented well is [the visualization in the Wikipedia page's example](http://en.wikipedia.org/wiki/Ascii85#Example).)
4. If the last block to be encoded contains less than four bytes, it's padded with nulls to a total length of four bytes, and then after encoding, the same number of characters are removed as were added in the padding step.
5. If a block to be encoded contains all nulls, then that block is encoded as a simple ```z``` (ASCII 122) rather than the fully-encoded value of ```!!!!!```.
6. The final encoded value is surrounded by ```<~``` at the start and ```~>``` at the end. In the wild, whitespace can be inserted as needed (e.g., line breaks for mail transport agents); in this kata, **whitespace shouldn't be added to the final encoded value** for the sake of checking the fidelity of the encoding.
7. Decoding applies the above in reverse; each block of five encoded characters is taken as its ASCII character codes, multiplied by powers of 85 according to the position in the block of five characters (again, see the Wikipedia example visualization), and then broken into four separate bytes to determine the corresponding binary values.
8. If a block to be decoded contains less than five characters, it is padded with ```u``` characters (ASCII 117), decoded appropriately, and then the same number of characters are removed from the end of the decoded block as ```u```s were added.
9. All whitespace in encoded values is ignored (as in, it's removed from the encoded data before the data is broken up into the five-character blocks to be decoded).
<br>
To make your testing easier, two functions are preloaded for your use if you wish:
* ```generateRandomReadableStrings(num, len)```: generates an array of *num* strings of length *len* containing only readable ASCII characters
* ```generateRandomBinaryData(num, len)```: generates an array of *num* strings of length *len* containing binary data (ASCII code 0—255)
| algorithms | PLAIN, PLAIN_FILLER = (4, 256, 0), chr(0)
ASC85, ASC85_FILLER = (5, 85, 33), "u"
ZIPPR = "!!!!!", "z"
def toAscii85(data):
return "<~%s~>" % "" . join(map(lambda x: x . replace(* ZIPPR), quantize(PLAIN_FILLER, data, PLAIN, ASC85)))
def fromAscii85(data):
return "" . join(quantize(ASC85_FILLER, '' . join(data[2: - 2]. split()). replace(* ZIPPR[:: - 1]), ASC85, PLAIN))
def quantize(filler, data, source, target):
if data:
size = source[0]
tail = size - len(data) % size
data += tail * filler * (tail is not size)
quants = [parse(block, target) for block in chop(data, source)]
quants[- 1] = quants[- 1][:(None, - tail)[tail is not size]]
return quants
return []
def chop(data, (size, width, offset)):
for point in xrange(0, len(data), size):
yield sum((ord(data[point + i]) - offset) * width * * (size - i - 1) for i in xrange(size))
def parse(block, (size, width, offset)):
return "" . join(chr(block / (width * * k) % width + offset) for k in xrange(size))[:: - 1]
| ASCII85 Encoding & Decoding | 5277dc3ff4bfbd9a36000c1c | [
"Binary",
"Strings",
"Algorithms"
] | https://www.codewars.com/kata/5277dc3ff4bfbd9a36000c1c | 5 kyu |
Given an array of positive or negative integers
<code> I= [i<sub>1</sub>,..,i<sub>n</sub>]</code>
you have to produce a sorted array P of the form
<code>[ [p, sum of all i<sub>j</sub> of I for which p is a prime factor (p positive) of i<sub>j</sub>] ...]</code>
P will be sorted by increasing order of the prime numbers.
The final result has to be given as a string in Java, C#, C, C++ and as an array of arrays in other languages.
#### Example:
```fortran
I = (/12, 15/); // result = "(2 12)(3 27)(5 15)"
```
```python
I = [12, 15] # result = [[2, 12], [3, 27], [5, 15]]
```
```elixir
I = [12, 15] # result = [{2, 12}, {3, 27}, {5, 15}]
```
```rust
I = [12, 15] # result = [(2, 12), (3, 27), (5, 15)]
```
```swift
I = [12, 15] # result = [(2, 12), (3, 27), (5, 15)]
```
```ruby
I = [12, 15] # result = [[2, 12], [3, 27], [5, 15]]
```
```java
I = {12, 15}; // result = "(2 12)(3 27)(5 15)"
```
```cpp
I = {12, 15}; // result = "(2 12)(3 27)(5 15)"
```
```c
I = {12, 15}; // result = "(2 12)(3 27)(5 15)"
```
```csharp
I = {12, 15}; // result = "(2 12)(3 27)(5 15)"
```
```clojure
I = [12, 15] ; result = [[2, 12], [3, 27], [5, 15]]
```
```haskell
I = [12, 15] -- result = [(2,12),(3,27),(5,15)]
```
```javascript
I = [12, 15]; //result = [[2, 12], [3, 27], [5, 15]]
```
```coffeescript
I = [12, 15] # result = [[2, 12], [3, 27], [5, 15]]
```
```typescript
I = [12, 15]; //result = [[2, 12], [3, 27], [5, 15]]
```
```php
I = [12, 15]; //result = [[2, 12], [3, 27], [5, 15]]
```
```cobol
I = [12, 15]
* result = [[2, 12], [3, 27], [5, 15]]
```
[2, 3, 5] is the list of all prime factors of the elements of I, hence the result.
**Notes:**
- It can happen that a sum is 0 if some numbers are negative!
Example: I = [15, 30, -45]
5 divides 15, 30 and (-45) so 5 appears in the result, the sum of the numbers for which 5 is a factor is 0 so we have [5, 0] in the result amongst others.
- In Fortran - as in any other language - the returned string is not permitted to contain any redundant trailing whitespace: you can use dynamically allocated character strings.
| algorithms | from collections import defaultdict
def sum_for_list(lst):
def factors(x):
p_facs = []
i = 2
while x > 1 or x < - 1:
if x % i == 0:
p_facs . append(i)
x / /= i
else:
i += 1
return list(set(p_facs))
fac_dict = defaultdict(int)
for i in lst:
for fac in factors(i):
fac_dict[fac] += i
return sorted([[k, v] for k, v in fac_dict . items()])
| Sum by Factors | 54d496788776e49e6b00052f | [
"Arrays",
"Algorithms",
"Mathematics"
] | https://www.codewars.com/kata/54d496788776e49e6b00052f | 4 kyu |
# Task
Given a rectangular `matrix` and integers `a` and `b`, consider the union of the ath row and the bth (both 0-based) column of the `matrix`. Return sum of all elements of that union.
# Example
For
```
matrix = [[1, 1, 1, 1],
[2, 2, 2, 2],
[3, 3, 3, 3]]
a = 1 and b = 3 ```
the output should be `12`.
Here `(2 + 2 + 2 + 2) + (1 + 3) = 12`.
# Input/Output
- `[input]` 2D integer array `matrix`
2-dimensional array of integers representing a rectangular matrix.
Constraints: `1 ≤ matrix.length ≤ 5, 1 ≤ matrix[0].length ≤ 5, 1 ≤ matrix[i][j] ≤ 100.`
- `[input]` integer `a`
A non-negative integer less than the number of matrix rows.
Constraints: `0 ≤ a < matrix.length.`
- `[input]` integer `b`
A non-negative integer less than the number of matrix columns.
Constraints: `0 ≤ b < matrix[i].length. `
- `[output]` an integer | games | def crossing_sum(matrix, row, col):
return sum(matrix[row]) + sum(line[col] for line in matrix) - matrix[row][col]
| Simple Fun #61: Crossing Sum | 5889ab4928c08c08da00009b | [
"Puzzles"
] | https://www.codewars.com/kata/5889ab4928c08c08da00009b | 7 kyu |
# Area of an annulus
When given the length of the arrow as `a`, where `a` is an `integer` and `≥ 1`,
calculate the area of the annulus (the grey ring).

Round the answer to two decimal places.
In case you need pi, you can use the standard `Math.PI`/`M_PI`. | games | from math import pi
def annulus_area(r):
return round(r * r / 4 * pi, 2)
| Area of an annulus | 5896616336c4bad1c50000d7 | [
"Mathematics",
"Puzzles"
] | https://www.codewars.com/kata/5896616336c4bad1c50000d7 | 7 kyu |
#Sort the columns of a csv-file
You get a string with the content of a csv-file. The columns are separated by semicolons.<br>
The first line contains the names of the columns.<br>
Write a method that sorts the columns by the names of the columns alphabetically and incasesensitive.
An example:
```
Before sorting:
As table (only visualization):
|myjinxin2015|raulbc777|smile67|Dentzil|SteffenVogel_79|
|17945 |10091 |10088 |3907 |10132 |
|2 |12 |13 |48 |11 |
The csv-file:
myjinxin2015;raulbc777;smile67;Dentzil;SteffenVogel_79\n
17945;10091;10088;3907;10132\n
2;12;13;48;11
----------------------------------
After sorting:
As table (only visualization):
|Dentzil|myjinxin2015|raulbc777|smile67|SteffenVogel_79|
|3907 |17945 |10091 |10088 |10132 |
|48 |2 |12 |13 |11 |
The csv-file:
Dentzil;myjinxin2015;raulbc777;smile67;SteffenVogel_79\n
3907;17945;10091;10088;10132\n
48;2;12;13;11
```
There is no need for prechecks. You will always get a correct string with more than 1 line und more than 1 row. All columns will have different names.
Have fun coding it and please don't forget to vote and rank this kata! :-)
I have created other katas. Have a look if you like coding and challenges. | algorithms | def sort_csv_columns(csv_file_content, sep=';', end='\n'):
'''Sort a CSV file by column name.'''
csv_columns = zip(* (row . split(sep)
for row in csv_file_content . split(end)))
sorted_columns = sorted(csv_columns, key=lambda col: col[0]. lower())
return end . join(sep . join(row) for row in zip(* sorted_columns))
| Sort the columns of a csv-file | 57f7f71a7b992e699400013f | [
"Strings",
"Arrays",
"Algorithms",
"Sorting"
] | https://www.codewars.com/kata/57f7f71a7b992e699400013f | 6 kyu |
In her trip to Italy, Elizabeth Gilbert made it her duty to eat perfect pizza. One day she ordered one for dinner, and then some Italian friends appeared at her room. The problem is that there were many people who ask for a piece of pizza at that moment, and she had a knife that only cuts straight.
Given the number of pizza cuts, find the maximum amount of pieces of pizza that you can get (not necessarily of equal size). If the number of cuts is negative, return `-1` instead (or `Nothing` in Haskell). | algorithms | def max_pizza(n):
return n * (n + 1) / / 2 + 1 if n >= 0 else - 1
| Pizza pieces | 5551dc71101b2cf599000023 | [
"Mathematics",
"Algorithms"
] | https://www.codewars.com/kata/5551dc71101b2cf599000023 | 6 kyu |
# # Task:
* #### Complete the pattern, using the special character ```■ □```
* #### In this kata, we draw some histogram of the sound performance of ups and downs.
# # Rules:
- parameter ```waves``` The value of sound waves, an array of number, all number in array >=0.
- return a string, ```■``` represents the sound waves, and ```□``` represents the blank part, draw the histogram from bottom to top.
