Watermark Removal Model
Model Summary
The Watermark Removal model is an image processing model based on neural networks. It is designed to remove watermarks from images while preserving the original image quality. The model utilizes an encoder-decoder structure with skip connections to maintain fine details during the watermark removal process.
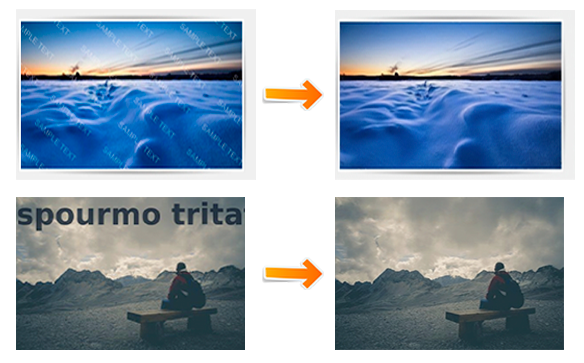
Model Details
Model Description
- Developed by: FODUU AI
- Model type: Computer Vision - Image Processing
- Task: Remove watermark from image
Usage Guide
Installation Requirements
pip install torch torchvision
pip install Pillow matplotlib numpy
or you can run :
pip install -r requirements.txt
Model Loading and Inference
import torch
from torchvision import transforms
from PIL import Image
from watermark_remover import WatermarkRemover
import numpy as np
image_path = "path to your test image" # Replace with the path to your test image
device = torch.device("cuda" if torch.cuda.is_available() else "cpu")
# Load the trained model
model = WatermarkRemover().to(device)
model_path = "path to your model.pth" # Replace with the path to your saved model
model.load_state_dict(torch.load(model_path, map_location=device))
model.eval()
transform = transforms.Compose([transforms.Resize((256, 256)),
transforms.ToTensor(),])
watermarked_image = Image.open(image_path).convert("RGB")
original_size = watermarked_image.size
input_tensor = transform(watermarked_image).unsqueeze(0).to(device)
with torch.no_grad():
output_tensor = model(input_tensor)
predicted_image = output_tensor.squeeze(0).cpu().permute(1, 2, 0).clamp(0, 1).numpy()
predicted_pil = Image.fromarray((predicted_image * 255).astype(np.uint8))
predicted_pil = predicted_pil.resize(original_size, Image.Resampling.LANCZOS)
predicted_pil.save("predicted_image.jpg", quality=100)
Limitations and Considerations
- Performance may vary depending on watermark complexity and opacity
- Best results achieved with semi-transparent watermarks
- Model trained on 256x256 images; performance may vary with different resolutions
- GPU recommended for faster inference
Training Details
- Dataset: The model was trained on a custom dataset consisting of 20,000 images with watermarks in various styles and intensities.
- Training Time: The model was trained for 200 epochs on an NVIDIA GeForce RTX 3060 GPU.
- Loss Function: The model uses a combination of MSE (Mean Squared Error) and perceptual loss to optimize watermark removal quality.
Model Evaluation
The model has been evaluated using Peak Signal-to-Noise Ratio (PSNR) and Structural Similarity Index (SSIM) on a test set of watermarked images, achieving an average PSNR of 30.5 dB and an SSIM of 0.92.
Compute Infrastructure
Hardware
NVIDIA GeForce RTX 3060 card
Software
The model was trained on Jupyter Notebook environment.
Model Card Contact
For inquiries and contributions, please contact us at [email protected]
@ModelCard{
author = {Nehul Agrawal and
Priyal Mehta},
title = {Watermark Removal Using Neural Networks},
year = {2025}
}