# # Example:
```
draw([1,2,3,4])
□□□■
□□■■
□■■■
■■■■
draw([1,2,3,3,2,1])
□□■■□□
□■■■■□
■■■■■■
draw([1,2,3,3,2,1,1,2,3,4,5,6,7])
□□□□□□□□□□□□■
□□□□□□□□□□□■■
□□□□□□□□□□■■■
□□□□□□□□□■■■■
□□■■□□□□■■■■■
□■■■■□□■■■■■■
■■■■■■■■■■■■■
draw([5,3,1,2,4,6,5,4,2,3,5,2,1])
□□□□□■□□□□□□□
■□□□□■■□□□■□□
■□□□■■■■□□■□□
■■□□■■■■□■■□□
■■□■■■■■■■■■□
■■■■■■■■■■■■■
draw([1,0,1,0,1,0,1,0])
■□■□■□■□
```
| games | def draw(waves):
m = max(waves)
rotHist = [('■' * v). rjust(m, '□') for v in waves]
return '\n' . join(map('' . join, zip(* rotHist)))
| ■□ Pattern □■ : Wave | 56e67d6166d442121800074c | [
"ASCII Art",
"Puzzles"
] | https://www.codewars.com/kata/56e67d6166d442121800074c | 6 kyu |
# Brainscrambler
[Brainscrambler](https://esolangs.org/wiki/Brainscrambler) is an Esoteric programming language.
A Brainscrambler program consists of a string containing
* command characters
* decimal numbers, which are the input of the program.
Brainscrabler memory is made up of three stacks storing integers :
`A`, `B`, and `C`.
The "current stack" starts out at `A`, then is rotated to `B`, then to `C`, then back to `A` again, etc.
The "current value" is the number at the top of the current stack.
In other words :
* `A` is to the right of `C` and to the left of `B`
* `B` is to the right of `A` and to the left of `C`
* `C` is to the right of `B` and to the left of `A`
Your task is to create an interpreter for Brainscrambler.
You have to write the method ```read(input)``` of the object ```Interpreter```.
This method takes as parameter a ```string``` representing the source code of the Brainscrambler program.
Its output shall be a string containing all the values that have been sent to the output during the execution of the program.
You must execute the commands until you reach the end of the input string.
## Commands
The eleven possible commands in the Brainscrambler language:
- `+` Increment the current number.
- `-` Decrement the current number.
- `<` Move the current number to the stack to the "left".
- `>` Move the current number to the stack to the "right".
- `*` Push a zero onto the current stack.
- `^` Pop the current number and discard it.
- `#` Rotate between stacks.
- `,` Input a number and push it to the current stack. This number will consist of the decimal digits immediatly to the right of the `,` character. After reading the number, the program resumes at the command to the right of the last digit.
- `.` Output the current number.
- `[` Enter the loop.
- `]` Jump to the corresponding `[` bracket if the current number is > `0`.
## Specifications
* All stacks have an original value of 0
* The input numbers are decimal and in the big-endian order.
* When popping from the stack, if the stack is empty, the current number will be undefined. If the current number is undefined :
* If a `.` or `<` or `>` operation appears, do not add anything to the output or shift anything.
* If a `+` or `-` appears, set the current number to `0`.
* If a `]` appears, do not reenter the loop (as ```undefined > 0 === false```).
* Between ```read()``` calls :
* The output is reset;
* The stacks keep their values;
* The current stack stays the same.
* The loops are ```do [] while``` loops, i.e. the loop body is executed BEFORE evaluating the current value
* Loops can be nested
## Examples
#### Simple Program
```javascript
const interpreter = new Interpreter();
interpreter.read('*+.');
'1'
```
```python
interpreter = Interpreter()
interpreter.read('*+.')
'1'
``` | reference | class Interpreter:
def __init__(self):
self . stacks = [[0], [0], [0]]
self . stk = 0
def read(self, code):
res, cur, ln, loop = [], 0, len(code), []
while cur < ln:
if code[cur] in '+-':
if not self . stacks[self . stk]:
self . stacks[self . stk]. append(0)
else:
self . stacks[self . stk][- 1] += [- 1, 1][code[cur] == '+']
elif code[cur] in '<>' and self . stacks[self . stk]:
val = self . stacks[self . stk]. pop()
self . stacks[(self . stk + [- 1, 1][code[cur] == '>']) % 3]. append(val)
elif code[cur] == '*':
self . stacks[self . stk]. append(0)
elif code[cur] == '^':
self . stacks[self . stk]. pop()
elif code[cur] == '#':
self . stk = (self . stk + 1) % 3
elif code[cur] == ',':
fd = next((k for k, x in enumerate(
code[cur + 1:], cur + 1) if not x . isdigit()), ln)
self . stacks[self . stk]. append(int(code[cur + 1: fd]))
cur = fd - 1
elif code[cur] == '.' and self . stacks[self . stk]:
res . append(self . stacks[self . stk][- 1])
elif code[cur] == '[':
loop . append(cur)
elif code[cur] == ']':
if self . stacks[self . stk] and self . stacks[self . stk][- 1] > 0:
cur = loop[- 1]
else:
loop . pop()
cur += 1
return '' . join(map(str, res))
| Brainscrambler - Esoteric programming #3 | 56941f177c0a52aef50000a2 | [
"Interpreters",
"Esoteric Languages"
] | https://www.codewars.com/kata/56941f177c0a52aef50000a2 | 5 kyu |
Write a function ```sort_cards()``` that sorts a shuffled list of cards, so that any given list of cards is sorted by rank, no matter the starting collection.
All cards in the list are represented as strings, so that sorted list of cards looks like this:
```['A', '2', '3', '4', '5', '6', '7', '8', '9', 'T', 'J', 'Q', 'K']```
Example:
```
>>> sort_cards(['3', '9', 'A', '5', 'T', '8', '2', '4', 'Q', '7', 'J', '6', 'K'])
['A', '2', '3', '4', '5', '6', '7', '8', '9', 'T', 'J', 'Q', 'K']
```
Hint: *Tests will have many occurrences of same rank cards, as well as vary in length. You can assume though, that input list is always going to have at least 1 element.* | algorithms | def sort_cards(cards):
return sorted(cards, key="A23456789TJQK" . index)
| Sort deck of cards | 56f399b59821793533000683 | [
"Fundamentals",
"Algorithms",
"Lists",
"Sorting"
] | https://www.codewars.com/kata/56f399b59821793533000683 | 7 kyu |
Write a function `groupIn10s` which takes any number of arguments, groups them into tens, and sorts each group in ascending order.
The return value should be an array of arrays, so that numbers between `0` and`9` inclusive are in position `0`, numbers between `10` and `19` are in position `1`, etc.
Here's an example of the required output:
```javascript
const grouped = groupIn10s(8, 12, 38, 3, 17, 19, 25, 35, 50)
grouped[0] // [3, 8]
grouped[1] // [12, 17, 19]
grouped[2] // [25]
grouped[3] // [35, 38]
grouped[4] // undefined
grouped[5] // [50]
```
```csharp
int[][] grouped = Kata.GroupIn10s(new int[] { 8, 12, 38, 3, 17, 19, 25, 35, 50 });
grouped[0] // [3, 8]
grouped[1] // [12, 17, 19]
grouped[2] // [25]
grouped[3] // [35, 38]
grouped[4] // null
grouped[5] // [50]
```
```python
grouped = group_in_10s(8, 12, 38, 3, 17, 19, 25, 35, 50)
grouped[0] # [3, 8]
grouped[1] # [12, 17, 19]
grouped[2] # [25]
grouped[3] # [35, 38]
grouped[4] # None
grouped[5] # [50]
```
```ruby
grouped = group_in_10s(8, 12, 38, 3, 17, 19, 25, 35, 50)
grouped[0] # [3, 8]
grouped[1] # [12, 17, 19]
grouped[2] # [25]
grouped[3] # [35, 38]
grouped[4] # nil
grouped[5] # [50]
```
``` haskell
groupIn10s [8, 12, 3, 17, 19, 24, 35, 50] `shouldBe` [[3,8],[12,17,19],[24],[35],[],[50]]
```
```lambdacalc
# group-in-10s : List Number -> List (List Number)
group-in-10s [ 8, 12, 3, 17, 19, 24, 35, 50 ] -> [[3,8],[12,17,19],[24],[35],[],[50]]
```
~~~if:lambdacalc
### Encodings
purity: `LetRec`
numEncoding: `Scott`
export `nil, cons, foldl` for your `List` encoding
~~~
| reference | from collections import defaultdict
def group_in_10s(* args):
if not args:
return []
tens = defaultdict(list)
for n in sorted(args):
tens[n / / 10]. append(n)
return [tens . get(d, None) for d in range(max(tens) + 1)]
| Group in 10s | 5694d22eb15d78fe8d00003a | [
"Arrays",
"Lists",
"Fundamentals"
] | https://www.codewars.com/kata/5694d22eb15d78fe8d00003a | 6 kyu |
A poor miner is trapped in a mine and you have to help him to get out !
Only, the mine is all dark so you have to tell him where to go.
In this kata, you will have to implement a method `solve(map, miner, exit)` that has to return the path the miner must take to reach the exit as an array of moves, such as : `['up', 'down', 'right', 'left']`. There are 4 possible moves, `up`, `down`, `left` and `right`, no diagonal.
`map` is a 2-dimensional array of boolean values, representing squares. `false` for walls, `true` for open squares (where the miner can walk). It will never be larger than 5 x 5. It is laid out as an array of columns. All columns will always be the same size, though not necessarily the same size as rows (in other words, maps can be rectangular). The map will never contain any loop, so there will always be only one possible path. The map may contain dead-ends though.
`miner` is the position of the miner at the start, as an object made of two zero-based integer properties, x and y. For example `{x:0, y:0}` would be the top-left corner.
`exit` is the position of the exit, in the same format as `miner`.
Note that the miner can't go outside the map, as it is a tunnel.
Let's take a pretty basic example :
```javascript
var map = [[true, false],
[true, true]];
solve(map, {x:0,y:0}, {x:1,y:1});
// Should return ['right', 'down']
```
```python
map = [[True, False],
[True, True]];
solve(map, {'x':0,'y':0}, {'x':1,'y':1})
# Should return ['right', 'down']
```
```ruby
map = [[true, false],
[true, true]];
solve(map, {'x'=>0,'y'=>0}, {'x'=>1,'y'=>1})
# Should return ['right', 'down']
```
```haskell
let map = [[True, False],
[True, True]]
solve map (0,0) (1,1)
-- Should return [R, D]
``` | games | # name of direction, name of opposite, translation function
dirs = [
('left', 'right', lambda (x, y): (x - 1, y)),
('right', 'left', lambda (x, y): (x + 1, y)),
('up', 'down', lambda (x, y): (x, y - 1)),
('down', 'up', lambda (x, y): (x, y + 1)),
]
def solve(map, miner, exit):
# we turn dictionaries into tuples for nicer lambdas
return do_solve(map, (miner['x'], miner['y']), (exit['x'], exit['y']), None)
def do_solve(map, miner, exit, block):
if not allowed(map, miner):
return None
if miner == exit:
return []
for dir in dirs:
if dir[0] != block:
path = do_solve(map, dir[2](miner), exit, dir[1])
if path != None:
return [dir[0]] + path
def allowed(map, miner):
x, y = miner[0], miner[1]
return x >= 0 and x < len(map) and y >= 0 and y < len(map[0]) and map[x][y]
| Escape the Mines ! | 5326ef17b7320ee2e00001df | [
"Data Structures",
"Algorithms",
"Puzzles",
"Graph Theory"
] | https://www.codewars.com/kata/5326ef17b7320ee2e00001df | 5 kyu |
Linked Lists - Insert Nth
Implement an InsertNth() function (`insert_nth()` in PHP) which can insert a new node at any index within a list.
InsertNth() is a more general version of the Push() function that we implemented in the first kata listed below. Given a list, an index 'n' in the range 0..length, and a data element, add a new node to the list so that it has the given index. InsertNth() should return the head of the list.
```javascript
insertNth(1 -> 2 -> 3 -> null, 0, 7) === 7 -> 1 -> 2 -> 3 -> null)
insertNth(1 -> 2 -> 3 -> null, 1, 7) === 1 -> 7 -> 2 -> 3 -> null)
insertNth(1 -> 2 -> 3 -> null, 3, 7) === 1 -> 2 -> 3 -> 7 -> null)
```
```php
insert_nth(1 -> 2 -> 3 -> NULL, 0, 7); // 7 -> 1 -> 2 -> 3 -> NULL
insert_nth(1 -> 2 -> 3 -> NULL, 1, 7); // 1 -> 7 -> 2 -> 3 -> NULL
insert_nth(1 -> 2 -> 3 -> NULL, 3, 7); // 1 -> 2 -> 3 -> 7 -> NULL
insert_nth(1 -> 2 -> 3 -> NULL, 4, 7); // throws new InvalidArgumentException
```
```csharp
Node.InsertNth(1 -> 2 -> 3 -> null, 0, 7) => 7 -> 1 -> 2 -> 3 -> null
Node.InsertNth(1 -> 2 -> 3 -> null, 1, 7) => 1 -> 7 -> 2 -> 3 -> null
Node.InsertNth(1 -> 2 -> 3 -> null, 3, 7) => 1 -> 2 -> 3 -> 7 -> null
Node.InsertNth(1 -> 2 -> 3 -> null, -2, 7) // throws new ArgumentException
```
You must throw/raise an exception (`ArgumentOutOfRangeException` in C#, `InvalidArgumentException` in PHP) if the index is too large.
The push() and buildOneTwoThree() (`build_one_two_three()` in PHP) functions do not need to be redefined. The `Node` class is also preloaded for you in PHP.
Related Kata in order of expected completion (increasing difficulty):<br>
<a href="http://www.codewars.com/kata/linked-lists-push-and-buildonetwothree">Linked Lists - Push & BuildOneTwoThree</a><br>
<a href="http://www.codewars.com/kata/linked-lists-length-and-count">Linked Lists - Length & Count</a><br>
<a href="http://www.codewars.com/kata/linked-lists-get-nth-node">Linked Lists - Get Nth Node</a><br>
<a href="http://www.codewars.com/kata/linked-lists-insert-nth-node">Linked Lists - Insert Nth Node</a><br>
<a href="http://www.codewars.com/kata/linked-lists-sorted-insert">Linked Lists - Sorted Insert</a><br>
<a href="http://www.codewars.com/kata/linked-lists-insert-sort">Linked Lists - Insert Sort</a><br>
<a href="http://www.codewars.com/kata/linked-lists-append">Linked Lists - Append</a><br>
<a href="http://www.codewars.com/kata/linked-lists-remove-duplicates">Linked Lists - Remove Duplicates</a><br>
<a href="http://www.codewars.com/kata/linked-lists-move-node">Linked Lists - Move Node</a><br>
<a href="http://www.codewars.com/kata/linked-lists-move-node-in-place">Linked Lists - Move Node In-place</a><br>
<a href="http://www.codewars.com/kata/linked-lists-alternating-split">Linked Lists - Alternating Split</a><br>
<a href="http://www.codewars.com/kata/linked-lists-front-back-split">Linked Lists - Front Back Split</a><br>
<a href="http://www.codewars.com/kata/linked-lists-shuffle-merge">Linked Lists - Shuffle Merge</a><br>
<a href="http://www.codewars.com/kata/linked-lists-sorted-merge">Linked Lists - Sorted Merge</a><br>
<a href="http://www.codewars.com/kata/linked-lists-merge-sort">Linked Lists - Merge Sort</a><br>
<a href="http://www.codewars.com/kata/linked-lists-sorted-intersect">Linked Lists - Sorted Intersect</a><br>
<a href="http://www.codewars.com/kata/linked-lists-iterative-reverse">Linked Lists - Iterative Reverse</a><br>
<a href="http://www.codewars.com/kata/linked-lists-recursive-reverse">Linked Lists - Recursive Reverse</a><br>
Inspired by Stanford Professor Nick Parlante's excellent [Linked List teachings.](http://cslibrary.stanford.edu/103/LinkedListBasics.pdf) | reference | class Node (object):
def __init__(self, data, nxt=None):
self . data = data
self . next = nxt
def insert_nth(head, index, data):
if index == 0:
return Node(data, head)
if head and index > 0:
head . next = insert_nth(head . next, index - 1, data)
return head
raise ValueError
| Linked Lists - Insert Nth Node | 55cacc3039607536c6000081 | [
"Linked Lists",
"Data Structures",
"Fundamentals"
] | https://www.codewars.com/kata/55cacc3039607536c6000081 | 6 kyu |
# Linked Lists - Get Nth
Implement a `GetNth()` function that takes a linked list and an integer index and returns the node stored at the `Nth` index position. `GetNth()` uses the C numbering convention that the first node is index 0, the second is index 1, ... and so on.
So for the list `42 -> 13 -> 666`, `GetNth(1)` should return `Node(13)`;
```javascript
getNth(1 -> 2 -> 3 -> null, 0).data === 1
getNth(1 -> 2 -> 3 -> null, 1).data === 2
```
```csharp
Node.GetNth(1 -> 2 -> 3 -> null, 0).Data == 1
Node.GetNth(1 -> 2 -> 3 -> null, 1).Data == 2
```
The index should be in the range `[0..length-1]`. If it is not, or if the list is empty, `GetNth()` should throw/raise an exception (`ArgumentException` in C#, `InvalidArgumentException` in PHP, `Exception` in Java).
**Related Kata in order of expected completion (increasing difficulty):**
* [Linked Lists - Push & BuildOneTwoThree](http://www.codewars.com/kata/linked-lists-push-and-buildonetwothree)
* [Linked Lists - Length & Count](http://www.codewars.com/kata/linked-lists-length-and-count)
* [Linked Lists - Get Nth Node](http://www.codewars.com/kata/linked-lists-get-nth-node)
* [Linked Lists - Insert Nth Node](http://www.codewars.com/kata/linked-lists-insert-nth-node)
* [Linked Lists - Sorted Insert](http://www.codewars.com/kata/linked-lists-sorted-insert)
* [Linked Lists - Insert Sort](http://www.codewars.com/kata/linked-lists-insert-sort)
* [Linked Lists - Append](http://www.codewars.com/kata/linked-lists-append)
* [Linked Lists - Remove Duplicates](http://www.codewars.com/kata/linked-lists-remove-duplicates)
* [Linked Lists - Move Node](http://www.codewars.com/kata/linked-lists-move-node)
* [Linked Lists - Move Node In-place](http://www.codewars.com/kata/linked-lists-move-node-in-place)
* [Linked Lists - Alternating Split](http://www.codewars.com/kata/linked-lists-alternating-split)
* [Linked Lists - Front Back Split](http://www.codewars.com/kata/linked-lists-front-back-split)
* [Linked Lists - Shuffle Merge](http://www.codewars.com/kata/linked-lists-shuffle-merge)
* [Linked Lists - Sorted Merge](http://www.codewars.com/kata/linked-lists-sorted-merge)
* [Linked Lists - Merge Sort](http://www.codewars.com/kata/linked-lists-merge-sort)
* [Linked Lists - Sorted Intersect](http://www.codewars.com/kata/linked-lists-sorted-intersect)
* [Linked Lists - Iterative Reverse](http://www.codewars.com/kata/linked-lists-iterative-reverse)
* [Linked Lists - Recursive Reverse](http://www.codewars.com/kata/linked-lists-recursive-reverse)
Inspired by Stanford Professor Nick Parlante's excellent [Linked List teachings.](http://cslibrary.stanford.edu/103/LinkedListBasics.pdf)
http://cslibrary.stanford.edu/103/LinkedListBasics.pdf
http://cslibrary.stanford.edu/105/LinkedListProblems.pdf | reference | class Node (object):
def __init__(self, data):
self . data = data
self . next = None
def get_nth(node, index):
v = - 1
n = node
while n:
v += 1
if v == index:
return n
n = n . next
raise ValueError
| Linked Lists - Get Nth Node | 55befc42bfe4d13ab1000007 | [
"Linked Lists",
"Data Structures",
"Fundamentals"
] | https://www.codewars.com/kata/55befc42bfe4d13ab1000007 | 7 kyu |
Linked Lists - Length & Count
Implement `length` to count the number of nodes in a linked list.<br>
```javascript
length(null) => 0
length(1 -> 2 -> 3 -> null) => 3
```
```csharp
Node.Length(nullptr) => 0
Node.Length(1 -> 2 -> 3 -> nullptr) => 3
```
```cpp
Length(null) => 0
Length(1 -> 2 -> 3 -> null) => 3
```
```cobol
NodeListLength(null) => 0
NodeListLength(1 -> 2 -> 3 -> null) => 3
```
```lambdacalc
length nil # 0
length (1 -> 2 -> 3 -> nil) # 3
```
~~~if-not:csharp,lambdacalc
Implement Count() to count the occurrences of an integer in a linked list.
~~~
~~~if:csharp
Implement Count() to count the occurrences of a that satisfy a condition provided by a predicate which takes in a node's Data value.
~~~
~~~if:lambdacalc
Implement `count` to count the occurrences of an element in a linked list. A function `eq` is preloaded for you to make comparisons, which will return `True = \ a _ . a` or `False = \ _ b . b`.
~~~
```javascript
count(null, 1) => 0
count(1 -> 2 -> 3 -> null, 1) => 1
count(1 -> 1 -> 1 -> 2 -> 2 -> 2 -> 2 -> 3 -> 3 -> null, 2) => 4
```
```csharp
Node.Count(null, value => value == 1) => 0
Node.Count(1 -> 3 -> 5 -> 6, value => value % 2 != 0) => 3
```
```cpp
Count(null, 1) => 0
Count(1 -> 2 -> 3 -> nullptr, 1) => 1
Count(1 -> 1 -> 1 -> 2 -> 2 -> 2 -> 2 -> 3 -> 3 -> nullptr, 2) => 4
```
```cobol
NodeListCount(null, 1) => 0
NodeListCount(1 -> 2 -> 2 -> 2 -> 2 -> 3 -> 3 -> null, 2) => 4
```
```lambdacalc
count nil 1 # 0
count (1 -> 2 -> 3 -> nil) 1 # 1
count (1 -> 1 -> 2 -> 1 -> 2) 2 # 2
```
I've decided to bundle these two functions within the same Kata since they are both very similar.
~~~if:lambdacalc
### Lambda Calculus Notes
In the LC translations of this series, we use the Scott encoding of a linked list. In this encoding, a list is a function which accepts two arguments. If the list is `nil` then it returns the first argument (the default case), otherwise it will pass its data and tail to the second argument. In practice this looks like this:
```lambdacalc
my-list (default-case) (\ data next . result)
```
For this kata, `nil` is defined for you in `Preloaded` like this:
```lambdacalc
nil = \ n _ . n
```
In addition, we also use the [Scott encoding](https://github.com/codewars/lambda-calculus/wiki/encodings-guide#scott-numerals) of numbers.
~~~
The `push()`/`Push()` and `buildOneTwoThree()`/`BuildOneTwoThree()` functions do not need to be redefined.
Related Kata in order of expected completion (increasing difficulty):<br>
<a href="http://www.codewars.com/kata/linked-lists-push-and-buildonetwothree">Linked Lists - Push & BuildOneTwoThree</a><br>
<a href="http://www.codewars.com/kata/linked-lists-length-and-count">Linked Lists - Length & Count</a><br>
<a href="http://www.codewars.com/kata/linked-lists-get-nth-node">Linked Lists - Get Nth Node</a><br>
<a href="http://www.codewars.com/kata/linked-lists-insert-nth-node">Linked Lists - Insert Nth Node</a><br>
<a href="http://www.codewars.com/kata/linked-lists-sorted-insert">Linked Lists - Sorted Insert</a><br>
<a href="http://www.codewars.com/kata/linked-lists-insert-sort">Linked Lists - Insert Sort</a><br>
<a href="http://www.codewars.com/kata/linked-lists-append">Linked Lists - Append</a><br>
<a href="http://www.codewars.com/kata/linked-lists-remove-duplicates">Linked Lists - Remove Duplicates</a><br>
<a href="http://www.codewars.com/kata/linked-lists-move-node">Linked Lists - Move Node</a><br>
<a href="http://www.codewars.com/kata/linked-lists-move-node-in-place">Linked Lists - Move Node In-place</a><br>
<a href="http://www.codewars.com/kata/linked-lists-alternating-split">Linked Lists - Alternating Split</a><br>
<a href="http://www.codewars.com/kata/linked-lists-front-back-split">Linked Lists - Front Back Split</a><br>
<a href="http://www.codewars.com/kata/linked-lists-shuffle-merge">Linked Lists - Shuffle Merge</a><br>
<a href="http://www.codewars.com/kata/linked-lists-sorted-merge">Linked Lists - Sorted Merge</a><br>
<a href="http://www.codewars.com/kata/linked-lists-merge-sort">Linked Lists - Merge Sort</a><br>
<a href="http://www.codewars.com/kata/linked-lists-sorted-intersect">Linked Lists - Sorted Intersect</a><br>
<a href="http://www.codewars.com/kata/linked-lists-iterative-reverse">Linked Lists - Iterative Reverse</a><br>
<a href="http://www.codewars.com/kata/linked-lists-recursive-reverse">Linked Lists - Recursive Reverse</a><br>
Inspired by Stanford Professor Nick Parlante's excellent [Linked List teachings.](http://cslibrary.stanford.edu/103/LinkedListBasics.pdf) | reference | class Node (object):
def __init__(self, data):
self . data = data
self . next = None
def length(node):
leng = 0
while node:
leng += 1
node = node . next
return leng
def count(node, data):
c = 0
while node:
if node . data == data:
c += 1
node = node . next
return c
| Linked Lists - Length & Count | 55beec7dd347078289000021 | [
"Linked Lists",
"Data Structures",
"Fundamentals"
] | https://www.codewars.com/kata/55beec7dd347078289000021 | 6 kyu |
Linked Lists - Push & BuildOneTwoThree
Write push() and buildOneTwoThree() functions to easily update and initialize linked lists. Try to use the push() function within your buildOneTwoThree() function.
Here's an example of push() usage:
```javascript
var chained = null
chained = push(chained, 3)
chained = push(chained, 2)
chained = push(chained, 1)
push(chained, 8) === 8 -> 1 -> 2 -> 3 -> null
```
```csharp
Node chained = null;
chained = Node.Push(chained, 3);
chained = Node.Push(chained, 2);
chained = Node.Push(chained, 1);
Node.Push(chained, 8) => 8 -> 1 -> 2 -> 3 -> null
```
```lambdacalc
chained1 = nil
chained2 = push chained1 3
chained3 = push chained2 2
chained4 = push chained3 1
push chained4 8 # 8 -> 1 -> 2 -> 3 -> nil
```
The push function accepts head and data parameters, where head is either a node object or null/None/nil. Your push implementation should be able to create a new linked list/node when head is null/None/nil.
The buildOneTwoThree function should create and return a linked list with three nodes: ```1 -> 2 -> 3 -> null```
~~~if:lambdacalc
### Lambda Calculus Notes
In the LC translations of this series, we use the Scott encoding of a linked list. In this encoding, a list is a function which accepts two arguments. If the list is `nil` then it returns the first argument (the default case), otherwise it will pass its data and tail to the second argument. In practice this looks like this:
```lambdacalc
my-list (default-case) (\ data next . result)
```
For this kata, `nil` is defined for you in `Preloaded` like this:
```lambdacalc
nil = \ n _ . n
```
*Note: Scott lists in LC typically use the `cons` constructor instead of `push`, which accepts arguments in reverse order.*
~~~
Related Kata in order of expected completion (increasing difficulty):<br>
<a href="http://www.codewars.com/kata/linked-lists-push-and-buildonetwothree">Linked Lists - Push & BuildOneTwoThree</a><br>
<a href="http://www.codewars.com/kata/linked-lists-length-and-count">Linked Lists - Length & Count</a><br>
<a href="http://www.codewars.com/kata/linked-lists-get-nth-node">Linked Lists - Get Nth Node</a><br>
<a href="http://www.codewars.com/kata/linked-lists-insert-nth-node">Linked Lists - Insert Nth Node</a><br>
<a href="http://www.codewars.com/kata/linked-lists-sorted-insert">Linked Lists - Sorted Insert</a><br>
<a href="http://www.codewars.com/kata/linked-lists-insert-sort">Linked Lists - Insert Sort</a><br>
<a href="http://www.codewars.com/kata/linked-lists-append">Linked Lists - Append</a><br>
<a href="http://www.codewars.com/kata/linked-lists-remove-duplicates">Linked Lists - Remove Duplicates</a><br>
<a href="http://www.codewars.com/kata/linked-lists-move-node">Linked Lists - Move Node</a><br>
<a href="http://www.codewars.com/kata/linked-lists-move-node-in-place">Linked Lists - Move Node In-place</a><br>
<a href="http://www.codewars.com/kata/linked-lists-alternating-split">Linked Lists - Alternating Split</a><br>
<a href="http://www.codewars.com/kata/linked-lists-front-back-split">Linked Lists - Front Back Split</a><br>
<a href="http://www.codewars.com/kata/linked-lists-shuffle-merge">Linked Lists - Shuffle Merge</a><br>
<a href="http://www.codewars.com/kata/linked-lists-sorted-merge">Linked Lists - Sorted Merge</a><br>
<a href="http://www.codewars.com/kata/linked-lists-merge-sort">Linked Lists - Merge Sort</a><br>
<a href="http://www.codewars.com/kata/linked-lists-sorted-intersect">Linked Lists - Sorted Intersect</a><br>
<a href="http://www.codewars.com/kata/linked-lists-iterative-reverse">Linked Lists - Iterative Reverse</a><br>
<a href="http://www.codewars.com/kata/linked-lists-recursive-reverse">Linked Lists - Recursive Reverse</a><br>
Inspired by Stanford Professor Nick Parlante's excellent [Linked List teachings.](http://cslibrary.stanford.edu/103/LinkedListBasics.pdf)
I'm aware that there are better ways to create linked lists (class methods, reference pointers, etc.), but not all languages have the same features. I'd like to keep the basic API consistent between language translations for this kata. | reference | class Node (object):
def __init__(self, data, next=None):
self . data = data
self . next = next
def push(head, data):
return Node(data, head)
def build_one_two_three():
return Node(1, Node(2, Node(3)))
| Linked Lists - Push & BuildOneTwoThree | 55be95786abade3c71000079 | [
"Linked Lists",
"Fundamentals"
] | https://www.codewars.com/kata/55be95786abade3c71000079 | 7 kyu |
You will be given an array of objects representing data about developers who have signed up to attend the next coding meetup that you are organising.
Given the following input array:
```javascript
var list1 = [
{ firstName: 'Nikau', lastName: 'R.', country: 'New Zealand', continent: 'Oceania', age: 39, language: 'Ruby' },
{ firstName: 'Precious', lastName: 'G.', country: 'South Africa', continent: 'Africa', age: 22, language: 'JavaScript' },
{ firstName: 'Maria', lastName: 'S.', country: 'Peru', continent: 'Americas', age: 30, language: 'C' },
{ firstName: 'Agustin', lastName: 'V.', country: 'Uruguay', continent: 'Americas', age: 19, language: 'JavaScript' }
];
```
```python
list1 = [
{ "first_name": "Nikau", "last_name": "R.", "contry": "New Zealand", "continent": "Oceania", "age": 39, "language": "Ruby" },
{ "first_name": "Precious", "last_name": "G.", "contry": "South Africa", "continent": "Africa", "age": 22, "language": "JavaScript" },
{ "first_name": "Maria", "last_name": "S.", "contry": "Peru", "continent": "Americas", "age": 30, "language": "C" },
{ "first_name": "Agustin", "last_name": "V.", "contry": "Uruguay", "continent": "Americas", "age": 19, "language": "JavaScript" }
]
```
```ruby
list1 = [
{ "first_name"=>"Nikau", "last_name"=>"R.", "contry"=>"New Zealand", "continent"=>"Oceania", "age"=>39, "language"=>"Ruby" },
{ "first_name"=>"Precious", "last_name"=>"G.", "contry"=>"South Africa", "continent"=>"Africa", "age"=>22, "language"=>"JavaScript" },
{ "first_name"=>"Maria", "last_name"=>"S.", "contry"=>"Peru", "continent"=>"Americas", "age"=>30, "language"=>"C" },
{ "first_name"=>"Agustin", "last_name"=>"V.", "contry"=>"Uruguay", "continent"=>"Americas", "age"=>19, "language"=>"JavaScript" }
]
```
```crystal
list1 = [
{ "first_name"=>"Nikau", "last_name"=>"R.", "contry"=>"New Zealand", "continent"=>"Oceania", "age"=>39, "language"=>"Ruby" },
{ "first_name"=>"Precious", "last_name"=>"G.", "contry"=>"South Africa", "continent"=>"Africa", "age"=>22, "language"=>"JavaScript" },
{ "first_name"=>"Maria", "last_name"=>"S.", "contry"=>"Peru", "continent"=>"Americas", "age"=>30, "language"=>"C" },
{ "first_name"=>"Agustin", "last_name"=>"V.", "contry"=>"Uruguay", "continent"=>"Americas", "age"=>19, "language"=>"JavaScript" }
]
```
Write a function that returns the array sorted alphabetically by the programming language. In case there are some developers that code in the same language, sort them alphabetically by the first name:
```javascript
[
{ firstName: 'Maria', lastName: 'S.', country: 'Peru', continent: 'Americas', age: 30, language: 'C' },
{ firstName: 'Agustin', lastName: 'V.', country: 'Uruguay', continent: 'Americas', age: 19, language: 'JavaScript' },
{ firstName: 'Precious', lastName: 'G.', country: 'South Africa', continent: 'Africa', age: 22, language: 'JavaScript' },
{ firstName: 'Nikau', lastName: 'R.', country: 'New Zealand', continent: 'Oceania', age: 39, language: 'Ruby' }
];
```
```python
[
{ "first_name": "Maria", "last_name": "S.", "contry": "Peru", "continent": "Americas", "age": 30, "language": "C" },
{ "first_name": "Agustin", "last_name": "V.", "contry": "Uruguay", "continent": "Americas", "age": 19, "language": "JavaScript" },
{ "first_name": "Precious", "last_name": "G.", "contry": "South Africa", "continent": "Africa", "age": 22, "language": "JavaScript" },
{ "first_name": "Nikau", "last_name": "R.", "contry": "New Zealand", "continent": "Oceania", "age": 39, "language": "Ruby" }
]
```
```ruby
[
{ "first_name"=>"Maria", "last_name"=>"S.", "contry"=>"Peru", "continent"=>"Americas", "age"=>30, "language"=>"C" },
{ "first_name"=>"Agustin", "last_name"=>"V.", "contry"=>"Uruguay", "continent"=>"Americas", "age"=>19, "language"=>"JavaScript" },
{ "first_name"=>"Precious", "last_name"=>"G.", "contry"=>"South Africa", "continent"=>"Africa", "age"=>22, "language"=>"JavaScript" },
{ "first_name"=>"Nikau", "last_name"=>"R.", "contry"=>"New Zealand", "continent"=>"Oceania", "age"=>39, "language"=>"Ruby" }
]
```
```crystal
[
{ "first_name"=>"Maria", "last_name"=>"S.", "contry"=>"Peru", "continent"=>"Americas", "age"=>30, "language"=>"C" },
{ "first_name"=>"Agustin", "last_name"=>"V.", "contry"=>"Uruguay", "continent"=>"Americas", "age"=>19, "language"=>"JavaScript" },
{ "first_name"=>"Precious", "last_name"=>"G.", "contry"=>"South Africa", "continent"=>"Africa", "age"=>22, "language"=>"JavaScript" },
{ "first_name"=>"Nikau", "last_name"=>"R.", "contry"=>"New Zealand", "continent"=>"Oceania", "age"=>39, "language"=>"Ruby" }
]
```
Notes:
- The input array will always be valid and formatted as in the example above.
- The array does not include developers coding in the same language and sharing the same name.
<br>
<br>
<br>
<br>
<br>
This kata is part of the **Coding Meetup** series which includes a number of short and easy to follow katas which have been designed to allow mastering the use of higher-order functions. In JavaScript this includes methods like: `forEach, filter, map, reduce, some, every, find, findIndex`. Other approaches to solving the katas are of course possible.
Here is the full list of the katas in the **Coding Meetup** series:
<a href="http://www.codewars.com/kata/coding-meetup-number-1-higher-order-functions-series-count-the-number-of-javascript-developers-coming-from-europe">Coding Meetup #1 - Higher-Order Functions Series - Count the number of JavaScript developers coming from Europe</a>
<a href="https://www.codewars.com/kata/coding-meetup-number-2-higher-order-functions-series-greet-developers">Coding Meetup #2 - Higher-Order Functions Series - Greet developers</a>
<a href="https://www.codewars.com/kata/coding-meetup-number-3-higher-order-functions-series-is-ruby-coming">Coding Meetup #3 - Higher-Order Functions Series - Is Ruby coming?</a>
<a href="https://www.codewars.com/kata/coding-meetup-number-4-higher-order-functions-series-find-the-first-python-developer">Coding Meetup #4 - Higher-Order Functions Series - Find the first Python developer</a>
<a href="https://www.codewars.com/kata/coding-meetup-number-5-higher-order-functions-series-prepare-the-count-of-languages">Coding Meetup #5 - Higher-Order Functions Series - Prepare the count of languages</a>
<a href="https://www.codewars.com/kata/coding-meetup-number-6-higher-order-functions-series-can-they-code-in-the-same-language">Coding Meetup #6 - Higher-Order Functions Series - Can they code in the same language?</a>
<a href="http://www.codewars.com/kata/coding-meetup-number-7-higher-order-functions-series-find-the-most-senior-developer">Coding Meetup #7 - Higher-Order Functions Series - Find the most senior developer</a>
<a href="https://www.codewars.com/kata/coding-meetup-number-8-higher-order-functions-series-will-all-continents-be-represented">Coding Meetup #8 - Higher-Order Functions Series - Will all continents be represented?</a>
<a href="https://www.codewars.com/kata/coding-meetup-number-9-higher-order-functions-series-is-the-meetup-age-diverse">Coding Meetup #9 - Higher-Order Functions Series - Is the meetup age-diverse?</a>
<a href="https://www.codewars.com/kata/coding-meetup-number-10-higher-order-functions-series-create-usernames">Coding Meetup #10 - Higher-Order Functions Series - Create usernames</a>
<a href="https://www.codewars.com/kata/coding-meetup-number-11-higher-order-functions-series-find-the-average-age">Coding Meetup #11 - Higher-Order Functions Series - Find the average age</a>
<a href="https://www.codewars.com/kata/coding-meetup-number-12-higher-order-functions-series-find-github-admins">Coding Meetup #12 - Higher-Order Functions Series - Find GitHub admins</a>
<a href="https://www.codewars.com/kata/coding-meetup-number-13-higher-order-functions-series-is-the-meetup-language-diverse">Coding Meetup #13 - Higher-Order Functions Series - Is the meetup language-diverse?</a>
<a href="https://www.codewars.com/kata/coding-meetup-number-14-higher-order-functions-series-order-the-food">Coding Meetup #14 - Higher-Order Functions Series - Order the food</a>
<a href="https://www.codewars.com/kata/coding-meetup-number-15-higher-order-functions-series-find-the-odd-names">Coding Meetup #15 - Higher-Order Functions Series - Find the odd names</a>
<a href="https://www.codewars.com/kata/coding-meetup-number-16-higher-order-functions-series-ask-for-missing-details">Coding Meetup #16 - Higher-Order Functions Series - Ask for missing details</a>
<a href="https://www.codewars.com/kata/coding-meetup-number-17-higher-order-functions-series-sort-by-programming-language">Coding Meetup #17 - Higher-Order Functions Series - Sort by programming language</a> | reference | def sort_by_language(arr):
return sorted(arr, key=lambda x: (x["language"], x["first_name"]))
| Coding Meetup #17 - Higher-Order Functions Series - Sort by programming language | 583ea278c68d96a5fd000abd | [
"Functional Programming",
"Data Structures",
"Arrays",
"Fundamentals",
"Algorithms",
"Sorting"
] | https://www.codewars.com/kata/583ea278c68d96a5fd000abd | 7 kyu |
Oh No!
The song sheets have been dropped in the snow and the lines of each verse have become all jumbled up.
<h1>Task</h1>
```if-not:python
You have to write a comparator function which can sort the lines back into their correct order, otherwise Christmas will be cancelled!
```
```if:python
You have to write a sorting function which can organize the lines back into their correct order, otherwise Christmas will be cancelled!
```
Reminder: Below is what the final verse *should* look like
```
On the 12th day of Christmas my true love gave to me
12 drummers drumming,
11 pipers piping,
10 lords a leaping,
9 ladies dancing,
8 maids a milking,
7 swans a swimming,
6 geese a laying,
5 golden rings,
4 calling birds,
3 French hens,
2 turtle doves and
a partridge in a pear tree.
```
<h1>Background</h1>
A `Java` [comparator function](https://docs.oracle.com/javase/8/docs/api/java/util/Comparator.html) is described as below. Other languages are similar:
> `int compare(T o1, T o2)`
Compares its two arguments for order. Returns a negative integer, zero, or a positive integer as the first argument is less than, equal to, or greater than the second. | reference | def song_sorter(lines):
return sorted(lines, key=lambda x: ['On', '12', '11', '10', '9', '8', '7', '6', '5', '4', '3', '2', 'and', 'a']. index(x . split()[0]))
| The 12 Days of Christmas | 57dd3a06eb0537b899000a64 | [
"Strings",
"Sorting",
"Fundamentals"
] | https://www.codewars.com/kata/57dd3a06eb0537b899000a64 | 7 kyu |
## Task
Some people are standing in a row in a park. There are trees between them which cannot be moved.
Your task is to rearrange the people by their heights in a non-descending order without moving the trees.
## Example
For `a = [-1, 150, 190, 170, -1, -1, 160, 180]`, the output should be
`[-1, 150, 160, 170, -1, -1, 180, 190]`.
## Input/Output
- `[input]` integer array `a`
If a[i] = -1, then the ith position is occupied by a tree. Otherwise a[i] is the height of a person standing in the ith position.
Constraints:
`5 ≤ a.length ≤ 30,`
`-1 ≤ a[i] ≤ 200.`
- `[output]` an integer array
`Sorted` array a with all the trees untouched. | games | def sort_by_height(a):
s = iter(sorted(x for x in a if x != - 1))
return [x if x == - 1 else next(s) for x in a]
| Simple Fun #88: Sort By Height | 589577f0d1b93ae32a000001 | [
"Puzzles",
"Sorting",
"Algorithms"
] | https://www.codewars.com/kata/589577f0d1b93ae32a000001 | 7 kyu |
You're continuing to enjoy your new piano, as described in <a href="https://www.codewars.com/kata/piano-kata-part-1">Piano Kata, Part 1</a>. You're also continuing the exercise where you start on the very first (leftmost, lowest in pitch) key on the 88-key keyboard, which (as shown below) is the note A, with the little finger on your left hand, then the second key, which is the black key A# ("A sharp"), with your left ring finger, then the third key, B, with your left middle finger, then the fourth key, C, with your left index finger, and then the fifth key, C#, with your left thumb. Then you play the sixth key, D, with your right thumb, and continue on playing the seventh, eighth, ninth, and tenth keys with the other four fingers of your right hand. Then for the eleventh key you go back to your left little finger, and so on. Once you get to the rightmost/highest, 88th, key, C, you start all over again with your left little finger on the first key.
<a href="http://tachyonlabs.com/miscimages/piano-keyboard-with-notes-clipart.jpg" target="_blank"><img src="http://tachyonlabs.com/miscimages/piano-keyboard-with-notes-clipart.jpg"></a>
(If the Codewars Instructions pane resizes the above piano keyboard image to be too small to read the note labels of the black/sharp keys on your screen, <a href="http://tachyonlabs.com/miscimages/piano-keyboard-with-notes-clipart.jpg" target="_blank">click here to open a copy of the image in a new tab or window</a>.)
This time, in addition to counting each key press out loud (not starting again at 1 after 88, but continuing on to 89 and so forth) to try to keep a steady rhythm going and to see how far you can get before messing up, you're also saying the name of each note. You wonder whether this may help you develop perfect pitch in addition to learning to just *know* which note is which, and -- as in <a href="https://www.codewars.com/kata/piano-kata-part-1">Piano Kata, Part 1</a> -- helping you to learn to move smoothly and with uniform pressure on the keys from each finger to the next and back and forth between hands.
The function you are going to write will explore one of the patterns you're experiencing in your practice: Given the number you stopped on, which note was it? For example, in the description of your piano exercise above, if you stopped at 5, your left thumb would be on the fifth key of the piano, which is C#. Or if you stopped at 92, you would have gone all the way from keys 1 to 88 and then wrapped around, so that you would be on the fourth key, which is C.
Your function will receive an integer between 1 and 10000 (maybe you think that in principle it would be cool to count up to, say, a billion, but considering how many years it would take it is just not possible) and return one of the strings "A", "A#", "B", "C", "C#", "D", "D#", "E", "F", "F#", "G", or "G#" indicating which note you stopped on -- here are a few more examples:
```
1 "A"
12 "G#"
42 "D"
100 "G#"
2017 "F"
```
Have fun!
| reference | def which_note(count):
return "A A# B C C# D D# E F F# G G#" . split()[(count - 1) % 88 % 12]
| Piano Kata, Part 2 | 589631d24a7323d18d00016f | [
"Fundamentals"
] | https://www.codewars.com/kata/589631d24a7323d18d00016f | 6 kyu |
## Your Story
"A *piano* in the home meant something." - *Fried Green Tomatoes at the Whistle Stop Cafe*
You've just realized a childhood dream by getting a beautiful and beautiful-sounding upright piano from a friend who was leaving the country. You immediately started doing things like playing "<a href="https://en.wikipedia.org/wiki/Heart_and_Soul_(1938_song)#Musical_format">Heart and Soul</a>" over and over again, using one finger to pick out any melody that came into your head, requesting some sheet music books from the library, signing up for some MOOCs like <a href="https://www.coursera.org/learn/develop-your-musicianship">Developing Your Musicianship</a>, and wondering if you will think of any good ideas for writing piano-related katas and apps.
Now you're doing an exercise where you play the very first (leftmost, lowest in pitch) key on the 88-key keyboard, which (as shown below) is white, with the little finger on your left hand, then the second key, which is black, with the ring finger on your left hand, then the third key, which is white, with the middle finger on your left hand, then the fourth key, also white, with your left index finger, and then the fifth key, which is black, with your left thumb. Then you play the sixth key, which is white, with your right thumb, and continue on playing the seventh, eighth, ninth, and tenth keys with the other four fingers of your right hand. Then for the eleventh key you go back to your left little finger, and so on. Once you get to the rightmost/highest, 88th, key, you start all over again with your left little finger on the first key. Your thought is that this will help you to learn to move smoothly and with uniform pressure on the keys from each finger to the next and back and forth between hands.
<img src="http://tachyonlabs.com/miscimages/piano-keyboard-clipart.jpg">
You're not saying the names of the notes while you're doing this, but instead just counting each key press out loud (not starting again at 1 after 88, but continuing on to 89 and so forth) to try to keep a steady rhythm going and to see how far you can get before messing up. You move gracefully and with flourishes, and between screwups you hear, see, and feel that you are part of some great repeating progression between low and high notes and black and white keys.
## Your Function
The function you are going to write is not actually going to help you with your piano playing, but just explore one of the patterns you're experiencing: Given the number you stopped on, was it on a black key or a white key? For example, in the description of your piano exercise above, if you stopped at 5, your left thumb would be on the fifth key of the piano, which is black. Or if you stopped at 92, you would have gone all the way from keys 1 to 88 and then wrapped around, so that you would be on the fourth key, which is white.
Your function will receive an integer between 1 and 10000 (maybe you think that in principle it would be cool to count up to, say, a billion, but considering how many years it would take it is just not possible) and return the string "black" or "white" -- here are a few more examples:
```
1 "white"
12 "black"
42 "white"
100 "black"
2017 "white"
```
Have fun! And if you enjoy this kata, check out the sequel: <a href="https://www.codewars.com/kata/piano-kata-part-2">Piano Kata, Part 2</a>
| reference | def black_or_white_key(key_press_count):
return "black" if (key_press_count - 1) % 88 % 12 in [1, 4, 6, 9, 11] else "white"
| Piano Kata, Part 1 | 589273272fab865136000108 | [
"Fundamentals"
] | https://www.codewars.com/kata/589273272fab865136000108 | 6 kyu |
# Esolang Interpreters #3 - Custom Paintfuck Interpreter
## About this Kata Series
"Esolang Interpreters" is a Kata Series that originally began as three separate, independent esolang interpreter Kata authored by [@donaldsebleung](http://codewars.com/users/donaldsebleung) which all shared a similar format and were all somewhat inter-related. Under the influence of [a fellow Codewarrior](https://www.codewars.com/users/nickkwest), these three high-level inter-related Kata gradually evolved into what is known today as the "Esolang Interpreters" series.
This series is a high-level Kata Series designed to challenge the minds of bright and daring programmers by implementing interpreters for various [esoteric programming languages/Esolangs](http://esolangs.org), mainly [Brainfuck](http://esolangs.org/wiki/Brainfuck) derivatives but not limited to them, given a certain specification for a certain Esolang. Perhaps the only exception to this rule is the very first Kata in this Series which is intended as an introduction/taster to the world of esoteric programming languages and writing interpreters for them.
## The Language
Paintfuck is a [borderline-esoteric programming language/Esolang](http://esolangs.org) which is a derivative of [Smallfuck](http://esolangs.org/wiki/Smallfuck) (itself a derivative of the famous [Brainfuck](http://esolangs.org/wiki/Brainfuck)) that uses a two-dimensional data grid instead of a one-dimensional tape.
Valid commands in Paintfuck include:
- `n` - Move data pointer north (up)
- `e` - Move data pointer east (right)
- `s` - Move data pointer south (down)
- `w` - Move data pointer west (left)
- `*` - Flip the bit at the current cell (same as in Smallfuck)
- `[` - Jump past matching `]` if bit under current pointer is `0` (same as in Smallfuck)
- `]` - Jump back to the matching `[` (if bit under current pointer is nonzero) (same as in Smallfuck)
The specification states that any non-command character (i.e. any character other than those mentioned above) should simply be ignored. The output of the interpreter is the two-dimensional data grid itself, best as animation as the interpreter is running, but at least a representation of the data grid itself after a certain number of iterations (explained later in task).
In current implementations, the 2D datagrid is finite in size with toroidal (wrapping) behaviour. This is one of the few major differences of Paintfuck from Smallfuck as Smallfuck terminates (normally) whenever the pointer exceeds the bounds of the tape.
Similar to Smallfuck, Paintfuck is Turing-complete **if and only if** the 2D data grid/canvas were unlimited in size. However, since the size of the data grid is defined to be finite, it acts like a finite state machine.
More info on this Esolang can be found [here](http://esolangs.org/wiki/Paintfuck).
## The Task
Your task is to implement a custom Paintfuck interpreter `interpreter()`/`Interpret` which accepts the following arguments in the specified order:
1. `code` - **Required**. The Paintfuck code to be executed, passed in as a string. May contain comments (non-command characters), in which case your interpreter should simply ignore them. If empty, simply return the initial state of the data grid.
2. `iterations` - **Required**. A non-negative integer specifying the number of iterations to be performed before the final state of the data grid is returned. See notes for definition of 1 iteration. If equal to zero, simply return the initial state of the data grid.
3. `width` - **Required**. The width of the data grid in terms of the number of data cells in each row, passed in as a positive integer.
4. `height` - **Required**. The height of the data grid in cells (i.e. number of rows) passed in as a positive integer.
A few things to note:
- Your interpreter should treat all command characters as **case-sensitive** so `N`, `E`, `S` and `W` are **not** valid command characters
- Your interpreter should initialize all cells within the data grid to a value of `0` regardless of the width and height of the grid
- In this implementation, your pointer must always start at the **top-left hand corner** of the data grid (i.e. first row, first column). This is important as some implementations have the data pointer starting at the middle of the grid.
- One iteration is defined as one step in the program, i.e. the number of command characters evaluated. For example, given a program `nessewnnnewwwsswse` and an iteration count of `5`, your interpreter should evaluate `nesse` before returning the final state of the data grid. **Non-command characters should not count towards the number of iterations.**
- Regarding iterations, the act of skipping to the matching `]` when a `[` is encountered (or vice versa) is considered to be **one** iteration regardless of the number of command characters in between. The next iteration then commences at the command **right after** the matching `]` (or `[`).
- Your interpreter should terminate normally and return the final state of the 2D data grid whenever **any** of the mentioned conditions become true: (1) All commands have been considered left to right, or (2) Your interpreter has already performed the number of iterations specified in the second argument.
- The return value of your interpreter should be a representation of the final state of the 2D data grid where each row is separated from the next by a CRLF (`\r\n`). For example, if the final state of your datagrid is
```
[
[1, 0, 0],
[0, 1, 0],
[0, 0, 1]
]
```
... then your return string should be `"100\r\n010\r\n001"`.
Good luck :D
## Kata in this Series
1. [Esolang Interpreters #1 - Introduction to Esolangs and My First Interpreter (MiniStringFuck)](https://www.codewars.com/kata/esolang-interpreters-number-1-introduction-to-esolangs-and-my-first-interpreter-ministringfuck)
2. [Esolang Interpreters #2 - Custom Smallfuck Interpreter](http://codewars.com/kata/esolang-interpreters-number-2-custom-smallfuck-interpreter)
3. **Esolang Interpreters #3 - Custom Paintfuck Interpreter**
4. [Esolang Interpreters #4 - Boolfuck Interpreter](http://codewars.com/kata/esolang-interpreters-number-4-boolfuck-interpreter) | algorithms | def interpreter(code, iterations, width, height):
code = "" . join(c for c in code if c in "[news]*")
canvas = [[0] * width for _ in range(height)]
row = col = step = count = loop = 0
while step < len(code) and count < iterations:
command = code[step]
if loop:
if command == "[":
loop += 1
elif command == "]":
loop -= 1
elif command == "n":
row = (row - 1) % height
elif command == "s":
row = (row + 1) % height
elif command == "w":
col = (col - 1) % width
elif command == "e":
col = (col + 1) % width
elif command == "*":
canvas[row][col] ^= 1
elif command == "[" and canvas[row][col] == 0:
loop += 1
elif command == "]" and canvas[row][col] != 0:
loop -= 1
step += 1 if not loop else loop / / abs(loop)
count += 1 if not loop else 0
return "\r\n" . join("" . join(map(str, row)) for row in canvas)
| Esolang Interpreters #3 - Custom Paintf**k Interpreter | 5868a68ba44cfc763e00008d | [
"Esoteric Languages",
"Interpreters",
"Algorithms",
"Tutorials"
] | https://www.codewars.com/kata/5868a68ba44cfc763e00008d | 4 kyu |
# Esolang Interpreters #2 - Custom Smallfuck Interpreter
## About this Kata Series
"Esolang Interpreters" is a Kata Series that originally began as three separate, independent esolang interpreter Kata authored by [@donaldsebleung](http://codewars.com/users/donaldsebleung) which all shared a similar format and were all somewhat inter-related. Under the influence of [a fellow Codewarrior](https://www.codewars.com/users/nickkwest), these three high-level inter-related Kata gradually evolved into what is known today as the "Esolang Interpreters" series.
This series is a high-level Kata Series designed to challenge the minds of bright and daring programmers by implementing interpreters for various [esoteric programming languages/Esolangs](http://esolangs.org), mainly [Brainfuck](http://esolangs.org/wiki/Brainfuck) derivatives but not limited to them, given a certain specification for a certain Esolang. Perhaps the only exception to this rule is the very first Kata in this Series which is intended as an introduction/taster to the world of esoteric programming languages and writing interpreters for them.
## The Language
Smallfuck is an [esoteric programming language/Esolang](http://esolangs.org) invented in 2002 which is a sized-down variant of the famous [Brainfuck](http://esolangs.org/wiki/Brainfuck) Esolang. Key differences include:
- Smallfuck operates only on bits as opposed to bytes
- It has a limited data storage which varies from implementation to implementation depending on the size of the tape
- It does not define input or output - the "input" is encoded in the initial state of the data storage (tape) and the "output" should be decoded in the final state of the data storage (tape)
Here are a list of commands in Smallfuck:
- `>` - Move pointer to the right (by 1 cell)
- `<` - Move pointer to the left (by 1 cell)
- `*` - Flip the bit at the current cell
- `[` - Jump past matching `]` if value at current cell is `0`
- `]` - Jump back to matching `[` (if value at current cell is nonzero)
As opposed to Brainfuck where a program terminates only when all of the commands in the program have been considered (left to right), Smallfuck terminates when any of the two conditions mentioned below become true:
- All commands have been considered from left to right
- The pointer goes out-of-bounds (i.e. if it moves to the left of the first cell or to the right of the last cell of the tape)
Smallfuck is considered to be Turing-complete **if and only if** it had a tape of infinite length; however, since the length of the tape is always defined as finite (as the interpreter cannot return a tape of infinite length), its computational class is of bounded-storage machines with bounded input.
More information on this Esolang can be found [here](http://esolangs.org/wiki/Smallfuck).
## The Task
Implement a custom Smallfuck interpreter `interpreter()` (`interpreter` in Haskell and F#, `Interpreter` in C#, `custom_small_fuck:interpreter/2` in Erlang) which accepts the following arguments:
1. `code` - **Required**. The Smallfuck program to be executed, passed in as a string. May contain non-command characters. Your interpreter should simply ignore any non-command characters.
2. `tape` - **Required**. The initial state of the data storage (tape), passed in **as a string**. For example, if the string `"00101100"` is passed in then it should translate to something of this form within your interpreter: `[0, 0, 1, 0, 1, 1, 0, 0]`. You may assume that all input strings for `tape` will be non-empty and will only contain `"0"`s and `"1"`s.
Your interpreter should return the final state of the data storage (tape) **as a string** in the same format that it was passed in. For example, if the tape in your interpreter ends up being `[1, 1, 1, 1, 1]` then return the string `"11111"`.
*NOTE: The pointer of the interpreter always starts from the first (leftmost) cell of the tape, same as in Brainfuck.*
Good luck :D
## Kata in this Series
1. [Esolang Interpreters #1 - Introduction to Esolangs and My First Interpreter (MiniStringFuck)](https://www.codewars.com/kata/esolang-interpreters-number-1-introduction-to-esolangs-and-my-first-interpreter-ministringfuck)
2. **Esolang Interpreters #2 - Custom Smallfuck Interpreter**
3. [Esolang Interpreters #3 - Custom Paintfuck Interpreter](http://codewars.com/kata/esolang-interpreters-number-3-custom-paintf-star-star-k-interpreter)
4. [Esolang Interpreters #4 - Boolfuck Interpreter](http://codewars.com/kata/esolang-interpreters-number-4-boolfuck-interpreter)
| algorithms | def interpreter(code, tape):
tape = list(map(int, tape))
ptr = step = loop = 0
while 0 <= ptr < len(tape) and step < len(code):
command = code[step]
if loop:
if command == "[":
loop += 1
elif command == "]":
loop -= 1
elif command == ">":
ptr += 1
elif command == "<":
ptr -= 1
elif command == "*":
tape[ptr] ^= 1
elif command == "[" and tape[ptr] == 0:
loop += 1
elif command == "]" and tape[ptr] == 1:
loop -= 1
step += 1 if not loop else loop / / abs(loop)
return "" . join(map(str, tape))
| Esolang Interpreters #2 - Custom Smallfuck Interpreter | 58678d29dbca9a68d80000d7 | [
"Esoteric Languages",
"Interpreters",
"Algorithms",
"Tutorials"
] | https://www.codewars.com/kata/58678d29dbca9a68d80000d7 | 5 kyu |
*For the rest of this Kata, I would recommend considering "fuck" to be non-profane.*
# Esolang Interpreters #1 - Introduction to Esolangs and My First Interpreter (MiniStringFuck)
## About this Kata Series
"Esolang Interpreters" is a Kata Series that originally began as three separate, independent esolang interpreter Kata authored by [@donaldsebleung](http://codewars.com/users/donaldsebleung) which all shared a similar format and were all somewhat inter-related. Under the influence of [a fellow Codewarrior](https://www.codewars.com/users/nickkwest), these three high-level inter-related Kata gradually evolved into what is known today as the "Esolang Interpreters" series.
This series is a high-level Kata Series designed to challenge the minds of bright and daring programmers by implementing interpreters for various [esoteric programming languages/Esolangs](http://esolangs.org), mainly [Brainfuck](http://esolangs.org/wiki/Brainfuck) derivatives but not limited to them, given a certain specification for a certain Esolang. Perhaps the only exception to this rule is the very first Kata in this Series which is intended as an introduction/taster to the world of esoteric programming languages and writing interpreters for them.
## What is an esoteric programming language?
~~~if:bf
*If you are solving this Kata in Brainfuck, you are probably already familiar with what an Esolang is so feel free to skip this part of the Description.*
~~~
An [esoteric programming language](http://esolangs.org), otherwise known as an **Esolang**, is an informal computer programming language that is generally not designed for serious practical use. There are a few main aims/themes among the vast majority of such languages:
1. **Achieve Turing-completeness in as few commands (instructions) as possible.** There are currently a number of implemented Esolangs that have been proven to be Turing-complete, [Brainfuck](http://esolangs.org/wiki/Brainfuck) being the most popular of them all, comprised of no more than 8 distinct commands. Despite having only 8 commands, it has been [objectively proven to be Turing-complete](http://www.hevanet.com/cristofd/brainfuck/utm.b). However, Brainfuck is not the Turing-complete programming language with the fewest commands. [Boolfuck](http://esolangs.org/wiki/Boolfuck), a derivative of Brainfuck which operates on bits (`0`s and `1`s) and contains **7 commands** only, has also been proven to be Turing-complete through reduction from Brainfuck. Another less-known Esolang called [Etre](http://codewars.com/wiki/Etre) contains as few as **3 commands** yet has been proven to be Turing-complete through the translation of a Minsky Machine to Etre.
2. **To be as hard to program in as possible.** The famous [Brainfuck](http://esolangs.org/wiki/Brainfuck) Esolang is well known as a **Turing tarpit** - that is, a Turing-complete programming language where it is very hard to write a useful program in reality. However, Brainfuck is most definitely not the hardest Esolang to program in. For example, its close cousin, Boolfuck, which operates on bits (mentioned above) is even harder to program in. There have also been a small number of implemented Esolangs which are so difficult to program in that no one has ever successfully written a single program in it from scratch - the only programs generated from these languages came from computers!
3. **As a joke.** Many Esolangs out there have been invented solely as a joke. Examples include [Ook!](http://esolangs.org/wiki/Ook!) and [Bitxtreme](http://esolangs.org/wiki/bitxtreme).
Although there is no clear-cut definition as to when a programming language is esoteric (or not), Esolangs can generally be identified by the following features/traits:
1. Minimalistic - having as few instructions as possible
2. Plays with new concepts - for example, [Befunge](http://esolangs.org/wiki/Befunge), another very popular Esolang, is interpreted in **two dimensions** as opposed to the usual linear way of interpreting code
3. Themed - this is a trait of many joke Esolangs. For example, some may be fashioned like Shakespearean plays and others like cooking recipes
4. Not clearly documented - Many Esolangs out there have not been described in great detail with perhaps only a few code examples on the entire Internet. Some Esolangs have not even been implemented yet!
5. Contain incomplete specs - New Esolangs are being invented every day. Some Esolangs on the Internet are still a work-in-progress and their commands and behaviour have not been finalised yet.
Nevertheless, Esolangs are generally fun to program in, experiment with and write interpreters for. A great deal can be learned about certain concepts and theories in Computer Science just by studying and programming in a well-designed Esolang such as Brainfuck or Befunge.
Next off, I will introduce you to a simple, minimalistic Esolang called **MiniStringFuck**.
## The Language
[MiniStringFuck](http://esolangs.org/wiki/MiniStringFuck) is a derivative of the famous [Brainfuck](http://esolangs.org/wiki/Brainfuck) which contains a **memory cell** as its only form of data storage as opposed to a memory tape of 30,000 cells in Brainfuck. The **memory cell** in MiniStringFuck initially starts at `0`. MiniStringFuck contains only 2 commands as opposed to 8:
- `+` - Increment the memory cell. If it reaches `256`, wrap to `0`.
- `.` - Output the value of the memory cell as a character with code point equal to the value
For example, here is a MiniStringFuck program that outputs the string `"Hello, World!"`:
```
++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++.+++++++++++++++++++++++++++++.+++++++..+++.+++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++.++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++.+++++++++++++++++++++++++++++++++++++++++++++++++++++++.++++++++++++++++++++++++.+++.++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++.++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++.+++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++.
```
And here is another program that prints the uppercase English alphabet:
```
+++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++.+.+.+.+.+.+.+.+.+.+.+.+.+.+.+.+.+.+.+.+.+.+.+.+.+.
```
Any characters in a MiniStringFuck program other than `+` or `.` are simply non-command characters (no-ops, i.e. do nothing) and therefore can serve as comments in the program.
## The Task
Time to write your first Esolang interpreter :D
Your task is to implement a MiniStringFuck interpreter `myFirstInterpreter()`/`my_first_interpreter()`/`Interpreter()`/`interpret()` `MyFirstInterpreter()` (depending on your language) which accepts exactly 1 required argument `code`/`$code`/`strng` which is the MiniStringFuck program to be executed. The output of the program should then be returned by your interpreter as a string.
~~~if:elixir
For Elixir you have to implement `MiniStringFuck.execute/1`.
~~~
~~~if:erlang
For Erlang you have to implement `mini_string_fuck:my_first_interpreter/1`.
~~~
~~~if:java
For Java you'll implement the `MyFirstInterpreter` class which accepts 1 String in it's constructor and returns the output from the `interpret` method.
~~~
~~~if:bf
For Brainfuck you have to implement a program that accepts a MiniStringFuck program of varying length, interpret it according to the specs provided above, and return its output (if any). You may assume that the interpreter used to interpret your Brainfuck program uses a standard implementation: `30000` unsigned wrapping 8-bit cells on a non-cylindrical tape (going too far to the left/right of the memory tape throws an error), EOF is denoted by `byte(0)` (**not** `byte(-1)`), etc.
~~~
Since this is the first time you are about to write an interpreter for an Esolang, here are a few quick tips:
- If you are afraid that your interpreter will be confused by non-command characters appearing in the MiniStringFuck program, you can try to remove all non-command characters from the `code` input before letting your interpreter interpret it
- The memory cell in MiniStringFuck only ever contains a single integer value - think of how it can be modelled in your interpreter
- If you are stuck as to how to interpret the string as a program, try thinking of strings as an array of characters. Try looping through the "program" like you would an array
- Writing an interpreter for an Esolang can sometimes be quite confusing! It never hurts to add a few comments in your interpreter as you implement it to remind yourself of what is happening within the interpreter at every stage
*NOTE: If you would not like to name your interpreter as* `myFirstInterpreter()`/`my_first_interpreter()`*, you can optionally rename it to either* `miniStringFuck()`/`mini_string_fuck()` *or* `interpreter()` *instead - the test cases will handle the rest for you.* **Not available in Java, Go, Swift, TypeScript, Haskell, Elixir, C++, C#, Rust, R, Erlang, F#, Factor, COBOL and NASM**; *irrelevant to Brainfuck solvers* ;)
Good luck :D
~~~if:bf
*NOTE: If you are having difficulty keeping track of your program in Brainfuck, you may want to use [this Brainfuck visualizer](http://fatiherikli.github.io/brainfuck-visualizer) to aid you in debugging your code.*
~~~
## Kata in this Series
1. **Esolang Interpreters #1 - Introduction to Esolangs and My First Interpreter (MiniStringFuck)**
2. [Esolang Interpreters #2 - Custom Smallfuck Interpreter](http://codewars.com/kata/esolang-interpreters-number-2-custom-smallfuck-interpreter)
3. [Esolang Interpreters #3 - Custom Paintfuck Interpreter](http://codewars.com/kata/esolang-interpreters-number-3-custom-paintf-star-star-k-interpreter)
4. [Esolang Interpreters #4 - Boolfuck Interpreter](https://www.codewars.com/kata/esolang-interpreters-number-4-boolfuck-interpreter)
| algorithms | def my_first_interpreter(code):
memory, output = 0, ""
for command in code:
if command == "+":
memory += 1
elif command == ".":
output += chr(memory % 256)
return output
| Esolang Interpreters #1 - Introduction to Esolangs and My First Interpreter (MiniStringFuck) | 586dd26a69b6fd46dd0000c0 | [
"Interpreters",
"Esoteric Languages",
"Algorithms",
"Tutorials"
] | https://www.codewars.com/kata/586dd26a69b6fd46dd0000c0 | 6 kyu |
Given the root node of a binary tree (but not necessarily a binary search tree,) write three functions that will print the tree in pre-order, in-order, and post-order.
A Node has the following properties:
```javascript
var data; // A number or string.
Node left; // Undefined if there is no left child.
Node right; // Undefined if there is no right child.
```
```python
data # A number or a string
left # A Node, which is None if there is no left child.
right # A Node, which is None if there is no right child.
```
```haskell
The structure of a tree looks like:
data Tree a = Nil | Node (Tree a) a (Tree a)
```
Pre-order means that we
1.) Visit the root.
2.) Traverse the left subtree (left node.)
3.) Traverse the right subtree (right node.)
In-order means that we
1.) Traverse the left subtree (left node.)
2.) Visit the root.
3.) Traverse the right subtree (right node.)
Post-order means that we
1.) Traverse the left subtree (left node.)
2.) Traverse the right subtree (right node.)
3.) Visit the root.
Let's say we have three nodes.
```javascript
var a = new Node("A");
var b = new Node("B");
var c = new Node("C");
a.left = b;
a.right = c;
```
```python
a = Node("A")
b = Node("B")
c = Node("C")
a.left = b
a.right = c
```
```haskell
a = Node b "A" c
b = Node Nil "B" Nil
c = Node Nil "C" Nil
```
Then,
preOrder(a) should return ["A", "B", C"]
inOrder(a) should return ["B", "A", "C"]
postOrder(a) should return ["B", "C", A"]
What would happen if we did this?
```javascript
var d = new Node("D");
c.left = d;
```
```python
d = Node("D")
c.left = d
```
```haskell
d = Node Nil "D" Nil
c = Node d "C" Nil
```
preOrder(a) should return ["A", "B", "C", "D"]
inOrder(a) should return ["B", "A", "D", "C"]
postOrder(a) should return ["B", "D", "C", "A"] | algorithms | # Pre-order traversal
def pre_order(node):
return [node . data] + pre_order(node . left) + pre_order(node . right) if node else []
# In-order traversal
def in_order(node):
return in_order(node . left) + [node . data] + in_order(node . right) if node else []
# Post-order traversal
def post_order(node):
return post_order(node . left) + post_order(node . right) + [node . data] if node else []
| Binary Tree Traversal | 5268956c10342831a8000135 | [
"Binary Trees",
"Trees",
"Recursion",
"Data Structures",
"Algorithms"
] | https://www.codewars.com/kata/5268956c10342831a8000135 | 5 kyu |
Given the node object:
```
Node:
val: <int>,
left: <Node> or null,
right: <Node> or null
```
write a function `compare(a, b)` which compares the two trees defined by Nodes a and b and returns `true` if they are equal in structure and in value and `false` otherwise.
Examples:
```
1 1
| \ | \
2 3 2 3
=> true
1 1
| \ | \
3 3 2 3
=> false (values not the same 2 != 3)
1 1
| \ |
2 3 2
|
3
=> false (structure not the same)
``` | algorithms | def compare(a, b):
return a . val == b . val and compare(a . left, b . left) and compare(a . right, b . right) if a and b else a == b
| Binary Tree Compare | 55847fcd3e7dadc9f800005f | [
"Binary Trees",
"Algorithms"
] | https://www.codewars.com/kata/55847fcd3e7dadc9f800005f | 6 kyu |
# Story
Hereinafter, `[space]` refers to `" "`, `[tab]` refers to `"\t"`, and `[LF]` refers to `"\n"` for illustrative purposes. This does not mean that you can use these placeholders in your solution.
In esoteric language called [Whitespace](http://compsoc.dur.ac.uk/whitespace/), numbers are represented in the following format:
* first character represents the sign: `[space]` for plus, `[tab]` for minus;
* characters after that are the binary representation of the absolute value of the number, with `[space]` for 0, `[tab]` for 1, the rightmost bit is the least significand bit, and no leading zero bits.
* the binary representation is immediately followed by `[LF]`.
# Notes
* Valid Whitespace number must always have at least two characters: a sign and the terminator. In case there are only two characters, the number is equal to zero.
* For the purposes of this kata, zero must always be represented as `[space][LF]`.
* In Whitespace, only space, tabulation and linefeed are meaningful characters. All other characters are ignored. However, for the purposes of this simple kata, please do not add any other characters in the output.
* In this kata, input will always be a valid negative or positive integer.
* For your convenience, in this kata we will use `unbleach()` function when evaluating your results. This function replaces whitespace characters with `[space]`, `[tab]`, and `[LF]` to make fail messages more obvious. You can see how it works in Example Test Cases.
# Examples
* `1` in Whitespace is `" \t\n"`.
* `0` in Whitespace is `" \n"`.
* `-1` in Whitespace is `"\t\t\n"`.
* `2` in Whitespace is `" \t \n"`.
* `-3` in Whitespace is `"\t\t\t\n"`.
# More on Whitespace
If you liked this kata, you may also enjoy a much harder Whitespace-related kata: [Whitespace interpreter](http://www.codewars.com/kata/whitespace-interpreter) by @jacobb. | reference | from string import maketrans
def whitespace_number(n):
return (' \n' if n == 0 else
'{:+b}\n' . format(n). translate(maketrans('+-01', ' \t \t')))
| Convert integer to Whitespace format | 55b350026cc02ac1a7000032 | [
"Binary",
"Fundamentals"
] | https://www.codewars.com/kata/55b350026cc02ac1a7000032 | 6 kyu |
Oh no! Ghosts have reportedly swarmed the city. It's your job to get rid of them and save the day!
In this kata, strings represent buildings while whitespaces within those strings represent ghosts.
So what are you waiting for? Return the building(string) without any ghosts(whitespaces)!
Example:
```
"Sky scra per" -> "Skyscraper"
```
If the building contains no ghosts, return the string:
```
"You just wanted my autograph didn't you?"
``` | reference | message = "You just wanted my autograph didn't you?"
def ghostbusters(building):
return building . replace(' ', '') if ' ' in building else message
| Ghostbusters (whitespace removal) | 5668e3800636a6cd6a000018 | [
"Fundamentals",
"Regular Expressions",
"Strings"
] | https://www.codewars.com/kata/5668e3800636a6cd6a000018 | 7 kyu |
Here is a new kata that Codewars asked me to do related to interviewing and working in a production setting.
You might be familar with and have used Angular.js. Among other things, it lets you create your own filters that work as functions. You can then put these in a page to perform specific data changes, such as shortening a number to display a more concise notation.
In this kata, we will create a function which returns another function (or process, in Ruby) that shortens very long numbers. Given an initial array of values replace the Xth power of a given base. If the input of the returned function is not a numerical string, it should return the input itself as a string.
Here's an example, which is worth a thousand words:
```javascript
filter1 = shortenNumber(['','k','m'],1000);
filter1('234324') == '234k';
filter1('98234324') == '98m';
filter1([1,2,3]) == '1,2,3';
filter2 = shortenNumber(['B','KB','MB','GB'],1024);
filter2('32') == '32B';
filter2('2100') == '2KB';
filter2('pippi') == 'pippi';
```
```python
filter1 = shorten_number(['','k','m'],1000)
filter1('234324') == '234k'
filter1('98234324') == '98m'
filter1([1,2,3]) == '[1,2,3]'
filter2 = shorten_number(['B','KB','MB','GB'],1024)
filter2('32') == '32B'
filter2('2100') == '2KB';
filter2('pippi') == 'pippi'
```
```csharp
var filter1 = shortenMethods.ShortenNumberCreator(new string[] { "", "k", "m" }, 1000);
filter1("234324") == "234k";
filter1("98234324") == "98m";
filter1(new int[] { 1,2,3 }) == "1,2,3";
var filter2 = shortenMethods.ShortenNumberCreator(new string[] { "B", "KB", "MB", "GB" }, 1024);
filter2("32") == "32B";
filter2("2100") == "2KB";
filter2("pippi") == "pippi";
```
```ruby
filter1 = shorten_number(['','k','m'],1000)
filter1.call('234324') == '234k'
filter1.call('98234324') == '98m'
filter1({}) == '{}'
filter2 = shortenNumber(['B','KB','MB','GB'],1024)
filter2.call('32') == '32B';
filter2.call('2100') == '2KB';
filter2.call('pippi') == 'pippi'
```
If you like to test yourself on kata related to actual work and interviews, consider trying this kata where you will build [a breadcrumb generator](http://www.codewars.com/kata/563fbac924106b8bf7000046) | algorithms | def shorten_number(suffixes, base):
def filter(num_string):
try:
num = int(num_string)
except:
return str(num_string)
suf_index = 0
while num > base and suf_index < len(suffixes) - 1:
num = num / / base
suf_index += 1
return str(num) + suffixes[suf_index]
return filter
| Number Shortening Filter | 56b4af8ac6167012ec00006f | [
"Angular",
"Algorithms"
] | https://www.codewars.com/kata/56b4af8ac6167012ec00006f | 6 kyu |